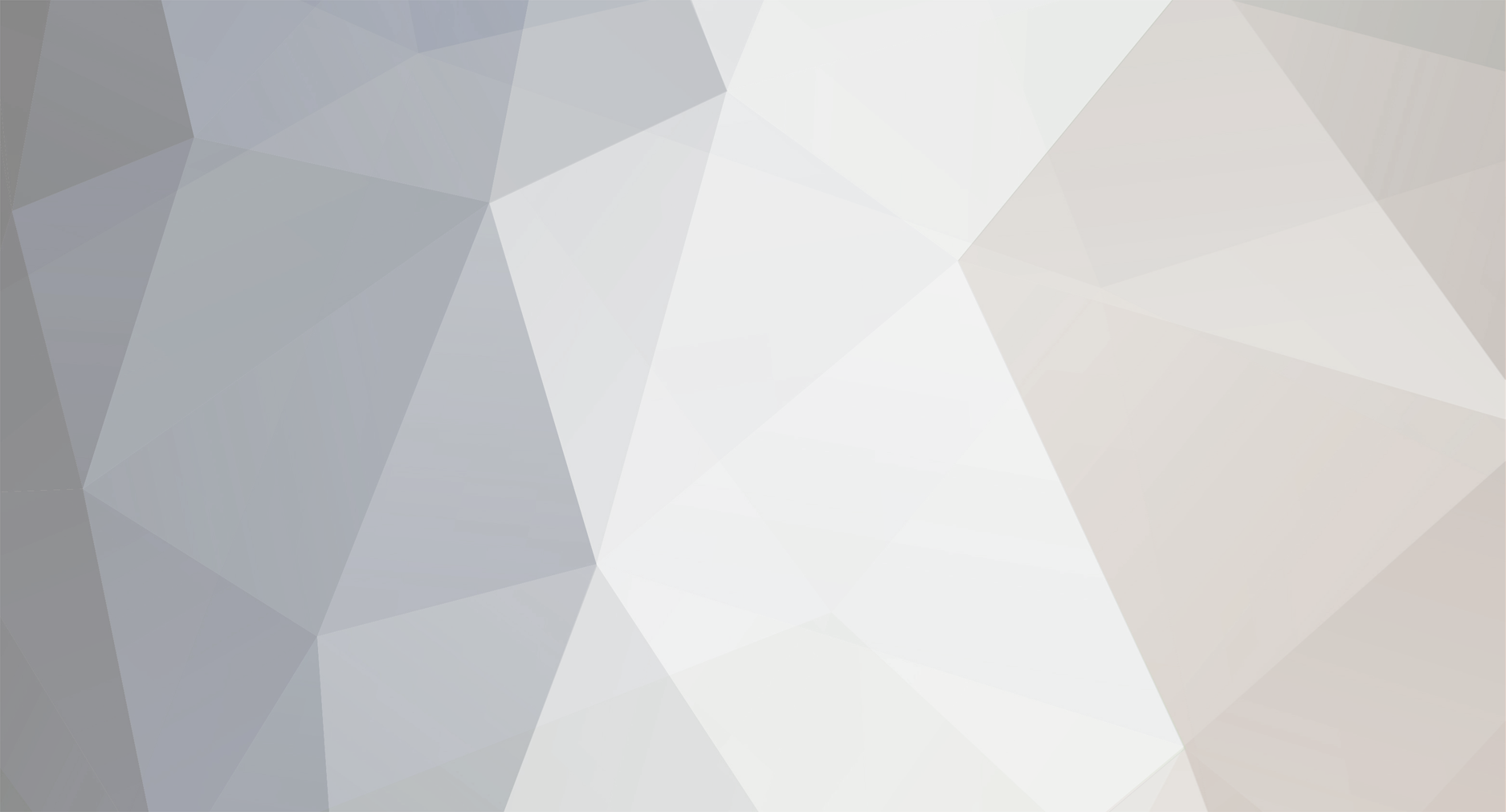
mtorbin
Members-
Posts
65 -
Joined
-
Last visited
Everything posted by mtorbin
-
OOP PHP: Where to put a "variable assigning" function
mtorbin replied to mtorbin's topic in PHP Coding Help
That's an interesting suggestion. I'm still very much learning OOP and the proper way to write things (thanks, BTW, to the great suggestions made at this forum). I always like to write things to make my life easier in the long run, though it was my understanding that if you can boil functions/classes down to their most simplistic format, it's better to have a bunch of things that each do one thing rather then a few things that each do many things. Is this the right way of looking at this? - MT -
OOP PHP: Where to put a "variable assigning" function
mtorbin replied to mtorbin's topic in PHP Coding Help
So, in reference to my script this line: $this->setEventVars($sportSchedules,$counter); should really be this line: self::setEventVars($sportSchedules,$counter); yes? - MT -
OOP PHP: Where to put a "variable assigning" function
mtorbin replied to mtorbin's topic in PHP Coding Help
Ah, got it! It took some tweaking but I finally got the concept down. Here's the final script, enjoyably much leaner: <?php class TranslateXML { public function __construct($type,$url) { global $newXML; $this->scheduleXML = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n<events>\n"; $data = simplexml_load_file($url); $counter = 0; $sportSchedules = Array(); switch($type) { case 'mlb': include 'class/class.MlbSchedule.php'; foreach($data->{'sports-schedule'}->{'baseball-mlb-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new MlbSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->setEventVars($sportSchedules,$counter); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nba': include 'class/class.NbaSchedule.php'; foreach($data->{'sports-schedule'}->{'nba-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NbaSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->setEventVars($sportSchedules,$counter); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nfl': include 'class/class.NflSchedule.php'; foreach($data->{'sports-schedule'}->{'football-nfl-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NflSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->setEventVars($sportSchedules,$counter); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nhl': include 'class/class.NhlSchedule.php'; foreach($data->{'sports-schedule'}->{'hockey-nhl-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NhlSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->setEventVars($sportSchedules,$counter); $this->scheduleXML .= $this->buildNode(); $counter++; } break; } } function getNewXml() {return $this->scheduleXML;} function setEventVars($myOBJ,$myCount) { $this->myGameID = $myOBJ[$myCount]->getGameID(); $this->myHomeTeamName = $myOBJ[$myCount]->getTeamName('home'); $this->myHomeTeamCity = $myOBJ[$myCount]->getTeamCity('home'); $this->myVisitTeamName = $myOBJ[$myCount]->getTeamName('visit'); $this->myVisitTeamCity = $myOBJ[$myCount]->getTeamCity('visit'); $this->myGameDate = $myOBJ[$myCount]->getGameDate(); $this->myGameTime = $myOBJ[$myCount]->getGameTime(); $this->myVenueID = $myOBJ[$myCount]->getVenueID(); $this->myPerfID = $myOBJ[$myCount]->getPerfID(); $this->myGenre = $myOBJ[$myCount]->getPcomGenre(); } function buildNode() { $tempData = "\t\t<event_id>" . $this->myGameID . "</event_id>\n"; $tempData .= "\t\t<event_title>" . $this->myVisitTeamCity . ' ' . $this->myVisitTeamName . ' (away) vs. ' . $this->myHomeTeamCity . ' ' . $this->myHomeTeamName . " (home)</event_title>\n"; $tempData .= "\t\t<perf_id>" . $this->myPerfID . "</perf_id>\n"; $tempData .= "\t\t<addl_perfs></addl_perfs>\n"; $tempData .= "\t\t<venue_id>" . $this->myVenueID . "</venue_id>\n"; $tempData .= "\t\t<event_desc>" . $this->myVisitTeamCity . ' ' . $this->myVisitTeamName . ' (away) vs. ' . $this->myHomeTeamCity . ' ' . $this->myHomeTeamName . " (home) [" . $this->myGameDate . ' @' . $this->myGameTime . "]</event_desc>\n"; $tempData .= "\t\t<genres>\n"; $tempData .= "\t\t\t<genre>" . $this->myGenre . "</genre>\n"; $tempData .= "\t\t</genres>\n"; $tempData .= "\t\t<showtimes></showtimes>\n"; $tempData .= "\t\t<schedule>\n"; $tempData .= "\t\t\t<performance date=\"" . $this->myGameDate . "\">" . $this->myGameTime . "</performance>\n"; $tempData .= "\t\t</schedule>\n"; $tempData .= "\t</event>\n"; return $tempData; } } ?> Guys thanks for all of your help. Nightslyr, I had one question about your post: Are you saying instead of using "$this->" I should use "self::"? What is the difference between the two? - MT -
OOP PHP: Where to put a "variable assigning" function
mtorbin replied to mtorbin's topic in PHP Coding Help
UPDATE: Mark, I posted the function above the constructor exactly as I have it listed above. While it didn't error out, it also didn't assign the vars. I have a feeling this is because it doesn't know what "this" is anymore, right? How would I correct this? - MT -
OOP PHP: Where to put a "variable assigning" function
mtorbin replied to mtorbin's topic in PHP Coding Help
Sure, sorry about that: function setEventVars() { $this->myGameID = $sportSchedules[$counter]->getGameID(); $this->myHomeTeamName = $sportSchedules[$counter]->getTeamName('home'); $this->myHomeTeamCity = $sportSchedules[$counter]->getTeamCity('home'); $this->myVisitTeamName = $sportSchedules[$counter]->getTeamName('visit'); $this->myVisitTeamCity = $sportSchedules[$counter]->getTeamCity('visit'); $this->myGameDate = $sportSchedules[$counter]->getGameDate(); $this->myGameTime = $sportSchedules[$counter]->getGameTime(); $this->myVenueID = $sportSchedules[$counter]->getVenueID(); $this->myPerfID = $sportSchedules[$counter]->getPerfID(); $this->myGenre = $sportSchedules[$counter]->getPcomGenre(); } I had put this AFTER the constructor but it seems like I need to put it BEFORE the constructor as Mark pointed out, yes? - MT -
Hey all, I'm trying to optimize a class file that I've written and I'm hoping you can help me. Here is the class file that I've written: <?php class TranslateXML { public function __construct($type,$url) { global $newXML; $this->scheduleXML = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n<events>\n"; $data = simplexml_load_file($url); $counter = 0; $sportSchedules = Array(); switch($type) { case 'mlb': include 'class/class.MlbSchedule.php'; foreach($data->{'sports-schedule'}->{'baseball-mlb-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new MlbSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->myGameID = $sportSchedules[$counter]->getGameID(); $this->myHomeTeamName = $sportSchedules[$counter]->getTeamName('home'); $this->myHomeTeamCity = $sportSchedules[$counter]->getTeamCity('home'); $this->myVisitTeamName = $sportSchedules[$counter]->getTeamName('visit'); $this->myVisitTeamCity = $sportSchedules[$counter]->getTeamCity('visit'); $this->myGameDate = $sportSchedules[$counter]->getGameDate(); $this->myGameTime = $sportSchedules[$counter]->getGameTime(); $this->myVenueID = $sportSchedules[$counter]->getVenueID(); $this->myPerfID = $sportSchedules[$counter]->getPerfID(); $this->myGenre = $sportSchedules[$counter]->getPcomGenre(); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nba': include 'class/class.NbaSchedule.php'; foreach($data->{'sports-schedule'}->{'nba-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NbaSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->myGameID = $sportSchedules[$counter]->getGameID(); $this->myHomeTeamName = $sportSchedules[$counter]->getTeamName('home'); $this->myHomeTeamCity = $sportSchedules[$counter]->getTeamCity('home'); $this->myVisitTeamName = $sportSchedules[$counter]->getTeamName('visit'); $this->myVisitTeamCity = $sportSchedules[$counter]->getTeamCity('visit'); $this->myGameDate = $sportSchedules[$counter]->getGameDate(); $this->myGameTime = $sportSchedules[$counter]->getGameTime(); $this->myVenueID = $sportSchedules[$counter]->getVenueID(); $this->myPerfID = $sportSchedules[$counter]->getPerfID(); $this->myGenre = $sportSchedules[$counter]->getPcomGenre(); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nfl': include 'class/class.NflSchedule.php'; foreach($data->{'sports-schedule'}->{'football-nfl-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NflSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->myGameID = $sportSchedules[$counter]->getGameID(); $this->myHomeTeamName = $sportSchedules[$counter]->getTeamName('home'); $this->myHomeTeamCity = $sportSchedules[$counter]->getTeamCity('home'); $this->myVisitTeamName = $sportSchedules[$counter]->getTeamName('visit'); $this->myVisitTeamCity = $sportSchedules[$counter]->getTeamCity('visit'); $this->myGameDate = $sportSchedules[$counter]->getGameDate(); $this->myGameTime = $sportSchedules[$counter]->getGameTime(); $this->myVenueID = $sportSchedules[$counter]->getVenueID(); $this->myPerfID = $sportSchedules[$counter]->getPerfID(); $this->myGenre = $sportSchedules[$counter]->getPcomGenre(); $this->scheduleXML .= $this->buildNode(); $counter++; } $this->scheduleXML .= "</events>\n"; break; case 'nhl': include 'class/class.NhlSchedule.php'; foreach($data->{'sports-schedule'}->{'hockey-nhl-schedule'}->{'game-schedule'} as $schedule) { $this->scheduleXML .= "\t<event>\n"; $scheduleOBJ = new NhlSchedule($schedule,$data); $sportSchedules[$counter] = $scheduleOBJ; $this->myGameID = $sportSchedules[$counter]->getGameID(); $this->myHomeTeamName = $sportSchedules[$counter]->getTeamName('home'); $this->myHomeTeamCity = $sportSchedules[$counter]->getTeamCity('home'); $this->myVisitTeamName = $sportSchedules[$counter]->getTeamName('visit'); $this->myVisitTeamCity = $sportSchedules[$counter]->getTeamCity('visit'); $this->myGameDate = $sportSchedules[$counter]->getGameDate(); $this->myGameTime = $sportSchedules[$counter]->getGameTime(); $this->myVenueID = $sportSchedules[$counter]->getVenueID(); $this->myPerfID = $sportSchedules[$counter]->getPerfID(); $this->myGenre = $sportSchedules[$counter]->getPcomGenre(); $this->scheduleXML .= $this->buildNode(); $counter++; } break; } } function getNewXml() {return $this->scheduleXML;} function buildNode() { $tempData = "\t\t<event_id>" . $this->myGameID . "</event_id>\n"; $tempData .= "\t\t<event_title>" . $this->myVisitTeamCity . ' ' . $this->myVisitTeamName . ' (away) vs. ' . $this->myHomeTeamCity . ' ' . $this->myHomeTeamName . " (home)</event_title>\n"; $tempData .= "\t\t<perf_id>" . $this->myPerfID . "</perf_id>\n"; $tempData .= "\t\t<addl_perfs></addl_perfs>\n"; $tempData .= "\t\t<venue_id>" . $this->myVenueID . "</venue_id>\n"; $tempData .= "\t\t<event_desc>" . $this->myVisitTeamCity . ' ' . $this->myVisitTeamName . ' (away) vs. ' . $this->myHomeTeamCity . ' ' . $this->myHomeTeamName . " (home) [" . $this->myGameDate . ' @' . $this->myGameTime . "]</event_desc>\n"; $tempData .= "\t\t<genres>\n"; $tempData .= "\t\t\t<genre>" . $this->myGenre . "</genre>\n"; $tempData .= "\t\t</genres>\n"; $tempData .= "\t\t<showtimes></showtimes>\n"; $tempData .= "\t\t<schedule>\n"; $tempData .= "\t\t\t<performance date=\"" . $this->myGameDate . "\">" . $this->myGameTime . "</performance>\n"; $tempData .= "\t\t</schedule>\n"; $tempData .= "\t</event>\n"; return $tempData; } } ?> As you can see in the switch statement, each of the four options does a lot of the same thing. I'd like to wrap those assignment statements in a function to cut down on size. However, whenever I try to add the function to the constructor, I get one of two errors (depending upon whether I use the prefix "$this->" with the call to the function: Fatal error: Call to undefined method TranslateXML::setEventVars() OR Fatal error: Call to undefined function setEventVars() I believe that the function needs to be encapsulated within the constructor but I think I'm missing something very small but VERY important. Any help would be much appreciated.
-
Hey all, I'm in the process of writing a small script that pings blogsearch.google.com via REST client. The problem is that I don't think that Google works as nicely as they'd like you too believe. Here's what's going on: We have about seventy or so blogs that we're pinging Google with. The process basically performs a file_get_contents which returns either a 200 alert or an error. Immediately after the ping process is complete, I download their changes.xml document to see if any of our requested URLs have been accepted. Sometimes I get lucky and a few actually make it into the changes.xml file. However, when I try to search for any of our blogs in their blogsearch, I don't get any responses. My guess is that the blobsearch is being pinged so quickly and so frequently by everyone and their second cousins that it doesn't have time to ingest everything. Has anyone else had this experience? If you have any suggestions on how best to deal with this, please let me know. Thanks, - MT p.s. here is my script if that helps any of you: <?php if(file_exists("changes.xml")) {unlink("changes.xml");} $blogsURL = "[document containing blog links]"; //links should be in the following format: //<google>http://blogsearch.google.com/ping?name=[blog_name]&url=[blog_url]&changesURL=[blog_feed.rss]</google> $counter = 0; $blogsData = file_get_contents($blogsURL); preg_match_all("/(?<=<google>)(.*)(?=<\/google>)/isU", $blogsData, $linksFound); foreach($linksFound[0] as $link) { $counter++; $link = substr($link,34,strlen($link)); $link = "http://blogsearch.google.com/ping?" . getEscapeChars($link,"esc"); $myURL = $link; $myData = file_get_contents($myURL); $blogName = substr($link, 39, (strpos($link,"&url")-39)); $blogName = str_replace("+", " ", str_replace("%27", "'", str_replace("%3A", ":", str_replace("%2C", ",", str_replace("%21", "!", $blogName))))); if($myData == "Thanks for the ping.") {print("The url for '" . getEscapeChars($blogName,"unesc") . "' was accepted.\n");} else {print("The url for '" . getEscapeChars($blogName,"unesc") . "' was not accepted.\n");} } print("Total Blogs: " . $counter . "\n\n"); exec("curl http://blogsearch.google.com/changes.xml?last=900 -o changes.xml"); print("\n"); function getEscapeChars($myChars,$myProcess) { if($myProcess == "esc") {$myChars = str_replace(":","%3A",str_replace("/","%2F",str_replace("+","%2B",$myChars)));} else {$myChars = str_replace("%3A",":",str_replace("%2F","/",str_replace("%2B"," ",$myChars)));} return $myChars; } ?>
-
Thanks. I've never used those but maybe it's time I did. - MT CORRECTION: Sorry, it's late in the day. I've used SimpleXML but in this case, it couldn't be done because the XML that I'm reading was non-standard so I had to use preg_match_all to grab chunks and rebuild.
-
that was sorta my thinking too because TextWrangler identifies a difference between "\r" and "\n". Thank you, at least I'm a lot closer then I was to figuring this one out. I think going forward I'm going to stick to Eclipse. - MT
-
In the hex editor, all of the affected areas contain: 0D 0A 20 20 - MT
-
I'll definitely give that a go. What's odd, and somewhat sad, is that I checked this file in other text editors (both PC and Mac) and this is the ONLY one that displays double carriage returns. I'm using TextWrangler by Bare Bones. I've used this particular text editor for many years without issue. I wonder why THIS script created THIS problem. Anyways, I'm going to try your suggestion and report back. I hate to just walk away from a problem and write it off as a "software bug" without being sure. - MT
-
Hey all, I have an odd issue that I've never quite seen before and I'm wondering if it has something to do with the way that fwrite is working (possibly coupled with issues in my data). Without a lengthy explanation of extraneous details, I have an array with each item in the array having something like the following (spacing was intentional): $myArray[1] = " <xmlTag>[DATA]</xmlTag> <newTag>[DATA]</newTag> <thirdTag>[DATA]</thirdTag>"; However, when it gets written to the file, I have this (again, spacing is intentional): <xmlTag>[DATA]</xmlTag> <newTag>[DATA]</newTag> <thirdTag>[DATA]</thirdTag> (note the double carriage returns) I've run print outputs all throughout the script to test what's going on and I'm viewing the responses in Terminal (on Mac). All of the print outputs display correctly. The only thing that displays incorrectly is the final data written in the text file. Has anyone else seen this before? Thanks, - MT
-
Installation or Syntax Problems
mtorbin replied to mtorbin's topic in PHP Installation and Configuration
Yes, I'm running this from the Terminal command line: Here is the output form the phpinfo(): HP Version => 5.2.6 System => Darwin blackDeath.local 9.6.0 Darwin Kernel Version 9.6.0: Mon Nov 24 17:37:00 PST 2008; root:xnu-1228.9.59~1/RELEASE_I386 i386 Build Date => Sep 19 2008 11:23:02 Configure Command => '/SourceCache/apache_mod_php/apache_mod_php-44.1/php/configure' '--prefix=/usr' '--mandir=/usr/share/man' '--infodir=/usr/share/info' '--disable-dependency-tracking' '--with-apxs2=/usr/sbin/apxs' '--with-ldap=/usr' '--with-kerberos=/usr' '--enable-cli' '--with-zlib-dir=/usr' '--enable-trans-sid' '--with-xml' '--enable-exif' '--enable-ftp' '--enable-mbstring' '--enable-mbregex' '--enable-dbx' '--enable-sockets' '--with-iodbc=/usr' '--with-curl=/usr' '--with-config-file-path=/etc' '--sysconfdir=/private/etc' '--with-mysql-sock=/var/mysql' '--with-mysqli=/usr/bin/mysql_config' '--with-mysql=/usr' '--with-openssl' '--with-xmlrpc' '--with-xsl=/usr' '--without-pear' Server API => Command Line Interface Virtual Directory Support => disabled Configuration File (php.ini) Path => /etc Loaded Configuration File => (none) PHP API => 20041225 PHP Extension => 20060613 Zend Extension => 220060519 Debug Build => no Thread Safety => disabled Zend Memory Manager => enabled IPv6 Support => enabled Registered PHP Streams => php, file, data, http, ftp, compress.zlib, https, ftps Registered Stream Socket Transports => tcp, udp, unix, udg, ssl, sslv3, sslv2, tls Registered Stream Filters => string.rot13, string.toupper, string.tolower, string.strip_tags, convert.*, consumed, convert.iconv.*, zlib.* This program makes use of the Zend Scripting Language Engine: Zend Engine v2.2.0, Copyright (c) 1998-2008 Zend Technologies _______________________________________________________________________ Configuration PHP Core Directive => Local Value => Master Value allow_call_time_pass_reference => On => On allow_url_fopen => On => On allow_url_include => Off => Off always_populate_raw_post_data => Off => Off arg_separator.input => & => & arg_separator.output => & => & asp_tags => Off => Off auto_append_file => no value => no value auto_globals_jit => On => On auto_prepend_file => no value => no value browscap => no value => no value default_charset => no value => no value default_mimetype => text/html => text/html define_syslog_variables => Off => Off disable_classes => no value => no value disable_functions => no value => no value display_errors => STDOUT => STDOUT display_startup_errors => Off => Off doc_root => no value => no value docref_ext => no value => no value docref_root => no value => no value enable_dl => On => On error_append_string => no value => no value error_log => no value => no value error_prepend_string => no value => no value error_reporting => no value => no value expose_php => On => On extension_dir => /usr/lib/php/extensions/no-debug-non-zts-20060613 => /usr/lib/php/extensions/no-debug-non-zts-20060613 file_uploads => On => On highlight.bg => <font style="color: #FFFFFF">#FFFFFF</font> => <font style="color: #FFFFFF">#FFFFFF</font> highlight.comment => <font style="color: #FF8000">#FF8000</font> => <font style="color: #FF8000">#FF8000</font> highlight.default => <font style="color: #0000BB">#0000BB</font> => <font style="color: #0000BB">#0000BB</font> highlight.html => <font style="color: #000000">#000000</font> => <font style="color: #000000">#000000</font> highlight.keyword => <font style="color: #007700">#007700</font> => <font style="color: #007700">#007700</font> highlight.string => <font style="color: #DD0000">#DD0000</font> => <font style="color: #DD0000">#DD0000</font> html_errors => Off => Off ignore_repeated_errors => Off => Off ignore_repeated_source => Off => Off ignore_user_abort => Off => Off implicit_flush => On => On include_path => .: => .: log_errors => Off => Off log_errors_max_len => 1024 => 1024 magic_quotes_gpc => On => On magic_quotes_runtime => Off => Off magic_quotes_sybase => Off => Off mail.force_extra_parameters => no value => no value max_execution_time => 0 => 0 max_input_nesting_level => 64 => 64 max_input_time => -1 => -1 memory_limit => 128M => 128M open_basedir => no value => no value output_buffering => 0 => 0 output_handler => no value => no value post_max_size => 8M => 8M precision => 14 => 14 realpath_cache_size => 16K => 16K realpath_cache_ttl => 120 => 120 register_argc_argv => On => On register_globals => Off => Off register_long_arrays => On => On report_memleaks => On => On report_zend_debug => Off => Off safe_mode => Off => Off safe_mode_exec_dir => /usr/local/php/bin => /usr/local/php/bin safe_mode_gid => Off => Off safe_mode_include_dir => no value => no value sendmail_from => no value => no value sendmail_path => /usr/sbin/sendmail -t -i => /usr/sbin/sendmail -t -i serialize_precision => 100 => 100 short_open_tag => On => On SMTP => localhost => localhost smtp_port => 25 => 25 sql.safe_mode => Off => Off track_errors => Off => Off unserialize_callback_func => no value => no value upload_max_filesize => 2M => 2M upload_tmp_dir => no value => no value user_dir => no value => no value variables_order => EGPCS => EGPCS xmlrpc_error_number => 0 => 0 xmlrpc_errors => Off => Off y2k_compliance => On => On zend.ze1_compatibility_mode => Off => Off ctype ctype functions => enabled curl cURL support => enabled cURL Information => libcurl/7.16.3 OpenSSL/0.9.7l zlib/1.2.3 date date/time support => enabled "Olson" Timezone Database Version => 2008.2 Timezone Database => internal Default timezone => America/New_York Directive => Local Value => Master Value date.default_latitude => 31.7667 => 31.7667 date.default_longitude => 35.2333 => 35.2333 date.sunrise_zenith => 90.583333 => 90.583333 date.sunset_zenith => 90.583333 => 90.583333 date.timezone => no value => no value dom DOM/XML => enabled DOM/XML API Version => 20031129 libxml Version => 2.6.16 HTML Support => enabled XPath Support => enabled XPointer Support => enabled Schema Support => enabled RelaxNG Support => enabled exif EXIF Support => enabled EXIF Version => 1.4 $Id: exif.c,v 1.173.2.5.2.25 2008/03/12 17:33:14 iliaa Exp $ Supported EXIF Version => 0220 Supported filetypes => JPEG,TIFF filter Input Validation and Filtering => enabled Revision => $Revision: 1.52.2.42 $ Directive => Local Value => Master Value filter.default => unsafe_raw => unsafe_raw filter.default_flags => no value => no value ftp FTP support => enabled hash hash support => enabled Hashing Engines => md2 md4 md5 sha1 sha256 sha384 sha512 ripemd128 ripemd160 ripemd256 ripemd320 whirlpool tiger128,3 tiger160,3 tiger192,3 tiger128,4 tiger160,4 tiger192,4 snefru gost adler32 crc32 crc32b haval128,3 haval160,3 haval192,3 haval224,3 haval256,3 haval128,4 haval160,4 haval192,4 haval224,4 haval256,4 haval128,5 haval160,5 haval192,5 haval224,5 haval256,5 iconv iconv support => enabled iconv implementation => libiconv iconv library version => unknown Directive => Local Value => Master Value iconv.input_encoding => ISO-8859-1 => ISO-8859-1 iconv.internal_encoding => ISO-8859-1 => ISO-8859-1 iconv.output_encoding => ISO-8859-1 => ISO-8859-1 json json support => enabled json version => 1.2.1 ldap LDAP Support => enabled RCS Version => $Id: ldap.c,v 1.161.2.3.2.12 2007/12/31 07:20:07 sebastian Exp $ Total Links => 0/unlimited API Version => 3001 Vendor Name => OpenLDAP Vendor Version => 20327 libxml libXML support => active libXML Version => 2.6.16 libXML streams => enabled mbstring Multibyte Support => enabled Multibyte string engine => libmbfl Multibyte (japanese) regex support => enabled Multibyte regex (oniguruma) version => 4.4.4 Multibyte regex (oniguruma) backtrack check => On mbstring extension makes use of "streamable kanji code filter and converter", which is distributed under the GNU Lesser General Public License version 2.1. Directive => Local Value => Master Value mbstring.detect_order => no value => no value mbstring.encoding_translation => Off => Off mbstring.func_overload => 0 => 0 mbstring.http_input => pass => pass mbstring.http_output => pass => pass mbstring.internal_encoding => ISO-8859-1 => no value mbstring.language => neutral => neutral mbstring.strict_detection => Off => Off mbstring.substitute_character => no value => no value mysql MySQL Support => enabled Active Persistent Links => 0 Active Links => 0 Client API version => 5.0.67 MYSQL_MODULE_TYPE => external MYSQL_SOCKET => /var/mysql/mysql.sock MYSQL_INCLUDE => -I/usr/include/mysql MYSQL_LIBS => -L/usr/lib/mysql -lmysqlclient Directive => Local Value => Master Value mysql.allow_persistent => On => On mysql.connect_timeout => 60 => 60 mysql.default_host => no value => no value mysql.default_password => no value => no value mysql.default_port => no value => no value mysql.default_socket => no value => no value mysql.default_user => no value => no value mysql.max_links => Unlimited => Unlimited mysql.max_persistent => Unlimited => Unlimited mysql.trace_mode => Off => Off mysqli MysqlI Support => enabled Client API library version => 5.0.67 Client API header version => 5.0.67 MYSQLI_SOCKET => /var/mysql/mysql.sock Directive => Local Value => Master Value mysqli.default_host => no value => no value mysqli.default_port => 3306 => 3306 mysqli.default_pw => no value => no value mysqli.default_socket => no value => no value mysqli.default_user => no value => no value mysqli.max_links => Unlimited => Unlimited mysqli.reconnect => Off => Off odbc ODBC Support => enabled Active Persistent Links => 0 Active Links => 0 ODBC library => iodbc ODBC_INCLUDE => -I/usr/include ODBC_LFLAGS => -L/usr/lib ODBC_LIBS => -liodbc Directive => Local Value => Master Value odbc.allow_persistent => On => On odbc.check_persistent => On => On odbc.default_db => no value => no value odbc.default_pw => no value => no value odbc.default_user => no value => no value odbc.defaultbinmode => return as is => return as is odbc.defaultlrl => return up to 4096 bytes => return up to 4096 bytes odbc.max_links => Unlimited => Unlimited odbc.max_persistent => Unlimited => Unlimited openssl OpenSSL support => enabled OpenSSL Version => OpenSSL 0.9.7l 28 Sep 2006 pcre PCRE (Perl Compatible Regular Expressions) Support => enabled PCRE Library Version => 7.6 2008-01-28 Directive => Local Value => Master Value pcre.backtrack_limit => 100000 => 100000 pcre.recursion_limit => 100000 => 100000 PDO PDO support => enabled PDO drivers => sqlite2, sqlite pdo_sqlite PDO Driver for SQLite 3.x => enabled PECL Module version => (bundled) 1.0.1 $Id: pdo_sqlite.c,v 1.10.2.6.2.3 2007/12/31 07:20:10 sebastian Exp $ SQLite Library => 3.3.7 posix Revision => $Revision: 1.70.2.3.2.18 $ Reflection Reflection => enabled Version => $Id: php_reflection.c,v 1.164.2.33.2.50 2008/03/13 15:56:21 iliaa Exp $ session Session Support => enabled Registered save handlers => files user sqlite Registered serializer handlers => php php_binary Directive => Local Value => Master Value session.auto_start => Off => Off session.bug_compat_42 => On => On session.bug_compat_warn => On => On session.cache_expire => 180 => 180 session.cache_limiter => nocache => nocache session.cookie_domain => no value => no value session.cookie_httponly => Off => Off session.cookie_lifetime => 0 => 0 session.cookie_path => / => / session.cookie_secure => Off => Off session.entropy_file => no value => no value session.entropy_length => 0 => 0 session.gc_divisor => 100 => 100 session.gc_maxlifetime => 1440 => 1440 session.gc_probability => 1 => 1 session.hash_bits_per_character => 4 => 4 session.hash_function => 0 => 0 session.name => PHPSESSID => PHPSESSID session.referer_check => no value => no value session.save_handler => files => files session.save_path => no value => no value session.serialize_handler => php => php session.use_cookies => On => On session.use_only_cookies => Off => Off session.use_trans_sid => 0 => 0 SimpleXML Simplexml support => enabled Revision => $Revision: 1.151.2.22.2.39 $ Schema support => enabled sockets Sockets Support => enabled SPL SPL support => enabled Interfaces => Countable, OuterIterator, RecursiveIterator, SeekableIterator, SplObserver, SplSubject Classes => AppendIterator, ArrayIterator, ArrayObject, BadFunctionCallException, BadMethodCallException, CachingIterator, DirectoryIterator, DomainException, EmptyIterator, FilterIterator, InfiniteIterator, InvalidArgumentException, IteratorIterator, LengthException, LimitIterator, LogicException, NoRewindIterator, OutOfBoundsException, OutOfRangeException, OverflowException, ParentIterator, RangeException, RecursiveArrayIterator, RecursiveCachingIterator, RecursiveDirectoryIterator, RecursiveFilterIterator, RecursiveIteratorIterator, RecursiveRegexIterator, RegexIterator, RuntimeException, SimpleXMLIterator, SplFileInfo, SplFileObject, SplObjectStorage, SplTempFileObject, UnderflowException, UnexpectedValueException SQLite SQLite support => enabled PECL Module version => 2.0-dev $Id: sqlite.c,v 1.166.2.13.2.10 2007/12/31 07:20:11 sebastian Exp $ SQLite Library => 2.8.17 SQLite Encoding => iso8859 Directive => Local Value => Master Value sqlite.assoc_case => 0 => 0 standard Regex Library => Bundled library enabled Dynamic Library Support => enabled Path to sendmail => /usr/sbin/sendmail -t -i Directive => Local Value => Master Value assert.active => 1 => 1 assert.bail => 0 => 0 assert.callback => no value => no value assert.quiet_eval => 0 => 0 assert.warning => 1 => 1 auto_detect_line_endings => 0 => 0 default_socket_timeout => 60 => 60 safe_mode_allowed_env_vars => PHP_ => PHP_ safe_mode_protected_env_vars => LD_LIBRARY_PATH => LD_LIBRARY_PATH url_rewriter.tags => a=href,area=href,frame=src,form=,fieldset= => a=href,area=href,frame=src,form=,fieldset= user_agent => no value => no value tokenizer Tokenizer Support => enabled xml XML Support => active XML Namespace Support => active libxml2 Version => 2.6.16 xmlreader XMLReader => enabled xmlrpc core library version => xmlrpc-epi v. 0.51 php extension version => 0.51 author => Dan Libby homepage => http://xmlrpc-epi.sourceforge.net open sourced by => Epinions.com xmlwriter XMLWriter => enabled xsl XSL => enabled libxslt Version => 1.1.12 libxslt compiled against libxml Version => 2.6.16 EXSLT => enabled libexslt Version => 1.1.12 zlib ZLib Support => enabled Stream Wrapper support => compress.zlib:// Stream Filter support => zlib.inflate, zlib.deflate Compiled Version => 1.2.3 Linked Version => 1.2.3 Directive => Local Value => Master Value zlib.output_compression => Off => Off zlib.output_compression_level => -1 => -1 zlib.output_handler => no value => no value Additional Modules Module Name Environment Variable => Value MANPATH => /usr/share/man:/usr/local/share/man:/usr/X11/man TERM_PROGRAM => Apple_Terminal TERM => xterm-color SHELL => /bin/bash TMPDIR => /var/folders/xz/xz32xxmfENeyp4S68o-abU+++TM/-Tmp-/ Apple_PubSub_Socket_Render => /tmp/launch-2T9Z8i/Render TERM_PROGRAM_VERSION => 240 OLDPWD => /Users/mtorbin USER => mtorbin COMMAND_MODE => unix2003 SSH_AUTH_SOCK => /tmp/launch-OYkmPh/Listeners __CF_USER_TEXT_ENCODING => 0x1F6:0:0 PATH => /usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin:/usr/X11/bin PWD => /Volumes/120gb_wd/scripts/php/brightcoveMigration LANG => en_US.UTF-8 SHLVL => 1 HOME => /Users/mtorbin LOGNAME => mtorbin DISPLAY => /tmp/launch-tB7IWM/:0 SECURITYSESSIONID => 632ca0 _ => /usr/bin/php PHP Variables Variable => Value _SERVER["MANPATH"] => /usr/share/man:/usr/local/share/man:/usr/X11/man _SERVER["TERM_PROGRAM"] => Apple_Terminal _SERVER["TERM"] => xterm-color _SERVER["SHELL"] => /bin/bash _SERVER["TMPDIR"] => /var/folders/xz/xz32xxmfENeyp4S68o-abU+++TM/-Tmp-/ _SERVER["Apple_PubSub_Socket_Render"] => /tmp/launch-2T9Z8i/Render _SERVER["TERM_PROGRAM_VERSION"] => 240 _SERVER["OLDPWD"] => /Users/mtorbin _SERVER["USER"] => mtorbin _SERVER["COMMAND_MODE"] => unix2003 _SERVER["SSH_AUTH_SOCK"] => /tmp/launch-OYkmPh/Listeners _SERVER["__CF_USER_TEXT_ENCODING"] => 0x1F6:0:0 _SERVER["PATH"] => /usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin:/usr/X11/bin _SERVER["PWD"] => /Volumes/120gb_wd/scripts/php/brightcoveMigration _SERVER["LANG"] => en_US.UTF-8 _SERVER["SHLVL"] => 1 _SERVER["HOME"] => /Users/mtorbin _SERVER["LOGNAME"] => mtorbin _SERVER["DISPLAY"] => /tmp/launch-tB7IWM/:0 _SERVER["SECURITYSESSIONID"] => 632ca0 _SERVER["_"] => /usr/bin/php _SERVER["PHP_SELF"] => sandbox.php _SERVER["SCRIPT_NAME"] => sandbox.php _SERVER["SCRIPT_FILENAME"] => sandbox.php _SERVER["PATH_TRANSLATED"] => sandbox.php _SERVER["DOCUMENT_ROOT"] => _SERVER["REQUEST_TIME"] => 1241054903 _SERVER["argv"] => Array ( [0] => sandbox.php ) _SERVER["argc"] => 1 _ENV["MANPATH"] => /usr/share/man:/usr/local/share/man:/usr/X11/man _ENV["TERM_PROGRAM"] => Apple_Terminal _ENV["TERM"] => xterm-color _ENV["SHELL"] => /bin/bash _ENV["TMPDIR"] => /var/folders/xz/xz32xxmfENeyp4S68o-abU+++TM/-Tmp-/ _ENV["Apple_PubSub_Socket_Render"] => /tmp/launch-2T9Z8i/Render _ENV["TERM_PROGRAM_VERSION"] => 240 _ENV["OLDPWD"] => /Users/mtorbin _ENV["USER"] => mtorbin _ENV["COMMAND_MODE"] => unix2003 _ENV["SSH_AUTH_SOCK"] => /tmp/launch-OYkmPh/Listeners _ENV["__CF_USER_TEXT_ENCODING"] => 0x1F6:0:0 _ENV["PATH"] => /usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin:/usr/X11/bin _ENV["PWD"] => /Volumes/120gb_wd/scripts/php/brightcoveMigration _ENV["LANG"] => en_US.UTF-8 _ENV["SHLVL"] => 1 _ENV["HOME"] => /Users/mtorbin _ENV["LOGNAME"] => mtorbin _ENV["DISPLAY"] => /tmp/launch-tB7IWM/:0 _ENV["SECURITYSESSIONID"] => 632ca0 _ENV["_"] => /usr/bin/php PHP License This program is free software; you can redistribute it and/or modify it under the terms of the PHP License as published by the PHP Group and included in the distribution in the file: LICENSE This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. If you did not receive a copy of the PHP license, or have any questions about PHP licensing, please contact license@php.net. -
I'm not sure if this is an installation problem or a syntax problem but I'm hoping you all can point me in the right direction. Here is the code: $files1 = scandir("/Volumes/my_dir/"); print_r($files1); The error I got was this: Fatal error: Call to undefined function scandir() Yet when I run php -v I get this: PHP 5.2.6 (cli) (built: Sep 19 2008 11:28:54) Copyright (c) 1997-2008 The PHP Group Zend Engine v2.2.0, Copyright (c) 1998-2008 Zend Technologies I installed Entropy's latest php for Mac. Any suggestions would be greatly appreciated. - MT
-
D'oh! Sometimes it just helps to type things out. Here's what the function should look like: function compareDates($firstDate, $secondDate) { $firstDate = strtotime($firstDate[0]); $secondDate = strtotime($secondDate[0]); if($firstDate == $secondDate) { return 0; } else if ($firstDate < $secondDate) { return 1; } else { return -1; } } Thanks for the forum! - MT
-
Please note there is a typo above. The function should read: function compareDates($firstDate, $secondDate) { if($firstDate[0] == $secondDate[0]) { return 0; } else if ($firstDate[0] < $secondDate[0]) { return 1; } else { return -1; } } This does not resolve the problem however.
-
I have a class I've built who's main goal is compare unix time stamps and reorder them in an array accordingly. I have them reordering ok, but I think the ordering determination is a big askew. Here is the function: function compareDates($firstDate, $secondDate) { if($firstDate[1] == $secondDate[1]) { return 0; } else if ($firstDate[1] < $secondDate[1]) { return 1; } else { return -1; } } which is initiated here: usort($assetList, 'compareDates'); Here is a sample of what the array might look like: $assetList[0][0] = "2008-02-06T07:53:55.297-04:00"; $assetList[0][1] = "080605_pc_PBT_retailup"; $assetList[1][0] = "2008-03-04T14:01:48.293-04:00"; $assetList[1][1] = "080604_pc_PBT_maria_sludge"; $assetList[2][0] = "2009-06-03T12:26:22.773-04:00"; $assetList[2][1] = "080603_pc_PBT_hummer"; $assetList[3][0] = "2008-06-06T12:16:22.157-04:00"; $assetList[3][1] = "080602_pc_PBT_wachovia2"; $assetList[4][0] = "2007-05-09T13:37:59.697-04:00"; $assetList[4][1] = "080530_pc_PBT_redlasso"; Is this the best way to do this? Why are the dates coming back incorrectly sorted? Thanks, - MT
-
I wanted to post this here because I figured it was well deserved. Awhile back I posted a thread on whether or not I should be using a class to define my structures and my functions. There was a lot of positive feedback on this and the end result is that I've learned how much more powerful classes are and I now almost exclusively write my PHP in OOP (thanks of course, to you guys). The side effect of this is that I'm now an much more effective programmer and a more valuable asset to my company. Thanks guys! - MT
-
Hey all, I don't know if this is possible but I want to know if there's a declaration that I can call to CANCEL a previously declared style. Consider the following: At some point in my page, I have something like this (fictional example): <style type="text/css">.myStyle{background-color:#ff0000; width:500px;}</style> Later on, I want to REMOVE the width declaration (not patch over it, I know how to do that) so that the only active declaration is the background-color. Is this possible? Thanks, - MT
-
simplexml_load_file errors: How Do Plan for Bad XML?
mtorbin replied to mtorbin's topic in PHP Coding Help
Neil, Thanks for this! I'll give it a go now. - MT -
I have a process that I've build which will load multiple XML documents into memory using simplexml_load_file. The problem is that some of the documents contain data that is screwy. Specifically, we have file URLs that contain +, ˙ and & which obviously causes the simplexml_load_file to fail. Any suggestions on this? Modifying the XML before simplexml_load_file is not an option because we're downloading it from a vendor site. Thanks, - MT
-
Yes, please excuse me. I did mean Ubuntu 8.10. I've since come to realize that (in my limited knowledge of Linux) that 8.10 does not OOTB work with the MSI Wind, or am I wrong?
-
I have an XML Feed which contains a bunch of assets, each having a ton of variables. I just built a class that can return this data but I'm curious what the best way to return this data is. Should I: 1) Write it as a CSV to an external file? 2) Pass it over as a multi-dimensional array (i.e. 2300 or so assets each with 20 or so variables) What suggestions do you guys have?
-
I just bought an MSI Wind (used) and I wanted to install Ubuntu on it. I've had a ton of trouble with 8.10 so I'm wondering if anyone else has used something else, specifically with the MSI Wind, that they like. Thanks, - MT
-
Finally, for those of you who are like me and need a written example of this, here's what worked for me: exec("NET USE Z: \\123.456.78.910\myDIR /USER:username password"); . . . [script runs] . . . exec("NET USE Z: /DELETE"); This will, if I wrote it properly in php, mount my remote server, run my script which is located locally, and then dismount from the remote server. Thanks again to Aaron for pointing me in the right direction! - MT