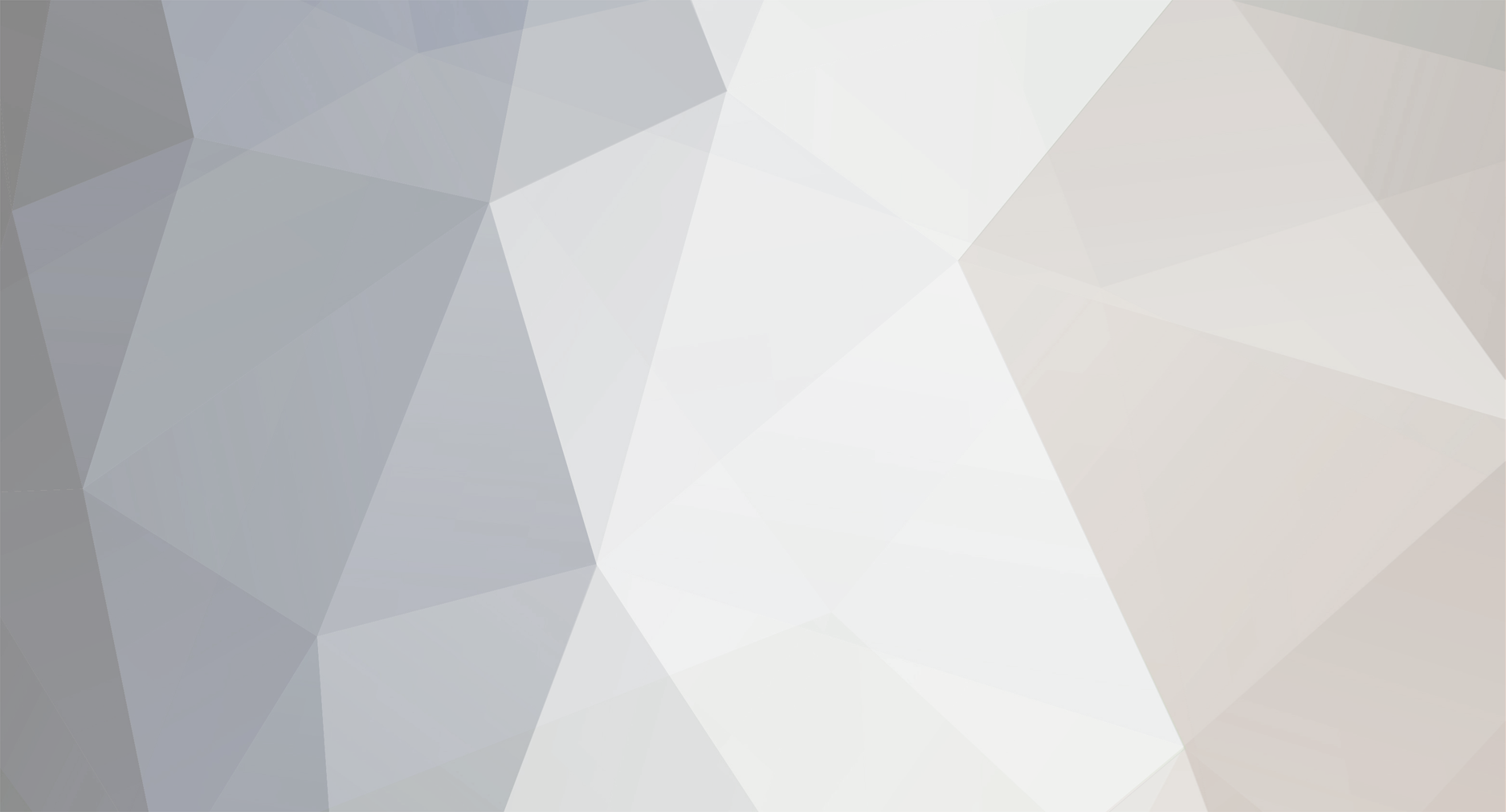
emehrkay
-
Posts
1,214 -
Joined
-
Last visited
Posts posted by emehrkay
-
-
I've never used JS with an xml file, but if it works the same as a regular HTML file then this shouldnt be too difficult.
First you want to get a collection of the title elements by doing
var items = document.getElementsByTagName('title');
Loop through that array and with every iteration run a check for your string
var collection = []; //empty array for(var x in items){ //this is the check var title_text = items[x].innerHtml; var reg_str = /Recipe of the Week/i; if(reg_str.test(title_text)){ var parent_node = items[x].parentNode; collection.push(parent_node); } }
now collection will be an array of all of the items who has a title with the string in it. To access the other nodes, you can either do
collection[0].childNodes[0]; //for the title, if the title is always the first childnode
or
collection[0].getElementsByTagName('link')[0]; //this will give you the link for that item
I havent tested this out so let me know if it doesnt work for you
-
You should really remove it from being inline, it will be a lot easier to handle and you can do simple things like if else statements to do what you want
var element = document.getElementById('element_id'); var clicked = false; element.onclick = function(){ clicked = (clicked) ? false : true;\ //do somehting }; element.onmouseover = function(){ if(!clicked){ //do soemthing } };
-
well the reason why it works in firefox is because your logic is flawed.
you want to check if shift and enter is pressed, but you never define sPressed as boolean and sPressed never changes in firefox so !sPressed returns true.
Run the code that i posted, you never get 'shift' in the log when shift is pressed
Do a search, possibly quirskmode will be your best resource, for crossbrowser keycodes and how to check for them
-
just move it outside of the loop
-
You are/were probably overwriting global variables which is very very easy to do in JS
-
does event.shiftKey work crossbrowser?
You may want to check that
//this is what i added to firebug and shift is never logged function hitkey(event) { console.log(event); var sPressed; if(event.shiftKey){ console.log('shift'); }else{ sPressed = false; } if (event.keyCode == 13 && !sPressed) { alert('send'); } } window.onkeyup = function(e){ hitkey(e); };
-
im pretty sure that user_input is undefined or numeric...probably undefined.
What you need to grab is the innerHTML of the option not the value.
-
I've just been casting it inside of the function, but I wonder why you're not able to limit it in the params
function foo(array $bar = array()){
$bar = (object) $bar;
}
-
I dont know what it is called exactly, but you know what I'm talking about (this is the reason why I'd probably fail the cert exam).
Anyway, when you cast the type of data that a function variable should be, can you specify an object?
I've tried a few things
function foo((object) array $bar = array()){} //cannot cast function foo((object) $bar = array()){} //cannot cast function foo(object $bar){} //cannot instanciate class $bar
Is it even possible?
thanks
-
That camel code is amazing
-
@tibberous - I like that one line because it is the perfect example of how the language is supposed to be used. My original code (the one I posted was wrong using % for minutes) works just the same, but it doesnt take advantage of the JavaScript Object structure.
pad() was a prototype to the String object written by the same guy. That too was pretty well written.
String.prototype.pad = function(l, s){ return ((l -= this.length) > 0 ) ? (s = new Array(Math.ceil(l / s.length) + 1).join(s)).substr(0, s.length) + this + s.substr(0, l - s.length) : this; };
-
-
You just have to know how to select elements. once you have them selected, all of the css properties are available to you.
In your example
var first_p = document.getElementsByTagName('p')[0];
first_p.sytle.color; //blue
-
element.style.whatever_attribute_you_need
-
I wrote this lil block of javascript to convert seconds to minutes:seconds for a count down timer
var m, s; m = (time % 60) ? Math.floor(time % 60) : 00; s = (time >= 60) ? Math.floor(time % 60) : time; if(time == 60){ m = 1; s = 00; }else if(time < 10){ s = '0' + s; } return m + ':' + s;
And then for whatever reason, I searched the web for the same thing and came across this
return Math.floor(seconds / 60).toFixed().pad(2,'0') + ':' + (seconds % 60).toFixed().pad(2, '0');
I was kinda jealous that I didnt come up with that. My block looks like amateur hour
-
Is this an attempt to make PHP a strong typed language?
It may be simpler to create a rule that people have to use the defined methods to set your properties...thats just my way of saying that I dont have an answer for your question.
If you want all fo the properties to use the setter, why declare some as public? Set them all to either private or protected and when you need to change or retrieve them, use simple getters and setters
-
Why do you want to make it an array? What are you trying to do? You may be adding more work
-
Elements are objects and the attributes are properties of that object
lets say you have this situation
input.element = {
type: 'text',
value: 'some value',
id: 'ele_id'
};
So you access it like
input.element.type or input.element['type'];
YOu can loop through the attributes using a for in
for(var x in input.element){
console.log(input.element[x]);
}
-
doesnt work in firefox...
Are you testing cross-browser?
I would suggest get yourself a copy of firefox and the firebug plugin. The script crashed right on that line of code...
-
This seems to be the error
self.status = "Current Tag is: " + window.event.srcElement.tagName;
what are you trying to do here? You cannot edit the status bar in browsers anymore
-
post it all, html included
-
Having the script in the head or the body doesnt matter, it all gets put to the memory.
You need to do
y.onkeyup = function(){func();}; because you need that function (func) to fire when a key is pressed
try
y.onkeyup = function(){
alert('key pressed');
};
-
did you try
y.onkeyup = function(){
sz(this);
};
?
-
is there any reason why there is a syntax error?
function sz(t) { a = t.value.split('\n'); b=1; for (i=0;i<a.length;i++) { if (a[i].length >= t.cols) b+= Math.floor(a[i].length/t.cols); } b+= a.length; if (b > t.rows) t.rows = b; }
i copied straight from the site, and it didnt work, i changed their x to my i and it still doesnt work, any ideas?
My guess, without looking at the rest of your code, is that you are using global variables when you dont write var in front of them. But there could be an error with my original code. try
y.onkeyup = function(){
sz(this);
}
Counting table rows
in Javascript Help
Posted
Well, it is becuase the javascript is run before the table is actually loaded into the document
do
onload = function(){
//add the functions that you want to run on page load here
};
or you can simply put the js at the bottom of the page to ensure it fires after loading, but window.onload or onload does just fine