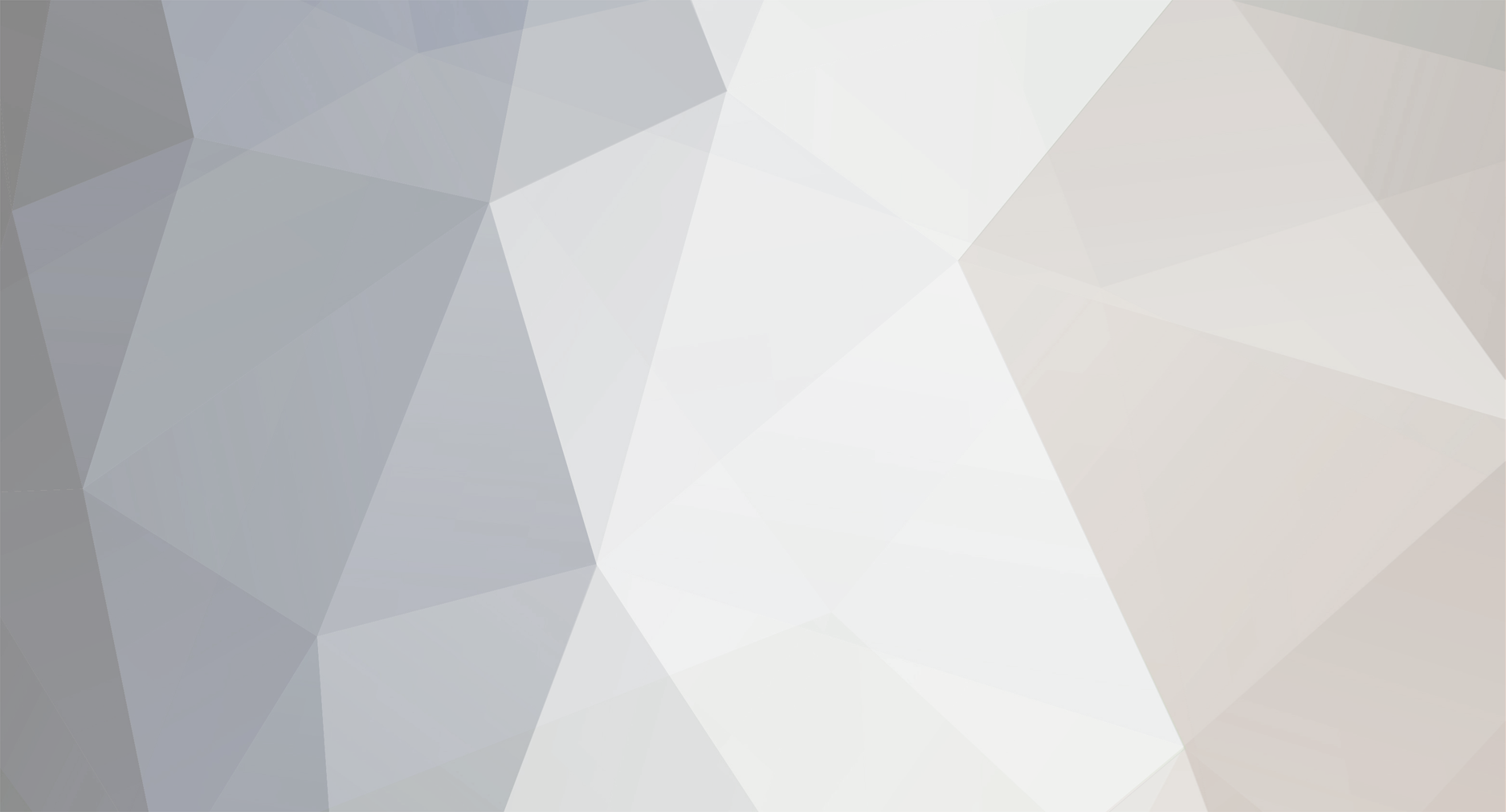
BrianM
-
Posts
217 -
Joined
-
Last visited
Never
Posts posted by BrianM
-
-
Problem solved!
Thanks for the help, rhodesa!
-
I fixed that problem, now I'm getting this error
Notice: Undefined variable: encrypt_password in C:\Program Files\Apache Group\Apache2\htdocs\test.php on line 15
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Testing</title> </head> <?php if(isset($_POST['submit'])) { $_POST['password'] = $password; $encrypt_password = md5($password); } echo 'This is your password, encrypted:' . $encrypt_password; // line 15 echo '<form action="</test.php>" method="post">'; echo '<input type="password" name="password" />'; echo '<input type="submit" name="submit" />'; echo '</form>'; ?> <body> </body> </html>
-
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Testing</title> </head> <?php if(isset($_POST['submit'])) { $_POST['password'] = $password; $encrypt_password = md5($password); } echo 'This is your password, encrypted:' . $encrypt_password; echo '<form action='</test.php>' method='post'>'; // line 17 echo '<input type='password' name='password' />'; echo '<input type='submit' name='submit' />'; echo '</form>'; ?> <body> </body> </html>
Parse error: parse error in C:\Program Files\Apache Group\Apache2\htdocs\test.php on line 17
The error isn't very informative. Any advice?
-
Yes, what were the results?
-
Yes, what are the results?
-
<html> <head> <script src="selectuser.js"></script> </head> <body> <form id="form1" name="form1" method="post" action="checking.php"> <p><strong>KEV Claim</strong></p> <table width="497" border="0"> <tr> <td width="284">Claim</td> <td width="203"><select name="name" id="name" onChange="showUser(this.value)"> <?php $host = 'localhost'; $user = 'root'; $password = 'admin'; $dbase = 'claim'; $dblink = mysql_connect($host, $user, $password); mysql_select_db($dbase, $dblink); $query = "SELECT staff_no, name FROM user ORDER BY name"; $result = mysql_query($query); while($myrow = mysql_fetch_row($result)) { echo "<option value=\"" . $myrow[0] . "\">" . $myrow[1]."</option>"; } ?> </select></td> </tr> </table> <p> </p> <p> <input type="submit" name="Submit" value="Submit"> </p> </form> </body> </html>
<?php $host = 'localhost'; $user = 'root'; $password = 'admin'; $dbase = 'claim'; $dblink = mysql_connect($host, $user, $password); mysql_select_db($dbase, $dblink); echo "<table border=3 align=center width=90%>"; echo "<tr><td>ID</td>"; echo "<td>Month</td>"; echo "<td>Start Date </td>"; echo "<td>End Date</td>"; echo "<td>Name</td>"; echo "<td>Staff No</td>"; echo "<td>Details</td>"; echo "<td>Amount</td>"; echo "<td>GL code</td>"; echo "<td>Bank </td>"; echo "<td>Account No. </td><tr>"; $name = $_POST['name']; $query = "SELECT report1.id, month, start_date, end_date, user.name, report1.staff_no, details, amount, gl_code, user.bank, user.acc_no FROM user, report1 WHERE report1.staff_no = '$name'"; $result = mysql_query($query, $dblink); while($myrow = mysql_fetch_array($result)) { echo "<tr><td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td>"; echo "<td>" . $myrow . "</td></tr>"; } echo "</table>"; ?>
-
Any advice?
-
So state again what exactly it is that your wanting the page to do when you click the submit button.
-
$name = $_POST['name']; $query = "SELECT report1.id, month, start_date, end_date, user.name, report1.staff_no, details, amount, gl_code, user.bank, user.acc_no FROM user, report1 WHERE report1.staff_no = '$name'"; $result = mysql_query($query, $dblink); while($myrow = mysql_fetch_array($result)) { echo "<tr><td>" . $myrow[0] . "</td>"; echo "<td>" . $myrow[1] . "</td>"; echo "<td>" . $myrow[2] . "</td>"; echo "<td>" . $myrow[3] . "</td>"; echo "<td>" . $myrow[4] . "</td>"; echo "<td>" . $myrow[5] . "</td>"; echo "<td>" . $myrow[6] . "</td>"; echo "<td>" . $myrow[7] . "</td>"; echo "<td>" . $myrow[8] . "</td>"; echo "<td>" . $myrow[9] . "</td>"; echo "<td>" . $myrow[10] . "</td></tr>"; }
Try that.
-
$query = "SELECT report1.id, month, start_date, end_date, user.name, report1.staff_no, details, amount, gl_code, user.bank, user.acc_no FROM user, report1 WHERE user.name = '$name'";
That should work.
-
Rohan, now you want to help with the script I posted!?
-
Is there any certain order I should put the code, or a syntax error I made?
-
Well, I was on a little vacation for a few days, back now.
I tried the code and my page doesn't display anything when I add in what you gave me to use :| arg. I get nothing with error_reporting(E_ALL); ... so can anyone give me a hand and point me in the right direction, please?
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>MPS - Send Client Data</title> </head> <?php $mysql_hostname = "localhost"; $mysql_username = "brian"; $mysql_password = ""; $mysql_database = "mps"; $mysql_connect = mysql_connect("$mysql_hostname", "$mysql_username", "$mysql_password") or die(mysql_error()); mysql_select_db("$mysql_database") or die(mysql_error()); mysql_select_db("$mysql_database"); $result = mysql_query("SELECT * FROM `08-12345-1`"); if(isset($_POST['text'])) { $text = $_POST['text']; $resultsUpdate = mysql_query("UPDATE table SET text='$text' WHERE id=4"); } $resultsShow = mysql_query("SELECT id, text FROM table WHERE id=4") or die(); $valuesShow = mysql_fetch_array($resultsShow); ?> <table> <tr> <td> <?php if($_GET['action'] != 'edit') { echo $valuesShow['text'] . '<br /><br />'; echo "a href='http://www.game-zero.org/mps/projects/project_08-12345-1.php?action=edit'>Click here to edit the text</a>"; } else { ?> <form name="formEdit" method="post" action="http://www.game-zero.org/mps/projects/project_08-12345-1.php"> <textarea name="text"><?php echo $valuesShow['text']; ?></textarea> <input type="submit" name="Submit" value="Submit" /> </form> <?php } ?> </td> </tr> </table> <?php echo "<table border=1> <tr> <th> Permit Process </th> <th> Req </th> <th> Submittal Date </th> <th> Comments Received Date </th> <th> Resubmit Date </th> <th> Comments Received Date </th> <th> Resubmit Date </th> <th> Comments Received Date </th> <th> Resubmit Date </th> <th> Comments Received Date </th> <th> Resubmit Date </th> <th> Approval Date </th> <th> Permit Number </th> <th> CoC Req Date </th> <th> CoC Submittal Date </th> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr>"; echo "<td><center><b>" . $row['PermitProcess'] . "</b></center></td>"; echo "<td><center><b>" . $row['Req'] . "</b></center></td>"; echo "<td><center><b>" . $row['SubmittalDate'] . "</b></center></td>"; echo "<td><center><b>" . $row['CommentsReceivedDate1'] . "</b></center></td>"; echo "<td><center><b>" . $row['ResubmitDate1'] . "</b></center></td>"; echo "<td><center><b>" . $row['CommentsReceivedDate2'] . "</b></center></td>"; echo "<td><center><b>" . $row['ResubmitDate2'] . "</b></center></td>"; echo "<td><center><b>" . $row['CommentsReceivedDate3'] . "</b></center></td>"; echo "<td><center><b>" . $row['ResubmitDate3'] . "</b></center></td>"; echo "<td><center><b>" . $row['CommentsReceivedDate4'] . "</b></center></td>"; echo "<td><center><b>" . $row['ResubmitDate4'] . "</b></center></td>"; echo "<td><center><b>" . $row['ApprovalDate'] . "</b></center></td>"; echo "<td><center><b>" . $row['PermitNumber'] . "</b></center></td>"; echo "<td><center><b>" . $row['CoCReqDate'] . "</b></center></td>"; echo "<td><center><b>" . $row['CoCSubmittalDate'] . "</b></center></td>"; echo "</tr>"; } echo "</table> </font>"; mysql_close($mysql_connect); ?> <body> </body> </html>
-
Is there any trick to using background-color with tables, because it doesn't seem to want to work for me. Here is an example of how I am writing my code, maybe somebody can tell me what I'm doing wrong and point me in the right direction.
<table class="login">
...
</table>
and in my style sheet linked to the page I have something like
table.login {
background-color: #FFFFFF;
}
Thanks for any help/advice.
-
Thank you very much, schme16!
Well my ISP is blocking port 80 then. *shakes fist* I used to have business internet with them about a year ago, which ran around $90/month, and apparently that's what it takes to open the port. Just another way for them to get more money, because the guy that owns it obviously isn't happy with the hundreds of millions (or maybe even more) I'm sure that he has.
-
Can somebody please just give me a 'yes' or 'no'? People are obviously reading the post as it states, but I don't know why you wont give a simple 'yes' or 'no', it only takes about 5 seconds.
-
Can somebody please let me know?
-
I know this doesn't really belong here, but it's about the only forums with members actually viewing it this time of morning. I am having a bit of trouble getting users outside of my LAN to access my website on port 80 so I am wondering if anyone here can give me a hand and let me know if you can view it on port 81; http://www.game-zero.org:81/ if you can view this website (wont say much besides, "Site under construction...") then this means my ISP has blocked port 80 on my account and I'll need to contact them tomorrow sometime. So if anyone reading this can view my website, please let me know here so I can take appropriate action. http://www.game-zero.org:81/
-
I can definitely see this working. Although, yes, I will have to alter tables and what not. I'll report back and let you know how it goes.
-
-
Way to put it lightly.
I try, I try. Well then again, he's just learning, and two, he didn't write that script himself, so really it was somebody else's mistake. But just a lesson learned in clean/formal code.
-
Whoa. I completely forgot about the last else. That would mess everything up.
It would have spelled disaster.
As for the $PHP_SELF; you were using, try to get used to using a full global variable like so;
<?php $_SERVER['PHP_SELF']; ?>
-
Or I was going to say, use this right here. I rewrote it just a bit, but made sure you wont get any errors when you load the page.
<html> <head> <title>Personal INFO</title> </head> <?php if (isset($_POST['submit'])) { echo "Hello, ".$_POST['first_name']." ".$_POST['last_name'].".<br /><br />"; echo "You are ".$_POST['gender'].", and you like "; echo $_POST['food'].".<br /><br />"; echo "".$_POST['quote'].".<br /<br />"; echo "You're favorite time is ".$_POST['TofD'].", and you passed ".$_POST['education']."!"; } ?> <body> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> First Name:<input type="text" size="12" maxlength="12" name="first_name"> Last Name:<input type="text" size="12" maxlength="36" name="last_name"> Gender: Male:<input type="radio" value="Male" name="gender"> Female:<input type="radio" value="Female" name="gender"> Please choose type of residence: Steak:<input type="checkbox" value="Steak" name="food"> Pizza:<input type="checkbox" value="Pizza" name="food"> Chicken:<input type="checkbox" value="Chicken" name="food"> <textarea rows="5" cols="20" name="quote" wrap="physical">Enter your favorite quote!</textarea> Select a Level of Education: <select name="education"> <option value="Jr.High">Jr.High</option> <option value="HighSchool">HighSchool</option> <option value="College">College</option></select> Select your favorite time of day: <select name="TofD" size="3"> <option value="Morning">Morning</option> <option value="Day">Day</option> <option value="Night">Night</option></select> <input type="submit" value="submit" name="submit"> </form> </body> </html>
-
Thank you very much, both of you!
Why wont my table update? :|
in PHP Coding Help
Posted
Here is my code:
I execute
UPDATE `08-12345-1` SET Req='some_text' WHERE PermitProcess='COUNTY PRE-APP MEETING'
in the phpMyAdmin query window and it works just fine, updates the table in my browser, but when I go to use the same statement in this script, it doesn't want to cooperate... :| anyone see any problem with it? I'll be really excited if somebody can figure this out, as I've been at this same part of my website for about a week now, with no further progress due to this problem.