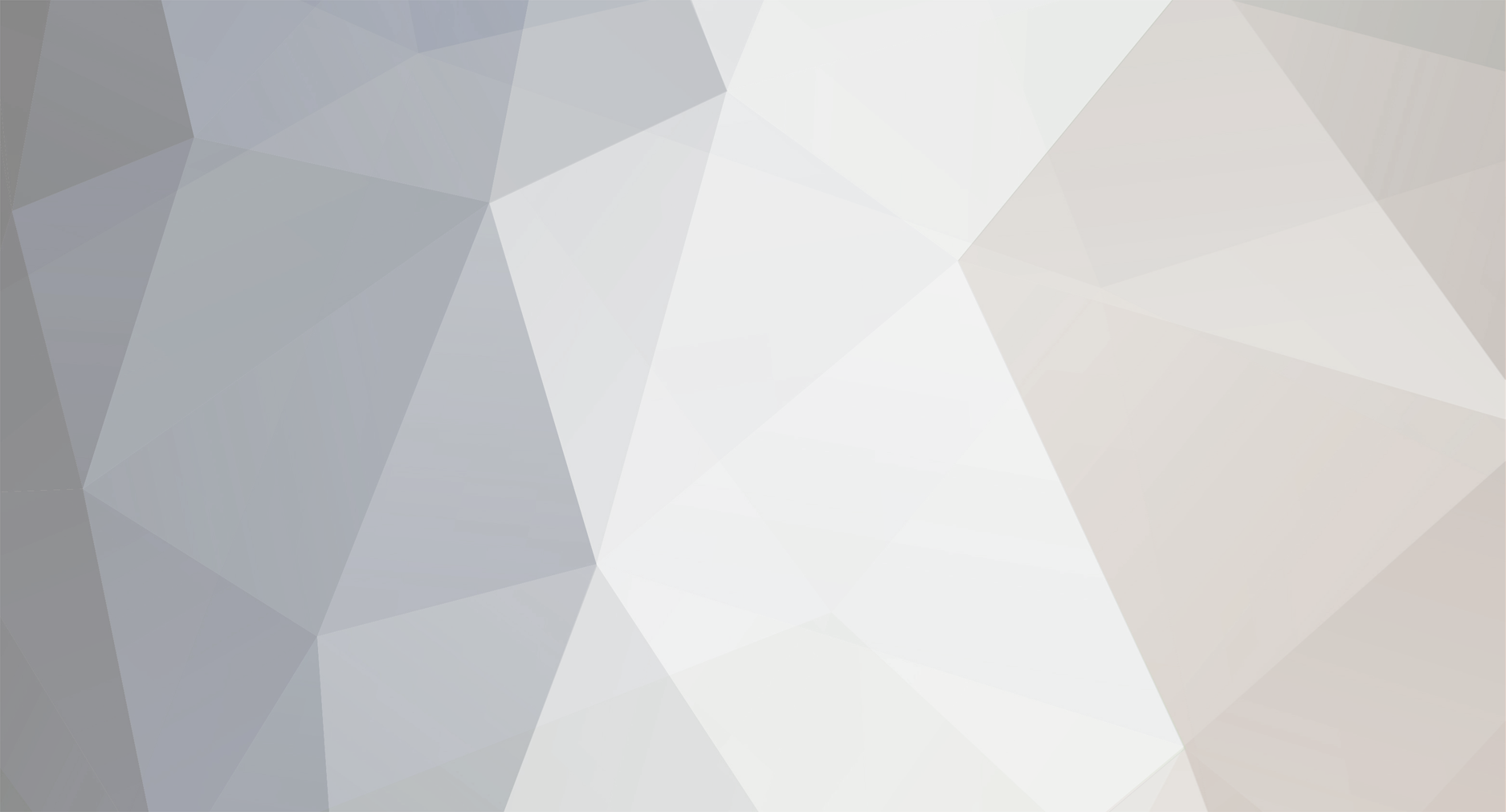
atrum
Members-
Posts
184 -
Joined
-
Last visited
About atrum
- Birthday 09/10/1984
Profile Information
-
Gender
Male
atrum's Achievements

Regular Member (3/5)
0
Reputation
-
So it has recently occurred to me that my method of error handling is sloppy and cumbersome. What I want to do is record all of the errors that occur and allow the page to finish loading. Then display them in a designated error panel on my page. Granted that if I come across a major error I do want to stop the script but for minor errors such as incorrect credentials or some other custom error I may want to display I want to neatly display them all without stopping the script. Can anyone offer any advice?
-
Oh yeah I forgot I still had this old link up. I fixed it here http://icca.exiled-alliance.com. I changed the name of it so that it was more general. I still haven't put anything in those tabs. Kinda lost interest in this thing but it gets a lot of use at my work place. Every time my web server goes down, I get spammed by people wanting to know when it would be fixed haha.
-
Ok, thanks Ignace.
-
So there really is no right or wrong way to use them then other than trying to keep them primarily for Setter use. Cool, thank you Thorpe.
-
So, I recently discovered what method chaining is and how it can be used. My question about method chaining is in regards to best practice. What I want to know is what the correct way to implement methods that can be chained are, and what pitfalls will I need to watch out for when using them?
-
Help separating http header information from json encoded string.
atrum replied to atrum's topic in PHP Coding Help
Here is the solution I came up with using Curl instead of Fsockopen. <?php //Curl Test Function define("HOST","api.host.com"); //API HOST define("ACCT_INFO","/account/information/1.0/"); //Defines the api path for getting account and product details. define("KEY_ID","XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"); //Defines key identification code. define("SECRET_KEY","XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"); //Defines secret response. function hmacsha1($secret, $string){ $blocksize = 64; $hashfunc = 'sha1'; if (strlen($secret) > $blocksize) $secret = pack('H*', $hashfunc($secret)); $secret = str_pad($secret,$blocksize,chr(0x00)); $ipad = str_repeat(chr(0x36),$blocksize); $opad = str_repeat(chr(0x5c),$blocksize); $hmac = pack('H*',$hashfunc(($secret^$opad).pack('H*',$hashfunc(($secret^$ipad).$string)))); return $hmac; } function fetch_info($customer,$action,$method){ if($customer!==""){ if($action!==""){ $accept = 'application/json'; // Assign json application $proxy = "https://".HOST.$action.$customer; // Assign the proxy (URL/URI) $path = HOST.$action.$customer; // Authorization Header $date = gmdate('r'); // GMT based timestamp $string = join("\n", array("GET", $accept, $date, $proxy)); // Join string with new lines $signature = base64_encode(hmacsha1(SECRET_KEY, $string)); // Create authorization signature $ch = curl_init(); //Initialize the Curl Session. //Set all the necessary headers to authenticate. $headers = array( "$method $path HTTP/1.1", "Host: ".HOST, "Accept: $accept", "Content-Type: $accept", "Authorization: VKEY ".KEY_ID.":$signature", "Date: $date", "Connection: Close" ); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); //Feeds the header details into the request. curl_setopt($ch, CURLOPT_HEADER, 0); //Mostly for debugging. if 3rd argument is 1, include header response. curl_setopt($ch, CURLOPT_URL, $proxy); //Sets the URL/PATH. curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); //Allows returned data to be stored in a variable for later use. $results = json_decode(curl_exec($ch), true); //Returned data in an associative array. curl_close($ch); //Close the curl session return $results; //Returns an array of data requested. }else{ $error = "Missing Argument: [Action]"; return $error; } }else{ $error = "Missing Argument: [Customer ID]"; return $error; } } ?> -
Hey man, thanks a lot for the help on that. That is a much cleaner way of doing this.
-
I got it figured out. So far it works out exactly the same when I manually work out the numbers. <?php //Initialize Variables if($_POST['num1']!=="" || $_POST['num2']!==""){ $num1 = $_POST['num1']; $num2 = $_POST['num2']; }else{ die("Both fields require a number"); } //Function calulates the Greatest Common Factor from 2 given numbers. function gcd($num1,$num2){ while($num1 !== $num2){ if($num1 > $num2){ //While $num1 is equal $num2 $num1 = $num1 - $num2; //Subtract $num1 from $num2 $gcd = $num1; }else{ $num2 = $num2 - $num1; //Subtract $num1 from $num2 $gcd = $num2; } if($gcd <=1){ //Checks if the gcd is lower than 1. if it is, then stop the loop and make gcd equal to the number before we hit 0 $gcd = $num1; break; } } $result = "The GCD of ".$_POST['num1'] ." and ". $_POST['num2']." is : ".$gcd; return $result; } echo gcd($_POST['num1'],$_POST['num2']); ?>
-
Hello everyone. So in my programing class which is starting out doing flow charts. We were asked to do a flow chart that shows the process of calculating the GCD from 2 numbers. I wanted to try it out for real in php. The issue I am having is that after the first time its used, any attempt to use it after that results in a never ending loop that ends up tanking my server, and I have to restart apache to fix the problem. I am trying to come up with a solution to prevent that but I am drawing a blank. Looping is still somewhat new to me. At least when dealing with just numbers. Here is my code,. If anyone can offer any advice I would appreciate it. <?php //Initialize Variables if($_POST['num1']!=="" || $_POST['num2']!==""){ $num1 = $_POST['num1']; $num2 = $_POST['num2']; }else{ die("Both fields require a number"); } //Function calulates the Greatest Common Factor from 2 given numbers. function gcd($num1,$num2){ while($num1 !== $num2){ if($num1 > $num2){ //While $num1 is equal $num2 $num1 = $num1 - $num2; //Subtract $num1 from $num2 $gcd = $num1; }else{ $num2 = $num2 - $num1; //Subtract $num1 from $num2 $gcd = $num2; } } $result = "The GCD of ".$_POST['num1'] ." and ". $_POST['num2']." is : ".$gcd; return $result; } echo gcd($_POST['num1'],$_POST['num2']); ?>
-
Hello all, I am working on a project that has a function in it that I don't completely understand. From what I can understand about it; it appears to create a hash from 2 strings. The parts I don't understand is the methods it is going about it with. Here is the function. If someone could please break this down for me into its parts with a brief explanation of what each part is doing, I would be very appreciative. <?php function hmacsha1($secret, $string){ $blocksize = 64; $hashfunc = 'sha1'; if (strlen($secret) > $blocksize) $secret = pack('H*', $hashfunc($secret)); $secret = str_pad($secret,$blocksize,chr(0x00)); $ipad = str_repeat(chr(0x36),$blocksize); $opad = str_repeat(chr(0x5c),$blocksize); $hmac = pack('H*',$hashfunc(($secret^$opad).pack('H*',$hashfunc(($secret^$ipad).$string)))); return $hmac; } ?>
-
Help separating http header information from json encoded string.
atrum replied to atrum's topic in PHP Coding Help
Yo, Gizmola. I got this all working through curl now. So much easier than fsockopen. Thanks again for your help! -
I actually got it figured out. I think that example is very out of date or something. I don't even need the fopen function to make it work. I have a new issue now, but I will make a new post since it's not related to curl or fopen. Thanks though. Here is basically what I did. <?php $ch = curl_init(); //Initialize the Curl Session. $headers = array( "GET $path HTTP/1.1", "Host: $host", "Accept: $accept", "Content-Type: $accept", "Authorization: VKEY $keyid:$signature", "Date: $date", "Connection: Close" ); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_URL, $proxy); $results = curl_exec($ch); curl_close($ch); ?>
-
hello all, I am trying to teach my self how to use curl to get the contents of a remote file. I am using the example provided on the php.net website under the curl example. <?php //Curl driven verio api test $ch = curl_init("http://curtisdorris.com/"); //Initialize the Curl Session. $fp = fopen("index.php","r"); //Open the file for reading only curl_setopt($ch, CURLOPT_FILE, $fp); curl_setopt($ch, CURLOPT_HEADER, 0); curl_exec($ch); curl_close($ch); fclose($fp); ?> I am getting the following warnings and I am unable to find any information as to why I am getting them. It still works but I really want to take care of these warnings before I continue further. Warning: fopen(index.php) [function.fopen]: failed to open stream: No such file or directory in /home/UID/www/tools.exiled-alliance.com/apitest/curltest.php on line 4 Warning: curl_setopt(): supplied argument is not a valid File-Handle resource in /home/UID/www/tools.exiled-alliance.com/apitest/curltest.php on line 6 Can anyone offer any in-sight as to wtf is going on?
-
Help separating http header information from json encoded string.
atrum replied to atrum's topic in PHP Coding Help
Awesome. I will let you know if I need any help. Thanks. -
Help separating http header information from json encoded string.
atrum replied to atrum's topic in PHP Coding Help
The authentication required is over www-auth. Can curl or fopen do that? I've only used curl and fopen. At there most basic