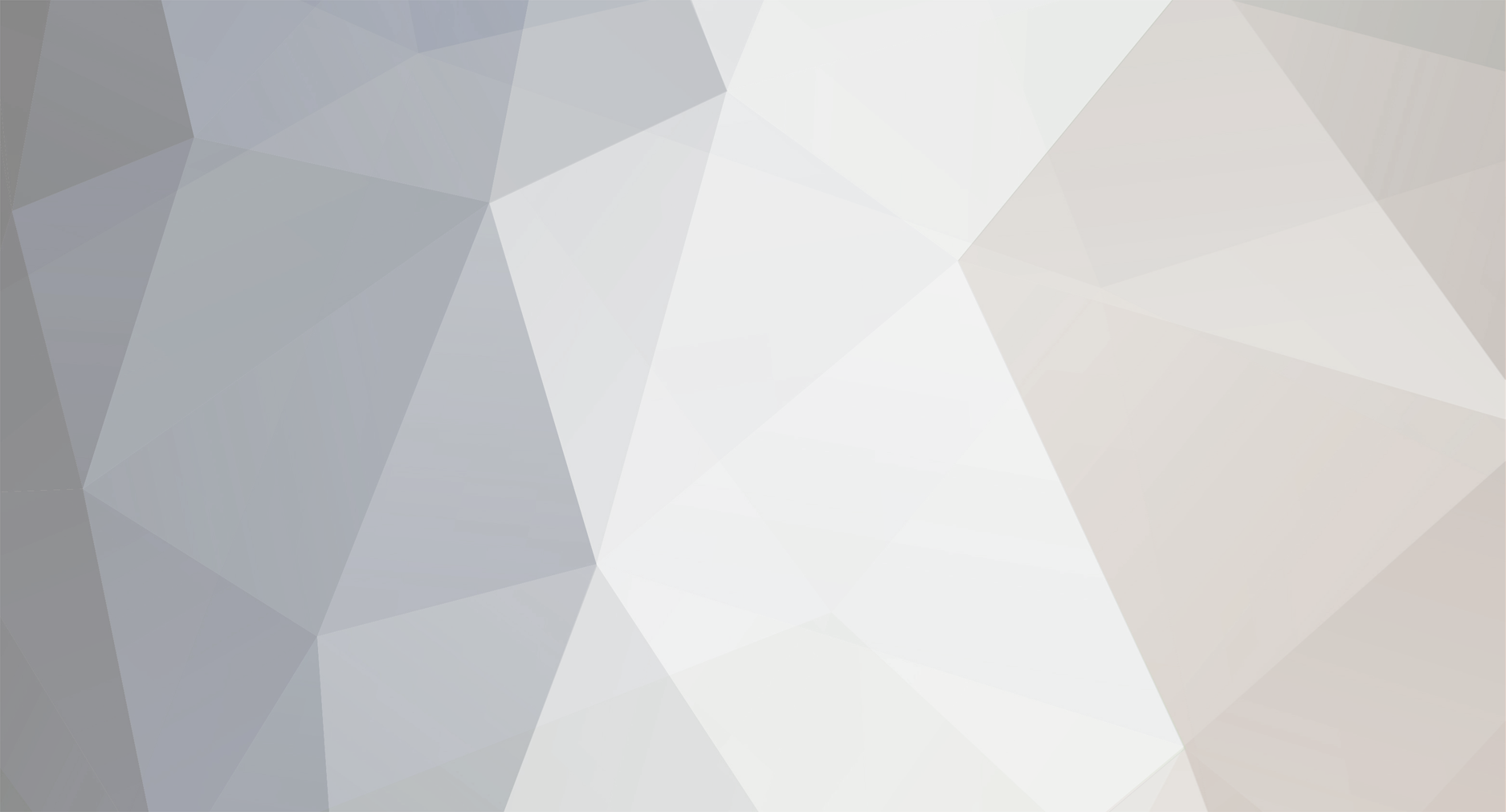
next
Members-
Posts
140 -
Joined
-
Last visited
Everything posted by next
-
[SOLVED] db connection class (prob another easy one)
next replied to CaptainChainsaw's topic in PHP Coding Help
You have an error in your syntax. In constructor for instance: $this->$_dbhost=$dbhost; should be: $this->_dbhost=$dbhost; properties don't have a '$' prefix. -
I'm a bit confused with OOP, why even use regular methods like: $user = new User($a); $user->promote(); if the same can be accomplished in static method much easier: User::promote($a) I just feel like making all of my classes static. What am i missing in this picture?
-
thorpe, thanks this answers my question perfectly! NathanLedet , nah, it would append that to my current location. New Coder , this was a work around that i was using until now, the problem is that if you change files location it's not going to work anymore.
-
I'm trying to redirect when user is logged out: http://localhost/ahk_php/sidebar_links/logout.php to http://localhost/ahk_php/index.php here's my code, logout.php: <?php session_start(); require_once($_SERVER['DOCUMENT_ROOT'] . '/ahk_php/config.php'); session_destroy(); header('location: ' . $_SERVER['HTTP_HOST'] . '/ahk_php/index.php'); ?> But here instead of: http://localhost/ahk_php/index.php i get: http://localhost/ahk_php/sidebar_links/localhost/ahk_php/index.php Why is it appending instead of replacing url completely?
-
Actually i see it the other way around, if you decide to change the way information is passed in a form you just need to change the method and $_REQUEST takes care of the rest, but i'm new to PHP to know all the cons. Btw, it worked, thanks!
-
corbin, yeah i usaly prefer to be more specific with global too, but this time decided to try out $_REQUEST. I tested it, and it does pass necessary info. Nightslyr, thanks i'll try it out!
-
lol, i always do that, thanks i'll try it out
-
I wrapped PDO in my class but have a weird problem with my execute method: <?php class Database { private static $dbh; private function __construct() {} private static function connect() { if(!isset(self::$dbh)) { try { self::$dbh = new PDO(DB_DSN, DB_USER, DB_PASSWORD, array(ATTR_PERSISTENT => true)); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } } return self::$dbh; } public static function close() { self::$dbh = null; } public static function execute($sql) { try { $handle = self::connect(); $stmt = $handle->prepare($sql); $stmt->execute(); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } } public static function getAll($sql, $style = PDO::FETCH_ASSOC) { $result = null; try { $handle = self::connect(); $stmt = $handle->prepare($sql); $stmt->execute(); $result = $stmt->fetchAll($style); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } return $result; } public static function getRow($sql, $style = PDO::FETCH_ASSOC) { $result = null; try { $handler = self::connect(); $stmt = $handler->prepare($sql); $stmt->execute(); $result = $stmt->fetch($style); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } return $result; } } ?> If you will look at getAll or getRow you'll notice that i have to type in the same lines of code: $handler = self::connect(); $stmt = $handler->prepare($sql); $stmt->execute(); these lines make up "execute" method: public static function execute($sql) { try { $handle = self::connect(); $stmt = $handle->prepare($sql); $stmt->execute(); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } } So if i try to replace getAll with this: public static function getAll($sql, $style = PDO::FETCH_ASSOC) { $result = null; try { $self::execute($sql); $result = $stmt->fetchAll($style); } catch(PDOException $e) { self::close(); trigger_error($e->getMessage(), E_USER_ERROR); } return $result; } i get an error: Why is this happening? Those are the same lines of code. Any idea?
-
$_REQUEST can be used regardless of what method is used in a form.
-
I'm trying to figure out how to create a User class, but i'm stuck and have no clue how to proceed. I'm currently stuck with "register" method and it's error display. Here's what i have: User class: <?php class User { private $user_name; private $password; private $keep_logged_in; private $found; public $errors; public function __construct($user_name, $password) { $this->user_name = $user_name; $this->password = $password; } public function logIn() { $found = Database::getRow("CALL user_exists('$this->user_name', '$this->password')"); if($found) { session_register($this->user_name); $_SESSION['user_name'] = $this->user_name; } header('location: index.php'); } public function register() { $found = Database::getRow("CALL name_taken('$this->user_name')"); if($found) $errors .= "<li>user name is taken.</li>\r\n"; if(strlen($this->user_name) > 50) $errors .= "<li>user name is to long</li>\r\n"; if(!$errors) { Database::execute("CALL user_register('$this->user_name', '$this->password')"); header('location: index.php'); } else $this->displayErrors(); } public function displayErrors() { echo "<ul>\r\n"; echo $errors; echo "</ul>\r\n"; } } ?> Registration page: <?php require_once('header.php'); if($_REQUEST['submit']) { $user_name = trim($_POST['username']); $password = trim($_REQUEST['password']); $user = new User($user_name, $password); $user->register(); } ?> <form action="<?php $_SERVER['PHP_SELF']; ?>" method="post" id="registration"> <label for="username">User name:</label><input name="username" type="text" id="username" size="15" maxlength="50" /> <label for="password">Password:</label><input name="password" type="password" id="password" size="15" maxlength="100" /> <input name="submit" type="submit" id="submit" value="register" /> </form> Script does detect if user name is already taken or if it's to long, but it doesn't display errors, how can i fix it? Also, does anyone know a good tutorial on such class? Thanks.
-
Variable declaration is still optional, so PPP is not required. Of course at first i made them private but it just gave me similar error: <?php class User { private $user_name = trim(mysql_real_escape_string($_REQUEST['username'])); private $password = trim(md5($_REQUEST['password'])); private $keep_logged_in = isset($_REQUEST['remember']) ? true : false; public static $logged_in = false; public static $error_msg; public static function logIn() { if(isset($user_name) && isset($password)) { $found = Database::execute('CALL user_exists($user_name)') ? true : false; if($found) { session_register($user_name); self::$logged_in = true; } else self::$error_msg = "<p id=\"error\">Sorry, user name and password don't match.</p>"; header('location: ' . INDEX_PAGE); return self::$logged_in; } } } ?> gives me: this didn't make any sense, so i removed "private". This looks like a nice article that you reference, but i already know that, can you be a bit more specific with "improperly use classes"? Thanks.
-
<?php class User { $user_name = trim(mysql_real_escape_string($_REQUEST['username'])); $password = trim(md5($_REQUEST['password'])); $keep_logged_in = isset($_REQUEST['remember']) ? true : false; public static $logged_in = false; public static $error_msg; public static function logIn() { if(isset($user_name) && isset($password)) { $found = Database::execute('CALL user_exists($user_name)') ? true : false if($found) { session_register($user_name); self::$logged_in = true; } else self::$error_msg = "<p id=\"error\">Sorry, user name and password don't match.</p>"; header('location: ' . INDEX_PAGE); exit(); return self::$logged_in; } } } ?> When i run my index page i get an error: this is line 3: i tried removing trim and real_escape, but it didn't help. Any ideas on what might be wrong here ??? Thanks.
-
I doesn't show any PEAR info.
-
So does this mean that i already have PEAR armed and ready to use on my machine if i run PHP 5.2.5? I have WOS(server on a stick) package, how can i check if i already have PEAR and what version? Thanks.
-
Thanks for the info, i googled esnipe, didn't know something like this existed, lol. Yes, that is partially what i want to do. Where do i start? Thanks again
-
no, i want php to parse a text file and send retrieved information to eBay, example: auction number: 11111111111111, amount: $50.00 i want php to bid $50 on auction 1111111111111.
-
Sorry for making mess, problem solved. Thanks.
-
I can't seem to figure out how to display array elements on my page. $result = Database::getAll('CALL entry_preview(100)'); if i use print_r($result) is get the following: i tried foreach($result as $key => $value) { echo $key . "<br />" . $value . "<br />"; but this isn't working. Please help. Thanks!
-
So far i like MySql Tools a lot(official MySql GUI). Very good piece of software. Supports procedures, functions, views, tabbed interface, nice help and overview on the right side, pretty and clean interface, easy access to Query Browser from Administration and vice-versa. Few drawbacks that i found so far: 1) interface is not resizable. 2) It has some issues with OUT variables in procedures, i had to use cmd to see the return value. Alternative is HeidiSql, but i don't think that this one supports procedures, at least i failed at figuring out how to create and manage them. Another alternative is PhpMyAdmin, but quite frankly managing databases online is ridiculous, way to slow. However it support all MySql features. All the above is free, MySql Tools can be found on MySql official site.
-
i think it's to late with masking sensitive information . If you will read those errors you'll understand that your user has no password set and apparently your $mysql_password variable has some value assigned to it, therefore you are failing to connect. Correct this and the rest should work just fine. Cmon, you should know that it's against math rules to divide by 0, you need to fix you expression.
-
it just said that i have a syntax error at line 6, nothing specific. Thanks for your help, i'll use TIMESTAMP instead.
-
i'm trying to create a table, but get an error at this line for some reason: date_registered DATE DEFAULT(CURRENT_DATE) says i have a syntax error. Any ideas how to fix this?
-
I have a text file filled with auction item numbers and amounts, how can i make php parse txt file and send that info to ebay? I know how to work with files in php, but i have no clue how to pass that info to eBay. Can you point me in the right direction?
-
Ok, so i checked out smarty and to be honest i'm really disappointed. Things that i didn't like: a) syntax b) "if(blah = blah)" is not the same as "if (blah = blah)", smarty pretty much forces you to you a space. I'm pretty sure there is more, but i don't want to look. What template engine is easy to learn with a nice syntax and is not that picky? Thanks.