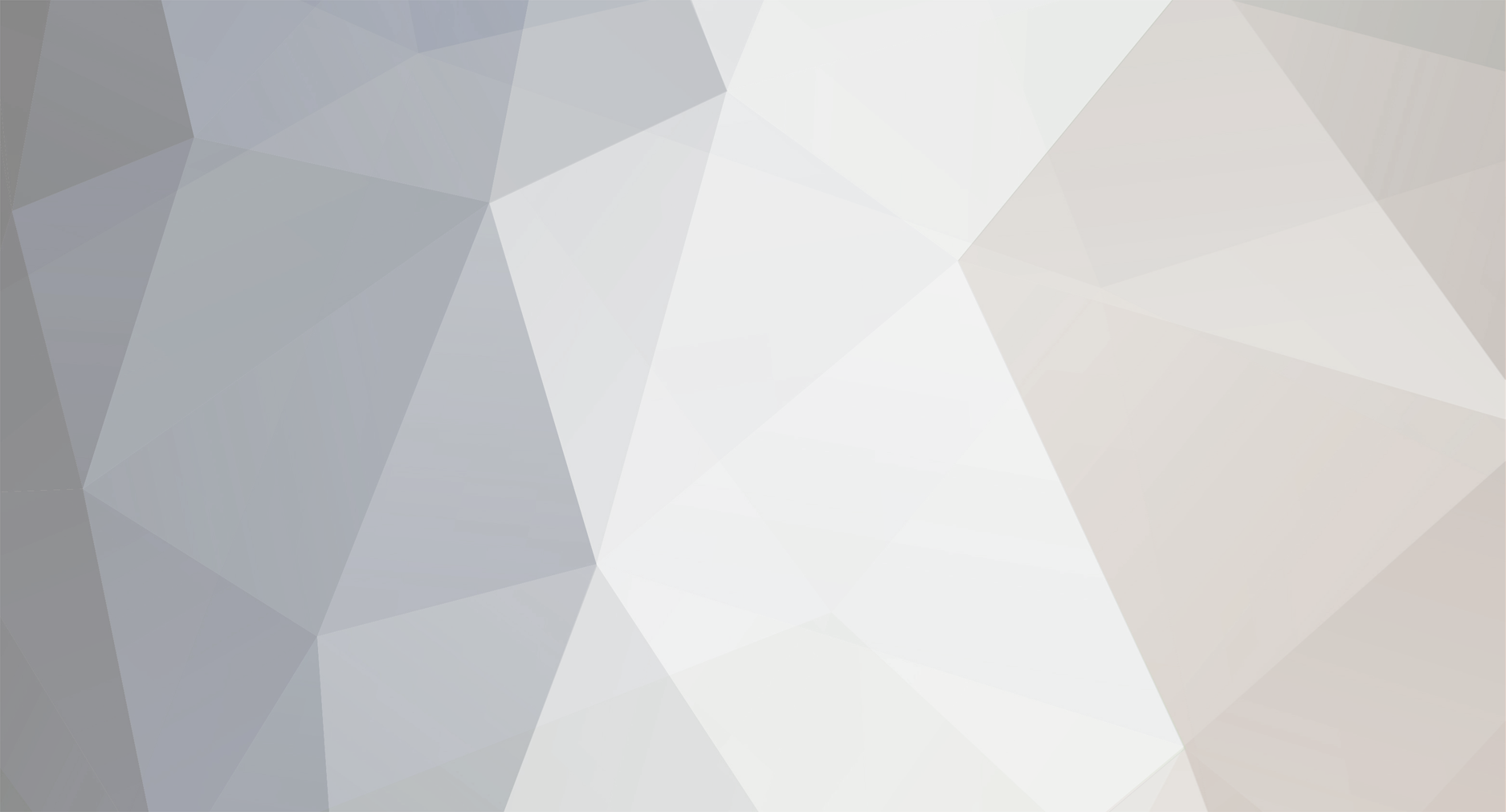
mac007
Members-
Posts
194 -
Joined
-
Last visited
Everything posted by mac007
-
Hello, all: I'm trying to learn and do a simple foreach loop so I can upload multiple files at once, but all I actually get in umy "picts" directory is an "Array" file... maybe I got the $key variable in wrong places? Thanks! <code> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1" /> <title>Untitled Document</title> </head> <body> <?php if(isset($_FILES['picture']['tmp_name'])) { $fileArray = $_FILES['picture']['tmp_name']; foreach ($fileArray as $key) { move_uploaded_file($key,"picts/" . $_FILES['picture']['name']); } } ?> <form method="post" enctype="multipart/form-data"> <label> <input type="file" name="picture[]" id="picture[]" /> <br /> <input type="file" name="picture[]" id="picture[]" /> <br /> <input type="file" name="picture[]" id="picture[]" /> </label> <p> <input type="submit" name="button" id="button" value="Submit" /> </p> </form> </body> </html> </code>
-
Hi, all: I need to have a form, where people can select different quantities of different products, and then submit all at once to cart. I was reading that one can use the "multiple_products_cart_quantity" form structure, so I set this code up, that has 2 product id's, but it's not working right, only added the last item. What am I doing wrong?? appreciate the help! Thanks! <form name="multiple_products_cart_quantity" action='http://www.mysite.com/shopping-carts/zen-cart/index.php?main_page=product_info&action=add_product' method='post' enctype='multipart/form-data'> <input type="text" name="cart_quantity" value="1" maxlength="6" size="4" /><br /><br /><input type="hidden" name="products_id" value="1" /> <input type="text" name="cart_quantity" value="1" maxlength="6" size="4" /><br /><br /><input type="hidden" name="products_id" value="2" /> <input type="image" src="includes/templates/template_default/buttons/english/button_in_cart.gif" alt="Add to Cart" title=" Add to Cart " /> </form>
-
Hello, all: I'm trying to sanitize/secure my query, and it all seems ok when I test it with most special-characters... but when I try to test the single quote (') like this... www.mysite.com/page.php?category=' Then it gives me this error: "You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''''' at line 1" It seems to do it only when I test it on the category variable... it only does it with single quotes, it's Ok with double quotes; so I dont get it... So if I test it with the other variables like this... http://www.sitetemplates101.com/workCategories.php?category=1&type=' http://www.sitetemplates101.com/workCategories.php?category=1&type=2&filter=' Then it works fine, it simply refreshes or disregards entry... See here below the code-snippet i have... what am I doing wrong??? Thanks!! PS. Forgot to mention I have .htaccess to have magic-quotes OFF <CODE> // THESE ARE VARIABLES $colname1_worksRS = "-1"; $colname2_worksRS = "-1"; $colname3_worksRS = "-1"; if (isset($_GET['category'])) { $colname1_worksRS = mysql_real_escape_string($_GET['category']);} if (isset($_GET['type'])) { $colname2_worksRS = mysql_real_escape_string($_GET['type']);} if (isset($_GET['filter'])) { $colname3_worksRS = mysql_real_escape_string($_GET['filter']);} // THIS IS COMPOUND SELECT STATEMENT ACCORDING TO CALLED VARIABLES $query_worksRS = "SELECT * FROM works"; if (!empty($_GET['category'])) { $query_worksRS .= " WHERE Type = '$colname1_worksRS'"; } if (!empty($_GET['type'])) { $query_worksRS .= " AND Subject = '$colname2_worksRS'"; } if ((!empty($_GET['filter'])) && $_GET['filter'] == 'Price') { $query_worksRS .= " ORDER BY Price DESC"; } elseif ($_GET['filter'] == 'Size') { $query_worksRS .= " ORDER BY Size DESC"; }else { $query_worksRS .= " ORDER BY ProductID DESC"; } </CODE>
-
Hi, all: I am trying trying to integrate one of these 2 carts for our store, but we do lots of landing pages where we then pass a hidden "source" code either thru url or thru post-form in order to track which of these landing pages people came from that bought a product, so on url it would be appended like this: http://www.mysite.com/.... &source=mediaworld and on form like this... <input type="hidden" name="source" value="mediaworld" /> But I noticed these carts dont really read product info thru a normal form, but more like thru functions... is there a way to bypass this and force to take the added product-field info?? Though it does look like Zen does have a minimalized form... Thanks... appreciate any help...
-
why do I still get error when placing a ' in url even after I sanitize...
mac007 replied to mac007's topic in PHP Coding Help
Thanks PFM; but I did try that too... instead of the filter_input() functio, but it still did the same thing... Maybe I'm not setting it up correctly?? -
Hi, all: need some help clarifying couple things about sanitizing... As you can see in the code below, I am using FILTER_INPUT() function to sanitize the url variables being sent, since these are being used to make SELECT data based on what variables are being called... but when I add a single-quote to the string to test it like this: page.php?category=' Then I get an error: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''''' at line 1 I also get similar errors if I type url like: page.php?category=1' But it doesnt givbe me any errors if I do any other of the special-characters like <, >, ", &, or stuff of that sort. It's funny, cause if I have url like this: page.php?category=18&type=' I dont get any errors! Appreciate the help... Thanks // THESE ARE VARIABLES $colname1_worksRS = filter_input(INPUT_GET, 'category', FILTER_SANITIZE_SPECIAL_CHARS); $colname2_worksRS = filter_input(INPUT_GET, 'type', FILTER_SANITIZE_SPECIAL_CHARS); $colname3_worksRS = filter_input(INPUT_GET, 'filter', FILTER_SANITIZE_SPECIAL_CHARS); // THIS IS COMPOUND SELECT STATEMENT ACCORDING TO CALLED VARIABLES $query_worksRS = "SELECT * FROM works WHERE 1"; if (!empty($_GET['category'])) { $query_worksRS .= " AND Type = '$colname1_worksRS'"; } if (!empty($_GET['type'])) { $query_worksRS .= " AND Subject = '$colname2_worksRS'"; } if ((!empty($_GET['filter'])) && $_GET['filter'] == 'Price') { $query_worksRS .= " ORDER BY Price DESC"; } elseif ($_GET['filter'] == 'Size') { $query_worksRS .= " ORDER BY Size DESC"; }else { $query_worksRS .= " ORDER BY ProductID DESC"; } [code]
-
Hi, all: Have a general question on mysql_pconnect and mysql_free_result()... If one uses the "permanent" connection (mysql_pconnect), should I, as standard practice, always "close" any recordset-queries with mysql_free_result()?? what kind of problems would come up if I didnt?? And then again, if I was using just the "non-permanent" connection (mysql_connect), am I to assume that one doesnt need to "close" any queries then?? Thanks!
-
quick question on mysql_pconnect and mysql_free_result()...
mac007 posted a topic in PHP Coding Help
Hi, all: Have a quick question on mysql_pconnect and mysql_free_result()... If one uses the "permanent" connection (mysql_pconnect), should I, as standard practice, always "close" any recordset-queries with mysql_free_result()?? what kind of problems would come up if I didnt?? appreciate any feedback... -
Thanks Premiso... Yeah, well, it's a very long page, so I just wanted overall guidance since not sure where to even begin... I'll check for loops... already found a repeating mysql_select_db line in there. Could it also be any of these: 1) that I been refreshing page over and over again as I make changes and test page... coudl it be that I keep calling the connection too many times? 2) my connection call is "permanent" (mysql_pconnect), and some of these recordsets I didnt "close" (mysql_free_result), coudl that be an issue?? Thanks!
-
Hello, All: Sometimes I get this "Out of memory" warning when I load my page... this page has several recordsets that pull all kinds of records from a mysql-database. What would be the reasons I get this warning? or what steps should I take to avoid that from happening? could it be repetitive scripts? or repetitive connection calls?? Appreciate any help...
-
hey, Andy: well, I worked out... kind of, I guess anyway; I ended up duplicating a whole new recordset off the original one... renaming all references by adding SUM at end of recordset-variables. $query_RecordsetSUM = "SELECT *, SUM(noorder) AS monthSum FROM ucn WHERE 1"; if (!empty($_GET['agentid'])) { $query_RecordsetSUM .= " AND agentid = '$agent'"; } if (!empty($_GET['skillname'])) { $query_RecordsetSUM .= " AND skillname = '$skillname'"; } if (!empty($_GET['ani'])) { $query_RecordsetSUM .= " AND ani = '$ani'"; } if (!empty($_GET['dnis'])) { $query_RecordsetSUM .= " AND dnis = '$dnis'"; } if (!empty($_GET['startdate1'])) { $query_RecordsetSUM .= " AND startdate BETWEEN '$startingdate1' AND '$startingdate2'"; } $query_RecordsetSUM .= " GROUP BY first ORDER BY startdate DESC"; $RecordsetSUM = mysql_query($query_RecordsetSUM, $natureCityMembers) or die(mysql_error()); $row_RecordsetSUM = mysql_fetch_assoc($RecordsetSUM); and the SUM line I changed to this... $monthSum = $row_RecordsetSUM['monthSum']; I didnt try, listign the colum names... I did was able to get it thru at first by putting GROUP BY line at very end of select-statement, but before the ORDER BY, but then I noticed it was then GROUPING the records, so that if "John" appeared 8 times, it would only show 1 time. That's why I thought the only way woudl be to use the GROUP BY on a duplicated recordset.. and looks like it worked! Thanks a lot, you got me in the right direction!
-
Yes, Andy, I want to use the $Recordset1 query. Here's the whole $Recordset1 select statement, which is kind of long cause I have a form-filtering setup to pull specific records based on form-choice submissions... mysql_select_db($database_natureCityMembers, $natureCityMembers); $agent = $_GET['agentid']; $skillname = $_GET['skillname']; $ani = $_GET['ani']; $dnis = $_GET['dnis']; $startdate1 = $_GET['startdate1']; $daydate1 = $_GET['daydate1']; $yeardate1 = $_GET['yeardate1']; $startingdate1 = $yeardate1."-".$startdate1."-".$daydate1; $startdate2 = $_GET['startdate2']; $daydate2 = $_GET['daydate2']; $yeardate2 = $_GET['yeardate2']; $startingdate2 = $yeardate2."-".$startdate2."-".$daydate2; $query_Recordset1 = "SELECT * FROM ucn WHERE 1"; if (!empty($_GET['agentid'])) { $query_Recordset1 .= " AND agentid = '$agent'"; } if (!empty($_GET['skillname'])) { $query_Recordset1 .= " AND skillname = '$skillname'"; } if (!empty($_GET['ani'])) { $query_Recordset1 .= " AND ani = '$ani'"; } if (!empty($_GET['dnis'])) { $query_Recordset1 .= " AND dnis = '$dnis'"; } if (!empty($_GET['startdate1'])) { $query_Recordset1 .= " AND startdate BETWEEN '$startingdate1' AND '$startingdate2'"; } $query_Recordset1 .= " ORDER BY startdate DESC"; $query_limit_Recordset1 = sprintf("%s LIMIT %d, %d", $query_Recordset1, $startRow_Recordset1, $maxRows_Recordset1); $Recordset1 = mysql_query($query_limit_Recordset1, $natureCityMembers) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); Appreciate the help!
-
Hello, all: well, I just realized my Sum() mysql-select statement is adding all records as oppposed to just the ones being returned. Problem is I have 2 select statements. This first one selects a set or records based on a variety of filtering-options... $row_Recordset1 = mysql_fetch_assoc($Recordset1); and this select-statement, SUMS the "noorder" column, but it SUMS all records in db, as opposed to just the ones being pulled from statement above... how can I link statements so it sums just records being pulled?? <?php $select = mysql_query("SELECT SUM(noorder) AS monthsum FROM ucn"); $monthSum=mysql_fetch_array($select); echo $monthSum['monthsum']; ?> Appreciate any help...
-
Hello, all: I have several of these type of lines, each of them representing a column, where I simply sum() each column (in this example, these are "return_useave" & "return_success", then I divide that amount by number of rows, and then I multiply by 100 to get a percentage number. It works fine throughout, specially when several rows come up, or if no-rows come up, BUT if only 1 row comes up, and sum is 0, then the "return_usesave" column shows "100%", instead of 0%. Really weird, cause exact same script shows just fine for other the "return_success" column! Seems like it's picking up a 1 in the (sum), but cant see how if sum seems like should be 0... cause if change the value to 1 in the DB, then it becomes 200%!... Appreciate any help! This column shows wrong: <?php $select = mysql_query("SELECT SUM(return_usesave) AS monthsum FROM ucn"); $monthSum=mysql_fetch_array($select); echo number_format($monthSum['monthsum'] / $totalRows_Recordset1*100); ?> But this column shows just fine: <?php $select = mysql_query("SELECT SUM(return_success) AS monthsum FROM ucn"); $monthSum=mysql_fetch_array($select); echo number_format($monthSum['monthsum'] / $totalRows_Recordset1)*100; ?>
-
Need to have simple "search" form filter records from database
mac007 replied to mac007's topic in PHP Coding Help
I see what you are saying Syed... I will try your code a bit later today. Hope I can make it work... makes so much sense. Also, you are right Ben, I'll switch that to POST after I get my code finalized. Many times it's easier for me to use GET when I'm in testing mode... Let you guys know how it goes... -
Hello, all: I am trying to do like a mini-search script, where records are filtered based on submitted-form values. Basically, sample submitted-fields may be like: agent and skillname. Thing is, I want to have it so one can search from a combination of both terms or just by itself. In other words, one could enter just "John" and all the Jonhs would come up, or just enter "tv" under skillname and all the TV skillnames would then show up. But then, let's say I also want to be able to further filter this search, like submit both "john" and "tv" and only those records that have those in common woudl show up. Thing is, I will need to add even more filtering fields (like agentid, date, type, etc)... I setup this if-else script here, and seems to work. But it's pretty amateurish I know, so there must be a better way to have it do the filtering. Appreciate any help... $agent = $_GET['agent']; $skillname = $_GET['skillname']; if (!empty($_GET['agent']) && !empty($_GET['skillname'])) { $query_Recordset1 = "SELECT * FROM ucn WHERE agentid = '$agent' AND skillname = '$skillname' ORDER BY startdate DESC"; } elseif (!empty($_GET['agent'])) { $query_Recordset1 = "SELECT * FROM ucn WHERE agentid = '$agent' ORDER BY startdate DESC"; } elseif (!empty($_GET['skillname'])) { $query_Recordset1 = "SELECT * FROM ucn WHERE skillname = '$skillname' ORDER BY startdate DESC"; } else { $query_Recordset1 = "SELECT * FROM ucn ORDER BY startdate DESC"; }
-
I have the folloiwing javascript "hide/show" code, that shows a user specific checkboxes depending on what gets clicked... problem is, any clicked checkboxes that are within these "hide/show" divs retain their checked values even if one hides them... so, seems javascript is simply hiding whole selected "checkbox" and sending value thru anyways.. So, do I need DOM-Javascript? so actual divs are completely removed or added? But I'm not a javascript guy, and I'm havign a hard time modifyign this to do that... Appreciate any help! here's javascript: <script type="text/javascript"> <!-- function toggle_visibility(id) { var e = document.getElementById(id); if(e.style.display == 'none') e.style.display = 'block'; else e.style.display = 'none'; } //--> </script> <script type="text/javascript"> <!-- function toggle_visibilityNone(id) { var e = document.getElementById(id); if(e.style.display == 'block') e.style.display = 'none'; else e.style.display = 'none'; } //--> </script> <script type="text/javascript"> <!-- function toggle_visibilityYes(id) { var e = document.getElementById(id); if(e.style.display == 'none') e.style.display = 'block'; else e.style.display = 'block'; } //--> </script> And here is HTML FORM code: <form action="<?php echo $editFormAction; ?>" id="insertForm" name="insertForm" method="POST"> <p> </p> <table width="375" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td colspan="3" align="center"><img src="../images/nc_title_logo.gif" width="342" height="54" /></td> </tr> <tr> <td colspan="3"> </td> </tr> <tr> <td> </td> <td colspan="2" align="center"> </td> </tr> <tr> <td width="314"><span class="style5">No Order completed in this call </span></td> <td width="61" colspan="2" align="center"> <input name="noorder" type="checkbox" id="noorder" value="checkbox" onclick="toggle_visibility('box');" /> </td> </tr> <tr> <td colspan="3"> </td> </tr> <tr> <td colspan="3"><div id="box"> <table width="100%" border="0" cellpadding="0" cellspacing="0"> <tr> <td width="100%"><table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr class="color-table"> <td > </td> <td align="left"> </td> <td align="left"> </td> </tr> <tr class="color-table"> <td > </td> <td align="left"><strong>YES</strong></td> <td align="left"><strong>NO</strong></td> </tr> <tr> <td width="80%" class="color-table"> 1) Did you attempt an Upsell?</td> <td class="color-table"><input type="radio" name="upsell" value="Y" onclick="toggle_visibilityYes('upsellBox');"/></td> <td align="center" class="color-table"><input type="radio" name="upsell" value="N" onclick="toggle_visibilityNone('upsellBox');"/></td> </tr> </table></td> </tr> <tr> <td class="color-table"> </td> </tr> <tr> <td class="color-table"><div id="upsellBox"> <table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="80%" class="color-table"> 2) Was the Upsell successful? </td> <td class="color-table"><input type="radio" name="upsellStatus" value="Y" /></td> <td align="center" class="color-table"><input type="radio" name="upsellStatus" value="N" /></td> </tr> <tr> <td class="color-table"> </td> <td class="color-table"> </td> <td align="center" class="color-table"> </td> </tr> </table> </div></td> </tr> <tr> <td class="color-table-2"> </td> </tr> <tr> <td class="color-table-2"><table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td class="color-table-2"> </td> <td align="left" class="color-table-2"><strong>YES</strong></td> <td colspan="2" align="left" class="color-table-2"><strong>NO</strong></td> </tr> <tr> <td width="80%" class="color-table-2"> 3) Did you attempt a Cross-Sell? </td> <td class="color-table-2"><input type="radio" name="cross" value="Y" onclick="toggle_visibilityYes('crossBox');"/></td> <td colspan="2" align="center" class="color-table-2"><input type="radio" name="cross" value="N" onclick="toggle_visibilityNone('crossBox');"/></td> </tr> </table></td> </tr> <tr> <td class="color-table-2"> </td> </tr> <tr> <td><div id="crossBox"> <table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="80%" class="color-table-2"> 4) Was the Cross-Sell successful? </td> <td class="color-table-2"><input type="radio" name="crossStatus" value="Y" /></td> <td align="center" class="color-table-2"><input type="radio" name="crossStatus" value="N" /></td> </tr> <tr> <td class="color-table-2"> </td> <td class="color-table-2"> </td> <td align="center" class="color-table-2"> </td> </tr> </table> </div></td> </tr> </table> </div> </td> </tr> <tr> <td> </td> <td colspan="2" align="center"> </td> </tr> <tr> <td><input name="agentid" type="hidden" id="agentid" value="<?php echo $_GET['agentid']; ?>" /> <input name="first" type="hidden" id="first" value="<?php echo $_GET['first']; ?>" /> <input name="last" type="hidden" id="last" value="<?php echo $_GET['last']; ?>" /> <input name="startdate" type="hidden" id="startdate" value="<?php $newdate = $_GET['startdate']; $newdateMonth = substr($newdate,0,2); $newdateDay = substr($newdate,2,2); $newdateYear = substr($newdate,4,4); $newFinalDate = $newdateYear . "-" . $newdateMonth . "-" . $newdateDay; echo $newFinalDate; ?>" /> <input name="inqueue" type="hidden" id="inqueue" value="<?php echo $_GET['inqueue']; ?>" /> <input name="skill" type="hidden" id="skill" value="<?php echo $_GET['skill']; ?>" /> <input name="skillname" type="hidden" id="skillname" value="<?php echo $_GET['skillname']; ?>" /> <input name="contactid" type="hidden" id="contactid" value="<?php echo $_GET['contactid']; ?>" /> <input name="masterid" type="hidden" id="masterid" value="<?php echo $_GET['masterid']; ?>" /> <input name="dnis" type="hidden" id="dnis" value="<?php echo $_GET['dnis']; ?>" /> <input name="ani" type="hidden" id="ani" value="<?php echo $_GET['ani']; ?>" /> </td> <td colspan="2" align="center"><input type="submit" name="Submit" value="Submit" /></td> </tr> </table> <input type="hidden" name="MM_insert" value="insertForm"> </form>
-
Hi, PFM... thanks for the tip! it worked beautifully! This is the code I used if it helps anybody... <?php $newdate = $_GET['startdate']; $newdateMonth = substr($newdate,0,2); $newdateDay = substr($newdate,2,2); $newdateYear = substr($newdate,4,4); $newFinalDate = $newdateYear . "-" . $newdateMonth . "-" . $newdateDay; echo $newFinalDate; ?> the final echo line is what I used to populate my hidden "date" form field... which inserts the date into my table's DATE field.
-
Hi, all: I have this problem, where I want to be able to insert a date that's coming from url-variable, like this... www.site.com/page.php?name=john&date=06152009 Also, as you see, the date-variable format comes as month, day, year. So how can I insert this date into my mysql db table? I can usually insert current times into my table with functions like NOW(), and other similar stuff, but how do I grab this variable and make it possible to insert back into my DATE field in my table?? thanks!! appreciate any help...
-
sorry, didnt answer earlier... had to step out for a while.. yes, it's a mysql database; but the validation is in php first. Then the value gets UPDATED in this mysql statement: $updateQuery = mysql_query("UPDATE BUDGETS SET Jan=$Jan, Feb=$Feb, Mar=$Mar, Apr=$Apr, May=$May, Jun=$Jun, Jul=$Jul, Aug=$Aug, Sep=$Sep, Oct=$Oct, Nov=$Nov, BUDGETS.Dec=$Dec, notes='$notes' WHERE recordid = $accountid AND recordcustid = '$member'");
-
Thanks, Ken... I tried your code but still doing same thing... I reversed if / elseif statements, and added === as you suggested; but still no luck... $Sep= $_POST['Sep']; if(is_null($Sep)) { $Sep = 'NULL';} elseif ($Sep === 0) { $Sep = "0.0";} I dotn get why mysql is not understanding the is_null() function... I mean that pretty much indicates that field is blank, doenst it? as opposed to empty()...
-
Hi PFM... thanks for your help, I got this to work, kind off with this code: $Sep= $_POST['Sep']; if ($Sep == 0) { $Sep = "0.0";} BUT NOW, if the form fields are left blank, then it automatically inserts 0.00 (not blank, which I also want the DB fields to remain). I tried to modify to this, but didnt seem to work... $Sep= $_POST['Sep']; if ($Sep == 0) { $Sep = "0.0";} elseif(is_null($Sep)) { $Sep = 'NULL';} Any ideas? Thanks again...