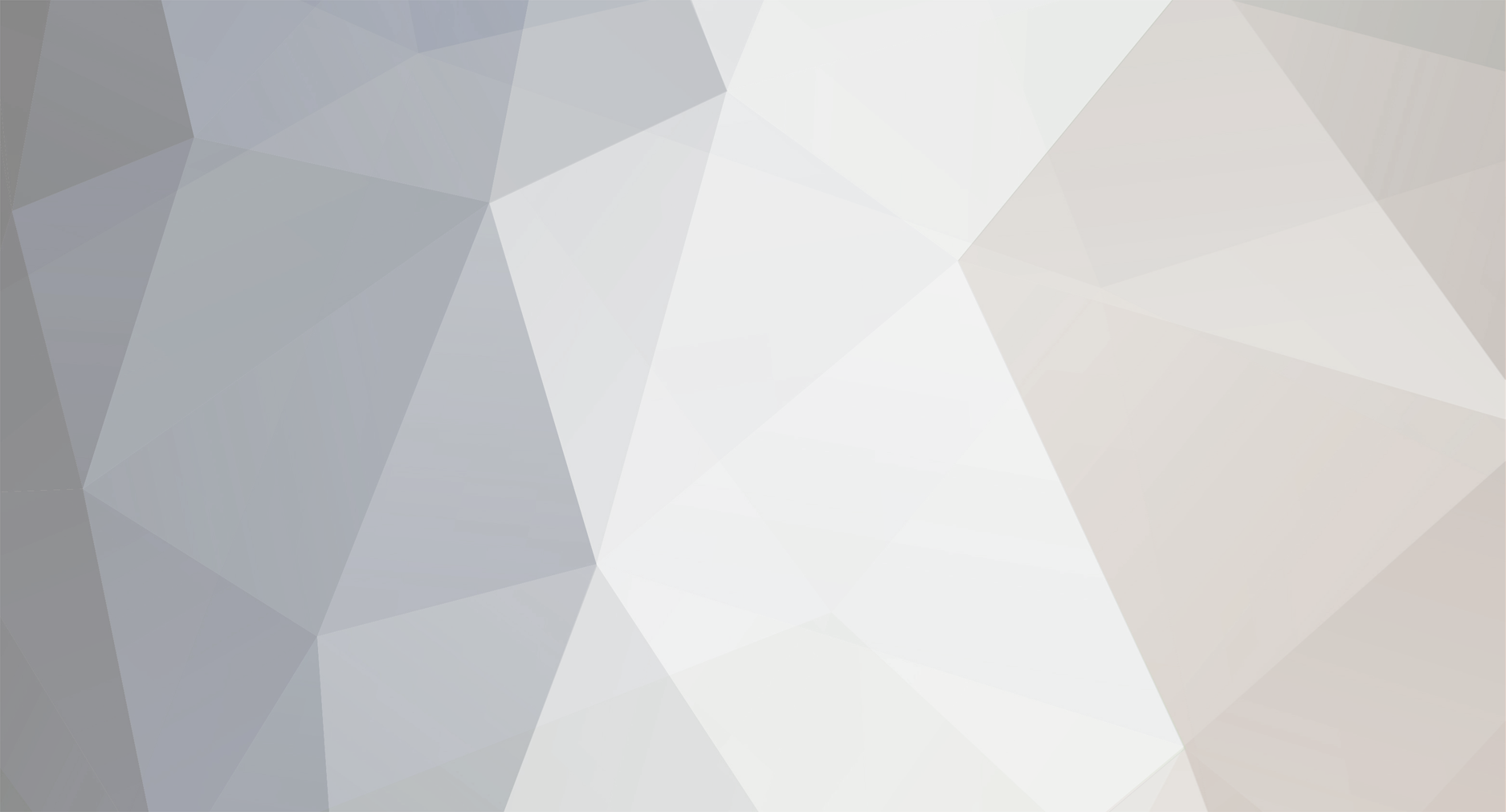
jonsjava
-
Posts
1,579 -
Joined
-
Last visited
Posts posted by jonsjava
-
-
I found one mention on the web about this issue. try this:
class UtilityController extends Zend_Controller_Action
instead of
class UtilityController extends CustomControllerAction
-
better solution:
create a field on the user table that is to track if they are banned:
ALTER TABLE ADD `banned`INT(1) NOT NULL DEFAULT VALUE='0';
if you ban someone, you just have the script change thier banned value to 1, and add a check to the login that says
.... AND `banned` != 1;
-
Compare IPs
Won't work on anybody but Static IP addresses.
-
I can't remeber the wordpress code for this, so I'll just guess it(you should be able to find it from this):
where it says:
<div class="Title"><h1><a href="{url}">{site_name}</a></h1></div>
change it to
<div class="Title"><img src="http://url.to.image.com/image.jpg"></div>
-
He would get an error if there was a SQL error, hence the or die statement.
I'm getting tired. I've been helping where I can on these forums since this morning, and am now starting to make mistakes, and miss stuff. maybe I should stop for the night. I've been coding at work as well....
-
The code looks good, so my guess is the SQL connection, or the table name.
-
I know you installed pear, but did you install the PEAR module:
[root@localhost:~]pear install (module name)
-
um...that's what I said.
-
try this slightly modified version of my last attempt:
<body> <?php $Conn=mysql_connect("localhost","fierm","13183"); if(!$Conn){ echo "failed"; } else{ mysql_select_db("fierm"); $User=$_POST["user"]; $Pass=$_POST["pass"]; $Conf=$_POST["confirm"]; if($Pass == $Conf){ $result=mysql_query("SELECT * FROM user WHERE usr_name='$User' AND `password`='{$Pass}' LIMIT 1;") or die("It failed on the first query :".mysql_error()); $cou=mysql_num_rows($result); if($cou == 1) { echo "user already exists"; } else{ $result = mysql_query("SELECT * FROM user WHERE usr_name='{$User}' LIMIT 1;") or die("It failed on the second query :".mysql_error()); if (mysql_num_rows($result) != 1){ mysql_query("Insert into user (usr_name,pwd) Values ('$User','$Pass')"); echo "New User Was Added"; } } } else{ echo "passwords do not match"; } } ?> </body> <body> <?php $Conn=mysql_connect("localhost","fierm","13183"); if(!$Conn){ echo "failed"; } else{ mysql_select_db("fierm"); $User=$_POST["user"]; $Pass=$_POST["pass"]; $Conf=$_POST["confirm"]; if($Pass == $Conf){ $result=mysql_query("SELECT * FROM user WHERE usr_name='$User' AND `password`='{$Pass}' LIMIT 1;") or die("It failed on the third query :".mysql_error()); $cou=mysql_num_rows($result); if($cou == 1) { echo "user already exists"; } else{ $result = mysql_query("SELECT * FROM user WHERE usr_name='{$User}' LIMIT 1;"); if (mysql_num_rows($result) != 1){ mysql_query("Insert into user (usr_name,pwd) Values ('$User','$Pass')"); echo "New User Was Added"; } } } else{ echo "passwords do not match"; } } ?> </body>
***EDITED to add error checking
-
um... have you *installed* the pear class?
-
I agree. I'm betting that your variables are not named "pass" and "confirm"
-
<?php $comment = "this is a test"; $clean = str_replace(" ", "" $comment); ?>
-
<body> <?php $Conn=mysql_connect("localhost","fierm","13183"); if(!$Conn){ echo "failed"; } else{ mysql_select_db("fierm"); $User=$_POST["user"]; $Pass=$_POST["pass"]; $Conf=$_POST["confirm"]; if($Pass == $Conf){ $result=mysql_query("SELECT * FROM user WHERE usr_name='$User' AND `password`='{$Pass}' LIMIT 1;"); $cou=mysql_num_rows($result); if($cou>0) { echo "user already exists"; } else{ $result = mysql_query("SELECT * FROM user WHERE usr_name='{$User}' LIMIT 1;"); if (mysql_num_rows($result) != 1){ mysql_query("Insert into user (usr_name,pwd) Values ('$User','$Pass')"); echo "New User Was Added"; } } } else{ echo "passwords do not match"; } } ?> </body>
-
yes, you are correct. at the head of all files, (including the login script) add:
<?php session_start(); ?>
and in the login script, add this as well:
<?php $_SESSION['is_valid'] = true; //change the session variable name to what you want, just remember it for all files $_SESSION['username'] = $row['user_name']; $_SESSION['user_level'] = $row['userlevel']; ?>
and for all the files that need to know user level, or username, etc...
<?php if ($_SESSION['is_valid'] == true){ if ($_SESSION['user_level'] == 0){ //do something for general users } if ($_SESSION['user_level'] == 1){ //do something for admins } } ?>
for more info, just ask, and we shall answer
-
What error are you receiving?
-
it asks if you want to repost the data, because it generated the previous page with a _POST
to fix this, do something like this ("proxy page")
for the search field:
//search page <form method="POST" action="page1.php"> //rest of stuff here
for page1
<?php $go = array(); $go .= $_POST['variable1']; $go .= $_POST['variable2']; //keep on this style until you have it all $search_url = "search.php?".$go['0']; $count = 1; foreach($go as $value){ if ($value != 0){ $search_url .= "&var".$count."=".$value; $count++; } } header("location:".$search_url); ?>
and the final page, the search page:
<?php //Search page: do your search by parsing through the $_GET vars $var1 = addslashes($_GET['var1']); $var2 = addslashes($_GET['var2']); $var3 = addslashes($_GET['var3']); //and so on. //then add the $vars into your query ?>
**EDITED to add some security into the get variables
-
could you let us see the code, so we can see how it's giving you the error? We can't do much unless we know how it's doing the search and redirect.
-
if you want/need any code checked via Zend 5.5.1, post it here, and I'll run it, and give you the errors it spits out (if you wanna fix stuff yourself, but don't have an IDE to do it in). I have an official license for the application, so I can freely offer this and not worry about someone getting me for having a cracked copy
-
I agree with BlueSky. We need the code, and then we could help you, or even flesh out a decent fix for you in a matter of minutes (well...quickly, at any rate)
-
I think this will do what you need *didn't error check it too thoroughly, though*:
<?php include '../includes/db_connect.php'; include '../includes/config_table.inc.php'; $user_name = $_POST["user_name"]; $user_password = $_POST["user_password"]; $verify_username = strlen($user_name); $verify_pass = strlen($user_password); if ($verify_pass > 0 && $verify_username > 0) { $salt = substr($user_password, 0, 2); $userPswd = crypt($user_password, $salt); $sql = "SELECT * FROM `$user` WHERE user_name='$user_name' AND user_password='$userPswd' LIMIT 1;"; $result = mysql_query($sql); if (mysql_num_rows($result) == 1){ $row = mysql_fetch_assoc($result); $user_level = $row['userlevel']; if ($user_level == 1) { echo "Logged In Sucessfully. Please wait while you are redirected"; echo "<meta http-equiv='refresh' content='2; url=setadmincookie.php?u=$username&p=$user_password'>"; } elseif ($user_level == 2){ echo "Logged In Sucessfully. Please wait while you are redirected"; echo "<meta http-equiv='refresh' content='2; url=setcookie.php?u=$username&p=$user_password'>"; } } else{ echo 'Login failed. Username and Password did not match database entries.'; } } else { echo "Form was not completed. Please go back and make sure that the form was fully completed."; } mysql_close(); ?>
**EDIT**
Forgot to do a couple things. give me a minute to finish this up.
**EDIT2**
Fixed forgotten things. should work now.
-
Not exactly what you were expecting, but why add overhead if you don't gotta?
<?php require_once 'library/db.php'; if (!($conn = mysql_connect('localhost', 'root', ''))) { showError(); } if (!(mysql_select_db('itsupport', $conn))) { showError(); } if (!($result = mysql_query('select * from itsupport', $conn))) { showError(); } if(!isset($cmd)) print "<tr> <td>User_ID</td> <td>Problem</td> <td>Problem_ID</td> <td>User_email</td> <td>Office_Number</td> <td>Phone_Number</td> <td>User_Name</td> <td> </td> <td> </td> <tr>"; while ($row =mysql_fetch_array($result)) { echo "<tr>"; echo "<td>" . $row[user_ID] . "</td> <td>" . $row[Problem] . " </td> <td>" . $row[Problem_ID] . "</td> <td>" . $row[user_email] . "</td> <td>" . $row[Office_Number] . " </td> <td>" . $row[Phone_Number] . " </td> <td>" . $row[user_Name] . " </td>"; echo "<td><a href=\"selectedit.php?id=$row[user_ID]\"><img border=\"0\" src=\"images/edit.gif\"></a>"; echo "<td><a href=\"delete.php?id=$row[user_ID]\"><img border=\"0\" src=\"images/delete.gif\"></a>"; echo "</tr>"; } mysql_close($conn);
-
you need to run a Join statement to do this right. I hate them, so I am going to let someone who loves MySQL answer this in full for you.
**looks like someone already did.
-
solution:
str_replace("\n", "", $data);
-
<html> <head> <title>Database is Updating...</title> </head> <?php $id = $_GET["id"]; $facpr = $_GET["facpr"]; ?> <body bgcolor="#FFFFFF"> <?php $username = "root"; $password = "*****"; //gotta love the fact I left the real password in there. glad it's just a test box. gotta change it now. $hostname = "localhost"; $dbhandle = mysql_connect($hostname, $username, $password); echo "Connected to Server<br>"; mysql_select_db("testing",$dbhandle); echo "Connected to Salt Lake City Database<p>"; mysql_query("UPDATE training SET firstaidcpr = '$facpr' WHERE id='$id'"); echo "Database has been updated. You will be returned to the training page momentarily. <meta http-equiv='refresh' content='3;URL=training.php' >" ?> </body> </html>
works great for me. I had it updating a test user just fine for me.
Getting PHP to print a page
in PHP Coding Help
Posted
I agree. I recommend you download ApacheFriends Xampp. It's free, and easy to install