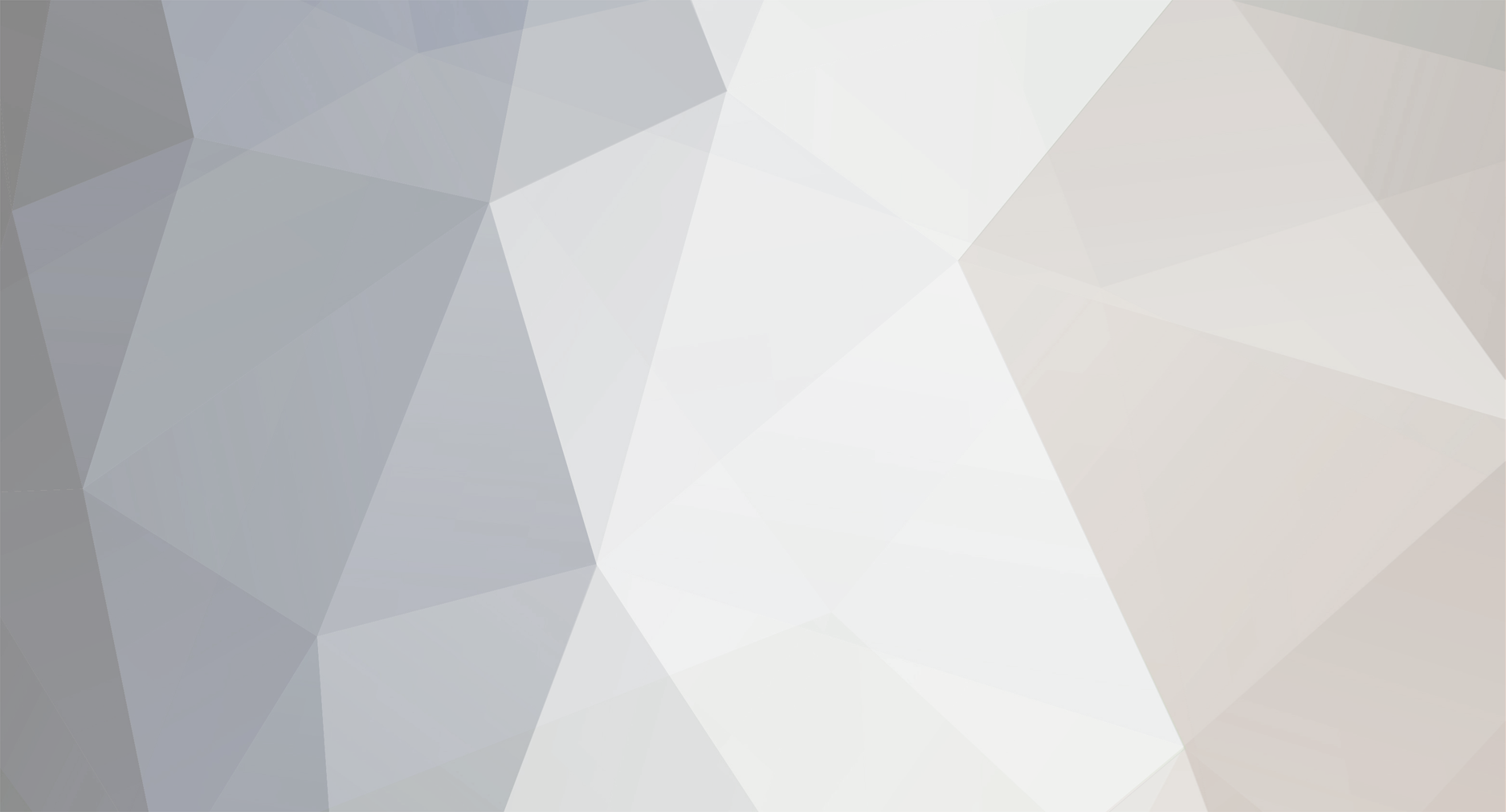
davey_b_
Members-
Posts
34 -
Joined
-
Last visited
Never
Everything posted by davey_b_
-
any help???
-
here's the JS (I think) var Behaviour = { list : new Array, register : function(sheet){ Behaviour.list.push(sheet); }, start : function(){ Behaviour.addLoadEvent(function(){ Behaviour.apply(); }); }, apply : function(){ for (h=0;sheet=Behaviour.list[h];h++){ for (selector in sheet){ list = document.getElementsBySelector(selector); if (!list){ continue; } for (i=0;element=list[i];i++){ sheet[selector](element); } } } }, addLoadEvent : function(func){ var oldonload = window.onload; if (typeof window.onload != 'function') { window.onload = func; } else { window.onload = function() { oldonload(); func(); } } } } Behaviour.start(); /* document.getElementsBySelector(selector) - returns an array of element objects from the current document matching the CSS selector. Selectors can contain element names, class names and ids and can be nested. For example: elements = document.getElementsBySelect('div#main p a.external') Will return an array of all 'a' elements with 'external' in their class attribute that are contained inside 'p' elements that are contained inside the 'div' element which has id="main" -- Works in Phoenix 0.5, Mozilla 1.3, Opera 7, Internet Explorer 6, Internet Explorer 5 on Windows -- Opera 7 fails */ function getAllChildren(e) { // Returns all children of element. Workaround required for IE5/Windows. Ugh. return e.all ? e.all : e.getElementsByTagName('*'); } document.getElementsBySelector = function(selector) { // Attempt to fail gracefully in lesser browsers if (!document.getElementsByTagName) { return new Array(); } // Split selector in to tokens var tokens = selector.split(' '); var currentContext = new Array(document); for (var i = 0; i < tokens.length; i++) { token = tokens[i].replace(/^\s+/,'').replace(/\s+$/,'');; if (token.indexOf('#') > -1) { // Token is an ID selector var bits = token.split('#'); var tagName = bits[0]; var id = bits[1]; var element = document.getElementById(id); if (tagName && element.nodeName.toLowerCase() != tagName) { // tag with that ID not found, return false return new Array(); } // Set currentContext to contain just this element currentContext = new Array(element); continue; // Skip to next token } if (token.indexOf('.') > -1) { // Token contains a class selector var bits = token.split('.'); var tagName = bits[0]; var className = bits[1]; if (!tagName) { tagName = '*'; } // Get elements matching tag, filter them for class selector var found = new Array; var foundCount = 0; for (var h = 0; h < currentContext.length; h++) { var elements; if (tagName == '*') { elements = getAllChildren(currentContext[h]); } else { elements = currentContext[h].getElementsByTagName(tagName); } for (var j = 0; j < elements.length; j++) { found[foundCount++] = elements[j]; } } currentContext = new Array; var currentContextIndex = 0; for (var k = 0; k < found.length; k++) { if (found[k].className && found[k].className.match(new RegExp('\\b'+className+'\\b'))) { currentContext[currentContextIndex++] = found[k]; } } continue; // Skip to next token } // Code to deal with attribute selectors if (token.match(/^(\w*)\[(\w+)([=~\|\^\$\*]?)=?"?([^\]"]*)"?\]$/)) { var tagName = RegExp.$1; var attrName = RegExp.$2; var attrOperator = RegExp.$3; var attrValue = RegExp.$4; if (!tagName) { tagName = '*'; } // Grab all of the tagName elements within current context var found = new Array; var foundCount = 0; for (var h = 0; h < currentContext.length; h++) { var elements; if (tagName == '*') { elements = getAllChildren(currentContext[h]); } else { elements = currentContext[h].getElementsByTagName(tagName); } for (var j = 0; j < elements.length; j++) { found[foundCount++] = elements[j]; } } currentContext = new Array; var currentContextIndex = 0; var checkFunction; // This function will be used to filter the elements switch (attrOperator) { case '=': // Equality checkFunction = function(e) { return (e.getAttribute(attrName) == attrValue); }; break; case '~': // Match one of space seperated words checkFunction = function(e) { return (e.getAttribute(attrName).match(new RegExp('\\b'+attrValue+'\\b'))); }; break; case '|': // Match start with value followed by optional hyphen checkFunction = function(e) { return (e.getAttribute(attrName).match(new RegExp('^'+attrValue+'-?'))); }; break; case '^': // Match starts with value checkFunction = function(e) { return (e.getAttribute(attrName).indexOf(attrValue) == 0); }; break; case '$': // Match ends with value - fails with "Warning" in Opera 7 checkFunction = function(e) { return (e.getAttribute(attrName).lastIndexOf(attrValue) == e.getAttribute(attrName).length - attrValue.length); }; break; case '*': // Match ends with value checkFunction = function(e) { return (e.getAttribute(attrName).indexOf(attrValue) > -1); }; break; default : // Just test for existence of attribute checkFunction = function(e) { return e.getAttribute(attrName); }; } currentContext = new Array; var currentContextIndex = 0; for (var k = 0; k < found.length; k++) { if (checkFunction(found[k])) { currentContext[currentContextIndex++] = found[k]; } } // alert('Attribute Selector: '+tagName+' '+attrName+' '+attrOperator+' '+attrValue); continue; // Skip to next token } if (!currentContext[0]){ return; } // If we get here, token is JUST an element (not a class or ID selector) tagName = token; var found = new Array; var foundCount = 0; for (var h = 0; h < currentContext.length; h++) { var elements = currentContext[h].getElementsByTagName(tagName); for (var j = 0; j < elements.length; j++) { found[foundCount++] = elements[j]; } } currentContext = found; } return currentContext; } /* That revolting regular expression explained /^(\w+)\[(\w+)([=~\|\^\$\*]?)=?"?([^\]"]*)"?\]$/ \---/ \---/\-------------/ \-------/ | | | | | | | The value | | ~,|,^,$,* or = | Attribute Tag */
-
Hey... Does any one know how I can change this list in to submit buttons or links. Its for changing currency from pounds, euros, dollars etc. Appreciate any help on this. <form name="frmCurrencyType" method="post"> <table cellpadding="0" cellspacing="0" border="0" width="100%"> <tr> <td> <select name="currency_type" onChange="Javascript:document.frmCurrencyType.submit();"> <option value="">--Select--</option> <?php $select_currency = "SELECT ncurrency_id,vcurrency_name FROM ".$tableprefix."currency_master WHERE vactive = 'Y'"; $result_currency = mysql_query($select_currency) or die(mysql_error()); while($currency_row = mysql_fetch_array($result_currency)) { if($_SESSION['SESS_currency_type'] == $currency_row['ncurrency_id']) { $currency_selected = "selected"; } else { $currency_selected = ""; } ?> <option value="<?php echo $currency_row['ncurrency_id']?>" <?php echo $currency_selected?>><?php echo $currency_row['vcurrency_name']?></option> <?php } ?> </select> </td> </tr> </table> </form>
-
solved it.... if(!(isset($pagenum))) { $pagenum = 1; } $result=mysql_query($sql) or die(mysql_error()); $totalrows = mysql_num_rows($result); $limit=8; $page = $_GET['page']; // <-- added the line you said!!! if(empty($page)) { $page = 1; } $limitvalue = ($page - 1) * $limit; $query_string = " LIMIT $limitvalue, $limit"; $sql = $sql.$query_string;
-
could it be a problem with the MySQL query ? ?
-
ok... so I've changed to following.. still not working. <?php /* if(!(isset($pagenum))) { $pagenum = 1; } */ $result=mysql_query($sql) or die(mysql_error()); $totalrows = mysql_num_rows($result); $limit=8; if(empty($page)) { $page = 1; } if(isset($_GET['page'])) { $pagenum = $_GET['page']; } else{ $pagenum = 1; //if $_GET['page'] isnt set then it is page one } $limitvalue = ($page - 1) * $limit; $query_string = " LIMIT $limitvalue, $limit";
-
So where should that go ? ?
-
OK, here is the full code... the site being effected is http://www.trueurbangift.co.uk/mmviii/index.php and it effects the whole site where the products are being listed. <?php if($_GET['featured'] == 'Y') { $sql = "SELECT product_id,product_name,product_image_small,product_stock,product_short_desc,product_price,discount FROM ".$tableprefix."products WHERE featured='Y' AND deleted != 'Y' ORDER BY date_added DESC"; $featured_image = "featured_items1.gif"; $newitem_image = "new_items.gif"; } else { $sql = "SELECT product_id,product_name,product_image_small,product_stock,product_short_desc,product_price,discount FROM ".$tableprefix."products WHERE deleted != 'Y' ORDER BY date_added DESC"; $featured_image = "featured_items.gif"; $newitem_image = "new_items_h.gif"; } ?> <div align="left" id="items"> <!--items display area start --> <div align="left" id="items_top_area"> <?php if(!isset($_GET['featured']) || $_GET['featured'] == 'N') { ?> <img src="<?php echo $newitem_image?>" alt="" width="92" height="33" /> <a href="index.php?featured=Y" onMouseOut="MM_swapImgRestore()" onMouseOver="MM_swapImage('Image30','','featured_items1.gif',1)"> <img src="<?php echo $featured_image?>" alt="Featured Items" name="Image30" width="127" height="33" border="0" id="Image30" /> </a> <?php } else { ?> <a href="index.php?featured=N" onMouseOut="MM_swapImgRestore()" onMouseOver="MM_swapImage('ImageNew','','new_items1.gif',1)"> <img src="<?php echo $newitem_image?>" border="0" alt="" name="ImageNew" width="92" height="33" /> </a> <img src="<?php echo $featured_image?>" alt="Featured Items" name="Image30" width="127" height="33" border="0" id="Image30" /> <?php } ?> </div> <div align="center" id="items_display_area"> <?php $prodimagedir = "products/"; if(!(isset($pagenum))) { $pagenum = 1; } $result=mysql_query($sql) or die(mysql_error()); $totalrows = mysql_num_rows($result); $limit=8; if(empty($page)) { $page = 1; } $limitvalue = ($page - 1) * $limit; $query_string = " LIMIT $limitvalue, $limit"; $sql = $sql.$query_string; $result=mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($result) > 0 ) { while($row = mysql_fetch_array($result)) { if(intval($row["product_stock"]) > 0) { $productinstock = true; } else { $productinstock = false; } if($row["product_image_small"] == "") { $imageurl = "<img class='item_thumb' src='".$prodimagedir . "clear1.gif' width='50' height='50' border='0' >"; } else { if(!function_exists('gd_info')) { $imageurl = "<img class='item_thumb' src='".$prodimagedir.urlencode($row["product_image_small"])."' border='0' width='50' height='50' class='item_thumb'>"; } else { $imageurl = "<img class='item_thumb' src='getimagethumbnail.php?imagename=".urlencode($row["product_image_small"])."' width='50' height='50' border='0' class='item_thumb'>"; } } ?> <?php $currency_attributes = displayCurrencyType(); //********** Display discount and set up price formatting ************** $price = number_format(($currency_attributes[1] *$row["product_price"]),2 ,".","" ); $discountstr=""; if($row["discount"] !="0"){ $price = number_format(($currency_attributes[1] *$row["product_price"] - ($price*$row["discount"])/100),2 ,".","" ); $discountstr=" <font color=red size=1>(".$row["discount"]."% off)</font>"; } ?> <!--Display the product details--> <div align="center" class="item_block_featured"> <div align="center" class="item_block_links"> <table width="412" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="65" valign="top"><table width="50" height="50" border="0" cellspacing="0" cellpadding="0"> <tr> <td align="center"><a href="productdetails.php?productid=<?php echo $row["product_id"]?>"><?php echo $imageurl?></a></td> </tr> </table> <a href="productdetails.php?productid=<?php echo $row["product_id"]?>"></a></td> <td width="10" align="left" valign="top"><a href="productdetails.php?productid=<?php echo $row["product_id"]?>"><img src="images/clear1.gif" alt="1" width="10" border="0" /> </a> </td> <td width="477" align="left" valign="top"><h4 class="productHead"><a href="productdetails.php?productid=<?php echo $row["product_id"]?>"><?php if(strlen($row["product_name"])>40) $prd_name = substr($row["product_name"],0,40)."..."; else $prd_name = $row["product_name"]; echo $prd_name.$discountstr; ?> </a></h4> <?php if(strlen($row["product_short_desc"])>75) $prd_short_desc = substr($row["product_short_desc"],0,75)."..."; else $prd_short_desc = $row["product_short_desc"]; echo (htmlentities($prd_short_desc)); //echo $prd_short_desc; ?></td> <td width="85" align="center" valign="middle"><h4 class="productHead"> <?php if ($row["product_price"] =="0") { echo ""; } else { $pricestr= "" .$currency_attributes[0].$price.""; echo $pricestr; } ?> </h4> <?php if ($row["product_stock"] =="N") { ?> <img src="classified_btn.gif" alt="Classified" border="0" /> <?php } else { if($productinstock) { ?> <div align="center" class="item_block_links"> <a href="homepage_addtocart.php?productid=<?php echo $row["product_id"]?>"><img src="view_cart_btn.gif" alt="View Cart" border="0" /></a> <?php } } ?> <a href="productdetails.php?productid=<?php echo $row["product_id"]?>"><img src="more_btn.gif" alt="More" border="0" /></a><a href="productdetails.php?productid=<?php echo $row["product_id"]?>"></a></td> </tr> </table> </div> </div> <!--Display the product details--> <?php } } if($page > 1) { $pageprev = $page-1; $previous_page = "<a href=".$_SERVER['PHP_SELF']."?page=$pageprev alt='Previous' border='0'><img src='previous_btn.gif' alt='Previous' border='0' /></a>"; } $numofpages = ceil($totalrows / $limit); if($page < $numofpages) { $pagenext = ($page + 1); $next = "<a href=".$_SERVER['PHP_SELF']."?page=$pagenext alt='Next' border='0'><img src='next_btn.gif' alt='Next' border='0'/></a> "; } ?> <div align="left" class="float_clear1"></div> <div align="left" class="more_links"> <div align="left" class="previous"> <?php echo $previous_page?> </div> <div align="left" class="next"> <?php echo $next?> </div> </div> </div> <!--items display area end --> </div>
-
Hi... I'm pretty new to php so i could be missing the obvious. My "next page" button reloads the first page even though it registers as "index.php?page=2" Here's my code, I've removed a chunk that displays the list so it doesn't take too much space Appreciate any help on this... Dave <?php if($_GET['featured'] == 'Y') { $sql = "SELECT product_id,product_name,product_image_small,product_stock,product_short_desc,product_price,discount FROM ".$tableprefix."products WHERE featured='Y' AND deleted != 'Y' ORDER BY date_added DESC"; } else { $sql = "SELECT product_id,product_name,product_image_small,product_stock,product_short_desc,product_price,discount FROM ".$tableprefix."products WHERE deleted != 'Y' ORDER BY date_added DESC"; }?> <?php if(!(isset($pagenum))) { $pagenum = 1; } $result=mysql_query($sql) or die(mysql_error()); $totalrows = mysql_num_rows($result); $limit=8; if(empty($page)) { $page = 1; } $limitvalue = ($page - 1) * $limit; $query_string = " LIMIT $limitvalue, $limit"; $sql = $sql.$query_string; $result=mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($result) > 0 ) { while($row = mysql_fetch_array($result)) { if(intval($row["product_stock"]) > 0) { $productinstock = true; } else { $productinstock = false; } ?> <!-- BODY CODE REMOVE TO STREAMLINE --> <?php } } if($page > 1) { $pageprev = $page-1; $previous_page = "<a href=".$_SERVER['PHP_SELF']."?page=$pageprev alt='Previous' border='0'><img src='previous_btn.gif' alt='Previous' border='0' /></a>"; } $numofpages = ceil($totalrows / $limit); if($page < $numofpages) { $pagenext = ($page + 1); $next = "<a href=".$_SERVER['PHP_SELF']."?page=$pagenext alt='Next' border='0'><img src='next_btn.gif' alt='Next' border='0'/></a> "; } ?> <div align="left" class="float_clear1"></div> <div align="left" class="more_links"> <div align="left" class="previous"> <?php echo $previous_page?> </div> <div align="left" class="next"> <?php echo $next?> </div></div>