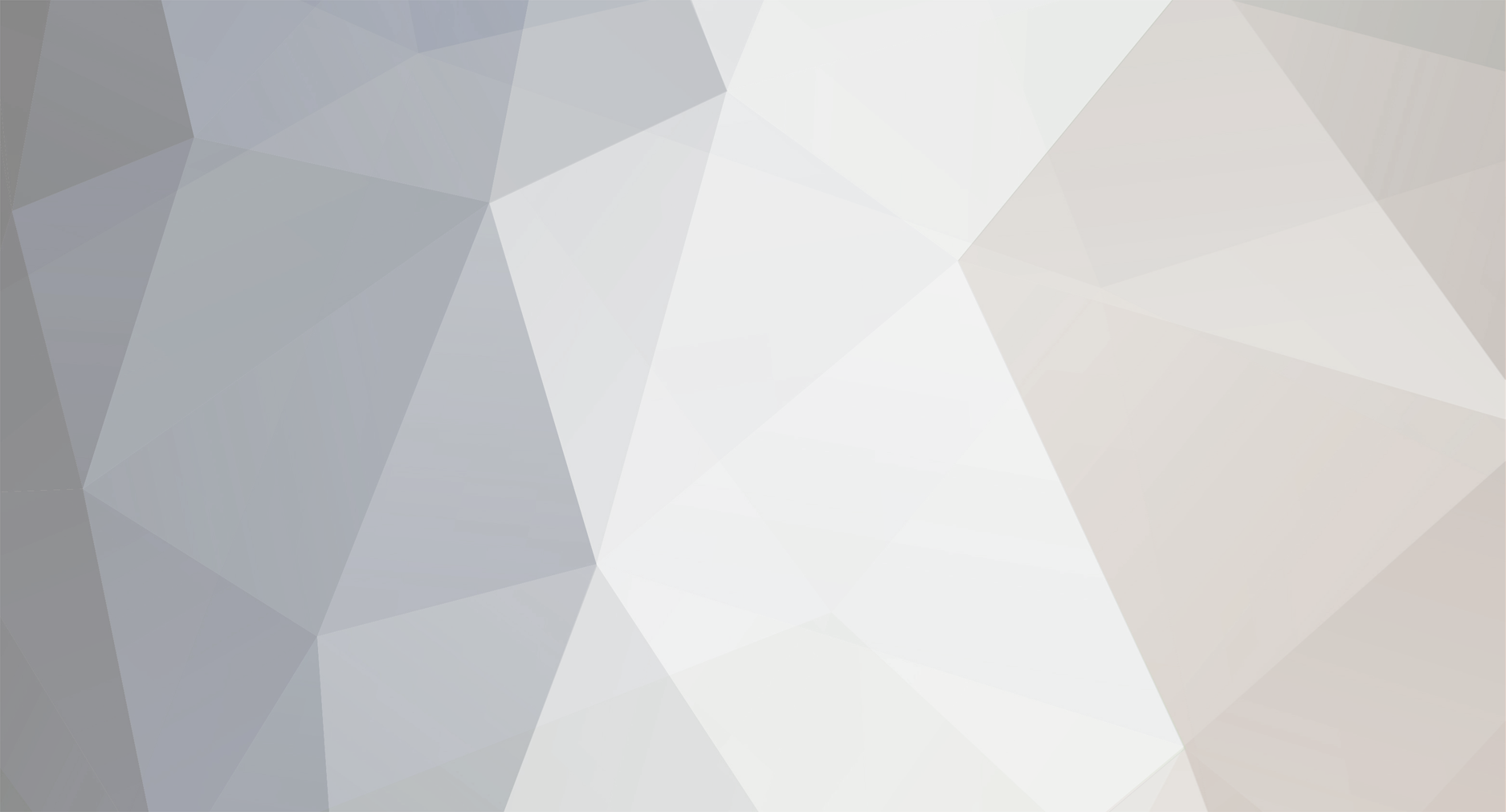
phorman
Members-
Posts
49 -
Joined
-
Last visited
Never
Everything posted by phorman
-
I am not sure you can do this, this is a SERIOUS SECURITY BREACH if you can, as many well known payment gateways claim that if you iFrame to them, then customers information they enter is secure. This instills confidence to the customer to continue with the order. You may not be trying to do this, but its for this reason that I think the browsers will not let you submit form to two places. As for a tracking pixel, or pixie, its a piece of code given to you by the tracker. Some tracking pixels are javascript code, while others is a image link. You will need to contact the person who hired you for the specific tracking pixel. An example of a pixel would be: <img src="www.cj.com?id=feASEsdgsd">
-
Retrieve files from outside the 'public_html' directory
phorman replied to cs.punk's topic in PHP Coding Help
The headers method is really the only correct way to do what you are wanting to do. Why don't you like it. The problem is that you don't want just anybody seeing the photos, you want to control it by who has access to see what. You could do it the way you described, but unlinking a file deletes it. Why copy the file only so you can display the file and then delete the file. PHP is a scripting language that can serve up text, html, css, images of any type, .pdf files... the list goes on. What determines what the browser sees is the MIME type in the header. Set the header MIME type to .jpeg, or .gif, or .png, whichever the image is you want to display, then use the code you have written but take out the copy and the unlink statemets. Now just echo out the image. You would now have a dynamic link to your files that you can store in your database whenever you need to show a picture. So when your program builds the interface, you end up with: < img src="image_viewer.php?id=aWRTDGhdfhaw"> which when looked at on the monitor displays your image, neither copied or deleted. -
PHP has built in functions to help you in that regard, specifically for that task. include, require, include_once, and require_once. The difference are that require will not allow your program to continue, unless the file actually exists. Include will try to include the file, but if it does not exist, it will skip it, and continue. The _once option means that it will include the file only once no matter how many times you call it from other libraries. So... You could say.. require("header.php"); require("left_side.php"); require("footer.php"); Also, the files you are including could end in .html , they do not have to be .php files, unless you have scripts that need to be processed inside them.
-
Ok, everything stated is almost correct, except..... NEVER use curly brackets in echo.... Curly brackets, while they will work in echo, are meant for print statements only. There is an overhead to processing a string with brackets, and on a small site it is minimal, but learn to code correctly and when working on bigger sites, you will see the payoff. " echo " is a special function if you will that does not require the output to be in quotes in the first place. If you want to append something with an echo statement, use a comma. The following are all valid echo statements. echo $start; echo $start["now"]; echo "Start Time: ", $start["now"], " End Time: ", $end["now"]; echo $start->now();
-
Don't forget to always level the playing field by setting all margins and padding to 0 as some browsers have different default margins and padding for their elements.
-
Hard to describe, I am looking for something like 'return' but for IF
phorman replied to cs.punk's topic in PHP Coding Help
Goto? Yuck!!! If you must use a goto, set what you want to do in a function, and then call the function.. This helps document your code as you go too, and is one step closer to OOP. function doSomething() { // THIS IS WHERE I WANT IT TO GO echo "Your username is too long"; echo "blah blah"; // form data } /*** MAIN PROGRAM BODY ****/ if ($sumbited) {//process form if ($error) { doSomething(); } } or you could do if ( $submitted && $error ) { doSomething(); } -
Help with Status Bar, using the Basic Start working Example
phorman replied to phorman's topic in Javascript Help
I had another thought, and that was that maybe this is a limitation of ajax, and it would be better to use comet instead. I have tried all kinds of combinations and no success yet. I will attempt to implement comet, and see if that helps matters. -
I can't figure out how to run multiple requests right.
phorman replied to HaLo2FrEeEk's topic in Javascript Help
can I see some of the modified php code, with the functions add_link_to_db, and build_select_statement, or equivalant? with the revised php code earlier, you could call ajax request to index.php in the main body onload, and again in the onsubmit for the form. When called the first time, no form elements would be retrieved, so it would just show the dropdown. When called fromt the form onsubmit, the form elements would be there, so it would update the database and then display your dropdown. Since all these were called from either onload or onsubmit, it would still have the ajax functionality you were wanting. -
I can't figure out how to run multiple requests right.
phorman replied to HaLo2FrEeEk's topic in Javascript Help
ok, I have now looked at your main code, and noticed that you have not named your submit button. I would do that. -
I can't figure out how to run multiple requests right.
phorman replied to HaLo2FrEeEk's topic in Javascript Help
hmm.. after seeing your code, i question whether AJAX needs to be used on anything except making sure the user has entered a valid url into the input box. Until they do, I would disable the insert button. Now to fix your problem, I would first determine whether the insert button was pressed ( IF ($_REQUEST["SUBMIT"]) Replace SUBMIT with whatever you named your INSERT button. <?php if ($_REQUEST["SUBMIT"]) { // put your current code to add to the database in here // I prefer to make functions out of alot of code lines, as it helps document what your trying to accomplish // and this helps after six months of not looking at the code to go in and fix with relative ease. // not to mention it prepares the code for possible OOP later. add_link_to_db(); } build_select_statement(); ?> So... the way this works is that it will first check to see if any input came from the form, and if so add the information to the database. Regardless of the whether input was sent, it will then rebuild the screen with the new dropdown. Adding the new URL to the list if there was one. -
As this appears to be a non-web game, am I to assume that your users will have some sort of executable file to run the game? If so, then you can use FTP to "hide" the backend of the game. Just ship the game with an (Apache/PHP) framework. If this is to be a web based game, and your users will not have to run an executable to play, ie they just visit a website, then you do not need a form, or even FTP. Just store the levels on the server, and call the files from their current location, depending on what level they are on. If you want users to submit their own level creations to your server, then use a form.
-
I can't figure out how to run multiple requests right.
phorman replied to HaLo2FrEeEk's topic in Javascript Help
ok, why not put the two functions in the same php code that gets executed. place them in the order you need them to fire off so that therecords will be written. As for your urls being displayed when the page is loaded, you can do this in one of two ways. Either use the onload of the body to fire off a javascript that calls an ajax function to populate the data, or have your page be a .php, and build the page with a while loop to populate the fields and then display the output. I prefer the latter, just in case your visitors have javascript turned off, they will still have a dropdown to choose from. -
The code is very insecure.. You must pass any $_REQUEST, $_POST, $_GET variables through mysql_escape_string() function. Are you looking for a way someone could circumvent your current security? google SQL Injection
-
break the directory apart with something like explode, and look for the numbered occurance your interrested in. If its the directory thats one away from root then: <?php $directory = dirname($_SERVER["REQUEST_URI"]); $dir_array = explode("/",$directory); $lookingfor = $dir_array[1]; ?>
-
[SOLVED] How to store a path in mysql db for this ftp upload script ?
phorman replied to redpedia's topic in PHP Coding Help
this is not the best script I have seen that will do the job, as you are doing any verifications, but to answer your question: add the following after the line : $fp = fopen($localfile, ‘r’); $path = getCWD; // returns the current directory you are in. $fullpath = $path . $localfile; then use $fullpath as the path to store in the database. Also, you need to check out: http://us2.php.net/manual/en/features.file-upload.php This is by the book the way you need to process uploaded files. You may even want to throw in a filesize check to see if they are exceeding some kind of quota. -
verify the page encoding your using, and also your database encoding if this is coming from a database.
-
Ok, I see what you are attempting to do, and if your game allows a place to call php code, you can use php to call FTP functions to send files to the server in the background, without a form having to be submitted. Contrary to the message title, this is not a basic question. To point you in the right direction, checkout www.php.net and specifically search for FTP to see what I mean.
-
the main thing I see is that you have strict error checking turned on. in your etc/php.ini file, change this behaviour.
-
Your variable enddate is not the array you think it is. It is an array, but it is string. Soo, when you ask for $enddate[0] you are infact getting the 2 from 2008, the index 0 of the string array. If you want the enddates stored in array so that you can call the $enddate[0] and get the desired full date, change the following: <?php $enddate=mysql_result($result,$i,"EndDate"); ?> to <?php $enddate[$i]=mysql_result($result,$i,"EndDate"); ?>
-
I have program that examines a specific directory, and under that directory is another directory of authors/Artists. Then inside the author/artist directory is all the songs, or lectures given by the individual or group. My program enters all the information into a Mysql Database (indexed) and allows for quick retrieval of requested content. My problem is that the inserting process can take some time, and therefore I want a way to be able to display the current status of the process. Here is what I have that I thought would work: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> <style type="text/css"> <!-- #status { height: 40px; width: 740px; background-image: url(template/images/bg_status.png); } #status #update { background-image: url(template/images/status.png); font-family: Arial, Helvetica, sans-serif; font-size: 24px; font-style: normal; font-weight: bold; color: #FFFFFF; height: 35px; padding-top: 5px; text-align: center; overflow: hidden; } --> </style> <script type="text/javascript" language="javascript" src="scripts/ajaxrequest.js"></script> <script type="text/javascript"> <!-- function updateProgress() { //MyAjaxRequest("none","batchmedia.php?action=process"); upd = document.getElementById("update"); do { MyAjaxRequest('status','batchmedia.php?action=show_status'); } while (upd.value != "100%"); } //--> </script> </head> <body> <div id="status" name="status"></div> <form> <input type="button" name="start" value="Start" onclick="updateProgress()" /> <div id="update" style="width:1%; "> 0% </div> </form> </body> </html> This is the output generated by the batchmedia.php?action=show_status call: <div id="update" style="width:100%; "> 100% </div> The percentages are passed using php code. This works, except I keep getting "Access Denied" or timeouts when this code runs. The errors usally take a few minutes to pop up, and then when I answer them, the solution works as expected. The error seems to come from : "An exception of type "Access denied was not handled"....... coming from: //located in ajaxrequest.js MyHttpRequest.open("get",url_encode(file +query_string),true); It should also be noted that this error is coming from Windows where no access is set on the file in the first place. After it works in my test environment, I will move it to my linux server environment and test again. Any help in this matter would be greatly appreciated. Additional Thoughts: May be its re-calling the script too soon, and some sort of pause should take place. I came to this after removing the loop and just running through it once. It worked without a problem. When I introduce the loop, is when it stops working correctly.
-
While you may be new to PHP, if you know anything about Visual Basic, javascript or other OOP (Object Oriented Programming), its the same as writing: this._Link or in Excel Macros: activesheet.select;
-
Why not use a simple SQL JOIN SELECT STATEMENT: SELECT * from table1 join table2 on table1.seller_number = table2.buyer_number Then, if this statement returns any rows, you have your answer as to if the two numbers match in the database, and can then determine with the rest of your code whether a buyer or seller was trying to login and give the appropriate error.
-
While I agree with Craygo, I do not presume to know why you are doing it this way, but to accomplish what you asked, it can be written: <?php while($row1 = mysql_fetch_assoc($result1)) { $sku="sku_[color=red]1[/color]"; $$sku = row1["sku"]; $quanitity = "quantity_[color=red]1[/color]"; $$quantity = row1["quantity"]; }; ?>
-
This is how you would accomplish the task. <?php for ($i=0;$i<10;$i++) { $seed = "seed$i"; $$seed =$array[0]; } ?> The part that makes this possible is that two "$" dollar signs together will form a variable name with the string contained in the second $ variable. I do however question the need for this in this example as any variable with numbers assigned after it should be stored in an array to make it easier to loop, sort, or move the variables around if necessary.
-
I see two syntax error with the code. 1. The only time you need to escape your array call is in a print statement. So, the fix is to remove the {} brackets around the if statement. 2. On line 65, when setting the variable to equal something, only use one = sign. Otherwise you are comparing instead of setting the value equal. The code should look like: <?php $rct = 1; 61 //get each row 62 while($dbRow = mysql_fetch_array($result, MYSQL_ASSOC)) 63 { 64 if ($dbRow['got_it'] == '1'){ 65 $bg_color = "#90EE90"; 66 }else{ 67 if ($rct % 2 == 0) 68 $bg_color = "#F5F5F5"; 69 else 70 $bg_color = "#F0F8FF"; 71 } ?>