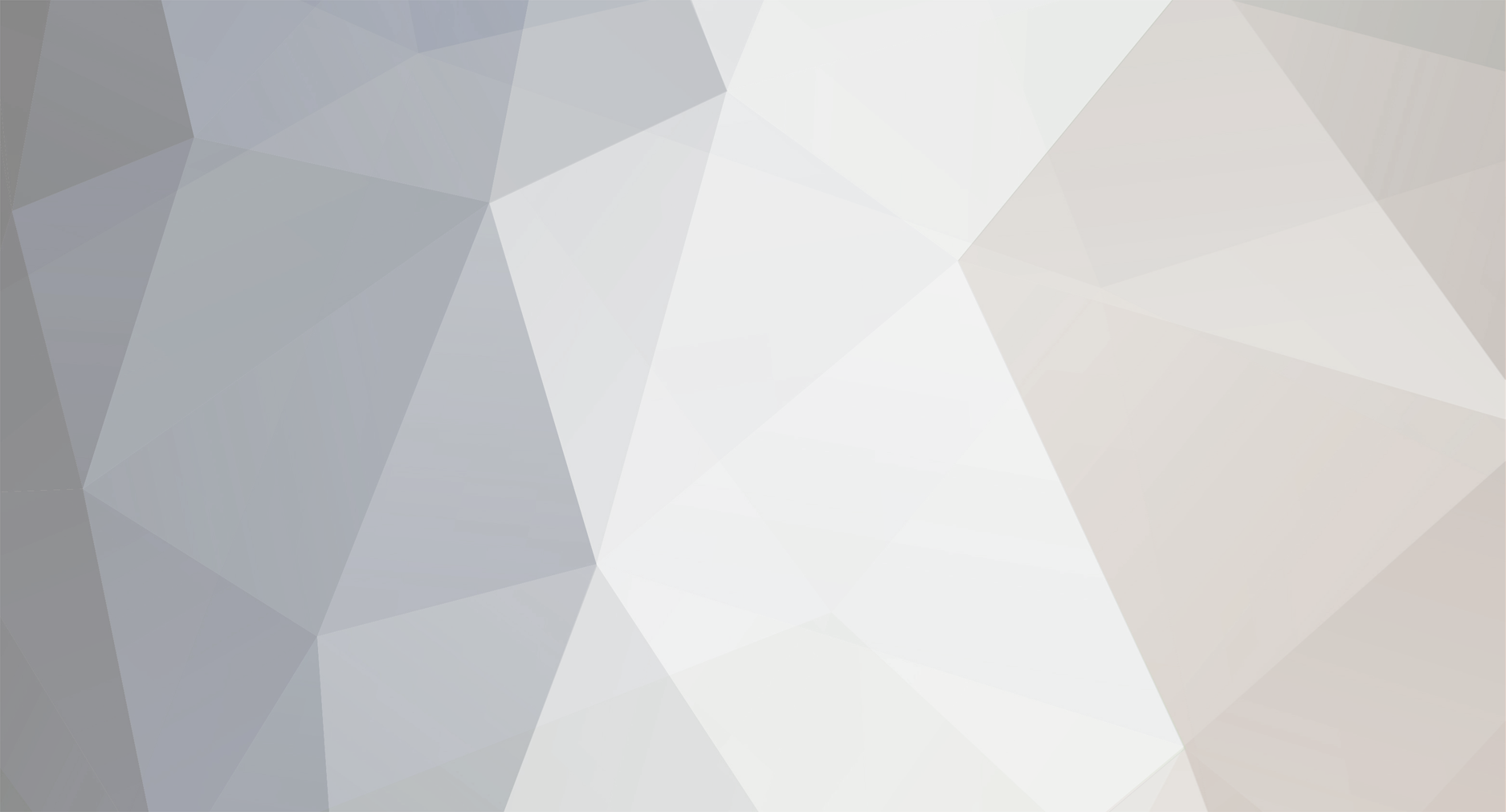
jrws
-
Posts
124 -
Joined
-
Last visited
Never
Posts posted by jrws
-
-
How about a tutorial on MYSQL sub-queries? You know explain with examples, that would help heaps
-James-
-
Thanks for that, what about numerical data? Something like is_numeric($code)?
-
Hey guys,
Currently I am using a protect function:
function clean($string) { if (get_magic_quotes_gpc()) { $string = stripslashes($string); } elseif (!get_magic_quotes_gpc()) { $string = addslashes(trim($string)); } $string = trim($string); //$string = escapeshellcmd($string);//Uncomment if the website uploading to allows this. $string = mysql_real_escape_string($string); $string = stripslashes(strip_tags(htmlspecialchars($string))); return $string; }
This is used to sanitize the user input, however I recently downloaded a program for firefox that checks against sql attacks, and I have seven possible attacks that can happen:
SQL Injection String Test Results username Submitted Form State: * password: * login: Login * remember_me: Remember Me Results: Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1 UNION ALL SELECT 1,2,3,4,5,6,name FROM sysObjects WHERE xtype = 'U' -- Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1 AND ASCII(LOWER(SUBSTRING((SELECT TOP 1 name FROM sysobjects WHERE xtype='U'), 1, 1))) > 116 Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: '; DESC users; -- Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1' AND 1=(SELECT COUNT(*) FROM tablenames); -- Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1'1 Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1 AND USER_NAME() = 'dbo' Error string found: 'You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use' Tested value: 1'1
So can I secure against these? Or are they nothing to worry about?
-
Thanks man, I would never have of figured it out otherwise, please correct me if I am wrong;
What I had done was make it have white space before it?
Thanks again
-James-
-
Hey guys,
I don't know how to fix this problem, I have tried trim and everything. Please have a look at the picture included to see what I mean by white space:
Its annoying because as I have said, I can't figure it out. Its not the database I have checked it, anyway here is the code to go along with it.
<?php //View News $title = 'News'; require_once ('libary/header.php'); if (isset($_GET['id']) && is_numeric($_GET['id']) && isset($_GET['edit']) &&is_numeric($_GET['id'])) { $id = $_GET['id']; $sql = "SELECT * FROM news WHERE id = '$id'"; $result = mysql_query($sql)or die(mysql_error()); $row = mysql_fetch_array($result); $u_id = $row['u_id']; if ($_SESSION['u_id'] == $u_id || $_SESSION['u_level'] == 6){ if(!isset($_POST['submit'])){ ?><form action="<? echo $PHP_SELF; ?>" method = "post"> Title:<input type="text" name = "title" value="<?=$row['title'];?>" size="32"><br> Author:<input type = "text" name = "author" disabled = "true" value = "<?=$row['author'];?>"><br> <textarea name = "data" id = "textbox"> <?=trim($row['data']);?> </textarea> <br> <input type = "submit" value = "Submit" name = "submit"> </form><? }else{ $error = array(); $title = clean($_POST['title']); $data = clean($_POST['data']); $update = "UPDATE news SET title = '$title', data = '$data' WHERE id='$id'"; $update_result = mysql_query($update)or die(mysql_error()); if($update_result){ echo 'Successfully updated article!<a href="'.$siteURL.'view_news.php?id='.$id.'"> View the updated post</a>'; }else{ echo '<div class="error">Did not update article successfully. Please try <a href="'.$siteURL.'view_news.php?id='.$id.'&edit='.$id.'">again</a></div>'; } } }else{ echo 'Not authorised to view this page!'; } } elseif (isset($_GET['id']) && is_numeric($_GET['id'])) { $id = clean($_GET['id']); $sql2 = mysql_query("SELECT * FROM `news` WHERE id='$id'") or die(mysql_error()); $row = mysql_fetch_array($sql2); $u_id = $row['u_id']; $title = $row['title']; $data = trim(nl2br($row['data'])); $author = $row['author']; echo '<h1>' . strtoupper($title) . '</h1>'; echo '<small>By :<a href="' . $siteURL . 'profile.php?id=' . $row['u_id'] . '">' . $author . '</a><br></small>'; echo '<p>' . $data . '</p>'; if ($_SESSION['u_id'] == $u_id || $_SESSION['u_level'] == 6) { echo '<br> <a href="?id=' . $id . '&edit=' . $id . '">Edit this post</a>'; } else { } } else { //Pagination $table = 'news'; $rowsPerPage = 3; $data1 = 'title'; $data2 = 'data'; $link = 'post'; include_once ('Pagnation.php'); pagnation($table, $rowsPerPage, $data1, $data2, $link); /*Pagination*/ } require_once ('libary/footer.php'); ?>
-
I dunno what it would be counted as, because I am displaying the information via PHP, however I am using a database. I asume the latter, and I now realise I have probably posted in the wrong place.
-James-
-
Hi there,
Once again I have a question, how or what command would one use to find out how many times something reoccurs in a table? For example if there is a multiple of uid, how do I find out how many a specific uid occurs? Here is an example
id uid
1 2
2 2
3 4
4 1
5 6
6 2
And I want to count how many times uid 2 appears, which would be 3 times. Would I use something like SUM or COUNT?
-
Thanks,
However I am not exactly re-inventing the wheel, the whole point of the exercise is to learn. I am looking at the video now. Thanks again.
-
Hey there all,
I know that you will suggest that I just use an already made forum, however I want to undertake the creation of a forum to help me learn to relate MYSQL stuff. I have already successfully made a news system and a user system, the next project is a forum. However, I am at a loss as to where to start, I have a couple of ideas but I would like to know more, if possible. I have been searching for tutorials, however I am unable to find what I want. So far this is what I have.
Table : Catergory id primary key, auto increment, int; name varchar 100; Table: Forum id primary key, auto increment, int; parent int; name varchar 100; description blob 500;
And that is all I have, however I don't know what other information I should have, I am thinking to have another table-Topic- and for that a reply table.
Any help is appreciated.
Thanks,
-James-
-
Thanks mate, I didn't realize.
-
Here's the mysql query:
INSERT INTO forum_sub_cats(name,desc) VALUES('Random','Random Description')
I have checked that everything exists, and those values are pseudo values mind you, however it spits this error:
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'desc) VALUES('Random','Random Description')' at line 1
So whats wrong with it?
It will accept this one:
INSERT INTO forum_sub_cats(name,admin)VALUES('$name','$admin')
And for the life of me I can't find an answer..
-
Hey guys,
Not so much a problem as just a question. At the moment I am using a remember me function that includes grabbing the password. However I am concerned about crackers, even though I am using md5. So I was wondering whats the best way to use a remember function.
At the moment here's what happens
User logins
If user successfully logins and does not select remember me, store information as sessions otherwise as cookies. Checks cookie data against database to prevent hacking. If it doesn't exist logouts user, otherwise creates a session with the information.
So that's it so far, what can I do to make it more secure? Or do you think it is enough?
-
nada...welp i have figured something wierd out....this snipped will always say Signed In...no clue why...i made that session up...it doesn't exist anywhere except right there...there shouldn't be ANYTHING in it, especially a word.
if ($_SESSION['author'] = "yes") { echo "Signed In"; } else { echo "Log in please"; }
Just thought I would pint where the error was; you where trying to change the variable instead of checking it. So where you've said if ($_SESSION['author'] = "yes") that means change the session to yes when it should of been
if ($_SESSION['author'] == "yes")
Notice the difference? There is an extra equals sign.
-
To be honest, no idea. It was a function suggested by someone here, so I took the suggestion.
-
Hi guys I am creating a news system so that I can learn about PHP and Mysql, however I have run into a problem when submiting news, all new breaks appear as
r
when inserted into the database, I've tried using both Long Text field and a long blob field, however the results are always the same. I protect the fields from sql injections and from XSS attack by using a function, which is
function clean($string) { if (get_magic_quotes_gpc()) { $string = stripslashes($string); } elseif (!get_magic_quotes_gpc()) { $string = addslashes(trim($string)); } $string = trim($string); $string = escapeshellcmd($string); $string = mysql_real_escape_string($string); $string = stripslashes(strip_tags(htmlspecialchars($string))); return $string; }
So I wish to know, it is the protect function that is causing this, or is there something else I can do?
This is also the insert code of the section:
$title = clean($_POST['title']); $data = clean($_POST['data']); $author = clean($_SESSION['username']); $u_id = $_SESSION['u_id']; $alreadyExists = mysql_query("SELECT * FROM news WHERE title = '$title'")or die(mysql_error()); if(mysql_num_rows($alreadyExists)>1){ echo '<div class="error">News all ready exists! Please go <a href="'.$siteURL.'new_news.php">Back</a></div>'; }else{ $q = "INSERT INTO news(title,data,author,submit_date,u_id)VALUES('$title','$data','$author',now(),'$u_id')"; $r = mysql_query($q) or die(mysql_error()); $id = mysql_insert_id(); if ($r) { echo 'News successfully added!<br> Please click <a href="'.$siteURL.'view_news.php">here</a> to view the news. Or click <a href="'.$siteURL.'view_news.php?id='.$id.'">here</a> to view your news.'; } }
Here is the view code, mind you I've only just added the nl2br code:
if (isset($_GET['id']) && is_numeric($_GET['id'])) { $id = clean($_GET['id']); $sql2 = mysql_query("SELECT * FROM `news` WHERE id='$id'") or die(mysql_error()); $row = mysql_fetch_array($sql2); $title = $row['title']; $data = nl2br($row['data']); $author = $row['author']; echo '<h1>' . strtoupper($title) . '</h1>'; echo '<small>By :<a href="'.$siteURL.'profile.php?id='.$row['u_id'].'">'.$author.'</a><br></small>'; echo '<p>' . $data . '</p>';}
I just realized now, do I actually need to clean the id after checking that its numeric?
I have just added an edit function, but it appears something is wrong because as I get the data from the database, it leaves a large blank space, it also doesn't get the title;
I have added trim to the code but get the same results, here is the edit part of the code:
if (isset($_GET['id']) && is_numeric($_GET['id']) && isset($_GET['edit']) &&is_numeric($_GET['id'])) { $id = $_GET['id']; $sql = "SELECT * FROM news WHERE id = '$id'"; $result = mysql_query($sql)or die(mysql_error()); $row = mysql_fetch_array($result); $u_id = $row['u_id']; if ($_SESSION['u_id'] == $u_id || $_SESSION['u_level'] == 6){ ?><form action="<? echo $PHP_SELF; ?>" method = "post"> Title:<input type="text" name = "<?=$row['title'];?>" size="32"><br> Author:<input type = "text" name = "author" disabled = "true" value = "<?=$row['author'];?>"><br> <textarea rows="6" cols="40" name = "data"> <?=trim($row['data']);?> </textarea> <br> <input type = "submit" value = "Submit" name = "submit"> </form><? }else{ echo 'Not authorised to view this page!'; } }
-
This would only be on a testing server since I cannot test mail on my localhost, ie my machine as I don't know what program I need, and the programs I have used don't work
-
That's because as soon as you answer the secret question, it doesn't process the form at all, but rather just takes you back to the main part of the page, the user selection.
-
Oh sorry, I thought I had. Basically this is the flow chart:
User enters username
|
V
Checks that username exists
Exists Doesn't -> Throw an error
|
v
Displays the secret question, gets user to answer
Right answer Wrong answer-> Throw an error
|
v
Displays a new password form for user to enter password
Update is successful Not successful-> Throw an error
|
V
redirects back to login
The problem is that it doesn't get to the new password form. I hope that has clarified it.
-
Hey guys this is really annoying me, as I have never come across this type of problem before. The problem is this, after the form searches the database for the username, after successfully getting the secret question when that form is submitted it takes you back the the original page.
<?php include_once ('libary/header.php');//Includes style sheet, config and functions if (!isset($_POST['submit'])) { ?> <p>Please enter your username below.</p> <form method="POST" action="<?= $PHP_SELF; ?>"> Username:<input type = "text" name="username" ><br> <input type = "submit" name = "submit" value ="Submit"> </form> <?php } else { $username = clean($_POST['username']); $sql = "SELECT secretQuestion FROM user WHERE username = '$username' AND u_level>=1 LIMIT 1"; $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) != 0) { $row = mysql_fetch_array($result); $secretQuestion = ($row['secretQuestion']); if (!isset($_POST['secretAnswer'])) { ?><p>Please enter your answer to your secret question (CaSe SenSeTiVe).</p> <form method="POST" action="<?= $PHP_SELF; ?>"> Secret Question:<input type = "text" value="<?= $secretQuestion; ?>" ><br> Answer:<input type = "text" name="answer" ><br> <input type = "submit" name = "secretAnswer" value ="Submit"> </form> <?php }else{ echo 'Processing here.'; } }else { echo '<div class="error">The username you have supplied does not exist, or is not activated! Please supply another username.<a href="' . $siteURL . 'login.php?newPassword">Back</a></div>'; } } ?>
-
Bump (hope this is allowed) >_<
-
I have just added an edit function, but it appears something is wrong because as I get the data from the database, it leaves a large blank space, it also doesn't get the title;
I have added trim to the code but get the same results, here is the edit part of the code:
if (isset($_GET['id']) && is_numeric($_GET['id']) && isset($_GET['edit']) &&is_numeric($_GET['id'])) { $id = $_GET['id']; $sql = "SELECT * FROM news WHERE id = '$id'"; $result = mysql_query($sql)or die(mysql_error()); $row = mysql_fetch_array($result); $u_id = $row['u_id']; if ($_SESSION['u_id'] == $u_id || $_SESSION['u_level'] == 6){ ?><form action="<? echo $PHP_SELF; ?>" method = "post"> Title:<input type="text" name = "<?=$row['title'];?>" size="32"><br> Author:<input type = "text" name = "author" disabled = "true" value = "<?=$row['author'];?>"><br> <textarea rows="6" cols="40" name = "data"> <?=trim($row['data']);?> </textarea> <br> <input type = "submit" value = "Submit" name = "submit"> </form><? }else{ echo 'Not authorised to view this page!'; } }
-
Hi guys I am creating a news system so that I can learn about PHP and Mysql, however I have run into a problem when submiting news, all new breaks appear as
r
when inserted into the database, I've tried using both Long Text field and a long blob field, however the results are always the same. I protect the fields from sql injections and from XSS attack by using a function, which is
function clean($string) { if (get_magic_quotes_gpc()) { $string = stripslashes($string); } elseif (!get_magic_quotes_gpc()) { $string = addslashes(trim($string)); } $string = trim($string); $string = escapeshellcmd($string); $string = mysql_real_escape_string($string); $string = stripslashes(strip_tags(htmlspecialchars($string))); return $string; }
So I wish to know, it is the protect function that is causing this, or is there something else I can do?
This is also the insert code of the section:
$title = clean($_POST['title']); $data = clean($_POST['data']); $author = clean($_SESSION['username']); $u_id = $_SESSION['u_id']; $alreadyExists = mysql_query("SELECT * FROM news WHERE title = '$title'")or die(mysql_error()); if(mysql_num_rows($alreadyExists)>1){ echo '<div class="error">News all ready exists! Please go <a href="'.$siteURL.'new_news.php">Back</a></div>'; }else{ $q = "INSERT INTO news(title,data,author,submit_date,u_id)VALUES('$title','$data','$author',now(),'$u_id')"; $r = mysql_query($q) or die(mysql_error()); $id = mysql_insert_id(); if ($r) { echo 'News successfully added!<br> Please click <a href="'.$siteURL.'view_news.php">here</a> to view the news. Or click <a href="'.$siteURL.'view_news.php?id='.$id.'">here</a> to view your news.'; } }
Here is the view code, mind you I've only just added the nl2br code:
if (isset($_GET['id']) && is_numeric($_GET['id'])) { $id = clean($_GET['id']); $sql2 = mysql_query("SELECT * FROM `news` WHERE id='$id'") or die(mysql_error()); $row = mysql_fetch_array($sql2); $title = $row['title']; $data = nl2br($row['data']); $author = $row['author']; echo '<h1>' . strtoupper($title) . '</h1>'; echo '<small>By :<a href="'.$siteURL.'profile.php?id='.$row['u_id'].'">'.$author.'</a><br></small>'; echo '<p>' . $data . '</p>';}
I just realized now, do I actually need to clean the id after checking that its numeric?
-
Alright I will give it a try
-
Sorry didn't mean to lose you. Basically I don't know if I want a where clause like 'SELECT * FROM $table WHERE something = '$something';
Or if I needed a join
What I want to do is find out how many posts as user has made, the way I have made the tables they only would share two things, the author as the username of the poster and the user id as the id of the user (am I making sense?)
So then I would like to count how many times the user id is repeated in the news table, perhaps author would be better. After that I want PHP to spit out the results. I am going to see if I can find some open source software which may have the answer.
Thanks again,
Hopefully I made a bit more sense this time.
PHPFreaks Tutorials Wishlist
in PHPFreaks.com Website Feedback
Posted
Wow, didn't realize it was that simple. For myself, it is hard to get my head around on how to use. Well thanks for clearing that up, I will just try and google a good tutorial.
-James-