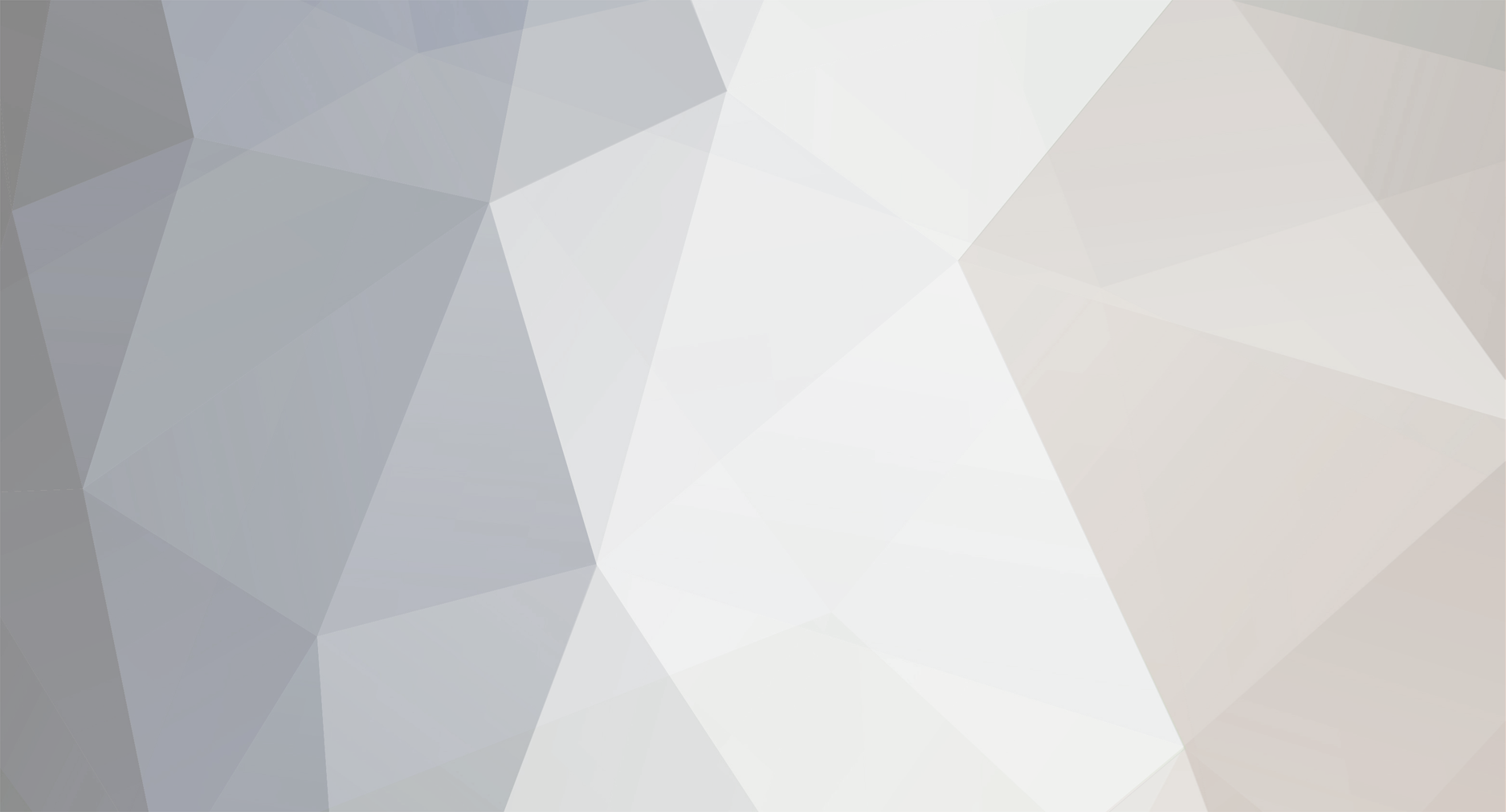
jrws
Members-
Posts
124 -
Joined
-
Last visited
Never
Everything posted by jrws
-
Hey guys, I have a problem. I made a simple login script but it won't protect against sql injections. I changed it so that it would echo the username in the login form, and it echoed: Here is the code I used (Blanked out password and user) <?php /* Login Page User can login here, if they cannot, displays a register page. As soon as they login they are redirected to welcome.php Has the added functionality to make a cookie, from the remember me button /*/ session_start(); $host = '****'; $user = '***'; $password = ''; $database = 'site'; $con = mysql_connect($host, $user, $password) or die('Error: Cannot Connect'); $condb = mysql_select_db($database); function safe($string) { if (get_magic_quotes_gpc()) { $string = stripslashes($string); } $string = mysql_real_escape_string($string); $string = strip_tags($string); return trim($string); } function encrypt($string) { $string = md5(sha1($string)); return $string; } if (isset($_POST['login'])) { $user = safe($_POST['user']); $pass = safe($_POST['pass']); $pass1 = encrypt(safe($_POST['pass'])); if(!ctype_alnum($user) OR !ctype_alnum($pass)){ return $errorA; } $error = array(); $q = mysql_query("SELECT * FROM user WHERE username = '$user' AND password = '$pass1' LIMIT 1") or die('Unable to connect...'); if (!$q OR $errorA) { $error[] = 'Appologises, username or password does not exist or are incorrect...</span>'; } if (count($error) > 0) { echo "The following errors occured:</br>"; foreach ($error as $err) { echo "<span style=\"color: red;\">$err<br>"; } echo 'Click to go <a href="#" onClick="history.go(-1)">Back</a>'; } //else { // if (isset($_POST['remember'])) { // setcookie("cookname", $_SESSION['username'], time() + 60 * 60 * 24 * 100, "/"); // setcookie("cookpass", $_SESSION['password'], time() + 60 * 60 * 24 * 100, "/"); //} //else { // $_SESSION['username'] = $user; // $_SESSION['password'] = $pass; // } echo "<meta http-equiv=\"Refresh\" content=\"0;url=welcome.php\"> Going to welcome page<br>Click <a href=\"welcome.php\">here</a> if you are not automatically redirected."; //} } else { ?> <form action="<? $_SERVER['PHP_SELF'] ?>" method="post"> Username:<input type ="text" name= "user"><br> Password:<input type ="password" name= "pass"><br> <input type="submit" name = "login" value = "Login"> <input type="checkbox" name="remember"> <font size="2">Remember me next time </form> <? } mysql_close(); ?> To see what I mean in a live example go to (my) site and login only as 'OR'x=x and it will auto redirect to welcome page... http://sandstorm.net46.net
-
Either a forum, blog or cms tutorial would be much appreciated.
-
alright I will try that. It worked, so now do I add the protect function (for injection attacks)? -edit- Thanks guys, you have given me an idea for future debugs.
-
Indeed it is, I was just thinking to use md5+ a salt, but I thought, just try it wilth sha1 as well. @MrAdam I don't think it is, because I have another test, but it doesn't search for user level, it just looks for username and password, and it works.
-
I am testing on a Windows machine, but I will edit, thanks.
-
//Encypts user password or a string, can be used for the activation code. function encrypt($string){ $string=md5(sha1(md5(sha1($string)))); return $string; } //Sanitizes user input function protect($string) { if (get_magic_quotes_gpc()) { //Check if magic_quotes is enabled $string = stripslashes($string); } $string = mysql_real_escape_string($string); //escape harmful characters $string = strip_tags($string); //remove HTML tags return trim($string); //Shorten string and return it } 2.Well I just tried what you suggested about the username and password. Not matter what the password the outcome is always: e8555537f6031e44c5c6937a3d62956a 3. echo "<div align=center><b>Oops! Your login is wrong. Please try again.</b></div>";
-
<?php include_once('functions.php'); if(isset($_POST['submit'])){ $username = protect($_POST['user']); $password = protect(encrypt($_POST['password'])); $query = "SELECT username, password,u_LV FROM user WHERE username ='$username' AND password = '$password' AND u_lv BETWEEN 1 AND 6"; $result = mysql_query($query)or die("Error, please contact staff. $bugE"); if(mysql_num_rows($result)>0){ Header("Location: logged_in.php"); } else { //Failed login, give error message. echo "<div align=center><b>Oops! Your login is wrong. Please try again.</b></div>"; } } close()//Close Database ?> Alright that's the code, now the problem. I am not sure but I think it is in the SQL syntax. So what should happen is this: user+password = login check; login check = query database, see if username and password match or even exist AND their user level is 1 to 6 (1 = activated gets progressively higher in user stats i.e. 6 = admin). IF login check fails (username/password don't match OR user not 1-6) give error message. Else login the user. So thats what should happen, but what is happening is that no matter what, login check fails and give the error message echo "<div align=center><b>Oops! Your login is wrong. Please try again.</b></div>"; What I want to know is what the problem is, and how I can fix it and identify it in the future as I am sure you guys get tired of solving code's for newbies/dunces like me.
-
Thanks I will try it, if it works thank you, if it doesn't well then I will have to find the problem.
-
Hey I was wondering how to do this, I know how to do too mysql selects: SELECT username AND password FROM user WHERE username='John' AND password = 'test123'; But how can I do it for three or more arguments? For example, this is the code I want, but it doesn't work: SELECT username AND password AND u_LV FROM user WHERE username ='$username' AND password = '$password' AND u_lv BETWEEN 1 AND 6; I don't get any errors but the code does not function as it should (Login a user)
-
Thanks so much man I will try again, you explained it so it shouldn't happen again. -edit- Working! Thanks so much, I will have to make sure I nest correctly
-
@Shauno, yes at least it is for the program I am using (PHP designer 2008) I will test it again on local host. @Ken2k7, the error message is a custom error incase the user is not found. I am not sure, but I think the SQL is fine else{//Failed login, give error message. echo "<div align=center><b>Oops! Your login is wrong. Please click back and try again.</b></div>"; -edit- Just tested using a browser, same problem.
-
Thanks guys, man I hate typo's, but thanks again, at least now I can make sure I check my code like I should! >< Thanks again. -edit- It didn't work, still displays the error message when it shouldn't... I changed it as suggested but it seems to still have the same problem. So no the only code changed is if(isset($_POST['submit'])){
-
My problem is that this code is supposed to execute AFTER the submit button is pressed. But that is not so, it just executes the code reguardless and as such gives the error message (the else statement). I would like to know what I did wrong and how to fix it as well as identify it in the future. <?php include_once('functions.php'); if(isset($_POST['Submit'])){ $username = protect($_POST['user']); $password = protect(encrypt($_POST['password'])); $query = "SELECT username, password AND u_LV FROM user WHERE username ='$username' , password = '$password' AND u_lv BETWEEN 1 AND 6"; $result = mysql_query($query)or die("Error, please contact staff. $bugE"); if(mysql_num_rows($result)>0){ $r=mysql_fetch_array($result);//Fetch arrays $login_username=$r["username"];//Make $login_username = to there username session_register("login_username");//Register a session Header("Location: logged_in.php"); } }else{//Failed login, give error message. echo "<div align=center><b>Oops! Your login is wrong. Please click back and try again.</b></div>"; } close()//Close Database ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <link rel="stylesheet" href="c3cd01d0/main.css" type="text/css" media="screen" /> <meta http-equiv="Content-Type" content="text/html; charset=us-ascii" /> <title>Registration</title> <style type="text/css"> /*<![CDATA[*/ div.c1 {text-align: left} /*]]>*/ </style> </head> <body> <p>All fields are required.</p> <form id="register" name="register" method="post" action="<? echo $HTTP_SERVER_VARS['PHP_SELF']; ?>"> <table width="292" border="0" cellspacing="0" cellpadding="3"> <tr> <th width="286" scope="row"> <div class="c1"> Username : <input name="user" type="text" maxlength="32" /> </div> </th> </tr> <tr> <th scope="row"> <div class="c1"> Password : <input name="pass" type="password" maxlength="32" /> </div> </th> </tr> </table><input type="submit" value="Submit" name="submit" /><input type="reset" name="Reset" value="Reset" /> <p>Or perhaps you would like to <a href="http://sandstorm.net46.net/register.php">register</a></p> </form> </body> </html>
-
Nevermind. Disregard, I didn't read you comments. But a question why just the username? And Not the password as well?
-
Alright I guess, I didn't make it clear, I will try again OK so what happens is that I have the $error array. This contains errors to display when something happens that I don't want to happen for example having a user enter blank info. The problem is these errors are now displaying even when there are no errors to display. I hope that clears it up.
-
Hey again guys, got errors again: http://pastebin.com/m7f64852c Thats the code (Bit big for forum) And the errors display no matter even if there are no errors. Anybody can help
-
Thanks you guys
-
Alright heres my problem, I am designing a website using my own code, I used the suggested error array error display. So what happens is no matter even if there are no errors when it is sent it still outputs all possible errors. Please help me rectify this. I am testing on local host ATM. Heres the code, and as far as I can tell it is perfectly fine. I was going to add more error checking but until I figure out why it doesn't work I have omitted it. <?php /** * Register page */ session_start(); include ("includes/functions.php"); if (isset($_POST['submit'])) { $error = array(); $user = protect($_POST['user']); $disname = protect($_POST['disname']); $pass = protect($_POST['pass']); $passcon = protect($_POST['passcon']); $email = protect($_POST['email']); $emailcon = protect($_POST['emailcon']); //Start Error checking if(!$user||!$disname||!$pass||!$passcon||!$email||!$emailcon){ $error[] = "One or more fields are not filled in, please try again.</span><br>"; } if(count($error) > 0) { echo "The following errors occured:</br>"; foreach($error as $err) { echo"<span style=\"color: red;\">$err"; } } else{ echo "Success!"; } } ?> <html> <body> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <link rel="stylesheet" href="c3cd01d0/main.css" type="text/css" media="screen"> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Registration</title> </head> <p>All fields are required.</p> <form id="register" name="register" method="post" action="<? echo $HTTP_SERVER_VARS['PHP_SELF']; ?>"> <table width="292" border="0" cellspacing="0" cellpadding="3"> <tr> <th width="286" scope="row"><div align="left">Desired Username : <input name="user" type="text" maxlength="32" /> </div></th> </tr> <tr> <th scope="row"><div align="left">Display Name: <input name="disname" type="text" maxlength="64" /> </div></th> </tr> <tr> <th scope="row"><div align="left">Desired Password : <input name="pass" type="password" maxlength="32" /> </div></th> </tr> <tr> <th scope="row"><div align="left">Confirm Password : <input name="passcon" type="password" maxlength="32" /> </div></th> </tr> <tr> <th scope="row"><div align="left">Email: <input type="text" name="email" /> </div></th> </tr> <tr> <th scope="row"><div align="left">Confirm Email : <input type="text" name="emailcon" /> </div></th> <tr> <th scope="row"><div align="left">Confirm Code (Bot protection): <input type="text" name="code" /><?code()?> </div></th> </tr> </table> <input type="submit" value = "Submit" name="submit"> <input type="reset" name="Reset" value="Reset"> <p>Or perhaps you would like to<a href="http://sandstorm.net46.net/login.php"> login </a></p> </form> </body> </html> <? footer(); ?> //Protect code: function protect($String) { $String = trim($String); $String = mysql_real_escape_string($String); $String = addslashes($String); $String = strip_tags($String); $String = stripslashes($String); //return $String; }
-
Not quite what I mean. This is what I mean Articles.php require login, user presses on link to go to articles.php and since they are not logged in go to login.php (this would use sessions). User would then login/signup and then be redirected to the page they where previously on. Does this make it move understandable?
-
What I am wondering is how people redirect users to pages I will give an example to clarify: User enters a site where some pages are only accessible by being registered/logged in and other pages are not. User enters a page where login is required and logs in. He is then able to continue looking at the page instead of being redirected to the main page. Obviously this is accomplished by a header, but what does the header contain? Please and thank you.
-
cool thanks. So would I just place the passwords in a SQL table or database? Also how could I implement that the admins and mods can only see the passwords?
-
Ok so lets see if I can explain it better: Once a password has been used, it randomises again. The other thing was, show the admins and mods what the current password is. So this could happen. When somebody wins the prize admins know the password and pass it along to the winner, they then claim their prize and the password changes. Does this make more sense?
-
What I want to know if it is possible (and if so how to implement it) is this: Pick a random password from an array and change ever 24 hours OR when a user uses the password, show admins and mods password so they do not have to look around. I was thinking that this will probably require MySQL for the User Database but I have no idea where to even start, I thought this idea was interesting so if you can help it is appreciated