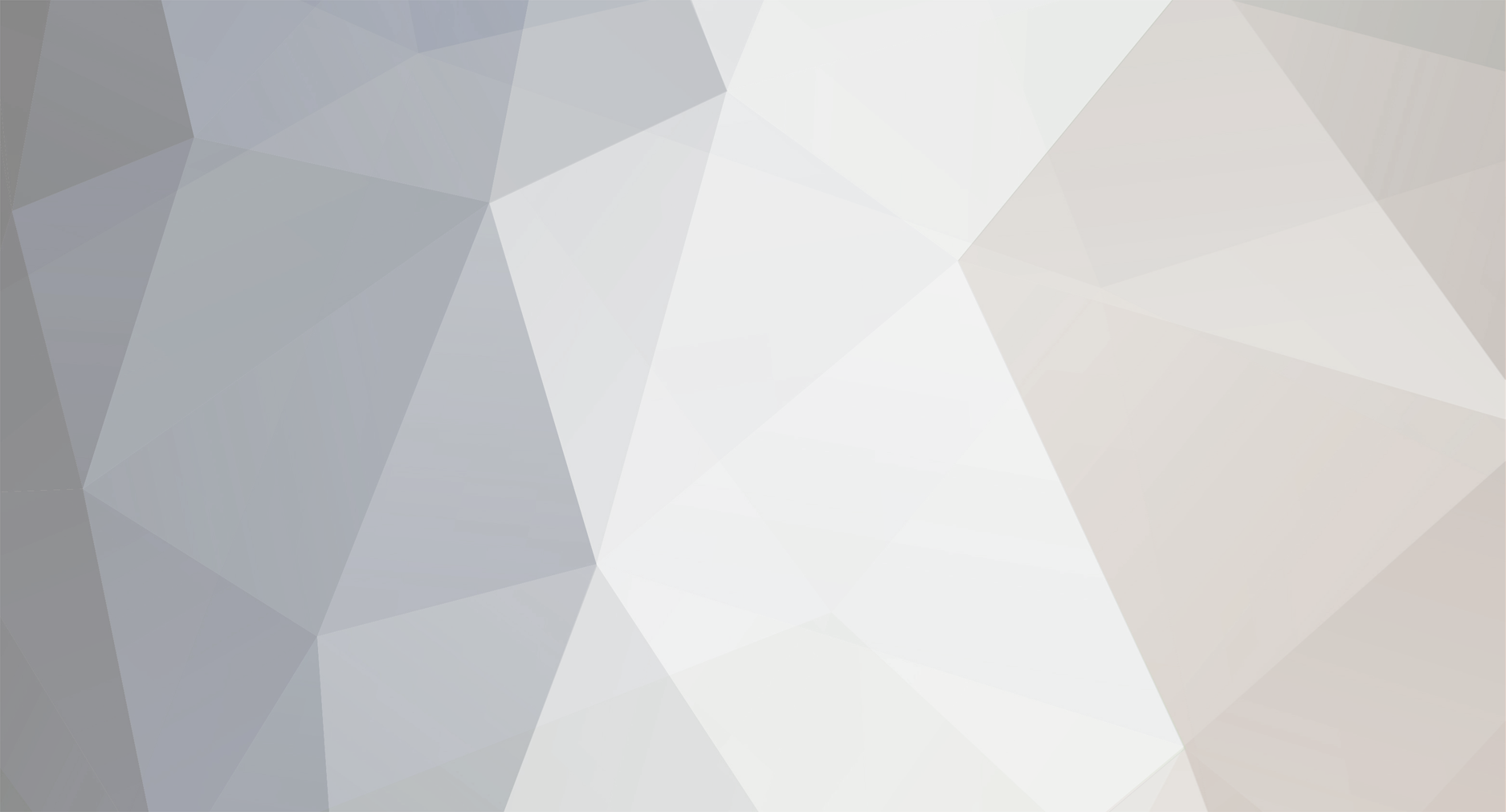
dragon_sa
Members-
Posts
520 -
Joined
-
Last visited
Everything posted by dragon_sa
-
set a default option value for the first option of every box then check for that option, eg if the value of the select box equals first option do nothing and move on, if value of select box does not equal first option then do something. I usually set the first option to something like --Select-- $select=$_POST['select']; foreach ($select AS $selected => $value) { if ($value!='--Select--') { do something } } $value will be the value of the selected box, if you name all your boxes select[somevar], this will create an array to loop through. $selected with be the somevar you put in the square brackets to uniquely identify each box
-
Cookie is not created and Page is not redirect properly....
dragon_sa replied to ankit.pandeyc012's topic in PHP Coding Help
instead of print_r($_COOKIE); use echo $_COOKIE["TestCookie"]; see if that gives you the value we set in the example code I posted -
echo out the ids to make sure you are getting them also the first item in the array starts at 0 $update_weed_world_id = $update_weed_world_data_while['id']; echo $update_weed_world_data_while['id']; $update_weed_world_basis[$update_weed_world_id] = $update_weed_world_data_while['weed_basis']; $update_weed_world_factor[$update_weed_world_id] = $update_weed_world_data_while['weed_factor'];
-
try this $Uid=$update_weed_world_data_while['id']; $update_weed_world_basis[$Uid] = $update_weed_world_data_while['weed_basis']; $update_weed_world_factor[$Uid] = $update_weed_world_data_while['weed_factor'];
-
see this reference http://bugs.php.net/bug.php?id=22108 discusses the effects of BOM
-
// scale to width $main_width = 160; $main_height = $img_height * ($main_width / $img_width); $original= imagecreatefromjpeg($img); you wont need the if statements this will do the trick
-
Cookie is not created and Page is not redirect properly....
dragon_sa replied to ankit.pandeyc012's topic in PHP Coding Help
what if you try this on a blank php page on the linux server <?php $value='my test cookie'; setcookie("TestCookie", $value); print_r($_COOKIE); ?> See if this prints out your newly set cookie -
Cookie is not created and Page is not redirect properly....
dragon_sa replied to ankit.pandeyc012's topic in PHP Coding Help
This will show you if the cookie is set [print_r($_COOKIE);/php] -
If max_ids is an auto increment unique id then that would suffice, if not then would need to use timestamp for date as suggested by revraz
-
Cookie is not created and Page is not redirect properly....
dragon_sa replied to ankit.pandeyc012's topic in PHP Coding Help
try to echo $row['LoginId'] after the query and see if it gives you a result also the php manual says to put cookie name in double quotes. eg setcookie("LoginIdCookie",$row['LoginId']); -
$query="SELECT * FROM table ORDER BY max_ids DESC LIMIT 3"; if you want for a particular user where you have set the variable of the userid $query="SELECT * FROM table WHERE userid='$userid' ORDER BY max_ids DESC LIMIT 3";
-
remove session array works for 1 but not 2 items
dragon_sa replied to dragon_sa's topic in PHP Coding Help
Rather than recoding several pages which call the session variables I found a bit of trickery using the onclick event on a checkbox. <input type="checkbox" name="remove[<?php echo $basket_counter; ?>]" value="<?php echo $basket_counter; ?>" onclick="document.formName.submit();" /> So now each time a checkbox is ticked it submits the form and removes that one item, then I can use the update cart button to modify the values of other session variables all at once using the current index system of the sessions. -
It should be echoed inside the while loop aswell if there is to be more than 1 result, if there is only supposed to be 1 result then there is no need for the while loop at all
-
point taken, to check we could use opendir to list the files from the download directory and put them into an array, then check to see if the requested file is in the array, if it is send the file if it isnt send an error message and return
-
remove session array works for 1 but not 2 items
dragon_sa replied to dragon_sa's topic in PHP Coding Help
How would that go for deleting multiple items in a loop, as the problem I am having now seems to be the $index number changes for each item after removal then the index numbers I have tagged for removal after that are for the wrong items. eg 10 items in the cart index 0-9 I send the trigger to remove 4, 7 and 9 4 removes fine on first loop but 8 becomes 7 and is then removed on second loop then on the third loop 9 has also become 7 so nothing gets removed as it is now the last item and there is no 9 to remove -
It looks like is because you are uploading a png file and not a jpg file so it is causing the error
-
The first part of the script points to the directory of downloadable files, as long as you only kept the files for download in that directory the core site php files would be safe
-
remove session array works for 1 but not 2 items
dragon_sa replied to dragon_sa's topic in PHP Coding Help
For me that would be an easy way but I want to reduce the number of calls to the database. I think sessions are good way to store temporary information before its entered into the database. -
<html> <?php if ($adult==0) { $class="note-general"; echo "<p class='$class'> This is the general </p>"; } if ($adult==1) { $class="note-warning"; echo "<p class='$class'> And this is the warning </p>"; } ?> </html>
-
remove session array works for 1 but not 2 items
dragon_sa replied to dragon_sa's topic in PHP Coding Help
I am suspecting that the issue may be as each time it loops the item positions change and then the set $remove_positions become incorrect, if this is the case how would I get around this to remove all items selected at once?? -
I found this to help you out create a file called download.php and put this code in it <?php $path = $_SERVER['DOCUMENT_ROOT']."/"; // change the path to fit your websites document structure $fullPath = $path.$_GET['download_file']; if ($fd = fopen ($fullPath, "r")) { $fsize = filesize($fullPath); $path_parts = pathinfo($fullPath); $ext = strtolower($path_parts["extension"]); switch ($ext) { case "pdf": header("Content-type: application/pdf"); // add here more headers for diff. extensions header("Content-type: application/php"); header("Content-Disposition: attachment; filename=\"".$path_parts["basename"]."\""); // use 'attachment' to force a download break; default; header("Content-type: application/octet-stream"); header("Content-Disposition: filename=\"".$path_parts["basename"]."\""); } header("Content-length: $fsize"); header("Cache-control: private"); //use this to open files directly while(!feof($fd)) { $buffer = fread($fd, 2048); echo $buffer; } } fclose ($fd); exit; ?> Be sure to look at the top of the script to make sure the path to your files is correct then on the page you want to download files from ad links like this <a href="download.php?download_file=file_to_download.php">Download Here</a>
-
apc is not bundled with php you will need to ask the server admins to install this for you if it is not already installed
-
When I remove 1 session item using this it works fine when I select more than 1 item to be removed I get unexpected results, I have checked the outputted html to see what positions get echoed into the remove check box and they are correct, so I guess the problem lies in my remove code here is the part of the form that sends the remove check box <?php for ($basket_counter=0;$basket_counter<$_SESSION['ses_basket_items'];$basket_counter++) { $price=sprintf("%01.2f",$ses_basket_price[$basket_counter]); $quantity=$ses_basket_amount[$basket_counter]; $code=$ses_basket_stockcode[$basket_counter]; $itemID=$ses_basket_id[$basket_counter]; $name=$ses_basket_name[$basket_counter]; $image=$ses_basket_image[$basket_counter]; $size=$ses_basket_size[$basket_counter]; $sizechoice=$ses_basket_sizechoice[$basket_counter]; // create array from sizes and make the option box selections if ($sizechoice!="" && (!in_array($sizechoice, array('One Size', 'one size', 'free size', 'Free Size', 'Adjustable', 'adjustable')))) { // add size trigger $sizelist="add"; //create the array $sizepick=explode(",", $sizechoice); } else { $sizepick=$sizechoice; } // adjust gst for AU customers if ($country=='AU') { $price=sprintf("%01.2f",($price*1.1)); $unit=sprintf("%01.2f",($price/$quantity)); } else { $unit=sprintf("%01.2f",($price/$quantity)); } ?> <tr> <td align='center' class='rescon' style="border-bottom:solid #330000 1px;"><input type="checkbox" name="remove[<?php echo $basket_counter; ?>]" value="<?php echo $basket_counter; ?>" /></td> and here is the part of the code that processes the removal of the items $remove = $_POST['remove']; if (isset($remove) && ($remove!='')) { foreach($remove AS $key => $remove_position){ array_splice ($ses_basket_name, $remove_position, 1); array_splice ($ses_basket_amount, $remove_position, 1); array_splice ($ses_basket_price, $remove_position, 1); array_splice ($ses_basket_stockcode, $remove_position, 1); array_splice ($ses_basket_image, $remove_position, 1); array_splice ($ses_basket_size, $remove_position, 1); array_splice ($ses_basket_sizechoice, $remove_position, 1); array_splice ($ses_basket_id, $remove_position, 1); $_SESSION['ses_basket_items']--; } } I am using a form with checkboxes named remove[$remove_position] and the positions in the form are correct for each item in the array, but when I select more than 1 item to delete they are not always the correct items only the first 1 selected. What am I doing wrong here??
-
How Radio button checked automatically??
dragon_sa replied to ankit.pandeyc012's topic in PHP Coding Help
try this <input type='radio' name='Sex' style='width:16px; border:0;' value='Male'<?php if($row['Sex']=='Male') echo "checked='checked'";?>'/>Male <input type='radio' name='Sex' '<?php if($row['Sex']=='Female') echo "checked='checked'";?>' style='width:16px; border:0;' 'value='Female' />Female -
Modify or delete multiple items from a session
dragon_sa replied to dragon_sa's topic in PHP Coding Help
Should I be echoing the itemID in the square brackets to use as a key for each action? eg name=remove"[itemID]" name="size[itemID]' name="quantity[itemID]" then what would be the best way to perform the actions?