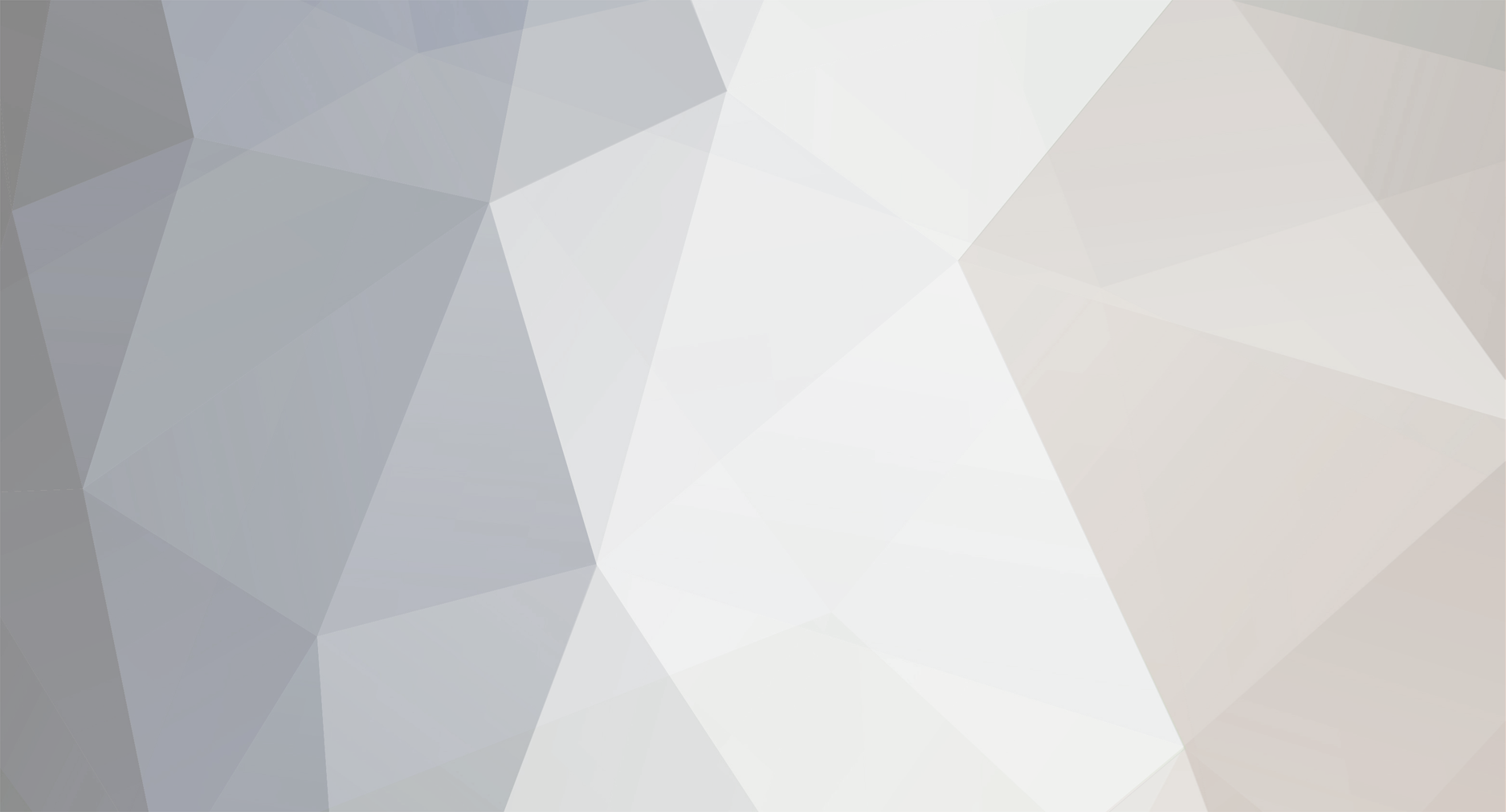
JayVee
Members-
Posts
21 -
Joined
-
Last visited
Never
Everything posted by JayVee
-
How would I go about rewriting this php in javascript? Its a simple cookie check with a redirect based on cookie value. else if (!isset($_COOKIE['legal'])) { header("Location: /index.php"); } else if($_COOKIE['legal'] == "no"){ header("Location: /index.php"); }
-
Ok tried that and pages still failed to index. Nothing on my site is indexing in google. (I'm using google webmaster tools to submit sitemap and show diagnostics.) Is it possible to recreate this problem locally with a different value other than googlebot so that I can test my code? That way I could see if my code was working correctly?
-
Ok I rewrote it as this <?php if ( strstr($_SERVER['HTTP_USER_AGENT'], "Googlebot" ) == true ){ // condition (1) //User has the GoogleBot user agent, but is it a real google bot? $host = gethostbyaddr($_SERVER['REMOTE_ADDR']); if ( substr($host, (strlen($host)-13)) == 'googlebot.com' ){ // condition (2) //real bot } } else if (!isset($_COOKIE['legal'])) { //condition (3) header("Location: /index.php"); } else if($_COOKIE['legal'] == "no"){ //condition (4) header("Location: /index.php"); } ?> It seems to work but google is still failing to index pages. Any advice?
-
Ok tried that but it bypassed the cookie security on the site. Pages displayed even if cookie didn't exist. <?php if ( strstr($_SERVER['HTTP_USER_AGENT'], "Googlebot" ) == true ){ // condition (1) //User has the GoogleBot user agent, but is it a real google bot? $host = gethostbyaddr($_SERVER['REMOTE_ADDR']); if ( substr($host, (strlen($host)-13)) == 'googlebot.com' ){ // condition (2) //real bot } else if (!isset($_COOKIE['legal'])) { //condition (3) header("Location: /index.php"); } //The code below is executed even if conditions (1) and (2) are true. if($_COOKIE['legal'] == "no"){ //condition (4) header("Location: /index.php"); } } ?> I want condition 1 and 2 to be checked. If true then I want the page to be viewable without any more condition checks. If false then I want both condition 3 and 4 to be checked. Do I need to put condition 4 into an ELSE IF statement?
-
Yes. Once the bot is legitimate I want the code to do nothing. If the bot is not legitimate or it's someone who is trying to access the page without gaining the proper cookie value I want the code to redirect back to 'index.htm' for a login form.
-
I'm trying to allow indexing of pages in my site which require a cookie. (I want the code to detect indexing by searchbots and to bypass the restrictions). Here is my code. It doesn't work. if ( strstr($_SERVER['HTTP_USER_AGENT'], "Googlebot" ) == true ){ //User has the GoogleBot user agent, but is it a real google bot? $host = gethostbyaddr($_SERVER['REMOTE_ADDR']); if ( substr($host, (strlen($host)-13)) == 'googlebot.com' ) { } //real bot else //fake bot or general access to page if(!isset($_COOKIE['legal'])) { header("Location: /index.php"); } if($_COOKIE['legal'] == "no") { header("Location: /index.php"); } Even is I get this to work it will only work for Google. Is there a better way to go about this. I don't need to log the activity of the searchbots. I just want them to gain access to the site.
-
I have a site which requires cookies to view a page content. Its an alcohol related site and legally needs to have an age restriction on site to validate user age. All the php age/cookie existence checking code works fine but I'm wondering if there is any php code I can add to allow search engines read pages without having the appropriate cookie/ cookie values.
-
I'm looking for code/files to allow a user to submit a passport type image. Ultimately I want the user to submit an image, be asked to crop this image via an interface and the resulting cropped image to be submitted. Is it possible to set the constraints in code so that the images are proportionally the same? Can anyone recommend a simple crop image editor using php?
-
Ok I have it working now. Huge thanks to Garethp for your time. Here are all the scripts. Home page php <?php /*if the cookie already exists from a previous remember me button click then skip this page*/ if($_COOKIE['legal'] == "yes") { header("Location: home.php"); } if (isset($_POST['checkage'])) { $day = ''; $month = ''; $year = ''; $minage = ''; $remember = 'O'; if(isset($_POST['day'])) { $day = $_POST['day']; } if(isset($_POST['month'])) { $month = $_POST['month']; } if(isset($_POST['year'])) { $year = $_POST['year']; } if(isset($_POST['country'])) { $minage = $_POST['country']; } $rembox=$_POST['remember']; $birthstamp = mktime(0, 0, 0, $month, $day, $year); $diff = time() - $birthstamp; $age_years = floor($diff / 31556926); if($age_years >= $minage) { if($rembox=="yes") { setcookie('legal', 'yes', time() + 31556926); } else{ setcookie('legal', 'yes', 0); } $url = '/bntest/home.php'; } else { setcookie('legal', 'no', 0); $url = '/bntest/minor.php'; } header('Location: '.$url.''); } /*echo "<p>".$_COOKIE["legal"]."</p>";*/ ?> Home page form (I cut the years back to 1960) and the legal age for most countries is included. <form id="form1" name="form1" method="post" action=""> <select name="country" id="country"> <option value="99" selected="selected">Choose Country</option> <option value="18">Ireland</option> <option value="99">Albania</option> <option value="18">Antigua</option> <option value="18">Argentina</option> <option value="99">Armenia</option> <option value="18">Australia</option> <option value="16">Austria</option> <option value="18">Azerbaijan</option> <option value="18">Bahamas</option> <option value="18">Barbados</option> <option value="18">Belarus</option> <option value="16">Belgium</option> <option value="18">Belize</option> <option value="18">Bermuda</option> <option value="18">Bolivia</option> <option value="18">Brazil</option> <option value="18">Bulgaria</option> <option value="19">Canada</option> <option value="18">China</option> <option value="18">Chile</option> <option value="18">Columbia</option> <option value="18">Costa Rica</option> <option value="18">Croatia</option> <option value="16">Cuba</option> <option value="17">Cyprus</option> <option value="18">Czech Republic</option> <option value="18">Denmark</option> <option value="18">Dominican Republic</option> <option value="18">Ecuador</option> <option value="21">Egypt</option> <option value="18">Estonia</option> <option value="18">Finland</option> <option value="18">Fiji</option> <option value="16">France</option> <option value="18">Georgia</option> <option value="16">Germany</option> <option value="17">Greece</option> <option value="18">Guam</option> <option value="16">Haiti</option> <option value="18">Hong Kong</option> <option value="18">Honduras</option> <option value="18">Hungary</option> <option value="20">Iceland</option> <option value="21">India</option> <option value="18">Israel</option> <option value="16">Italy</option> <option value="18">Jamaica</option> <option value="20">Japan</option> <option value="18">Kenya</option> <option value="18">Liechtenstein</option> <option value="17">Luxembourg</option> <option value="19">South Korea</option> <option value="18">Latvia</option> <option value="18">Lebanon</option> <option value="18">Lithuania</option> <option value="18">Macedonia</option> <option value="18">Malaysia</option> <option value="16">Malta</option> <option value="18">Mexico</option> <option value="18">Moldova</option> <option value="18">Morocco</option> <option value="18">Nepal</option> <option value="16">Netherlands</option> <option value="18">New Zealand</option> <option value="18">Nigeria</option> <option value="18">Norway</option> <option value="18">Paraguay</option> <option value="18">Peru</option> <option value="18">Philippines</option> <option value="18">Poland</option> <option value="16">Portugal</option> <option value="18">Puerto Rico</option> <option value="18">Romania</option> <option value="18">Russia</option> <option value="18">Serbia</option> <option value="18">Slovakia</option> <option value="18">Singapore</option> <option value="18">South Africa</option> <option value="18">Spain</option> <option value="18">Sri Lanka</option> <option value="18">Sweden</option> <option value="16">Switzerland</option> <option value="18">Taiwan</option> <option value="20">Thailand</option> <option value="18">Turkey</option> <option value="18">Uganda</option> <option value="18">Ukraine</option> <option value="21">U.A.E.</option> <option value="18">UK</option> <option value="21">USA</option> <option value="18">Venezuela</option> <option value="18">Other</option> </select> <select name="day" id="day"> <option value="1">Day</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> <option value="10">10</option> <option value="11">11</option> <option value="12">12</option> <option value="13">13</option> <option value="14">14</option> <option value="15">15</option> <option value="16">16</option> <option value="17">17</option> <option value="18">18</option> <option value="19">19</option> <option value="20">20</option> <option value="21">21</option> <option value="22">22</option> <option value="23">23</option> <option value="24">24</option> <option value="25">25</option> <option value="26">26</option> <option value="27">27</option> <option value="28">28</option> <option value="29">29</option> <option value="30">30</option> <option value="31">31</option> </select> <select name="month" id="month"> <option value="1" selected="selected">Month</option> <option value="1">January</option> <option value="2">February</option> <option value="3">March</option> <option value="4">April</option> <option value="5">May</option> <option value="6">June</option> <option value="7">July</option> <option value="8">August</option> <option value="9">September</option> <option value="10">October</option> <option value="11">November</option> <option value="12">December</option> </select> <select id="year" name="year"> <option selected="selected" value="2008">Year</option> <option value="1998">1998</option> <option value="1997">1997</option> <option value="1996">1996</option> <option value="1995">1995</option> <option value="1994">1994</option> <option value="1993">1993</option> <option value="1992">1992</option> <option value="1991">1991</option> <option value="1990">1990</option> <option value="1989">1989</option> <option value="1988">1988</option> <option value="1987">1987</option> <option value="1986">1986</option> <option value="1985">1985</option> <option value="1984">1984</option> <option value="1983">1983</option> <option value="1982">1982</option> <option value="1981">1981</option> <option value="1980">1980</option> <option value="1979">1979</option> <option value="1978">1978</option> <option value="1977">1977</option> <option value="1976">1976</option> <option value="1975">1975</option> <option value="1974">1974</option> <option value="1973">1973</option> <option value="1972">1972</option> <option value="1971">1971</option> <option value="1970">1970</option> <option value="1969">1969</option> <option value="1968">1968</option> <option value="1967">1967</option> <option value="1966">1966</option> <option value="1965">1965</option> <option value="1964">1964</option> <option value="1963">1963</option> <option value="1962">1962</option> <option value="1961">1961</option> <option value="1960">1960</option> </select> <input name="remember" type="checkbox" id="remember" value="yes" /> <input type="image" src="images/entersite.png" height="25" width="191" border="0" alt="Submit Form" name="checkage" value="submit"/> </form> and here is the include.php code to check on all pages within site <?php if(!isset($_COOKIE['legal'])) { header("Location: index.php"); } if($_COOKIE['legal'] == "no") { header("Location: index.php"); } ?>
-
How do I make a cookie expire at the end of a session? When i try setcookie('cookiename', 'yes', time() + 0); or setcookie('cookiename', 'yes', 0); the cookie expires instantly.
-
Almost there, I just about have this working. It worked with sessions so I wanted to try and get it working with Cookies so that the user could decide to set up a 'remember me' option and set a cookie with a year long expiry date. I have the cookies setting properly and the checking script on subsequent pages. I just can't get the $remember variable to add to the time() in the set cookie script. Here's the code. if (isset($_POST['checkage'])) { $day = ''; $month = ''; $year = ''; $minage = ''; $remember = 'O'; if(isset($_POST['day'])) { $day = $_POST['day']; } if(isset($_POST['month'])) { $month = $_POST['month']; } if(isset($_POST['year'])) { $year = $_POST['year']; } if(isset($_POST['country'])) { $minage = $_POST['country']; } if(isset($_POST['remember'])) { $remember = 31556926; } $birthstamp = mktime(0, 0, 0, $month, $day, $year); $diff = time() - $birthstamp; $age_years = floor($diff / 31556926); if($age_years >= $minage) { setcookie('legal', 'yes', time() + 31556926); $url = '/bntest/home.php'; } else { $_SESSION["legal"] = "No"; setcookie('legal', 'no', time() + 31556926); $url = '/bntest/minor.php'; } header('Location: '.$url.''); } When I try to use setcookie('legal', 'yes', time() + $remember) The page fails.
-
Thanks Garethp, the form is finally displaying. I'm going to check and see if I can get the sessions to check on each page in the site and I will paste up the code if it works.
-
Thanks Garethp, Ok I'm with you there. But if my code is included before my html and its the first time you load the page then the isset statement should be ignored (because no form data was sent). Then, on form submission page reloads and the code recognises that form data was sent and processes the script. Am I way off? My issue is that the if statement I have blocks the loading of the html on the page.
-
Can't see where I've got too many }. I want this code to run on submission of form and not as soon as the page is loaded. Regarding the ? and the : They work like true and false in the statement. They set the values to null if the Post field does not exist. I have removed them but still have the same problem.
-
Ok I have narrowed this down a bit. Is it possible that the "if (isset($_POST['checkage']))" statement has a syntax error. If I comment it out the page displays properly. Am I setting the Session variables properly from within an 'if' statement? Any help? <?php session_start(); if (isset($_POST['checkage'])) { $day = $_POST['day'] ? $_POST['day'] : ''; $month = $_POST['month'] ? $_POST['month'] : ''; $year = $_POST['year'] ? $_POST['year'] : ''; $minage = $_POST['country'] ? $_POST['country'] : ''; $birthstamp = mktime(0, 0, 0, $month, $day, $year); $diff = time() - $birthstamp; $age_years = floor($diff / 31556926); if($age_years >= $minage) { $_SESSION["Age"] = "Yes"; $url = '/home.htm'; } else { $_SESSION["Age"] = "No"; $url = '/minor.htm'; } header('Location: $url'); } ?>
-
Thanks Garethp I can see how using sessions is easier on the 'follow on' pages but this code still gives me a blank page. Here is the updated code. <?php session_start(); if(isset($_POST['checkage'])) { $day = ctype_digit($_POST['day']) ? $_POST['day'] : ''; $month = ctype_digit($_POST['month']) ? $_POST['month'] : ''; $year = ctype_digit($_POST['year']) ? $_POST['year'] : ''; $birthstamp = mktime(0, 0, 0, $month, $day, $year); $diff = time() - $birthstamp; $age_years = floor($diff / 31556926); if($age_years >= 18) { $_SESSION['Age'] = "Yes"; # The above line sets a cookie called "legal" throughout the entire domain and its value is yes. The cookie will expire next year, if it is not manually deleted. $url = '/home.htm'; } else { $_SESSION['Age'] = "No"; # You're not old enough, come back next year! $url = '/minor.htm'; } header('Location: $url'); } ?> Any ideas?
-
I'm trying to put an alcohol related website together and want to include a minimum age verification script on the site. The code I use is not redirecting properly (I get a blank page with no source code). I've never set a cookie before so I'm not sure where the script is falling down. Heres what appears before the head content on my page. <?php if(isset($_POST['checkage'])) { $day = ctype_digit($_POST['day']) ? $_POST['day'] : ''; $month = ctype_digit($_POST['month']) ? $_POST['month'] : ''; $year = ctype_digit($_POST['year']) ? $_POST['year'] : ''; $birthstamp = mktime(0, 0, 0, $month, $day, $year); $diff = time() - $birthstamp; $age_years = floor($diff / 31556926); if($age_years >= 18) { setcookie('legal', 'yes', 0, '/', '.'.$_SERVER['SERVER_NAME']); # The above line sets a cookie called "legal" throughout the entire domain and its value is yes. The cookie will expire next year, if it is not manually deleted. $url = '/home.htm'; } else { setcookie('legal', 'no', 0, '/', '.'.$_SERVER['SERVER_NAME']); # You're not old enough, come back next year! $url = '/minor.htm'; } header('Location: $url'); } ?> Any help would be appreciated.
-
Thanks for the replies. I used discomatts code and got it to work. Both where a great help, I owe ya, thanks.
-
How would I go about rewriting that query so that I could loop in my values?
-
I want to add array values to a sql query by using a loop but I'm unsure of the syntax. This is my array. if (array_key_exists('box', $_POST)) { $box1 = $_POST["box"]; } $myarray = $_POST['box']; I've echoed out the values so I know they are being passed correctly. Here's where I'm going wrong somewhere. $query_Recordset1 = "SELECT DISTINCT `id` ,`field1` , `field2` FROM `SomeTbl` WHERE "foreach ($box as $suggestion) { "field1` LIKE '%'.$suggestion.'%' OR field2` LIKE '%'.$suggestion.'%' AND"; }" LIMIT 0 , 30"; Also, I've seen a bug with this as it will leave an extra "AND" in the statement. Can I remove this? I know its probably riddled with errors but any help would be appreciated.
-
Having some trouble with this search code. I want a simple multi word search form but when I run this code only the last word of any search string returns. i.e. search for 'dog cat' - cat returns. Alternately search for 'cat dog' - dog returns. I'm pretty new to this so any help would be appreciated. Here's the code //search variable = data in search box or url if(isset($_GET['search'])) { $search = $_GET['search']; } //trim whitespace from variable $search = trim($search); $search = preg_replace('/\s+/', ' ', $search); //seperate multiple keywords into array space delimited $keywords = explode(" ", $search); //Clean empty arrays so they don't get every row as result $keywords = array_diff($keywords, array("")); //Set the MySQL query if ($search == NULL or $search == '%'){ } else { for ($i=0; $i<count($keywords); $i++) { $query = "SELECT * FROM CourseTbl " . "WHERE CourseID LIKE '%".$keywords[$i]."%'". " OR CourseTitle LIKE '%".$keywords[$i]."%'" . " OR CourseAims LIKE '%".$keywords[$i]."%'" . " ORDER BY CourseTitle"; } //Store the results in a variable or die if query fails $result = mysql_query($query) or die(mysql_error()); } if ($search == NULL or $search == '%'){ } else { //Count the rows retrived $count = mysql_num_rows($result); } echo "<html>"; echo "<head>"; echo "<title>Your Title Here</title>"; echo "<link rel=\"stylesheet\" type=\"text/css\" href=\"style.css\" />"; echo "</head>"; echo "<body onLoad=\"self.focus();document.searchform.search.focus()\">"; echo "<center>"; echo "<br /><form name=\"searchform\" method=\"GET\" action=\"searchfull.php\">"; echo "<input type=\"text\" name=\"search\" size=\"20\" TABINDEX=\"1\" />"; echo " <input type=\"submit\" value=\"search\" />"; echo "</form>"; //If search variable is null do nothing, else print it. if ($search == NULL) { } else { echo "You searched for <b><FONT COLOR=\"blue\">"; foreach($keywords as $value) { print "$value "; } echo "</font></b>"; } echo "<p> </p><br />"; echo "</center>"; //If users doesn't enter anything into search box tell them to. if ($search == NULL){ echo "<center><b><FONT COLOR=\"red\">Please enter a search parameter to continue.</font></b><br /></center>"; } elseif ($search == '%'){ echo "<center><b><FONT COLOR=\"red\">Please enter a search parameter to continue.</font></b><br /></center>"; //If no results are returned print it } elseif ($count <= 0){ echo "<center><b><FONT COLOR=\"red\">Your query returned no results from the database.</font></b><br /></center>"; //ELSE print the data in a table } else { //Table header echo "<center><table id=\"search\" bgcolor=\"#AAAAAA\">"; echo "<tr>"; echo "<td><b>COLUMN 1:</b></td>"; echo "<td><b>COLUMN 2:</b></td>"; echo "<td><b>COLUMN 3:</b></td>"; echo "<td><b>COLUMN 4:</b></td>"; echo "<td><b>COLUMN 5:</b></td>"; echo "<tr>"; echo "</table></center>"; //Colors for alternation of row color on results table $color1 = "#face9e"; $color2 = "#bdebf2"; //While there are rows, print it. while($row = mysql_fetch_array($result)) { //Row color alternates for each row $row_color = ($row_count % 2) ? $color1 : $color2; //table background color = row_color variable echo "<center><table bgcolor=".$row_color.">"; echo "<tr>"; echo "<td>".$row['CourseID']."</td>"; echo "<td>".$row['CourseTitle']."</td>"; echo "<td>".$row['CourseAims']."</td>"; echo "<td>".$row['CourseDuration']."</td>"; echo "<td>".$row['EntryRequirements']."</td>"; echo "</tr>"; echo "</table></center>"; $row_count++; //end while } //end if } echo "</body>"; echo "</html>"; if ($search == NULL or $search == '%') { } else { //clear memory mysql_free_result($result); } ?>