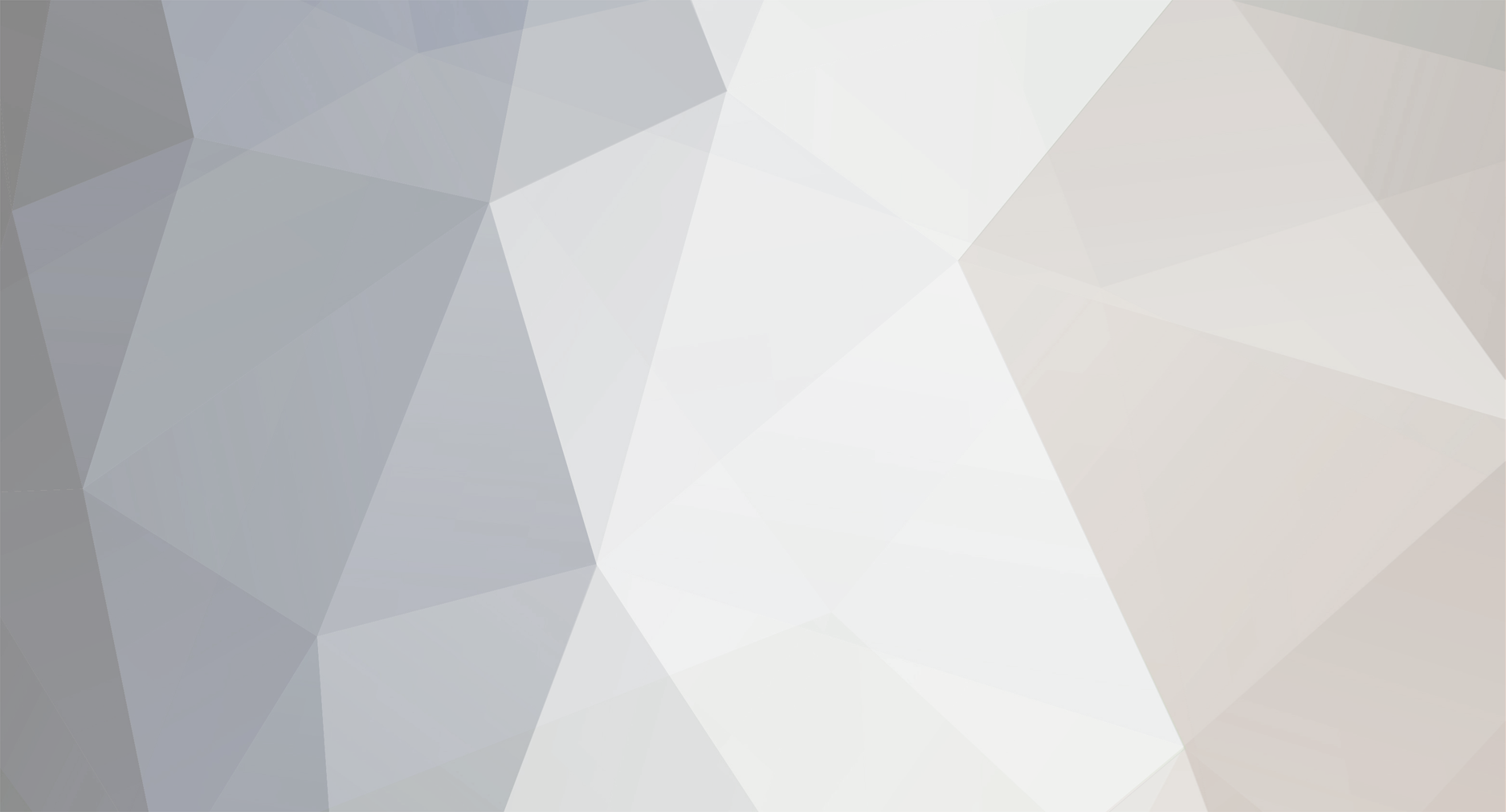
wiggst3r
-
Posts
94 -
Joined
-
Last visited
Never
Posts posted by wiggst3r
-
-
Any idea how I could do that?
-
Hi
I'm trying to write a news archive system, which, on the news page, displays one news article and links to the others in the db.
When I click on the link to one of them, I want it to display in another page, and just display that particular article.
I'm trying to write a while loop, which loop through the articles, and displays the individual article, based on it's id, but i'm a little stuck.
I've written a fucntion to display the news article
function get_one_article($id) { $id = mysql_real_escape_string($id); $query = "SELECT * FROM news WHERE id = '$id' LIMIT 1"; $result = @mysql_query ($query); // Run the query. $num = mysql_num_rows($result); $one_row = mysql_fetch_array($result, MYSQL_ASSOC); return $one_row; }
I want to put the following code into a while loop and get the news story for it's id
echo $one_row = get_one_article($_GET['id']);
When i var_dump that code, I can see the articles details, just can't seem to loop through and print it to the screen
Thanks
-
The test file is named download.txt
How could i implement this into that piece of code?
-
Hi
I have a script that adds files to a folder, that I get from FTP.
I download these files everyday and are txt files, but are iin CSV format.
In this folder I currently have 3 files. 1.csv, 2.csv and 3.csv
Rather than me renaming them everyday, I'm looking to write a script to auto-increment the filename.
So i'm looking to loop through the files in the folder, If there is no filename which is 4.csv, the file that has been downloaded will be renamed to this.
The next day, the file will be renamed to 5.csv etc
Does anyone know the code to do this?
Thanks
-
Hi
I'm trying to dowload a file from my FTP site to my local machine and i keep getting errors.
I'm using the following code:
$local_file = '/Users/mac/workspace/folder_test/' . $last_file; $server_file = '/folder_name/' . $last_file; // set up basic connection $conn_id = ftp_connect($ftp_server); // login with username and password $login_result = ftp_login($conn_id, $ftp_user_name, $ftp_user_pass); // try to download $server_file and save to $local_file if (ftp_get($conn_id, $local_file, $server_file, FTP_BINARY)) { echo "Successfully written to $local_file\n"; } else { echo "There was a problem\n"; } // close the connection ftp_close($conn_id);
The file I'm looking for is in the the folder, 'folder_name' on the ftp server and I'm trying to download it to my workspace on my mac.
I get the following errors:
Warning: ftp_get(/Users/mac/workspace/folder_test/File.txt) [function.ftp-get]: failed to open stream: No such file or directory in /Users/mac/workspace/folder_test/index.php on line 40Warning: ftp_get() [function.ftp-get]: Error opening /Users/mac/workspace/folder_test/File.txt in /Users/mac/workspace/folder_test/index.php on line 40
There was a problem
Any ideas?
-
The code you gave me works fine, as it finds the file at the bottom of the folder.
But it returns a file, that was created on 17/05/2008
I'd like it to loop through the files on the FTP and find the file that matches the string Transfer_20080617.txt, for example.
Where Transfer_20080617.txt, was the last file that was uploaded before todays date. There are other files and folders on the FTP, but I'm only concerned with finding the files
with the Transfer_2008xxxx.txt.
Any ideas?
-
Theres 2 folders
One called import and one called archive.
How would I remove this folder too?
Thanks
-
It only works when I use:
$last_file = $contents[count($contents) - 23 ];
-
Hi
Thanks for that
There's a problem though.
I have a folder named 'import', which was created on 17/12/2007 and the code you gave me, outputs
The last file created was importIt should say
The last file created was Transfer_20080619.txtAny ideas why?
-
Hi
I have an FTP server, that has files put onto it (supposedly daily, but not always). Each file has the name 'Transfer_20080620.txt' for example. The date changes depending on which day/month it is.
As the files are put on the FTP server early in the morning, I'm looking to find the date of the last filename that is on the server (so I know the script is running properly when I get to work).
What I'm looking to do, is find todays date (which I've done) and then loop through the file(names) and find the filename, which is closest to todays date.
So, if there were files called 'Transfer_20080610.txt' , 'Transfer_20080612.txt', 'Transfer_20080613.txt', 'Transfer_20080614.txt', 'Transfer_20080617.txt', the loop would find the file named
'Transfer_20080617.txt'
My code so far is:
<?php function ftp_dwn($server,$user,$pass,$fromDir,$toDir) { $ftp_server = $server; $ftp_user_name = $user; $ftp_user_pass = $pass; $conn_id = ftp_connect($ftp_server); $login_result = ftp_login($conn_id, $ftp_user_name, $ftp_user_pass); if((!$conn_id) || (!$login_result)) { echo "Could not Connect to the FTP Server"; exit(); } if(!file_exists($toDir)) // If our local directory doesn't exists let's create it mkdir($toDir); $contents = ftp_nlist($conn_id, $fromDir); // Compiles an array of all given folders in remote structure } print $contents; // Initialize FTP Server $ftp_server = "www.example.com"; $ftp_user_name = "xxxxxxx"; $ftp_user_pass = "xxxxxx"; $fromDir = "folder"; $toDir = "/Users/mac/workspace/test_ftp"; ftp_dwn($ftp_server,$ftp_user_name,$ftp_user_pass,$fromDir,$toDir); $today = date("Ymd"); print $today; ?>
Thanks
-
<?php $handle = fopen("results.csv", "r"); $race_time = 13; // column number of total time $mob_num = 10;// column number of mob num $url1 = '<a href="http://www.example.com">www.www.example.com</a> '; $url2 = '<a href="http://www.example.com">http://www.example.com</a> '; while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { echo "Congratulations on finishing the race, your time was " . $data[$race_time] . " Full race results at " . $url1 . $url2 . '<br><br>'; } fclose($handle); ?>
I have this so far, that loops through the results and displays each row to the screen.
I have a column, which will have UK mobile phone numbers in (e.g. 07777777777), one number per racer.
I'll need to validate this number and make sure it is in the right format, and then drop the '0' and add +44 to the start, so that the
sms(AQL) API can send the sms to the correct recipient. Which I'm not sure how I'd validate the mobile number.
I have the sms function that I could post, If anyone is willing to help me with?
Thanks
-
Hi
I have a csv with 17 columns.
I'm only interested in 2 columns though, one is a 'TotalTime' column and the other is a MobileNumber(will be used to send an sms at a later time)
What I'm looking to do, is loop through each row and append the time to pre-defined string, such as:
Congratulations on completing the race, your time was 00:00:00 Full race resutls results at www.example.comFor testing, I would like it to be outputted, in html to the screen (so i know it's working)
Any ideas how I could do this?
-
Hi
I'm trying to create a drag and drop functionality to a page.
I'm using a foreach loop to loop through records of a database and output them.
I have a problem though with the output on the page.
As I'm pulling records from 6 columns, all the inofmration within the output is all squashed together.
for example
AAThis is a test messageFridayExample1I want each of the column results to be nicely separated.
My code is as follows for the foreach loop:
<div id="listContainer"> <? foreach($list as $item) { ?> <div id="item_<?=$item['catid'];?>"><?=$item['briefed'];?><?=$item['category'];?><?=$item['needed'];?><?=$item['status'];?><?=$item['orderid'];?></div> <? } ?> </div>
Any ideas how I can separate these results (without using html spaces) for example
Thanks
-
It's in an admin panel.
There are 4 columns with details in that are gathered from a form.
The checkbox is there, so that when the admin has replied to a user that has entered their details, the admin can
check the checkbox, to signify that they have replied.
I want the checkbox to be constantly checked.
But I'm not sure how to do this.
-
Hi
I want to have a checkbox on a page that, when it has been checked and the user clicks on a submit button, the next time the user
visits the page, this checkbox is always checked.
How would I do this?
-
Hi guys
I've been working on a shopping cart using Zen Cart and I have a slight problem.
When you go to the shopping cart page (page 2 of 3), it lists the prices (sub-total, total etc) but I also included a 'Discount' price.
When this is used, I get a problem with the aligning of the prices, as the discount adds a minus (-)
So my prices are like this:
Sub-Total: £xx.xx
Standard Rate: £xx.xx
10% Discount: £-xx.xx
Total: £xx.xx
What I'm looking to do, is possibly run a loop, which will check for the word 'Discount', If the word is not found in the string (such as 'Sub-Total') It will add a html space ( ) at the end, so it's all lined up correctly.
But I'm not sure how I'd do that within the following code:
<?php /** * Displays order-totals modules' output */ for ($i=0; $i<$size; $i++) { ?> <div id="<?php echo str_replace('_', '', $GLOBALS[$class]->code); ?>"> <div class="totalBox larger forward"><?php echo $GLOBALS[$class]->output[$i]['text']; ?></div> <div class="lineTitle larger forward"><?php echo $GLOBALS[$class]->output[$i]['title']; ?></div> </div> <br class="clearBoth" /> <?php } ?>
Thanks
While and FTP
in PHP Coding Help
Posted
Hi guys
I'm doing a small project and I need some help.
I'm trying to query a database and find the last row that was inserted into the database determined by the date(e.g, 25th June - 2008-06-25) - which I've done (see code below)
I'm then trying to loop through some files on an FTP server, which are in the format, File_2008_06_25.txt, for example.
From then, I want to check that the last inserted row, if it was 25th june, loops through the files on the FTP and finds then next file, File_2008_06_26.txt and runs a function I've written, that processes the txt files contents, once that's done, it will be inserted into the database. So then, the last inserted date/file, would be 26th june etc
This should loop around, until it reaches todays date, when it should exit.
so what I'm looking to do is:
1) Get last row which was inserted into the database (date)
2) Search the FTP and check the date of the file, if not in the database, download and run a function
3) Go to next file, if not in database, download and run the the function etc etc (the loop)
4) If the last file on the FTP server has a date that is greater than todays' date, then the script should stop.
Can anyone point me in the right direction?
I'm really stuck
Thanks