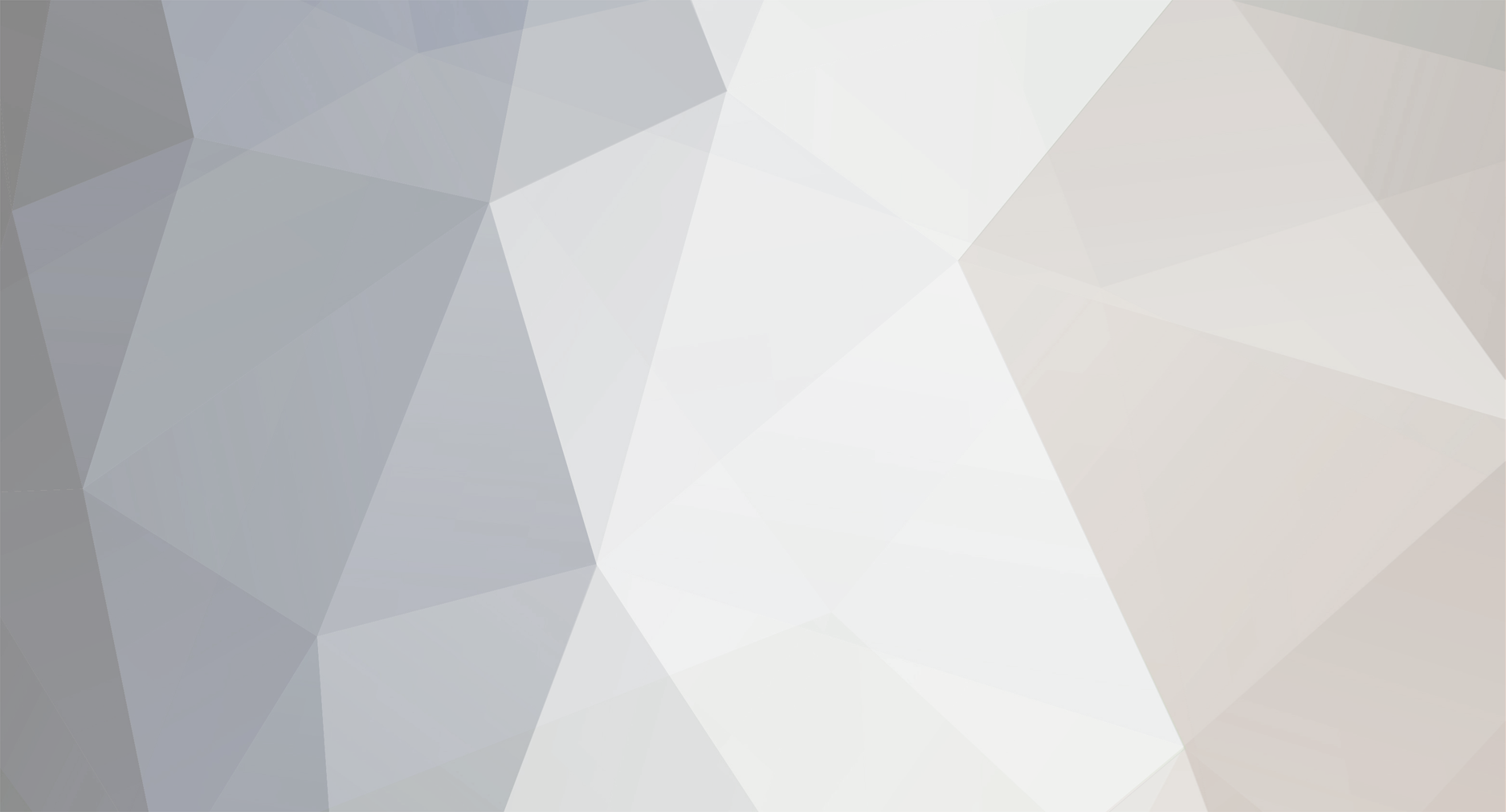
andrew_biggart
-
Posts
363 -
Joined
-
Last visited
-
Days Won
1
Posts posted by andrew_biggart
-
-
Thanks for this xyph, this has given me a better understanding about security, storing passwords and hashing. Everything you have shown me has been very useful.
Thank you for you patience and help. I shall keep this thread open as no doubt I will be back.
-
That is a very valid point about storing the username and password in a cookie. I will NOT do that again.
So what you are suggesting is that whenever a user logs in, I check the credentials, if they are correct I add a authcode to the database and the remember cookie and redirect. If the user goes off the site and then back on I check the database for the authcode cookie and if it exists skip the loggin process?
I'm just struggling to get my head around how the whole process works properly.
As for the md5 issues, I have created the below function to use instead of md5 which will hopefully make it a lot more secure. Do you agree?
<?php function md5_me($input){ $salt = "mysecretkeygoeshere"; $pass = md5($input.$salt); $pass = str_ireplace(array("a","c","e"),"",$pass); return md5($pass); ?> }
-
Surely I shouldn't have to check for the cookie variable as it only gets created if a user want's to be remembered so it shouldn't be essential.
-
Surely I should check if the session uid is set OR the cookie remembered is set instead of AND.
Would this be what your suggesting?
<?php session_start(); $url = (!empty($_SERVER['HTTPS'])) ? "https://".$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI'] : "http://".$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI']; $uid = $_SESSION['uid']; $check = $_COOKIE['remembered']; if (!isset($uid) OR !isset($check)) { header('location:login.php?redirect='.$url); exit(); } ?>
-
But surely if I do that then the users who have not checked the keep me logged in checkbox will not be able to access any of the other admin pages?
Could you give me an example of what you mean?
Also what is your opinion on the security of this login system?
Thanks
Andrew
-
I am trying to build my own custom login script.
What I am trying to achieve is once a user has logged in depending on wether they have checked the keep me logged in checkbox they have two options. If they haven't checked it then it creates session variables only, and if they have checked it it also creates cookie variable as well as the session variables.
If they then close their browser / tab without logging out and then revisit the site they will get redirected to login page because the active session variable is no longer there. As soon as they land on the loggin page, it automatically checks for the cookie variable and if it exists, it uses it to login and redirect them automatically.
However the problem that I am facing is that the session variable is still being trashed after a default amount of idle time and forcing a login.
My goal is that the user shouldn't have to re-login unless they have either clicked the logout button.
Can someone please have a look through my solution and advise me as to wether this is the correct method that I am implementing, if there is an easier way to achieve what I want, and is this a secure way to handle user logins.
Thanks in advance.
Andrew
Here is the check code I have placed at the top of each admin page.
<?php session_start(); $url = (!empty($_SERVER['HTTPS'])) ? "https://".$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI'] : "http://".$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI']; $uid = $_SESSION['uid']; if (!isset($uid)) { header('location:login.php?redirect='.$url); exit(); } ?>
Next we have the code for the login.php file.
<?php include ('functions.php'); ?> <?php get_header('login'); ?> <div id="login-result"> <?php connect(); $redirect = htmlspecialchars(mysql_real_escape_string(addslashes($_GET['redirect']))); if(isset($_COOKIE['remembered'])){ $username = htmlspecialchars(mysql_real_escape_string(addslashes($_COOKIE['remembered']['username']))); $password = htmlspecialchars(mysql_real_escape_string(addslashes($_COOKIE['remembered']['password']))); $sql = "SELECT * FROM usersT WHERE username='$username' AND password='$password'"; $result = mysql_query($sql); $count = mysql_num_rows($result); $row = mysql_fetch_array($result); $uid = $row['uid']; $fname = $row['firstname']; $lname = $row['lastname']; $role = $row['role']; if($count==1){ $sql2 = "UPDATE usersT SET status = '1' WHERE uid = '$uid'"; $result2 = mysql_query($sql2); if($result2){ session_register("uid"); session_register("uname"); session_register("ulevel"); $_SESSION["uid"] = $uid; $_SESSION["uname"] = $fname; $_SESSION["ufullname"] = $fname . " " .$lname; $_SESSION["urole"] = $role; $home = get_option('home'); if(!empty($redirect)) { header( 'Location: '. $redirect ) ; exit(); } else { header( $home ) ; exit(); } } } else { echo "<div class=\"error rounded5 shadow\">Invalid username or password!</div>"; } } else if (isset($_POST['admin_login'])){ if(isset($_POST["username"]) && isset($_POST["password"])){ $username_p = htmlspecialchars(mysql_real_escape_string(addslashes($_POST["username"]))); $password_p = htmlspecialchars(mysql_real_escape_string(addslashes($_POST["password"]))); $psw = md5($password_p); $sql3 = "SELECT * FROM usersT WHERE username='$username_p' AND password='$psw'"; $result3 = mysql_query($sql3); $count3 = mysql_num_rows($result3); $row3 = mysql_fetch_array($result3); $uid = $row3['uid']; $fname = $row3['firstname']; $lname = $row3['lastname']; $role = $row3['role']; if($count3==1){ $sql4 = "UPDATE usersT SET status = '1' WHERE uid = '$uid'"; $result4 = mysql_query($sql4); if($result4){ session_register("uid"); session_register("uname"); session_register("ulevel"); $_SESSION["uid"] = $uid; $_SESSION["uname"] = $fname; $_SESSION["ufullname"] = $fname . " " .$lname; $_SESSION["urole"] = $role; $home = get_option('home'); if(isset($_POST['remember'])) { setcookie("remembered[username]", $username, time() + 86400 * 365 * 2); setcookie("remembered[password]", $psw, time() + 86400 * 365 * 2); } if(!empty($redirect)) { header( 'Location: '. $redirect ) ; exit(); } else { header( $home ) ; exit(); } } } else { echo "<div class=\"error rounded5 shadow\">Invalid username or password!</div>"; } } } ?> </div><!-- / login-results --> <div id="login" class="rounded5 shadow"> <form name="loginform" id="loginform" action="<?php $_SERVER['PHP_SELF']; ?>" method="post"> <p> <label for="username">Username<br> <input type="text" name="username" id="username" class="rounded5" value="<?php echo $username_p; ?>" size="20" tabindex="10" /></label> </p> <p> <label for="password">Password<br> <input type="password" name="password" id="password" class="rounded5" value="<?php echo $password_p; ?>" size="20" tabindex="20" /></label> </p> <p class="submit"> Keep me logged in<input type="checkbox" name="remember" id="remember" /><br /><br /><a href="" class="left">Lost your password?</a> <input type="submit" name="admin_login" id="admin_login" class="btn rounded10 right" value="Log In" tabindex="100" /> </p> <div class="cleaner"></div><!-- / cleaner --> </form> </div><!-- / login--> <?php get_footer('login'); ?>
Finally here is the code I am using for the logout.php page.
<?php session_start(); include ('functions.php'); connect(); $uid = mysql_real_escape_string($_SESSION['uid']); $sql = "UPDATE usersT SET status = '0' WHERE uid = '$uid'"; $result = mysql_query($sql); if($result) { session_unset(); session_destroy(); if(isset($_COOKIE['remembered'])){ setcookie("remembered[username]", $username, time() - 3600); setcookie("remembered[password]", $psw, time() - 3600); header("location: login.php"); } exit(); } else { echo "You couldn't be logged out at this time."; } ?>
-
Bump!
-
I am using this jquery slider. (http://css-tricks.com/examples/StartStopSlider/ )
I was wondering how I would go about adding left and right arrows to control the slides and possibly pagination. Is this possible with the following code? Can anyone point me in the right directions, because my Javascript is limited.
Javascript
// SET THIS VARIABLE FOR DELAY, 1000 = 1 SECOND var delayLength = 5000; function doMove(panelWidth, tooFar) { var leftValue = $("#inner-slider-mover").css("left"); // Fix for IE if (leftValue == "auto") { leftValue = 0; }; var movement = parseFloat(leftValue, 10) - panelWidth; if (movement == tooFar) { $(".slide img").animate({ "top": -270 }, function() { $("#inner-slider-mover").animate({ "left": 0 }, function() { $(".slide img").animate({ "top": 0 }); }); }); } else { $(".slide img").animate({ "top": -270 }, function() { $("#inner-slider-mover").animate({ "left": movement }, function() { $(".slide img").animate({ "top": 0 }); }); }); } } $(function(){ var $slide1 = $("#slide-1"); var panelWidth = $slide1.css("width"); var panelPaddingLeft = $slide1.css("paddingLeft"); var panelPaddingRight = $slide1.css("paddingRight"); panelWidth = parseFloat(panelWidth, 10); panelPaddingLeft = parseFloat(panelPaddingLeft, 10); panelPaddingRight = parseFloat(panelPaddingRight, 10); panelWidth = panelWidth + panelPaddingLeft + panelPaddingRight; var numPanels = $(".slide").length; var tooFar = -(panelWidth * numPanels); var totalMoverwidth = numPanels * panelWidth; $("#inner-slider-mover").css("width", totalMoverwidth); sliderIntervalID = setInterval(function(){ doMove(panelWidth, tooFar); }, delayLength); });
Html
<div id="outer-slider"> <div id="inner-slider-left"> <a href="#" class="left-arrow">Left</a> </div><!-- / inner-slider-left --> <div id="inner-slider-mid"> <div id="inner-slider-mover"> <div id="slide-1" class="slide"> <div class="inner-slider-info"> <h1>The boys are back in town.</h1> <p>Donec gravida posuere arcu. Nulla facilisi. Phasellus imperdiet. Vestibulum at metus. Integer euismod. Nullam placerat rhoncus sapien. Ut euismod. Praesent libero. Morbi pellentesque libero sit amet ante. Maecenas tellus.</p> </div><!-- / inner-slider-info --> <a href="#"><img src="images/slide-1.jpg" alt="The boys are back in town." /></a> </div><!-- / slide-1 --> <div class="slide"> <div class="inner-slider-info"> <h1>Test 2.</h1> <p>Donec gravida posuere arcu. Nulla facilisi. Phasellus imperdiet. Vestibulum at metus. Integer euismod. Nullam placerat rhoncus sapien. Ut euismod. Praesent libero. Morbi pellentesque libero sit amet ante. Maecenas tellus.</p> </div><!-- / inner-slider-info --> <a href="#"><img src="images/slide-2.jpg" alt="The boys are back in town." /></a> </div><!-- / slide-2 --> <div class="slide"> <div class="inner-slider-info"> <h1>Test 3.</h1> <p>Donec gravida posuere arcu. Nulla facilisi. Phasellus imperdiet. Vestibulum at metus. Integer euismod. Nullam placerat rhoncus sapien. Ut euismod. Praesent libero. Morbi pellentesque libero sit amet ante. Maecenas tellus.</p> </div><!-- / inner-slider-info --> <a href="#"><img src="images/slide-2.jpg" alt="The boys are back in town." /></a> </div><!-- / slide-2 --> </div><!-- / inner-slider-mover --> </div><!-- / inner-slider-mid --> <div id="inner-slider-right"> <a href="#" class="right-arrow">Right</a> </div><!-- / inner-slider-right --> </div><!-- / outer-slider -->
CSS
.left-arrow, .right-arrow { width:34px; height:34px; position:relative; display:block; text-indent:-9999px; border:0; } .left-arrow { background:url(images/left-arrow-bg.jpg) 0 0 no-repeat; } .right-arrow { background:url(images/right-arrow-bg.jpg) 0 0 no-repeat; } #inner-slider-mover { width: 2502px; position: relative; } .inner-slider-info { width:240px; height:210px; position:relative; float:left; background-color:#29aae3; padding:30px; } .inner-slider-info p { font-family:Arial, Helvetica, sans-serif; font-size:14px; color:#fff; margin:0 0 15px 0; padding:0; } .slide { width:834px; height:270px; float: left; position: relative; } .slide img { position: absolute; top: 0; left: 300px; }
-
I have tried your solution of passing strung data and I'm still not getting any error messages, doesn't save the data in the database. The form is definitely submitting but nothing is being return. Any other ideas? Yes my .php file is in the same directory.
-
Thanks for the information about PHP_SELF vulnerability, didn't realize. How would I go about troubleshooting the send_form function? What do you recommend?
-
After adding an alert instead of a function to " $('#addclientform').submit(function() { " I have realised that this is not the issue. So my next guess is that the problem lys within the post data function but I can't seem to figure it out. Anyone?
-
I am trying to design a simple ajax form so I can learn ajax. I know the php page I have written works so the issue is definitely with the ajax. I think the problem is with the .submit any ideas? At the moment its not reaching the php page.
<script type="text/javascript"> $(document).ready(function() { //when submitting form $('#addclientform').submit(function() { send_form(); }); }); //function to sent the form function send_form(){ //get the client name and code var clientname = $('#clientname').val(); var clientcode = $('#clientcode').val(); //use ajax to run the check $.post("add-client-info.php", { clientname: clientname, clientcode : clientcode }, function(result){ //if the result is 1 if(result == 1){ //show that the username is available $('#addclient').html('<h1 class="success">' +clientname + ' has been added to the list.</h1>'); } else if(result == 2){ //show that the username is NOT available $('#addclient').html('<h1 class="fail">Please make sure you add both a client name and a client code.</h1>'); } else if(result == 3){ //show that the username is NOT available $('#addclient').html('<h1 class="fail">' +clientname + ' has not been added to the list.</h1>'); } }); } </script>
The form
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" id="addclientform"> <input type="submit" name="addclientbtn" id="addclientbtn" class="addclientbtn" value="Add Client" /> <div id="client-code-wrap"> <div id="client-code"><input type='text' id='clientcode' name='clientcode' class="client-input" value="Client Code" onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /></div> <div id='client-code-result'></div> </div> <div id="client-name-wrap"> <div id="client-name"><input type='text' id='clientname' name='clientname' class="client-input" value="Client Name" onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /></div> <div id='client-name-result'></div> </div> </form>
-
How embarrassing! Html error!
-
Can anyone see any reason why the inputs in this form do not save any values entered into the database. Yet the selects and textareas do!
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Job Sheet</title> <link rel="stylesheet" type="text/css" href="styles.css"> </head> <body> <?php if(isset($_POST['submitform'])) { include('config-jobs.php'); //Parse & Escape form values $jobclient = mysql_real_escape_string(trim($_POST['jobclient'])); $jobbriefedby = mysql_real_escape_string(trim($_POST['jobbriefedby'])); $jobmanager = mysql_real_escape_string(trim($_POST['jobmanager'])); $jobdesc = mysql_real_escape_string(trim($_POST['jobdesc'])); $jobdate = mysql_real_escape_string(trim($_POST['jobdate'])); $joblocation = mysql_real_escape_string(trim($_POST['joblocation'])); $jobbrief = mysql_real_escape_string(trim($_POST['jobbrief'])); $jobassets = mysql_real_escape_string(trim($_POST['jobassets'])); //Create & Run insert query $query = "INSERT INTO jobnumbers (jobclient, jobbriefedby, jobmanager, jobdesc, jobdate, joblocation, jobbrief, jobassets) VALUES ('$jobclient', '$jobbriefedby', '$jobmanager', '$jobdesc', '$jobdate', '$joblocation', '$jobbrief', '$jobassets')"; $result = mysql_query($query); if (!$result) { //Query failed, display error echo"Error: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n"; } else { //Display job id of inserted record. $id = mysql_insert_id(); $jobid = str_pad($id, 6, '0', STR_PAD_LEFT); $jobnum = "{$jobclient}-{$jobid}"; $query2 = "UPDATE jobnumbers SET jobnum = '$jobnum' WHERE jobid = '$id'"; $result2 = mysql_query($query2); if(!result2) { //Query failed, display error echo"Error 2: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n"; }else{ echo"A new job has been added to the system. The ref number is $jobnum."; } } } ?> <div id="job-sheet"> <div id="job-sheet-inner"> <h1 class="job-sheet-h1">Job Sheet</h1> <h2 class="job-sheet-h2">No work will be accepted without a completed job sheet</h2> <form action="#" method="post"> <table cellpadding="0" cellspacing="0"> <tr> <td class="job-sheet-td-left">Client:</td> <td class="job-sheet-td-right"> <select id="jobclient" name="jobclient" class="job-sheet-select"> <?php include('config-jobs.php'); // Retrieve data from database $sql=" SELECT * FROM clients ORDER BY client_name ASC "; $result=mysql_query($sql); // Start looping rows in mysql database. while($rows=mysql_fetch_array($result)){ ?> <option value="<?php echo $rows['client_code']; ?>"><?php echo $rows['client_name']; ?></option> <?php // close while loop } // close connection mysql_close(); ?> </select> </td> </tr> <tr> <td class="job-sheet-td-left">Briefed By:</td> <td class="job-sheet-td-right"> <select id="jobbriefedby" name="jobbriefedby" class="job-sheet-select"> <?php include('config-clients.php'); // Retrieve data from database $sql=" SELECT staff_name FROM staff_list ORDER BY staff_name ASC "; $result=mysql_query($sql); // Start looping rows in mysql database. while($rows=mysql_fetch_array($result)){ ?> <option><?php echo $rows['staff_name']; ?></option> <?php // close while loop } // close connection mysql_close(); ?> </select> </td> </tr> <tr> <td class="job-sheet-td-left">Account Manager:</td> <td class="job-sheet-td-right"> <select id="jobmanager" name="jobmanager" class="job-sheet-select"> <?php include('config-clients.php'); // Retrieve data from database $sql=" SELECT staff_name FROM staff_list ORDER BY staff_name ASC "; $result=mysql_query($sql); // Start looping rows in mysql database. while($rows=mysql_fetch_array($result)){ ?> <option><?php echo $rows['staff_name']; ?></option> <?php // close while loop } // close connection mysql_close(); ?> </select> </td> </tr> <tr> <td class="job-sheet-td-left">Job Description:</td> <td class="job-sheet-td-right"><input type="text" id="jobdesc" name"jobdesc" class="job-sheet-input" value="Test Description" /></td> </tr> <tr> <td class="job-sheet-td-left">Date Required:</td> <td class="job-sheet-td-right"><input type="text" id="jobdate" name"jobdate" class="job-sheet-input" value="Test Date" /></td> </tr> <tr> <td class="job-sheet-td-left">Assets Located:</td> <td class="job-sheet-td-right"><input type="text" id="joblocation" name"joblocation" class="job-sheet-input " value="digital/kisumu/images/spring-summer-images" /></td> </tr> <tr> <td class="job-sheet-td-top" colspan="2">Brief:</td> </tr> <tr> <td class="job-sheet-td-bot" colspan="2"><textarea id="jobbrief" name="jobbrief" class="job-sheet-text" >Include as much information as possible</textarea></td> </tr> <tr> <td class="job-sheet-td-top" colspan="2">Assets Required:</td> </tr> <tr> <td class="job-sheet-td-bot" colspan="2"><textarea id="jobassets" name="jobassets" class="job-sheet-text">Include details of any logos, fonts, images, colours etc.</textarea></td> </tr> <tr> <td class="job-sheet-bot" colspan="2"><input type="submit" id="submitform" name="submitform" class="job-sheet-submit" value="Submit Job" /></td> </tr> </table> </form> </div><!-- /job-sheet-inner --> </div><!-- /job-sheet --> </body> </html>
-
Its fine i have cracked the problem. Thanks
-
Ok I have been trying to get learn ajax and php contact forms for a few days now and I can't seem to get my head around why mine refuses to work. I know the form and the php does what it is suppost to do but I can't understand why when i link the whole thing together it doesn't work. As it sits at the moment its just sticking on the loading part of the ajax and refuses to execute the validate in the php file. Can anyone please point me in the right direction?
index.php (the form)
<div id="contact_wrapper"> <div id="contact_form"> <script src="js/ajax.form.js" language="javascript"></script> <form action="javascript:contact_form()" method="post"> <h1 class='contact_form_h' id='contact-loading'>Contact Us</h1> <div id="login_response"></div> <input type='text' name='name' id='name' class='contact_form_input' value='Name' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /> <input type='text' name='email' id='email' class='contact_form_input' value='Email' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /> <textarea name='enquiry' id='enquiry' class='contact_form_textarea' rows='10' cols='10' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;">Enquiry</textarea> <input type='submit' name='contact' id='contact' class='contact_form_submit' value='Contact Us' /> </form> </div> </div>
ajax.form.js (Ajax)
/* ---------------------------- */ /* XMLHTTPRequest Enable */ /* ---------------------------- */ function createObject() { var request_type; var browser = navigator.appName; if(browser == "Microsoft Internet Explorer"){ request_type = new ActiveXObject("Microsoft.XMLHTTP"); }else{ request_type = new XMLHttpRequest(); } return request_type; } var http = createObject(); /* -------------------------- */ /* Contact */ /* -------------------------- */ /* Required: var nocache is a random number to add to request. This value solve an Internet Explorer cache issue */ var nocache = 0; function contact_form() { // Optional: Show a waiting message in the layer with ID ajax_response document.getElementById('contact_wrapper').innerHTML = "<div id='contact_error'><img src='img/loader.gif' alt='loading' /> Loading...</div>" // Required: verify that all fileds are not empty. Use encodeURI() to solve some issues about character encoding. var name = encodeURI(document.getElementById('name').value); var email = encodeURI(document.getElementById('email').value); var enquiry = encodeURI(document.getElementById('enquiry').value); // Set the random number to add to URL request nocache = Math.random(); // Pass the form variables like URL variable http.open('get', 'send_email.php?name='+name+'&email='+email+'&enquiry='+enquiry+'&nocache = '+nocache); http.onreadystatechange = Reply; http.send(null); } function Reply() { if(http.readyState == 4){ var response = http.responseText; if(response == 1){ // if fields are empty document.getElementById('login_response').innerHTML = 'Please fill in all the fields.'; } else if(response == 2){ // if email isnt valid document.getElementById('login_response').innerHTML = 'Please enter a valid email address.'; } else if(response == 3){ // if email has been sent document.getElementById('login_response').innerHTML = 'Your email has been sent.'; } else if(response == 10){ // if email hasnt been sent document.getElementById('login_response').innerHTML = 'Your email has not been sent.'; } } }
send_email.php (php)
<?php //Require check email function require "check_email.php"; //Variables $err_name=stripslashes($_GET['name']); $err_email=stripslashes($_GET['email']); $err_enquiry=stripslashes($_GET['enquiry']); $to="xxx@xxxxxxxxxx.com"; $subject="Website Contact Form"; $from = stripslashes($_GET['name'])."<".stripslashes($_GET['email']).">"; $message = $err_enquiry; $headers = "From: $from\r\n" . "MIME-Version: 1.0\r\n" . "Content-Type: multipart/mixed;\r\n" . " boundary=\"{$mime_boundary}\""; //Check all form fields are filled in if ($_GET["name"]!='' && $_GET["name"]!='Name' && $_GET["email"]!='' && $_GET["email"]!='Email' && $_GET["enquiry"]!='' && $_GET["enquiry"]!='Enquiry') { //Check email address is valid if (isValidEmail($_GET['email'])){ //Send Mail if (@mail($to, $subject, $message, $headers)) { echo "3"; } else{ echo "10"; } } //Email isnt valid else{ echo"2"; } } else { echo"1"; } ?>
-
Yea it is definitely passing the php the correct values. The php is definitely doing its part so maybe its an issue with the reply function but i'm not sure what.
-
Not quite sure what you mean.
-
Yea I noticed that as I posted it. I currently have this.
<?php //Check if form is submitted //Require check email function require "check_email.php"; //Variables $err_name=stripslashes($_GET['name']); $err_email=stripslashes($_GET['email']); $err_enquiry=stripslashes($_GET['enquiry']); $to="andrew@peppermintdigital.com"; $subject="Website Contact Form"; $from = stripslashes($_GET['name'])."<".stripslashes($_GET['email']).">"; $message = $err_enquiry; $headers = "From: $from\r\n" . "MIME-Version: 1.0\r\n" . "Content-Type: multipart/mixed;\r\n" . " boundary=\"{$mime_boundary}\""; //Check all form fields are filled in if ($_GET["name"]!='' && $_GET["name"]!='Name' && $_GET["email"]!='' && $_GET["email"]!='Email' && $_GET["enquiry"]!='' && $_GET["enquiry"]!='Enquiry' ) { if (isValidEmail($_GET['email'])){ //Send Mail if (@mail($to, $subject, $message, $headers)) { echo "3"; } else{ echo "2"; } } else { echo "1"; } } else { echo"0"; } ?>
The problem I am having now is with the ajax part of the form. I have tested the php and it now works but when the form is hooked up by ajax its getting stuck.
Here is the ajax part of the form which alway echo's the please fill in all form fields error.
/* ---------------------------- */ /* XMLHTTPRequest Enable */ /* ---------------------------- */ function createObject() { var request_type; var browser = navigator.appName; if(browser == "Microsoft Internet Explorer"){ request_type = new ActiveXObject("Microsoft.XMLHTTP"); }else{ request_type = new XMLHttpRequest(); } return request_type; } var http = createObject(); /* -------------------------- */ /* LOGIN */ /* -------------------------- */ /* Required: var nocache is a random number to add to request. This value solve an Internet Explorer cache issue */ var nocache = 0; function send_email() { // Optional: Show a waiting message in the layer with ID ajax_response document.getElementById('login_response').innerHTML = "Loading..." // Required: verify that all fileds is not empty. Use encodeURI() to solve some issues about character encoding. var name = encodeURI(document.getElementById('name').value); var email = encodeURI(document.getElementById('email').value); var enquiry = encodeURI(document.getElementById('enquiry').value); // Set te random number to add to URL request nocache = Math.random(); // Pass the login variables like URL variable http.open('get', 'send_email.php?name='+name+'&email='+email+'&enquiry='+enquiry+'&nocache = '+nocache); http.onreadystatechange = Reply; http.send(null); } function Reply() { if(http.readyState == 4){ var response = http.responseText; if(response == 0){ // if fields are empty document.getElementById('login_response').innerHTML = 'Please fill in all the fields.'; } else if(response == 1){ // if email isnt valid document.getElementById('login_response').innerHTML = 'Please enter a valid email address.'; } else if(response == 2){ // if email has been sent document.getElementById('login_response').innerHTML = 'Your email has been sent.'; } else if(response == 3){ // if email hasnt been sent document.getElementById('login_response').innerHTML = 'Your email has not been sent.'; } } }
-
Post your code inside code brackets. It makes it easier to read and you have more chance or a response.
-
Ok this should be straight forward but my tired brain cannot work this out. I'm creating a simple contact form and for some reason the validation isn't working. It wont go past the check fields are filled in validation. Can anyone spot what I'm doing wrong?
<form method="get"> <h1 class='contact_form_h'>Contact Us</h1> <div id="login_response"></div> <input type='text' name='name' id='name' class='contact_form_input' value='Name' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /> <input type='text' name='email' id='email' class='contact_form_input' value='Email' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;" /> <textarea name='enquiry' id='enquiry' class='contact_form_textarea' rows='10' cols='10' onfocus="if(!this._haschanged){this.value=''};this._haschanged=true;">Enquiry</textarea> <input type='submit' name='contact' id='contact' class='contact_form_submit' value='Contact Us' /> </form>
<?php //Check if form is submitted if(isset($_GET['contact'])) { //Require check email function require "check_email.php"; //Variables $err_name=stripslashes($_GET['name']); $err_email=stripslashes($_GET['email']); $err_enquiry=stripslashes($_GET['enquiry']); $to="xxx@xxxx.com"; $subject="Website Contact Form"; $from = stripslashes($_GET['name'])."<".stripslashes($_GET['email']).">"; $message = $err_enquiry; $headers = "From: $from\r\n" . "MIME-Version: 1.0\r\n" . "Content-Type: multipart/mixed;\r\n" . " boundary=\"{$mime_boundary}\""; //Check all form fields are filled in if ($_GET["name"]!='' OR $_GET["name"]!='Name' OR $_GET["email"]!='' OR $_GET["email"]!='Email' ) { if (isValidEmail($_GET['email'])){ //Send Mail if (@mail($to, $subject, $message, $headers)) { echo "3 - sent"; } else{ echo "2 - not"; } } else { echo "1 - not valid"; } } else { echo"0 - Fill in"; } } ?>
Below is the check email script.
<?php // This function tests whether the email address is valid function isValidEmail($email){ $pattern = "^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$"; if (eregi($pattern, $email)){ return true; } else { return false; } } ?>
-
Ok I have had a look at this today with a fresh head and this is what I have come up with which will suit my needs.
<?php if(isset($_POST['submit'])) { // Connect to server and select database. $host = ""; // Host name $username = ""; // Mysql username $password = ""; // Mysql password $db_name = ""; // Database name mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); //Parse & escape POSTed values $category = mysql_real_escape_string(trim($_POST['category'])); $name = mysql_real_escape_string(trim($_POST['name'])); //Create & Run insert query $query = "INSERT INTO jobnumbers (category, name) VALUES ('$category', '$name')"; $result = mysql_query($query); if (!$result) { //Query failed, display error echo"Error: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n"; } else { //Display job id of inserted record. $id = mysql_insert_id(); $jobid = str_pad($id, 6, '0', STR_PAD_LEFT); $cat = htmlspecialchars((trim($_POST['category']))); $jobnum = "{$cat}-{$jobid}"; //Create & Run insert query $query2 = "INSERT INTO jobnumbers (jobnumber) VALUES ('$jobnum') WHERE jobid='$id' "; $result2 = mysql_query($query2); if (!$result2) { //Query failed, display error echo"Error 2: <br />\n" . mysql_error() . "<br />\nQuery:<br />\n{$query}\n"; } else { echo"Your job number for $name is $jobnum."; } } } ?>
I am having a problem with the second sql statement and I am getting the following error.
Error 2:
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'WHERE jobid='11'' at line 1
Query:
INSERT INTO jobnumbers (category, name) VALUES ('Web', '1234')
Any ideas?
-
I tried a different way of doing it which was to select the last auto increment number and add one to it to create the job number but it hasn't worked. I am going in the right direction with this?
<?php $host=""; // Host name $username=""; // Mysql username $password=""; // Mysql password $db_name=""; // Database name // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); $category = $_POST['category']; $name = $_POST['project-name']; if(isset($_POST['submit'])) { $sql = " SELECT job-id FROM job-numbers ORDER BY job-id DESC LIMIT 1 "; $result = mysql_query($sql); $rows = mysql_num_rows($result); $jobid = $rows['job-id']; $jobnumber = $jobid++; $newjobid = str_pad($jobnumber, 6, '0', STR_PAD_LEFT); $sql2="INSERT INTO job-numbers (category, name , jobnumber) VALUES ('$category', '$name', '$newjobid' )"; $result2=mysql_query($sql2); if ($result2) { echo"worked"; } else { echo"hasnt"; } } else {} ?> <form action="#" method="post"> <select id="category" name="category"> <option>Web</option> <option>Print</option> </select> <input type="text" name="project-name" id="project-name" /> <input type="submit" name="submit" id="submit" /> </form>
-
Ok I understand how padding works now but im failing to see how to link everything together to get my desired effect. Could you give me an example of the sql statement I would need to use?
Thank you for you help.
Securely storing passwords
in PHP Coding Help
Posted
I have recently done a lot of research on this topic myself as I am currently creating a custom CMS. I came across this useful link ( http://sunnyis.me/blog/secure-passwords/ ) which explains how to use the Phpass framework easily. It helped me lots, so hopefully you will find it useful as well.