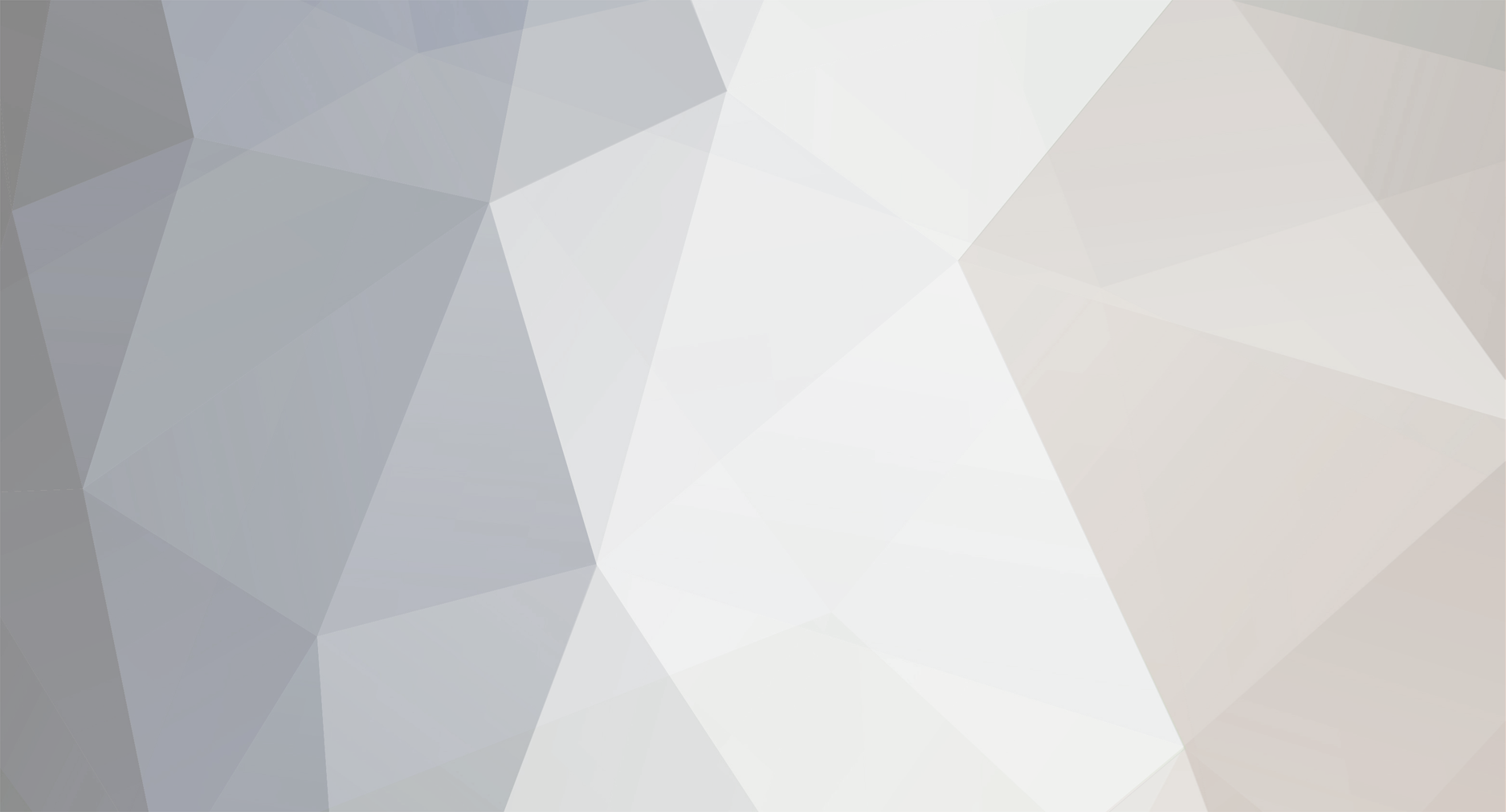
hansford
-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Posts posted by hansford
-
-
Check the url again: http://3elementsreview.com/blog/wp-content/themes/3elements/php-signup/sign-up-complete.php
make sure the url and page name are correct - check the file names.
-
First things first - you must first understand the fundamentals of programming - then you can pick up on any language even if you don't know it and figure things out.
if(is_wc_endpoint_url("order-received")) { echo "empty no text"; } else { echo "the below code"; }
That is an if/else statement. The argument in the "IF" clause is calling a function - wc_endpoint_url - that function is suppose to return true or false based on the argument it was passed which is "order-received". My only answer based on the information you gave is - check that function - is it returning anything at all, is it returning what it should be - true or false.
-
On the second page - you need to fill out form values so on page 3 those values are available in the $_POST array. You can do this through hidden form fields so the user doesn't see them.
-
Also, if you're returning results (plural) you need to return an array to ajax so that's why I used the dataType "json" in the ajax call.
The php would need to return the results as a json array like so.
<?php $args = array('result1','result2','result3'); echo json_encode($args);
-
I had to change a few things to get the code working on my system, so incorporate what you need into your current code.
<?php session_start(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <meta http-equiv="content-type" content="text/html; charset=UTF-8" /> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js" type="text/javascript"></script> <style type="text/css"> body{ font-family:Tahoma, Geneva, sans-serif; font-size:18px; } .content{ width:auto; margin:0 auto; } #searchid { width:200px; font-size:14px; } #result { position:absolute; width:200px; display:block; margin-top:-1px; border-top:0px; border:1px #CCC solid; background-color: white; z-index:1; } .show { border-bottom:1px #999 dashed; font-size:14px; padding:4px; width:auto; } .show:hover { background:#4c66a4; color:#FFF; cursor:pointer; } .selected-hint { background-color:#E2E1E1; } </style> <head> <link rel="stylesheet" type="text/css" href="css/jquery-ui.css"> <script type="text/javascript"> $(function(){ $(".search").keyup(function(e) { // don't want keyup to fire if we are scrolling if(e.which == 38 || e.which == 40) { return false; } var searchid = $(this).val(); var dataString = 'search='+ searchid; if(searchid!='') { $.ajax({ type: "POST", url: "search.php", dataType: "json", data: dataString, cache: false, success: function(html) { for(var i in html) { $("#result").append('<div>' + html[i] + '<div>'); } $("#result").show(); } }); }return false; }).keydown(function(e) { // check if result div is visible var visible = $('#result').is(':visible'); var key = e.which; // down arrow if(key == 40) { if(visible) { if($('#result div.selected-hint').length == 0) { var child = $('#result div:nth-child(1)'); child.addClass('selected-hint'); } else { if($('#result div.selected-hint').next('div').length) { $('#result div.selected-hint').removeClass('selected-hint').next('div').addClass('selected-hint'); } else { $('#result div.selected-hint').removeClass('selected-hint'); } } } } // up arrow if(key == 38) { if(visible) { if($('#result div.selected-hint').prev('div').length) { $('#result div.selected-hint').removeClass('selected-hint').prev('div').addClass('selected-hint'); } else { $('#result div.selected-hint').removeClass('selected-hint'); } } } }); jQuery("#result").live("click",function(e){ var $clicked = $(e.target); var $name = $clicked.find('.name').html(); var decoded = $("<div/>").html($name).text(); $('#searchid').val(decoded); var yourLinkHref= $(this).attr('href'); //window.location = "mainpage.php?TopicName=" + $name; }); jQuery(document).live("click", function(e) { var $clicked = $(e.target); if (! $clicked.hasClass("search")){ jQuery("#result").fadeOut(); } }); $('#searchid').click(function(){ jQuery("#result").fadeIn(); }); }); </script> </head> <div id="pagewrap"> <!---Header---> <div id="header"> <div align="right">Welcome <?php if(isset($_SESSION['MM_Username'])) { echo $_SESSION['MM_Username']; } ?> </div> <img src="images/HomeBackgound.jpg" alt="" width="100%" height="50" /> <!--Autocomplete--> <div class="content"> <input type="text" class="search" id="searchid" placeholder="Search For Topics" /> <div id="result"> </div> </div>
-
Post the associated html so we can see what's going wrong.
-
Just my opinion, others may feel different, but yes. I've went down the same road and it takes a lot to accomplish anything.
Maintenance was suppose to be easier yadi yadi - but because the code is so deep - it's not easier. The more time you think about writing perfectly uncoupled code the less time you spend being productive and actually writing code. Maybe the guru's have this all figured out and one day we may too, but for now - we need to write the best code we can without losing productivity.
-
If it's possible for the client to change the value - and how are they doing this? Anyways, it won't matter if they set it to a million clicks if each click is sent to the server and the server only registers one click per execution.
$('#button').click(function() { $.ajax({ url: 'click.php', type: 'POST', data: '&click=1', dataType: 'text', success: function($data) { $('#success').html('click added to database'); }, error: function($data) { $('#success').html('error'); } });
On the server
if(isset($_POST['click'])) { //no need to check post value as we //are only updating click by 1 //update user clicks in database by 1 echo 'click added!'; }
-
Where is the id coming from? (surplus_id) If the id is auto generated then you don't need to insert it.
Try using error reporting at the top of the php page to see if any
errors are generated during the transaction.
error_reporting(E_ALL); ini_set('display_errors',1);
Check if the rows were actually inserted by using something like:
printf("Rows updated: %d\n", mysql_affected_rows());
You should also look into using PDO for your database interactions to prevent sql injection attacks.
-
$msg_to_user = "";
When the page is first loaded, this variable remains empty.
On the second page load, this variable gets set - or should.
Thus we should be checking not for:
<?php if($msg_to_user !=''){ ?>
but for:
<?php if($msg_to_user == ''){ ?>
which would insert the name and address boxes into the form.
You can also set flags (variables of either true of false) for each section of code and then check that value before displaying that particular html section.
-
If you're trying to get the name value pairs here is a way to accomplish that.
// A list to be populated with the "key=value" pairs you want in your WHERE clause. $fields = array(); // Loop through the keys and add the field to the list if needed. foreach ($_POST as $name => $value) { if (!empty($value)) { $fields[] = sprintf("'%s' = '%s'", $name, $value); } }
-
You don't need to explicitly cast variables to new data types in PHP as it's done automatically.
Here is a link to the page in the manual: http://www.php.net/manual/en/language.types.type-juggling.php
-
Use a little jQuery to change the action attribute of the form upon submit.
$(document).ready(function() { if($('#age_form').length > 0) { $('#age_form').submit(function() { if($('#youngster:checked').val() == true) { $('#age_form').attr('action','http://127.0.0.1/test_page_3.php'); } else { $('#age_form').attr('action','http://127.0.0.1/test_page_2.php'); } }); } });
Don't forget to add jQuery link in the head of your form:
<head> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> </head>
Here is a sample form:
<form id="age_form" method="post" action=""> Under 19 years of age: <input type="radio" name="age_restriction" id="youngster" value="young" /><br /> 19 years of age or older: <input type="radio" name="age_restriction" value="old" checked /><br /> <input type="submit" value="submit"/> </form>
-
Added some additions.
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="refresh" content="5;url=entries.php"> </head> <body> <text><red><large><i>Processing may take up to five seconds. You will be redirected shortly.</i></large></red></text><p> <div style="display:block;"> <img src="base.gif" width="10" height="36"/> <img src="base2.gif" width="10" height="36"/> <img src="base3.gif" width="10" height="36"/> <img src="base4.gif" width="10" height="36"/> <img src="base5.gif" width="10" height="36"/> <img src="base6.gif" width="10" height="36"/> <?php $pic = array('50.gif','100.gif','200.gif','250.gif','150.gif','300.gif'); shuffle($pic); ?> <?php for( $i = 0; $i < 1; $i++) echo "<li style=\"display: inline;\"> <img src=\"$pic[$i]\" width=\"27\" height=\"36\"> </li>"; ?> </div> </body> </html>
-
Not quite sure of what you want - no code posted. But here's a little something that may/may not help.
$query = "SELECT * FROM table_name"; try { $con = new PDO('mysql:host=localhost;dbname=your_db_name', $username, $password); $con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $result = $con->prepare($query); $result->execute(); } catch(PDOException $e) { echo $e->getMessage(); } $table = "<table cellpadding=10 cellspacing=0 border=1>"; $table .= "<tr>"; $table .= "<th>Name</th><th>Contact</th><th>Code</th><th>Type</th><th>Quanitiy</th>"; $table .= "</tr>"; while($row = $result->fetch(PDO::FETCH_ASSOC)) { $table .= "<tr>"; $table .= "<td>" . $row['Name'] . "</td>"; $table .= "<td>" . $row['Contact'] . "</td>"; $table .= "<td>" . $row['Code'] . "</td>"; $table .= "<td>" . $row['Type'] . "</td>"; $table .= "<td>" . $row['Quanity'] . "</td>"; $table .= "</tr>"; } $table .= "</table>";
-
As was mentioned by another member, this code is not right.
<?php while ($line = mysql_fetch_array($result, MYSQL_ASSOC)) { ?> <option value="<php echo $line['table_number'];?>"> <?php echo $line['main_theme'];?> </option> <?php } ?>
Should be something like:
<?php while ($line = mysql_fetch_array($result, MYSQL_ASSOC)) { ?> <option value="<?php echo $line['table_number']; ?>"> <?php echo $line['main_theme'];?></option> <?php } ?>
-
This section of code - is 'value' a column in your database table - if not it has to be.
// Insert data into mysql $sql="INSERT INTO $tbl_name(value) VALUES('$select')"; $result=mysql_query($sql);
Also, you will get more help if you post the code instead of attaching files. Post the code in the flow order - meaning, page 1 with any forms that calls another page and so on. Post any error msgs you are getting. Post a better table layout - table name, column1, column2 etc
-
make sure you have display errors on at the top of your php pages during debugging - then remove for production environment
error_reporting(E_ALL);
ini_set('display_errors', '1');
I'll look at your code.
-
Yeah, I like to store in non-html chars. This code will remove the last span tag if it's not necessary.
//keep all paths with '/' symbol - even the last one $str = 'path1/path2/path3/path4'; $path = explode('/',$str); //remove last array element with / symbol and no path array_pop($path); $size = count($path); for($i = 0; $i < $size; $i++) { //if on the first and last array element - don't add > symbol and break loop if($size == 1) { $link = htmlentities('<a href="/Topics">' . $path[$i] . '</a>'); $path[$i] = $link; break; } //if on the last array element - don't add > symbol if($i == $size - 1) { $link = '<span class="navHere"><b>' . $path[$i] . '</b></span>'; } else { //else add > symbol $link = '<a href="/Topics">' . $path[$i] . '</a> > '; } $path[$i] = $link; } //no need to join with > symbol echo join($path, '');
-
$str = 'path1/path2/path3';
$path = explode('/',$str);
$size = count($path);
for($i = 0; $i < $size; $i++) {
if($i == $size - 1) {
$link = htmlentities('<span class="navHere"><b>' . $path[$i] . '</b></span>');
}
else {
$link = htmlentities('<a href="/Topics">' . $path[$i] . '</a>');
}
$path[$i] = $link;
}
echo join($path, ' > '); -
Problem appears in this section where hours is listed twice instead of minutes.
this_sel.find('.days').text(days); this_sel.find('.hours').text(hours); this_sel.find('.mins').text(hours); this_sel.find('.secs').text(seconds);
should be:
this_sel.find('.days').text(days); this_sel.find('.hours').text(hours); this_sel.find('.mins').text(minutes); this_sel.find('.secs').text(seconds);
-
I assume you're using WordPress. IMO - I would just let that file grow as needed. However, on the functions.php page you could group similar functions together and place a text header at the top of each section so you can quickly drill down to each section performing a search for the header text.
-
I agree with the Guru member - you need to learn the basics of programming in general and then PHP. I ran your code and there are multiple issues. You are not accessing the database columns properly for one:
$cats[] = $cat
should be:
$cats[] = $cat['some_column_in_table']
Also, you don't use opening and closing braces properly or return values on several functions.
function list() { $q="SELECT * FROM categories ORDER BY name"; $result=mysql_query($q); $cats=array(); while($cat=mysql_fetch_array($result)) $cats[]=$cat; return $cats; }
should be more along the lines of this:
function list() { $q="SELECT * FROM categories ORDER BY name"; $result=mysql_query($q); $cats=array(); while($cat=mysql_fetch_array($result)) { $cats[]=$cat['some_column_in_table']; } return $cats; }
You should also be using PDO to access the database as your code has been deprecated and will not work in PHP future releases.
-
Don't have time to write the code for you, but look at the ftp functions: ftp_fget and ftp_fput.
php get current time
in PHP Coding Help
Posted
Try - date_default_timezone_set() function.
http://php.net/manual/en/function.date-default-timezone-set.php