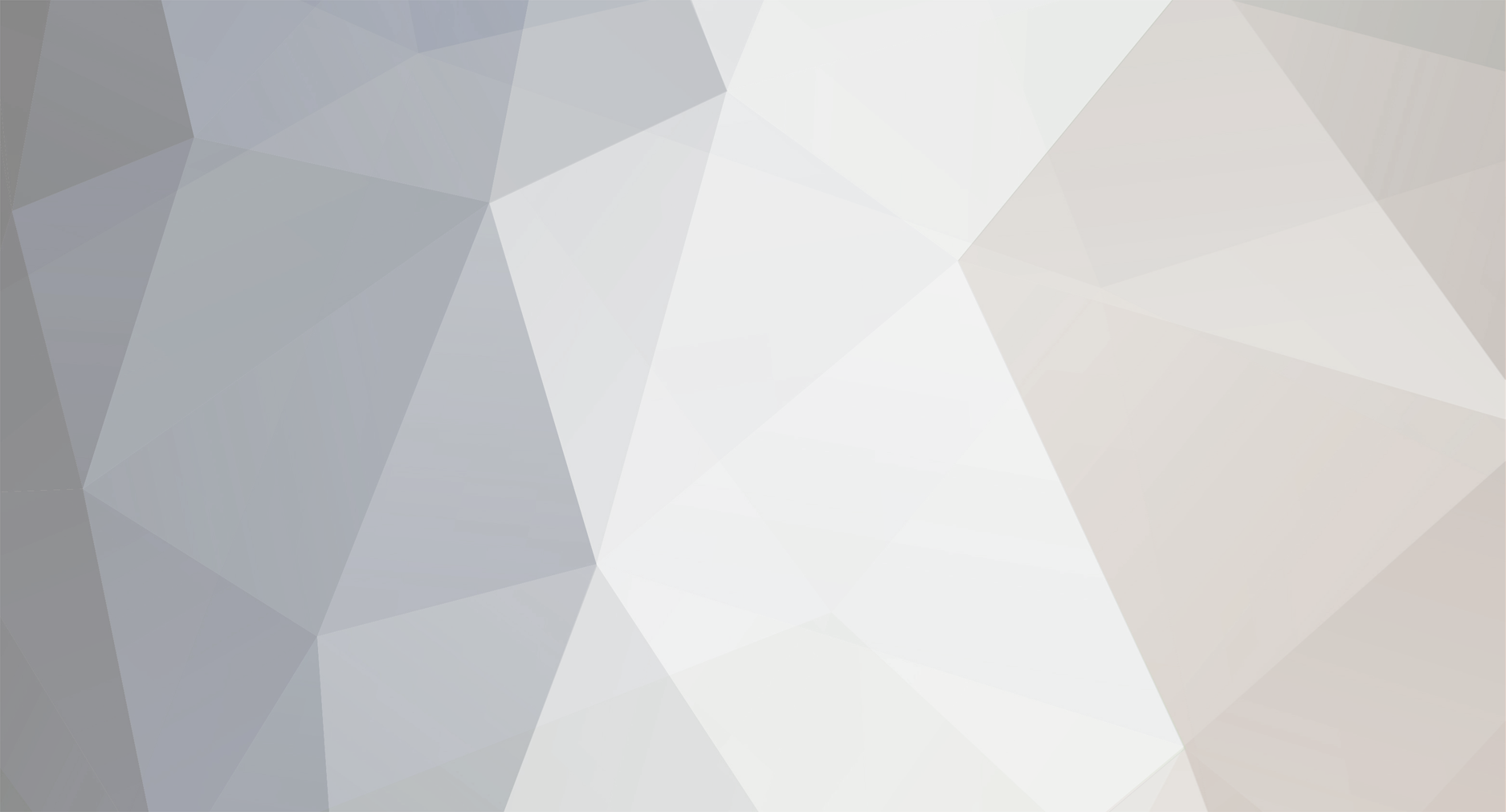
hansford
-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Posts posted by hansford
-
-
I don't see the rest of your code, but your attempted access is off.
$test = $_GET["account_id"]; foreach ($output['data'][$test] as $item) { echo $item['mark'] . '</br>'; }
-
You are only calling a static method of the class. In order to call the constructor you need to create an object of the class.
use foo as foo; //\foo\foo_bar::foo(); $foo = new \foo\foo_bar(); $foo::foo(); class foo_bar { function __construct() { $this->foobar(); } public static function foo() { print('This is foo'); } public function foobar() { print('<br />This is foobar'); } }
-
foreach ($cities as $city) {
if($city[0] == 'E') {
print_r($city);
}
} -
what does klasse.txt look like ? Post that file.
-
Looking over the code....
If $_GET['id'] is not set, then none of the variables are going to be defined.
$row is defined twice and only the second definition counts.
Put in some echo's to see what is going on along the code path.
$row=mysqli_fetch_assoc($result); $run = mysqli_query($conn,$sql); $row=mysqli_fetch_array($run);
-
You can try this for now:
$re = "/(\sF\d+.*[\r\n]{3,})/";
-
$output = array( 'data'=>array( '500020315'=>array( 'members'=>array( array('account_name'=>'zebra'), array('account_name'=>'gazelle'), array('account_name'=>'chiken'), array('account_name'=>'antelope') )))); usort($output['data']['500020315']['members'],function($a, $b) { $c = $a['account_name']; $d = $b['account_name']; if($c == $d) return 0; else if($c > $d) return 1; else return -1; });
-
"cleaning the URL",Totally and in a nut shell:1.You must use rawurlencode() for parts that come before "?"2.Use urlencode for all GET parameters (values that come after each "=")(POST parameters are automatically encoded).3.Use htmlspecialchars for HTML tag parameters and HTML text content
<?php
$url_page = 'example/page/url.php';
//page the link will request
$text = 'this is a simple string';
$id = '4334%3434';
$linktext = "<Clickit> & you will see it";
//text of the link, with HTML unfriendly characters
?>
<?php
// this gives you a clean link to use
$url = "http://localhost/";
$url .= rawurlencode($url_page);
$url .= "?text=" . urlencode($text);
$url .= "&id=" . urlencode($id);
// htmlspecialchars escapes any html that
// might do bad things to your html page
?>
<a href="<?php echo htmlspecialchars($url); ?>">
<?php echo htmlspecialchars($linktext); ?>
</a> -
http://codex.wordpress.org/Function_Reference/get_term
In the Examples section it appears to demonstrate how to get the slug.
$term = get_term( $term_id, $taxonomy ); Gives you term slug: e.g.: term-slug-example $slug = $term->slug;
-
echo str_replace(' ', '-',trim($citylower)); -
From the information we have been given, this process should not take any real time using a proper database setup.
I agree with Ch0cu3r, post the code requested so we can sort out what the real issue is.
-
@hansford, I changed the data type to BLOB, however, it stores the file as kind of binary file (see attached image please).
That is what it's suppose to do. It's a database, not a file system. In order to convert it back into a file, you need to write that data to a file with the proper file extension of whatever it used to be before you placed it in the database.
-
I agree with QuickOldCar,
PHP doesn't appear to be parsing the file. The PHP engine needs to be installed before that can happen.
I use WAMP as it packaged with all I need for a localhost development environment.
-
In my opinion, storing files in a database is not a good idea. Store the file location in the database and the file in the file system. If you do have to store a file in the database, then you need to be storing it as type BLOB.
-
1
-
-
This will make it more clear to you. http://php.net/manual/en/faq.passwords.php
-
You have a form with a method of "post" but are attempting to retrieve the id via $_GET....should match your form method...$_POST
-
define('br','<br />');
$lists = array(
array('id'=>'g','total'=>33,'goal'=>100),
array('id'=>'c','total'=>20,'goal'=>50),
array('id'=>'e','total'=>3,'goal'=>5));
$mid_array = array();
$highest_id = '';
$i = 0;
foreach($lists as $list)
{
$id = $list['id'];
$total = $list['total'];
$goal = $list['goal'];
$percent = ($total / $goal) * 100;
$mid_array[$i]['id'] = $id;
$mid_array[$i]['percent'] = $percent;
$i++;
}
usort($mid_array, function($a, $b){
$c = $a['percent'];
$d = $b['percent'];
if($c == $d) { return 0; }
if($c < $d) { return 1; }
return -1;
});
foreach($mid_array as $arg)
{
echo $arg['id'] . ' ' . $arg['percent'] . '%' . br;
} -
My Opinions:
When you bunch up your code like that it makes it difficult to follow and see where errors may be occurring.
I would enclose conditional statements within braces. Less prone to errors as what happens if you decide to add an additional statement.
If a function or variable name is more than one word long...each successive word should start with a capital letter or an underscore should be used between words for clarity.
function checkTitle( $title ) { if(strlen($title) > 55) { return getMsg('Title Is Too Long'); } if(! preg_match('/[a-z]/', $title)) { return getMsg('Title Is All Caps'); } }
-
To get it back into array format you could do something like so:
$path = 'path to file goes here'; $fp = @fopen($path, 'rb'); if($fp === false) { echo 'failed to create/open csv file'; exit; } $start = true; $headers = array(); $multi = array(); while(($data = fgetcsv($fp,4095)) !== false) { if($start) { $headers = array_values($data); $start = false; continue; } // combine headers with data array to form associative array $multi[] = array_combine($headers, $data); } print_r($multi);
-
I knew I was missing stuff. The code is very difficult to follow.
if (strlen)($password)) > 20) { // if (strlen($password) > 20) $error[] 'Username is too long'; } $login = login($username, $password); if login ==== false) { // if (login === false) $errors[] = "That username or password is incorrect. Please try again."; } else if { // No conditional statement. should be only else and not else if $_Session['user_id'] = $login; //as mentioned $_SESSION['user_id'] = $login; header('Location: index.php'); edit(); }
-
class MyObj
{
public function read()
{
//do something
}
}
MyObj = new MyObj(); // create new object
MyObj->read(); //this works
MyObj->write(); //fatal error as the there is no member function called "write" of this object -
This part of the code is not going to work.
if (strlen)($password)) > 20) { // if (strlen($password) > 20) $error[] 'Username is too long'; } $login = login($username, $password); if login ==== false) { // if (login === false) $errors[] = "That username or password is incorrect. Please try again."; } else if { $_Session['user_id'] = $login; header('Location: index.php'); edit(); }
-
It's difficult to tell where the logical error is from the information we are presented with. Can more than one user potentially have the same username and password ? Users can have the same password, but there should only be one user with that name.
-
I would be checking this part of the code based on some known username, password and status of both Y and N.
echo out the value of $row['active'];
Personally, I wouldn't use a character for this column to compare. I would use a Boolean value of 1 or 0;
if ($result) { // If uname and password match while($row = mysqli_fetch_assoc($query)) { if ($row['active'] == 'N') { // Account is not active echo "<p>Your account has not been activated! Please check your email inbox.</p><br />"; } else if ($row['active'] == 'Y') { // Account is active $_SESSION['uname'] = $_POST['uname']; header("Location: /profile"); }
if inside foreach loop not working
in PHP Coding Help
Posted
Then use this if you are not sure of the case: