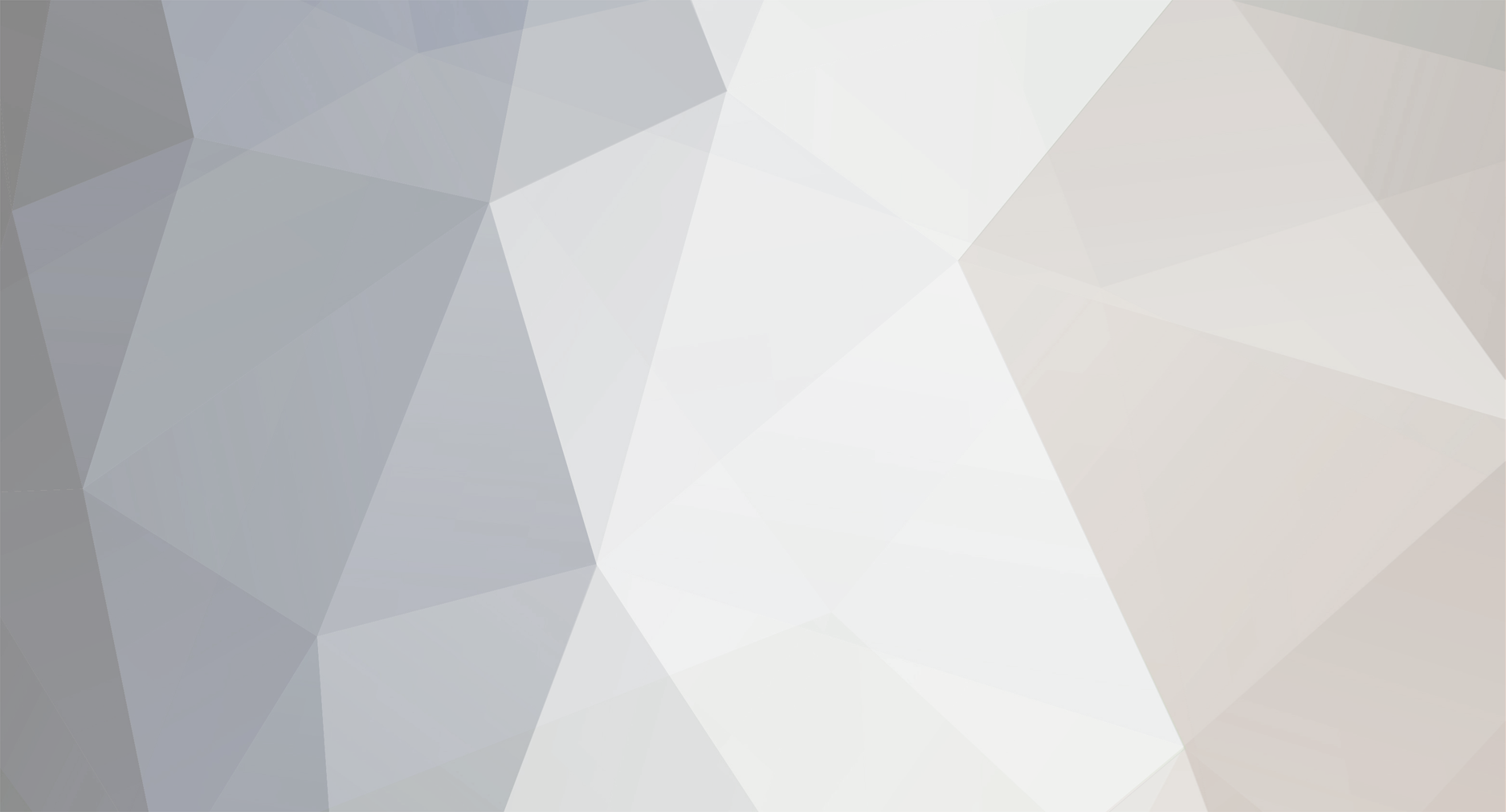
Stephen
Members-
Posts
200 -
Joined
-
Last visited
Never
Everything posted by Stephen
-
<?php $con = mysql_connect(localhost,root,qwertyztp99); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("demo", $con); $result = mysql_query("SELECT * FROM questions LIMIT 30"); while($row = mysql_fetch_array($result)){ $q_id = $row['question_id']; //display question here $choices = mysql_query("SELECT * FROM question_id WHERE question_id='$q_id'"); while($row2 = mysql_fetch_array($choices)) { //display answers here } } ?> Okay try that D: You forgot a ) after the while function. I missed it xD
-
Check your file, you probably have two new lines under the last line with text on it. Try deleting them and test it again.
-
Can you give me the table with the users in it's name, and the columns of it?
-
I don't know how order by rand() would work, so try this. <?php $con = mysql_connect(localhost,root,qwertyztp99); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("demo", $con); $result = mysql_query("SELECT * FROM questions LIMIT 30"); while($row = mysql_fetch_array($result)){ $q_id = $row['question_id']; //display question here $choices = mysql_query("SELECT * FROM question_id WHERE question_id='$q_id'"); while($row2 = mysql_fetch_array($choices){ //display answers here } } ?>
-
You could do something like: (by the way, the mysql_query should be the select one getting the emails from the db) <?php $_l=$_GET["l"]; if (!$_l) { $_l=0; } $_nl=$_l+1000; //Insert loop here //Put below where you put your query, just add the end of it to it (from the LIMIT on) mysql_query("[YOUR QUERY] LIMIT ".$_l.",1000"); //End loop echo("<html> <head> <META http-equiv='refresh' content='5;URL=page.php?l=".$_nl."'> </head> </html>"); ?>
-
<?php $page = $_GET["navigate"]; if (!$page) { include "index.php"; } else if($page=="Home") { include "index.php"; } else if($page=="ahome") { include "aph.html"; } else { echo "<b><h1>PHP Error</h1></b>"; } ?> Does that help? D:
-
<?php while (!feof($handle)) { $line=fgets($handle); //Insert your code for finding the variables... the string is $line mysql_query("INSERT INTO table VALUES('','','','etc.')"); } ?> Didn't test it. $handle is the fopen variable, then I put a comment for where you should find the new variables from $line. Just edit the mysql_query with the variables in the right order.
-
$sql="INSERT INTO question_id (ID, question) VALUES ('$_POST[question_ID]','$_POST[Cans1]','$_POST[ans1]','$_POST[Cans2]','$_POST[ans2]','$_POST[Cans3]','$_POST[ans3]','$_POST[C You put in too many values. It should be something like, $sql="INSERT INTO question_id (ID, question, answer) VALUES ('$_POST[question_ID]','$_POST[Cans1]','$_POST[ans1]')"; By the way, you'll need to add an answer column in question_id table.
-
Can you post the index page (and possibly the syle.css page)?
-
Try to try it yourself first and if you have problems post here. PHP.net is your friend. But heres a code: <?php $_hour=date("G"); //Returns the string value of the hour in 24 hour format //Below will check if its less than 5AM or greater than 6PM (18) if (intval($_hour)<5 && intval($_hour)>18) { ?> Your HTML code here <?php } else { echo("Please return again between 5AM and 6PM"); //Displays this message if it isn't between 5AM and 6PM } ?> I didn't test it, tell me if it works. By the way, intval converts from a string value to an integer value.
-
Do you get any errors, or does it just not log you in? EDIT: found one problem Find: $sql = "SELECT id, nickname, privilages FROM admin WHERE username = '$user_name' AND password = '$md5pass'"; Try using: $sql = "SELECT id, nickname, privilages FROM admin WHERE username = '$user' AND password = '$md5pass'";
-
Yeah, so many different kinds of loops, that's why I hate 'em!
-
Well if you want it to keep looping you can't just use if. Maybe try using this? D: <?php $i = 1; do { $newfile = file_get_contents("http://site.com/$i.htm"); echo $newfile; sleep(3); $i++; } while ($i<135); ?> Otherwise I think it should work? D: Unless your site doesn't allow file_get_contents.
-
You could try using file_get_contents(site); to get the site.
-
Maybe the $_POST variable is getting confused with the $_POST function thing? D: If you change the variable name from $_POST to something else, maybe it would work?
-
Populate A Drop Down Box With Values From Database!
Stephen replied to murtz's topic in PHP Coding Help
<?php include("connect.php"); $result = mysql_query("SELECT * FROM module WHERE study_year = '1'"); ?> <table border="2" align="center" cellpadding="10" cellspacing="2" bordercolor="#D6D6D6"> <tr bgcolor="#AFEBEF"> <td height="30" bordercolor="#D6D6D6"><p class="style19" style="text-align: left;"> Modules</p></td> </tr> <tr> <td><select name="readinglist"> <?php while($row = mysql_fetch_array($result)) { ?> <option><?php echo $row["module_name"]; ?></option> <?php }?> </select></td> </tr> </table> Try that now. I moved some things from in between the while loop. -
How do you send several values using a FOR loop in an email
Stephen replied to kpetsche20's topic in PHP Coding Help
I edited my post, try that again. Lmfao sorry I'm kinda tired right now. Forgot toedit the variables. Try this: <?php if(isset($_POST['submit'])) { ///GET EMAIL $sh = "SELECT * FROM users WHERE login = 'admin'"; $sh2 = mysql_query($sh); $body=""; $sql = "SELECT * FROM checkout WHERE username = '".$_SESSION['id']."'"; $run = mysql_query($sql); $data2 = mysql_fetch_array($sh2); $to = $data2['email']; while ($_rows=mysql_fetch_array($run)) { $body .= " Name: ".$_rows['name']." QTY: ".$_rows['qty']." "; } mail($to, 'Subject', $body) or die(mysql_error()); echo "Quote Sent Successfully"; $delete = "DELETE FROM checkout WHERE username = '".$_SESSION['id']."'"; mysql_query($delete) or die(mysql_error); } ?> -
Inside the mysql function at the last part you could put "LIMIT 0,9" and that would retrieve rows 1,2,3,4,5,6,7,8,9. And keep doing that. Sample : $_start=0; $_current=0; $_one=mysql_query("SELECT * FROM place"); $_onenum=mysql_num_rows($_one); do { $_two=mysql_query("SELECT * FROM place LIMIT ".$_start.",9"); while($_rows=mysql_fetch_array($_two)) { echo($_rows["row"]); } $_start+=9; $_current+=9; echo("<br /><br />"); } while ($_onenum>$_current); I think that should work, but I didn't test it. There is probably an easier way anyway.
-
How do you send several values using a FOR loop in an email
Stephen replied to kpetsche20's topic in PHP Coding Help
<?php if(isset($_POST['submit'])) { ///GET EMAIL $sh = "SELECT * FROM users WHERE login = 'admin'"; $sh2 = mysql_query($sh); $body=""; $sql = "SELECT * FROM checkout WHERE username = '".$_SESSION['id']."'"; $run = mysql_query($sql); $data2 = mysql_fetch_array($sh2); $to = $data2['email']; while ($_rows=mysql_fetch_array($_run)) { $body .= " Name: ".$data['name']." QTY: ".$data['qty']." "; } mail($to, 'Subject', $body) or die(mysql_error()); echo "Quote Sent Successfully"; $delete = "DELETE FROM checkout WHERE username = '".$_SESSION['id']."'"; mysql_query($delete) or die(mysql_error); } ?> Maybe this will work? Btw, I don't know why you have "or die(mysql_error);" next to the mail function, because I don't think it would cause a mysql error even if it had an error. D: -
I fixed it.
-
Can someone help me? I think it might be an array problem :/
-
[SOLVED] Just a quickie! :) 1 minute read and solve
Stephen replied to futurewii's topic in PHP Coding Help
<?php $_handle=mysql_connect("host","username","password"); $_db=mysql_select_db("database",$_handle); mysql_query("INSTERT INTO ".$_POST["console"]."reviews (review) VALUES ('User submitted review here')"); mysql_close($_handle); ?> Something like that? -
<?php if (!isset($_POST["submit"])) { ?> <form action="<? echo($_SERVER['PHP_SELF']); ?>" method="POST"> <input type="text" name="information" value="information" /> <input type="submit" name="submit" value="Submit" /> </form> <?php } else { $_handle=mysql_connect("host","username","password"); $_db=mysql_select_db("database",$_handle); mysql_query("INSTERT INTO tablename (information) VALUES ('".$_POST["text"]."')"); mysql_close($_handle); } ?> A very very very basic way to do it. You need to edit the things for it to work.
-
I'm not an expert, but is your File Uploads variable enabled? Mine says (in cpanel): "File Uploads file_uploads Whether to allow HTTP file uploads. On"
-
Okay well I attempted to make a script that should check the links of a thread and make sure they work (like the bot on a phpbb forum). For some reason it can't do alot of threads, only like 20 or something. If you have vbulletin and a section with RS/MU etc. links you can try this and tell me what it gets to D: And if it's a programming problem can someone help me? <?php /////////////////////////////////////////////////////////// // Script created by: Stephen (aka AADude) //////////////////// // Use: Checking to see if links are dead or not ////////////////// ////////////////////////////////////////////////////////// function ss_link_check($_url,$_type="RS") { //RS = RapidShare; MU = MegaUpload; ES = EasyShare; FF = FileFactory; SS = SendSpace; $_sites=array( "RS" => "Error", "MU" => "Unfortunately, the link you have clicked is not available.", "ES" => "File not found", "FF" => "Sorry, this file is no longer available. It may have been deleted by the uploader, or has expired.", "SS" => "Sorry, the file you requested is not available.", "FFFH" => "Your requested file is not found" ); $_fgc=file_get_contents($_url); if (preg_match("/".$_sites[$_type]."/",$_fgc) or $_fgc=="") { $_correct=false; } else { $_correct=true; } return $_correct; } //Include the vBulletin configuration file include ("../config.php"); //Search the DB if ($config['MasterServer']['usepconnect']==1) { $_connection=mysql_pconnect( $config['MasterServer']['servername'].":".$config['MasterServer']['port'], $config['MasterServer']['username'], $config['MasterServer']['password']); } else { $_connection=mysql_connect( $config['MasterServer']['servername'].":".$config['MasterServer']['port'], $config['MasterServer']['username'], $config['MasterServer']['password']); } mysql_select_db( $config['Database']['dbname'], $_connection); $_boards_array=array( 1 => "16", 2 => "20", 3 => "23", 4 => "26", 5 => "28", 6 => "32", 7 => "18" ); foreach ($_boards_array as $_key => $_cboard) { echo("<b>Board ID:</b> ".$_cboard."<br />"); $_query1=mysql_query('SELECT * FROM '.$config['Database']['tableprefix'].'thread WHERE forumid="'.$_cboard.'" ORDER BY threadid DESC'); while ($_rows1=mysql_fetch_array($_query1)) { $_threadid=$_rows1["threadid"]; $_firstpostid=$_rows1["firstpostid"]; $_query2=mysql_query('SELECT * FROM '.$config['Database']['tableprefix'].'post WHERE postid="'.$_firstpostid.'"'); while ($_rows2=mysql_fetch_array($_query2)) { $_pagetext=$_rows2["pagetext"]; $_link=preg_match_all("@\[(?i)url\](.*?)\[/(?i)url\]@si",$_pagetext,$_url,PREG_SET_ORDER); $_replace=preg_replace("@\[(?i)url\](.*?)\[/(?i)url\]@si","@\[(?i)url\](.*?)\[/(?i)url\]@si",$_pagetext,-1,$_count); $_not_working=0; foreach ($_url as $_key2 => $_site) { if (preg_match("/rapidshare/",$_url[$_key2][1])) { $_type="RS"; } else if (preg_match("/megaupload/",$_url[$_key2][1])) { $_type="MU"; } else if (preg_match("/easyshare/",$_url[$_key2][1])) { $_type="ES"; } else if (preg_match("/filefactory/",$_url[$_key2][1])) { $_type="FF"; } else if (preg_match("/sendspace/",$_url[$_key2][1])) { $_type="SS"; } else if (preg_match("/fastfreefilehosting/",$_url[$_key2][1])) { $_type="FFFH"; } else { $_type=0; } $phper=error_get_last(); $message=$phper[2]; echo($_url[$_key2][1]." / ".mysql_error($_connection)." / ".$message."<br />"); if ($_type!==0) { if (ss_link_check($_url[$_key2][1],$_type)) { //blah } else { $_not_working+=1; } } } $_delete=$_not_working/$_count; $_delete=$_delete*100; if ($_delete > 60) { mysql_query('UPDATE '.$config['Database']['tableprefix'].'thread SET forumid="14" WHERE threadid="'.$_threadid.'"'); mysql_query('UPDATE '.$config['Database']['tableprefix'].'post SET pagetext="'.$_pagetext.'[quote]One or more links were found dead. If you want this moved back please report this post or PM Stephen.[/quote]" WHERE postid="'.$_firstpostid.'"'); } } } } ?> VB 3.7.x btw. I put this in includes/cron/ folder. Okay, my problem is that the script suddenly stops the loop before all of the threads in all 7 of the boards were checked. I posted this on another site too. EDIT: If you want to see a hosted example please PM me and I will give you a link.