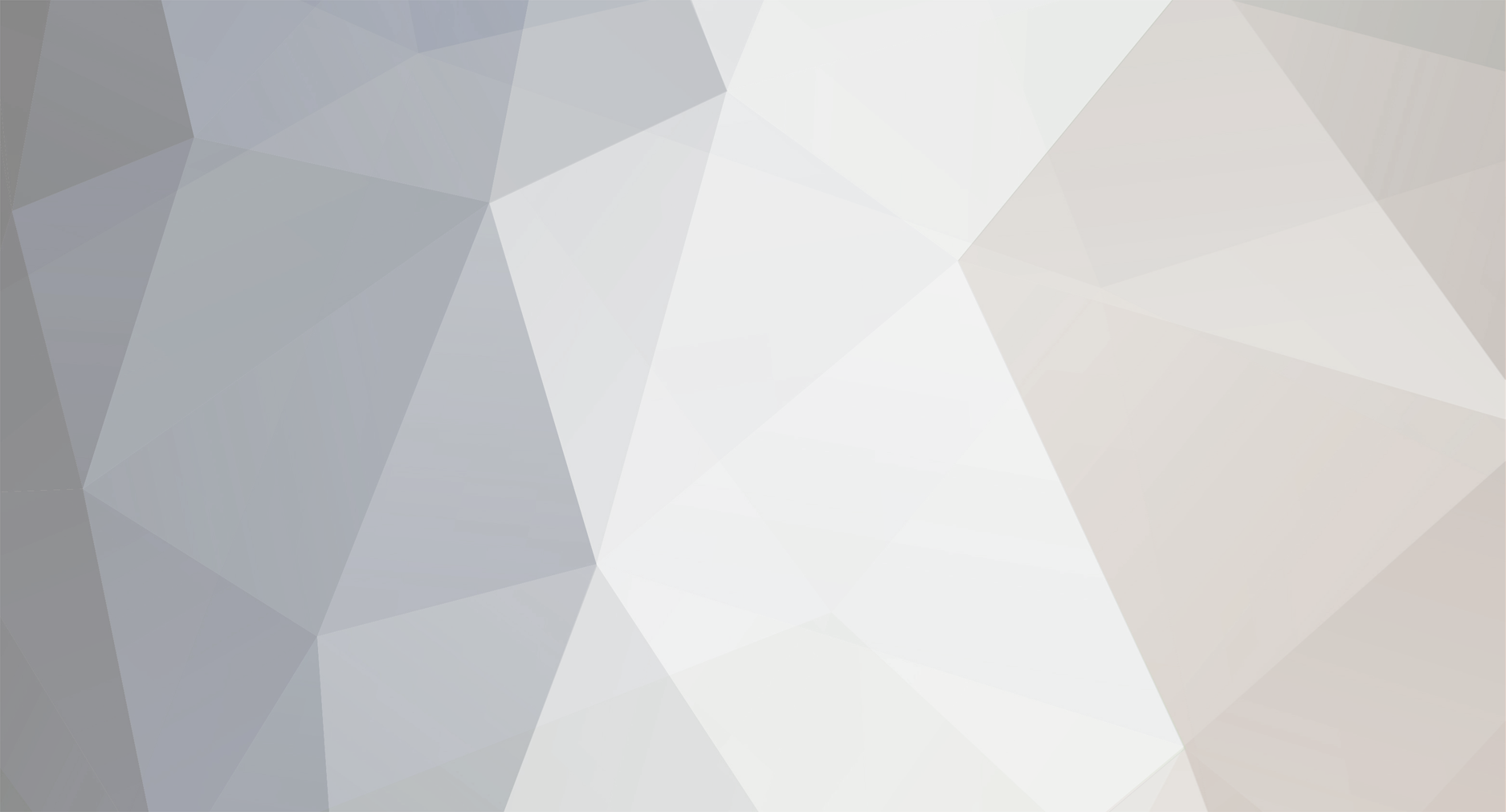
tfburges
Members-
Posts
79 -
Joined
-
Last visited
Never
Everything posted by tfburges
-
[SOLVED] Function for navigating/modifying a multidimensional array
tfburges replied to tfburges's topic in PHP Coding Help
Ahhhhh I figured it out! The problem was that I needed an ampersand before the name of the function, digDeep, so that PHP knew to return a reference (AKA pointer in C/C++). It looks like PHP isn't so different from C/C++ after all. This is what I had to do: (Note the placement of the ampersands before the variables and before the digDeep function.) /* This function "digs" into a multidimensional array and returns whatever it finds */ // 08.09.2009; 08.13.2009 function &digDeep($start,$depth) { if (is_array($depth)) { if (sizeof($depth) == 1) { $output = &$start[$depth[0]]; } else if (sizeof($depth) == 2) { $output = &$start[$depth[0]][$depth[1]]; } else if (sizeof($depth) == 3) { $output = &$start[$depth[0]][$depth[1]][$depth[2]]; } else if (sizeof($depth) == 4) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]]; } else if (sizeof($depth) == 5) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]]; } else if (sizeof($depth) == 6) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]]; } else if (sizeof($depth) == 7) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]]; } else if (sizeof($depth) == { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]]; } else if (sizeof($depth) == 9) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]]; } else if (sizeof($depth) == 10) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]]; } else if (sizeof($depth) == 11) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]]; } else if (sizeof($depth) == 12) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]]; } else if (sizeof($depth) == 13) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]]; } else if (sizeof($depth) == 14) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]]; } else if (sizeof($depth) == 15) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]][$depth[14]]; } else { $output = array('Array size of depth cannot be greater than 15.'); } } else if ($depth != "" && $depth != 0 && $depth != false) { $output = &$start[$depth]; } else { $output = &$start; } return $output; } /* Quick function for handling post data; can handle any number of elements that have been added by the PHP/javascript "Add Another" functions */ // 08.09.2009; 08.13.2009 function handlePostData($name,&$storeStart,$storeDepth) { if ($_POST['AddAnother_' . $name . '_num']) { $i = 0; $num = $_POST['AddAnother_' . $name . '_num']; if ($_POST[$name] && $num > 0) { $copy = $storeStart; foreach ($storeStart as &$sub) { unset($sub); } $storeStart['0'] = $copy; $storeLocation = &digDeep(&$storeStart['0'],$storeDepth); $storeLocation = $_POST[$name]; $i = 1; } while ($i <= $num) { if ($_POST[$name . '_num_' . $i]) { $storeLocation = &digDeep(&$storeStart[$i],$storeDepth); $storeLocation = $_POST[$name . '_num_' . $i]; } $i++; } } else { if ($_POST[$name]) { $storeLocation = &digDeep(&$storeStart,$storeDepth); $storeLocation = $_POST[$name]; } } } -
[SOLVED] Function for navigating/modifying a multidimensional array
tfburges replied to tfburges's topic in PHP Coding Help
Hmmm I still may not be clear. This example should help... Suppose there is only 1 AssetType, and so following variable exists: $_SESSION['configWizard']['AssetType']['_c']['Model']['_v'] (Model is a child of AssetType and has a value; hence, "_c" and "_v"... remember, this array structure comes from a large XML file.) And in the config wizard, the user creates at least one other AssetType... So to account for more than one key of the same name, the original AssetType must be moved to the following array like so: $_SESSION['configWizard']['AssetType']['_c']['Model']['_v'] --> $_SESSION['configWizard']['AssetType']['0']['_c']['Model']['_v'] ...so that any additional AssetTypes can be added whose structure will look something like: $_SESSION['configWizard']['AssetType']['1']['_c']['Model']['_v'] $_SESSION['configWizard']['AssetType']['2']['_c']['Could'][_c]['Be']['_v'] $_SESSION['configWizard']['AssetType']['3']['_c']['Anything']['_v'] Now, I've added a lot of comments/questions to my code to help explain what I'm trying to do... so please read: function handlePostData($name,&$storeStart,$storeDepth) { // name is simply the name of the input text field that is used to modify a particular key // storeStart should be the starting array to be modified, i.e. $_SESSION['configWizard']['etc'] // and the ampersand is before storeStart so that whatever variable passed is modified, not a copy // storeDepth is an array like: array('_c','Model','_v') so that the function can access // the correct key structure regardless of whether or not it is something like... // $_SESSION['configWizard']['AssetType']['_c']['Model']['_v'] // versus // $_SESSION['configWizard']['AssetType']['0']['_c']['Model']['_v'] if ($_POST['AddMore_' . $name . '_num']) { // this post variable is a hidden input that counts the number of extra elements // of the same key that were added $i = 0; $num = $_POST['AddMore_' . $name . '_num']; if ($_POST[$name] && $num > 0) { // if just post[name], meaning there was only 1 to begin with // (since if there was more than 1 to begin with, the post variable would be... // ...post[name_num_0],post[name_num_1],etc.) // and another element of same key was added (i.e., num > 0) $copy = $storeStart; // store a copy the data within the single key structure... // because... // $_SESSION['configWizard']['AssetType'] // ...needs to be copied to... // $_SESSION['configWizard']['AssetType']['0'] foreach ($storeStart as &$sub) { unset($sub); // since the entire structure under the starting position will be moved // everything under the starting position needs to be unset } $storeStart['0'] = $copy; // $_SESSION['configWizard']['AssetType'] // ...should now copied be to... // $_SESSION['configWizard']['AssetType']['0'] $storeLocation = &digDeep($storeStart['0'],$storeDepth); // the storeLocation should be a specific key structure beneath the newly moved/created array // the digDeep function should return the actual $_SESSION variable and not a copy $storeLocation = $_POST[$name]; // the posted data should replace the data in that particular location of memory $i = 1; // set the index to 1 so that it starts at 1 now since 0 is taken care of } while ($i <= $num) { if ($_POST[$name . '_num_' . $i]) { // each element added in the config wizard should have an input similar to... // name_num_0, name_num_1, etc. $storeLocation = &digDeep($storeStart[$i],$storeDepth); // the store location should become something like... // $_SESSION['configWizard']['AssetType']['1']['_c']['Model']['_v'] // then... // $_SESSION['configWizard']['AssetType']['2']['_c']['Model']['_v'] // for each one added/present // etc. $storeLocation = $_POST[$name . '_num_' . $i]; // store the respective posted data in memory } $i++; } } else { // there was only 1 element of a particular key and no extra elements were added, so... if ($_POST[$name]) { // make sure something was posted $storeLocation = &digDeep($storeStart,$storeDepth); // the storeLocation would still need to point to the correct address in memory $storeLocation = $_POST[$name]; } } } Now... I've been testing with the simplest case (where there was only 1 element of a particular key and no extra elements were added)... so the problem must lie within my digDeep function and/or the ampersand usage in the handlePostData function. Here's the digDeep function again: function digDeep(&$start,$depth) { // any changes/references to start should be direct, not a copy if (is_array($depth)) { // I made it this ridiculous if/else statement just to work out the kinks in my ampersand usage... // It used to be a for loop. if (sizeof($depth) == 1) { $output = &$start[$depth[0]]; } else if (sizeof($depth) == 2) { $output = &$start[$depth[0]][$depth[1]]; } else if (sizeof($depth) == 3) { $output = &$start[$depth[0]][$depth[1]][$depth[2]]; } else if (sizeof($depth) == 4) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]]; } else if (sizeof($depth) == 5) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]]; } else if (sizeof($depth) == 6) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]]; } else if (sizeof($depth) == 7) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]]; } else if (sizeof($depth) == { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]]; } else if (sizeof($depth) == 9) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]]; } else if (sizeof($depth) == 10) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]]; } else if (sizeof($depth) == 11) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]]; } else if (sizeof($depth) == 12) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]]; } else if (sizeof($depth) == 13) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]]; } else if (sizeof($depth) == 14) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]]; } else if (sizeof($depth) == 15) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]][$depth[14]]; } else { $output = array('Array size of depth cannot be greater than 15.'); } } else if ($depth != "" && $depth != 0 && $depth != false) { $output = &$start[$depth]; } else { $output = &$start; } return $output; // the output returned SHOULD be a direct reference to the location in memory, not a copy // ...but it does not seem to be working that way no matter what I do. } Also, here is an example of simple usage (testing the case of just 1 element, no extra): In theory, it may either be... handlePostData('Model',$_SESSION['configWizard']['AssetType'],array('_c','Model','_v')); ...or... handlePostData('Model',$_SESSION['configWizard']['AssetType']['_c']['Model'],false); ...but neither work. It MUST be my use of ampersands but I just can't figure it out! Do you see what the problem is? And does anyone know how to solve it? -
[SOLVED] Function for navigating/modifying a multidimensional array
tfburges replied to tfburges's topic in PHP Coding Help
I tried the following with various similar combinations of ampersand usage and to no avail... /* Quick function for handling post data; can handle any number of elements that have been added by the PHP/javascript "Add Another" functions */ // 08.09.2009 function handlePostData($name,&$storeStart,$storeDepth) { if ($_POST['AddMore_' . $name . '_num']) { $i = 0; $num = $_POST['AddMore_' . $name . '_num']; if ($_POST[$name] && $num > 0) { $copy = $storeStart; foreach ($storeStart as &$sub) { unset($sub); } $storeStart['0'] = $copy; $storeLocation = &digDeep($storeStart['0'],$storeDepth); $storeLocation = $_POST[$name]; $i = 1; } while ($i <= $num) { if ($_POST[$name . '_num_' . $i]) { $storeStart[$i] = $copy; $storeLocation = &digDeep($storeStart[$i],$storeDepth); $storeLocation = $_POST[$name . '_num_' . $i]; } $i++; } } else { if ($_POST[$name]) { $storeLocation = &digDeep($storeStart,$storeDepth); $storeLocation = $_POST[$name]; } } } /* This function "digs" into a multidimensional array and returns whatever it finds */ // 08.09.2009 function digDeep($start,$depth) { if (is_array($depth)) { /*foreach ($depth as $dig) { $start = $start[$dig]; }*/ if (sizeof($depth) == 1) { $output = &$start[$depth[0]]; } else if (sizeof($depth) == 2) { $output = &$start[$depth[0]][$depth[1]]; } else if (sizeof($depth) == 3) { $output = &$start[$depth[0]][$depth[1]][$depth[2]]; } else if (sizeof($depth) == 4) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]]; } else if (sizeof($depth) == 5) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]]; } else if (sizeof($depth) == 6) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]]; } else if (sizeof($depth) == 7) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]]; } else if (sizeof($depth) == { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]]; } else if (sizeof($depth) == 9) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]]; } else if (sizeof($depth) == 10) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]]; } else if (sizeof($depth) == 11) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]]; } else if (sizeof($depth) == 12) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]]; } else if (sizeof($depth) == 13) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]]; } else if (sizeof($depth) == 14) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]]; } else if (sizeof($depth) == 15) { $output = &$start[$depth[0]][$depth[1]][$depth[2]][$depth[3]][$depth[4]][$depth[5]][$depth[6]][$depth[7]][$depth[8]][$depth[9]][$depth[10]][$depth[11]][$depth[12]][$depth[13]][$depth[14]]; } else { $output = array('Array size of depth cannot be greater than 15.'); } } else if ($depth != "" && $depth != 0 && $depth != false) { $output = &$start[$depth]; } else { $output = &$start; } return $output; } -
[SOLVED] Function for navigating/modifying a multidimensional array
tfburges replied to tfburges's topic in PHP Coding Help
Now that I'm back... I reviewed what I posted and it may not be clear enough... but I think I included all relevant information. If something is unclear let me know. It looks like my problem is ampersand usage. I'm not sure if it's possible to use it in a for loop like I did but I don't see why not. I've always been unsure when and where to place the ampersands when I want to modify the original variable... I'll read up on it again but in the mean time, I'm pretty sure that's what my problem is if someone experienced can help. -
I'll try to make this as quick and concise as possible. I have a multidimensional array created by a function that parses xml files. The array structure can be best described by the following example... Given this XML: <SomeParent xml="blahBlah"> <OneElement>1.0</OneElement> <MultipleElementsOfSameName>Some value</MultipleElementsOfSameName> <MultipleElementsOfSameName>Another value</MultipleElementsOfSameName> <MultipleElementsOfSameName name="someAttribute">Some value</MultipleElementsOfSameName> </SomeParent> The following array will be created: Array ( [someParent] => Array ( [_a] => Array ( [xml] => blahBlah ) [_c] => Array ( [OneElement] => Array ( [_v] => 1.0 ) [MultipleElementsOfSameName] => Array ( [0] => Array ( [_v] => Some value ) [1] => Array ( [_v] => Another value ) [2] => Array ( [_a] => Array ( [name] => someAttribute ) [_v] => Some value ) ) ) ) ) Note that when multiple elements of the same name are present, that array key is created with numeric sub keys. Now, I have functions that generate forms based on the array and I would like to modify the respective values in the multidimensional array when post data is present. I have a javascript function that allows the user to add any number of extra elements of the same name (if a certain input to the form generation function is set to true but that's irrelevant)... and the names of the inputs would be something like "MultipleElementsOfSameName_num_0", "MultipleElementsOfSameName_num_1", "MultipleElementsOfSameName_num_2", etc. as more are added. I also have a hidden input that specifies how many the user has added (which is theoretically infinite). So... I have a function that handles post data: (Note that the second if statement in this function is for handling if there was only one element of a name present before, the array will need to move the original element's data to a key of '0' under that name to follow the correct structure of multiple elements.) /* Quick function for handling post data; can handle any number of elements that have been added by the PHP/javascript "Add Another" functions */ // 08.09.2009 function handlePostData($name,&$storeStart,$storeDepth) { if ($_POST['AddMore_' . $name . '_num']) { $i = 0; $num = $_POST['AddMore_' . $name . '_num']; if ($_POST[$name] && $num > 0) { $copy = $storeStart; foreach ($storeStart as &$sub) { unset($sub); } $storeStart['0'] = $copy; $storeLocation = digDeep(&$storeStart['0'],$storeDepth); $storeLocation = $_POST[$name]; $i = 1; } while ($i <= $num) { if ($_POST[$name . '_num_' . $i]) { $storeStart[$i] = $copy; $storeLocation = digDeep(&$storeStart[$i],$storeDepth); $storeLocation = $_POST[$name . '_num_' . $i]; } $i++; } } else { if ($_POST[$name]) { $storeLocation = digDeep(&$storeStart,$storeDepth); $storeLocation = $_POST[$name]; } } } The digDeep function is what I use for ensuring data retrieval from the multidimensional array regardless of whether or not an element it is singular or multiple. It is as follows: /* This function "digs" into a multidimensional array and returns whatever it finds */ // 08.09.2009 function digDeep($start,$depth) { if (is_array($depth)) { foreach ($depth as &$dig) { $start = &$start[$dig]; } } else if ($depth != "" && $depth != 0 && $depth != false) { $start = &$start[$depth]; } return $start; } I just added the ampersands ("&") hoping that would modify the original array's value(s) because that's what I want to happen when data is posted. I'm beginning to think the best way to handle something like this is to redo the "digDeep" function and make it something like... function digDeep($start, $depth) { if (sizeof($depth) == 0) { return $start; } else if (sizeof($depth) == 1) { return start[$depth[0]]; } else if (sizeof($depth) == 2) { return start[$depth[0]][$depth[1]]; } // etc. } I've actually gotta cut this a bit short right now but hopefully this will be enough to point me in the right direction.
-
Ahhhh I see! I assumed it would use the $link from earlier in the script. Thank you, sir.
-
Although in the error log it says "supplied argument is not a valid MySQL-Link resource." I googled this and I'm 99% sure I haven't run out of space, nor has MySQL run out of database connections. This is the code that is causing this: copyForm("form_name_value", $selected_form_name_value, "triggers", $new_form_name_value); And the function copyForm: function copyForm($where, $sel_form_name_value, $table_with_form_name_value, $new_form_name_value_to_insert) { $query_col_names = mysql_query("SELECT * FROM `$table_with_form_name_value` ORDER BY `id` DESC LIMIT 1", $link) or die ('MYSQL error: ' . mysql_error()); $num_cols = mysql_num_fields($query_col_names); $cols = "`"; $cols_array = array(); for ($i = 1; $i < $num_cols; $i++) { if ($i == $num_cols - 1) { $cols = $cols . mysql_field_name($query_col_names, $i) . "`"; } else { $cols = $cols . mysql_field_name($query_col_names, $i) . "`,`"; } $cols_array[$i] = mysql_field_name($query_col_names, $i); } $result_last_id = mysql_fetch_array($query_col_names); $last_id = $result_last_id['id']; $last_id_plus_one = $last_id; $values = "'"; $query_for_insert = mysql_query("SELECT * FROM `$table_with_form_name_value` WHERE `$where` = '$sel_form_name_value'", $link) or die ('MYSQL error: ' . mysql_error()); while ($result_for_insert = mysql_fetch_array($query_for_insert)) { for ($i = 1; $i < $num_cols; $i++) { $col_name = $cols_array[$i]; if ($i == $num_cols - 1) { $values = $values . $result_for_insert[$col_name] . "'"; } else { $values = $values . $result_for_insert[$col_name] . "','"; } } $last_id_plus_one++; mysql_query("INSERT INTO `$table_with_form_name_value` (`id`,$cols) VALUES ('$last_id_plus_one',$values)", $link) or die ('MYSQL error: ' . mysql_error()); mysql_query("UPDATE `$table_with_form_name_value` SET `$where` = '$new_form_name_value_to_insert' WHERE `id` > '$last_id'", $link) or die ('MYSQL error: ' . mysql_error()); } } All variables are accounted for (i.e. $selected_form_name_value and $new_form_name_value). Oh... and also, the line that the error log is referring to is: $query_col_names = mysql_query("SELECT * FROM `$table_with_form_name_value` ORDER BY `id` DESC LIMIT 1", $link) or die ('MYSQL error: ' . mysql_error()); I've used the same syntax hundreds of other times and never had a problem. I'm thinking it has something to do with the fact that it's within a function... ?
-
Querying a custom ODBC database through PHP/MySQL?
tfburges replied to tfburges's topic in MySQL Help
**Another edit (form wouldn't let me edit my last post again so I need to double post): Here's the code I'm using for the directory/file listing (I plan on modifying it to insert the directories/files into a MySQL): <?php function folderlist(){ $startdir = '\\\\xx.xx.xx.xx\\rbm\\RBM\\Cust'; $ignoredDirectory[] = '.'; $ignoredDirectory[] = '..'; if (is_dir($startdir)){ if ($dh = opendir($startdir)){ while (($folder = readdir($dh)) !== false){ if (!(array_search($folder,$ignoredDirectory) > -1)){ if (filetype($startdir . $folder) == "dir"){ $directorylist[$startdir . $folder]['name'] = $folder; $directorylist[$startdir . $folder]['path'] = $startdir; } } } closedir($dh); } } return($directorylist); } $folders = folderlist(); foreach ($folders as $folder){ $path = $folder['path']; $name = $folder['name']; echo '<a href="'. $path . $name .'">' . $name . '</a><br />'; } ?> All I really need to know is the syntax for an outside source "$startdir". Oh yeah... and the error message is: PHP Warning: Invalid argument supplied for foreach() in D:\\My Documents\\IRLCM_tech_form\\rbm_search.php on line 23 I do realize this thread is starting to be in the wrong forum... I'll post in another if needed. -
Querying a custom ODBC database through PHP/MySQL?
tfburges replied to tfburges's topic in MySQL Help
That's what I thought too. I've tried every possible combination of host names and user names and backslashes I could possibly think of. I must be missing something.... This is the odbc connection through PHP that I'm working with: (Forgive the redundancy of "RBM" lol... I replaced/deleted sensitive information with generic information.) $odbc_host = '\\\\xx.xx.xx.xx\\rbm'; $odbc_user = 'xx'; $odbc_pass = 'xx'; $odbc_dbname = 'db.rbm'; //$odbc_dbname = '\\\\xx.xx.xx.xx\\rbm\\RBM\\Cust\\South\\db.rbm'; (tried various combinations) $odbc_dsn = "DRIVER={CSI RBM 4.02 ODBC Driver};" . "CommLinks=tcpip(Host=$odbc_host);" . "DatabaseName=$odbc_dbname;" . "uid=$odbc_user; pwd=$odbc_pass"; $odbc_connect = odbc_pconnect($odbc_dsn, $odbc_user, $odbc_pass); if ($odbc_connect <= 0) { echo "Connection failed.<br>",$odbc_dsn; exit; } else { echo "Connection successful."; } This is the error message: PHP Warning: odbc_pconnect() [<a href='function.odbc-pconnect'>function.odbc-pconnect</a>]: SQL error: [sYWARE, Inc.][Dr. DeeBee ODBC Driver]Unable to connect, SQL state 08001 in SQLConnect in etc.php on line 21, referer: etc. Aaaand not that it matters much but... The data source is located on another machine on the network, but I can definitely access the machine because I have it mapped. In the mean time, unless someone knows what's wrong, I'll be working on a workaround (writing a script that pulls data into MS Access since I know that works and then querying Access through PHP... all for comparison with information from another (MySQL) database). To do this, I need to know how to get a directory/file listing so that I can right a batch file using odbcconf.exe (similar to odbcad32.exe (i.e. ODBC Data Source Administrator) but command line only) that adds the 1500+ rbm files to my data sources. Whoever designed this whole RBM thing wasn't thinking ahead... -
Querying a custom ODBC database through PHP/MySQL?
tfburges replied to tfburges's topic in MySQL Help
I'm just looking for a quick nudge in the right direction, hoping someone has experience in doing something similar and knows the best way to go about it so that I don't waste hours of my time trying something that either doesn't work or is really inefficient. That, and I was wondering if I was correct in my assumptions about needing to create a driver and the requirements to do so. -
Querying a custom ODBC database through PHP/MySQL?
tfburges replied to tfburges's topic in MySQL Help
None? -
The company I work for needs to query an outside datasource so it can be compared with the database I've created for them. The outside source is incredibly large (you have no idea...!) and requires a custom ODBC driver. I'm able to query it using Microsoft Access but not through PHP or MySQL. We've contacted the creators of the driver and they were of little to no help. I'm 99% sure I would need to create my own PHP extension to query the database through PHP. (I was hoping a generic ODBC connection would work as long as the driver was specified, but no.) I'm not at all familiar with creating PHP extensions and I'm not even sure I would be able to do it properly without the creators giving me the driver's source code. Nothing helpful shows up when googling the custom driver either. I could either somehow export everything using access or write a script that dumps data from the outside source into a text file (assuming I can use C++ to communicate with the database - don't know about this yet either), which would then be imported into MySQL... but I really don't want to do that, as it takes up way too much space and the information is already there (no point in having it in two places). Any suggestions?
-
[SOLVED] PHP Parse error: syntax error, unexpected '['...
tfburges replied to tfburges's topic in PHP Coding Help
LAWL. Can't win around here can I? I used to abbreviate my variables until someone told me I should be more descriptive. Now, I'm more descriptive and I'm told it's over the top. -
Is it possible that the driver simply can't handle queries from php? I've searched all over the place for information on this driver and it is apparently not used anywhere other than where I work LOL. I don't think I've found information on it in more than 3 places and they were of no help whatsoever.
-
That MySQL Driver stuff was some code I pulled off another web site that I was inquiring about. Turns out it's not what I'm looking for anyway. I'm positive that the driver I'm using now is the right one because I'm using the same one to query the database in Access. And I wish I could change the server but I can't without tons of work. The server isn't my doing anyway. All I really need to be able to do is retrieve some data from it through PHP. I hate it when stuff like this happens. It shouldn't be so complicated!
-
The database isn't mysql. It requires a different driver, i.e. the CSI RBM 4.02 ODBC Driver. I'm trying to contact the RBM guys and see if they can shed any light on the situation.
-
Thanks for the reply. I've tried this but to no avail. I just tried again just to make sure and I'm receiving the same message. $odbc_host = 'rbm1'; $odbc_user = 'user'; $odbc_pass = 'pass'; $odbc_dbname = 'Example.rbm'; $odbc_dsn = "DRIVER={CSI RBM 4.02 ODBC Driver};" . "CommLinks=tcpip(Host=$odbc_host;" . "DatabaseName=$odbc_dbname;" . "uid=$odbc_user; pwd=$odbc_pass"; $odbc_connect = odbc_pconnect($odbc_dsn, $odbc_user, $odbc_pass); echo $odbc_dsn,"<br>"; if ($odbc_connect <= 0) { echo "Connection failed."; exit; } else { echo "Connection successful."; }
-
I'm positive. I can query the database through Microsoft Access. I'm getting the following error: Warning: odbc_pconnect() [function.odbc-pconnect]: SQL error: [sYWARE, Inc.][Dr. DeeBee ODBC Driver]Unable to connect, SQL state 08001 in SQLConnect in C:\Program Files\Apache Software Foundation\Apache2.2\htdocs\admin\notifications.php on line 20 DRIVER={CSI RBM 4.02 ODBC Driver};CommLinks=tcpip(Host=rbm1;DatabaseName=\\rbm1\rbmnet\RBMsuite\CustData\RbmS\Example.rbm;uid=user; pwd=pass Connection failed. And the code up to that point: $odbc_host = 'rbm1'; $odbc_user = 'user'; $odbc_pass = 'pass'; $odbc_dbname = '\\\\rbm1\\rbmnet\\RBMsuite\\CustData\\RbmS\\Example.rbm'; $odbc_dsn = "DRIVER={CSI RBM 4.02 ODBC Driver};" . "CommLinks=tcpip(Host=$odbc_host;" . "DatabaseName=$odbc_dbname;" . "uid=$odbc_user; pwd=$odbc_pass"; $odbc_connect = odbc_pconnect($odbc_dsn, $odbc_user, $odbc_pass); echo $odbc_dsn,"<br>"; if ($odbc_connect <= 0) { echo "Connection failed."; exit; } else { echo "Connection successful."; } (Sensitive information has of course been replaced.)
-
No one knows how to do this?
-
Changed every single slash to double slashes... still not working.
-
I just tried this and no worky... $odbchost = '10.20.30.40'; $odbcuser = 'user'; $odbcpass = 'pass'; $odbcdbname = 'directories\example.rbm'; $dsn = "DRIVER={CSI RBM 4.02 ODBC Driver};" . "CommLinks=tcpip(Host=$odbchost;" . "DatabaseName=$odbcdbname;" . "uid=$odbcuser; pwd=$odbcpass"; $conn = odbc_connect($dsn, $odbcuser, $odbcpass); if ($conn <= 0) { echo "Connection failed."; exit; } else { echo "Connection successful."; } I've also tried changing "CSI RBM 4.02 ODBC Driver" to the actual location of the driver dll.
-
Another question... why would I want to use a MySQL driver when there are perfectly applicable commands for odbc? Like this: $dsn = "DRIVER={MySQL ODBC 3.51 Driver};" . "CommLinks=tcpip(Host=$db_host);" . "DatabaseName=$db_name;" . "uid=$db_user; pwd=$db_pass"; Why not just stick with ODBC? And when using odbc_connect or odbc_pconnect, do I have to use the same syntax as the code above?
-
Suppose there's a server with the IP address 10.20.30.40 containing ODBC with data I'd like to retrieve, and suppose the database is located within the directory "first_dir\second_dir\example\source.rbm." What's the command to connect and reach that source? I've read through php's manual and googled a few different things but I haven't quite found what I need. This is where I am: $conn = odbc_connect('10.20.30.40', $user, $pass); if ($conn <= 0) { echo "Could not connect."; exit; } else { echo "Connection successful."; } I've tried listing the directory after the IP and a few other variations. What's the syntax? I'll keep searching...
-
Haha just kidding! I always do this... I tried one more search string on google right after I posted this topic and found it. It's just <a name="section"> and then when linked use <a href="page.php#section">. And lol... I figured most here would know it off the top of their head and more people read this than the other. And registering for another forum just for html help takes too long.
-
...for immediately going to a named section on a page? I know it always involves a "#" character and then the name of the section but I can't remember the html syntax for naming the section. I've googled everything I could think of and to no avail since google ignores the "#" character. I know the html for the link is always something like <a href="some_page.php#section">... In advance, sorry to post in the wrong section! It's just that I know this one is read most frequently and I'd like an answer ASAP! <3