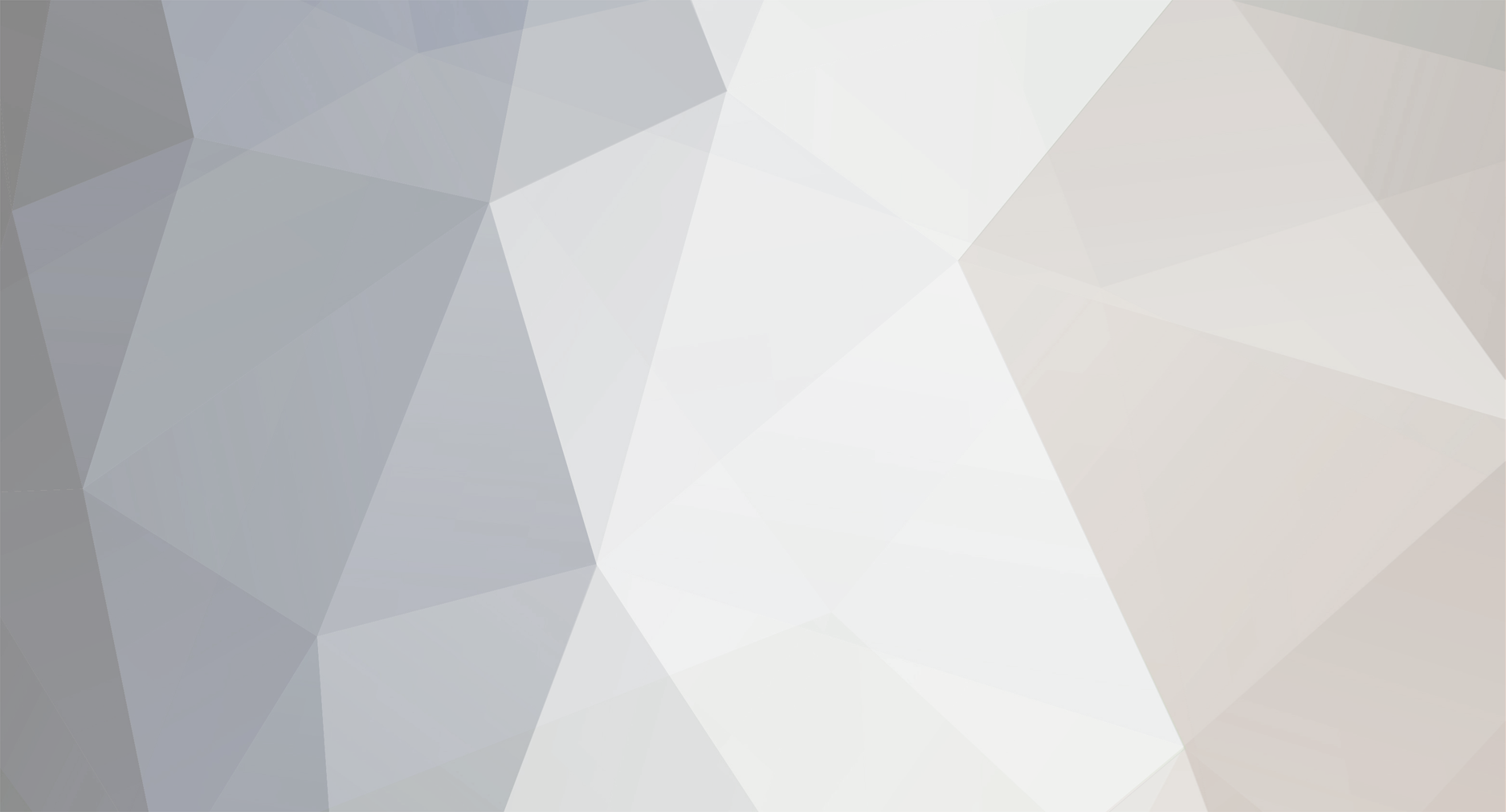
TransmogriBenno
-
Posts
61 -
Joined
-
Last visited
Never
Posts posted by TransmogriBenno
-
-
$mysql_rows=mysql_query("SELECT * FROM table"); $totalRows=mysql_num_rows($mysql_rows);
This is a really inefficient way to count the number of rows, at least try
SELECT COUNT(*) AS NumRows FROM table_name
In response to the OP, I would probably add a DATETIME column called LastUpdated, which you update when you process the rows. You can then set up a cron job to run your script every minute -- or continue down the meta refresh mode, if you want to rely on a browser to do the updates -- and when the script runs it gets the 20 (or however many) rows that have the oldest (or NULL) LastUpdated value.
-
The point of a class is to:
- encapsulate a set of data and the methods that will operate on it
- enforce strict controls on data
- support easy re-use and extension of existing code
There are lots of ways to use objects, the main one is as follows, creating your own data type that is used by several objects, each of which is an instance of the class:
class SillyString { private $str; function __construct ($value) { $this->str = $value; } function show () { echo '"', $this->str, "\"<br>\n"; } }
In PHP 5 there are few drawbacks (there is a very slight perfomance loss, but that's no drama). PHP 4 had a terrible object model.
-
I would say that it's a lot better than having a contact e-mail address displayed that can be parsed and stored in a spammer's database.
I've been putting contact forms on sites for years and haven't ever had a problem. What would be the point of a spammer sending stuff to the same person over and over, through a web form where the owner of the form knows exactly where the spammer came from?
-
How do you mean?
You control where the e-mail will be sent, so I'm not sure how anyone could send spam from it.
-
I like TinyMCE - http://tinymce.moxiecode.com/
You can configure it to do whatever formatting you like.
-
But each product is an object, so perhaps (I'm not familiar with vardump's output, and I'm assuming the class vars are public):
$this->products[$k]['product']->model
$this->products[$k]['product']->sku
In this code,
foreach($this->products as $k => $v){ for($i=0; $i < $this->products[$k]['quantity'] ; $i++){ $ups_rc->addProduct($prod); $usps_rc->addProduct($prod); } }
Where does $prod come from? Seems to be an error.
-
Try putting {} around the array element that's included in the string, i.e.
$datakey[$keys[$i]] = "<DATA:{$keys[$i]} />";
Also, assuming that's the end of the function, you're missing a closing brace. The indentation is pretty bad.
-
Perhaps.. the installer script for the package will probably do it for you, though.
-
You need the GD libraries, which are installed in various ways depending on your hosting environment. I compile them in.
-
When including a variable in a string, you should enclose it in {}. If the variable is an array element, you must enclose it. Also, you removed the quotes around the match term, which is an SQL string and needs the quotes.
So perhaps
AGAINST ( '{$_POST['search']}' ) ");
Instead of
AGAINST ( $_POST['search'] ) ");
-
You're using a POST form, when the original URL is a GET request.
file_get_contents should work just fine.
-
-
I hope you remembered to use mysql_query for the query I wrote there, I usually just write the query as a string so that people can use whatever wrapper functions they use to do the actual work. I use a hand-written one called execute_query, for example.
-
True that, whoops. It should read: tell your host to turn register_globals OFF, it's pure evil.
-
Perhaps the script is timing out - turn on error reporting and see what you get.
-
@DarkWater
I agree re: storing additional data in the session, under 2 conditions:
1. The other data needs to be displayed somewhere (it might not, I build a lot of systems in which this is the case) - best to store as little data in the session as possible.
2. The session is updated as soon as the row is updated by the user, e.g. if there's an "update my details" form - stale data is ugly.
There is also an issue if the actions of admins or others affect the situation, e.g. if an admin bans or deletes a user, you need to check the database every time they view a page, otherwise they can stay on until their session times out.
-
It seems that you haven't initialised $search and are relying on register_globals being on - this is bad practice.
Try using $_POST['search'] instead of just $search - and tell your host to turn register_globals OFF, it's pure evil.
-
Hidden form fields are awful; use sessions. You only need to store one value - the username, or whatever is the primary key of the table that stores the users - since you've already done the check against the database.
-
I think it's
SELECT * FROM posts WHERE date >= DATE_SUB(NOW(), INTERVAL 2 DAY);
Also, I highly recommend doing date comparisons either all in SQL or all in PHP, since the times (on a badly configured server) may be different in the two contexts. I've seen it happen before.
-
I don't think date is an appropriate solution to the problem, because the OP is looking for time elapsed, which isn't a timestamp value (it's the difference between two timestamp values).
If you want to use terms like 'years', 'months' etc. then you need a proper function that cares about how many days in each month, whether there are leap years, etc. If you just want days or weeks, then all you need to do is just divide the seconds into the relevant fields:
<?php $seconds_per_minute = 60; $seconds_per_hour = $seconds_per_minute * 60; $seconds_per_day = $seconds_per_hour * 24; $seconds_per_week = $seconds_per_day * 7; $weeks = floor ($uptime / $seconds_per_week); $uptime -= $weeks * $seconds_per_week; $days = floor ($uptime / $seconds_per_day); $uptime -= $days * $seconds_per_day; ... ?>
-
You can read the database into a tree and then traverse the tree to output it however you like.
e.g.
class TreeNode { private $parent; private $children; function __construct () { $this->parent = null; $this->children = array (); } function addChild (TreeNode $child) { $this->children[] = $child; $new_child->setParent ($this); } function setParent ($parent) { if ($parent !== null and !($parent instanceof TreeNode)) throw new Exception ('Invalid parent'); $this->parent = $parent; } }
The loading of nodes from the database is the fun part. Basically you loop through your set of rows and attempt to find parents for each node (remove each row from the set as it's added to the tree as a node). Obviously you have to set up a root somewhere. You count the number of children assigned to parents during each iteration of the loop. If it's ever zero, you should break out because there are orphan nodes, meaning your data or algorithm is flawed. The other exit condition would be when the number of nodes that are yet to be assigned to parents is zero.
-
for ($cntr = 0; $cntr < $num_rows_sect_mach; $cntr += 1) { echo " <input type=checkbox name=",$delete_field_mach[$cntr]," value=1> "; }
Should be this:
for ($cntr = 0; $cntr < $num_rows_sect_mach; $cntr += 1) { echo " <input type=checkbox name=\"delete_field_mach[{$cntr}]\" value=1> "; }
Also, you can group the IDs of the rows you want to delete in an array, then you only have to use one delete query, e.g.:
$delete_ids = array (); for ($cntr = 0; $cntr < $num_rows_sect_mach; $cntr += 1) { $delete_field_mach = (isset($_POST['delete_field_mach'][$cntr]) && $_POST['delete_field_mach'][$cntr] == '1')? 1 : 0; if ($delete_field_mach == '1') { $delete_ids[] = (int) mysql_result($result_field_desc_mach, $cntr); } } if (count ($delete_ids) > 0) { "DELETE FROM reports WHERE field_desc IN (". implode (', ', $delete_ids). ")"; }
-
Are the two values posted at the same time, or is the form re-loaded?
In the former case:
settype ($_POST['a'], 'int'); settype ($_POST['b'], 'int'); INSERT INTO table_name set field_name = {$_POST['a']}; INSERT INTO table_name set field_name = {$_POST['b']}; $result = $_POST['a'] + $_POST['b']; echo $result;
Of course, it's bad practice to echo on a POST form result page, it should just redirect somewhere.
In the latter case:
settype ($_POST['a'], 'int'); INSERT INTO table_name set field_name = {$_POST['a']}; SELECT SUM(field_name) AS Total FROM table_name; // fetch $row echo $row['Total'];
-
Also, if your variable names are two words, you should use_underscores or useCamelCaps (depending on your preference and the preference of those you share code with, I prefer underscores) so it's easier to read, and it also helps with spelling errors - $thisisnotanicevariablenameisit.
Also, I find enclosing variable names in {} to be much nicer on the eyes than switching in and out of strings and concatenating.
e.g.
"UPDATE odb_upload SET {$column_name} = '{$_POST[$i]}' ..."
rather than
"UPDATE odb_upload SET ".$column_name."='".$_POST[$i]."' ..."
It also means you can include array elements inside strings.
[SOLVED] MySql Order By
in PHP Coding Help
Posted
Not so, he is just missing string quotes around the field names, MySQL can do the summation work for him.
The reason I put the query into its own variable $q and made it echo is so you can see what the actual query you're running is. You can then plug that into a DB front-end (like PhpMyAdmin) to see what the result set is if need be.
Also, you should check out http://php.net/mysql_error