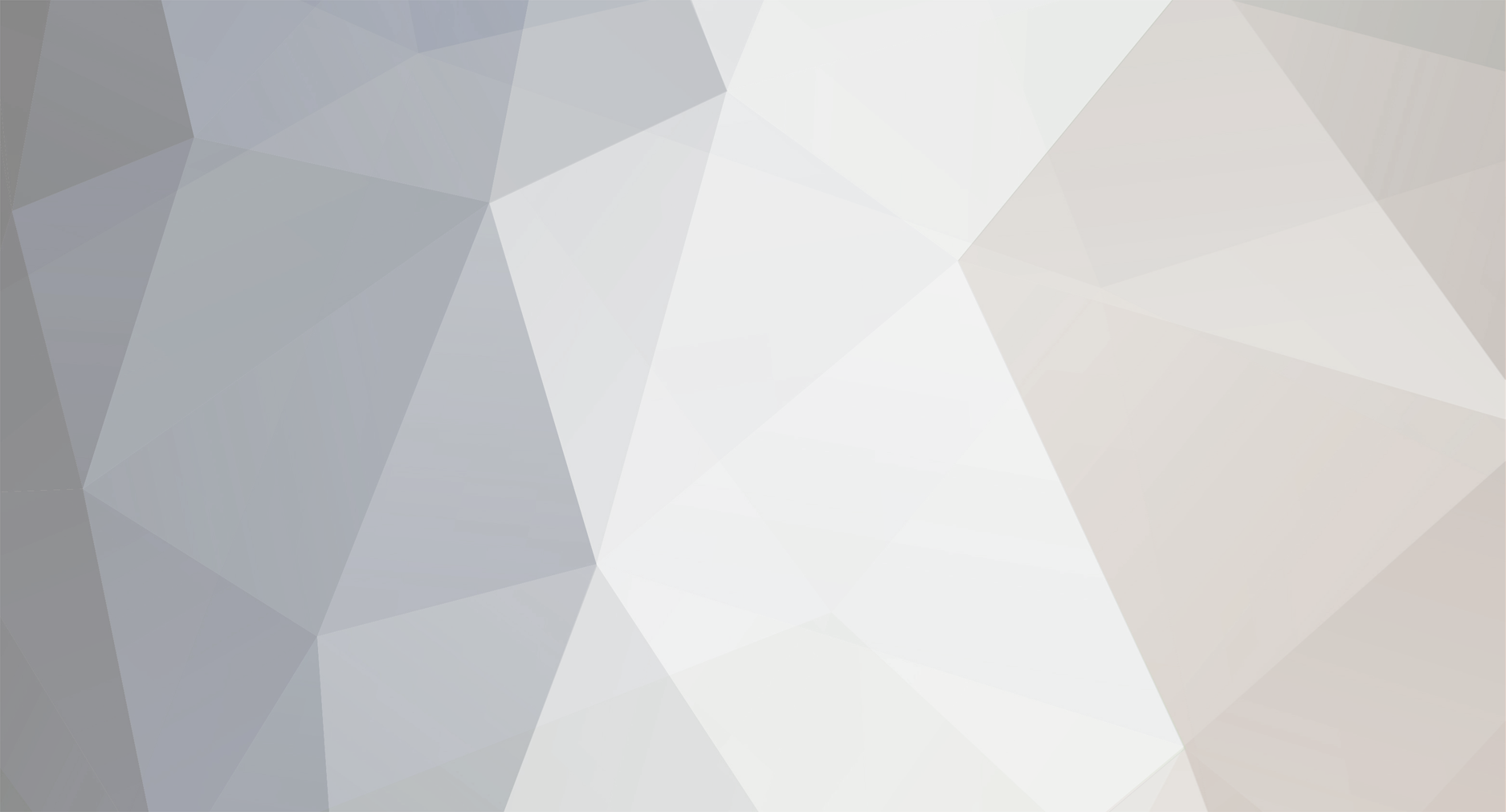
rmbarnes82
Members-
Posts
37 -
Joined
-
Last visited
Never
Everything posted by rmbarnes82
-
Hi, Your using the variable $i to mean two different things, and this is where your problem comes from. Your while loop runs the first time, when $i ==0. Then the for loop runs, after which $i==23 (you increment $i up to 23 in the for loop). Then you print the first activation code, then increment $i again, so now $i==24. The code jumps to the start of the while loop, but since $i is now 24 the $i < 18 fails, and the loop terminates. This code should fix your problem: <?php $i = 0; $allow = "abcdefghijkmnpqrstuvwxyz23456789"; while ($i < 18) { // Generates 8 Digit Activation Number srand((double)microtime()*1000000); for($y=0; $y<24; $y++) { $activation .= $allow[rand()%strlen($allow)]; } echo $activation . "<br />"; $i++; } Robin
-
Hi, Does anyone know of a php function to re-key an array with sequential integers starting at 0? For example, lets say the function to do this is called array_rekey(): <?php $myArray = array(3=>'a', 8=>'b', 102=>'c'); array_rekey($myArray); print_r($myArray); ?> I am of course assuming here that the function takes the array by reference, and so the ouput would be: Array ( [0] => 'a' [1] => 'b' [2] => 'c' ) array_fill_keys() won't work for this. If there isn't a function that already exists to do this, please ignore. Thanks, Robin
-
Hi, What database system are you using? I presume it's MySQL. If so, why are you using ids like 01 and 011. Why not use integer ids like 1, 2, 3, 4 which are sequential. If you use sequential ids, you can tell MySQL to auto increment the ids for you. So say the last entry has id of 11, when you insert a new one MySQL will automatically give the new row an id of 12. Robin
-
[SOLVED] my script work but some errors is displayed
rmbarnes82 replied to prince198's topic in PHP Coding Help
You'll get a reply quicker if you print the full error messages, and tell us which line in your code sample they relate to. -
Timestamp - Setting itself on load and again on submit
rmbarnes82 replied to TripleDES's topic in PHP Coding Help
Replace: if (!isset($_SESSION['tstamp'])) { session_start(); $_SESSION['tstamp'] = date('Ymd.His'); } with: session_start(); if (!isset($_SESSION['tstamp'])) { $_SESSION['tstamp'] = date('Ymd.His'); } You need to start the session before trying to see if the timestamp is set in it. Robin -
[SOLVED] Array - seaching inside serialised array
rmbarnes82 replied to williamZanelli's topic in PHP Coding Help
Hi, My mistake: SELECT * FROM my_table WHERE my_column LIKE %12%; Should have had quotes: SELECT * FROM my_table WHERE my_column LIKE "%12%"; However, this still won't work properly. You won't get an error, it's syntactically correct. Think, however, what "%12%" will match. You only want the number 12, but this also matches 121, 123, 1201, 512 ect, so rows without the number 12 in could be returned. To get it right the query you'd have to right would be really slow if run over a large number of rows. When you start to get errors like this, quick fixes become bug ridden nightmares, and the 'long' fix is actually much quicker then the 'quick' fix. -
Hi, When is crashes do you get a 'stack overflow' error by any chance? If so that means that you are infinitely recursing somewhere, probably here: $Data = (file_exists('./Templates/'.$Data)) ? $this->LoadFile($Data) : $Data; When using recursion, you must first determine your base case. This is a condition, which when met means you will stop recursing. If you don't have a base case, or the base case is never met, your program will infinitely recurse causing a crash. Robin
-
[SOLVED] Array - seaching inside serialised array
rmbarnes82 replied to williamZanelli's topic in PHP Coding Help
Hi, You asked if your entire approach to this was wrong, and it is. cooldude832 hit the nail on the head when he told you that your DB structure was wrong, and now I will go into some detail on how you can correct this. Ok, the problem is that you wish to store an arbitrary number of items in a single database table. As you have correctly observed, having one column for each item wont work as you don not know how many items there may be in the future. Also, although serializing and array into a single column, or imploding a comma separated list into a column may seem to work fine for updates / inserts it is very slow and verging on impossible to select based on an item in this column. Lets say you have a numeric, comma separated list in a column e.g. 2,12,45,192. Say you want to select all columns which have '12' in them: SELECT * FROM my_table WHERE my_column LIKE %12%; The above SQL will not work as desired. I will leave you to figure out why this is. Now for the proper way. Lets say we have a website selling TVs. The database schema for the TV table may look like this: tv ---- id title size price Each TV can have any number of options. These, at the current time include HD Ready Widescreen Flatpanel Remote Control However more options may be added later. We now need a second table, which we will call options. It could look like this: option --------- id title Our only problem now is that there is nothing to relate the two tables. We don't know which options are associated with which TVs. The two tables have what we call a 'many to many' or 'n to n' relationship with each other. This means a TV can relate to 0 or n options, and an option in turn can relate to 0 or n TVs. To achieve this we need a join table: tv_option ------------ tv_id option_id Note for this table there should be a dual primary key over each column in the table. This stops a tv having the same option more than once. Ok, now say a given tv with id 213 was HD ready(id 1) and widescreen (id 2), there would be the following entries in the tv_option table: tv_id option_id 213 1 213 2 to select all HD Ready TVs you'd do: SELECT t.* FROM tv t INNER JOIN tv_option o ON t.id = o.tv_id WHERE o.option_id = 1; Note I'm selecting based on option ID and not option title, as it's so much quicker and easier to search a database based on a numerical id as opposed to a string. This may mean you need some constants in you PHP cod so you have the option IDs to hand: define('OPTION_HD', 1); define('OPTION_WIDESCREEN', 2); But it does mean you would have to add constants each time you added items to the option table, so you may want to select on title rather than id. Don't just read up on all the possible MySQL commands, also learn about relational database theory (e.g. one to many relationships, many to many relationships, foreign keys etc.) . If you don't know this stuff you'll never be a great programmer, and will continually run into problems like this. -
Timestamp - Setting itself on load and again on submit
rmbarnes82 replied to TripleDES's topic in PHP Coding Help
Hi, I presume you are using a form which self submits (e.g. has a blank action and submits back to the same script), and so the timestamp is set 1) When the form loads 2) When the form is submitted. The solution to this is to check if the form has been submitted. If it has, don't bother setting the timestamp. For example: 1) Add a hidden field to your form which you will check in order to ascertain if the form has been submitted or not: <input type="hidden" name="postback" id="postback" value="1" /> 2) Then in your script check for the existence of the postback variable and only set the timestamp if the variable does not exist (e.g. the form has not been posted yet). if(!isset($_POST['postback'])) $timestamp = date('Ymd.His'); Please not I am assuming you are using a post form and not a get form. -
I've just started using Zend Framework for a personal project, and what I really like about it is it's modularity. This means if I don't like how any part of the framework works, I don't have to use that part of it. In addition to that it means that it isn't too hard to use some of Zend's classes in other frameworks, or indeed without actually using a framework at all. It does look like it may be hard to do certain things though. Zend is MVC based which means I should easily be able to do certain things, for example: have an xml (for rss) or JSON (for ajax) view instead of an HTML one, and have the same controller be able to switch between different view types, say based upon some parameter given in the HTTP Request. I have cast my eye over Symfony and it looks good, this may be the framework I start using in my day job (I don't get to make the decision about this). One framework I have used professionally in projects I have inherited is CakePHP. I do not like this framework. The view and controller side of things seems ok, but how they handle the model is awful. I think there is a huge misconception within the PHP community that the model should be a type of object, rather than just a layer of your application. This is shown in Cake by the fact the model classes all extend the same model base class. This gives the impression of separate 'models', when the model should be a collection of classes that combined model the business logic of your application, and give it permanence (e.g. via the database). I would actually go so far as to have the model layer split into two separate sub layers: the persistence layer and the logic layer. The logic layer may be aware that the persistence layer exists, but does not care about the details of it. Also the model in Cake and similar frameworks assumes I will be using a database to persist items from my model, and the model classes seem very tightly coupled to the idea that there is a one to one mapping between a database table and a model. I may, however, want to use a view which spans multiple tables and not a single table, not sure if Cake allows me to do this easily. Further still, what happens if part (or all) of my database doesn't use a database for persistence at all. These days with Google providing things like blogs via RSS / Atom and web services providing functionality like mailing lists, using a web service as a persistence layer instead of a database isn't so much of an edge case anymore, so frameworks need to be able to handle these things effortlessly. People may say that this is not what MVC is for, but the opposite is true. MVC is meant to allow me to easily switch between different types of Models, Views and Controllers. This is one of the main purposes of MVC. Robin