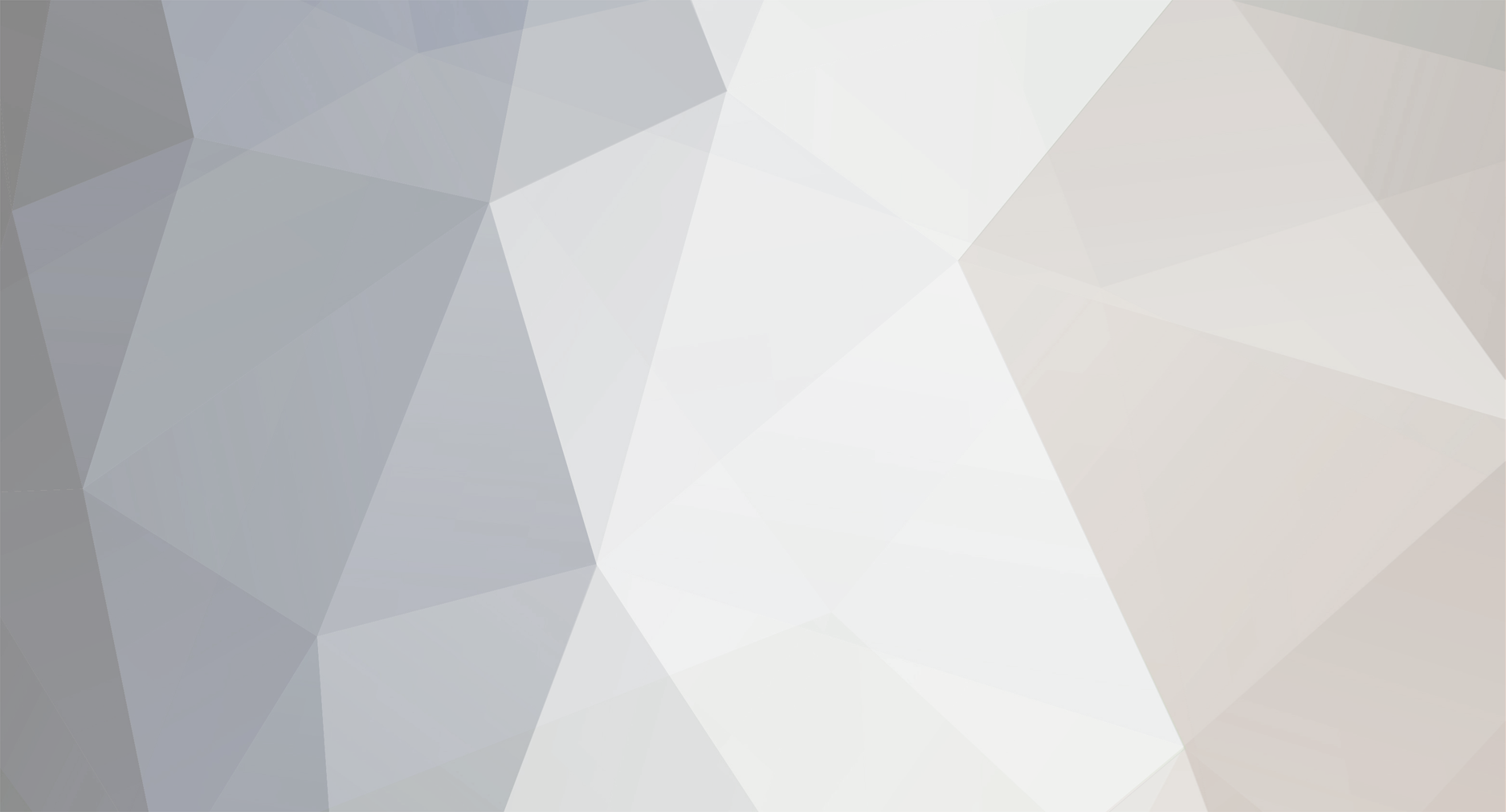
needs_upgrade
Members-
Posts
126 -
Joined
-
Last visited
Everything posted by needs_upgrade
-
Hi guys! I feel ashamed because my client complained that there are inconsistencies in the system that i've developed. He said that some of the figures in the system do not tally with the actual value in their stock room. The system my follow the FIFO rule and i suspect the problem is how i implemented it. Here are the details how i did it: CREATE TABLE IF NOT EXISTS `purchases` ( `purchase_id` int(10) unsigned NOT NULL auto_increment, `or_num` varchar(10) collate latin1_general_ci NOT NULL, `supplier_id` int(4) unsigned NOT NULL default '0', `user_id` int(2) unsigned NOT NULL default '0', `delivery_date` date NOT NULL default '0000-00-00', `delivery_cost` decimal(7,2) unsigned NOT NULL default '0.00', `received_by` int(2) NOT NULL, `notes` varchar(255) collate latin1_general_ci NOT NULL, `line_total` decimal(13,3) unsigned NOT NULL default '0.000', `balance` decimal(13,3) unsigned NOT NULL default '0.000', `pmode` int(1) unsigned NOT NULL COMMENT '1 if cash; 2 if check', `cancelled` int(1) NOT NULL, PRIMARY KEY (`purchase_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci ; CREATE TABLE IF NOT EXISTS `purchase_details` ( `purchase_detail_id` int(15) unsigned NOT NULL auto_increment, `purchase_id` int(10) unsigned NOT NULL, `product_id` int(5) unsigned NOT NULL, `quantity` decimal(8,3) unsigned NOT NULL, `unit_price` decimal(10,3) unsigned NOT NULL default '0.000', `unit_discount` decimal(8,3) unsigned NOT NULL default '0.000', `net_price` decimal(13,3) NOT NULL, `balance` decimal(8,3) NOT NULL, PRIMARY KEY (`purchase_detail_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci ; CREATE TABLE IF NOT EXISTS `sales` ( `sale_id` int(10) unsigned NOT NULL auto_increment, `or_num` varchar(10) collate latin1_general_ci NOT NULL, `customer_id` int(4) unsigned NOT NULL default '0', `user_id` int(2) unsigned NOT NULL default '0', `delivery_date` date default '0000-00-00', `due_date` date NOT NULL, `delivery_cost` decimal(7,2) default '0.00', `received_by` varchar(150) collate latin1_general_ci NOT NULL, `notes` varchar(255) collate latin1_general_ci default NULL, `line_total` decimal(13,3) unsigned NOT NULL default '0.000', `balance` decimal(13,3) unsigned NOT NULL default '0.000', `revenue` decimal(10,3) NOT NULL, `pmode` int(1) unsigned NOT NULL COMMENT '1 if cash; 2 if check', `cancelled` int(1) NOT NULL COMMENT '1 if cancelled; 2 if draft', PRIMARY KEY (`sale_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci ; CREATE TABLE IF NOT EXISTS `sale_details` ( `sale_detail_id` int(15) unsigned NOT NULL auto_increment, `sale_id` int(10) unsigned NOT NULL, `purchase_detail_id` int(15) unsigned NOT NULL, `product_id` int(5) unsigned NOT NULL, `acq_price` decimal(10,3) NOT NULL, `quantity` decimal(7,2) unsigned NOT NULL, `unit_price` decimal(10,3) unsigned NOT NULL default '0.000', `unit_discount` decimal(8,3) unsigned NOT NULL default '0.000', `net_price` decimal(13,3) NOT NULL, PRIMARY KEY (`sale_detail_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci ; i have added the "purhchase_detail_id" field in the "sale_details" table because it is foreign key to the "purchase_detail_id" in the "purchase_details" table. here's the code i use in processing the sale (taking the products from the purchase_details table); function Add2Sale($product_id, $quantity, $sale_id, $unit_price, $unit_discount, $net_price) { // while quantity requested is not zero while ($quantity > 0) { // get the oldest purchase in the inventory $sql = "SELECT DATEDIFF(p.delivery_date, NOW()) AS diff, pd.purchase_detail_id, pd.unit_price - pd.unit_discount, pd.balance FROM purchases p, purchase_details pd WHERE p.purchase_id=pd.purchase_id AND pd.balance > 0 AND pd.product_id='$product_id' ORDER BY diff LIMIT 0, 1"; $res = mysql_query($sql); $num = mysql_num_rows($res); if ($num > 0) { $row = mysql_fetch_array($res); // information about the purchase of the product $purchase_detail_id = $row[1]; $acq_price = $row[2]; $bal = $row[3]; if ($quantity <= $bal) { // get the changes in purchae_detail after the insert of sale_detail $new_bal = $bal - $quantity; $net_price = $quantity * ($unit_price - $unit_discount); mysql_query("INSERT INTO sale_details(sale_id, purchase_detail_id, product_id, acq_price, quantity, unit_price, unit_discount, net_price) VALUES('$sale_id', '$purchase_detail_id', '$product_id', '$acq_price', '$quantity', '$unit_price', '$unit_discount', '$net_price')") or die(mysql_error()); mysql_query("UPDATE purchase_details SET balance='$new_bal' WHERE purchase_detail_id='$purchase_detail_id' LIMIT 1") or die(mysql_error()); // quantity requested will be zero $quantity = 0; } else { // insert the sale detail and update the purchase_detail balance to zero $net_price = $bal * ($unit_price - $unit_discount); mysql_query("INSERT INTO sale_details(sale_id, purchase_detail_id, product_id, acq_price, quantity, unit_price, unit_discount, net_price) VALUES('$sale_id', '$purchase_detail_id', '$product_id', '$acq_price', '$bal', '$unit_price', '$unit_discount', '$net_price')") or die(mysql_error()); mysql_query("UPDATE purchase_details SET balance=0 WHERE purchase_detail_id='$purchase_detail_id' LIMIT 1") or die(mysql_error()); // update the remaining balance of quantity $quantity = $quantity - $bal; } } else { $quantity = 0; } mysql_free_result($res); } } // end here's how i show the values in the inventory: $sql = "SELECT p.product_id, CONCAT(p.product_name,' ',p.description), m.manufacturer_id, m.manufacturer_name, p.sell_price FROM products p, manufacturers m WHERE p.manufacturer_id = m.manufacturer_id ORDER BY $sortby LIMIT $from, $max_results"; $getlist = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_array($getlist)) { print "<tr> <td align=left><a class=tddata href='product.php?product_id=$row[0]'>$row[1]</a></td> <td align=left><a class=tddata href='manufacturer.php?manufacturer_id=$row[2]'>$row[3]</td> <td align=right>"; $sell = number_format($row[4], 2); print "$sell</td>"; $sql = "SELECT SUM(pd.balance), SUM((pd.unit_price - pd.unit_discount) * pd.balance), p.reorder_level FROM purchase_details pd, products p WHERE pd.product_id = '$row[0]' AND pd.product_id = p.product_id GROUP BY pd.product_id"; $pres = mysql_query($sql); $prow = mysql_fetch_array($pres); print "<td align=right>$prow[0]</td> <td align=right>"; $gval = number_format($prow[1], 2); print "$gval</td> </tr>"; } Would you guys be kind to criticize my approach. can you recommend better approaches? or maybe you can give me some sources or links. Thank you very much guys.
-
How to get local machine details with php
needs_upgrade replied to needs_upgrade's topic in PHP Coding Help
Even the details of the machine of the server??? -
How can i get the local machine details with php? Is it possible to get the key number of the OS or the ID of the processor or other parts of the local machine? Thanks so much guys.
-
Making a software Trial Version
needs_upgrade replied to needs_upgrade's topic in Application Design
thanks a lot ngreenwood6. that's what i really need. -
Making a software Trial Version
needs_upgrade replied to needs_upgrade's topic in Application Design
Yes, I am talking about a web application. Specifically, I'm using PHP and MySQL. -
Hello guys! One of my clients suggested that I should be making trial versions of my work to attract more clients. An my concern is how to LIMIT piracy. Would you guys be kind to give me some insights? These are what i have in mind: 1. A function that will delete some php codes (add / del / edit / etc) upon expiration of the software. (But what if someone deleted the function before it expires?) 2. A function that will delete the tables in the MySQL DB (Again, someone might delete the function) 3. Is there a way to limit access from a database after expiration? I know that there is no way to stop piracy, but is there a way to limit it? Most of my clients are medical doctors (most of them are not into programming). I am hoping for some insights/suggestions. Thanks and more power to you guys.
-
Working with images AND MySQL for a Dental Application
needs_upgrade replied to needs_upgrade's topic in Application Design
I dont know much about javascript, is it possible to change the color of each square? Would you be kind to give me some sources? Thanks so much corbin. Thank you daniel as well. -
Hello Guys! My client have shown me a chart she made wherein she can easily see all the teeth and their conditions. There are several kind of teeth and have different numbers of divisions/areas. Each division/area can bee in good condition, bad(damaged/decaying), coated or missing. I can easily do it if information for each tooth will be text-based. But my client wanted to include the chart in the system so that it will be much easier on her part. What came into my mind is to save images representing each condition of the tooth. But there would be many combination. Where should i start? Can you give me any idea on how to do it? Although i have started scanning about php image processing and zend drawing
-
Hello guys. I have a string of characters in my mysql table (together with the newlines, multiple spaces, etc). I wanted to show the strings in HTML exactly how the user entered it. I used ereg_replace to replace '\n' with '<br>', multiple spaces with ' ' and tab with five ' '. My problem is with '\n'. It omits the letter n followed by the character new line. How should i do it? thanks guys
-
Table design for hotel reservation system
needs_upgrade replied to needs_upgrade's topic in Application Design
thanks cooldude for your modifications. but still, it depends upon the business rule of the hotel. some hotels require payment for reservations and if you dont check in on time, service is considered sold. i find mysql date functions very useful, actually. they are very helpful in report generation. -
Table design for hotel reservation system
needs_upgrade replied to needs_upgrade's topic in Application Design
thanks for the reply guys. -
Hi guys! Assuming that i already have the room table, how should i design the table for room resevations? this is what i have in mind.... CREATE TABLE room_reservations ( room_reservation_id INT(6) NOT NULL AUTO_INCREMENT, room_id INT(4) NOT NULL, check_in DATE NOT NULL, check_out DATE NOT NULL, PRIMARY KEY(room_reservation_id) ); Would this work? For example, if room#1 has a reservation from january 15 - 20, that room#1 will not be showed if i search for any rooms available for january 17. or if i have selected room#1, it will show the days it is available thnx guys
-
what if the user checked many boxes, how will i be able to know which purchase_ids have been checked/selected?
-
Hello guys! I'm not used to checkboxes and I have this code: $sql = "SELECT purchase_id, or_num, DATE_FORMAT(delivery_date, '%b %e, %Y'), line_total, balance FROM purchases WHERE supplier_id='$supplier_id' AND cancelled=0 AND balance > 0 ORDER BY purchase_id DESC"; $sres = mysql_query($sql); $snum = mysql_num_rows($sres); if ($snum > 0) { print "<center><table class=data width=400 border=1><thead class=data>"; print "<tr><td align=center colspan=5>Payable Purchases From Supplier</td></tr>"; print "<tr> <td align=center width=30></td> <td align=center width=70>O.R. #</td> <td align=center width=100>Delivery Date</td> <td align=center width=100>Line Total</td> <td align=center width=100>Balance</td> </tr></thead><tbody class=data>"; while ($srow = mysql_fetch_array($sres)) { print "<tr> <td align=center><input type=checkbox name=pid value=$srow[0]></td> <td align=center><a class=tddata href='purchase.php?purchase_id=$srow[0]'>$srow[1]</a></td> <td align=center>$srow[2]</td> <td align=right>"; $linet = number_format($srow[3], 2); print "$linet</td> <td align=right>"; $bal = number_format($srow[4], 2); print "$bal</td> </tr>"; } } print "<tr><td align=center colspan=5> <input type=hidden name=supplier_id value='$supplier_id'> <input type=submit value=Submit></td></tr>"; print "</tbody></table></form></center>"; My question is, how can i catch all the purchase_ids when i click the submit button? Thanks so much guys.
-
Hello guys! I just wanted to know what are the advantages of VB.net over VB and/or vice versa.... Another question: if in php we can do this: <PHP? $sql = "SELECT product_id, product_name, product_description FROM products ORDER BY product_name"; $res = mysql_query($sql); while ($row = mysql_fetch_array($res)) { print "<tr> <td><a href='product.php?product_id=$row[0]'>$row[1]</a></td> <td>$row[2]</td> </tr>"; } ?> how can i do it with vb/vb.net? i've heard that i can use the datagrid. but i dont know how to do it. thnx for any help guys. more power to you all.
-
I've done this before... call a javascript function that refreshes the page and adds a variable on the URL.
-
How can I make PHP and Javascript work TOGETHER?
needs_upgrade replied to needs_upgrade's topic in Javascript Help
I haven't dealt with it. I've been searching for some related code but i can't find any. would you be kind to refer me to any tutorial related to my problem? thnx -
Hello guys! I have a dropdown box name wherein i can select between products and i have two uneditable text boxes. How can i make php and javascript work together so that upon onChange on the dropdown box, the product's selling price and quantity available will be automatically populated on the two uneditable text boxes? I don't have any problem with SQL statements only on the part where php will pass the data to javascript. Please help or refer me to any tutorial. I've been stucked here for several days now. Thank you guys in advanced. More power to you all.
-
Hello guys! I need help in working javascript and php together. I have these two tables in my MySQL database: CREATE TABLE `products` ( `product_id` int(10) unsigned NOT NULL auto_increment, `product_code` varchar(10) NOT NULL, `product_name` varchar(30) NOT NULL, `selling_price` decimal(10,2) unsigned NOT NULL default '0.00', PRIMARY KEY (`product_id`) ); CREATE TABLE `purchase_details` ( `purchase_detail_id` int(15) unsigned NOT NULL auto_increment, `purchase_id` int(10) unsigned NOT NULL, `product_id` int(5) unsigned NOT NULL, `quantity` decimal(7,2) unsigned NOT NULL, `unit_price` decimal(10,3) unsigned NOT NULL default '0.000', `unit_discount` decimal(8,3) unsigned NOT NULL default '0.000', `net_price` decimal(13,3) NOT NULL, `balance` decimal(8,2) NOT NULL, PRIMARY KEY (`purchase_detail_id`) ); And in my php file named add_sale, i have this form: $show_form = "<form name='AddSaleForm' action='add_sale.php' method='post'> <table class=data border=1><thead class=data>"; $show_form .= "<tr><td align=center colspan=4><b>Product Details</b></td></tr>"; $show_form .= "<tr> <td align=center>Product Name</td> <td align=center>Selling Price</td> <td align=center>Qty Available</td> <td align=center>Qty To Be Sold</td> </tr></thead><tbody class=data>"; for ($i=1; $i<21; $i++) { $show_form .= "<tr> <td align=left><select name='product_id$i' onChange=\"Populate_selling_price_and_quantity_available()\"><option value=>Product Name</option>"; // select only the products that are non-zero in values in the inventory $sql = "SELECT p.product_id, p.product_name FROM products p, purchase_details pd WHERE pd.product_id = p.product_id AND SUM(pd.balance) > 0 ORDER BY p.product_name"; $res = mysql_query($sql); while ($row = mysql_fetch_array($res)) { show_form .= "<option value=$prow[0]>$prow[1]</option>"; } $show_form .= "</select></td> <td align=center><input readonly=1 type=text class=TextBox size=10 name='selling_price$i'></td> <td align=center><input readonly=1 type=text class=TextBox size=10 name='quantity_available$i'></td> <td align=center><input type=text class=TextBox size=3 name='quantity_2_b_sold$i' onblur=\"check_if_quantity_2_b_sold_IS_NOT_GREATER_THAN_quantity_available()\"></td> </tr>"; } $show_form .= "<tr><td align=center colspan=4> <input type=reset> <input onClick=\"EvalAddSaleForm()\" type=button value=Submit></td></tr>"; $show_form .= "</tbody></table></form>"; print $show_form; What i need is: 1. If I have selected a product in the drop down menu named product_id, the Populate_selling_price_and_quantity_available() function will a. show the selling price in the uneditable selling_price textbox and b. show the quantity available in the uneditable quantity_available textbox. // the sql for showing the quantity available: "SELECT SUM(balance) FROM purchase_details WHERE product_id = '$selected_product_id_from_drop_down_menu'"; 2. If i have entered a value in the quantity_2_b_sold textbox, the check_if_quantity_2_b_sold_IS_NOT_GREATER_THAN_quantity_available() function will automatically check that its value is not greater than the value in the uneditable selling_price textbox Thank you very much in advanced guys. More power to you all.
-
[SOLVED] Develop a site with PAYPAL trainsaction
needs_upgrade replied to needs_upgrade's topic in MySQL Help
thnx vicodin. -
Develop a site with PAYPAL trainsaction
needs_upgrade replied to needs_upgrade's topic in Miscellaneous
Thanks dude! More power to you. -
Hello everyone! I am planning to develop a site that offers ambulatory care. Health packages for men/women/children are being offered with respective prices. How can i make a function that would allow PAYPAL transact with the customer and paypal will be able to insert into my transactions table (MYSQL ofcourse) the vital information about the customer (example: payment date, payment method, amount paid, package bought, REFERENCE NUMBER, etc any help that would lead me to solving my problem is highly appreciated. thank you all
-
Hello everyone! I am planning to develop a site that offers ambulatory care. Health packages for men/women/children are being offered with respective prices. How can i make a function that would allow PAYPAL transact with the customer and paypal will be able to insert into my transactions table the vital information about the customer (example: payment date, payment method, amount paid, package bought, REFERENCE NUMBER, etc any help that would lead me to solving my problem is highly appreciated. thank you all