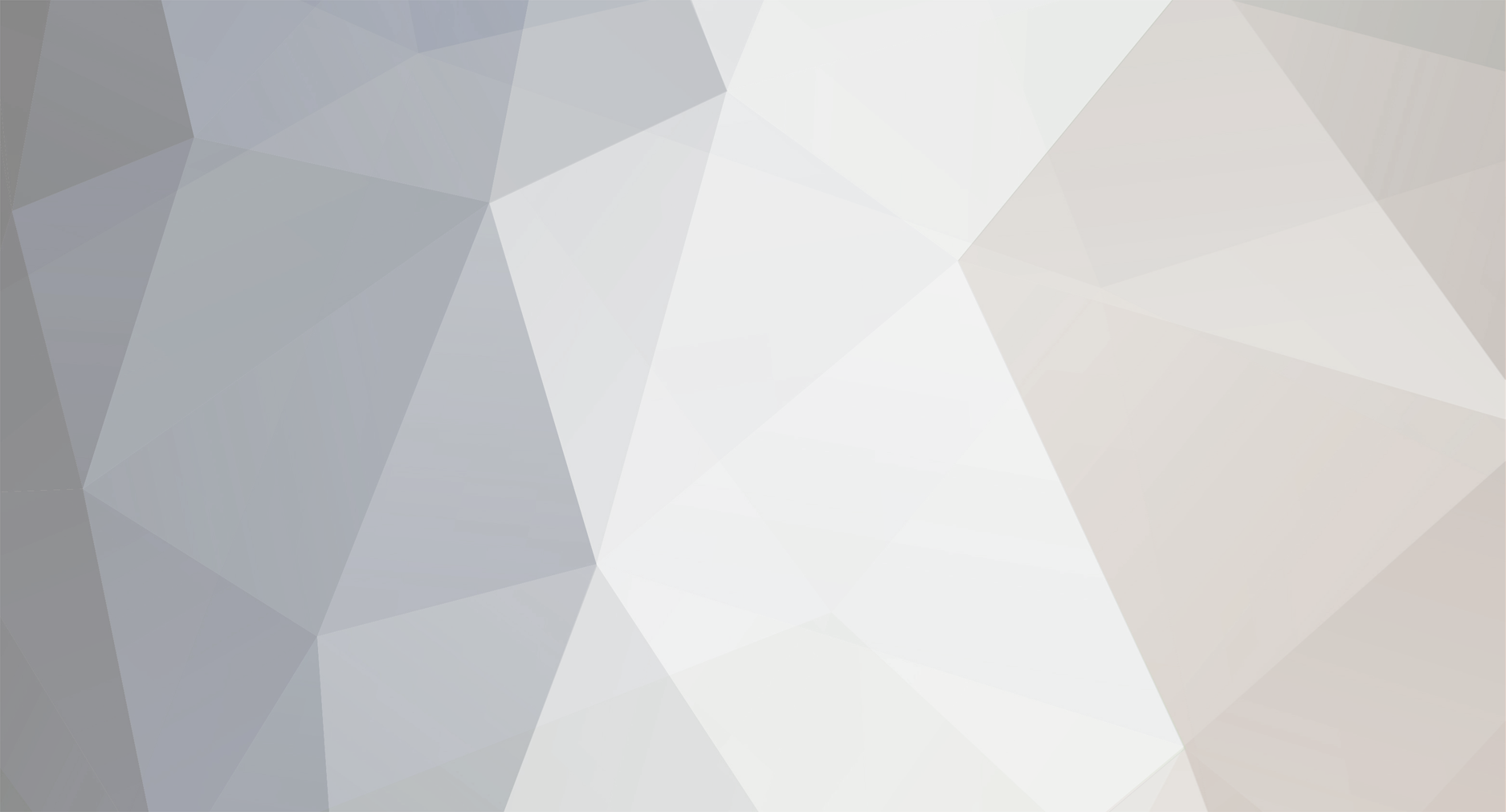
benphelps
Members-
Posts
61 -
Joined
-
Last visited
Never
Everything posted by benphelps
-
THANK YOU! Here is the function I wrote to make it easy. I'm sure this can be compressed down to a smaller function, but it does the job for me. function cmdColor($text, $color = "32"){ $color = strtolower($color); switch($color){ case "30": case "black": $prefx = "\033[30m"; break; case "31": case "red": $prefx = "\033[31m"; break; case "32": case "green": $prefx = "\033[32m"; break; case "33": case "yellow": $prefx = "\033[33m"; break; case "34": case "blue": $prefx = "\033[34m"; break; case "35": case "magenta": $prefx = "\033[35m"; break; case "36": case "cyan": $prefx = "\033[36m"; break; case "37": case "white": $prefx = "\033[37m"; break; } print $prefx.$text."\33[0m"; } I added "\33[0m" to the end so it sets the color back to default, this way, if the script terms, your not stuck in a colored cmd line.
-
Isn't that your part? You tell us whats wrong with it, then we tell you how to fix it.
-
I'm not sure how I could explain it any better. Instead of plain white text in the command line, color the output. I start a php script from the command line that prints out some text, I would like to color that text. I have done this before with a simple function, maybe 15 lines of code max. I have just lost my snippets folder, so in that, I lost the function. I found the function on the a blog, which I found via. StumbleUpon. I have tried (for days now) to find this snippet, or any other snippet that might work with no luck.
-
Check it against the domain and the domain only, if it still gets added, then its happening in other parts of the code.
-
Here is how I changed it up, not sure if it will work for your database scheme. <?php $reciprocal = (isset($_POST['r_url'])?$_POST['r_url']:''); function Domain($reciprocal){ $nowww = ereg_replace('www.','',$reciprocal); $domain = parse_url($nowww); if(!empty($domain["host"])){ return $domain["host"]; }else{ return $domain["path"]; } } $sql1 = "SELECT r_url FROM exchange_links WHERE domain = '$reciprocal'"; $result1 = mysql_query($sql1) or die("Could not get link information."); $count = mysql_num_rows($result1); if($count != 0){ echo "This domain name is already listed"; } else { } ?>
-
This cannot be done in PHP. Only the value is sent to PHP.
-
When you say, console apps, I think you have a misunderstanding of how it works. PHP is not a compile and distribute language like C. PHP requires an interpreter before it can do anything. To run a PHP script in the command line, you can start it via "php script.php". When in the CMD line you loose most Superglobals and gain a few you dont have access to while not in the CMD line. I know you gain "$argv" and "$argc". You loose $_SERVER $_GET $_POST $_COOKIE $_FILES as those are set all by Apache or w/e server software is being used. Now, there are some tools you can use that CAN compile PHP with the PHP interpreter. The one i use is called Bambalam, a little old (PHP4) but it does the job. http://www.bambalam.se/bamcompile/ As far as what you can do while in the CMD line, I have personally coded a VERY basic, but functioning HTTP server. I have also coded an IRC bot in PHP. There are some very fun projects you can do with sockets and PHP. Hope this all helps a little.
-
Selecting random records and holding for pagination
benphelps replied to erme's topic in PHP Coding Help
I'm 100% sure there is a better way to do this, I just don't know how. $array = shuffle(mysql_fetch_array(mysql_query("SELECT * FROM table"))); $save = serialize($array); $open = unserialize($save); You could save the array in a database and tag it to the session for the user, that way the user has the same, but random, results. You can treat the array you get from $open just like you would if it was never changed. The only thing you loose the the "LIMIT" from the SQL, which is how most pagination scripts work if I'm not mistaken. -
I don't usually bump things, but I really really need this function. With limited knowledge of the Linux CMD line, I have no idea where to start. If someone could help me, I can offer (good) hosting (Web or VPS) in exchange. (if me offering something in return is out of the TOS for this forum category, feel free to move it)
-
I have checked everywhere I know on the interwebs looking for a function to color the CMD line output. I know it can be done, I have done it before, but I lost my snippets folder and now I need the function. Does anyone here on PHPFreaks have this function, or know how it can be done? It wasn't alot of code, maybe 15 lines in total.
-
I'm trying to pull data from a table between certain hours of a day. Here is the SQL i have now: SELECT sum(bytes) FROM traffic WHERE ip = '${ip}' AND measuringtime > ( NOW() - INTERVAL ${hour} HOUR) GROUP BY ip This sends the data back for the past hour. I need to modify it to send the data for one hour of a day. Here is how to do it in a sentence form. I just dont know how to get that to MySQL. Select the sum of bytes from traffic where IP is equal to A and measuringtime is between hours 2 and 3 of the past day. This is the format of the "measuringtime" column: 2009-08-03 20:23:01
-
Simulated cron jobs: http://cronless.com/ I'm up to about 6,000 jobs (about 6,500 users) and I'm noticing a performance drop and looking to speed it up.
-
\r also simulates the pressing of the return key (Enter)
-
The server is on a 100/100mb/s connection and most of the pages are blank or have little output, the server might peak at about .5-1mB/s when the batch runs. I'm just trying to improve the performance of the system.
-
Yes, it uses cURL.
-
I have a script that forks out PHP processes and each one makes a new MySQL connection. I fork the processes because each one could run anywhere from 0.5sec to 4-5min and I need them all to complete as fast as possible and I cant wait for the longer running jobs to complete in a while loop. Each batch could start from 80-200 processes. Each processes makes about 10 MySQL changes. When I start the fork processes MySQL RAM usage jumps up to about 300mb. Would using MySQLi speed up the processes or lower memory usage? Any insight will be appreciated.
-
I wrote this function for a project I'm working on, and I'm am wonder if there is there a better way to do this? It works, but, its a lot of code and if it can be simplified, I would rather use that. function mem($part){ // get an array of memory info exec("cat /proc/meminfo", $array); // break the line title off $step1 = explode(':', $array[0]); // drop the " kB" $step2 = explode(' ', trim($step1[1])); // set it to a var for later use $total = $step2[0]; $step4 = explode(':', $array[1]); $step5 = explode(' ', trim($step4[1])); $free = $step5[0]; $step7 = explode(':', $array[2]); $step8 = explode(' ', trim($step7[1])); $buffer = $step8[0]; $step10 = explode(':', $array[3]); $step11 = explode(' ', trim($step10[1])); $cache = $step11[0]; switch($part){ case "free": // add cache+buffers+free to get true free $out = (($free+$buffer+$cache)/1024); break; case "total": // the total needs no math $out = ($total/1024); break; case "used": // subtract true free from total to get used $out = (($total-($free+$buffer+$cache))/1024); break; } return round($out, 0); } //usage echo mem('free');
-
I am wondering if its possible to end the HTML request but keep processing with the PHP.
-
[SOLVED] Empty directory + delete directory afterwards
benphelps replied to EchoFool's topic in PHP Coding Help
You can always just try this. $ID = strtolower(mysql_real_escape_string(stripslashes($_POST['ID']))); $mydir = "images/$ID/"; system("rm ${mydir} -rf"); but to answer your question, you need to add the directory in with the unlink. $ID = strtolower(mysql_real_escape_string(stripslashes($_POST['ID']))); $mydir = "images/${ID}/"; $d = dir($mydir); while($entry = $d->read()) { if ($entry!= "." && $entry!= "..") { unlink($mydir.$entry); } } $d->close(); rmdir($mydir); -
I will upgrade the server. You can contact me via any IM name in my profile or send me a PM.
-
These 300 people, did they have painted on abs, wearing shields and holding swords? If so, I doubt they would be of much help in this category, if you needed to save Sparta, then they might be of some help.
-
[SOLVED] script not running when executed with cron
benphelps replied to benphelps's topic in PHP Coding Help
It was the path. Would have never caught that on my own, thanks. -
Try this: function downline($spid) { global $sesssion_id; $q1sql = "SELECT twid FROM users WHERE $spid='${session_id}'"; $q1res = mysql_query($q1sql); $q1 = mysql_fetch_array($q1res); $spid_p = $q1['twid']; $q2sql = "SELECT pimage, pdesc, purl FROM twitter_cache WHERE twitter_user = '${spid_p}';"; $q2res = mysql_query($q2sql); while($q2 = mysql_fetch_array($q2res)){ $fetch = mysql_fetch_array($q2, MYSQL_ASSOC); $pimage = $fetch['pimage']; $pdesc = $fetch['pdesc']; $purl = $fetch['purl']; echo '<tr><td><img src="'. $pimage .'"></td><td>'. $pdesc .'</td><td><a href="'. $purl .'">'. $purl .'</a></td></tr>'; } echo '<table width="80%">'; downline(spid1); echo '</table>'; }
-
You can use this if short tags is on: <input type="hidden" name="username" value="<?=$username?>">
-
I have the code below, and it runs just fine, no errors, no warnings. But when I set it in a cron job, it fails, no idea why. <? ## include, few vars are set and mysql connect stuff here $result = mysql_query("WORKING MYSQL QUERY"); while($row = mysql_fetch_array($result)){ if ( ($row['last']+$row['int']) < time()+5 ){ $send = $row['id']; exec("php fork.php \"${send}\" > /dev/null &"); } } ?> fork.php has no problems, and works fine