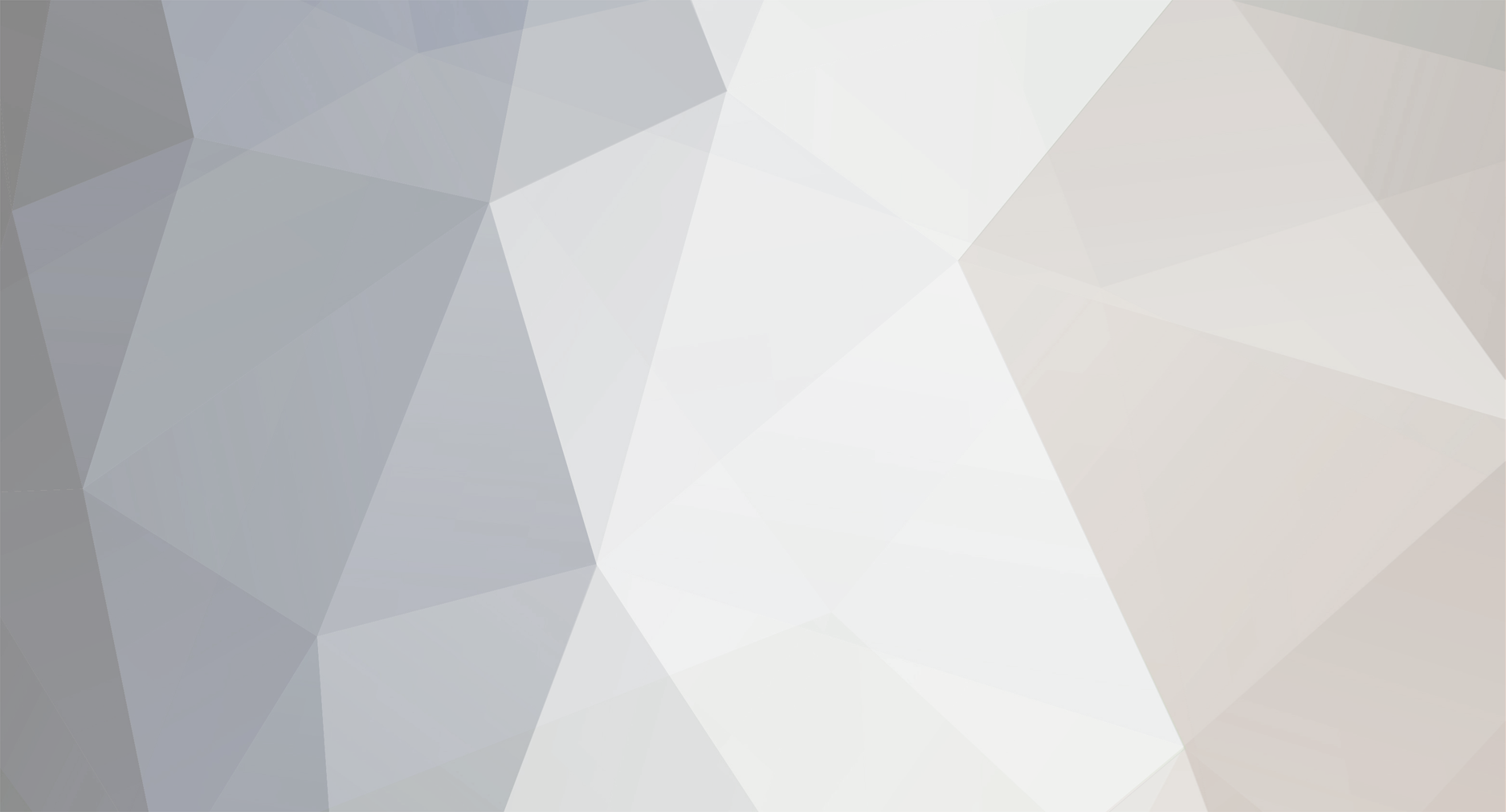
ecopetition
Members-
Posts
101 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
ecopetition's Achievements

Member (2/5)
0
Reputation
-
Hey all, I'm making a little game that contains lots of individual phases - when phase one is complete, it advances to phase two, then three, and so on, until a condition is met that ends the game and starts a new one. I'm using the following jQuery AJAX to refresh the page that the game is played on if a certain condition is met (update() checks if it's time for the next phase, and refresh() does it if is): <script> function refresh() { $.ajax({ type: 'POST', url: 'index.php', data: 'refresh=true', timeout: 0, success: function(data) { $("#current_game").html(data); }, error: function (XMLHttpRequest, textStatus, errorThrown) { $("#notice_div").html('Error contacting server. Retrying in 60 seconds.'); window.setTimeout(update, 60000); } }); }; function update() { $.ajax({ type: 'POST', url: 'check_time.php', data: 'checktime=true', timeout: 0, success: function(data) { $(".time_remaining").html(data); window.setTimeout(update, 1000); var time = data; if(time<=0) { $(".time_remaining").html("Reloading the page now."); $(".time_remaining_end").html("Reloading the page now."); refresh(); } else { $(".time_remaining").html("There are "+data+" seconds remaining in this phase." ); $(".time_remaining_end").html("There are "+data+" seconds until the next game." ); } }, error: function (XMLHttpRequest, textStatus, errorThrown) { $("#notice_div").html('Error contacting server. Retrying in 60 seconds.'); window.setTimeout(update, 60000); } }); }; $(document).ready(update); </script> Now, I'm caching the game parameters. The game parameters change each time the phase advances, so I need to delete that cache file, cache_game.php. I was trying to do this by deleting the cache everytime the phase_up() function is called, using the (extremely stripped down for clarity) script (which works with my $cache class): <?php function phase_up() { global $db, $cache; if(condition1) { ...; } else { if(condition2) { ...; } else { ...; exit; } $sql = "UPDATE games SET phase_details = ... WHERE game_id = ..."; $db->query($sql); } $cache->delete('game'); } ?> Yet when my AJAX calls this through index.php, it doesn't advance the phase. Manually refreshing the page does advance the phase. The AJAX just tells index.php to call phase_up(). Any help provided would be invaluable, this has been driving me crazy for hours!
-
Hi, I'm relatively new to ajax so am wondering if someone can point me in the direction of a tutorial for what I want to do: I have a page index.php, which calls a function that determines parameters for the display on the body of the page. These parameters change every couple of minutes, so a new page body is generated regularly. Is it possible for a user to be viewing the page in the browser and have the browser constantly checking if a new body has yet been generated, and is this a PHP-intensive process, when hundreds of users could be doing the same thing at once? Many thanks
-
Thanks for your reply thorpe, but this function returns the difference in the arrays, how would one use that to see if it's a subarray of the parent array? I read the notes for the function and didn't get anything similar to what I want, also Googled and it seems to have very few results for "subarray". Thanks again.
-
Hi, I've checked the PHP manual and Googled this but can't find anything that assists me. Does anybody know if there exists a function issubset($childarray, $parentarray) that returns true if $childarray is a subset of $parentarray? That is, if all the elements in $childarray are included in $parentarray. Many thanks
-
Hi all, I have the following array, which represents option_id => number_of_votes Array ( [3] => 4 [5] => 5 [2] => 11 ) Is it possible to look at the value side of the array and neglect the smallest values, and pick a vote winner? Also, if we get the case: Array ( [3] => 4 [5] => 11 [2] => 11 ) can we pick a random winner between option 5 and option 2? Thanks so much for all your help, let me know if more information will be useful.
-
Well that's the thing, I'm not receiving any errors, my success function runs, but the PHP doesn't. I don't see where I've gone wrong, I posted my code in the hope you can help out.
-
Could you help me with why this script doesn't work: jQuery: $(function() { $('.error').hide(); $('.failure').hide(); $('.success').hide(); $(".submit").click(function() { if (!$("input[@name='name']:checked").val()) { $('.error').show(); return false; } var vote_radio = $("input.vote_radio").val(); var dataString = 'vote='+ vote_radio; $.ajax({ type: "POST", url: "index.php", data: dataString, success: function() { $('.success').show(); $('.failure').hide(); }, error: function() { $('.failure').show(); $('.success').hide(); } }); return false; }); }); PHP: if(isset($_POST['vote'])) { $vote_id = htmlspecialchars($_POST['vote']); $insert_sql = $db->build_query('INSERT', array( 'game_id' => $game_id, 'vote_for' => $vote_id, 'ip_address' => $_SERVER['SERVER_ADDR'], 'time' => time() )); $sql = "INSERT INTO votes $insert_sql"; $db->query($sql); } Thanks again
-
I must admit that I'm completely lost. Why doesn't this work (in index.php): if(isset($_POST['submit'])) { $vote_id = htmlspecialchars($_POST['vote']); register_vote($vote_id); } Thank you
-
Thanks all. Now, I have a final question. I'm a newbie with Ajax, so I'm wondering, what code do I need in index.php that starts the processing of the form data? Thank you again
-
Thanks, it looks more promising, but it produces a value of 1 for every radio option?
-
Hello, I'm needing some help with the following HTML, it's a simple voting script: <form name="vote_form" action=""> <input type="radio" name="vote_radio" id="vote_radio" value="1"/>Option 1<br/> <input type="radio" name="vote_radio" id="vote_radio" value="2"/>Option 2<br/> <input type="radio" name="vote_radio" id="vote_radio" value="3"/>Option 3<br/> <div class="error">You must select an option to vote for.</div> <input type="submit" name="submit" class="submit" id="submit" value="submit" /> </form> I'm looking to submit the script using Ajax (and jQuery), and am looking to use the following code: <script> $(function() { $('.error').hide(); $(".submit").click(function() { if (!$("input[@name='name']:checked").val()) { $('.error').show(); return false; } var vote_radio = ????; var dataString = 'vote='+ vote_radio; $.ajax({ type: "POST", url: "index.php", data: dataString, success: function() { alert ('Done.'); }); } }); return false; }); }); </script> It goes without saying that this script doesn't work. What I can't figure out is how to define the radio button that's been selected (vote_radio), so the dataString fails. Can anyone help? Thanks a lot.
-
Can anybody help?
-
How do I apply this when the condition is "trapped" within an array?
-
Hey, I have the following two arrays, of which I want to use bits of each from to get another array. Array 1: Array ( [0] => Array ( [shop_id] => 1 [shop_name] => Aldi [shop_district] => 6 ) [1] => Array ( [shop_id] => 2 [shop_name] => Greggs [shop_district] => 6 ) [2] => Array ( [shop_id] => 3 [shop_name] => Tesco [shop_district] => 6 ) [3] => Array ( [shop_id] => 4 [shop_name] => Morrisons [shop_district] => 6 ) [4] => Array ( [shop_id] => 5 [shop_name] => Ryman [shop_district] => 6 ) [5] => Array ( [shop_id] => 6 [shop_name] => Boots [shop_district] => 6 ) [6] => Array ( [shop_id] => 7 [shop_name] => Superdrug [shop_district] => 6 ) [7] => Array ( [shop_id] => 8 [shop_name] => City [shop_district] => 2 ) [8] => Array ( [shop_id] => 9 [shop_name] => Lizard [shop_district] => 6 ) [9] => Array ( [shop_id] => 10 [shop_name] => Asda [shop_district] => 8 ) [10] => Array ( [shop_id] => 11 [shop_name] => Tesco [shop_district] => 2 ) [11] => Array ( [shop_id] => 12 [shop_name] => Tesco [shop_district] => 8 ) ) Array ( [0] => Array ( [shop_id] => 1 [shop_name] => Aldi [shop_district] => 6 ) [1] => Array ( [shop_id] => 2 [shop_name] => Greggs [shop_district] => 6 ) [2] => Array ( [shop_id] => 3 [shop_name] => Tesco [shop_district] => 6 ) [3] => Array ( [shop_id] => 4 [shop_name] => Morrisons [shop_district] => 6 ) [4] => Array ( [shop_id] => 5 [shop_name] => Ryman [shop_district] => 6 ) [5] => Array ( [shop_id] => 6 [shop_name] => Boots [shop_district] => 6 ) [6] => Array ( [shop_id] => 7 [shop_name] => Superdrug [shop_district] => 6 ) [7] => Array ( [shop_id] => 8 [shop_name] => City [shop_district] => 2 ) [8] => Array ( [shop_id] => 9 [shop_name] => Lizard [shop_district] => 6 ) [9] => Array ( [shop_id] => 10 [shop_name] => Asda [shop_district] => 8 ) [10] => Array ( [shop_id] => 11 [shop_name] => Tesco [shop_district] => 2 ) [11] => Array ( [shop_id] => 12 [shop_name] => Tesco [shop_district] => 8 ) ) Array 2: Array ( [0] => Array ( [sUM(item_price)] => 31.23 [item_shop] => 1 ) [1] => Array ( [sUM(item_price)] => 1.65 [item_shop] => 2 ) [2] => Array ( [sUM(item_price)] => 41.23 [item_shop] => 3 ) [3] => Array ( [sUM(item_price)] => 7.98 [item_shop] => 4 ) [4] => Array ( [sUM(item_price)] => 4.49 [item_shop] => 7 ) ) I'd love if anyone could tell me how I can generate an array that will combine bits of these two arrays, so that using the first array I can get a shop_name and shop_district for each item_shop (aka shop_id). Then I want to get an array with entries that look something like this: [0] => Array ( [sUM(item_price)] => 31.23 [shop_name] => Aldi [shop_district] => 6 ) Thanks so much for your help, it means a lot to me.