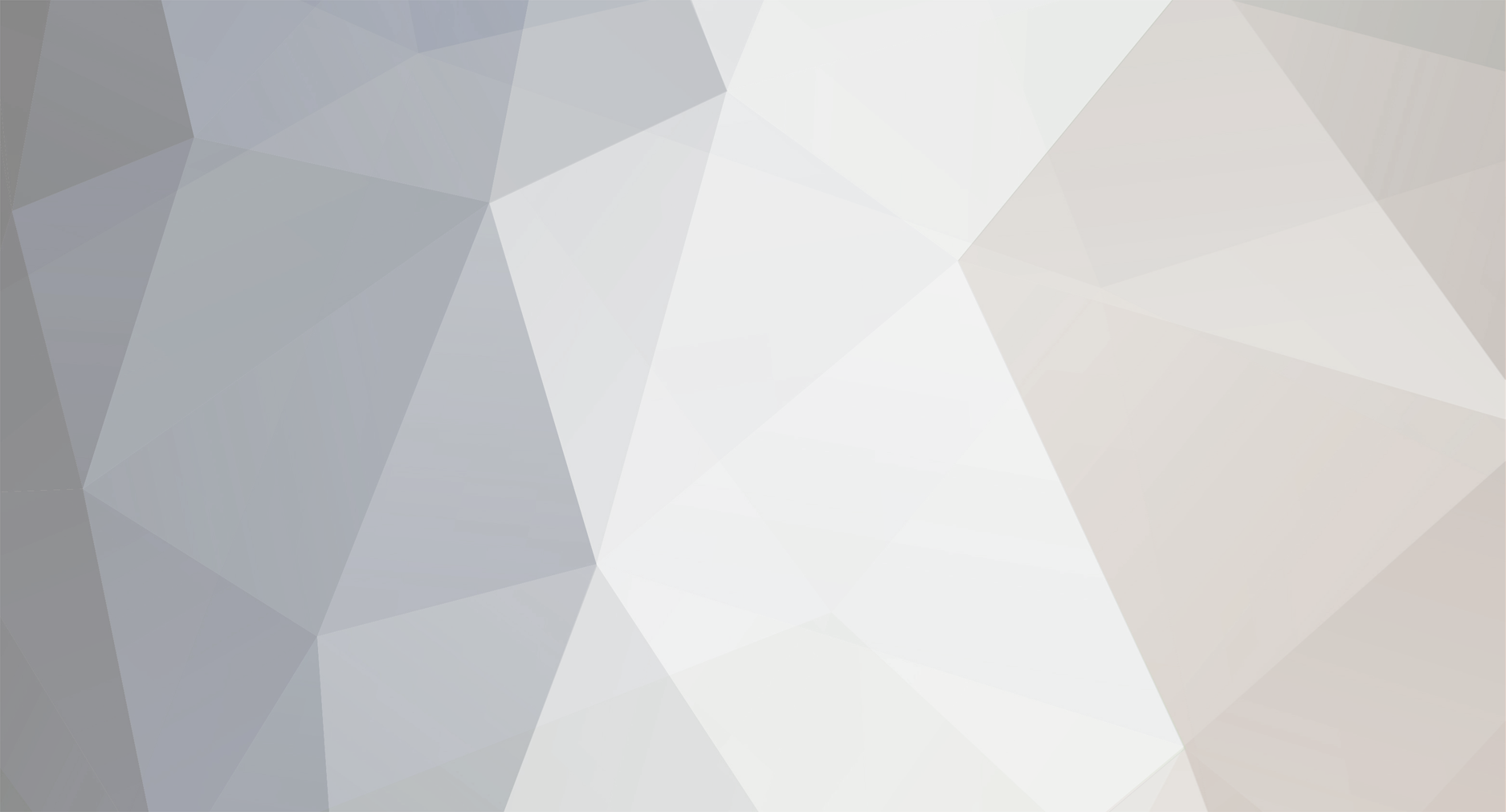
Benjigga
Members-
Posts
11 -
Joined
-
Last visited
Never
Everything posted by Benjigga
-
Here is a link to screenshots of what is happening, because I'm crappy at describing things: http://imgur.com/a/ahvPA Of course I've messed the code up somewhere, but I can't for the life of me figure out where. I have a simple page for someone to enter text in a textbox to save in a database to display on another page. There are 10 textboxes on the page. I can enter text into any and all textboxes and it'll save the data in the database, EXCEPT if I enter text into the 2nd textbox. If there's anything in the 2nd textbox after I click submit, it will delete whatever was in the 2nd textbox and move all data in each textbox (except textbox 1, which stays in the same place) down one element. And it pushes the data in the final textbox off the page (presumably because there's no other textbox to accept its data). Here is the code to the two pages affected. Any help would be appreciated! <?php // start the session session_start(); if (!session_is_registered($_SESSION['myusername'])) { header ('location:./login.php'); } $i=1; require_once('./dbconnect.php'); $sql="SELECT `event` FROM `events`"; $result=mysql_query($sql); mysql_close(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <title>Admin Portal</title> </head> <body> <form name="events" method="post" action="eventlogging.php"> <table width="800" border="0" align="center" cellpadding="0" cellspacing="1" bgcolor="#CCCCCC"> <tr> <td> <table width="100%" border="0" cellpadding="3" cellspacing="0" bgcolor="#FFFFFF"> <tr> <td colspan="3" bgcolor="#CCCCCC"> <strong><p><strong>Admin Portal</strong></p><p align="right"><small><a href="./logout.php">Logout</a></small> </td> </tr> <?php while ($i<11) { echo ' <tr align="center"> <td align="right" width="100">Event ' . $i . ' </td> <td width="800"> <textarea name=event' . $i . ' cols=40 rows=5>'; $row = mysql_fetch_array($result); if ($row) { $msg=$row[0]; echo $msg; }; $i++; echo '</textarea> </td> </tr> '; } ?> <tr> <td colspan="2"> <center><input type="submit" name="Submit" value="Submit" /></center> <input type="hidden" name="submitted" value="true" /> </td> </tr> </td> </tr> </table> </td> </tr> </table> </form> </body> </html> <?php $position = 1; $dbControl = 9; $event = array(); if (!empty($_POST['event1'])) { $event[] = $_POST['event1']; } if (!empty($_POST['event2'])) { $event[] = $_POST['event']; } if (!empty($_POST['event2'])) { $event[] = $_POST['event']; } if (!empty($_POST['event3'])) { $event[] = $_POST['event3']; } if (!empty($_POST['event4'])) { $event[] = $_POST['event4']; } if (!empty($_POST['event5'])) { $event[] = $_POST['event5']; } if (!empty($_POST['event6'])) { $event[] = $_POST['event6']; } if (!empty($_POST['event7'])) { $event[] = $_POST['event7']; } if (!empty($_POST['event8'])) { $event[] = $_POST['event8']; } if (!empty($_POST['event9'])) { $event[] = $_POST['event9']; } if (!empty($_POST['event10'])) { $event[] = $_POST['event10']; } include ('./dbconnect.php'); mysql_query("DELETE FROM `events`"); foreach ($event as $msg) { mysql_query("INSERT INTO `events` (`position`, `event`, `date`) VALUES ('$position', '$msg', curdate() )") or die(mysql_error()); $position++; } mysql_close(); $position=1; /* foreach ($event as $msg) { echo $position . '. ' . $msg . '<br />'; $position++; } */ header("location:./admin.php"); ?>
-
!= worked for this but == didn't. Can someone please explain?
Benjigga replied to Benjigga's topic in PHP Coding Help
Example1: if (sql == 0) { Do 1 } else { Do 2 } In the first code, it was practically like the example above. In the second code, where I changed == to != I changed it to: Example2: if (sql != 0) { Do 2 } else { Do 1 } Understand? It worked with example 2, but not example 1. And I was just wondering if anyone could explain why? -
!= worked for this but == didn't. Can someone please explain?
Benjigga replied to Benjigga's topic in PHP Coding Help
Thanks for the heads up. I understand != means Not Equal To and == means Equal To, I just don't understand why in this situation Not Equal To worked and Equal To didn't? -
I'm making a registration page. I'm trying to get it to double check to see if an email address has already been submited. The == would let double email entries into the database. Here is the code that would let double entries in. $query = "SELECT user_id from USERS where email='$e'"; $result = mysql_query($query); if (mysql_num_rows($result) == 0) { $query = "INSERT INTO users (first_name, last_name, email, password, registration_date) VALUES ('$fn', '$ln', '$e', SHA('p'), NOW() )"; $result = @mysql_query ($query); if ($result) { echo '<h1 id="mainhead">Thank you!</h1> <p>You are now registered.</p><p><br /></p>'; include ('./includes/footer.inc'); exit(); } } else { echo '<h1 id="mainhead">Error!</h1> <p class="error">The email address has already been registered.</p>'; } Now here's what I changed it to and it stopped letting duplicate email addresses register. All I did was change == to != and switch the else statment with the if statement. Here it is. $query = "SELECT user_id from USERS where email='$e'"; $result = mysql_query($query); if (mysql_num_rows($result) != 0) { $query = "INSERT INTO users (first_name, last_name, email, password, registration_date) VALUES ('$fn', '$ln', '$e', SHA('p'), NOW() )"; $result = @mysql_query ($query); if ($result) { echo '<h1 id="mainhead">Error!</h1> <p class="error">The email address has already been registered.</p>'; } } else { echo '<h1 id="mainhead">Thank you!</h1> <p>You are now registered.</p><p><br /></p>'; include ('./includes/footer.inc'); exit(); } Can someone please explain to me why == wouldn't work but != did? I can't understand this. Thank you much!
-
[SOLVED] Syntax error while doing a tutorial in a book.
Benjigga replied to Benjigga's topic in MySQL Help
Those singlequotes were confusing me too when I was going through the book. The " ', ', " I'm assuming it's to add a comma between the last and first name. And that "SELECT CONTACT" is supposed to be "SELECT CONCAT" -
I'm going through Larry Ullman's PHP and MySQL book and I've written this script twice identical to how he has it in the book, but I keep getting a syntax error! I am using MySQL 5.0. Here is the error: You have an error in your SQL syntax. Check the manual that corresponds to your MySQL server version for the right syntax to use near '(last_name, first_name) AS name, DATE_FORMAT (registration_date Query: SELECT CONTACT (last_name, first_name) AS name, DATE_FORMAT (registration_date, '%M %d, %Y') AS dr FROM users ORDER BY registration_date ASC $query = "SELECT CONTACT(last_name, ', ', first_name) AS name, DATE_FORMAT (registration_date, '%M %d, %Y') AS dr FROM users ORDER BY registration_date ASC"; $result = @mysql_query ($query);
-
Okay, I see what you mean. This is obviously an HTML issue. Sorry for the mis-placed post. Quickly though- isn't there a clickable index to distribute things on certain "levels" of the page? I thought it was like "vindex="1"" or something. Anyone know what I'm talking about and can someone correct me in the name of it please?
-
I'm going through a tutorial in a book I have and I've created a really basic site. But the menu on the right side someimtes only half of each link is available to be clicked (like when you're at the "Home" link only the letter "r" of the "Calculator" is able to be clicked). www.kidsparkonline.com/index.php Please tell me if this is only my browsers problem or is anyone else experiencing this. If so, anyone have any idea how to fix such an issue? v/r Ben
-
Yes, this was exactly what I was looking for, thanks a lot!
-
I've read through a few tutorials and two books and I haven't found anything to explain this. What is the difference between the function $POST and $_POST? What is the purpose of the underscore? As far as I cal tell, $POST is for storing data and $_POST is for retrieving? Can someone please tell me if I'm right?