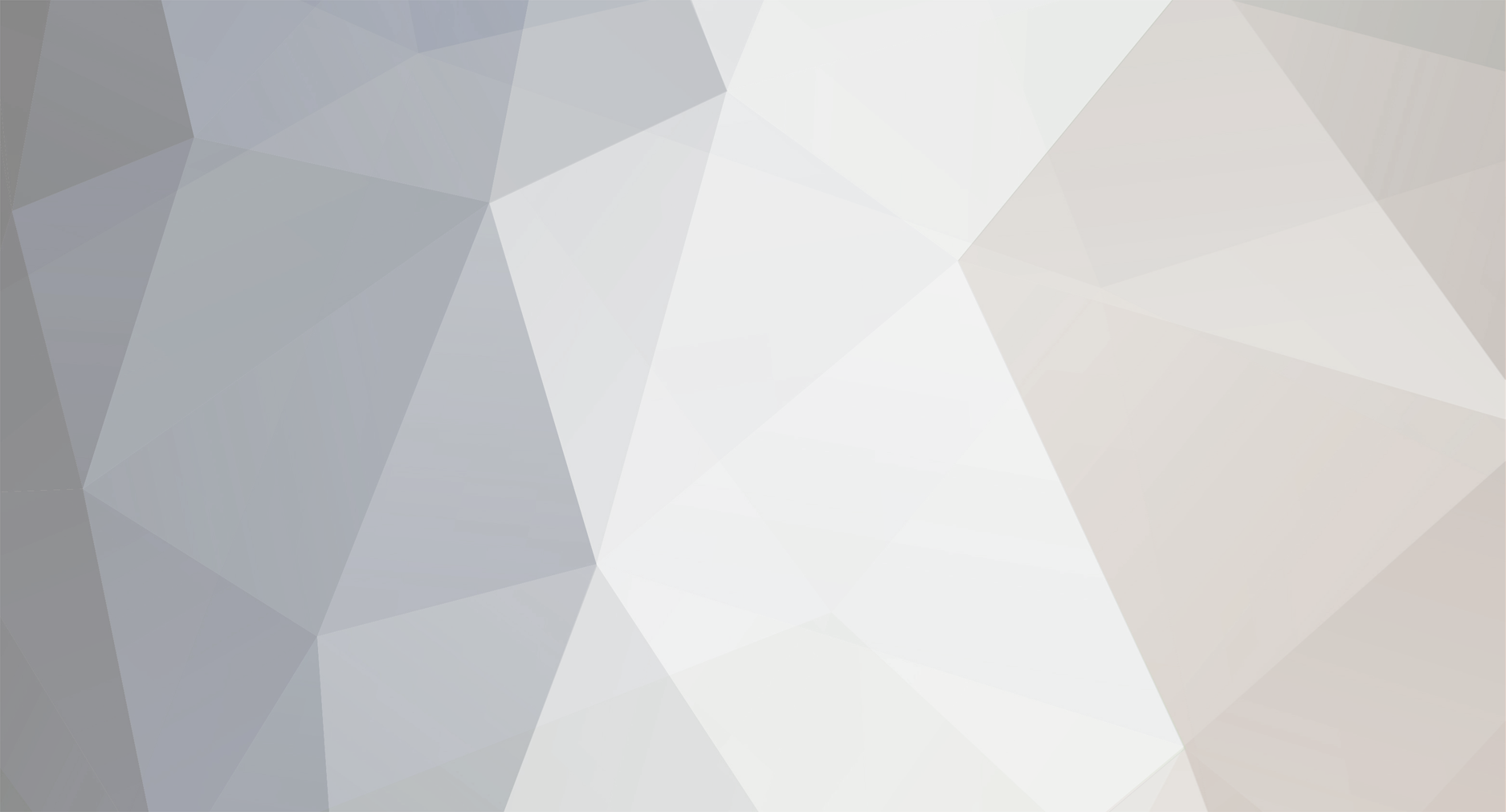
gaza165
-
Posts
472 -
Joined
-
Last visited
Never
Posts posted by gaza165
-
-
<?php session_start(); header("Expires: Mon, 26 Jul 1987 05:00:00 GMT"); // Date in the past header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); header("Cache-Control: no-store, no-cache, must-revalidate"); // HTTP/1.1 header("Cache-Control: post-check=0, pre-check=0", false); header("Pragma: no-cache"); include ('dbconnect.php'); $nick = $_GET['nick']; $message = $_GET['message']; $lastid = $_GET['lastid']; if ($lastid == 0) { $sql = "SELECT MAX(chat_id) FROM chat"; $res = mysql_query($sql); $lastid = mysql_fetch_row($res); $lastid = $lastid[0]; } $time_start = microtime(true); $sql = "SELECT * FROM chat WHERE chat_id > $lastid AND nick != $nick"; $result = mysql_query($sql); $time_end = microtime(true); $time = ($time_end - $time_start); $sql = "SELECT MAX(chat_id) FROM chat"; $res = mysql_query($sql); $lastid = mysql_fetch_row($res); $lastid = $lastid[0]; while ($row = mysql_fetch_array($result)) { $data = array('id'=>$row['chat_id'] , 'nick'=>ucfirst($row['nick']), 'message'=>$row['message'], 'time'=>$time); } $json = '{"latest":"'.$lastid.'","response":['; $json .= json_encode($data); $json .= ']}'; echo $json; $get = "SELECT * FROM chat"; $result = mysql_query($get); $numrows = mysql_num_rows($result); ?>
-
From my knowledge of MD5 encryption it always encrypts to a password 32 characters long.... in your database structure is your password field VARCHAR(32) in your registration table???
If your registration password field is 20 chars, you need to change it to hold 32 chars
-
<?php $time_start = microtime(true); $sql = "SELECT * FROM chat WHERE chat_id > $lastid"; $result = mysql_query($sql); $time_end = microtime(true); $time = ($time_end - $time_start); ?>
Im using this code to bring back records from my shoutbox, what i would like to do...when the user posts a message for it to be displayed to them straight away, and then bring it back from the database.
So what I am trying to do is write an SQL statement that brings back all the other messages apart from the ones that are submitted by the user user that is logged in.
Can anyone help me. thanks
Garry
-
Hi all,
I have been working on a shoutbox for ages, from the feedback i have been getting is that the problem with it is that messages take to long to be displayed.
Currently
1.) Users write a message and send it.
2.) It then is added to the database.
3.) Every 3 seconds a call to a php file called ajaxgetshoutbox.php is called to get the last message posted.
4.) This data is then put into JSON format.
4.) This is then outputted to the screen.
Obviously i cant put to much pressure on the server by posting a request to the database every second.
Have i gone about this all the wrong way?
Is there a way to improve responsivness of message sending??
If anyone would like to look at the code, please ask. Or you can view it live at http://shoutbox.thedesignmonkeys.co.uk
thanks
Garry
-
Would be better if we knew where the page is going to so we can give a better solution...
-
or something like
<select onChange="window.open('http://www.google.com','Google','width=400,height=200')">
-
You could always run two different queries??
-
Hi all,
I need to come up with an encryption alrogithm for a given password.. the thing is, i cant use the crypt function.
What is the best way to go about this.
thanks
Garry
-
well you can simply replace the space in your url with a + sign
using str_replace
<?php $url = "http://www.domain.com/blue widget"; $url = str_replace(" ","+",$url); echo $url; // http://www.domain.com/blue+widget ?>
-
http://shoutbox.thedesignmonkeys.co.uk
Hi guys, i was wondering if you could each have a look at this and tell me if you find any problems with it??
-
-
I have reworked my shoutbox so tell me what you think...
I have made posts to be submitted by the enter button too..
gimme some feedback..
thanks
Garry
-
Is there anyway I can get this to set it properly??
-
Hi all,
can someone tell me whenever i update my users timestamp on the server it always starts at 3600 but locally it always starts at 0. this is the code im running...
user_online.php
<?php session_start(); include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $difference = time() - $usertime; $idletime = 300; //3 Minutes echo $difference." is the difference<br/>"; echo $idletime." is the idletime<br/><br/>"; if($difference > 600) { echo $row['username']." is offline<br/>"; } else if($difference > 150) { $idletime++; echo $row['username']." is idle<br/>"; } else { echo $row['username']." is online<br/>"; } } ?>
update.php
<?php session_start(); include('dbconnect.php'); $username = $_SESSION['login']['username']; $sql = mysql_query("UPDATE users set last_activity = CURRENT_TIMESTAMP WHERE username = '$username'"); ?>
-
Ok....
The reason for the ONLINE/IDLE/OFFLINE thing is that it is a Chat Application written in JQuery/PHP. Much like the popular facebook, when a user has been inactive for a certain amount of time, it shows that user to be idle.
Then after a longer amount of time, it times the user out and they need to log in again. This is what I am trying to achieve here.
I have updated the code to this..
userstatus.php
<?php session_start(); include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $difference =time() - $usertime $idletime = $difference - 150; echo $difference." is the difference"; if($difference < -500) { echo $row['username']." is offline<br/>"; } else if($idletime <= 0) { $idletime++; echo $row['username']." is idle<br/>"; } else { echo $row['username']." is online<br/>"; } echo "--------------------------------<br/>"; } ?>
then the
update.php
<?php session_start(); include('dbconnect.php'); $username = $_SESSION['login']['username']; $sql = mysql_query("UPDATE users set last_activity = CURRENT_TIMESTAMP WHERE username = '$username'"); ?>
-
I am trying to write a script to let me see who is online, who is idle and who is offline. This is the script I have so far.
<?php session_start(); include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $difference = $usertime - time(); $idletime = $difference - 150; if($difference < -500) { echo $row['username']." is offline<br/>"; } else if($idletime <= 0) { $idletime++; echo $row['username']." is idle<br/>"; } else { echo $row['username']." is online<br/>"; } echo "--------------------------------<br/>"; } ?>
Everytime a message is sent, i run the script below to update the users last activity timestamp ahead so it knows there has been activity from the user so get it to say they are online.
After an amount of inactivity i want it to say they are idle.
<?php session_start(); include('dbconnect.php'); $username = $_SESSION['login']['username']; $sql = mysql_query("UPDATE users set last_activity = CURRENT_TIMESTAMP + 100 WHERE username = '$username'"); ?>
The problem I am having is when the user sends the message, im not sure how much i have to update the time by for the countdown to start again.
Thanks
Garry
-
I really cannot guage anything from this as it is ASP....
What do you think is the best thing to update the time with everytime a message is sent.
update.php
<?php session_start(); include('dbconnect.php'); $username = $_SESSION['login']['username']; $sql = mysql_query("UPDATE users set last_activity = CURRENT_TIMESTAMP WHERE username = '$username'"); ?>
guestsonline.php
<?php include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $currenttime = time(); if($usertime < $currenttime) { echo "online"; } } ?>
-
this means it will set the $usertime into the future, then they will be online!!
-
<?php session_start(); include('dbconnect.php'); $username = $_SESSION['login']['username']; $sql = mysql_query("UPDATE users set last_activity = CURRENT_TIMESTAMP + 100 WHERE username = '$username'"); ?>
this code is rang everytime a new message is sent to show that the user is still online!!
-
nope this is what ive got
<?php include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $currenttime = time(); $offlinetime = $usertime - time() * 60 * 1; if($usertime < $currenttime) { echo $row['username']."IDLE<br/>"; }elseif($usertime < $offlinetime) { echo $row['username']."OFFLINE"; } else { echo $row['username']."ONLINE<br/>"; } } echo "</ul>"; ?>
it works between ONLINE and IDLE just not offline!!
-
$offlinetime = $usertime - time() * 60 * 10; if($usertime < $currenttime) { echo $row['username']."IDLE<br/>"; }elseif($usertime < $offlinetime) { echo $row['username']."OFFLINE"; } else { echo $row['username']."ONLINE<br/>"; }
-
Yeah there is something wrong with
if($usertime < (time() * 60 / 10)) {
it says that the user is offline when they are online i need it to check if it is 10 minutes in the past
-
<?php include("dbconnect.php"); $sql = mysql_query("SELECT last_activity,username FROM users"); echo "<ul class='online'>"; while($row = mysql_fetch_assoc($sql)) { $usertime = strtotime($row['last_activity']); $currenttime = time(); if($usertime < $currenttime) { echo $row['username']."IDLE<br/>"; } else { echo $row['username']."ONLINE<br/>"; } else if($usertime < ($currenttime * 60 * 10)) { echo $row['username']."OFFLINE"; } } echo "</ul>"; ?>
I get 'Parse error: parse error in C:\XAMPP\htdocs\Shoutbox\config\guests_online.php on line 21'
-
Well it works out how to change between online and idle but I want it to check if a user has been inactive for more than 10 min to have their session timeout and log them out
Learning OOP Programming
in PHP Coding Help
Posted
I have made a simple bit of OOP code which i was wondering if you guys could just check over, just to see if i have got the jist of OOP Programming....
index.php
class_lib.php
process.php