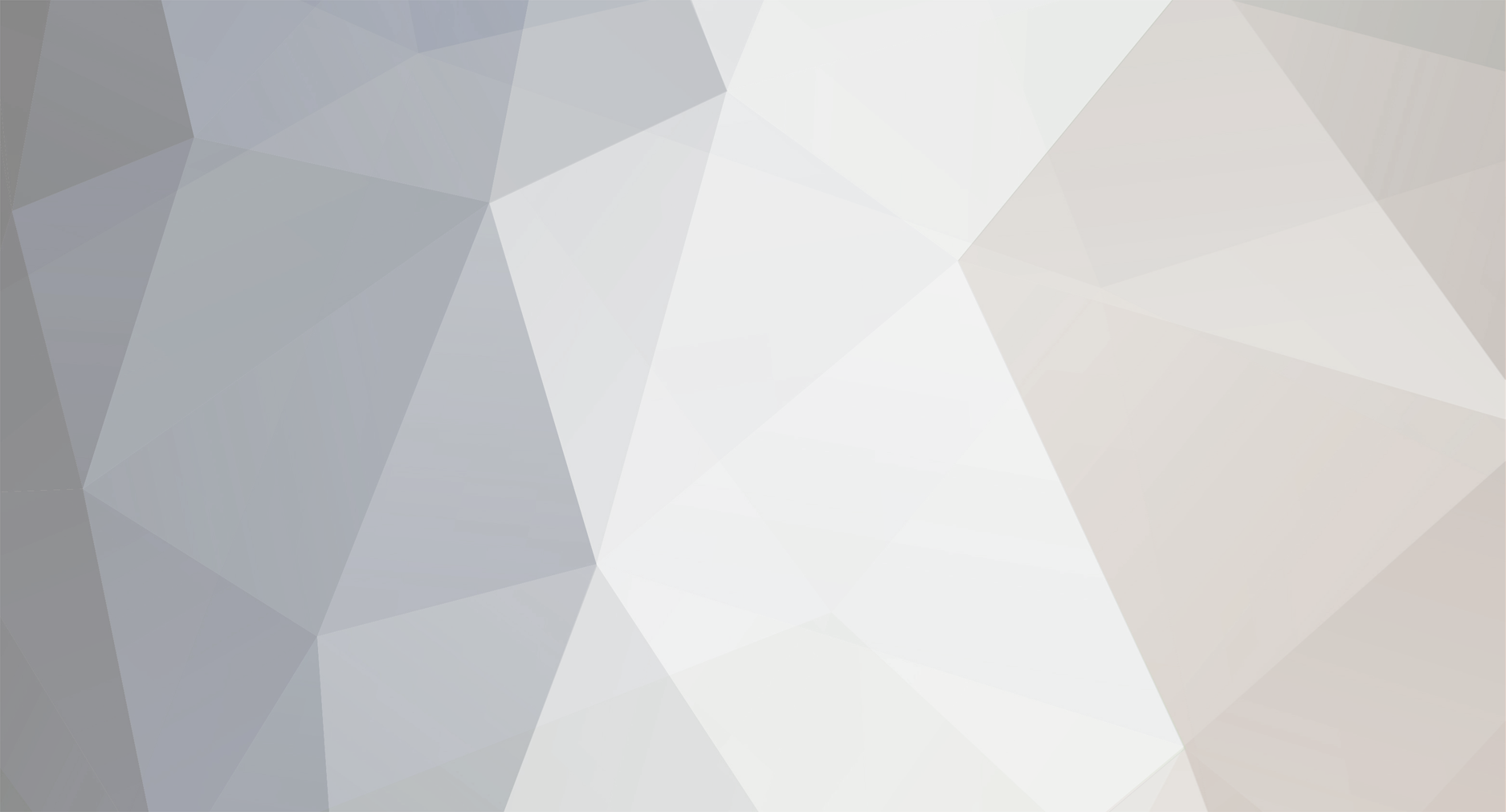
outchy
Members-
Posts
33 -
Joined
-
Last visited
Never
Everything posted by outchy
-
[SOLVED] Display information broken down by field
outchy replied to outchy's topic in PHP Coding Help
Sweet, that worked! Thank you very much. -
Hey guys, I have a MySQL database comprised of high school alumni members and I'm trying to display the information on a page broken down by one particular field (year) and having it repeat by year down the page. I would like the page to look something like this: CLASS of 1936 John Smith Karen Smith Mike Thomas Jane Warner CLASS of 1940 Tim Jones Mandy Minkle Rose Neelan Ralph Potter, etc. But I only know how to display all the information at once in one big list (see code below). Can anyone help guide me on how to achieve this? Thanks! <?php include('connection.php'); $result = mysql_query("SELECT * FROM `database` order by year asc, lastname asc"); // Define $color=1 $color="1"; echo '<head>'; echo '<title>WHS Alumni Association Members Database</title>'; echo '<link rel="stylesheet" href="style.css" type="text/css" media="screen" />'; echo '<script type="text/javascript" src="autoclear.js"></script>'; echo '</head>'; echo '<body>'; echo "<div id='container'>"; echo "<div class='pagename'> <a class='header' href='index.php'>WHS Alumni Association Members Database</a><br /><br /> <form method='post' action='search.php'> <input type='text' name='searchterm' id='search' value='Search' size='32' maxlength='32' onfocus='clearDefault(this)'> </form> </div> <!-- end pagename -->"; while($rows=mysql_fetch_array($result)){ if($color==1){ echo "<div class='bg1'>"; echo "<div class='pad'>"; echo "<span class='fullname'>"; if (!empty($rows['firstname'])) { echo "<span class='firstname'> ".stripslashes($rows['firstname'])." </span> <!-- end firstname -->"; } if (!empty($rows['middlename'])) { echo "<span class='middlename'> ".stripslashes($rows['middlename']).". </span> <!-- end middlename -->"; } if (!empty($rows['nickname'])) { echo "<span class='nickname'> "".stripslashes($rows['nickname'])."" </span> <!-- end nickname -->"; } if (!empty($rows['maidenname'])) { echo "<span class='maidenname'> (".stripslashes($rows['maidenname']).") </span> <!-- end maidenname -->"; } if (!empty($rows['lastname'])) { echo "<span class='lastname'> ".stripslashes($rows['lastname'])." </span> <!-- end lastname -->"; } echo "</span> <!-- end fullname -->"; if (!empty($rows['year'])) { echo "<div class='year'> <a href='".stripslashes($rows['year']).".php'>Class of ".stripslashes($rows['year'])."</a> </div> <!-- end year -->"; } if (!empty($rows['streetaddress'])) { echo "<div class='saddress'> ".stripslashes($rows['streetaddress'])." </div> <!-- end saddress -->"; } if (!empty($rows['mailingaddress'])) { echo "<div class='maddress'> ".stripslashes($rows['mailingaddress'])." </div> <!-- end maddress -->"; } if (!empty($rows['state'])) { echo "<div class='citystate'> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <!-- end citystate -->"; } if (!empty($rows['areacode'])) { echo "<div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <!-- end phone -->"; } if (!empty($rows['email'])) { echo "<div class='email'> <a href='mailto:".stripslashes($rows['email'])."'>".stripslashes($rows['email'])."</a> </div> <!-- end email -->"; } echo " </div> <!-- end pad --> </div> <!-- end bg1 --><br clear='all' />"; // Set $color==2, for switching to other color $color="2"; } else { echo "<div class='bg2'>"; echo "<div class='pad'>"; echo "<span class='fullname'>"; if (!empty($rows['firstname'])) { echo "<span class='firstname'> ".stripslashes($rows['firstname'])." </span> <!-- end firstname -->"; } if (!empty($rows['middlename'])) { echo "<span class='middlename'> ".stripslashes($rows['middlename']).". </span> <!-- end middlename -->"; } if (!empty($rows['nickname'])) { echo "<span class='nickname'> "".stripslashes($rows['nickname'])."" </span> <!-- end nickname -->"; } if (!empty($rows['maidenname'])) { echo "<span class='maidenname'> (".stripslashes($rows['maidenname']).") </span> <!-- end maidenname -->"; } if (!empty($rows['lastname'])) { echo "<span class='lastname'> ".stripslashes($rows['lastname'])." </span> <!-- end lastname -->"; } echo "</span> <!-- end fullname -->"; if (!empty($rows['year'])) { echo "<div class='year'> <a href='".stripslashes($rows['year']).".php'>Class of ".stripslashes($rows['year'])."</a> </div> <!-- end year -->"; } if (!empty($rows['streetaddress'])) { echo "<div class='saddress'> ".stripslashes($rows['streetaddress'])." </div> <!-- end saddress -->"; } if (!empty($rows['mailingaddress'])) { echo "<div class='maddress'> ".stripslashes($rows['mailingaddress'])." </div> <!-- end maddress -->"; } if (!empty($rows['state'])) { echo "<div class='citystate'> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <!-- end citystate -->"; } if (!empty($rows['areacode'])) { echo "<div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <!-- end phone -->"; } if (!empty($rows['email'])) { echo "<div class='email'> <a href='mailto:".stripslashes($rows['email'])."'>".stripslashes($rows['email'])."</a> </div> <!-- end email -->"; } echo " </div> <!-- end pad --> </div> <!-- end bg2 --><br clear='all' />"; // Set $color back to 1 $color="1"; } } echo "</div> <!-- end container -->"; echo '</body>'; mysql_close($connection); ?>
-
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
IT WORKS! Thank you guys so much, wow. Here is the final working code: <?php include('connection.php'); $result = mysql_query("SELECT * FROM `database` order by lastname desc"); // Define $color=1 $color="1"; echo '<head>'; echo '<title>Members Database</title>'; echo '<link rel="stylesheet" href="style.css" type="text/css" media="screen" />'; echo '</head>'; echo '<body>'; while($rows=mysql_fetch_array($result)){ if($color==1){ echo "<div class='bg1'>"; if (!empty($rows['year'])) { echo "<div class='year'> Class of ".stripslashes($rows['year'])." </div>"; } echo " <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> <div class='id'> ID: ".stripslashes($rows['id'])." </div> </div>"; // Set $color==2, for switching to other color $color="2"; } else { echo "<div class='bg2'>"; if (!empty($rows['year'])) { echo "<div class='year'> Class of ".stripslashes($rows['year'])." </div>"; } echo " <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> <div class='id'> ID: ".stripslashes($rows['id'])." </div> </div>"; // Set $color back to 1 $color="1"; } } echo '</body>'; mysql_close($connection); ?> -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
I tried the last suggestion and this is what I'm getting now: Parse error: syntax error, unexpected T_ENCAPSED_AND_WHITESPACE, expecting T_STRING or T_VARIABLE or T_NUM_STRING in /home/wareh5/public_html/database/db7.php on line 24 Line 24 claims to be: Class of \".stripslashes($rows['year']).\" This is how I plugged it in, does this look right? echo "<div class='bg1'>;" if (!empty($rows['year'])) { echo "<div class='year'> Class of \".stripslashes($rows['year']).\" </div>"; } echo " <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> ...etc. -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Okay, that did something... now it seems to be hiding the "year" field regardless of whether or not it actually contains data. So as before, it would say "Class of 1958", now it says "Class of". What I really want to do is hide the entire div that contains the empty field, so the if the field itself is blank, the entire div/line containing "Class of 1958" won't show up at all... does that make sense? -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Sure, here you go. It says line 20 is "<?php echo "<div class='bg1'>"; ?>" but even if I take it out, it still says it's line 20 so I'm not sure... <?php include('connection.php'); $result = mysql_query("SELECT * FROM `database` order by year desc"); // Define $color=1 $color="1"; echo '<head>'; echo '<title>Members Database</title>'; echo '<link rel="stylesheet" href="style.css" type="text/css" media="screen" />'; echo '</head>'; echo '<body>'; while($rows=mysql_fetch_array($result)){ if($color==1){ <?php echo "<div class='bg1'>"; ?> <div class='year' <?php if(strlen($rows['year'])==0) { echo " style=\"display:none;\""; } ?> > Class of <?php stripslashes($rows['year']); ?> </div> <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> </div>"; // Set $color==2, for switching to other color $color="2"; } else { <?php echo "<div class='bg2'>"; ?> <div class='year' <?php if(strlen($rows['year'])==0) { echo " style=\"display:none;\""; } ?> > Class of <?php stripslashes($rows['year']); ?> </div> <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> </div>"; // Set $color back to 1 $color="1"; } } echo '</body>'; mysql_close($connection); ?> -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Okay I'll try that. . Yes, sorry. The latest piece gives this error: Parse error: syntax error, unexpected '<' in /home/wareh5/public_html/database/db5.php on line 20 -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Yeah, I'm trying it every which way but so far I can't get it to work. The concept does seem right though, thank you for your help. Would it work better if I rewrote the page doing it some other way than in one big echo? If so, I'm definitely open to suggestion... -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Yeah as you can see I kind of hacked it together :/ Trying to learn through trial and error... Now it's saying: Parse error: syntax error, unexpected T_IF in /home/wareh5/public_html/database/db5.php on line 22 -
[SOLVED] Do not display entire divs containing empty fields
outchy replied to outchy's topic in PHP Coding Help
Thanks for the quick reply! When I plug that in, I get the following: Parse error: syntax error, unexpected T_ENCAPSED_AND_WHITESPACE, expecting T_STRING or T_VARIABLE or T_NUM_STRING in /home/wareh5/public_html/database/db5.php on line 22 I think it has something to do with the quotation marks but I can't figure out the proper syntax... -
[SOLVED] Do not display entire divs containing empty fields
outchy posted a topic in PHP Coding Help
I really need some help on this one. Right now, I have my page pulling information from my mysql database and displaying the following fields (within divs) like this: Year Name Address Phone Email But say, for example, if any of those fields in the database are empty, I'd like for that entire div containing that empty field to not display on the page at all. So if the person did not fill in the year field, it would output like this: Name Address Phone Email How could I make that work? Thanks so much for any help... <?php include('connection.php'); $result = mysql_query("SELECT * FROM `database` order by year desc"); // Define $color=1 $color="1"; echo '<head>'; echo '<title>Members Database</title>'; echo '<link rel="stylesheet" href="style.css" type="text/css" media="screen" />'; echo '</head>'; echo '<body>'; while($rows=mysql_fetch_array($result)){ if($color==1){ echo "<div class='bg1'> <div class='year'> Class of ".stripslashes($rows['year'])." </div> <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> </div>"; // Set $color==2, for switching to other color $color="2"; } else { echo "<div class='bg2'> <div class='year'> Class of ".stripslashes($rows['year'])." </div> <div class='name'> ".stripslashes($rows['firstname'])." ".stripslashes($rows['nickname'])." ".stripslashes($rows['maidenname'])." ".stripslashes($rows['lastname'])." </div> <div class='address'> ".stripslashes($rows['streetaddress'])."<br /> ".stripslashes($rows['mailingaddress'])."<br /> ".stripslashes($rows['city']).", ".stripslashes($rows['state'])." ".stripslashes($rows['zipcode'])." </div> <div class='phone'> (".stripslashes($rows['areacode']).") ".stripslashes($rows['prefix'])."-".stripslashes($rows['suffix'])." </div> <div class='email'> ".stripslashes($rows['email'])." </div> </div>"; // Set $color back to 1 $color="1"; } } echo '</body>'; mysql_close($connection); ?> -
Yeah that works, I'll just forego the drop down altogether. I don't really need it anyhow. Thanks!
-
Yes, I finally put all the connection info in a separate file so I now know that's not the issue. I also took out a space between the equal sign and the dollar sign and that changed the error. Now it says: PHP Parse error: syntax error, unexpected T_VARIABLE in /Applications/MAMP/htdocs/test2/sortby.php on line 16 Which corresponds to: if(!isset($select) || $select == 1) {
-
Sorry about that. I found the error log file and this is what it's telling me: PHP Parse error: syntax error, unexpected T_VARIABLE in /Applications/MAMP/htdocs/test2/sortby.php on line 16 This is line 16: $select= $_POST['droppy']; Is there something wrong with that syntax?
-
Okay cool, that works if I replace this line... $result = mysql_query("SELECT * FROM shows ORDER BY dateofshow DESC"); with this line... $result = mysql_query("SELECT * FROM shows WHERE LOWER(CONCAT_WS(' ',opener1,opener2,opener3,opener4,opener5,opener6)) LIKE LOWER('%$search%')"); but then my other drop down options won't work anymore. Is there a way to combine them so I can search for dateofshow, headliner, venue, AND opener?
-
That doesn't show anything either... actually, I've never been able to see any echos at all when having this blank page issue. In fact, I think I remember you helping me before when I was having a similar problem. I'm using MAMP on a Mac, not sure if that would have anything to do with it.
-
I did both things you suggested and it's still showing up as a blank page upon submit. Here's what I'm using currently: <?php $con = mysql_connect("localhost","root","root"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("test2", $con); //gets the variables the user selected from the form. $select= $_POST['select']; if(!isset($select) || $select == 1){ $order = "DESC"; }else{ $order = "ASC"; } $result = mysql_query("SELECT * FROM shows ORDER BY dateofshow $order") or die (mysql_error()); echo '<table width="740" border="0" align="center" cellpadding="12" cellspacing="0" class="main">'; echo '<tr class="topper"><th>Date</th><th>Headliner</th><th>Venue</th><th>Opener</th></tr>'; while($rows=mysql_fetch_array($result)){ echo "<tr><td valign=\"top \">".date('Y > M d',strtotime($rows['dateofshow']))."</td><td valign=\"top \">".$rows['headliner']."</td><td valign=\"top \">".$rows['venue']."<br />".$rows['city'].", ".$rows['state']."</td><td valign=\"top \">".$rows['opener1']."<br />".$rows['opener2']."<br />".$rows['opener3']."<br />".$rows['opener4']."<br />".$rows['opener5']."<br />".$rows['opener6']."</td></tr>"; } echo '</table><br /></center></body>'; mysql_close($con); ?> Any other thoughts? Thanks for all of your help, btw.
-
That looks a lot better than mine... but it didn't work. I get a blank page when I hit the submit button.
-
I almost have this working... I have a concert database where I'm trying to create a simple drop down which will allow me to select either "ascending" or "descending" and have it display the results accordingly by date. It's sort of working, but it's only showing one date for "descending" and two dates for "ascending", and then it goes into an infinite loop each time. Here are my two files: sortform.htm: <html> <body> <form name="form1" method="post" action="sortby.php"> <table width="188" border="0" cellpadding="0" cellspacing="0"> <tr> <td><select name="select"> <option value="1">sort by DESC</option> <option value="2">sort by ASC</option> </select></td> <td width><input type="image" src="graphics/query.jpg" alt="submit" title="submit" value="Submit"></td> </tr> </table> </form> </body> </html> sortby.php: <?php $con = mysql_connect("localhost","root","root"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("test2", $con); $result = mysql_query("SELECT * FROM shows"); //gets the variables the user selected from the form. $select= $_POST['select']; echo '<table width="740" border="0" align="center" cellpadding="12" cellspacing="0" class="main">'; echo '<tr class="topper"><th>Date</th><th>Headliner</th><th>Venue</th><th>Opener</th></tr>'; while($rows=mysql_fetch_array($result)){ //If the user hasnt selected a sorting preference, just display all the records which havent expired if($select=='1') { $result = mysql_query("SELECT * FROM shows ORDER BY dateofshow DESC"); echo "<tr><td valign=\"top \">".date('Y > M d',strtotime($rows['dateofshow']))."</td><td valign=\"top \">".$rows['headliner']."</td><td valign=\"top \">".$rows['venue']."<br />".$rows['city'].", ".$rows['state']."</td><td valign=\"top \">".$rows['opener1']."<br />".$rows['opener2']."<br />".$rows['opener3']."<br />".$rows['opener4']."<br />".$rows['opener5']."<br />".$rows['opener6']."</td></tr>"; } else { $result = mysql_query("SELECT * FROM shows ORDER BY dateofshow ASC"); echo "<tr><td valign=\"top \">".date('Y > M d',strtotime($rows['dateofshow']))."</td><td valign=\"top \">".$rows['headliner']."</td><td valign=\"top \">".$rows['venue']."<br />".$rows['city'].", ".$rows['state']."</td><td valign=\"top \">".$rows['opener1']."<br />".$rows['opener2']."<br />".$rows['opener3']."<br />".$rows['opener4']."<br />".$rows['opener5']."<br />".$rows['opener6']."</td></tr>"; } } echo '</table><br /></center></body>'; mysql_close($con); ?>
-
I have a concert database where I've recently implemented a simple search form. I have it working so I can successfully search for "dateofshow", "headliner", and "venue" by choosing the proper option from a drop down list and typing the search string into a text field. Now, I would like to be able to add "opener" to that drop down list, but the problem is I don't have just one database entry called "opener"... I have six separate entries ("opener1", "opener2", "opener3", "opener4", "opener5" and "opener6"). Is there a way to add a variable called $opener and have it search through all six entries at once, perhaps with some sort of wildcard character at the end of the word opener? My code is below: <? //connect to mysql //change user and password to your mySQL name and password mysql_connect("localhost","root","root"); //select which database you want to edit mysql_select_db("test2"); $search=$_POST["search"]; $type=$_POST["type"]; //get the mysql and store them in $result //change whatevertable to the mysql table you're using //change whatevercolumn to the column in the table you want to search $result = mysql_query("SELECT * FROM shows WHERE $type LIKE '%$search%'"); // Define $color=1 $color="1"; echo '<head>'; echo '<title>Search Results</title>'; echo '<link rel="stylesheet" href="style.css" type="text/css" media="screen" />'; echo '</head>'; echo '<body>'; echo '<center>'; echo '<br /><a href="index.php"><img src="niksshowlist.png" border="0"></a><br /><br />'; echo '<form method="post" action="search.php"> <select name="type" size="1"> <option value="dateofshow">Date</option> <option value="headliner">Headliner</option> <option value="venue">Venue</option> </select> <input type="text" name="search" size=25 maxlength=25> <input type="Submit" name="Submit" value="Search"> </form>'; echo '<table width="740" border="0" align="center" cellpadding="12" cellspacing="0" class="main">'; echo '<tr class="topper"><th>Date</th><th>Headliner</th><th>Venue</th><th>Opener</th></tr>'; //grab all the content while($r=mysql_fetch_array($result)) { //the format is $variable = $r["nameofmysqlcolumn"]; //modify these to match your mysql table columns $dateofshow=$r["dateofshow"]; $headliner=$r["headliner"]; $venue=$r["venue"]; $city=$r["city"]; $state=$r["state"]; $opener1=$r["opener1"]; $opener2=$r["opener2"]; $opener3=$r["opener3"]; $opener4=$r["opener4"]; $opener5=$r["opener5"]; $opener6=$r["opener6"]; if($color==1){ echo "<tr class='bg1'> <td valign=\"top \">".date('Y > M d',strtotime($dateofshow))."</td> <td valign=\"top \">$headliner</td> <td valign=\"top \">$venue<br />$city, $state</td> <td valign=\"top \">$opener1<br />$opener2<br />$opener3<br />$opener4<br />$opener5<br />$opener6</td> </tr>"; // Set $color==2, for switching to other color $color="2"; } else { echo "<tr class='bg2'> <td valign=\"top \">".date('Y > M d',strtotime($dateofshow))."</td> <td valign=\"top \">$headliner</td> <td valign=\"top \">$venue<br />$city, $state</td> <td valign=\"top \">$opener1<br />$opener2<br />$opener3<br />$opener4<br />$opener5<br />$opener6</td> </tr>"; // Set $color back to 1 $color="1"; } } echo '</table><br /></center></body>'; mysql_close($con); ?>
-
Thank you!!
-
I was wondering if there's a way to have a form page automatically redirect to a different url after a successful post to the database, instead of just showing the text "1 show added". Here is the code: <?php $con = mysql_connect("localhost","root","root"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("nik", $con); $sql="INSERT INTO test (dateofshow, headliner, place, opener) VALUES (CONCAT('$_POST[year]','-','$_POST[month]','-','$_POST[day]'),'$_POST[headliner]','$_POST[place]','$_POST[opener]')"; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } echo "1 show added"; mysql_close($con) ?>
-
Hooker just PM'd me this and it totally worked! (CONCAT('$_POST[year]','-','$_POST[month]','-','$_POST[day]'),'$_POST[headliner]','$_POST[place]','$_POST[opener]')"; To all of you really, thank you so much!
-
Still nothing happens. When I click Submit, it just returns a blank page with nothing in the page source.