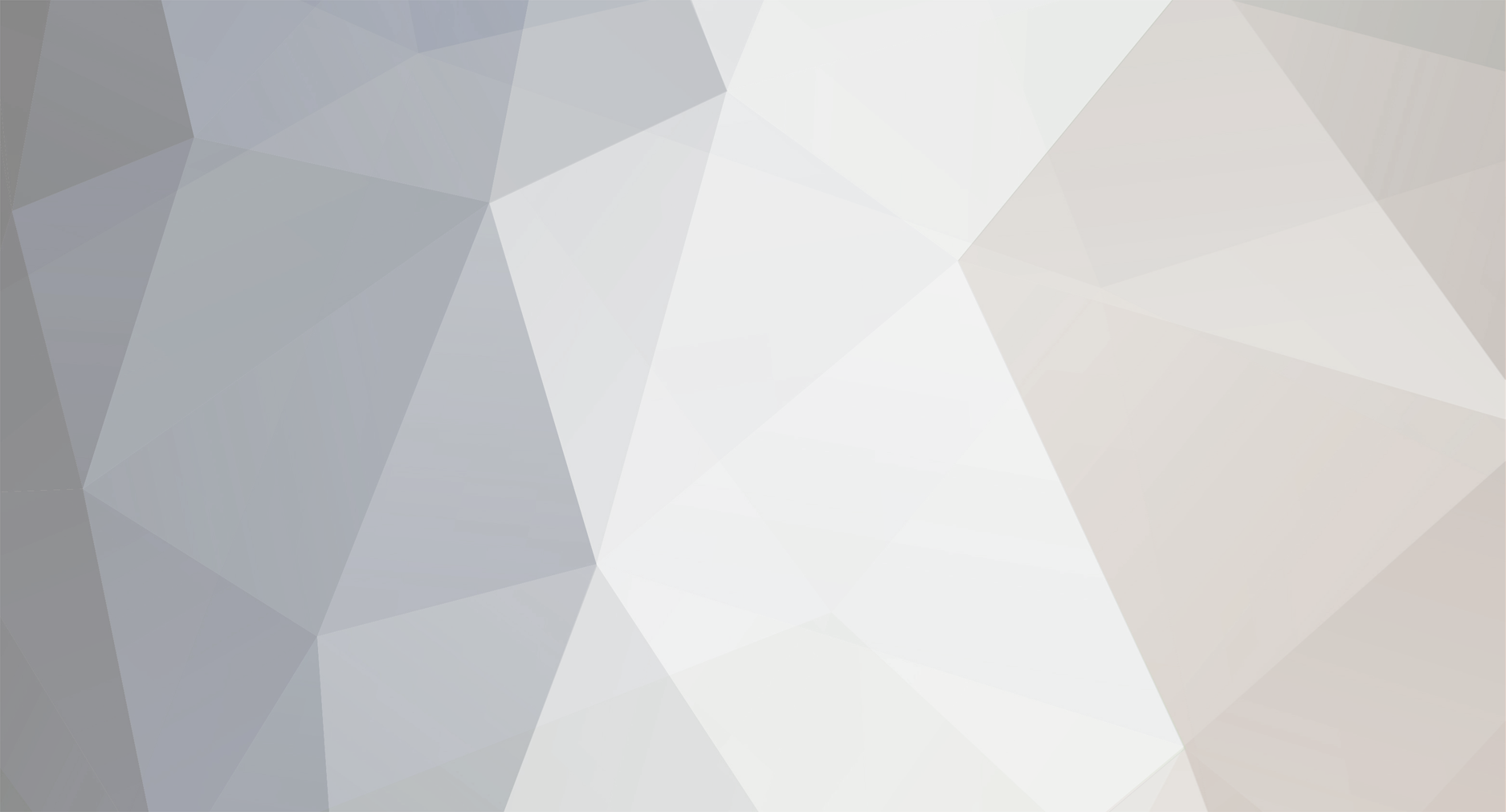
Vermillion
Members-
Posts
118 -
Joined
-
Last visited
Never
Everything posted by Vermillion
-
I like children and fantasy. My name comes from a fantasy RPG, in which Vermillion is a guy that protects a little girl. Vermillion is also a pretty color.
-
C Pointers (the actual thing not helpful tips)
Vermillion replied to natbob's topic in Other Programming Languages
Pointers are indeed a challenge the first time, but there's really nothing to be afraid of. While you are learning, just keep one thing in mind: Pointers allow you to pass a value by REFERENCE. Suppose you want to change the value of a variable through a function. Passing the values just like that wouldn't do the trick. Consider the following example: #include <iostream> using namespace std; void duplicate(int *p); int main(){ int a, b; a = 3; b = 4; cout << "Value of a before duplication: " << a; cout << endl << "Value of b before duplication: " << b; cout << endl << endl; duplicate(&a); duplicate(&b); cout << "Value of a after duplication: " << a; cout << endl << "value of b after duplication: " << b; cout << endl << endl; } void duplicate(int *p){ *p = *p * 2; } That will pring: Value of a before duplication: 3 Value of b before duplication: 4 Value of a after duplication: 6 Value of b after duplication: 8 If you try to pass a normal variable to the function, it is not going to work. Why? Because when you pass a variable to a function, only a copy is passed to it. The function works with they copy and then discards if. Now, if you work with a pointer, it is going to get "the address pointed at" by that pointer, and it is going to modify the variable itself. -
[SOLVED] Outputting Content Of A Database Correctly.
Vermillion replied to Vermillion's topic in PHP Coding Help
Thanks a lot, it works now. -
[SOLVED] Outputting Content Of A Database Correctly.
Vermillion replied to Vermillion's topic in PHP Coding Help
Oh, it is properly displayed to the users. I need it to display it properly to me for when I edit it. You know, like when you edit a post in a forum, you don't see all the tags in the text area, you see the linebreaks. And thanks about the second tip, I will see how I do about that. -
[SOLVED] Outputting Content Of A Database Correctly.
Vermillion replied to Vermillion's topic in PHP Coding Help
Output Script: <?php include "../../includes/dbdetails.php"; //Include database details. mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db($dbactual); $id = $_GET['id']; $query = "SELECT * FROM news WHERE id = '$id'"; $result = mysql_query($query); $rows = mysql_fetch_array($result); $title = $rows['title']; $body = nl2br(stripslashes($rows['body'])); echo "<form method=\"post\" action=\"editednews.php?id={$id} \">"; echo "<input type=\"text\" name=\"title\" value=\"$title\" /><br />"; echo "<textarea name=\"body\" cols=\"80\" rows=\"20\">$body</textarea><br />"; echo "<input type=\"submit\" value=\"Submit\" />"; echo "</form>"; ?> Input Script: <?php ################################################################## # SCRIPT: Add News # # SCRIPT NAME: addnews.php # # AUTHOR: Andrés Ibañez | Vermillion # ################################################################## require "../../includes/dbdetails.php"; $title = $_POST['title']; $body = $_POST['body']; $date = date("Y-m-d"); $time = date("G:i A"); mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db("vghack"); $body = mysql_real_escape_string($body); $title = mysql_real_escape_string($title); $query = "INSERT INTO news(title, body,time,date) VALUES('$title','$body','$time','$date')"; mysql_query($query); header("location:".$_SERVER['HTTP_REFERER']); ?> Optionally, this is the script with a little preview: <?php require "../../includes/dbdetails.php"; mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db("vghack"); $query = "SELECT * FROM news ORDER BY time DESC, date DESC"; $result = mysql_query($query); $num = mysql_num_rows($result); $i = 0; echo "<form method=\"post\" action=\"deletenews.php\">"; while($i < $num){ $id = mysql_result($result,$i,"id"); $title = mysql_result($result,$i,"title"); $body = mysql_result($result,$i,"body"); $date = mysql_result($result,$i,"date"); $time = mysql_result($result,$i,"time"); echo "<table class=\"newstitle\" onclick=\"toggleItem('_$id')\" width=\"100%\"><tr><td width=\"50%\">$title</td><td align=\"right\" width=\"100%\">$date at $time</td></tr></table>"; echo "<a href=\"deletenews.php?id=$id\" class=\"small_link\">Delete</a> - <a href=\"editnews.php?id=$id\" class=\"small_link\">Edit</a> - <input type=\"checkbox\" name=\"news_id[]\" value=\"$id\" />"; echo "<div class=\"bodynews\" id=\"_$id\" style=\"display:none;\">$body</div>"; $i++; } echo "<br /><br />"; echo "<input type=\"submit\" value=\"Delete Selected\" />"; echo "</form>"; ?> I will use the second option if I really can't figure it out. Still not working =(. -
[SOLVED] Outputting Content Of A Database Correctly.
Vermillion replied to Vermillion's topic in PHP Coding Help
Same thing happens. Except that neither the small preview works properly now. EDIT: Wait, it seems it was because I was using two ==, heh ;P. -
Okay yeah, so I have my news system, and news system has a script so I can edit the news. The script looks like this: <?php //Script to update news after submission. session_start(); include "../../includes/dbdetails.php"; mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db($dbactual); $id = $_SESSION['id']; $query = "SELECT * FROM access WHERE user_id = '$id' AND level = 8"; $result = mysql_query($query); $num = mysql_num_rows($result); if($num < 1){ echo "<strong>Hacking Attempt. Your IP and username have been logged.</strong>"; return 0; } include "../../includes/dbdetails.php"; $title = $_POST['title']; $body = $_POST['body']; $id = $_GET['id']; mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db($dbactual); $query = "UPDATE news SET title = '$title' WHERE id = '$id'"; mysql_query($query); $query = "UPDATE news SET body = '$body' WHERE id = '$id'"; mysql_query($query); mysql_close(); header("location:". "index.php"); ?> This is the script that adds the news: <?php ################################################################## # SCRIPT: Add News # # SCRIPT NAME: addnews.php # # AUTHOR: Andrés Ibañez | Vermillion # ################################################################## require "../../includes/dbdetails.php"; $title = $_POST['title']; $body = $_POST['body']; $date = date("Y-m-d"); $time = date("G:i A"); mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db("vghack"); $body = nl2br($body); $body = mysql_real_escape_string($body); $title = mysql_real_escape_string($title); $query = "INSERT INTO news(title, body,time,date) VALUES('$title','$body','$time','$date')"; mysql_query($query); header("location:".$_SERVER['HTTP_REFERER']); ?> Thing is, when I am editing one, the content is not displayed properly in the edit window. This is what is outputted. Notice that the script displays all the line break tags.
-
You basically want to prevent people from reserving another item if has already been reserved by someone, right?
-
[SOLVED] Searching For Values On An Array.
Vermillion replied to Vermillion's topic in PHP Coding Help
Thanks a lot guys, my register script works more than fine now. -
[SOLVED] Searching For Values On An Array.
Vermillion replied to Vermillion's topic in PHP Coding Help
And If I wanted to quote a different error message for each element that has the value of 1? Sorry, I can't seem to figure much about this out. -
[SOLVED] Searching For Values On An Array.
Vermillion replied to Vermillion's topic in PHP Coding Help
Sure. I have a class that registers the users to my site, and every function returns a value: 1 or 0. If a function returns 1, then there was a problem in the registration. So each element in the array will hold 1 or 0, and each key will represent an error message. <?php include "includes/class.register.php"; //Assuming there is user input data code here. $user -> new member; $register[0] = $user -> checkLength("Verm"); //If the username is 3 characters or long, return 0. If it returns 1, display an error message. Here the value will be 0. $register[1] = $user -> checkPassLength("aasdfadg"); // Same as before. This will return 0. $register[2] = $user -> checkMail("sdsdsd") // THis is invalid, therefore it will return 1. //And so on... ?> So suppose all the arrays contain 0, there will be nothing to process on the foreach loop, and I need to do something like "if array exists, do not register users. Else register the users". Ahhh, apologizes if that is not clear. My native language is not English and it is 2:58 AM here. If you need more details, I let me know and I will try to rephrase it. -
[SOLVED] Searching For Values On An Array.
Vermillion replied to Vermillion's topic in PHP Coding Help
Okay, thanks for that to both of you, I decided to do Cainmi's method, as it looks easier, and I don't get the other one at all. The only problem with the method I chose is that those values are actually generated. Sometimes, the array will have all the values set to 0, and since this methods creates another array, when all the values are at 0, the new array won't be generated, and I was wondering if there was a way to check wether an array exists or not. I tried doing a quick google search, but all the results talk about checking if an element exists, rather than if the whole array exists. -
[SOLVED] Searching For Values On An Array.
Vermillion replied to Vermillion's topic in PHP Coding Help
With that will I be able to return ALL of them with their respective values though, or only the first match? So I can get something like this: <?php $register[0] = 0; $register[1] = 1; $register[2] = 1; $register[3] = 1; $register[4] = 0; $register[5] = 1; $register[6] = 0; $register[7] = 1; //After using array search, I should only have $register[1], $register[2], $register[3], $register[5], and $register[7] ?> -
Suppose I have the following code: <?php $register[0] = 0; $register[1] = 1; $register[2] = 1; $register[3] = 1; $register[4] = 0; $register[5] = 1; $register[6] = 0; $register[7] = 1; ?> What would I need to do if I wanted to return, say, ONLY the ones that have "1" with their key values?
-
The main problem I see for now is the fact that you have too many "else" clauses. You can't do that. You either have: <?php if(1 == 1){ echo "True"; } else { echo "False"; } ?> In your case, it is not that case, you have: <?php if(1 == 1){ echo "True"; } else { echo "False"; } else { echo "False"; } ?> What you need to do in that case is either use the Switch statement or use the "elseif" clause: <?php $number = $_POST['value']; if($number == 1){ echo "Number is 1"; } elseif($number == 2) { echo "Number is 2"; } elseif ($number == 3){ echo "Number is 3"; } else { echo "Number is invalid!"; } ?> Again, that's what I saw at a quick glance, so even if that fixes your problem, you may get other problems. On another note, you should consider organizing your code more. I tried to look at it for you on Dreamweaver, but everything is flying around everywhere. It may be a good to comment where your blocks start and end, as well as using other indenting methods.
-
Never mind, I solved the issue by moving both mysql_connect(), mysql_select_db() to the top of class itself, instead of inside it. It is still inside the class file, but it works now. On another note, sorry for the double post, but the Modify link just dissapeared...
-
Hm, can you explain a bit more? Do you have the array? If so, can post it as well? Maybe I can help you there.
-
Thanks for the reply, although, I'm still having problems. When I just put the exact following MySQL codes outside of classes, it works, but now I am even trying on my class file itself, including the database details and everything, and nothing happens: <?php ################################################################################ # SCRIPT: Register Validator # # SCRIPT NAME: class.registervalidator.php # # AUTHOR: Andrés Ibañez | Vermillion. # ################################################################################ ################################################################################ # This is the main class for a member. However, other classes will be # # heredated from this one because of that, so everything here needs to be on # # protected: To allow the heredited classes to access members and functions, # # and not other classes. # ################################################################################ ################################################################################ # The script that will work with this class, or with the objects of this # # class, should include the "dbdetails.php" file on the includes folder. This # # is because this class will connect to the database. # ################################################################################ include "../includes/dbdetails.php"; class member{ protected $username; protected $password; protected $question; protected $answer; protected $email; protected $fname; protected $lname; protected $byear; protected $bmonth; protected $bday; protected $gender; protected $date; protected $time; protected $bdate; protected $ip; //User-related methods. function setUsername($username){ $this -> username = $username; } function getUsername(){ return $this -> username; } function checkChars($user){ if(preg_match("/[\[\]\^\\\{\}\*\"\'@\?\`\´#\|\=\(\)\+\:\°]/is", $user)){ return 1; } else { return 0; } } function checkExistence($username){ mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db($dbactual); if(mysql_num_rows(mysql_query("SELECT * FROM members WHERE username = '$username'")) > 0){ return 1; // IF it exists, return 1. } else { return 0; } } // Password-related methods. function valPassword($pass1, $pass2){ $passcount = strlen($pass); $pass1 = md5($pass1); $pass2 = md5($pass2); if($passcount < 6 || $pass1 != $pass){ return 0; } } function setPassword($pass1){ $this -> password = $pass1; } function getPassword(){ return $this -> password; } } $test = new member; $test -> setUsername("Vermillion"); echo $test -> getUsername(); $test -> checkExistence("Vermillion"); ?> Could there be something else causing the problem? Because if I have the following file: <?php class member{ protected $username; function setname($set){ $this -> username = $set; } function getname(){ return $this -> username; } } mysql_connect("localhost","root",""); mysql_select_db("vghack"); $user = new member; $user -> setname("Vermillion"); if(mysql_num_rows(mysql_query("SELECT * FROM members WHERE username = '".$user -> getname()."'")) > 0){ echo "Exists!"; } else { echo "Doesn't exist!"; } ?> Which is basically the same thing, except the MySQL functions are not in the class itself.
-
Okay yeah, I have this piece of code: <?php ################################################################################ # SCRIPT: Register Validator # # SCRIPT NAME: class.registervalidator.php # # AUTHOR: Andrés Ibañez | Vermillion. # ################################################################################ ################################################################################ # This is the main class for a member. However, other classes will be # # heredated from this one because of that, so everything here needs to be on # # protected: To allow the heredited classes to access members and functions, # # and not other classes. # ################################################################################ ################################################################################ # The script that will work with this class, or with the objects of this # # class, should include the "dbdetails.php" file on the includes folder. This # # is because this class will connect to the database. # ################################################################################ class member{ protected $username; protected $password; protected $question; protected $answer; protected $email; protected $fname; protected $lname; protected $byear; protected $bmonth; protected $bday; protected $gender; protected $date; protected $time; protected $bdate; protected $ip; //User-related methods. function setUsername($username){ $this -> username = $username; } function getUsername(){ return $this -> username; } function checkChars($user){ if(preg_match("/[\[\]\^\\\{\}\*\"\'@\?\`\´#\|\=\(\)\+\:\°]/is", $user)){ return 1; } else { return 0; } } function checkExistence($user){ mysql_connect($dbhost,$dbuser,$dbpass); mysql_select_db($dbactual); if(mysql_num_rows(mysql_query("SELECT * FROM members WHERE username = '$user'")) > 0){ return 1; // IF it exists, return 1. } else { return 0; } } // Password-related methods. function valPassword($pass1, $pass2){ $passcount = strlen($pass); $pass1 = md5($pass1); $pass2 = md5($pass2); if($passcount > 6 || $pass1 != $pass){ return 0; } } function setPassword($pass1){ $this -> password = $pass1; } function getPassword(){ return $this -> password; } } ?> If you see the checkExistence() method on it, you will see that it uses some MySQL stuff. So I include the class here: <?php include "vghack/classes/class.registervalidator.php"; include "vghack/includes/dbdetails.php"; ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <input type="text" name="user" /><input type="password" name="pass1" /><input type="password" name="pass2" /><input type="submit" /> </form> <?php if($_POST){ $user = new member; $user -> setUsername($_POST['user']); echo $user -> getUsername(); echo "<br /><br />"; echo $user -> checkChars($user -> getUsername()); echo "<br /><br /><br />"; echo $user -> valPassword($_POST['pass1'], $_POST['pass2']); echo "<br /><br />"; echo $user -> getPassword(); echo "<br /><br />"; echo $user -> checkExistence($_POST['user']); } ?> To see wether my stuff works or not, but I get a MySQL error: Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in E:\wamp\www\vghack\classes\class.registervalidator.php on line 73 I think the problem is the class, because I tried the code normally, outside a method, and it worked fine. Any help, please? -- Edit: Just noticed the O.O.P forum... I am not sure if this should be moved there, but if it is needed, I will appreciate it if it just moved and not closed.