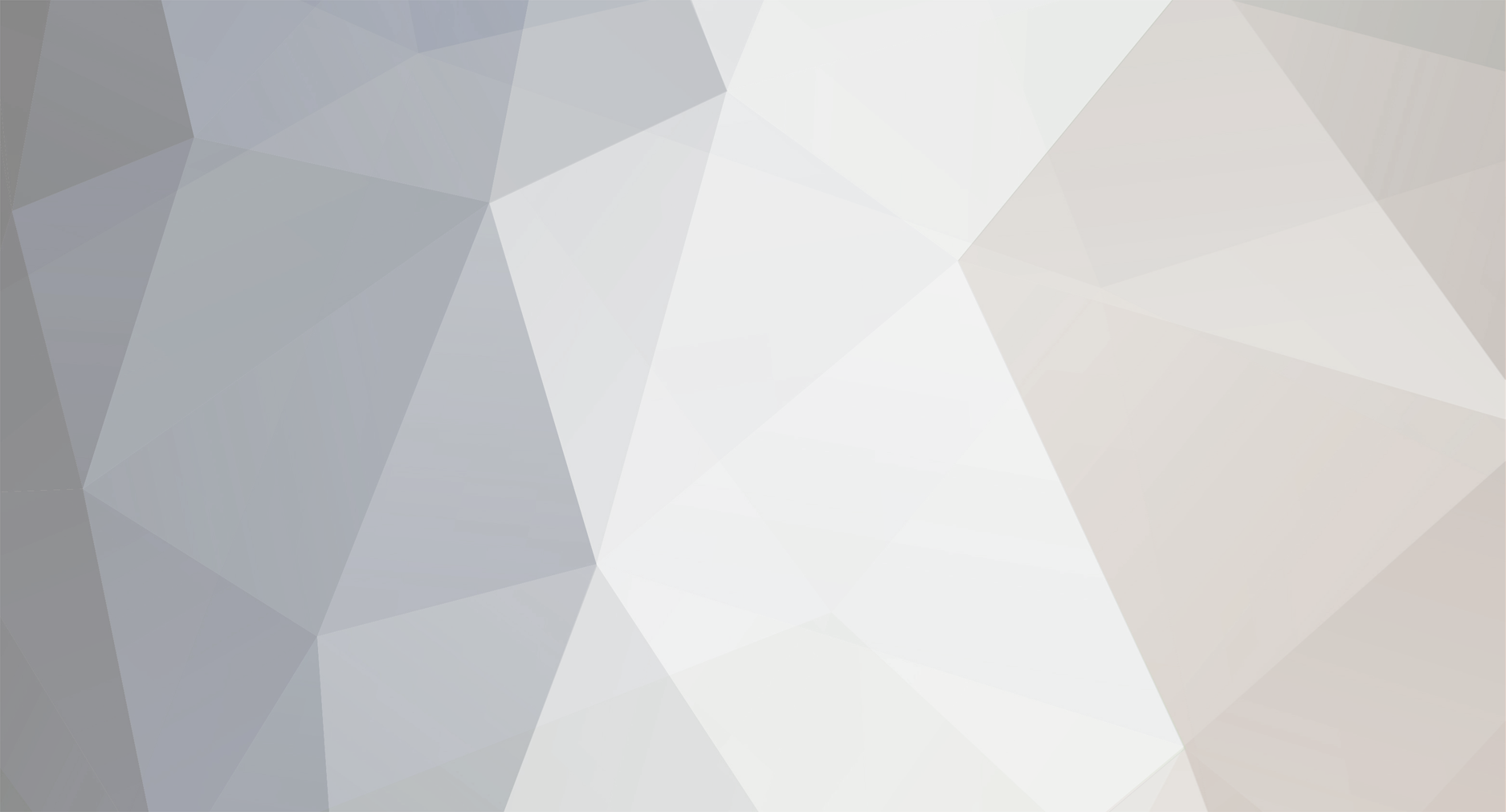
Eggzorcist
Members-
Posts
214 -
Joined
-
Last visited
Never
Everything posted by Eggzorcist
-
here is my class Its probably a variable scope issue, how do I go to fix this? Must I put a PDO object in all my objects that will use the database? class fetcher { function checkifDone( $value ){ if ( $value == 0 ) { echo 'Not Done'; } elseif ( $value == 1 ){ echo 'Done'; } } function grab_tasks( $userid ){ $grabber = $db->query('SELECT * WHERE user_id =' . $userid); $grabber->setFetchMode(PDO::FETCH_OBJ); while ( $row = $grabber->fetch() ){ $display = '<div>Task Name: ' . $row->name .'</div>'; $display .= '<div>Task Details: <p>'. $row->details .'</p></div>'; //$display .= '<div>'. $this->checkifDone($row->task_status) .'</div>'; $display .= '<div>Task Due: '. $row->task_due . '</div>'; echo $display; } } }
-
I made a small script and it says that my database object isn't an object. I connect to my pdo database via: <?php try { $db = new PDO("mysql:host=localhost;dbname=database", "root", "root"); $db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch(PDOException $e) { echo $e->getMessage(); } ?> The error I get is Notice: Undefined variable: db in /Users/JPFoster/Sites/jobiji_sandbox/extensions/tasks.php on line 21 Fatal error: Call to a member function query() on a non-object in /Users/JPFoster/Sites/jobiji_sandbox/extensions/tasks.php on line 21 here's where the error is $grabber = $db->query('SELECT * WHERE user_id =' . $userid); $grabber->setFetchMode(PDO::FETCH_OBJ); while ( $row = $grabber->fetch() ){ $display = '<div>Task Name: ' . $row->name .'</div>'; $display .= '<div>Task Details: <p>'. $row->details .'</p></div>'; //$display .= '<div>'. $this->checkifDone($row->task_status) .'</div>'; $display .= '<div>Task Due: '. $row->task_due . '</div>'; echo $display; } could anyone tell me where this issue is originating I followed a fairly simple tutorial that didn't seem to work. Thanks!
-
I've made my first object that will addition an array but it doens't seem to be working as it echo's out 0. here is my script <?php class A { public $sum = 0; function addition($values){ foreach ($values as $numbers){ $this->sum = $number + $this->sum; } echo $this->sum; } } $a = new A(); $array = array(10, 15, 25); echo $a->addition($array); ?> I don't see what I'm doing wrong. Any direction would be greatly appreciated.
-
I'm having a lot of trouble validating things using custom regex. I'm trying to validate usernames without spaces using numbers and/or letters with optional underscores. This is my code but it isn't validating anything, nothing is ever validated. Whatever I write it says the username isn't valid. if(isset($_POST['submit'])){ if(filter_var($_POST['value1'], FILTER_VALIDATE_REGEXP, array("options"=>array("regexp"=>"/^[\w.-]{{3},{28}}$/")))){ echo "Value is a valid username."; } else { echo "Value is NOT a valid username."; } } ?> Any help regarding this issue will be greatly appreciated. Thanks!
-
I found what was the problem. the input in the html must be the type of 'submit' rather than simply a type='button'. Thanks!
-
I'm not sure why this code isn't working... I've done this many times before and this time it isn't working at all... Here's the code I'm using: <?php if(isset($_POST['submit'])){ if(filter_var($_POST['value1'], FILTER_VALIDATE_EMAIL)){ echo "Value is a valid email address."; } else { echo "Value is NOT a valid email address."; } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Untitled Document</title> </head> <body> <form action="<?php echo $_SERVER['PHP_SELF'] ?>" method="POST"> <input name="value1" type="text" id="value1" size="40" /> <input id="submit" name="submit" value="Validate Value" type="button" /> </form> </body> </html> I'm wondering whether anything has changed, but I don't see how this couldn't work. Thanks for any additional information!
-
but if I'm looking for each array to send out as a json_encode() and if there is an error it will appear as such $error['message'] = "ERROR" so we can just check $error array, to seek for any "ERROR" in either of ['message'], ['name'], ['email']. Can't I make a really simple if statement using in_array()?
-
couldn't I try an in_array() function?
-
Thanks for the push. How is it possible to check the entire array of values to find if there are errors in them?
-
I've been thinking of using an assoc_array for validation code in php to have an associative of all the fields (name, email, message) and to see if there has been an "ERROR" declared in any of them? How would I first declare this array? and How would I check to see if they are empty or any of them contain "ERROR". Thanks
-
What is the proper methodology for declaring an empty assoc array? And checking if its still empty or not? Thanks!
-
Well I don't feel like I need a multi-dimensional array because most data will be already in the html and jquery... So all I want it to do is to store if its an error or not, so I can know which display error I should have slide open. I'm just worried, since I don't think this line is working for me: $error = array(NULL => '', NULL => '', NULL => ''); if(filter_var($name, FILTER_VALIDATE_REGEXP, array("options"=>array("regexp"=>"/^[A-Za-z](?=[A-Za-z0-9_.]{4,24}$)[a-zA-Z0-9_]*\.?[a-zA-Z0-9_]*$/"))) === false){ $error['name'] = "ERROR"; } else{ $error['name'] = NULL; } if ($error == NULL and $error != "ERROR"){ ... }
-
I've actually solved this problem. Thanks!
-
Hi, I have a few questions concerning controlling field validation using $error = array(); in a way to send back exactly which is false. This is what I'm doing. $error = array(NULL => '', NULL => '', NULL => ''); I check 3 fields: name, email, message and whether they are good or bad. As such //if the value is BAD I do: $error['message'] = "ERROR"; //if the value is good I do: $error['message'] = NULL; However, with this method I'm not sure how I can check whether any of these have an error... Also, is it the good way to declare an array for this functioning? Thank you very much!
-
Is it possible using the limiters {} to show only the minimum requirement with no maximum requirement. if(filter_var($message, FILTER_VALIDATE_REGEXP, array("options"=>array("regexp"=>"/{7}/"))) === false){ echo $error['message'] = "ERROR"; } I get an error with this, and without the backslashes too... Any other ways? Thanks!
-
I'm having trouble with listing a query with while loops... What am I doing wrong? $user = $database->escape_value($_SESSION['username']); while($row = mysql_fetch_assoc($database->query("SELECT * FROM project WHERE user = '{$user}'"))){ echo $row['name']; } THank you
-
I'm trying to do a query as such: SELECT * FROM projects WHERE user = {$user} and $user = a secured email. but I'm getting this error: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '@gmail.com' at line 1 Why am I getting this error? Thank you very much!
-
header('Location: index.php'); with ajax
Eggzorcist replied to Eggzorcist's topic in PHP Coding Help
However, I'm not quite sure how to detect a return to the ajax. How can I make it detect whether its a true or false login? Thanks -
I'm using jQuery ajax for my login script. However my linked php file with ajax doesn't change the screen with header('location') whats a way to change the page on login using ajax? Thanks
-
Why am I getting a session_start header problem?
Eggzorcist replied to Eggzorcist's topic in PHP Coding Help
so I can't put it in the __construct of my function even if its included at the very top? -
My session start is in my session object and I include it at the top of my page prior my html code, yet I still get this warning: Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /Users/Sites/writepaps/index.php:2) in /Users/Sites/writepaps/includes/functions/sessions.php on line 11 I include the object in index line 2. and line 11 is where my session start is in my construct function <?php require_once("database.php"); require_once("user.php"); class Sessions { public $logged_in = false; protected $username; protected $password; function __construct(){ session_start(); $this->check_login(); } .... Why is this happening?
-
class Sessions { public $logged_in = false; protected $username; protected $password; function __construct(){ session_start(); check_login(); } public function is_logged_in(){ return $this->logged_in; } public function check_login(){ if(isset($_SESSION['browser']) && $_SESSION['browser'] == $_SERVER['HTTP_USER_AGENT'] && isset($_SESSION['ip']) && $_SESSION['ip'] == $_SERVER['REMOTE_ADDR']){ if(isset($_SESSION['username'])){ $this->logged_in = true; } else { $this->logged_in = false; } } else { $this->logged_in = false; } } ....
-
Hi, I'm fairly new to OOP so please bare with me. I've been given to understand __construct is put forth in order to do that as soon as the object is created. Now I've created an object to control sessions. so here is my construct code includes a function within that object and session_start(). However, I get the error that the function within that object is non-existent but the function is right underneath the __construct function. should I put __construct last thing in my object? ANy help on this issue would be excellent! Thank you.
-
How can I make my script so I can send a mail, and if that mail is sent successfully, then it goes to header('Location: index.php') ? I tried but I keep getting an error or it doesnt do anything... Any help would be great, thanks
-
This is the very first time this is happening to me. I have a register form and when I click register and there is an error it clears the entire form but I've never gotten this before... I think it might be in my xhtml code. <?php require_once('functions.php'); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Register</title> </head> <body> <div id="error_message"> <?php if(isset($_POST['register'])){ register_user($_POST['email'], $_POST['pw1'], $_POST['pw2'], $_POST['firstname'], $_POST['lastname']); } ?> </div> <form id="register_user" method="post" action="<?php $_SERVER['PHP_SELF']; ?>"> <label>E-mail: <input type="text" name="email" id="email" />* </label> <label>Password: <input type="password" name="pw1" id="pw1" />* </label> <label>Comfirm: <input type="password" name="pw2" id="pw2" />* </label> <br><br> <label>First Name: <input type="text" name="firstname" id="firstname" />* </label> <label>Last Name: <input type="text" name="lastname" id="lastname" />* </label> <br><br> <input type="submit" id="register" name="register" value="Register" /> </form> </body> </html>