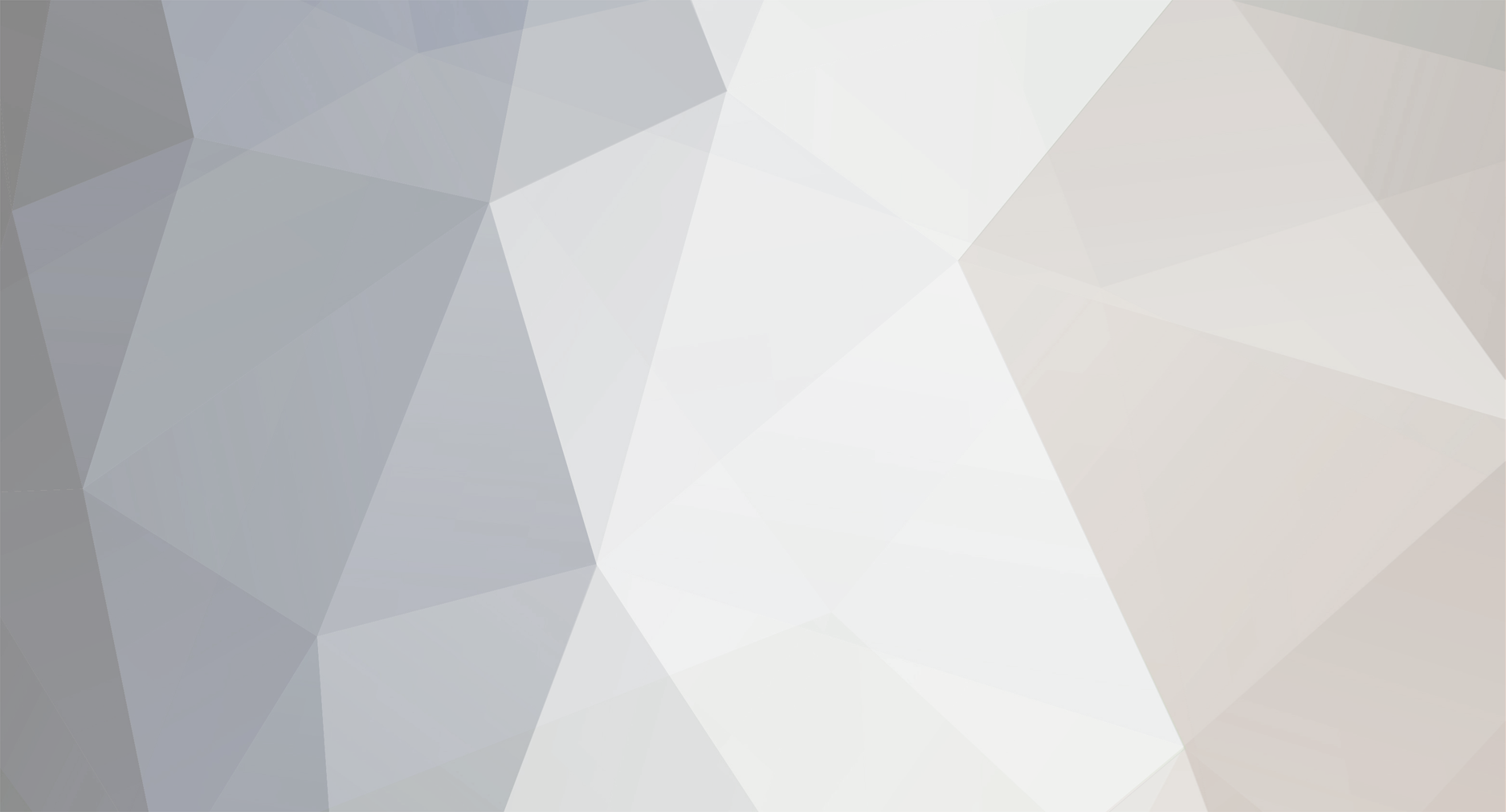
Brian W
-
Posts
867 -
Joined
-
Last visited
Posts posted by Brian W
-
-
try
"INSERT INTO `users` (`username`,`password`,`email`) VALUES (%s,%s,%s)"
for testing reasons. also use
mysql_query($insert) or die("Could not insert comment" . mysql_error().'<br>'.$insert);
that way you can see the query.
if that doesn't work, let us know what the query is (copy and past the output of the error report above)
-
Details please...
if a js problem, you'll want a debugger. I like Firebug for browser FireFox. it speaks English, not technical jib :-)
-
Please clarify what you want...
Here is my wild guess though:
You want to add the line
<img src="blabla.jpg">
to the $display variable?
if so,
$display .= '<img src="blabla.jpg">';
-
in code:
<?php //Find Rows $Result2= mysql_query("SELECT * FROM users WHERE ID='$ID'"); $Rows2= mysql_fetch_array($Result2); //Define User Variables $Pokémon= $Rows2['VolcanoValley']; $Pokémon2= $Rows2['VolcanoValley2']; //If Action Is Not Null if($Action=="catch") { //If Form Is Not Submitted if(!$Submit) { //Define Random Variables $PokémonRand= rand(1, 100); //If Pokémon Is Charmander if($PokémonRand >= 1 && $PokémonRand <= 25) { //Define Pokémon Variables $PokémonNumber= 1; $PokémonName="Charmander"; $PokémonImage="http://i13.tinypic.com/6xvsvw7.png"; } //If Pokémon Is Magby elseif($PokémonRand >= 26 && $PokémonRand <= 50) { //Define Pokémon Variables $PokémonNumber= 2; $PokémonName="Magby"; $PokémonImage="http://i10.tinypic.com/8also6f.png"; } //If Pokémon Is Numel elseif($PokémonRand >= 51 && $PokémonRand <= 75) { //Define Pokémon Variables $PokémonNumber= 3; $PokémonName="Numel"; $PokémonImage="http://i5.tinypic.com/73e7me8.png"; } //If Pokémon Is Nothing elseif($PokémonRand >= 76 && $PokémonRand <= 99) { //Define Pokémon Variables $PokémonNumber= 0; $PokémonName="Nothing"; $PokémonImage=""; } //If Pokémon Is Entei else { //Define Pokémon Variables $PokémonNumber= 4; $PokémonName="Entei"; $PokémonImage="http://i8.tinypic.com/7xxnyud.png"; } //If Pokémon Is Nothing if($PokémonName=="Nothing") { //Display Message echo "You have found nothing when searching for Pokémon.<br /><br /> <a href='map1.php?action=catch'><img border='0' src='http://i13.tinypic.com/8104aqe.jpg'></a>"; } //If Pokémon Is Not Nothing else { //Define Random Variables $PokémonRand2= rand(1, 49); //If Gender Is Male if($PokémonRand2 >= 1 && $PokémonRand2 <= 24) { //Define Gender Variables $PokémonGender="Male"; $PokémonGSign="(M)"; } //If Gender Is Female elseif($PokémonRand2 >= 25 && $PokémonRand2 <= 48) { //Define Gender Variables $PokémonGender="Female"; $PokémonGSign="(F)"; } //If Gender Is Ungendered elseif($PokémonRand2== 49) { //Define Gender Variables $PokémonGender="Ungendered"; $PokémonGSign="(U)"; } //If Gender Is Unknown else { //Define Gender Variables $PokémonGender="Male"; $PokémonGSign="(M)"; } //Update Information mysql_query("UPDATE users SET VolcanoValley='$PokémonNumber', VolcanoValley2='$PokémonGSign' WHERE ID='$ID'"); //Define User Variables //Display Form echo " <form action='?action=catch' method='POST'> <input type='hidden' name='Submit' value=1'> <img src='$PokémonImage' alt='$PokémonName' title='$PokémonName'><br /> <b>$PokémonName</b><br /><br /> You have encountered a wild $PokémonName <b>$PokémonGSign</b>!<br /><br /> <input type='submit' value='Catch $PokémonName' onclick=\"this.disabled='true'; this.value='Please Wait...'; this.form.submit();\"><br /><br /> </form>"; echo "<a href='map1.php?action=catch'><img border='0' src='http://i13.tinypic.com/8104aqe.jpg'></a>"; } } ?>
just to help me...
-
easy letter links
<?php $letters = "A B C D E F G H I J K L M N O P Q R S T U V W X Y Z"; $letters = explode(' ', $letters); foreach($letters as $alpha){ echo '<a href="?letter='.$alpha.'">'.$alpha.'</a> '; } ?>
-
with my version
<input type="submit" value="Submit" style="border:0px solid; width:150px; height:35px; background:url(Images/bg_button.gif);"/>you could add some javascript to ask them to confirm which is nice and if they don't have javascript enabled, the button simply just works without confirmation. unnecessary but cute...lol going above and beyond!
-
well, think this may be what you are looking for...
<?php $letter = $_GET['letter']; $system = $_GET['system']; $sql = "SELECT * FROM OSG_Games WHERE system_id = $system AND title LIKE '$letter%' ORDER BY title ASC"; $query = mysql_query($sql); while($row = mysql_fetch_array($query)) { echo $row['title']; echo "<br>"; }
-
I think this would also work... but am not 100%
<form method="post" action="unsub.php"> <div style="text-align: center;"> <span style="font-weight: bold;">Email Address </span> <input size="35" name="EmailAddress"> <br> </div> <input type="submit" value="Submit" style="border:0px solid; width:150px; height:35px; background:url(Images/bg_button.gif);"/> </form>
-
I've thought about this before... there isn't any easy way to do so as there isn't a SQL command to do so and when you start looking at even as little as 100+ records even a server side process of some sort gets ridiculous.
"why" is funny to me because whether they be crazy, dumb, or just OCD... it doesn't matter what they will do with it, the challenge of "how" is more important.
-
plain links don't submit forms. buttons or JavaScript can.
-
lol, asking why is funny.
"UPDATE `table_name` `key` = 3 WHERE `key` = 4"
table_name is your table name and key is the field that has those numbers
-
is there a reason u are using mysql_fetch_array() rather than simply using mysql_fetch_assoc()?
I'm not criticizing, just don't know what the advantage is for using mysql_fetch_array() ....
-
yeah, co-worker is an ASP developer.
So if system.byte is ASP, I guess that is not a mysql problem it is a ASP problem or both.
-
Okay, my co-worker who is an ASP developer has the entire office shitting bricks cuz the boss is shitting bricks. We moved a website onto our server yesterday seemingly without issues but not entirely without (of course)...
Though he is using ASP, this sounds like a mysql problem sorta.
What used to display First, middle, and last name and a float delimited by commas with something like CONCAT_WD(' ', Firstname, Middle, Last, Hours) now errors saying "system.byte[]".
What makes me think this is a mysql issue is that it worked on the old server which was running an older version of MySql, ODBC, and IIS6. Now it is on a system with the latest a greatest versions of mysql, ODBC and even IIS7. Huge environment changes like this make me paranoid... god bless testing the water and such but we didn't catch this bug till it hit production.
Any ideas? Thanks
-
Where I copied the headers from already had that. I changed it to ISO-8859-1 while using the UTF8_decode() but it still feed me the ?'s instead of symbols.
With UTF8_encode() and utf-8 as my charset i get:
â¦letâsand still if I have UTF8_decode() and utf-8 as my charset i get it with the ?'s
BUT! there is a BUT! I figured it out...
I used htmlspecialchars() with charset=UTF-8 and it worked!
I realized that what I needed was to not make it HTML safe but to make it HTML... lol
Thanks for the help guys.
-
I was just finished my test of utf8_decode() when I read your post, premiso...
my db handles the the symbols fine, just not the emails.
Little better now. This is what gets emailed now...
?let?sThis detail may or may not be effecting things. here is some of my code for the email:
$body = '<b>Summary: </b>"'.utf8_decode($_POST['Summary']).'"<br>'; $headers = "MIME-Version: 1.0\n"; $headers .= "Content-type: text/html; charset=us-ascii\n";
-
umm. lol
this is what I got with the same input as before in the example (...let’s)
matrix…let’sNot quite right I'd say. Any other ideas?
Thanks for the input zanus anyways, I'm going to keep looking following the same train of thought.
-
Whats up with the ads? They aren't even relevant! Make them something good (like software) and I'd click em. lol
I see em as Youtube advertisements... is that different for other people?
I won't see them for long though, I think I will join the donated list cuz this website is handy.
Thanks for the hard work guys
-
Need some help here with weird symbols. First off, here is the situation:
I have a form that posts text to a page to be processed. The input is a normal HTML text field.
The php page that processes the data first inputs the text into my mysql database and if successful, sends an email to an email address I've specified.
Sometimes I get weird things like "…let’s" (which is supposed to be ...let's). I've narrowed it down to the fact that some people like to copy text from things like MS WORD into my text field which doesn't email pretty when they use symbols that are not HTML friendly (Examples: "…" instead of "..."{there is a difference} and " ’ " instead of " ' ").
How do I convert their input into HTML friendly text?
Thanks in advance.
-
Your looking at needing Ajax my friend. You could find out how to do that yourself or you could pay one of the code monkeys around here to do it but It is in your favor to learn how it is done.
Here is a brief overview of how I would do it (which may not be the best way...)
Create three divs with id's "Cabinet", "Folder", "Item".
Create two other php pages that only contain a dynamically created menu based on a $_GET value.
:::This would likely be a unique ID passed from the above level:::
Use java script to trigger pulling in your "Folder" page into the div "Folder" with a URL variable to pass the "Cabinet" ID or whatnot.
Same thing for the "Folder" level to the "Item" level.
-
yeah, that would be a sure sign that someone needs to be fired. lol
Hope it does the job for ya. I'll tag this thread to notify, so let me know if it works else I'll help you.
-
THANK YOU FOR CLARIFYING!!! lol jk, but that does help.
on this line i gave you:
echo "<a href=\"paystubs.php?Date=".$Date."\">";
instead say
echo "<a href=\"paystubs.php?Date=".$endingdate."\">";
Then, in your query, say
...WHERE `date_column`=".$_GET['Date']."...
that should pass your block date (12152008) to the next page specified by the link above and make it usable for the query.
Additional comment: use
if(!is_numeric($_GET['Date'])){ die('Bad Date Input'); }
That will prevent SQL injection (type of hacking if you didn't know)...
-
oh yes, and to make it into a $_SESSION var, add this to the top of your pages that will use the $_SESSION var.
session_start();
and add this to that page
$_SESSION['the_name_of_the_session_you_want'] = $_GET['Date'];
Its hard for me to know what you want at this point because you'r a little all over the place. LOL
-
That is not a form dude. Your form won't use links like that. So, forget the form if you wan't, but you'll need to stop trying to use the like they are Inputs. Lets do this with $_GET then.
<?php $RESULTDS=mssql_query("SELECT DISTINCT M2.[EMPNO], PS.[PSTUB5] FROM MASTERL2 M2 LEFT JOIN PAYSTUBS PS ON PS.[PSTUB2]=M2.EMPNO WHERE M2.[EMPNO] = '".$_SESSION['empcode']."' ORDER BY PS.[PSTUB5]"); $RESULT=mssql_fetch_assoc($RESULTDS); mssql_data_seek($RESULTDS,0); echo "<CENTER>"; echo "<font size=2 color=#000000 face=arial>"; echo "Please select a stub to view:"; echo "</font>"; echo "</CENTER>"; echo "<BR>"; echo "<CENTER><font size=2 color=#0000ff face=arial>"; while ($RESULT = mssql_fetch_assoc($RESULTDS)) { $endingdate = $RESULT['PSTUB5']; $month = substr("$endingdate", -8, 2); $day = substr("$endingdate", -6, 2); $year = substr("$endingdate", -4, 4); $Date = $month."-".$day."-".$year; echo "<a href=\"paystubs.php?Date=".$Date."\">"; echo $Date; echo "</a>"; } echo "</font> </CENTER>"; echo "<BR>"; ?>
Sorry, changed your date format to use "-" instead of "/" because you shouldn't use / in the URL unless you are using it correctly (for directories). To use the variable as a $_GET variable: once its been clicked on and the variable is added to the URL query string, use echo $_GET['Date'].
I'm not sure how you are using advanced SQL and even some pretty non novice PHP functions and not be using forms correctly.
If you want to still use a drop down menu, you (or I) can do so by using some javascript.
BTW, why are you even bothering breaking the date up if your just going to put it back together?
[SOLVED] A simple time script
in PHP Coding Help
Posted
$time will be 0-23 according to http://us2.php.net/date...
best of luck