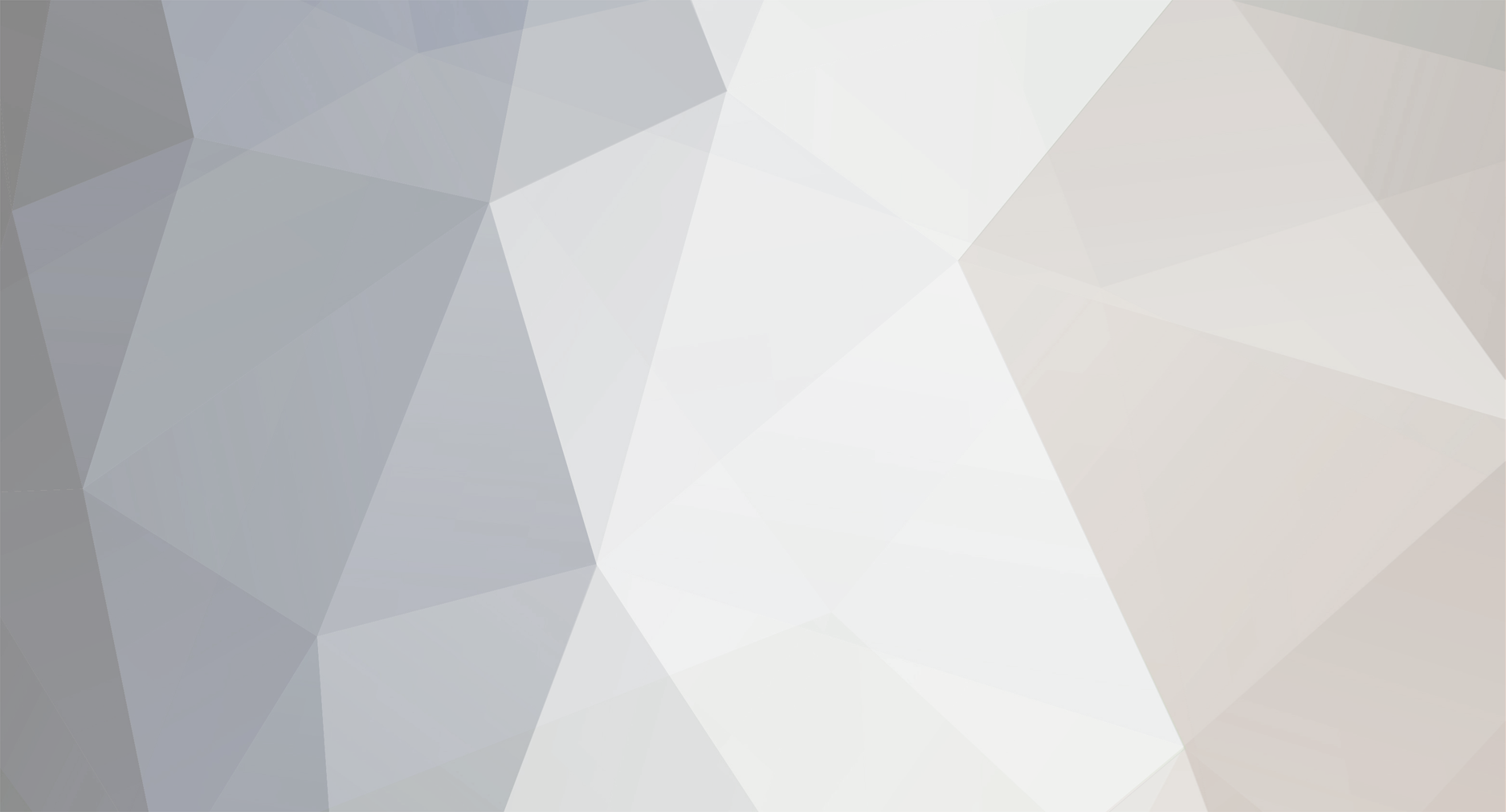
gsaldutti
Members-
Posts
32 -
Joined
-
Last visited
Everything posted by gsaldutti
-
I have a football pool where players pick FOUR teams to win each week, and the only rule is that they can't use the same team more than THREE times. So in my code, I have an basic array for all 32 teams.. $teamsArray = array( 'Philadelphia Eagles', 'Dallas Cowboys', 'New York Giants', 'Washingtong Redskins', 'Detroit Lions', 'Minnestoata Vikings', 'Green Bay Packers', 'Chicago Bears', 'Tampa Bay Buccs', 'New Orleans Saints', 'Carolina Panthers', 'Atlanta Falcons', 'Seattle Seahawks', 'San Francisco 49ers', 'St. Louis Rams', 'Arizona Cardinals', 'New York Jets', 'Miami Dolphins', 'Buffalo Bills', 'New England Patriots', 'Baltimore Ravens', 'Cincinnati Bengals', 'Pittsburgh Steelers', 'Cleveland Browns', 'Houston Texans', 'Tennessee Titans', 'Jacksonville Jaguars', 'Indianapolis Colts', 'Denver Broncos', 'Kansas City Chiefs', 'Oakland Raiders', 'San Diego Chargers', 'BYE'); Next I have a MySQL query to find out how many times teams have been used (see code below). Then it produces a little HTML box listing out each team that user has picked and how many times they were used. Works fine. Then right after that code, I have the submission form (with four DropDown lists, each showing all 32 teams). But that first listing of teams and the # of times used probably woudn't even be necessary AT ALL if I could just write some PHP that would look to the DropDown lists (they are just HTML SELECT lists with 32 OPTIONS....actually 33 because players can choose a BYE) and know specifically which teams in the list of 32 have been used THREE times. Ideally, it would just look to the "class" and change it from "teamdropdown" to "teamdropdownNO" and then in my CSS I could make the "teamdropdownNO" class have "text-decoration: strike-trough" (so the teams used 3 times had a line through them) or maybe just us "Display: none" so the teams used three times didn't even show up at all. But I cannot figure out how to change strings in the DropDown lists for just certain teams (i.e. the teams used three times) in the FOR LOOP. I've tried "str_replace" but cannot get it to work. I'm not asking anyone to write the code for me, but if someone can at least point me in the right direction of how this can/shoud be done, I would REALLY appreciate it. <form action="submit.php" method="post" /> <table id="submitform"> <?php echo "<div id='teamsused'>"; echo "<p class='reminder'>Remember, you can only use each team THREE times, maximum!</p>"; echo "<p class='teamsusedheader'>List of Teams Used (# of times used so far is in parentheses):</p>"; $query = "SELECT picks.teamid, COUNT(*) AS count, teams.teamname FROM picks, teams WHERE userid = '{$_SESSION['userid']}' AND picks.teamid = teams.teamid GROUP BY teamid ORDER BY count DESC"; $getTeams = mysql_query($query, $connection); if (!$getTeams) { die("Database query failed: " . mysql_error()); } while ($results = mysql_fetch_array($getTeams)) { if ($results['count'] > 3) { echo "<span class='four'>" . $results['teamname'] . " (" . $results['count'] . ") ***** You used this team TOO MANY TIMES.<br/> CONTACT GARY TO DISCUSS!!!!</span><br/>"; } elseif ($results['count'] == 3) { echo "<span class='three'>" . $results['teamname'] . " (" . $results['count'] . ") You CANNOT pick this team anymore</span><br/>"; } elseif ($results['count'] == 2) { echo "<span class='two'>" . $results['teamname'] . " (" . $results['count'] . ") You can only pick this team ONCE MORE</span><br/>"; } else { echo "<span class='one'>" . $results['teamname'] . " (" . $results['count'] . ")</span><br/>"; } } echo "</div>"; //Begin code for Pick Submission form for($x=1; $x<=4; $x++){ echo "\n<tr><td>Pick ".$x.':</td>'; echo "\n<td><select name=\"pickArray[]\"/>"; echo "\n<option class='' value=''></option>"; for($y=0; $y<count($teamsArray); $y++){ $teamID = $y+1; $teamdropdown = "\n<option class='teamdropdown' value='{$teamID}'>{$teamsArray[$y]}</option>"; echo $teamdropdown; } echo "\n</select>"; echo "\n</td>"; echo "</tr>"; } echo "\n<tr><td>Week:</td>"; echo "\n<td><select name=\"week\"/>"; echo "\n<option class='' value=''></option>"; for($x=2; $x<=17; $x++){ echo "\n<option class='' value='$x'>$x</option>"; } echo "\n</select>"; echo "\n".'<input type="submit" name="submit" value="Submit Picks" class="submitpicksbutton" /></td>'; echo '</tr></table></form>'; ?>
-
Ok, awesome, thanks. Please don't laugh at my inexperience, but how do I actually display the list of returned Teams? I wrote the following code but it just returns "Resource ID 25" (and I can't find any information on what this error means). There must be more required to display the teams than simply "echo $getTeams;" ... $query = "SELECT teamid,COUNT(*) AS count FROM picks WHERE userid = '{$_SESSION['userid']}' GROUP BY teamid ORDER BY teamid"; $getTeams = mysql_query($query, $connection); if (!$getTeams) { die("Database query failed: " . mysql_error()); } else { echo $getTeams;
-
Hi all, I have a football website where you pick FOUR winners each week, but you can only use each team THREE times throughout the year. There are 32 teams in the NFL. So I started writing code below which works, but is DEFINITELY not the best way to do it because I'm sure it can be simplified much more using a loop of some sort. I'm hoping someone here can point me in the right direction because I'm still pretty new to PHP. The code below simply grabs the specific teamid from the "picks" table and finds how many times the logged-in person picked that team. If it's = 3, that team's name shows up in a list of "teams they can't pick anymore." If it's more than 3, there is a warning message that they used them too many times and to contact me. As you can see, there is a block of bulky code for EACH of the 32 teams (I only included the Eagles and Cowboys below because I'm sure you get the idea). I imagine it's really bad programming to make the code connect to the databsae 32 separate times, so I'm hoping someone can tell me how to contact the database just once, and then do the same stuff below using a loop of some sort. Instead of $getEagles, I know I could just do $getTeams and NOT specify a specific "teamid" in the SQL Statement, but then I don't know how to manipulate the returned set of ALL the teams. Any help would be greatly appreciated. Thanks <?php $getEagles = "SELECT teamid FROM picks WHERE picks.userid = '{$_SESSION["userid"]}' AND picks.teamid = '1' "; $Eagles = mysql_query($getEagles, $connection); if (!$Eagles) { die("Database query failed: " . mysql_error()); } $numEagles = mysql_num_rows($Eagles); if ($numEagles == 3) { $teamsdone = "Eagles<br/> "; } elseif ($numEagles > 3) { $teamsdone = "<span class='problem'>Eagles (You picked them TOO MANY TIMES. Contact Gary about this.)<br/></span>"; } else { } $getCowboys = "SELECT teamid FROM picks WHERE picks.userid = '{$_SESSION["userid"]}' AND picks.teamid = '2' "; $Cowboys = mysql_query($getCowboys, $connection); if (!$Cowboys) { die("Database query failed: " . mysql_error()); } $numCowboys = mysql_num_rows($Cowboys); if ($numCowboys == 3) { $teamsdone .= "Cowboys<br/>"; } elseif ($numCowboys > 3) { $teamsdone .= "<span class='problem'>Cowboys (You picked them TOO MANY TIMES. Contact Gary about this.)<br/></span>"; } else { } //Then the same code above would be list THIRTY more times for the other 30 teams. Far from efficient. if (!$teamsdone) { echo "<p class='teamsdoneheader'>Teams you CANNOT pick again:</p>"; echo "<p class='teamsdone'> None yet </p>"; } else { echo "<p class='teamsdoneheader'>Teams you CANNOT pick again:</p>"; echo "<p class='teamsdone'>" . $teamsdone . "</p>"; } ?>
-
[SOLVED] Using ereg_replace MULTIPLE times?
gsaldutti replied to gsaldutti's topic in PHP Coding Help
I'm so sorry... I had ['row1'] written in my code later down the page by accident. i just caught it and when I changed it to ['row'] your code worked perfectly. Sorry about that. Thank you very much for your help. And as for your question about whether I wrote the code or not, I would say I wrote about half of it (and it took me a long time ). Someone helped me with sorting function and basically wrote all the parts dealing with that. Anyway, thanks again. I'm sure this is cake for you, but I'll get there some day -
[SOLVED] Using ereg_replace MULTIPLE times?
gsaldutti replied to gsaldutti's topic in PHP Coding Help
It does make sense, thank you. And using the arrays makes sense too, but it's not working for some reason. I replaced my original code with the following code and nothing outputs now... $find = array('RecordPlaceHolder', 'name'); $replace = array($totalWinLossText, 'name1'); $userResArray[$userCount]['row'] = str_replace($find, $replace, $userRow); $userResArray[$userCount]['percentage'] = $successPercentage; $userCount++; -
[SOLVED] Using ereg_replace MULTIPLE times?
gsaldutti replied to gsaldutti's topic in PHP Coding Help
Ok, I'm using str_replace now (thanks for the tip), but I still run into the same problem where I can only do ONE str_replace. If I list two in a row, it only does the second one. I'm sure I'm missing something SUPER obvious, but I can't figure it out. -
Hi all, I included the entire code at the bottom of this post (not sure if I need to or not) but the part I'm having trouble with is the ereg_replace function seen in this part below. $userResArray[$userCount]['row'] = ereg_replace('RecordPlaceHolder', $totalWinLossText, $userRow); $userResArray[$userCount]['percentage'] = $successPercentage; $userCount++; This way above works fine, but what if I also want to do ANOTHER ereg_replace, in addition to the one that is already there. I tried this way below but it only does the last (i.e. the second) ereg_replace... $userResArray[$userCount]['row'] = ereg_replace('RecordPlaceHolder', $totalWinLossText, $userRow); $userResArray[$userCount]['row'] = ereg_replace('name', 'name1', $userRow); $userResArray[$userCount]['percentage'] = $successPercentage; $userCount++; Any help with how to do MULTIPLE ereg_replaces would be greatly appreciated. Also, as a side question (and please don't laugh at my inexperience ), but can someone please briefly explain what the "['row']" index does (i.e. where it has "$userResArray[$userCount]['row'] "), and explain it like I'm a five year old . Is that index a default already built-in to PHP? Thanks! FULL CODE IF NECESSARY: <?php require_once("includes/session.php"); require_once("includes/connection.php"); require_once("includes/functions.php"); include_once("includes/form_functions.php"); $username=""; $password = ""; if(isset($_POST['submit'])) { $errors = array(); $required_fields = array('username','password'); $errors = array_merge($errors, check_required_fields($required_fields, $_POST)); $fields_with_lengths = array('username' => 30, 'password' => 30); $errors = array_merge($errors, check_max_field_lengths($fields_with_lengths, $_POST)); $username = trim(mysql_prep($_POST['username'])); $password = trim(mysql_prep($_POST['password'])); $hashed_password = sha1($password); if ( empty($errors) ) { // check database to see if username and hashed password exist there $query = "SELECT userid, username "; $query .= "FROM users "; $query .= "WHERE username = '{$username}' "; $query .= "AND hashed_password = '{$hashed_password}' "; $query .= "LIMIT 1"; $result_set = mysql_query($query); confirm_query($result_set); if (mysql_num_rows($result_set) > 0) { //username/password authenticated // and only 1 match $found_user = mysql_fetch_array($result_set); $_SESSION['userid'] = $found_user['userid']; $_SESSION['username'] = $found_user['username']; redirect_to("submit.php?userid={$_SESSION['userid']}"); } else { // username/password combo was not found in database $message = "Username/password combination is incorrect.<br /> Please make sure your caps lock key is off and try again."; } } } if(isset($_GET['logout']) && $_GET['logout'] == 1) { $message = "You are now logged out."; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>GSFL 2008 - League Standings</title> <link href="stylesheets/gsfl2008.css" media="all" rel="stylesheet" type="text/css" /> </head> <div><img src="images/gsfllogo.gif"></div> <div id="menu"> <p><a href="http://gdoot.com">Go to gdoot.com homepage</a></p> <p><a href="rules.php">Read the Rules</a></p> <p><a href="print.php" target="_blank">Printable Picks</a></p> </div> <div id="login"> <form action="index.php" method="post"> <table id="login"> <tr> <td>Username:</td> <td><input type="text" name="username" maxlength="30" value="<?php echo htmlentities($username); ?>" /></td> </tr> <tr> <td>Password:</td> <td><input type="password" name="password" maxlength="30" value="<?php echo htmlentities($password); ?>" /></td> </tr> <tr> <td colspan="2"><input type="submit" name="submit" value="Login to Submit Picks" class="submit" /></td> </tr> </table> </form> <?php if(!empty($message)) { echo "<p class=\"message\">" . $message . "</p>"; }?> </div> <?php function sortMultiArray($myRowArr, $assocOrNumericfieldIndex, $ascORdesc = 'asc'){ //Author: Dan //Refer by whichever type of index you know is available, (associative or numeric) //unset($myrow); //strange I had to unset this before using it. it was appending blank rows at the end for //some reason. actually doing the tradtional $myrow = array(); has the same effect $retArr = array(); $rowCount = count($myRowArr); if($rowCount){ $arrKeys = array_keys($myRowArr[0]); for ($x=0;$x<$rowCount;$x++) { $colarr[$x] = $myRowArr[$x][$assocOrNumericfieldIndex]; } //showArray($colarr); reset($arrKeys); //showArray($arrKeys); if(strtolower($ascORdesc) == 'asc'){ asort($colarr); // Use arsort=DESC or asort=ASC } elseif(strtolower($ascORdesc) == 'desc'){ arsort($colarr); // Use arsort=DESC or asort=ASC } $r = 0; for($sortedvar = reset($colarr); $sortedvar; $sortedvar= next($colarr)) { $rank = key($colarr); for ($y=0;$y<count($myRowArr[0]);$y++) { $retArr[$r][$arrKeys[$y]] = $myRowArr[$rank][$arrKeys[$y]]; } $r++; } } return($retArr); } echo "<p class=\"importantinfo\">Your \"pending\" picks will remain hidden until 12:59 PM on Sunday (NOTE: You can see your \"pending\" picks if you login)</p>"; $outputStart = "<div id='standings'> <table class=\"standings\" cellpadding='1' cellspacing='1'> <tr> <th class='name'>Player</th> <th>Week 1</th> <th>Week 2</th> <th>Week 3</th> <th>Week 4</th> <th>Week 5</th> <th>Week 6</th> <th>Week 7</th> <th>Week 8</th> <th>Week 9</th> <th>Week 10</th> <th>Week 11</th> <th>Week 12</th> <th>Week 13</th> <th>Week 14</th> <th>Week 15</th> <th>Week 16</th> <th>Week 17</th> </tr>"; $user_set = mysql_query("SELECT * FROM users", $connection); if (!$user_set) { die("Database query failed: " . mysql_error()); } $userResArray = array(); $userCount = 0; while ($users = mysql_fetch_array($user_set)) { $userRow = "<tr><td class='name'>{$users["firstname"]}" . " " . "{$users["lastname"]}<br/><img src=\"http://gdoot.com/gsfl/images/user_{$users["userid"]}.jpg\" /> <span class=\"record\">RecordPlaceHolder</span></td>"; /* $userRow .= '<td class="record">RecordPlaceHolder</td>'; */ $day = date("w"); $time = time("Hi"); $wins = 0; $losses = 0; for($x = 1; $x <= 17; $x++){ $userRow .= '<td>'; $sql = "SELECT teamname, result FROM teams, picks WHERE picks.userid = '{$users["userid"]}' AND picks.teamid = teams.teamid AND picks.week = '$x' "; $pick_set = mysql_query($sql, $connection); if (!$pick_set) { die("Database query failed: " . mysql_error()); } if(mysql_num_rows($pick_set)){ $userRow .= "<table class='inset' cellspacing='0' cellpadding='1'>\n"; while ($picks = mysql_fetch_array($pick_set)) { if ($picks['result'] == 'w') { $userRow .= "<tr><td class='pickwin' rowspan='1'>{$picks['teamname']}</td> </tr>\n"; $wins++; } elseif ($picks['result'] =='l') { $userRow .= "<tr><td class='pickloss' rowspan='1'>{$picks['teamname']}</td> </tr>\n"; $losses++; } elseif ((($picks['result'] == 'o') && ($day == 0) && ($time > "1259")) || ($day == 1)) { $userRow .= "<tr><td class='pickopen' rowspan='1'>{$picks['teamname']}</td> </tr>\n"; } else { $userRow .= "<tr><td class='pickopenhidden' rowspan='1'> <span class=\"hide\">{$picks['teamname']}</span></td> </tr>\n"; } } $userRow .= "</table>\n"; } else { $userRow .= ' '; } $userRow .= '</td>'; } $userRow .= "</tr>\n"; $totalPicks = $wins + $losses; $successPercentage = 0; $totalWinLossText = ''; if($totalPicks > 0){ $successPercentage = $wins / $totalPicks; $totalWinLossText = " $wins - $losses"; } $userResArray[$userCount]['row'] = ereg_replace('RecordPlaceHolder', $totalWinLossText, $userRow); $userResArray[$userCount]['percentage'] = $successPercentage; $userCount++; } $userResArraySorted = sortMultiArray($userResArray, 'percentage', 'desc'); $outputEnd = "</table>\n"; $outputEnd .= "</div>\n"; //Write the data table now echo $outputStart; for($x = 0; $x < count($userResArraySorted); $x++){ echo $userResArraySorted[$x]['row']; } echo $outputEnd; ?> </body> </html> <?php require_once("includes/footer.php"); ?>