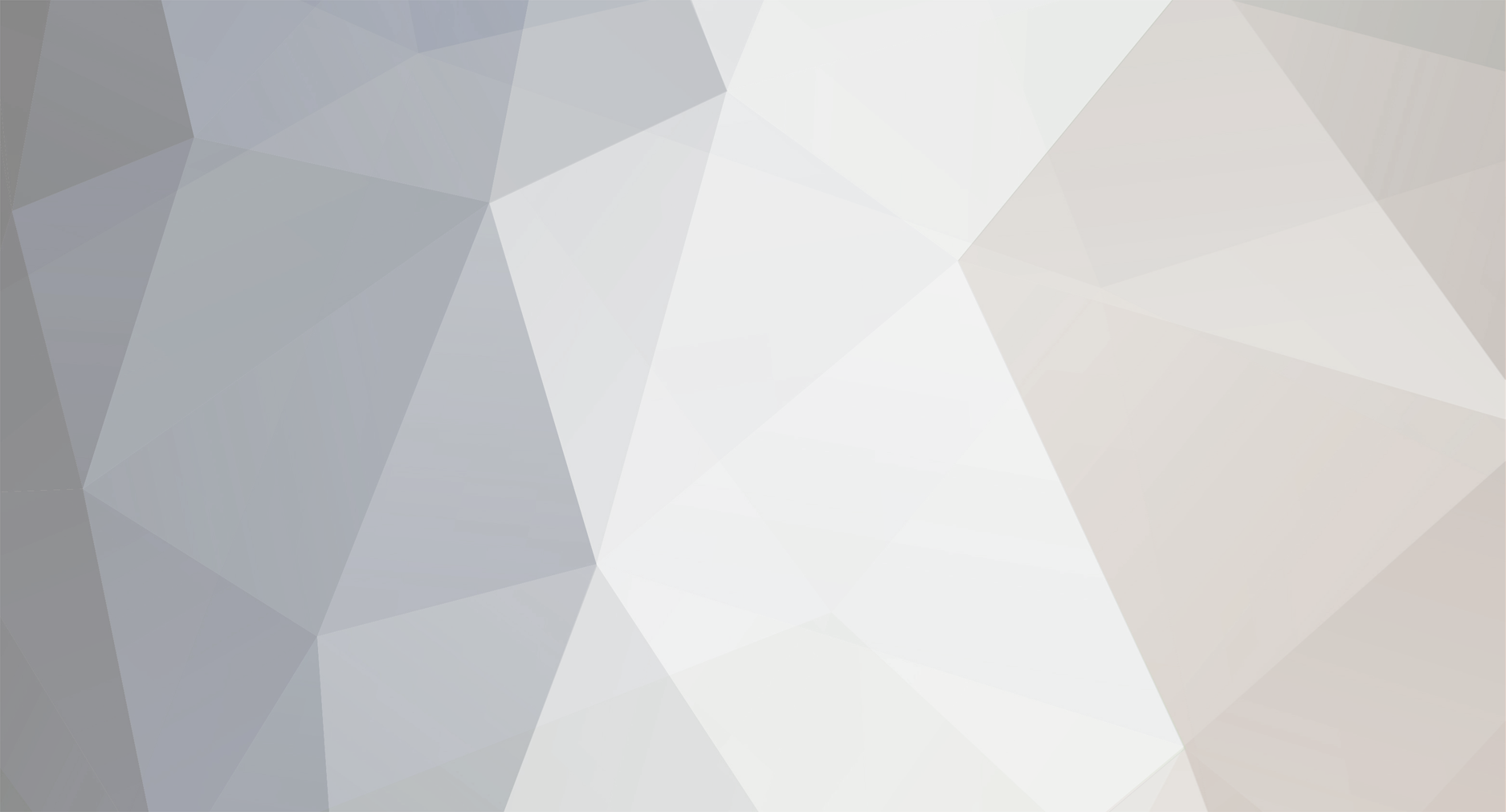
Dan06
Members-
Posts
103 -
Joined
-
Last visited
Never
Everything posted by Dan06
-
Thanks for the help. I followed your suggestions and got the code to work. Best, Dan
-
The following code is supposed to return a variable containing text, currently the code posts the data but returns an "undefined" variable. Can someone tell me what's wrong? Thanks. function ajaxPost(params, url){ var XMLHttpRequestObj = false; if (window.XMLHttpRequest){ XMLHttpRequestObj = new XMLHttpRequest(); } else if (window.ActiveXObject){ XMLHttpRequestObj = new ActiveXObject("Microsoft.XMLHttp"); } if (XMLHttpRequestObj){ XMLHttpRequestObj.open("POST", url, true); XMLHttpRequestObj.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); XMLHttpRequestObj.setRequestHeader("Content-length", params.length); XMLHttpRequestObj.setRequestHeader("Connection", "close"); XMLHttpRequestObj.onreadystatechange = function(){ if (XMLHttpRequestObj.readyState == 4 && XMLHttpRequestObj.status == 200){ var requestResponse = XMLHttpRequestObj.responseText; delete XMLHttpRequestObj; return (requestResponse); } } XMLHttpRequestObj.send(params); } }
-
Uploading multiple files - request entity or php issue?
Dan06 replied to Dan06's topic in PHP Coding Help
Thanks for the suggestion; I tried it but it didn't solve the problem. To figure out the problem, I printed the array, below is the result: Based on the above, my guess is the problem is the request entity. Anyone have anymore suggestions? -
I'm having trouble uploading multiple files at the same time. For example, if I send two files at the same time, the first file is uploaded but the second is seemingly ignored. I don't know if the problem is with the request entity or the php code. Does anyone know which is the problem? Thanks. Below is the "Request Entity" that is submitted to the server: --3D6atZKLBPujpCZzClN-wz3AJ0Eh-BBWlRr Content-Disposition: form-data; name="imgFile[]"; filename="Inbox_Msg_Display.GIF" Content-Type: application/octet-stream; charset=ISO-8859-1 Content-Transfer-Encoding: binary --3D6atZKLBPujpCZzClN-wz3AJ0Eh-BBWlRr-- --A2cG-IXHLNC2Rk7kF3fQ5-bQPQpuDwmUU Content-Disposition: form-data; name="imgFile[]"; filename="Table_Msg_Content.GIF" Content-Type: application/octet-stream; charset=ISO-8859-1 Content-Transfer-Encoding: binary --A2cG-IXHLNC2Rk7kF3fQ5-bQPQpuDwmUU-- Following is the code I'm using to process the request entity: for ($i = 0; $i < count($_FILES['imgFile']); $i++){ $targetPath = "profile_images/"; $targetPath = $targetPath . basename($_FILES['imgFile']['name'][$i]); move_uploaded_file($_FILES['imgFile']['tmp_name'][$i], $targetPath); }
-
I'm using a query that selects all records that have a particular recipient and matching ids. I want to refine the select criteria to include one record from each group based on recipient, message id, and latest date. The records are grouped based on "threadId." How can I do this? There are two tables involved: message_inbox and message_content. The message_content table has the following fields: msgId, threadId, msgParent, msgChild, msgDate, msgSubject, msgContent The message_inbox table has the following fields: msgRecipient, msgSender, msgId Here is my current mysql query: $messagesQuery = sprintf("SELECT msgSender, message_content.msgId, msgDate, msgSubject, msgContent FROM utilist.message_inbox, utilist.message_content WHERE msgRecipient = %s AND message_inbox.msgId = message_content.msgId", $format->formatValue($_SESSION["userId"]));
-
The problem seems to be caused because I assigned mysql_fetch_assoc($msgQueryResult) twice. Below is the entire code. I used the do rather than a while loop because when I used a while loop the image query did not work. class messageDetails{ public function displayDetails($msgLinkEnd){ $format = new stringFormat(); $dbconn = new dbConnection(); $msgParentQuery = sprintf("SELECT message_content.msgParent FROM utilist.message_content WHERE message_content.msgId = %s", $format->formatValue($msgLinkEnd)); $parentQueryResult = mysql_query($msgParentQuery, $dbconn->conn()) or die("Parent Message Query Error: " . $msgParentQuery . mysql_error()); $parentId = mysql_fetch_assoc($parentQueryResult); $messageQuery = sprintf("SELECT msgSender, message_content.msgId, message_content.msgParent, msgDate, msgContent FROM utilist.message_outbox, utilist.message_content WHERE message_content.msgId = %s AND message_outbox.msgId = message_content.msgId", $format->formatValue($parentId["msgParent"])); $msgQueryResult = mysql_query($messageQuery, $dbconn->conn()) or die("Outbox Message Query Error: " . $messageQuery . " " . mysql_error()); $msgFields = mysql_fetch_assoc($msgQueryResult); $membershipIdQuery = sprintf("SELECT membershipId FROM utilist.user_information WHERE userId = %s", $format->formatValue($msgFields["msgSender"])); $memIdQueryResult = mysql_query($membershipIdQuery, $dbconn->conn()) or die("Membership Id Query Error: " . $membershipIdQuery . " " . mysql_error()); $membershipIdValue = mysql_fetch_assoc($memIdQueryResult); $profileImgQuery = sprintf("SELECT profileImage FROM utilist.profile WHERE membershipId = %s", $format->formatValue($membershipIdValue["membershipId"])); $profileImgResult = mysql_query($profileImgQuery, $dbconn->conn()) or die("Profile Image Query Error: " . $profileImgQuery . " " . mysql_error()); $profileImgLoc = mysql_fetch_assoc($profileImgResult); while ($msgInfo = mysql_fetch_assoc($msgQueryResult)){ echo '<div class="displayMsg">' . '<img src="' . $profileImgLoc["profileImage"] . '" width="100px" height="100px" />' . ' ' . $msgInfo["msgDate"] . ' ' . '<a href=message_details.php?msgId=' . $msgInfo["msgId"] . '>' . $msgInfo["msgContent"] . '</a>' . '</div>'; } /* if ($msgInfo["msgParent"] != NULL || $msgInfo["msgParent"] != "NULL" || $msgInfo["msgParent"] != ""){ $this->displayDetails($msgInfo["msgId"]); } else{ return true; } */ } // end displayDetails function } // end messageDetails class
-
I have a php object that performs a series of sql queries and displays the results, the object is supposed to call itself if a particular value is not null. Currently, the object works correctly the first time through the code - the correct information is displayed. But when it comes to calling itself something goes wrong. The page displays the following error: Below is the php object code, anyone know where I've gone wrong? Thanks. class messageDetails{ public function displayDetails($msgLinkEnd){ $format = new stringFormat(); $dbconn = new dbConnection(); ... SQL QUERIES ... do{ echo '<div class="displayMsg">' . '<img src="' . $profileImgLoc["profileImage"] . '" width="100px" height="100px" />' . ' ' . $msgInfo["msgDate"] . ' ' . '<a href=message_details.php?msgId=' . $msgInfo["msgId"] . '>' . $msgInfo["msgContent"] . '</a>' . '</div>'; } while ($msgInfo = mysql_fetch_assoc($msgQueryResult)); if ($msgInfo["msgParent"] != NULL || $msgInfo["msgParent"] != "NULL" || $msgInfo["msgParent"] != ""){ $this->displayDetails($msgInfo["msgId"]); } else { return true; } } // end displayDetails function } // end messageDetails class
-
I'd like to create an app that performs multiple file upload. I'm new to java so I'm not sure exactly how to go about accomplishing my goal. Is it best to create an app and then use web start? Or is there another, possibly better way? Does anyone know of any tutorials, books, etc. that have examples or cover upload applications? Thanks
-
Thanks, changing = to IS resolved the issue.
-
I used parentheses as shown below: SELECT msgSender, message_content.msgId, msgDate, msgContent FROM utility.message_inbox, utility.message_content WHERE msgRecipient = "o4jd9sk1" AND (message_inbox.msgId = message_content.msgId AND message_content.msgChild = "NULL") but an empty result set was returned. It seems that the cause of the problem is the: AND message_content.msgChild = "NULL" statement. When I changed the aforementioned statement to: AND message_content.msgId = "1" the result set showed the expected content. Currently, the msgChild field is by default set to NULL, it's supposed to indicate that the current message is the last one in the thread since it has no children. Is the error in my statement or elsewhere?
-
How can I have a mysql query with 3 (or more) Where clauses (I know Where only takes 2). The following code doesn't work, but it demonstrates what I'd like to achieve: SELECT msgSender, message_content.msgId, msgDate, msgContent FROM utility.message_inbox, utility.message_content WHERE msgRecipient = "o4jd9sk1" AND message_inbox.msgId = message_content.msgId AND message_content.msgChild = "NULL"
-
Thanks for the clarification... I was confused because I incorrectly thought that each time the $msgInfo = mysql_fetch_assoc($msgQueryResult) code is called it automatically starts from the beginning, i.e. the first array set.
-
In my php page, in the head section I perform a few mysql queries. I then use these queries to display page content in the body. I don't understand why, but when I use a while loop only the latest/last set of queried information is displayed. However, when I use a Do while loop all the queried information is displayed. Can someone help me understand what is going on? Here is my code: <html> <head> <?php include("objects.php"); $dropmenu = new dropmenuDisplay(); $format = new stringFormat(); $dbconn = new dbConnection(); session_start(); $messagesQuery = sprintf("SELECT msgSender, message_content.msgId, msgDate, msgContent FROM utility.message_inbox, utility.message_content WHERE msgRecipient = %s AND message_inbox.msgId = message_content.msgId", $format->formatValue($_SESSION["userId"])); $msgQueryResult = mysql_query($messagesQuery, $dbconn->conn()) or die("Inbox Message Query Error: " . $messagesQuery . " " . mysql_error()); $msgInfo = mysql_fetch_assoc($msgQueryResult); $membershipIdQuery = sprintf("SELECT membershipId FROM utility.user_information WHERE userId = %s", $format->formatValue($msgInfo["msgSender"])); $memIdQueryResult = mysql_query($membershipIdQuery, $dbconn->conn()) or die("Membership Id Query Error: " . $membershipIdQuery . " " . mysql_error()); $membershipIdValue = mysql_fetch_assoc($memIdQueryResult); $profileImgQuery = sprintf("SELECT profileImage FROM utility.profile WHERE membershipId = %s", $format->formatValue($membershipIdValue["membershipId"])); $profileImgResult = mysql_query($profileImgQuery, $dbconn->conn()) or die("Profile Image Query Error: " . $profileImgQuery . " " . mysql_error()); $profileImgLoc = mysql_fetch_assoc($profileImgResult); ?> </head> <body> <?php while ($msgInfo = mysql_fetch_assoc($msgQueryResult)){ echo '<div class="displayMsg">' . '<img src="' . $profileImgLoc["profileImage"] . '" width="100px" height="100px" />' . ' ' . $msgInfo["msgDate"] . ' ' . $msgInfo["msgContent"] . '</div>'; } ?> </body> </html>
-
There were 2 problems: 1. var p = null; was inside the callback function, it needed to be outside of the function 2. The word suggestion in document.getElementById("suggestionBox")... was misspelled. Once the above two errors were fixed the code works fine.
-
I've made some progress with the code, but I'm having trouble with two aspects. 1. The following code is an ajax callback function, which inserts the responseText into the markup and binds an anonymous function to onkeypress. When the user presses the up or down arrows the current li position should be stored in variable p and adjusted based on the number of times up or down is pressed. The idea was that every time a new suggestion list is created/displayed the value of p would be returned to null. However, in my code, the code always returns the value of variable p to be 1 if up is pressed and 0 if down is pressed. function suggestionCallback(ajaxResponse){ if (!ajaxResponse.match(/Error/i) && ajaxResponse != ""){ $("#suggestionBox").show(); var suggestionList = document.getElementById("suggestionList"); suggestionList.innerHTML = ajaxResponse; var inputBox = document.getElementById("msgRecipient"); var li = suggestionList.getElementsByTagName("li"); var p = null; inputBox.onkeypress = function(e){ // If [TAB] or [ENTER] keys are pressed if ( e.keyCode == 9 || e.keyCode == 13 ){ } else if ( e.keyCode == 38 ){ // If the up key is pressed, select the previouse user or the last user if at the beginning p = (p == null) ? li.length-1 : p-1; if (p < 0){ p = li.length-1; } alert("p: " + p); updatePos( li[p] ); } else if ( e.keyCode == 40 ){ // if the down key is pressed, select the next user or the first user if at the end p = (p == null) ? 0 : p+1; if (p > li.length-1){ p = 0; } alert("p: " + p); updatePos( li[p] ); } }; // end binding of function to input.onkeypress } else { alert(ajaxResponse); } } // end suggestionCallback 2. The following combination of functions is supposed to remove a class from an li position and add a class to another li position depending on the value of variable p. Seemingly the problem is that the value of p and its resulting li[p] value is not being recognized in the functions that are supposed to perform the addition/removal of classes. Below is the code: function addClass( target, theClass ){ target.setAttribute("class", theClass); } function removeClass( target, theClass ){ target.setAttribute("class", ""); } // Change the highlight of the user that's currently selected function updatePos( elem ) { // Update the position to the currently selected element var elementPos = elem; // Get all the user li elements var li = document.getElementById("suggestonBox").getElementsByTagName("li"); // Remove the 'cur' class from the currently selected one for ( var i = 0; i < li.length; i++ ){ removeClass( li[i], "suggestionLight" ); } // And add the highlight to the current user item addClass( elementPos, "suggestionLight" ); } Help appreciated.
-
Yes, you're right $(document).ready(function(){...}); is jQuery and I am using it; I should have mentioned that I was using jQuery in my original post. No, I am not doing this coding exercise to learn jQuery, but rather to increase my knowledge/understanding of javascript. As you pointed out, there probably are jQuery autocomplete scripts I could download; but that would defeat the main purpose of this exercise. The main purpose is to create a working autocomplete code using mostly traditional js (rather than a library, i.e. jQuery or prototype). I used jQuery's dom ready function, because in my code I create elements on the fly so I needed to make sure certain dom elements were present. For another coding exercise I might create a generic dom ready function; but for now using a bit of jQuery made sense. Furthermore, the code I attached has been combined from 3 separate files (1 html, 1 js, 1 css) into 1 file for convenience of downloading and viewing. Lastly, Dj Kat, do you mean by: that I should use <script></script> rather than <script type="text/javascript"></script> ? Help and insight appreciated.
-
I need help finishing my auto suggest code; I need help positioning the suggestion list directly under the "To" text field and creating the ability to use the up and down keys to move from the "To" text field to the suggestion list. At this point, the code takes what the user inputs in the "To" text field, posts those values and using php/mysql returns a (li) list of values that match the input. However, the suggestion list appears under the "To" text field label rather than the "To" text field box. I've attached the html, javascript, and css code I've put together. Thanks for the help. [attachment deleted by admin]
-
Yes, what I attached back on Dec. 20th 5:40pm is really my HTML. What do you mean by? There is a doctype (see attachment from Dec. 20th entitled "dividerline"): <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> I don't understand what you are asking by
-
When I set the width to 100% the line appears, but the original problem persists.
-
With the severe weather, this is the earliest I could post the code. Attached is the html and css code, which contains the issue. While putting together the example page, I noticed that in my original css code posting I missed an important attribute from my code: iframe{display:none;} When this style attribute is applied to the page that is when the discrepancy with how firefox and IE displays the horizontal line occurs. Note: I did try floating the divider line, but that made the divider line disappear. [attachment deleted by admin]
-
I've attached two images - one of the code in IE, the other in Firefox. Notice that in Firefox the dividerline is flush with the content above it. Note: Firefox Version: 3.0.5, IE 7.0.5730.13 [attachment deleted by admin]
-
I meant to post this question in the AJAX section - I had multiple tabs open and I posted here by accident. But since, I got a response I'd like to clear up a couple of things. Rather than showing/hiding an image in the markup I want to display the gif in a div and then replace the gif with what the AJAX function returns. I attempted this earlier via: document.getElementById("targetDiv").innerHTML = <img src="images/progress.gif"/> I placed the above code right before I sent the parameters, i.e. document.getElementById("targetDiv").innerHTML = <img src="images/progress.gif"/> XMLHttpRequestObject.send(parameters); And using a callback function, the contents of the "targetDiv" is replaced with the AJAX response. Problem with the above code is instead of showing the activity indicator gif, what seems to be an image placeholder is shown and then it is replaced by the AJAX response content. Everything besides the activity indicator gif shows/acts as intended. Is the placement of my .innerHTML piece of code be wrong? Am I approaching my goal the wrong way?
-
I would like to have an AJAX activity indicator (a gif image I made) to display in a specific div while the AJAX activities are being completed. What is the best way to accomplish this?
-
First of all, thanks for taking the time to go through my code and helping to resolve the issue. In copying/pasting my code I missed the ending curly brace in my posting, but in my code I do have it. So, the closing brace is not the issue. However, after reading your post, I thought I'd try another browser, so I opened my code in Internet Explorer, rather than firefox; and in IE the divider line works as intended. Do you know what could be causing firefox to display the <hr> tag flush to the bottom of the floated object rather than with a top AND bottom margin of 15px?
-
The html for the document is: <html> <head> </head> <body> <div id="contentContainer"> <ul class="tabNav"> <li class="current"><a href="#">Basic</a></li> <li><a href="#">Profile</a></li> <li><a href="#">Photos</a></li> <li><a href="#">Messages</a></li> </ul> <div class="tabContainer"> <div class="tab current"> <fieldset class="profilePicture"><legend>Current Profile Picture</legend> <div id="profileImg"></div> </fieldset> <br /> <form name="profileImgForm" id="profileImgForm" enctype="multipart/form-data" method="post" action="image_upload.php" target="imgUploadFrame"> <label class="label">Upload Picture:<br/> <input type="file" name="imgFile" id="imgFile" /><input type="submit" name="saveProfileImg" value="Save" /></label> </form><iframe name="imgUploadFrame" id="imgUploadFrame"></iframe><br /> <label class="label">Click below to remove picture <br/> <input style="vertical-align:bottom" type="button" name="removeProfilePic" id="removeProfilePic" value="Remove Profile Picture"/></label> <hr class="divider"/> <div id="editAddress"></div> </div> // end current tab div <div class="tab"> …Content for Tab 2… </div> <div class="tab"> …Content for Tab 3… </div> <div class="tab"> …Content for Tab 4… </div> </div> </div> <!-- end of #contentContainer --> </body> </html> The tab css is: ul.tabNav {float:left; list-style: none; width: 100%; border-bottom: 1px solid #FF6600; margin: 20px 0 0 0; padding:0;} ul.tabNav li {border: 1px solid #FF6600; float: left; padding: 0; margin: 0 5px; margin-bottom: -1px; } ul.tabNav a {color: #333; display: block; padding: 10px; text-decoration: none; outline: 0;} ul.tabNav li.current {border-bottom-color: #fff;} ul.tabNav li.current a {background-color: #FF6600; color: #FFFFFF; text-decoration: underline; font-weight: bold;} div.tabContainer { clear: both; float: left; width: 100%; margin-top: 20px; } div.tabContainer div.tab { color: #000; display: none; } div.tabContainer div.current { display: block; } div.tab p:last-child { margin-bottom: 0; } The css for the content inside the tab is: .profilePicture{ width: 150px; height: 165px; } .profilePicture legend{ color: #000066; font-weight: bold; } .label { font-weight: bold; color: #000066; } div fieldset { margin-left: 40px; margin-right: 5px; float: left; .divider{ margin: 15px 0; clear: left; }