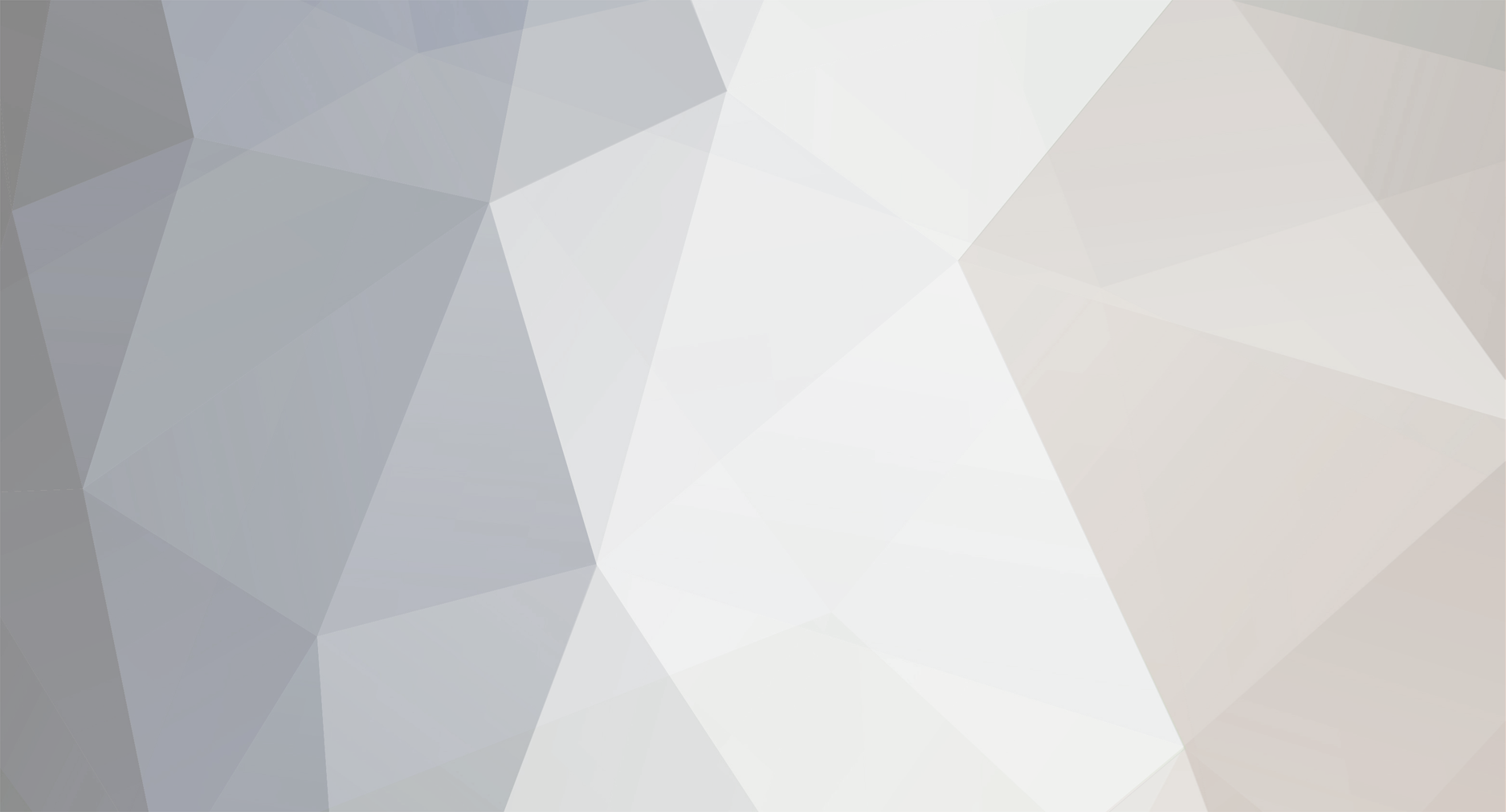
limitphp
-
Posts
706 -
Joined
-
Last visited
Posts posted by limitphp
-
-
I want to check to see if a songID exists in a playlist.
What would be the bare minimum way of doing it (least resources)?
this is what I am doing so far:
$querySong = "SELECT COUNT(id) FROM playlist_songs WHERE songID = '$songID' AND playlistID = '$playlistID'"; $resultSong = mysql_query($querySong) or die (mysql_error()); $flagSong = mysql_result($resultSong ,0); if ($flagSong==1) //song Exists
-
how about launching a new page, but making it nice and small and having that page play the song while they surf?
realistically, they'll keep that page minimized and out of the way.
-
There is no ONE best practice. Don't know exactly what you mean by some pages need the same variables. Are these "satic" in that they are the exact same values for each and every user (in that case use a config file that is included on all pages) or are these values static for each session/user (in that case use $SESSION).
It all depends on the implementation.
They are static for each session. Yeah, right now, I do use session variables.
-
I'm building a website that has many pages and is pretty complex.
Alot of pages use many variables.
Alot of pages use some of the same variables.
Alot of pages use the same include php pages that handle things like the menu.
Is there a best practice as far as structuring a php page?
Like having all the variables the php page will use listed somewhere, or having it organized a certain way?
Thanks
-
if that is what you want to do, go for it.
i'm still gonna push jQuery on you more though. i HIGHLY recommend trying it out when you get a chance. once you use it you will never go back
Ok. I tried looking at it.
So, I assume its a bunch of functions that ar in a js file...and anytime yuo want to use them you load that js file in your page?
And I assume the way they do things is alot easier than doing it by hand?
But I would still have to relearn everything. I spent all this time learning javascript....now I would have to spend all this time relearning some functions that people wrote for javascript.
It seemed pretty darn complicated for me when i looked at it.
-
if you don't use a framework, you have to use regex on the response body to pull out any bits that are between <script> tags, then run those fragments through JavaScript's eval() function.
did you even try the code i posted that uses jQuery? it works as is
I'm sorry, I didn't realize you had the source for the jQuery sitting on a website ready to go.
Looking at that code, though, i need to explain something:
on my ajax page.....in divs or forms like on a submit button
<input type=submit >
putting in onclick in their works, or putting javascript in any of the objects in divs or whatever as onclick=, or onMouseover=
those all work.
Just the javascript in the <script></script> tags doesn't work.
Now that i understand why it doesn't work, you have to get it manuualy and eval it (thanks for all the info and explanation)....I'll just put all my functions I need to call in a global js file.
thanks for all the info.
-
jQuery is easier than any non-framework AJAX code.
Anyway, you code PHP and you somehow don't know what parse means? Huh?
Anyway, parsing, in regards to scripting languages like JavaScript (or PHP) is when the parser takes the code and turns it in to something useful. It's essentially knowing what instructions to do based on certain 'tokens.' For example, the PHP engine converts an echo construct into something useful. Or, it may parse an fopen command into the system function call of fopen.
Trust me; using something predone to parse JS in AJAX will be much easier than trying to extract JS content from between <script> tags and eval() it.
A lot of times I see people use JS in AJAX returns when they don't really need to. Are you sure you need to?
I'm not sure yet...but there have been times when it seemed conveinet to just write the functions I needed on the ajax php page then on some global js page.
-
Because the AJAX object doesn't care what is in the content. It's only job is to load the content, not parse it.
You must tell the code to parse the other code (if that strange wording made sense).
What do you mean by parse the code?
I've tried looking at jQuery....it seems too complicated for me...
its was much easier for me to use the example from this forum.
-
What do you use to do the AJAX?
When a browser loads a page, it will look through the page for javascript and execute it. You loose this functionality with AJAX. Check out a JS Library like jQuery, and use it's AJAX functions. Their functions will parse the ajax response for JavaScript and eval() it so it gets run
I'm pretty much using the example on phpfreak forums:
http://www.phpfreaks.com/forums/index.php/topic,115581.0.html
Why does the page that gets loaded via ajax lose its javascript functionality?
-
you need to do it a little differently:
<script type="text/javascript"> foobar = function ( var1 ) { alert(var1); } foobar('test'); </script>
edit: this also assumes your AJAX code parses and eval()s JavaScript for you. if you are using a JS library for your AJAX (like jQuery) you should be all set
I'm confused.
I have a page with a div on it
<div id='playlist_box'></div>
I use ajax to call a php page (ajax.php) to fill that div.
On the ajax page, if I put something as simple as :
<script>
alert('test');
</script>
nothing will happen. Its as if the ajax page ignores any javascript on it.
-
If I use ajax to load a box or etc. And that box is just a small php page, like playlist_ajx_songs.php
On that playlist_ajx_songs.php if I have
<script>
</script>
with a javascript function in their and I try to use that function, it doesn't work.
Why is that?
It seems, you can only call functions that were already loaded before the ajax page was inserted.
-
sorry....i put width='50%'
instead of width:50%;
-
I'm trying to set a left column, a center column (for all content) and a right column.
I'm setting the width in the divs by percent.....I tried px just in case...neight is working. Each div seems to remain 100% width and they are stacked on top of each other.
here's the code:
<div style='border:solid 1px; width='30%' margin-top:25px; float:left'> <font class='h1'>My Account ($username)</font> <p style='line-height:5px'> </p> <a href='javascript: onclick=changeArrow()' class='link5'><b>My Playlists ($playlist_count) <img id='arrow' border='0' src='img/down_arrow.bmp'/></b></a> <p style='line-height:5px'> </p> <div id='list_playlists'></div> </div> <div style='border:solid 1px; width='50%' margin-top:25px; float:left'> <div id='list_songs'></div> </div> <div style='border:solid 1px; width='20%' float:left'align='right'> <p style='line-height:10px'> </p> <font class='t1'><b>$fname $lname</b> <br> $email <br> Joined ".date('F, Y', strtotime($join_date))." </font> </div>
-
im trying to convert to full div layouts now. Used to be a hardcore table user but the code sometimes got to the point of insane and I couldn't figure it out sometimes.
I do admit that pages load quite a bit faster using div but i got a lot of learning to do. One thing I realized that IE and firefox don't handle div right for me. using a width of 100% shows properly in IE but in FF, its expanding off the screen by about 4px.
Feel the same as you right now trying to figure out the bugs. Tables were easier
Not sure but im right now about 60% div, 40% tables
ahh...the reason why div width is not same in IE and Firefox....is because of margin defaults in the body tag in firefox maybe....
set this in css:
body {margin:0px; overflow-y:scroll}
the y scroll will always make the browser set room for the vertical scroll...this way your width always stays the same....
actually...i just figured something out....the reason my div was shooting up next to the other 2 divs I had...was because I needed to set the "clear:both" in the div I wanted to goto the next line...
so right now....I'm back to:
all divs for layout...no tables for layout....
tables for tabular data of course, though....
-
Is using tables for layout outdated?
Want two columns?
Yes, you can do a div float left and a div float right.
But, below those two columns, if you want to go back to 3 columns or 1 column, any div you put underneath it will go up to the top and will not by default stay underneath the two floating divs. That is a huge flaw.
With tables you don't have to worry about this.
Maybe I'm missing something, but for now, I'm sticking with tables.
-
There is a problem with divs....
it seems in IE, you cannot float divs within a div that is floated.
In other words....
two columns (div1 float left, div2 float right)
in div2 if you put floated divs....No NO.....trouble....will not work in IE
tables do not have this problem....
for now....if I need floating divs inside of floating divs, I'll use a table instead.
-
For instance, if you just use "regular" HTML, will people still be able to see and use my website normally, even if they are not using Internet Explorer? For example, I always use Firefox. Other people use things like AOL or Opera to browse the web. Thanks for your help!
personally, as long as my site works in firefox and ie, I'm happy.
I don't do anything out of the ordinary.
Although, I'm still very wet behind the ears compared to most people here.
Oh, also, I use this in my css:
body {margin:0px; overflow-y:scroll}
form {margin:0px;padding:0px}
p {margin:0px;padding:0px}
the body one is very important. that way when you say 100% width in a div or table....it gives you true 100% width in firefox and ie.
and then use divs (as I just learned) to layout the infrastructure of your site.
-
thanks for the info, by the way!
you have freed me from my table prison!
: )
-
I have a div, and I set the style='width to 100%'
I have left and right padding. The div is taking up more than the page. There's a horizontal scroll bar. Is there a reason the div style='100%' width is bigger than the page?
nevermind....it was the padding that made it larger than the page.
I thought padding takes place inside the div, not outside. oh well, now I know...no big deal.
-
Well, there are a few different ways. One of the easiest is to do something like this:
HTML:
<div id="left"></div> <div id="right"></div>
CSS:
#left { width: 40%; float: left; } #right { width: 60%; float: left; }
So, is that the best way, using divs to layout the infrastructure?
-
You can set the display of the div to 'inline', and it will act like that. But it doesn't usually make sense to do that if you are using semantic markup.
On top of that, using tables for page layout is outdated. They are only supposed to be used for tabular data - i.e. data that is cross referencing two category types.
That site I linked you to - HTML dog - has a really good reputation for their tutorials. I never actually used them myself (I learned CSS a different way), but if you want to make pages the way that they are being made these days, then I would really suggest spending some time doing the tutorials on that site.
Good luck!
tables for layout is outdated?
Lets say I have a page where I want two columns (column 1 I want to be 60% width of page, column 2 I want to be 40% width), how do I do this without tables?
-
A div by default is as wide as it can be. So if the item its contained in has padding, then the div will be as the inside of the containing element, minus the paddings that that element has. If the div itself has a margin, then the width of the margins will also be subtracted from the width of the div.
You should probably read more about the box model to understand how this all works. It's essential if you want to learn CSS and use it well.
Browsers have default settings for the padding and width on each element (sometimes the default is zero). To remove default paddings and margins, you will have to explicitly set them to zero.
Well, I read thought he link. thanks.
Problem is, I tried setting the margins and padding of both the td and the div inside the td all to 0.
The div still stays as wide as the td (50%).
Why do divs stay as wide as the objects they are in and tables do not?
If I have "asdasdasd" inside a table with no width specified....the table is only as wide as that text.
if I have "asdasdasd" inside a div with no width specified...the div is as wide as the object it is inside....regardless of padding and margins.
Is there anyway to get a div to act like a table in that respect, besides using float?
-
so if a div is in a td where the width is 50% of the page, the default width of the div will be 50%?
That is the behavior in firefox. Is there a default value that makes this happen?
margin or something? Something I can set to 0 in the css style sheet?
thanks
-
try
SELECT a.playlistID, COUNT( b.id ) FROM playlist_songs as a LEFT JOIN playlist_songs as b ON a.playlistID=b.playlistID WHERE a.songID = '1' GROUP BY a.playlistID
not tested
It worked!
thank you.....
Thats wierd, left joining the same table with itself. I'm learning more everyday.
thanks again.
[SOLVED] Help With Using Include PHP File
in PHP Coding Help
Posted
I have alot of pages that will display songs. I use different styles of displaying the songs. Users can choose to use either style. There will be a checkbox that will allow them to change the style via ajax.
In order to organize my code a little bit better, I would like to put each style of displaying the songs in their own include files.
So, I'll have an include file called: inc_table_view.php and one called inc_vote_view.php
In the pages that will use these include files, there will be a variable called $viewID that will control which one to use.
In the php code on the pages that will use these includ files, could I do something like:
Does that seem like a logical way to handle this?
Thanks for any advice...