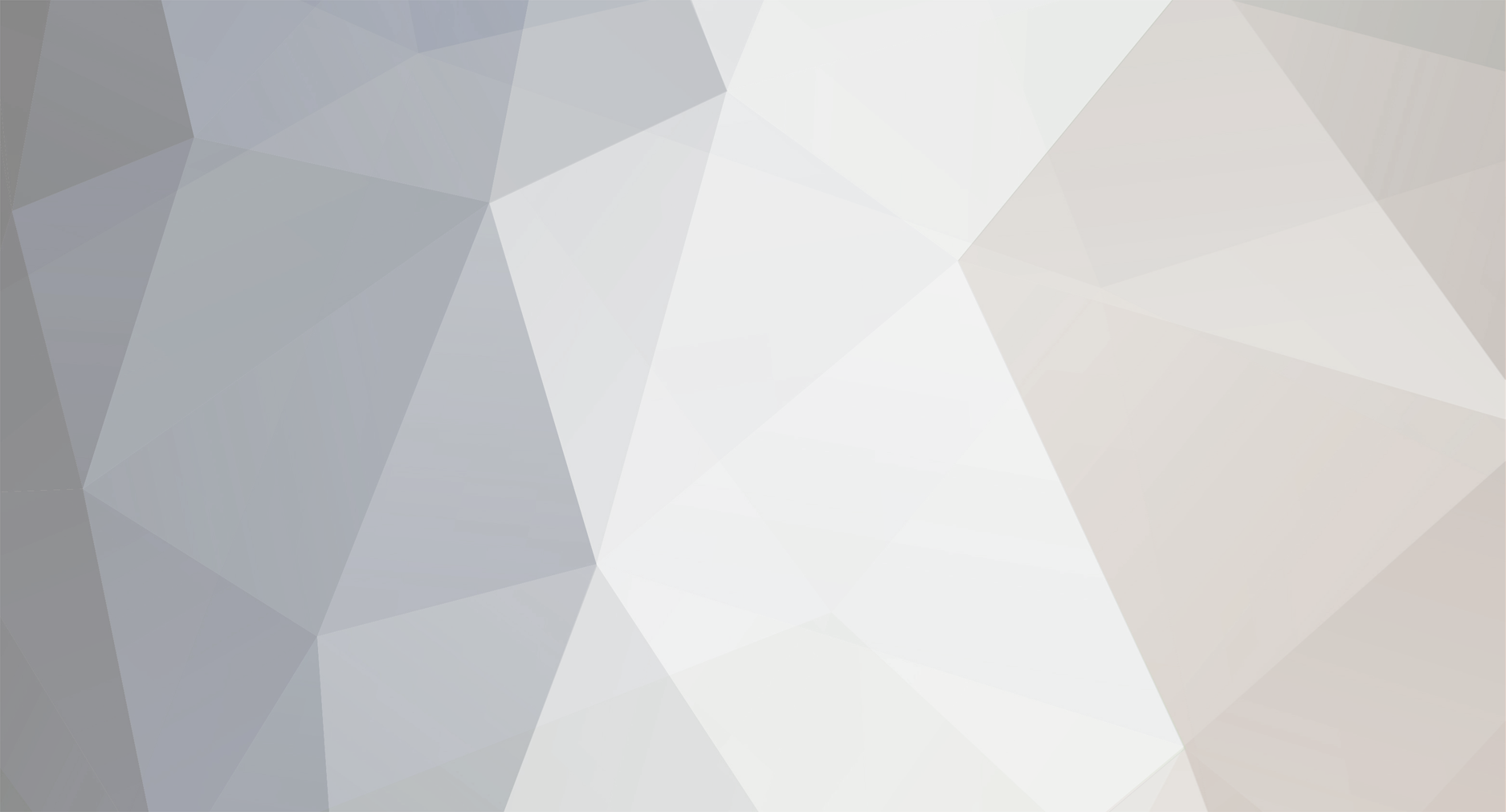
toplay
-
Posts
973 -
Joined
-
Last visited
Never
Posts posted by toplay
-
-
well, first, a '-' is also a special character, so you would need to escape it with a '\'
but '-' and '+' are included with '.', so that would be redundant. you should never need a . inside []
A dash ('-') doesn't need to be escaped ('\') inside a character class when it's at the beginning or end.
Also, discomatt is correct.
It's well worth buying this regex software:
and this book - Mastering regular expressions:
http://www.oreilly.com/catalog/regex3/
-
Issues:
1) You should not use just "date" for a column name because it's not meaningful enough and more importantly it's a MySQL reserved word. Change it to something else..i.e. date_created
2) When holding date data, use MySQL date, datetime, or timestamp data types and not a VARCHAR. This will then allow you to use many available date and time functions on that column.
3) You need to validate all input (i.e. from $_POST['Date']) to make sure it's in YYYY-MM-DD format (for a date data type). If you simply want to insert todays date, just use curdate() (without quotes) as the column value instead.
4) You should verify every query first before proceeding to execute subsequent commands. Your create probably didn't work (because you're using 'date' as the column name). If you insist on using 'date' as the name of your column, then you must enclose it in backtick marks (i.e. `date`).
Good luck.
-
From the code posted, I don't see where you set $game !!
-
Same as the above (I copied it) but a little tidier:
if (trim($_POST['subject']) == '') && (trim($_POST['post']) == '')) { $subject = mysql_real_escape_string(strip_tags($_POST['subject'])); $comment = mysql_real_escape_string($_POST['post']); } else { echo 'Empty field found'; }
You have your "if" condition wrong. That will execute the mysql_real_escape_string() on empty fields. I think you meant to use "!=" instead of "==".
-
Does $_SESSION['user'] hold a name or an email?
In your insert change these things:
Change:
'$_SESSION[user]',
To this:
'{$_SESSION['user']}',
Change:
'&_POST[price]'
to:
'$_POST[price]'
-
-
"SET" is only specified once. Check the mysql manual for proper syntax. Try this (untested):
$query = "UPDATE `user_settings SET `name` = '{$new_name}', `contact` = '{$new_contact}', `age_race` = '{$new_age_race}', `non_member` = '{$new_non_member}' WHERE id = '{$id}'";
-
You're missing a right curly brace to close off this line:
if (isset($_POST['submit'])) {
-
Make life easier on yourself and use a template engine. It will help you separate code/business logic from visual styling.
I highly recommend Smarty http://www.smarty.net
-
Use:
echo urlencode($categoryname);
Then in your indextest.php retrieve the value by using $_GET as in $_GET['category'].
-
Sometimes the error isn't on the line designated but earlier in the code...always look a line or more above too.
Change this:
$sql = auth->query("SELECT `id` FROM `tutorials` ORDER BY `id` DESC LIMIT 1"));
To this - you have an extra right parenthesis at the end:
$sql = auth->query("SELECT `id` FROM `tutorials` ORDER BY `id` DESC LIMIT 1");
-
You've answered your own question, or rather pointed out the problem by stating "behind a firewall and basically inaccessible". So, how do you expect MySQL to magically do anything?
-
1) I don't see where you're passing $_POST[sel_review] value to the view_review.php script. You need to pass it perhaps in a session or in the URL and view_review.php will use $_GET to retrieve the value (and not $_POST).
2) If you're only going to be reading one row, then don't use a while loop in view_review.php - just do a fetch once. If your query is meant to retrieve more than one row, then you need to put the echo of the book info inside the while loop.
-
Try something like this (untested):
SELECT
DISTINCT t1.id
, t2.`time`
FROM
table1 t1
JOIN
table2 t2
USING
(id)
ORDER BY
t2.`time`
;
or try:
SELECT
t1.id
, t2.`time`
FROM
table1 t1
JOIN
table2 t2
USING
(id)
GROUP BY
t1.id
ORDER BY
t2.`time`
;
-
See substr() function examples in the manual at http://www.php.net
-
This way returns 6 rows containing a count and an identifier (column_name) to tell for which column the count is for:
SELECT 's1t1' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s1t1) > 0
UNION
SELECT 's1t2' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s1t2) > 0
UNION
SELECT 's1t3' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s1t3) > 0
UNION
SELECT 's2t1' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s2t1) > 0
UNION
SELECT 's2t2' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s2t2) > 0
UNION
SELECT 's2t3' AS column_name, count(*) AS total_row_count FROM itmc_anmeldungen WHERE LENGTH(s2t3) > 0
If you want just one row back that has the total for each column, then you would do something like this:
SELECT
SUM(IF(LENGTH(s1t1) > 0, 1, 0)) AS total_s1t1_count
, SUM(IF(LENGTH(s1t2) > 0, 1, 0)) AS total_s1t2_count
, SUM(IF(LENGTH(s1t3) > 0, 1, 0)) AS total_s1t3_count
, SUM(IF(LENGTH(s2t1) > 0, 1, 0)) AS total_s2t1_count
, SUM(IF(LENGTH(s2t2) > 0, 1, 0)) AS total_s2t2_count
, SUM(IF(LENGTH(s2t3) > 0, 1, 0)) AS total_s2t3_count
FROM itmc_anmeldungen
# optional: you could specify a where clause here
;
-
Doing:
SELECT * FROM itmc_anmeldungen WHERE s1t1 > "" or ... WHERE LENGTH(s1t1) > 0
and using mysql_num_rows() will return the actual rows and how many there are.
This:
SELECT count(*) AS total_non_empty_s1t1 itmc_anmeldungen WHERE s1t1 > "" or ... WHERE LENGTH(s1t1) > 0
will just return one row containing a value which would correspond to the number of rows that have something in s1t1 column.
I think that's what you're talking about. You didn't mention what type of column s1t1 (char, numeric, decimal, etc.).
See manual for string functions:
http://dev.mysql.com/doc/refman/5.0/en/string-functions.html
Please don't specify any type of urgency in your posts because it's against forum rules. This is a free forum and there is no guarantee that anyone will answer let along in the time frame you might be looking for. If you need urgent help, consider posting in the freelancing section for paid help. Thanks.
-
get rid of or die() part
-
Show code.
-
Yes, that's where the add one would go, however, I'm not sure what your $userchoice holds as a value.
Based on your update shown, it would be like this:
UPDATE poll SET `votechoice1` = `votechoice1` + 1 WHERE ...
But I have a feeling that's not what you want or talking about.
-
You've got two mysql_fetch_array(), so get rid of the first one right underneath the query.
FYI: You can use a while loop instead of a for loop.
-
If you want to add one to an existing column in a table, you simply do:
UPDATE table_name SET column_name = column_name + 1
WHERE ...
-
But REPLACE changes the UID, and issues an implicit DELETE... not good.
Yes is does a delete and insert. If you're using an auto_increment column as the primary key it will give a new unique value if the existing key value isn't specified, otherwise it will be similar to an update. I didn't want to assume it was an auto_increment column.
dannyd, MySQL requires a primary key. It doesn't have to be an auto_increment, and a date/time could work provided that it will be unique. However, a date/time column is not usually used as a primary key (maybe as a secondary index with duplicates allowed).
You should think about what field would be unique for the info you're trying to save, and use that as the primary key.
-
You're not showing us your complete code or what your html_entities() function is really doing. So, your html_entities() function must be returning an array. It must be doing htmlentities() on each value of the array, I guess. If that's all your function is doing, then you don't need a function and can just use array_walk().
You can always save as-is and do the htmlentities() after retrieving the data (just before outputing/displaying).
FYI: I would reorganize the database in the first place so I wouldn't have to save serialized info in the table.
radio button validation
in Javascript Help
Posted
I deleted his other topic and unlocked this one...moved it to JS help.