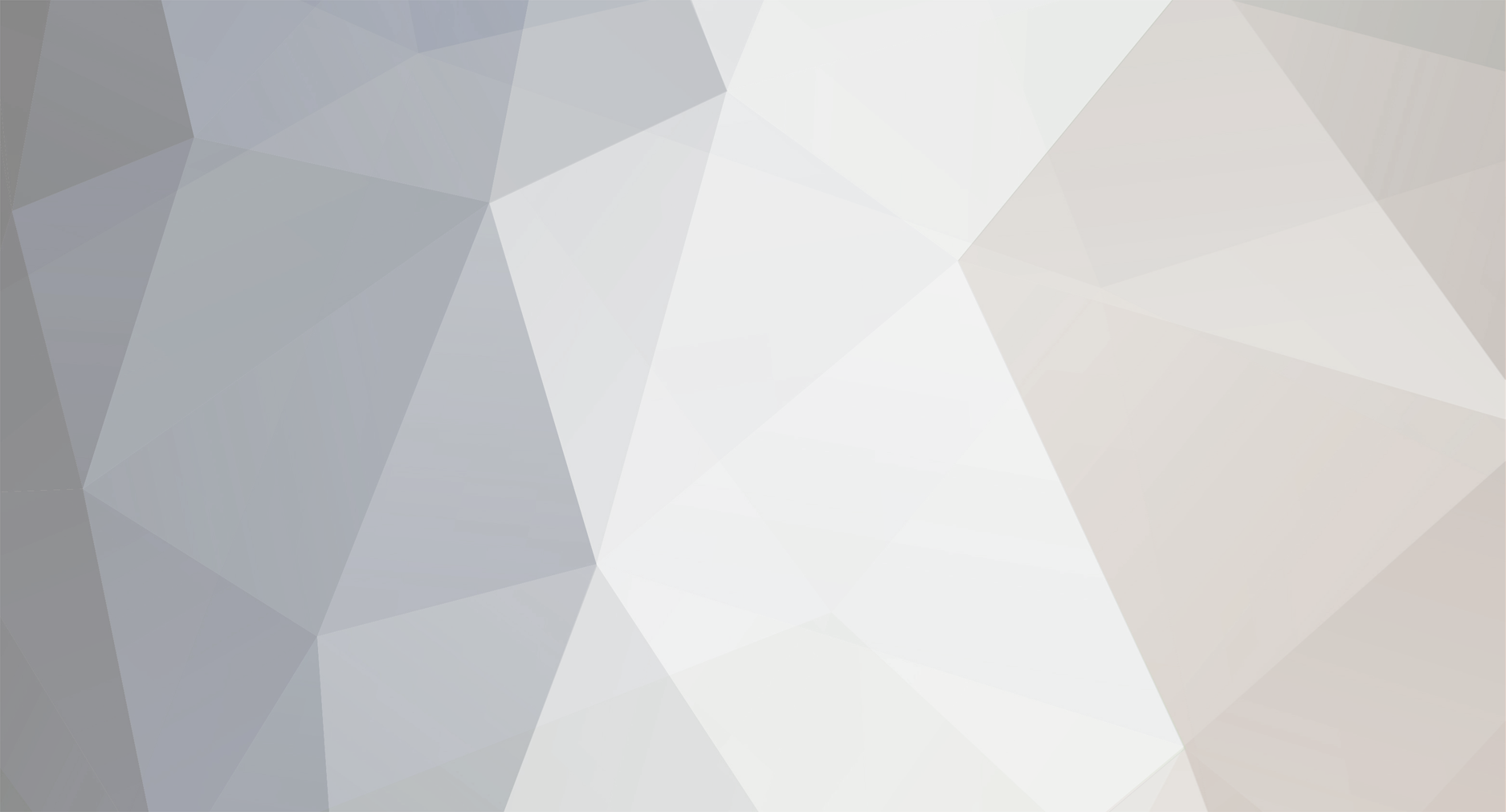
keeps21
Members-
Posts
91 -
Joined
-
Last visited
Everything posted by keeps21
-
[SOLVED] My first OOP script, not outputting value
keeps21 replied to keeps21's topic in PHP Coding Help
Thanks for the replies. I've now amended my code to the following which works as I expect it to. <?php /** * Filename: class.comment.php * Date: 22 October 2008 **/ // Include config.php - allows access to database. include('config.php'); class Comment { private $in_CommentId; private $in_Name; private $in_Email; private $in_Comment; public function __construct() { $this->in_Name = $name; $this->in_Email = $email; $this->in_Comment = $comment; } public function addComment() { $query = "INSERT INTO comments (name, email, comment) VALUES ( '{$this->in_Name}', '{$this->in_Email}', '{$this->in_Comment}')"; $result = mysql_query($query) or die ("MySQL Error"); if ($result) { echo 'Success!'; } else { echo 'Failed to add comment!'; } } public function getComments() { $query = "SELECT name, email, comment FROM comments"; $result = mysql_query($query); $this->in_Comments = array(); // Initialise array while ($row = mysql_fetch_assoc($result)) // Loop through query results and add rows into array { $this->in_Comments[] = $row['name'] . ' - ' . $row['email'] . ' - ' . $row['comment']; } return $this->in_Comments; } } // Call get comments function and output result. $in_comment = new Comment; $in_comment->getComments(); $comment_array = $in_comment->getComments(in_Comments); foreach ($comment_array as $row ) // Output each row { echo $row . '<br />'; } ?> The only other question I have about it is this: At the minute I'm concatenating the rows returned by the query $row['name'] . $row['email'] . $row['comment'] How would I got about creating an array where the first row would contain an individual value for name, one for email and one for comment, I guess what I want to do is create a multi dimensional array. -
Hi, I'm trying to get my head around OOP and have created the following script. All i want it to do at the minute is for the getComments method to return a value, which can then be echoed onto the screen. I can't figure out why it's not working, I'm sure I'm missing something really simple. Thanks for any help. <?php /** * Filename: class.comment.php * Date: 22 October 2008 **/ // Include config.php - allows access to database. include('config.php'); class Comment { private $in_CommentId; private $in_Name; private $in_Email; private $in_Comment; public $in_Comments; public function __construct() { $this->in_Name = $name; $this->in_Email = $email; $this->in_Comment = $comment; } public function addComment() { $query = "INSERT INTO comments (name, email, comment) VALUES ( '{$this->in_Name}', '{$this->in_Email}', '{$this->in_Comment}')"; $result = mysql_query($query) or die ("MySQL Error"); if ($result) { echo 'Success!'; } else { echo 'Failed to add comment!'; } } public function getComments() { $this->in_Comments = "Why isn't this displaying?"; return $this->in_Comments; } } // call get comments function and output result. $in_comment = new Comment(); $in_comment->getComments(); $value = $in_comment->getComments->$this->in_Comments; echo 'You said ' . $value; ?>
-
For anyone who's interested, the article that I got the code from is here http://www.talkphp.com/advanced-php-programming/1304-how-use-singleton-design-pattern.html
-
Just a quick query for you: If you have the following code, which is a singleton to create a database connection. Where does/could this code get the database connection details from? <?php class Database { // Store the single instance of Database private static $m_pInstance; private function __construct() { ... } public static function getInstance() { if (!self::$m_pInstance) { self::$m_pInstance = new Database(); } return self::$m_pInstance; } // Prevent singleton from being cloned private function __clone() { } } ?> Cheers
-
I found this tutorial quite useful. http://www.killerphp.com/tutorials/object-oriented-php/php-objects-page-1.php
-
I'd imagine you need to change the maximum upload size in the php.ini and it should be straight forward from there?
-
The $_GET needs to appear on the page which needs the information from the url.
-
Say you have a page with a list of users and by clicking on their name you can view their profile. This is how you could do it with $_GET users.php - lists users <html><head><title>Profile list</title></head> <body> <a href="view_profile.php?profile_id=1" > Profile of user 1</a> <a href="view_profile.php?profile_id=2" > Profile of user 2</a> <a href="view_profile.php?profile_id=3" > Profile of user 3</a> </body> </html> Notice the links, they have ?profile_id=1 and so on. When you click on that link you will be taken to a page called view_profile.php with the profile_id variable appended onto the end of the url. In this next page, to show the profile you need to 'get' that variable from the url. view_profile.php - view the profile of selected user. <?php // Get profile ID to show $profile_id = $_GET['profile_id']; // No error checking is done here so do not use this code as is. // you'll need to change the values here to suit your db $query = "SELECT profile_id, name, email FROM users WHERE profile_id = $profile_id"; $result = mysql_query($query) or die ("There was an error"); $row = mysql_fetch_row($result); ?> <html><head><title>Profile of User <?php echo $profile_id; ?></title></head> <body> <h1>Profile - <?php echo $row['id'] . ' - ' . $row['name'] ;?> </h1> Email - <?php echo $row['email'] ;?> </body> </html> You need to change the $row[''] to suit the db field value you want to show. $row['name'] shows data from the name column in your db $row['email'] shows data from the email column in your db I hope this clears things up a little for you.
-
Solved: I replaced return with echo in each of the methods.
-
note: when I say function I actually mean method
-
This is a script about books. details are title, author first name and last name, and price. I've created a class 'book', and functions to get the title, names and price which all work individually. However I've created a function to call the above 3 functions, but am getting an error: call to undefined function and can't figure out exactly why. any help would be appreciated. <?php class book { public $_Title; public $_FirstName; public $_LastName; public $_Price; public function __construct($title, $firstname, $lastname, $price) { $this->_Title = $title; $this->_FirstName = $firstname; $this->_LastName = $lastname; $this->_Price = $price; } public function getTitle() { return $this->_Title; } public function getAuthor() { return $this->_FirstName . ' ' . $this->_LastName; } public function getPrice() { return '£'.$this->_Price; } public function showBookDetails() { getTitle(); getAuthor(); getPrice(); } } $item1 = new book( "OOP and PHP", "Andrew", "Bell", 9.99 ); print $item1->getTitle(); print $item1->getAuthor(); print $item1->getPrice(); print $item1->showBookDetails(); $item2 = new book( "PHP Cookbook", "Joe", "Bloggs", 29.99 ); print $item2->getTitle(); print $item2->getAuthor(); print $item2->getPrice(); print $item2->showBookDetails(); ?>
-
You've lost me with this? I'm running 5.2.5. Is there an example you could give me for this to explain what you mean? Thanks
-
Hi I'm trying to get my head around OOP and MVC. I'm wondering whether I have it right with the following code - also attached as a zip file. model.php <?php class Student { /* These are some of the properties */ var $Name; var $Gender; var $GradeLevel; var $Schedule; /* Constructor. Called as soon as we create a student */ function Student($studentName, $studentGender) { $this->Name = $studentName; $this->Gender = $studentGender; } function goToClass() { } function rideTheBus($busNumber) { $statement = $this->Name . " rides bus number " . $busNumber; return $statement; } function skipSchool() { } function changeGender() { if ($this->Gender == "Female") { $this->Gender = "Male"; } else { $this->Gender = "Female"; } } } ?> view.php <?php include "model.php"; include "control.php"; print $a_student->Gender; print "<br>"; print $b_student->Gender; print "<br>"; print $c_student->Gender . " " . $c_student->Name; print $a_student->rideTheBus("65"); print $c_student->Gender; // this will print "Male" now... ?> control.php <?php $a_student = new Student("John","Male"); $b_student = new Student("Mary","Female"); $c_student = new Student("Larry","Female"); $c_student->changeGender(); ?> Any advice would be greatly appreciated. Thanks. [attachment deleted by admin]
-
Hi Is there an easier way to write this pseudo code script so that I don’t have to write the form out twice? At the moment I have separated the form html into a separate script and I'm just doing an include to call it in. if { // form has been submitted // process form if { //error when processing form // output error // show form (include form.html) } } else { // form hasn’t been submitted //show form (include form.html) } Thanks for any advice. Stephen