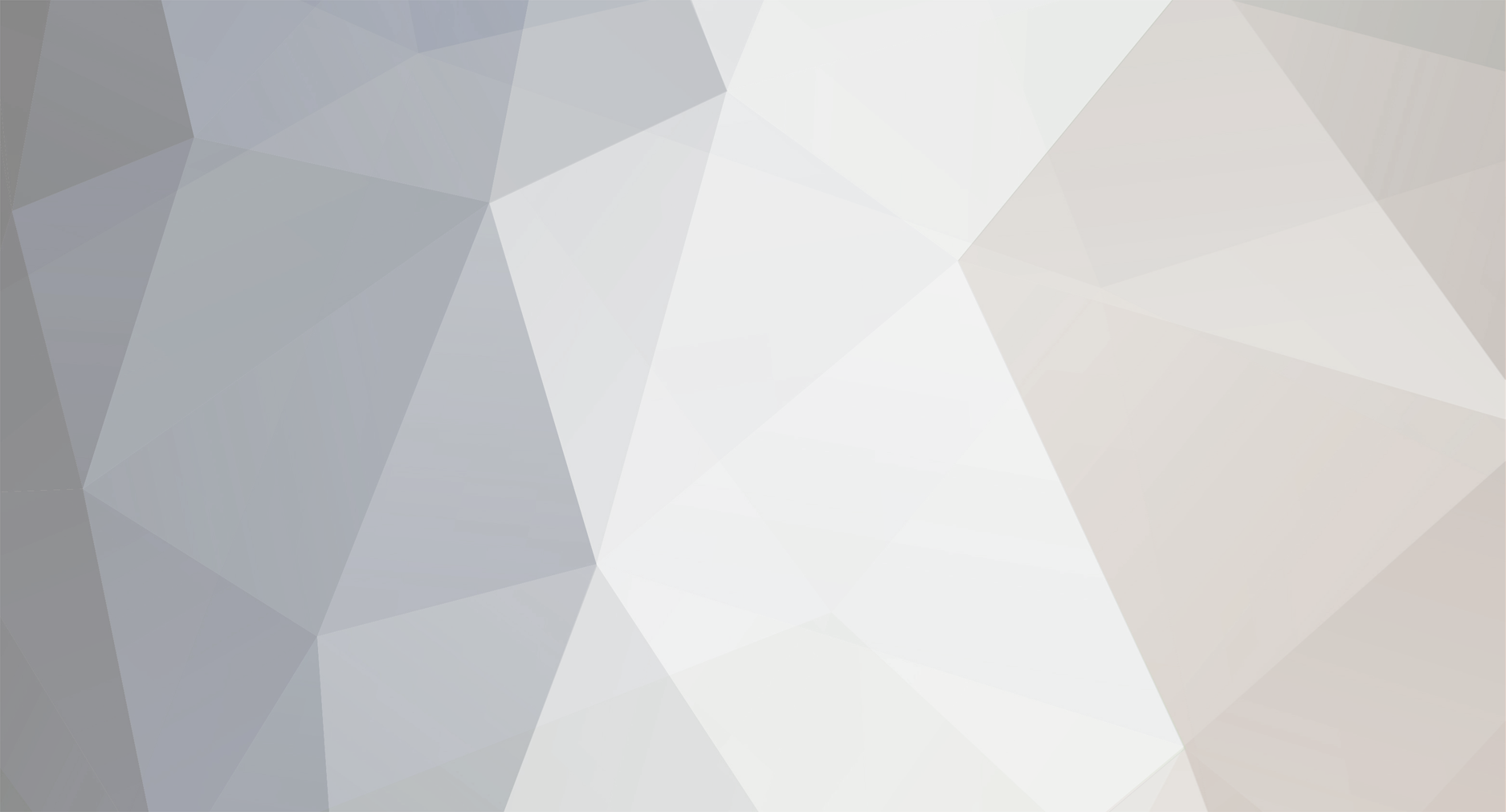
n3ightjay
-
Posts
64 -
Joined
-
Last visited
Never
Posts posted by n3ightjay
-
-
okay what are the data types of
cart_table( product_id
image
product_name
quantity
price
shipping_cost
total_cost
-
you have to remember to enQuote your string/charactor values with quotes and leave you numeric values open
<?php $qry="insert into cart_table(product_id,image,product_name,quantity,price,shipping_cost,total_cost) values(".$id.",'".$row['image']."','".$row['product_name']."',1,".$row['price'].",".$row['shipping_cost'].",".$row['price']."+".$row['shipping_cost'].")"; ?>
i think thats it im not sure of your db structure though also if your hardcoding your quantity value don't quote it out
-
sorry missed class= ....
<?=($tabParam == "home"?" class=selected":"");?>
ternary == if block inline (kinda)
if($tabParam == "home"){ echo "class=selected"; }else{ echo ""; }
-
isset() is not the same as !empty(). Since he has $cat = $_POST['category';, it'll be set no matter what. Example:
<?php $foo = ''; var_dump(isset($foo));
Outputs:
bool(true)
Good point got excited in trying to find multiple ways of answer the same question... assumed checking against zero was checking if it was empty ... therefore leading me down the path to isset()
-
try ...
row13
values(".$id.",".$row['image']."," .......
-
look up
-
I would use ternary "ifs"
<div id="navigation"> <div id="maintabs"> <a href="#"<?=($tabParam == "home"?" selected":"");?>><span>Home</span></a> <a href="#"<?=($tabParam == "reports"?" selected":"");?>><span>Reports</span></a> <a href="#"<?=($tabParam == "administration"?" selected":"");?>><span>Administration</span></a> <a href="#"<?=($tabParam == "logOut"?" selected":"");?>><span>Log Out</span></a> </div> <div id="subnav"> <a href="#"<?=($tabSubNav == "subNav1"?" selected":"");?>>Sub Nav Item #1 For Home</a> <a href="#"<?=($tabSubNav == "subNav2"?" selected":"");?>>Sub Nav Item #2 For Home</a> <a href="#"<?=($tabSubNav == "subNav3"?" selected":"");?>>Sub Nav Item #3 For Home</a> <a href="#"<?=($tabSubNav == "subNav4"?" selected":"");?>>Sub Nav Item #4 For Home</a> </div> </div>
-
damn ... im sure theres a million more ....
technically this qualifies as well
if(isset($cat)){
-
for loop would be the easiest then you already have a counter going but you could create a counter for a while loop.. sorry you'll have to figure out which of the following work better for you im to lazy to look through your code to figure out where / what your talking about
$counter = 0; while(true){ if($counter % 2 == 0){ $class = "class1"; }else{ $class = "class2"; } $counter++; }
or
for($i = 0;$i <= $whatever;$i++){ if($counter % 2 == 0){ $class = "class1"; }else{ $class = "class2"; } }
-
oh oh oh ... or
if(strlen($cat) != 0){
-
You 3rd if is comparing incomparable types (I don't know if thats the actuall definition of the solution but this works i tested it) ??? if you change it to
if($cat != ""){
or
if($cat != "0"){
it will work
-
Im not really sure i understand your question, but i think using mktime() in conjunction with your date function might lead you in the right direction:
-
my bad how embarassing .. thanks for the correction
-
<?php //weight in grams (A) $weightA = '600'; $priceA = '299.99'; //weight in kilograms (kg) (B) $weightB = '45.2'; $priceB = '699.21'; // cost for a $costPerKGA = $priceA / ($weightA * 1000); // cost for b $costPerKGB = $priceB / $weightB;
-
I know you clicked solved but i just had to finish this ... this will work for any matix setup in the layout order you wanted:
<table> <?php $result = array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25); $numRows = 5; $numCols = 3; for($i = 0; $i < $numRows; $i++){ echo "<tr>"; for($j = 0;$j < $numCols; $j++){ echo "<td>" . $result[($j*$numRows)+$i] . "</td>"; } echo "</tr>"; } ?> </table>
-
I created some logic while you posted your solution:
your current display logic:
<table> <? $result = array(1,2,3,4,5,6,7,8,9); for($i = 0; $i < 3; $i++){ echo "<tr>"; for($j = 0;$j < 3; $j++){ echo "<td>" . $result[($i*3)+$j] . "</td>"; } echo "</tr>"; } ?> </table>
logic to switch:
<table> <? $result = array(1,2,3,4,5,6,7,8,9); for($i = 0; $i < 3; $i++){ echo "<tr>"; for($j = 0;$j < 3; $j++){ echo "<td>" . $result[($j*3)+$i] . "</td>"; } echo "</tr>"; } ?> </table>
this is assuming you always have a 3x3 grid though i hope this helps
-
echo "<td bgcolor=".$rowcolor." style=\"padding-left:5px\" class='normal'><a href=javascript:createplayer(/'".$rfiles['vidlink']."') >".$rfiles['vidname']."</a></td></tr>";
looks like you have you apostophies messed up in "...createplayer(/'".$ ..... " switch it to "...createplayer('/".$ ...."
ie:
echo "<td bgcolor=".$rowcolor." style=\"padding-left:5px\" class='normal'><a href=javascript:createplayer('/".$rfiles['vidlink']."') >".$rfiles['vidname']."</a></td></tr>";
I hope this is fixes it .. a good check when something like this happens is to view the source to make sure the echo'd php is matching up you JS / HTML code
-
-
your welcome ... been there many times
-
2 trains of thought for ya... First is start with MySQL joins ... a quick google search says that 'left join' can combine multiple tables .. but i can't think of how to maintain the id.
the second would be 4 seperate querys for each query and just limiting the results to keep the page size small ... this would definately make maintaining the ids easier
-
<?php if ($row_pagecontent['subid'] != '5' || ($row_pagecontent['subid'] != '5' && mysql_num_rows($extras) > 0)): ?>
the div will always show if the pagecontent.subid != 5 because of the or(||) operator you will always have a logical truth.
try this:
<?php if ($row_pagecontent['subid'] != '5' && mysql_num_rows($extras) > 0): ?>
-
Instead of pulling in content based on a PHP ID variable, is it possible to pull in content based on the TLD (such as 'www.clientswebsite.com')
So, if URL equals 'clientswebsite.com', then insert 'this file or image'. If URL equals 'anotherclientwebsite.com', then insert 'this file or image' instead.
Yes it is : (definately not using cookies) something like:
<? if('$_SERVER["SERVER_NAME"].$_SERVER["REQUEST_URI"' == "www.clientswebsite.com"){ include(whatever); }elseif('$_SERVER["SERVER_NAME"].$_SERVER["REQUEST_URI"' == "www.anotherclientwebsite.com"){ include(whateverElse); } ?>
-
you have to take a field that is manditory ... like their name (if your not entering that) and check to make sure its not null then proceed for my example im assuming they have to enter their own first name:
this would go directly after you start your while loop
if($row['first'] != ""){ ?> ** Your Form with info in it ** <? }else{ ?> ** Your blank form ** <? } ?>
-
If i understand you correctly you don't need arrays at all ... you could simply
write the script to insert the info 'weekly_hits' as the query executes ie:
$query = mysql_query(your count query); while($hits = mysql_fetch_row($query)){ mysql_query(your insert query) }
but since you asked about arrays one way to do it would be (but this is a serious memory hog)
is
arrayVar[sid] = hits;
ie.
arrayVar[12] = 13;
arrayVar[21] = 61;
but there this creates a large essentially empty array unless your sid's are closer in frequency IE sid 1 sid 2 sid 3
Trying to use conditionals on mysql results
in PHP Coding Help
Posted
try