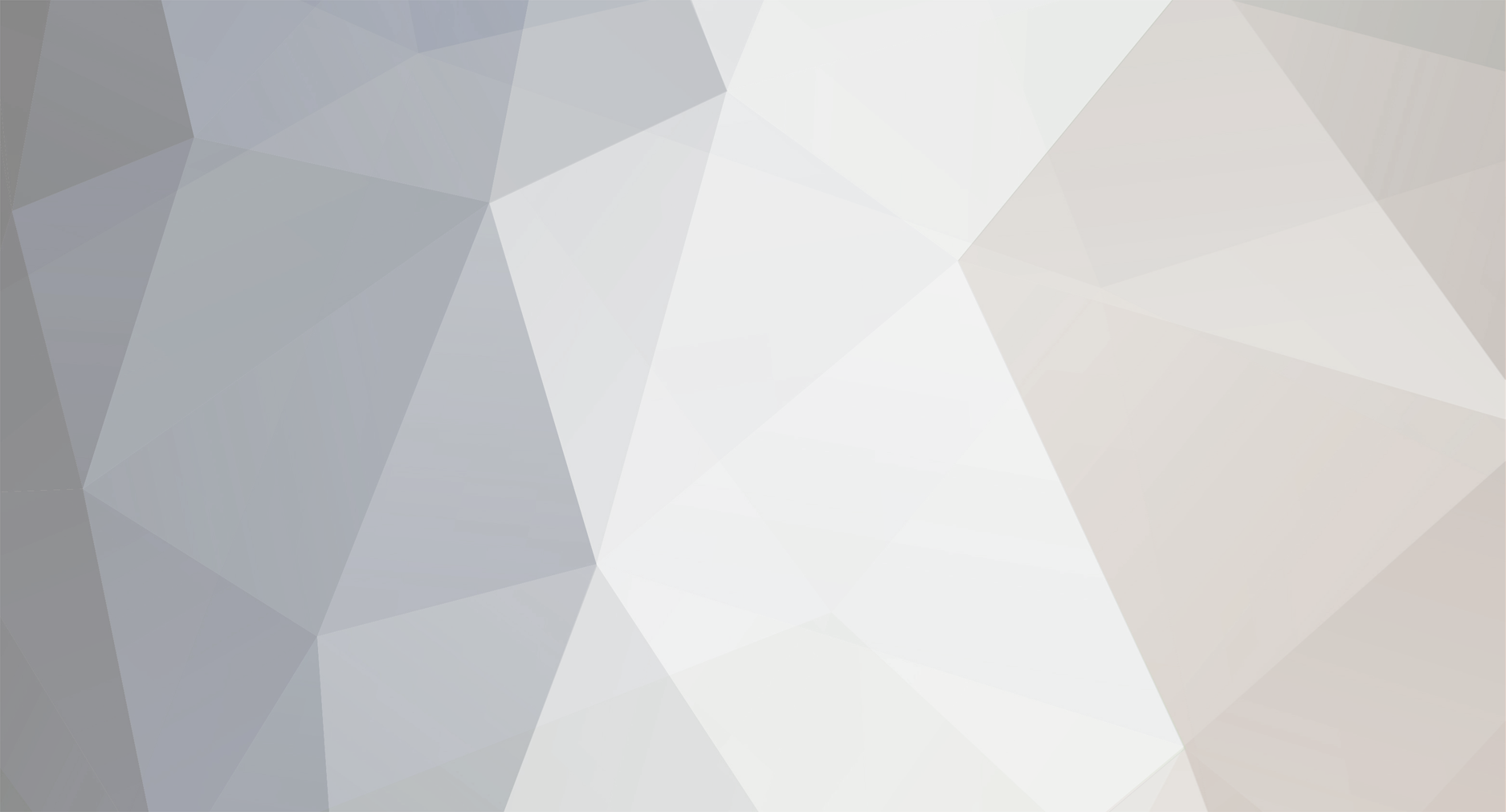
Jonob
Members-
Posts
77 -
Joined
-
Last visited
Everything posted by Jonob
-
OK, makes sense. Thank you.
-
Just getting my head around using $_SESSION to store variables. Lets assume that I am storing something like $_SESSION['company'] and I need to access this variable in all the functions in a file. Assume that my file looks as follows: <?php class test { function foo() { //Do something here } function bar() { //Do something else here } } ?> Is there an easy way for me to do something like $company = $_SESSION['company'] at the top of the php file (or class), and then use $company_id in each function? i.e. can I use $company_id as a variable in each function, without having to redeclare it inside each function??
-
...or so I have read However, being a newbie to php I have no idea how to get around this. Simplistically, I have many functions (across various classes and files) that must reference some variable - lets call it $status_id. I originally determine this variable via a sql query to the database. Am I meant to requery the database each time I want to know the value of $status_id?
-
Just in case anyone wants to know in the future, this is what got me the right answer: SELECT c.company_id, c.company_name, t1.* FROM company c LEFT JOIN (select max(status_date) as date, status_id, description, company_id from status group by company_id) as t1 ON c.company_id = t1.company_id
-
Hi all, Assuming I have the following structure table: company company_id company_name table: status status_id company_id (FK) status_date description How do I get all the records from company, and only the single most recent record (by status_date) from status? I want to show all fields from both tables, and if there is no record in status, then it should return nulls for each field in status. Thanks for any help.
-
Hi all, My dev environment is in eclipse, and I have tried both PDT and PHPElcipse. All works fine. I am trying to get to grips with using xdebug, and it all seems to be working fine. Part of what I need to do calls for me to install the xdebug helper from https://addons.mozilla.org/en-US/firefox/addon/3960 into Firefox. Whilst the addon installs fine, I dont get any icon on my firefox status bar. Ive even tried completely uninstalling firefox and reinstalling xdebug helper on a fresh firefox install, but no difference Unfortunately it seems that this helper is not supported at all, so I was hoping that someone here might have some idea on how to get around this. Thanks for any advice you can give.
-
[SOLVED] Best way to loop through an array and sum
Jonob replied to Jonob's topic in PHP Coding Help
Ahh, thats awesome, thank you. The only bit that you left out was GROUP BY a at the end -
[SOLVED] Best way to loop through an array and sum
Jonob replied to Jonob's topic in PHP Coding Help
Thanks for the replies. To be honest, that was my original approach, but I ran into a wall there...the reason being the data comes from 5 different tables. Maybe I am approaching this all wrong, but here is my query: SELECT b.bank_id, b.bank_name, IFNULL(ROUND(b.start_bal,2),0) as total_amount FROM bank b WHERE b.is_deleted = '0' AND b.company_id = 1 AND b.bank_type_id<4 AND b.is_deleted='0' UNION (SELECT i.bank_id, b.bank_name, ROUND(SUM(id.amount_in),2) as total_amount FROM income_detail id LEFT JOIN income i ON i.income_id = id.income_id LEFT JOIN bank b on i.bank_id = b.bank_id WHERE i.is_deleted = '0' AND b.company_id = 1 AND b.bank_type_id<4 AND b.is_deleted='0' group by i.bank_id) UNION (SELECT e.bank_id, b.bank_name, -ROUND(SUM(e.amount_in),2) as total_amount FROM expense e LEFT JOIN bank b on e.bank_id = b.bank_id WHERE e.is_deleted = '0' AND b.company_id = 1 AND b.bank_type_id<4 AND b.is_deleted='0' group by e.bank_id) UNION (SELECT a.bank_id, b.bank_name, -ROUND(SUM(a.amount_in),2) as total_amount FROM asset a LEFT JOIN bank b on a.bank_id = b.bank_id WHERE a.is_deleted = '0' AND b.company_id = 1 AND b.bank_type_id<4 AND b.is_deleted='0' group by a.bank_id) UNION (SELECT a.bank_id, b.bank_name, ROUND(SUM(a.amount_in),2) as total_amount FROM asset_disposal a LEFT JOIN bank b on a.bank_id = b.bank_id WHERE a.is_deleted = '0' AND b.company_id = 1 AND b.bank_type_id<4 AND b.is_deleted='0' group by a.bank_id) And no, these tables cannot be combined into one big table; they are normalised and in separate tables for a good reason. If, however, there is an easier way to get the sum, then I'd love to hear it. -
I have an array of arrays being returned from mysql as follows. Array ( [0] => Array ( [bank_id] => 1 [bank_name] => Bank 1 [total_amount] => 100.00 ) [1] => Array ( [bank_id] => 2 [bank_name] => Bank 2 [total_amount] => 0.00 ) [2] => Array ( [bank_id] => 1 [bank_name] => Bank 1 [total_amount] => 600.00 ) [3] => Array ( [bank_id] => 2 [bank_name] => Bank 2 [total_amount] => 300.00 ) [4] => Array ( [bank_id] => 3 [bank_name] => Bank 3 [total_amount] => -200.00 ) [5] => Array ( [bank_id] => 2 [bank_name] => Bank 2 [total_amount] => -10 ) ) What is the easiest way for me to loop through and return an array of unique [bank_id], [bank_name] and sum([total_amount]) for each bank_id? Using the above figures, I would like to return: Array ( [0] => Array ( [bank_id] => 1 [bank_name] => Bank 1 [sum] => 700.00 ) [1] => Array ( [bank_id] => 2 [bank_name] => Bank 2 [sum] => 290.00 ) [2] => Array ( [bank_id] => 3 [bank_name] => Bank 3 [sum] => -200.00 ) ) Thanks for any help you can provide.
-
Very nice, thank you
-
OK cool, thanks for the answer. Lets say I put 5 (linked) queries into the try block, will it roll back all of them if an error is caught in only the last one? If so, then I can definitely see the use in this.
-
Does anyone actually run mysql queries through a php try-catch block? I've come across code that looks something like: mysql_query("BEGIN"); try { $sql = "some sql statement"; mysql_query( $sql); } catch (Exception $E) { mysql_query("ROLLBACK"); return false; } mysql_query("COMMIT"); return true; Is this actually considered "good" practise? Or is a plain old mysql_query($sql) or die(mysql_error()); good enough?
-
Yup, that works. Fargin hell, that drove me mad. ??? Thank you so much for the help.
-
Have to say that I am totally stumped by this one. 1. I created the following a directory on my domain called testy as follows : www.mydomain.com/testy 2. I created a file called rename.php with the following code: <?php $old = "old.gz"; $new = "new2.gz"; if (file_exists($old)) { echo "<br>Original file (" . $old . ") exists"; } else { echo "<br>Original file (" . $old . ") does NOT exist, exiting..."; die(); } rename($old,$new); if (file_exists($new)) { echo "<br>Success!! New file (" . $new . ") created"; } else { echo "<br>Failure. New file (" . $new . ") was not created"; die(); } ?> 3. I placed a small file called old.gz in the above directory 4. I navigate on my browser to http://www.mydomain.com/testy/rename.php and I get the output: Original file (old.gz) exists Success!! New file (new.gz) created 5. I check ftp, and file has indeed been changed. OK cool, that that works fine. 6. I rename the file back to old.gz 7. I create a cron as follows: /usr/local/bin/php /home/foo/public_html/testy/rename.php 8. Cron runs, and I get an error message from the cron: Original file (old.gz) does NOT exist, exiting... 9. I log in via ftp and sure as hell old.gz is there 10. I go again in browser to http://www.mydomain.com/testy/rename.php and I get the same success message as above. Double check in ftp - yup, file name has been changed. Any ideas why this is working via http but not via cron? Any tips greatly appreciated.
-
More details: The existing code (stripped down for these purposes) is shown below. Basically, I take 2 variables: $gross_salary and $tax_code and put them into the function called get_tax_calc. This will return an array called $result, with $result['net_salary'] being the end calculation. For example, if we put $gross_salary = 50000, and $tax_code = 603, then we get $result['net_salary'] = 35470.60. What I would like to try do is reverse engineer this. So, given a net salary and tax code, can we calculate a gross salary? Thanks for any pointers or help that you can provide. <?php class earnings_sim { /** * Returns the full results of a tax calculation * from a given salary and tax code */ function get_tax_calc($gross_salary, $tax_code) { $personal_allowance = $this -> calc_personal_allowance($tax_code); $taxable = round(max($gross_salary - $personal_allowance,0),2); $paye = $this -> calc_tax ($taxable, "income"); $employee_ni = $this -> calc_tax ($gross_salary, "employee"); $result['gross_salary']= $gross_salary; $result['personal_allowance'] = $personal_allowance; $result['taxable'] = $taxable; $result['paye'] = $paye; $result['employee_ni'] = $employee_ni; $result['net_salary'] = round($gross_salary- $paye - $employee_ni,2); return $result; } /** * Calculates the personal allowance * from a given tax code */ function calc_personal_allowance($tax_code) { return (substr($tax_code,0,3) * 10) + 5; } /** * Calculates the amount of tax payable * $taxable = value to be worked on * $type = income, dividend, employee or employer */ function calc_tax($taxable, $type) { $weekly = false; $periods = 52; if ($type == "income" or $type == "dividend") { $data = $this -> get_tax(); $column = $type . "_rate"; } elseif ($type == "employee") { $data = $this -> get_tax_employee(); $column = "rate"; $weekly = true; } elseif ($type == "employer") { $data = $this -> get_tax_employer(); $column = "rate"; $weekly = true; } if ($weekly) { $taxable = round($taxable/$periods,2); } foreach ($data as $key => $value) { $lower = $data[$key]['lower_threshold']; $upper = $data[$key]['upper_threshold']; $rate = $data[$key][$column]; if ($taxable > $upper) { $tax += ($upper - $lower) * $rate; } else { $tax += ($taxable - $lower) * $rate; break; } } if ($weekly) { return round($tax * $periods,2); } else { return round($tax,2); } } /** * Tax tables for income and dividends */ function get_tax() { $result['0'] = array( "lower_threshold" => 0, "upper_threshold" => 34800, "income_rate" => 0.2, "dividend_rate" => 0.1) ; $result['1'] = array( "lower_threshold" => 34800, "upper_threshold" => 10000000, "income_rate" => 0.4, "dividend_rate" => 0.325 ); return $result; } /** * Tax tables for employee NI */ function get_tax_employee() { $result['0'] = array( "lower_threshold" => 0, "upper_threshold" => 105, "rate" => 0 ); $result['1'] = array( "lower_threshold" => 105, "upper_threshold" => 770, "rate" => 0.11, ); $result['2'] = array( "lower_threshold" => 770, "upper_threshold" => 10000000, "rate" => 0.01, ); return $result; } } ?>
-
Calculating Net salary from a given Gross salary is slightly complicated in the UK, given that there are two different taxes, each working off a different sliding scale. But no big deal, I've managed to get this part down right in php. However, I'm now trying to go the other way, and try calculate Gross salary from a given Net salary. I'm not sure this is even possible. So before I go down that route, I was just wondering if there are any PHP "solver" type functions out there like Excel's Solver or Goal Seek. These work on an iterative process, trying various 'input's until it narrows down the right answer. Failing that, if there are any maths boffins out there that would like to give this a try, let me know and I will provide more details
-
Lets say I have a (simplified) mysql query as follows: SELECT DATE_FORMAT(i.date,'%c') as income_month, DATE_FORMAT(i.date,'%Y') as income_year, sum(i.amount_ex) as income_amount FROM income i WHERE i.date>= "2008-01-01" AND i.date <= "2008-03-31" GROUP BY income_month, income_year Basically, I am grouping by month number and year between a specific date range. Is there any way for me to get all months returned in my date range (with a zero for the income_amount alias), even if no data actually exists for each month? For example, lets say that we have data in Jan and Feb, but not in March, so the sql returns something like: income_month, income_year, income_amount 1, 2008, 100 2, 2008, 200 3, 2008, 0 <-- This is the row that the sql auto creates
-
Thanks for the replies. The vast majority of my array variables are either int or float, so I would typecast those individually, such as (int)$data['company_id']; (float)$data['some_value']; I fully understand the array-walk or array_map, but I think its less resource intensive for me to just 'clean' the strings individually, since very few of the variables are going to be string type. clean_string($data['description']); For arrays that have a higher proportion of strings, then I would certainly use array_walk or array_map - thanks for the advice
-
I am using MySQL as a backend to a flex project, with PHP+AMFPHP as the interface. From a security point of view, I am in the process of typecasting variables that are passed from flex to my php services. For example, if its a get (i.e. sql SELECT) function against an _id field, then I just do something simple like the following, before passing it into my sql statement: (int)$company_id; For strings, I use something like: function clean_string($string) { trim($string); escapeshellarg($string); // Stripslashes if magic quotes is on if (get_magic_quotes_gpc()) { $string = stripslashes($string); } // Clean if not integer if (!is_numeric($string) || $string[0] == '0') { $string = mysql_real_escape_string($string); } return $string; } My question: In many cases, I am running INSERT queries based on an array of variables that is passed to PHP. Should I use the clean_string() function on each individual string variable, or can I run it on the whole constructed $sql string (i.e. after all the variables have been passed into the $sql string, but before its been executed)?
-
I have a flex application that uses mysql and php on the backend, which is run through an amfphp installation. Data is transferred between the database and the front end via amfphp. I have this all working 100%. In this scenario, there are a number of php files, each of which contains one class (and each class has the same name as the file name). Within each class there are a number of functions. I am now considering the security side of the app, and would like to implement $_SESSION variables. I have read up on how to work with sessions in PHP, but its been pretty difficult to try decipher how to implement it in a amfphp environment. Am I even on the right track here? 1. Once a user has been logged in, you store 1 or more pieces of data in the $_SESSION variable, such as the user_id or user_name. So, something like this: <?php class login { function do_login { session_start(); session_unset(); //Code to check if the login is correct //If login is valid then create the session if (!isset($_SESSION['userID'])) { $_SESSION['userID'] = $userID; } } } ?> 2. On all subsequent service calls, I need to check if(!isset($_SESSION['userID'])) { return "Error: Session not set"; } Where does this check take place? After the opening <?php tag, inside the class, or at the start of each function? Any other glaring errors or omissions? Thanks for any tips that you can give.
-
Thanks for the help. Much appreciated.
-
Thanks guys, works perfectly On the same topic, assuming the following: -I have one class in a php file -I have many functions in that class -I need to reference other classes from other php files in only ONE of those functions Is it considered OK coding practise to put the require_once("filename.php") code inside that single function? Or should it be just after the opening <?php tag?
-
New to php, but learning as I go. Lets say I have a php file called user.php class user { function first(); { Some code here return $result; } function second(); { //I want to refer to function first $test = $this -> first() return $test } } OK cool, so if I want to refer to another function in the same class, then I use $this -> Now, what happens if I have a second php file called (for example) company.php, and inside that file I have a class called company, and inside that class a function called get_company(). I want to use get_company() in user.php, so at the top of user.php I put require_once("company.php") Lets say I want to use the get_company function in the second() function. I've tried to reference it as $test = $this -> get_company() but it throws an error. I guess my question is: how do I reference a function in a different class?
-
I have a mysql table that stores a parent-child relationhsip in a hierarchy - this relationship may be one or many levels deep. The structure of my table is: user_provider_id: PK provider_id: FK parent_id: points to user_provider_id in the same table - this is what defines the parent-child relationship The table is returned to php, which it is processed via an array into a tree structure. The function that I am using, which works perfectly, is as follows: function provider_tree($provider_id) { $sql = "SELECT up.* FROM user_provider up WHERE provider_id = $provider_id"; $list = do_array($sql); // Set up indexing of the above list (in case it wasn't indexed). $lookup = array(); foreach($list as $item) { $lookup[$item['user_provider_id']] = $item; } // Now build tree. $tree = array(); foreach($lookup as $id => $foo) { $item = &$lookup[$id]; if($item['parent_id'] == 0) { $tree[$id] = &$item; } else if(isset($lookup[$item['parent_id']])) { $lookup[$item['parent_id']][$id] = &$item; } else { $tree['_orphans_'][$id] = &$item; } } return $tree; } As you can see, this will return all records (i.e. ALL user_provider_id) where provider_id = $provider_id. What I would like to do is also limit the tree to a specific user_provider_id, and only have the tree for that user_provider_id and all of its children (and their children, etc). I will obviously have to pass in $provider_id and $user_provider_id to the function. I could of course change the sql query to: $sql = "SELECT up.* FROM user_provider up WHERE up.provider_id = $provider_id AND (up.user_provider_id = $user_provider_id OR up.parent_id = $user_provider_id) "; But this will not return children of children and lower. So, I suspect that I need to retain my original sql query and do the tree processing in php, but limit the parent to $user_provider_id and all children of that parent. Not quite sure on the best way to approach this - any help greatly appreciated.
-
Hi all, New to this php and mysql thing, but getting there slowly but surely. I am running mysql 5.05 I have a query as follows, which works 100%: SELECT i.*,c.name, (SELECT SUM(amount_inc) FROM income_detail WHERE invoice_id = i.invoice_id) AS paid FROM invoice i LEFT JOIN counterpart c on i.counterpart_id = c.counterpart_id WHERE i.is_deleted = 'N' AND i.company_id = 1 AND i.counterpart_id = 1 ORDER BY i.date However, what I would like to do, is limit the results to cases where i.amount_inc>paid I tried the following, but it doesnt like the way that I referenced the 'paid' part of the code. SELECT i.*,c.name, (SELECT SUM(amount_inc) FROM income_detail WHERE invoice_id = i.invoice_id) AS paid FROM invoice i LEFT JOIN counterpart c on i.counterpart_id = c.counterpart_id WHERE i.is_deleted = 'N' AND i.company_id = 1 AND i.counterpart_id = 1 AND i.amount_inc > paid --This is the new line ORDER BY i.date What am I missing?