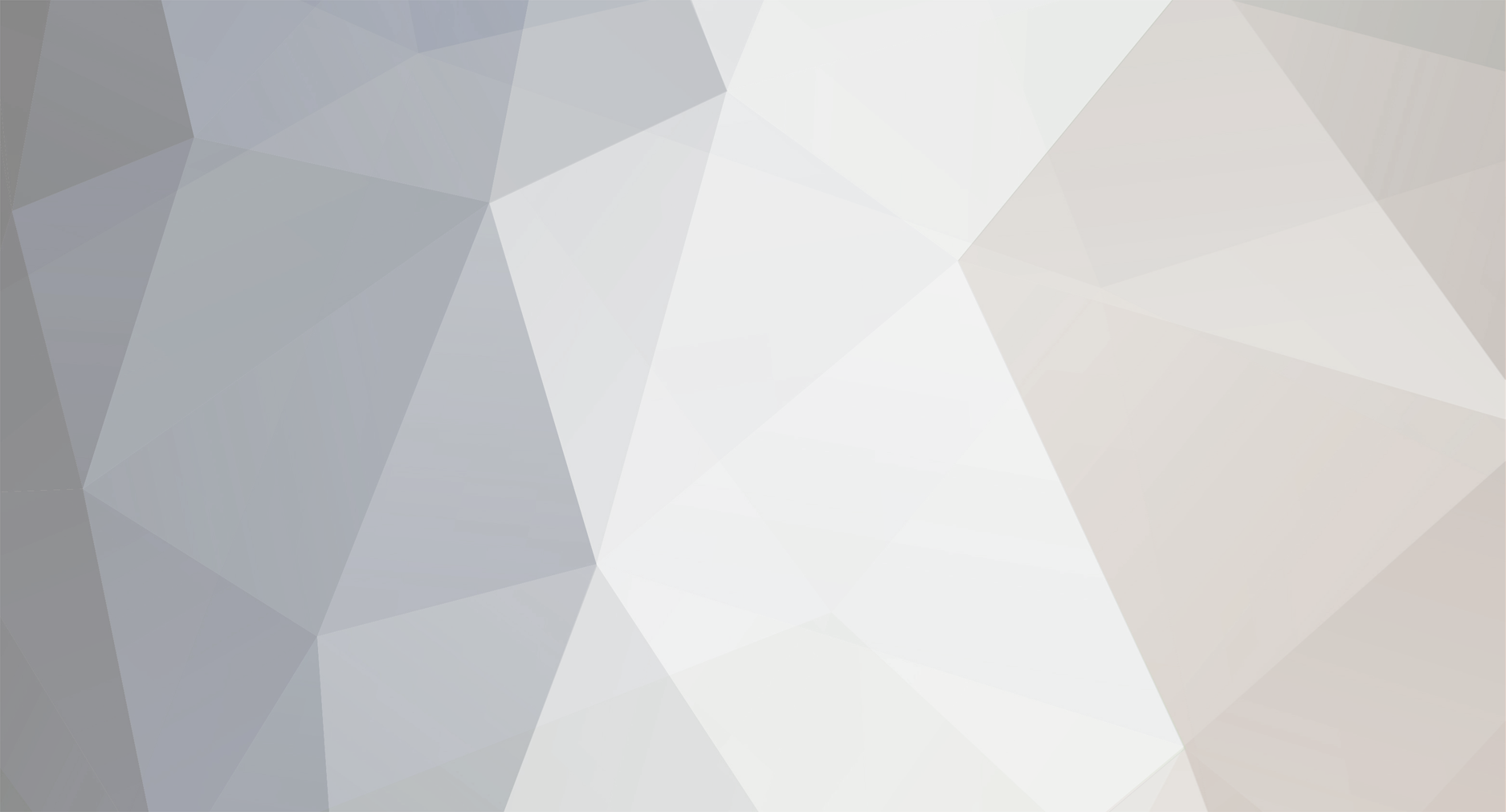
cpd
-
Posts
883 -
Joined
-
Last visited
-
Days Won
5
Posts posted by cpd
-
-
If you're using PDO you can use a different data structure. What you have at the minute is really confusing and can be massively simplified. I'd be happy to assist if you like...
And no I wasn't about to suggest PDO, that's an object for interfacing with a database...
-
Are you programming procedurally? If not I suggest you look at using a different data structure.
If you are, use a two dimensional array as already suggested.
E.g.
$a = array( "tile1" => array( "card1", "card2", "card3" ), "tile2" => array( ... ) );
-
Could you explain what you're trying to achieve? Provide a scenario and hopefully I can assist off that, along with anyone else.
-
To directly answer your question: it's because of the array. It doesn't resolve
$$something
where$something
is an array element as you have, to a variable variable.Now, why are you doing it this anyway? I've not once had to use a variable variable and have always advised to avoid it as there are cleaner methods.
-
http://www.html-form-guide.com/php-form/php-form-action-self.html
Scroll down to "What are PHP_SELF exploits and how to avoid them". I wouldn't use PHP_SELF as you have.
Have you connected to the database? Have you even created a database? What is the field type you're trying to insert to in the database as this dictates the format you use?
Do your research, come back with a specific problem once you've attempted it yourself.
-
-
Yes it can. Provided you define the relationship between the "Drops" properly.
I'm bored of reading posts where people can't be bothered to wrap their code in code tags.
-
Just goes to show how long I've not used MySQL for. I thought it was already deprecated.
-
Do you want a bib and high chair?
-
You could do it completely with SQL. Something along the lines of:
SELECT SUM(TIMESTAMPDIFF(SECOND, `start`, `finish`)) `total_seconds` FROM `table_name`
You can then format the seconds with something like
date("H\hrs i\m", $seconds)
. -
Scan the text file for the IP address, if you find it do nothing, if you don't add it to the text file.
-
Have you researched AJAX? It would appear not, try tackling it yourself first.
-
Change
if(preg_match("^[a-z0-9]+([_\\.-][a-z0-9]+)*" ."@"."([a-z0-9]+([\.-][a-z0-9]+)*)+"."\\.[a-z]{2,}"."$",$email )){
to
if(preg_match("#^[a-z0-9]+([_\\.-][a-z0-9]+)*@([a-z0-9]+([\.-][a-z0-9]+)*)+\\.[a-z]{2,}$#",$email )){
And thanks.
-
Your
if(preg_match...
has no ending delimiter and from the look of it no starting one either. I assume you've used ^ to signify the beginning of the string.Insert delimiters, e.g.
preg_match("#([A-Z0-9]+)#", $string)
where the # is the delimiter. -
So, in a single sentence:
"When the XML file exists but has no contents, i.e. does not contain an 'entries' element, I can't write to it".
And once again, I still stand by my original comments regarding breaking the problem down into smaller ones and this being a simple problem.
<?php $file = "entries.xml"; $default = "entries_default.xml"; // Does the file exist? Does it contain data? if(!file_exists($file) || ($xml = file_get_contents($file)) == "") { $xml = file_get_contents($default); } // Looad the XML in $dom = new DOMDocument("1.0", "UTF-8"); $dom->loadXML($xml); // Was the form submitted? if(isset($_POST['xml_append'])) { // Get the root node $root = $dom->getElementsByTagName("entries"); // Was the root node found? No, so create the file again using the default if($root->length == 0) { $dom->loadXML(file_get_contents($default)); } $root = $dom->getElementsByTagName("entries")->item(0); // Unset the submit button post data unset($_POST['xml_append']); // Create variables for each $_POST element extract($_POST); // Create the report element $report = $dom->createElement("report"); $report->appendChild($dom->createElement("timestamp", gmdate("Y-m-d H:i:s"))); $report->appendChild($dom->createElement("fname", $fname)); $report->appendChild($dom->createElement("lname", $lname)); $report->appendChild($dom->createElement("location", $location)); $report->appendChild($dom->createElement("type", $type)); $report->appendChild($dom->createElement("description", $description)); // Append the report $root->appendChild($report); // Save as XML $dom->save("entries.xml"); } // Get the last piece of data saved $reports = $dom->getElementsByTagName("report"); if($reports->length > 0) { $data = array(); $children = $reports->item(($reports->length-1))->childNodes; foreach($children as $child) { $data[$child->nodeName] = $child->textContent; } } ?> <html> <body> <form method="post"> <fieldset style="display: inline-block;"> <label>Fname</label><input type="text" name="fname" value="<?=(isset($data) ? $data["fname"] : "");?>" /><br /> <label>Lname</label><input type="text" name="lname" value="<?=(isset($data) ? $data["lname"] : "");?>" /><br /> <label>Location</label><input type="text" name="location" value="<?=(isset($data) ? $data["location"] : "");?>" /><br /> <label>Type</label><input type="text" name="type" value="<?=(isset($data) ? $data["type"] : "");?>" /><br /> <label>Description</label><input type="text" name="description" value="<?=(isset($data) ? $data["description"] : "");?>" /><br /> <input type="submit" name="xml_append" value="Save" /> </fieldset> </form> </body> </html>
A less than 5 minute edit to do what you want. This now performs two additional checks: 1) Checks if anything is in the XML file and if not uses the default; 2) Checks for an "entries" node and if it doesn't exist, uses the default.
-
I'm either seriously missing something, or have interpreted your posts correctly and you're failing to match the dots up.
<?php $file = "entries.xml"; // Does the file exist? if(file_exists($file)) { $xml = file_get_contents($file); } else { $xml = file_get_contents("entries_default.xml"); } // Looad the XML in $dom = new DOMDocument("1.0", "UTF-8"); $dom->loadXML($xml); // Was the form submitted? if(isset($_POST['xml_append'])) { // Get the root node $root = $dom->getElementsByTagName("entries")->item(0); // Unset the submit button post data unset($_POST['xml_append']); // Create variables for each $_POST element extract($_POST); // Create the report element $report = $dom->createElement("report"); $report->appendChild($dom->createElement("timestamp", gmdate("Y-m-d H:i:s"))); $report->appendChild($dom->createElement("fname", $fname)); $report->appendChild($dom->createElement("lname", $lname)); $report->appendChild($dom->createElement("location", $location)); $report->appendChild($dom->createElement("type", $type)); $report->appendChild($dom->createElement("description", $description)); // Append the report $root->appendChild($report); // Save as XML $dom->save("entries.xml"); } // Get the last piece of data saved $reports = $dom->getElementsByTagName("report"); if($reports->length > 0) { $data = array(); $children = $reports->item(($reports->length-1))->childNodes; foreach($children as $child) { $data[$child->nodeName] = $child->textContent; } } ?> <html> <body> <form method="post"> <fieldset style="display: inline-block;"> <label>Fname</label><input type="text" name="fname" value="<?=(isset($data) ? $data["fname"] : "");?>" /><br /> <label>Lname</label><input type="text" name="lname" value="<?=(isset($data) ? $data["lname"] : "");?>" /><br /> <label>Location</label><input type="text" name="location" value="<?=(isset($data) ? $data["location"] : "");?>" /><br /> <label>Type</label><input type="text" name="type" value="<?=(isset($data) ? $data["type"] : "");?>" /><br /> <label>Description</label><input type="text" name="description" value="<?=(isset($data) ? $data["description"] : "");?>" /><br /> <input type="submit" name="xml_append" value="Save" /> </fieldset> </form> </body> </html>
Default XML file is as before.
This uses a form to write data via PHP to an XML file. It does not submit a form to an XML file, that's just silly. If there are no entries, it still writes to the file. If there are entries, it still writes to the file. It also attempts to get the last entry and populates the fields with the data.
I'm still standing by my original comments. And once again this was modified in less than 5 minutes.
-
No, it won't.
Care to explain? I was under the impression it was going to be removed in the next major release?
-
Okay well whats your issue because you've not really specified one? And query_wrapper is doing a fair amount of work so we'd need to see it to ensure its correctly formatting the query.
-
Edit: Miss read your code. What does query_wrapper do?
-
You've likely not included the MySQL extension in your php.ini file.
That said, get away from mysql and use mysqli or PDO. The mysql extension will be removed in the next PHP release.
-
Hence why I asked you to print the query out so you could identify your own error, in this case you've had someone kind enough to state it.
-
You never said you needed to populate the form using data from the XML file. The problem you specified was that you had an issue appending to an XML file.
Does this mean you want to populate the form with the first entry or the last entry?
Do you want to overwrite what's already in the XML file or append to it as they are two completely different tasks?
-
Read it again, my previous answer still stands. I took your two posts combined and wrote the following script, tested it, and confirmed it works as you want it too; unless I've completely misinterpreted your OP.
$file = "entries.xml"; // Does the file exist? if(file_exists($file)) { $xml = file_get_contents($file); } else { $xml = file_get_contents("entries_default.xml"); } // Looad the XML in $dom = new DOMDocument("1.0", "UTF-8"); $dom->loadXML($xml); $root = $dom->getElementsByTagName("entries")->item(0); // Create the report element $report = $dom->createElement("report"); // Add the reports children. $report->appendChild($dom->createElement("timestamp", gmdate("Y-m-d H:i:s"))); $report->appendChild($dom->createElement("fname", "First name")); $report->appendChild($dom->createElement("lname", "Last name")); $report->appendChild($dom->createElement("location", "Location")); $report->appendChild($dom->createElement("type", "Report type")); $report->appendChild($dom->createElement("description", "This is my description")); // Append the report $root->appendChild($report); // Save as XML $dom->save($file);
entries_default.xml
<?xml version="1.0" encoding="UTF-8"?> <entries> </entries>
Written in 5-10 minutes at 2:30am. Like I said, the problem is easy to solve if you break it down.
Take the advice next time.
-
You really don't make sense but I think I've got the gist of what you're asking. If you want responses to a problem you should really spend more time writing your post, use code tags for any code you include and not describe a bulk of the problem in the topic title.
The problem
You want to return a user to the "add product" page after they've just added a new product.
The process
- User is on the addprod.php page that displays a form with an action value of "add_prod.php" or similar.
- add_prod.php creates a new record in the database for the new product and redirects to the addprod.php after the process is complete.
- addprod.php displays a success/error message dependent on the outcome.
Is this correct?
Don't use JavaScript to display a success message. Instead, use a session to store a success message and display it on the next page. You can then use the header function to redirect, i.e.
header("Location: ... ")
.Furthermore, you need to sanitise your data as you are open to SQL injection at the minute. I strongly suggest you reconsider your file names as well as they're very confusing. A large system with this sort of file naming would confuse the crap out of me.
Newbie needs help please
in PHP Coding Help
Posted
Not necessarily. Its dependent on the functionality of your PHP script. Many people move scripts outside the public domain for added security but if its a simple template script you don't necessarily have to.