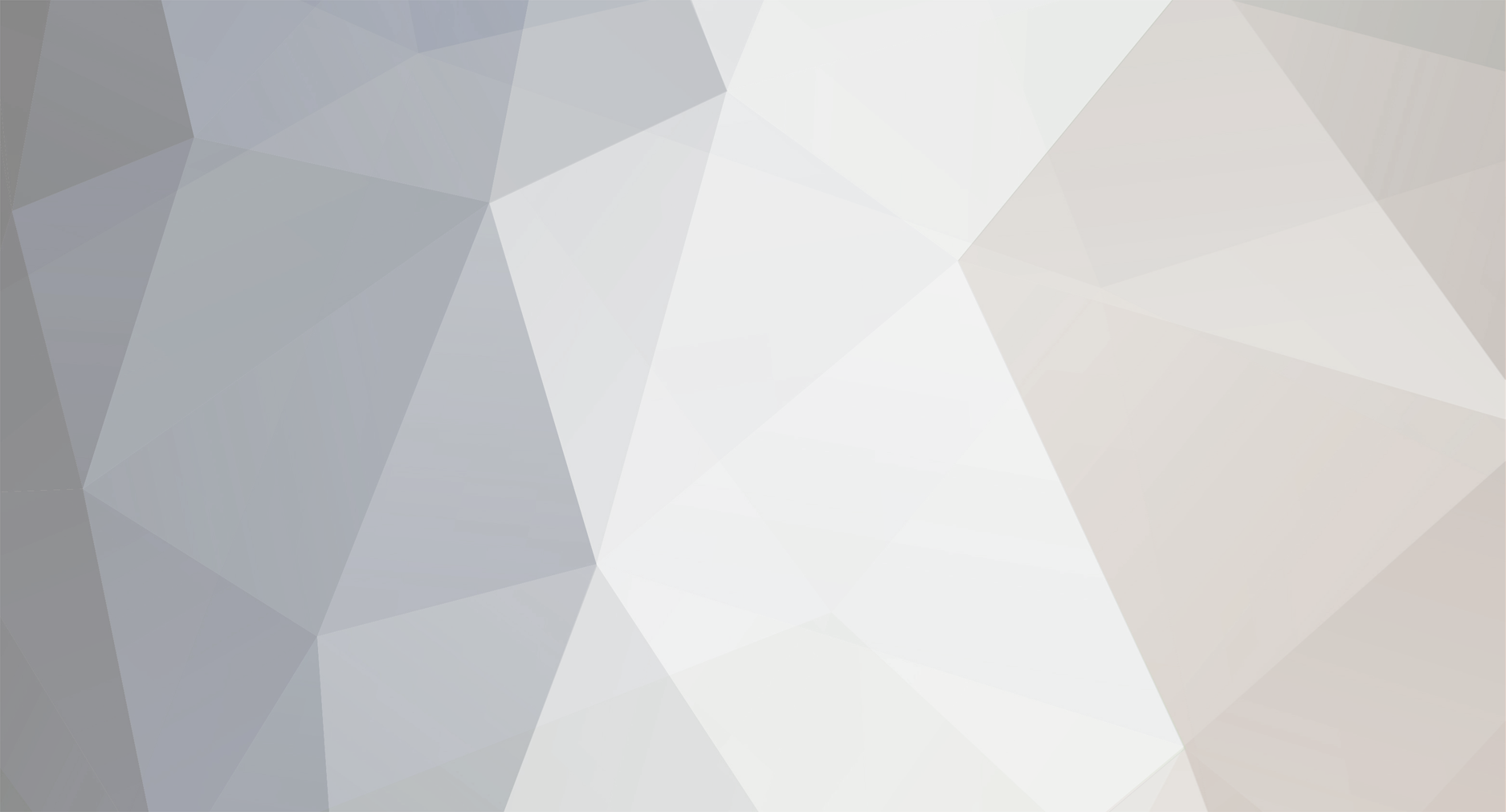
PravinS
-
Posts
459 -
Joined
-
Last visited
-
Days Won
2
Posts posted by PravinS
-
-
you can use isset() function to check if $_FILES['image'] is set of not like
if (isset($_FILES['image'])) { // your code here var_dump($_FILES['image']); // your code here }
may this will help you
-
you can check it like this
if (is_array($_POST['interest']) && count($_POST['interest']) > 0) { // code here }
-
-
i think your code should be like this
$event = array("Flash Flood Watch", "Flash Flood Warning", "Severe Thunderstorm Watch", "Severe Thunderstorm Warning", "Torndao Watch", "Torndao Warning"); $searchvalue = "Flash Flood Watch"; //as example if (in_array($searchvalue, $event)) { echo "Match found"; }
also go through in_array() function properly
-
-
give name as array for each field, as
<?php foreach ($items as $item) { echo'<div class="items-row"> <label for="name_'.$item['item_id'].'">'.$item['item_name'].'</label> <div class="item_input"><input type="text" name="quantity[]" id="'.$item['item_name'].'" value="0"></div> <input type="hidden" name="item_ids[]" value="'.$item['item_id'].'"> <input type="hidden" name="user_ids[]" value="'.$moving_basics_id.'"> </div>'; } ?>
-
your function should return the value like
<?php function test() { $response = "hello"; return $response; } ?>
and then you can call the function like this
<input type="textbox" name="xx" value="<?php echo test(); ?>" />
-
can you show us the actual code
-
thanks mate. its solve. it work perfectly on live server but its appearing "no database found" when running it offline. the database.php also include in the folder.
it means you haven't created database on your local machine or the database connection in database.php is not correct
-
at this line add mysql_error() like this and check what is the issue
$data=mysql_query("SELECT OT.nostaf, OT.otl_tkh_m, OT.otl_tkh_y ,OT.otl_jum_jam, OT.otl_jabatan FROM ot_line OT") or die(mysql_error());
-
mysql_select_db("ahamdabad_vpn_ip$", $dbc);$sql = "select main_system from ahamdabad_vpn_ip$";"ahamdabad_vpn_ip$" is you database or table name?also it is not good practice to use "$" in database of table name
-
use urlencode() and urldecode() functions
-
echo the SQL query and execute it in phpmyadmin
also write mysql_close($con); at the end the bottom of the code
-
check you SQL query, remove space between FROM and table name from SQL query
$query ="SELECT * From Section order by sectCode ASC";
-
your form method is post, so use POST while getting variable data
$destinationCode=$_POST['destinationcode']; $numberofadults=$_POST['numberofadults']; $numberofchildren=$_POST['numberofchildren']; $startdate=$_POST['stardate']; $enddate=$_POST['enddate'];
-
-
try uncommenting the if condition
<?php //$ab=1; $ab = ''; if(isset($_GET['id'])) $ab= $_GET['id']; echo $ab; ?>
-
-
You can also use TCPDF
-
-
input to "mysql_real_escape_string()" function should be string and you have passed array to it, so you are getting this error
if possible paste your code here
-
try using this SQL query
SELECT received, SUM(amount) as amt FROM TABLE_NAME GROUP BY received;
-
if you want to use server side valiation then you can use "filter_var" function to check the email or else you can use javascript email validation
-
try replacing this code
<form action="add_bk_insert.php"> Name <input type="text" name="name"/><br /> E-mail <input type="text" name="email" /><br /> Contact <input type="text" name="contact" /><br /> Requirement <input type="text" name="requirement" /><br /> <input type="submit" value="Submit" name="btnSubmit"/> </form>
<?php mysql_connect("localhost","root",""); mysql_select_db("test"); if (isset($_POST['btnSubmit'])) { $name = isset($_POST[name]) ? $_POST[name] : ''; $email_id = isset($_POST[email_id]) ? $_POST[email_id] : ''; $contact = isset($_POST[contact]) ? $_POST[contact] : ''; $requirement = isset($_POST[requirement]) ? $_POST[requirement] : ''; $order = "INSERT INTO sal2 (name,email_id, contact,requirement) VALUES('".$name."','".$email_id."','".$contact."','".$requirement."')"; $result = mysql_query($order); if($result) { echo "Inserted"; //include_once("testimonials.php"); } else{ echo("<br>Input data is fail"); } } ?>
There is a mistake somewhere....
in PHP Coding Help
Posted
your php code should be like this