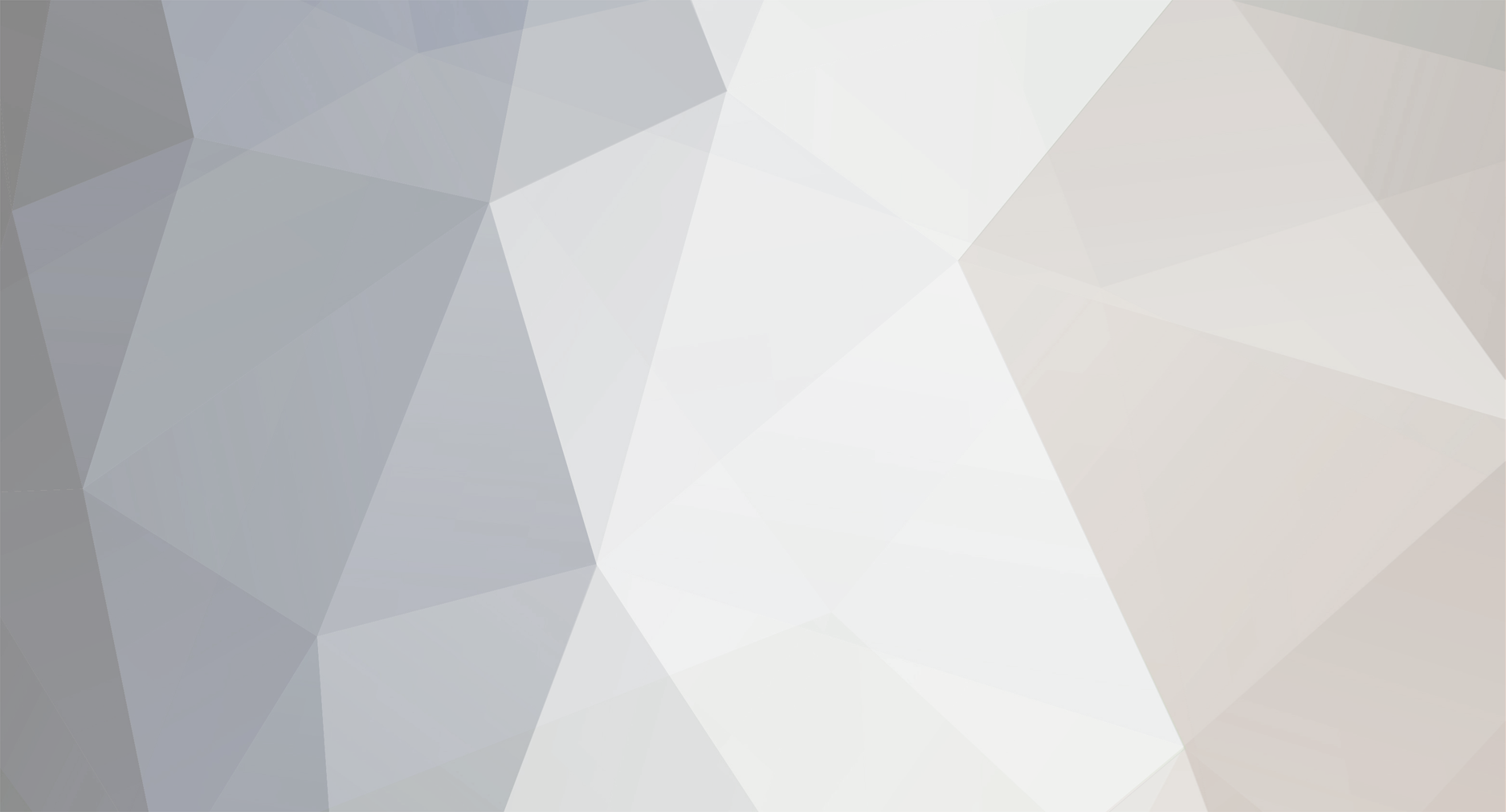
Omzy
-
Posts
314 -
Joined
-
Last visited
Never
Posts posted by Omzy
-
-
Hi Salathe,
Basically the most recent code I posted is meant to grab all links (with a class value of 'listinglink') from a given page.
I now want to run the code from my original post upon each of those links. So basically it's going to go into each of those links, find the required <P> tag and output it's data underneath the link.
So a sample output would be:
Link 1
P tag content
Link 2
P tag content
...and so on.
I tried using another curl() within the foreach loop but it doesn't seem to work.
P.S. thanks for the helpful tip on the foreach loop!
-
salathe,
Perhaps you can help me out with my final xpath query -
I've created a scrape script which fetches all links on a page:
$dom = new DOMDocument(); @$dom->loadHTML($html); $xpath = new DOMXPath($dom); $links = $xpath->query("//a[@class='listinglink']"); $i=0; foreach($links as $item) { $href = $links->item($i); $url = $href->getAttribute('href'); echo '<a href="'.$url.'">'.$url.'</a><br/>'; $i++ }
I now need to extend this further - it needs to go in to each link and perform the xpath query from my original post. Do you have any idea how I can do this?
-
holy sh*t! that is beautful! thanks buddy!
-
Basically I generated an XPath query that finds and outputs a <P> tag from a given page.
The problem I am having is that this <P> tag contains <BR> tags within it's content. For example:
<p>
100 New Drive
<br>
New Town
<br>
Manchester
<br>
M1 AAA
</p>
$address = $xpath->evaluate("/html/body/table/tr[5]/td[4]/p"); echo $address->item(0)->nodeValue;
This outputs:
100 New DriveNew TownManchesterM1 AAA
Ideally I want the <P> tag to be created into an array which is split up upon each <BR> tag. I can then put the data from this array into their own fields in the database.
Anybody got any suggestions on how to do this?
-
Anybody in the forum able to help at all?
-
Anyone able to help at all?
-
I've created a scrape script which fetches all links on a page:
$dom = new DOMDocument(); @$dom->loadHTML($html); $xpath = new DOMXPath($dom); $links = $xpath->query("//a[@class='listinglink']"); $i=0; foreach($links as $item) { $href = $links->item($i); $url = $href->getAttribute('href'); echo '<a href="'.$url.'">'.$url.'</a><br/>'; }
I now need to extend this further - it needs to go in to each link and get the content of a particular <p> tag on the page. So for example the page output should be as follows:
Link 1
P tag content
Link 2
P tag content
...and so on. The Xpath of the P element I need is "/html/body/form/center/table/tbody/tr[5]/td[4]/table/tbody/tr[3]/td/p"
Can anyone assist me with this?
-
Anybody in the forum able to offer any suggestions?
-
Anyone able to help?
-
Basically on my page I have 3 identical forms, for example:
<form method="post" action=""> <input type="text" name="name" value="<?php echo isset($_POST['name']) ? $_POST['name'] : null ?>" /> <input type="text" name="email" value="<?php echo isset($_POST['email']) ? $_POST['email'] : null ?>" /> <input type="hidden" name="form1" /> </form>
The hidden variable at the end helps me identify which form was submitted.
I've implemented validation and all is fine, apart from one thing. Whenever a form is returned back with validation errors, the matching fields on the other forms also get populated. Obviously this is because they all share the same 'name' attribute, but I have a requirement to keep the same names across all forms.
I could of course extend the isset() check to also check for the hidden field, but this creates too much replication.
Anybody have an elegant solution to this slight problem?
-
Here, I managed to figure this out for myself:
Controller:
$query="SELECT name, location, comments, date FROM reviews WHERE prod_id='$prod_id'"; $results=mysql_query($query); $numrows=mysql_num_rows($results); while($row=mysql_fetch_assoc($results)) { $name[]=$row['name']; $loc[]=$row['location']; $com[]=$row['comments']; $date[]=$row['date']; }
View:
for($i=0; $i<$numrows; $i++) { echo $name[$i]; echo $loc[$i]; echo $com[$i]; echo $date[$i]; }
I cannot beleive nobody here was able to tell me this, it would have saved me a lot of time.
-
Yes I know how to assign variables.
I want to have the variables defined in the Controller file, ready to be used in the view file.
View file should only have to loop through the resultset and echo out the variables, eg. $name or $location.
-
Not yet. Basically this is how it should work:
Controller:
-Run the query
-Get the resultset
-Put the resultset into an array
View:
-Loop through the array and output the data
Ideally I want to assign friendly variables to the $row array so they can be easily referenced in the view, e.g. $name instead of $row['name'].
Surely someone must have a solution to this simple scenario.
-
I'm not too conviced that's the correct way of doing it, but it won't work for me anyway because I'm including the controller file in the view file, not the other way round.
-
while($row=mysql_fetch_assoc($results)){
That's performing the query in the view file - ideally I would like the resultset to be available to the view file beforehand.
-
I suggest you re-read my original post...
Ideally I would like to do all the processing in the controller file and then be able to loop through the results in my view file. -
No, like I said I'm wanting to output the data in a separate view file.
-
I'm trying to output the list of product reviews from a table but I'm a bit stumped at the moment.
Table Reviews:
id
prod_id
comments
name
location
date
Obviously a product can have more than one review. I check that $prod_id matches prod_id field in the Reviews table.
Here is the code I have at the moment:
$query="SELECT * FROM reviews WHERE prod_id='$prod_id'"; $results=mysql_query($query); $numrows=mysql_num_rows($results); $row=mysql_fetch_assoc($results);
This code is in my controller file. So in my view file I need to output this data but I'm not sure if I should be using a while() loop or a foreach loop(). Ideally I would like to do all the processing in the controller file and then be able to loop through the results in my view file.
-
I've created a scrape script which fetches all links on a page:
$dom = new DOMDocument(); @$dom->loadHTML($html); $xpath = new DOMXPath($dom); $links = $xpath->query("//a[@class='listinglink']"); $i=0; foreach($links as $item) { $href = $links->item($i); $url = $href->getAttribute('href'); echo '<a href="'.$url.'">'.$url.'</a><br/>'; }
I now need to extend this further - it needs to go in to each link and get for example the content of all the <p> tags on the page. So for example the page output should be as follows:
Link 1
P tag 1 content
P tag 2 content
P tag 3 content
Link 2
P tag 1 content
P tag 2 content
...and so on. Can anyone assist me with this?
-
Any other suggestions?
-
Ideally I'd like to specify the labels in my controller and be able to output these in my view.
-
Cheers teamatomic.
Seems good but I'd rather not do it that way to be honest, the code I provided was a simplified version - my full code has around 15-20 fields and not all are mandatory. So I've got several isset() checks to set the $message elements - these checks would have to be replicated for my $content variable if I use the method you have suggested...
-
I have the following in my controller:
$message['name'] = htmlentities(strip_tags(trim($_POST['name']))); $message['email'] = htmlentities(strip_tags(trim($_POST['email']))); $to = "info@mywebsite.com"; $subject = "Website Enquiry"; $content = html_entity_decode(implode("\n\n", $message)); $content.= "\n\nIP Address: ".$_SERVER['REMOTE_ADDR']; $headers = "From: ".strip_tags(trim($_POST['name']))." <".strip_tags(trim($_POST['email'])).">"; @mail($to,$subject,$content,$headers);
And the following in my view:
echo '<tr><th><b>Name: </b></th><td>'.$message['name'].'</td></tr>'; echo '<tr><th><b>Email Address: </b></th><td>'.$message['email'].'</td></tr>';
This works as expected - the POST data is output to the page and is also sent in the email. However the email does not contain the field labels, for example 'Name: ' and 'Email Address: '.
This is obviously because I don't include the labels in their respective $message[] elements, as I need to enclose these in HTML tags in the view. I don't intend to include any HTML code in my controller, so does anyone have any idea how I can satisfy both requirements?
-
Anyone able to advise? Thanks.
rewrite rule for no page extension
in Apache HTTP Server
Posted
I'm basically after the rewrite rules to omit the .php extension of the URL, with and without the trailing slash, so for example:
localhost/page should redirect to localhost/page/
localhost/page/ should redirect to localhost/page.php
Currently I have this code:
RewriteCond %{REQUEST_FILENAME}\.php -f
RewriteRule ^(.*)$ $1.php
RewriteRule ^(.*)/$ $1.php
It does not seem to work for the URL with the trailing slash.