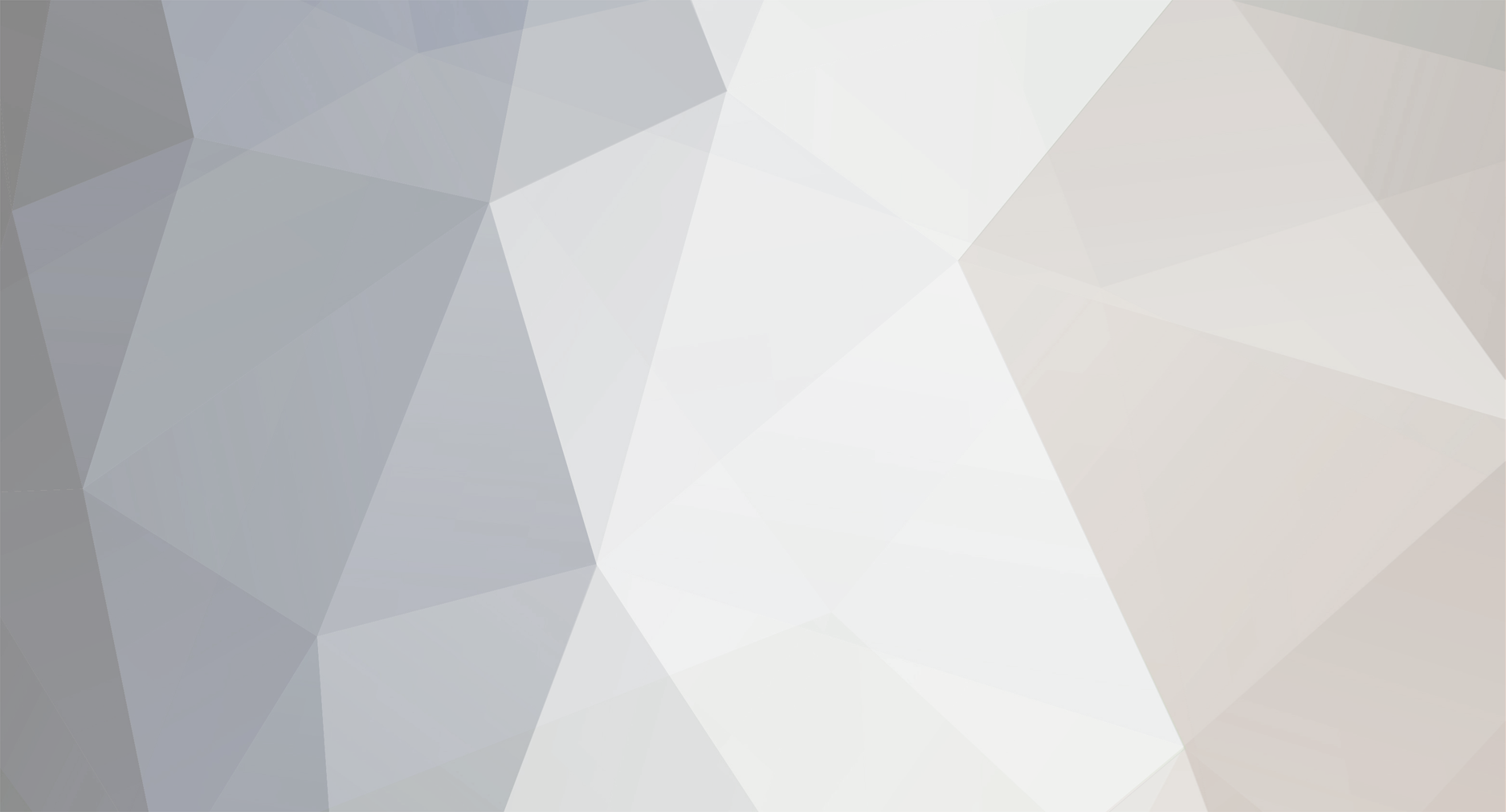
ejaboneta
-
Posts
168 -
Joined
-
Last visited
Posts posted by ejaboneta
-
-
Thanks! But just in case anyone looks to this post for help... HAVING should go after GROUP BY....
SELECT firstname, lastname, count( concat( firstname, lastname ) ) AS counter FROM leads GROUP BY concat( firstname, lastname ) HAVING counter >1 ORDER BY `counter` DESC LIMIT 0 , 30
-
I'm trying to make a "find duplicates" script. My query counts how many rows each "firstname lastname" combination is in the database. I want to only retrieve rows with more than one count. I get an unknown 'counter' column error when I run it. How can I get around this or is there a better way?
SELECT firstname, lastname, count( concat( firstname, lastname ) ) AS counter
FROM leads
WHERE `counter` > 1
GROUP BY concat( firstname, lastname )
ORDER BY `counter` DESC
-
I don't think its the query. I think its when IE trys to render the 2000 row table that it slows down. In both IE and Firefox, the page starts to display the results. But IE freezes up until the whole page is able to be displayed. In Firefox, you can scroll down to the bottom of the table and see the rows being loaded.
The table contents amount to almost 1MB.
-
I have a page that needs to display thousands of rows from a database... paging isnt an option... In firefox, the page displays and lets the user interact with the page while its still downloading. In IE, the page takes a long time to load and freezes up which of course frustrates my users who use IE. Is there anyway to make IE work more like Firefox and not freeze up?
-
I have a page that has a link "Schedule A Call" when it is clicked, a page is loaded into a div with ajax. The page that is loaded has a form to schedule an appointment, and a calendar so that a user can click a date and it will be loaded into the form. The page with the form works alone but not when it is loaded into a div.
This is the page that loads into the div:
http://leadstest.vanbergins.com/list/schedulecall.php
This is how I refer to the textfield in the link
<?php <a href=\"#\" onclick=\"document.scheduleform.scheduledate.value='$scheduletime'; return false;\">$date</a> ?>
-
I'm trying to fix a bunch of formatting errors that resulted in 1900 dates being converted into 2000 dates...
I'm trying to change 12/20/2012 to 12/20/1912. I can replace any /20 with /19 but I dont any days to be mixed up and being changed from 12/20/2012 to 12/19/1912.
I tried doing "/20.{2}$" but of course it I'll end up with 12/20/19. Sorry I'm new to regex so I am pretty lost.
<?php $data = '12/20/2012'; $birthstr = "/\/20/"; $birthrep = '/19'; $birthday = preg_replace($birthstr,$birthrep,$data); ?>
-
The first line of each csv I upload goes missing! Anyone know why?
<?php $row = 0; $handle = fopen("$file", "r"); $data = fgetcsv($handle, 1000, ","); while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { $num = count($data); $newvalues[firstname] = $data[0]; $newvalues[lastname] = $data[1]; $newvalues[birthday] = $data[3]; $newvalues[address] = $data[4]; $newvalues[city] = $data[5]; $newvalues[state] = $data[6]; $newvalues[zip] = $data[7]; $newvalues[phone] = $data[9]; $newvalues[sex] = $data[11]; $row++; echo "<br>"; $fdf_file=time(). rand(0,100) . '.fdf'; $fdf_dir=dirname(__FILE__).'/results'; $pdf_doc='Cigna.pdf'; $fdf_data=createFDF('Cigna.pdf',$newvalues); if($fp=fopen($fdf_dir.'/'.$fdf_file,'w')){ fwrite($fp,$fdf_data,strlen($fdf_data)); echo $fdf_file,' written successfully.'; }else{ die('Unable to create file: '.$fdf_dir.'/'.$fdf_file); } } fclose($handle); ?>
-
this seems kind of pointless? It doesn't look like you need to use eval at all
I guess not. Well I'm not skilled enough to figure everything out on my own so I had to ask.
-
try
<?php $values = array(); for($h=1; $h<$rowcount; $h++) { $val = array(); ##### GET VALUES ##### for($e=1; $e<$fieldcount; $e++) { $val[] = $_POST[$e][$h]; } $values[] = "('". implode("', '", $val) . "')"; } $values = implode(",\n",$values); ?>
Perfect! For what its worth, I found a workarond by using eval() to change $_POST[3] to $post3, and then another eval on $post3[4]. But that works better! Thanks!
-
Well the post comes from a dynamic amount of values from a dynamic amount of fields. I was trying to keep it simple and just ask for what i needed but maybe I should be more detailed.
I'm trying to allow my users to upload CSVs into our database. The problem is that the CSVs come from different places and so the field order and amount may be different. So I made a script that asks the user which columns in the CSV go into which column in the database. Then the next page, containing this code, formats the values to be inserted into the database.
Hopefully this helps to be more clear....
### FOR EACH ROW ### for($h=1; $h<$rowcount; $h++) { $values = $values . "$comma("; ##### GET VALUES ##### for($e=1; $e<$fieldcount; $e++) { $str1 = "\$_POST[$e][$h]"; eval("\$values = \$values . \"'$comma1$str1'\";"); $comma1 = ','; } $values = $values . ")"; $comma = ','; }
the result should look like:
('Joe','Smith','1234 Main St'),
('Sally','Smith','431 North St')
but I keep getting:
('Array[1]','Array[1]','Array[1]')
('Array[2]','Array[2]','Array[2]')
-
$e = '3'; $h = '4'; $str1 = "\$_POST[value$e][$h]"; eval("\$values = \"'$str1'\";"); echo $values;
I'm trying to get is the value of $_POST[3][4]... but the eval is processing the $_POST[3] and leaving the [4]. So what I end up with is Array[4]....
Any help?
-
-
The used command is not allowed with this MySQL version
-
So thanks to GoDaddys restrictions, I cant use mysql "LOAD DATA INFILE". My best solution is to use php to parse the csv files and insert them into the database. I'm not an "expert" so I don't know exactly how to do this. I'm sure I can find out but I wanted to ask first if there was an easier way or if anyone knows of a good guide to parsing or working with CSVs. Thanks
-
I'm an IT-Networking Major at Heald College in California. I'm about to enter the Planning Phase of my graduation project and I am at a loss on what I'd like to do. Anyone wanna give me some ideas? It doesn't necessarily have to be networking related but something to do with computers. I am usually more creative so something to get me started would be good enough. I'm sure I can tie in networking once I'm "inspired". Anything solid or abstract would be great! Thanks
-
Excuse my ignorance but I'd like to know the differences between scripting in php and C++. I do realize the obvious differences in php being interpreted and C++ being compiled. I'd just like to know how hard it would be for me to start learning C++ only having scripted in PHP. I've read that PHP is based on C/C++.
-
I'm basically making a spreadsheet application that is one big form with multiple rows. I want the users to be able to update multiple rows at once. The problem is that I don't want rows that were not changed to be updated.
How can I have the field be enabled when the user clicks or changes it? I know I can do this with check boxes but I don't want the user to have to click the checkbox to do it. So perhaps a checkbox that is checked when the user changes a field? I don't know much javascript so any advice will help.
-
I was reading on php security issues and i came across session hijacking. I was thinking about having the ip address stored in the session and matched before loading the page. Would that make sense? Is there a better way to protect against session id hijacking?
-
I've read the 2 pages and i was just a little confused. The conversation seemed to be going back and forth. And sorry, I didn't watch the video. I don't have working speakers connected.
-
Nope, thats from 2005. Matt Cutts spoke at WordCamp San Francisco 2007 conference saying that, Google will be going to treat underscores and dashes the same. Thats some time ago now.
Would it be safe to use underscores then? How about dots? I need a seperator in addition to dashes.
-
I'm trying to set Apache to forward urls that would include a persons first name and last name. I would then use the first and last name to grab the content from a database
example:
http://example.com/profiles/michael-jackson
forwards to
http://example.com/profiles.php?first=michael&last=jackson
I've got it working using
profiles/([a-zA-Z]*)(-*)([a-zA-Z]*)
from this I take the first part as the first name and the third part as the last name since the second part is "-".
I'm having problems with middle names and names with hypens. Like if the name was Neil Patrick Harris, it would become Neil-Patrick-Harris and my code would take "Neil" as the first name and "Patrick" as the last name.
I thought since I'd be using php to grab the actual content, later I could just grab the last word and use that as the last name but then there'd be the problem with hyphenated names. "John Doe-Smith" would become "John-Doe-Smith" and only "Smith" would be taken as the last name.
Any help on how I can do this? I figured the solution would be a regex one since thats how I'm formatting all this.
-
Google do currently, put more weight into urls with keywords. So using a url like This-is-the-title.html actually gives your site an unfair advantage, above those who use dynamic urls.
I would hardly call that an unfair advantage, an advantage, or advencement yes, but not an unfair one. A tiny bit of planning at the start of your project can give you a dynamic site (dynamic URIs) using normal URIs.
Take Codeigniter the framework for example;
http://www.example.com/about/the-team
A simple htaccess file gets rid of the index.php file in the URI and gives you a fully dynamic site. The above will load the 'about' controller and run the 'the-team' method.
So would
http://www.example.com/article1
better than
http://www.example.com/article1.php
??
-
I'm not sure this is done in PHP but I've seen some sites that have URLs like
example.com/article1
instead of
example.com/articles?id=1
How can I do this? I want more userfriendly addresses for my content.
-
What I meant was say I use my normal email to send the email to test@mysite.com.
What I want is to go to mysite.com/mail.php and see the message displayed there on the website.
I actually have been googling like crazy and found out about imap functions. But now i have a new problem. I can get the messages but they are displayed with a bunch of extra stuff i dont need. In this message, the body says "hello"
--0-497195976-1246665334=:79916 Content-Type: text/plain; charset=us-ascii hello --0-497195976-1246665334=:79916 Content-Type: text/html; charset=us-asciihello
--0-497195976-1246665334=:79916--
code:
$content = imap_body($conn, $i);
echo $content;
Loading in the wrong div
in Javascript Help
Posted
My site uses this as a generic code to load pages into a div. Sometimes when the page loads 2 or more, they get mixed up and load in the wrong div. How can I prevent this?