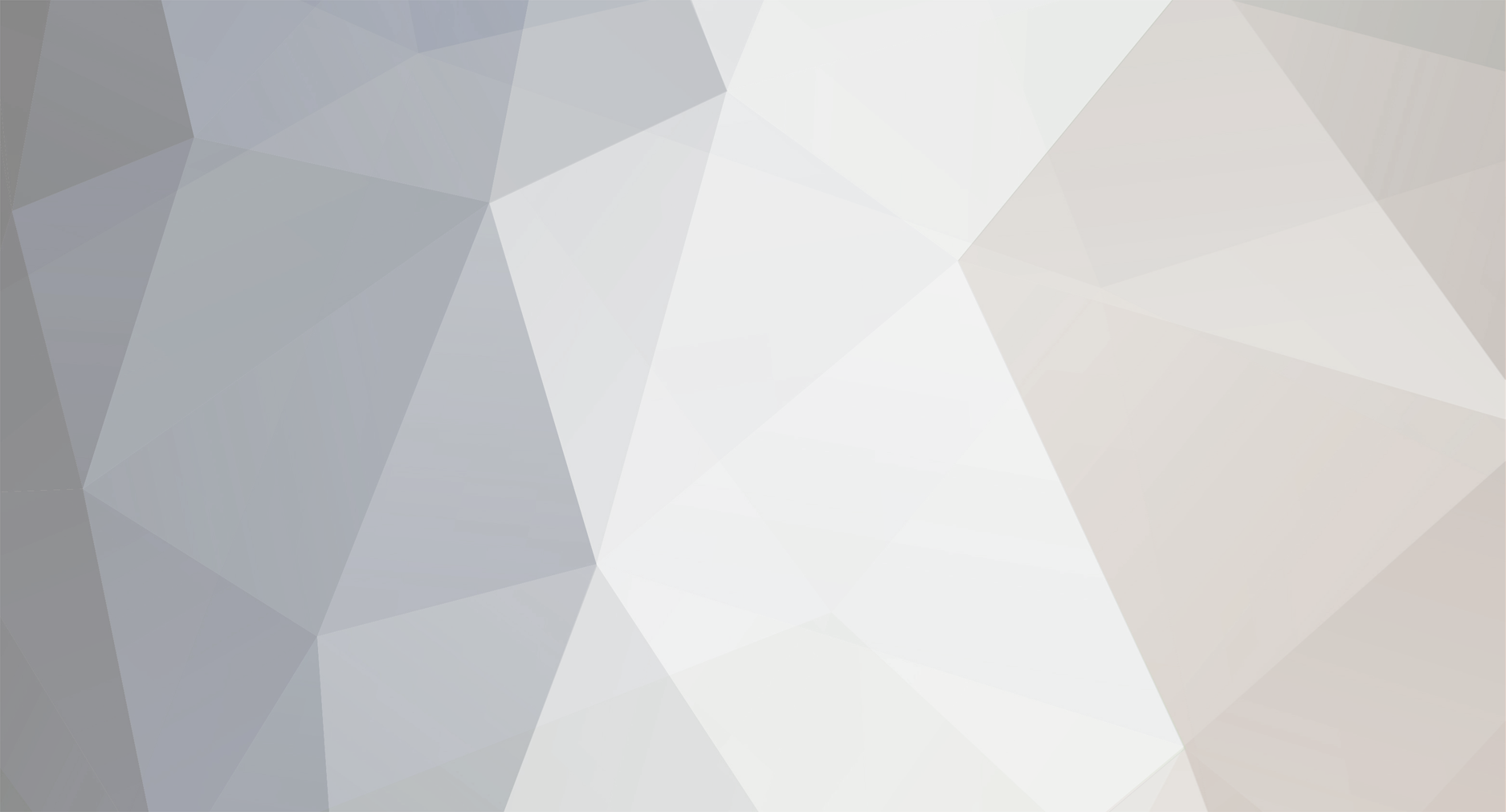
ialsoagree
-
Posts
406 -
Joined
-
Last visited
Posts posted by ialsoagree
-
-
http://www.phpfreaks.com/forums/index.php/topic,293165.msg1387567.html#msg1387567
PHP isn't going to be able to do this for you, you'll probably need javascript or if you only have 2 frames, you can set the target of your link not to a specific frame, but to the parent window and refresh the entire page.
-
ALTER TABLE table_name AUTO_INCREMENT = x
Where x is the new auto_increment value (the 1st number that will be given to the next record).
-
Im kinda new to php and i got the script from a tutorial.
Well, hopefully my response addressed your problem, mysql_select_database isn't a function (unless you define it as one).
However, PHP does have the built in function mysql_select_db:
-
$db = mysql_select_db($dbname, $connection);
Keep in mind that this returns a bool, so $db will either be TRUE or FALSE.
-
It's coming from right here:
print('<form action="grad.php?quesid=$quesid"
I believe you're looking for:
print('<form action="grad.php?quesid='.$quesid.'"
Keep in mind, you're using single quotes for this string, so variables inside single quotes are NOT parsed:
$example_string = 'stuff'; echo 'This is the first example: '.$example_string; // This is the first example: stuff echo 'This is the second example: $example_string'; // This is the second example: $example_string echo "This is the third example: $example_string"; // This is the third example: stuff
-
For stings, you can do things like stripping white space and removing HTML, but mostly you'll just need to escape the string. If you're using a MySQL database and need to query with the product's ID, using mysql_real_escape_string() will help.
If you're using just numbers for your product ID, casting the $_GET['product_id'] as in int, with (int)$_GET['product_id'] will tell PHP to to simplify the string into an integer. If you're only passing numbers then this prevents a potential hacker/user from putting in anything but numbers.
-
if ($orderby==prospect_id)
Should probably be
if ($orderby=='prospect_id')
-
That error means that you don't have a column named date_created in that table, perhaps it's named something else?
-
Give this a go:
DELETE FROM $table WHERE date_created < date_add(CURRENT_DATE, INTERVAL $numdays day)
(Note singular 'day' and not 'days').
The MySQL 5.1 manual entry for date_add:
http://dev.mysql.com/doc/refman/5.1/en/date-and-time-functions.html#function_date-add
-
If you *just* need the number or rows (but not the actual info in the rows themselves) you should use:
SELECT COUNT(*) FROM sent_messages WHERE status = 'unread' AND to_id = '$user->id'
If you need the info in the rows too, your current query will work, you just need to use mysql_num_rows($query) to get the number of results.
-
Have you tried echo mysql_error(); after your $result = line?
Also, you realize that you're doing date_add right? Every date_created is going to be a smaller number than a date in the future (in other words, your script will delete every entry that wasn't created at least $numdays days in the future).
-
You just forgot your else statement is all...
if ($orderby=prospect_id) { // display this query if prospect_id is chosen $prospectEntries = mysql_query("SELECT * from prospects ORDER BY ".mysql_real_escape_string($orderby)." DESC ".$queryLimit) or trigger_error(mysql_error()); } else { // if not prospect_id display other results in ASC order $prospectEntries = mysql_query("SELECT * from prospects ORDER BY ".mysql_real_escape_string($orderby)." ASC ".$queryLimit) or trigger_error(mysql_error()); }
-
This will partly depend on how you send the delete request, but if done correctly, $_POST['checkedItems'] will be unset if nothing is checked.
In other words:
if (isset($_POST['checkedItems'])) { // At least 1 item checked } else { // Nothing checked }
-
(The above solution is a lot more elegant - and probably more efficient too - than mine, I have some comments about using a link instead of a button below though)
How to delete the items, specifically, is going to depend on your database (of course) but I'll do my best to give you something generic (written for MySQL, since that's what I use) you can tailor to your use. Make sure that before you do delete anything, the user requesting the delete actually has permission to delete each of the files requested.
There's nothing to stop a user from building a POST or GET request with any message ID they want to put in (even if the messages don't belong to them) so plan accordingly!
$delete = 'DELETE FROM your_messages_table_name WHERE '; $start = TRUE; $i=0; foreach ($_POST['checkedItems'] as $value) { if (!$start) $delete .= ' OR '; else $start = FALSE; $delete .= 'message_id_column = '.$value; $i++ } $delete .= ' LIMIT '.$i; // Delete is now ready to run on your server, but you should make sure // that the user actually has permission to delete each of the ID's they're trying to delete!
As for the issue of using a link instead of a button. This is a javascript issue really. There's a few ways you could do this. I'd recommend this...
<input name="checkedItems[]" id="message_check_'.$r['unique_database_id'].'" type="checkbox" value="'.$r['unique_database_id'].'" />
Have your delete url look something like...
<a onClick="deleteMessages()" style="cursor:pointer;">Delete Checked Messages</a>
(A quick note, cursor:pointer may not work on IE, you may have to use cursor:hand in addition to cursor:pointer, or another style).
(I'm suddenly getting really lazy because I've been writing this post for about 10-15 minutes, so here's a quick note about what you need to do, and if you need specific examples than hopefully someone can help you, or I can continue later).
In your loop where you pull each message row, you need to create a series of if then javascript statements that checks each individual checkbox (they each have unique ID's, so you can pull their ID's using getElementById) to see if it is checked or unchecked (and if it's checked, to build a string that will take the user to a delete page and sends the ID's in checkedItems[], the request can be POST or GET, just change the PHP above from $_POST to $_GET if you switch to GET).
Then outside your loop, you have to put that javascript statement you made into the deleteMessages javascript function that builds the GET or POST request and sends it for the user.
-
I have a message system that i have just made on my site. when the user clicks on their message in their inbox it takes them to inbox.php?id=the id of the message, and this is displayed through the (isset($_GET['id'])) method. But whats to stop the user typing into the address bar inbox.php?id=22 or whatever. This would allow the user to see messages sent to and from other members. all they hve to do is get lucky with the id. Is there anyway to get around this?
There's nothing to stop a user from typing in any url they want. Since you can't prevent that, you need to code accordingly. Instead of just displaying the message if someone requests it, display the message only when it's requested by one of the message's recipients, or the person who sent the message.
In other words, before you display the message, check to see if the user requesting the message is one of the people the message was to, or the person who sent the message. If it's not, display some kind of error, warn the user to stop trying to abuse the system, forward them to an index page, or whatever else you feel is appropriate.
-
Using require is essentially copying and pasting the contents of a file to where the require statement is. The difference between require and include is that a require will cause the script to fail if it can't access the file, an include will not (although it may still throw an error).
In any event, if you need access to the variable that lets you connect to the database, you don't need to include the entire database connection script, you just need to define the database connection variable outside of any blocks (so it's not scoped to a function, class, or any other block structure) and then include it in any blocked structures (like your Tracker methods) using the key word global and then the variable name.
For example:
// some php file requested by the user, say, index.php: require('database.php'); $db = new database_connection(); require('tracker.php');
// tracker.php: class Tracker { var $ip_address; var $time; function Logger($ip_address) { global $db; // etc. } function another_function() { global $db; // function stuff here } // etc.
-
Try...
$output_string = ''; $start = TRUE; foreach ($_SESSION['items'] as $key => $value) { if (!$start) $output_string .= ', '; else $start = FALSE; $output_string .= $key; } // Do whatever you want with $output_string
-
What code is in mysqli.php?
-
From what I'm gathering, the problem is that the header function doesn't work because you've already sent HTML to the browser. It's usually good practice to buffer all your output, and then when your script is done to send all the output back to the user. This helps you avoid situations like this one, where you've started preparing output but suddenly you need to change what you're doing but it's already too late.
There's 2 methods to do this, I personally like to use objects that store all the page's HTML as the PHP script executes, and then when I'm done, a method in the object outputs the HTML to the browser and ends the script.
But, another method is to use PHP's output buffering functions. When you start output buffering, which is as simple as calling a single function, PHP saves everything you tell your script to echo until you turn output buffering off at which point it sends everything you've echoed to the browser.
The nice part is that, header's aren't buffered, so you can still send headers, even after you've used echoes to send output.
The output buffering manual is here (in english):
http://php.net/manual/en/book.outcontrol.php
The specific functions you're looking for are:
http://www.php.net/manual/en/function.ob-start.php
and
-
In order to delete all the checked rows, you need to know which items are checked. This is your current checkbox (which is the same for every message because it's used in the while loop):
<input name="chkSample" type="checkbox" value="" />
The problem here is that every checkbox has the same name, and has no value. In order to be able to distinguish which checkboxes are checked, I'd recommend changing your checkbox code to this:
<input name="checkedItems[]" type="checkbox" value="'.$r['unique_database_id'].'" />
Obviously you need to change 'unique_database_id' to whatever the column that identifies each message uniquely is.
The list of checked items will be returned as an array in $_POST like so:
foreach ($_POST['checkedItems'] as $value) { echo $value; // Echoes the unique ID of each checked message }
-
I'm not sure I'm totally understanding what you're asking.
That function you posted seems like a great method for determining if the page exists (and I used the wrong _GET index, sorry about that).
From what you're saying, I think the problem is that you have a script that writes a generic header and you need to modify that header if you're displaying an error page. Is that what you're asking?
OR, are you saying that you can't use the header() function because information was already sent to the browser before?
-
This code didnt work.
Is there anything specific that didn't work.
-
switch ($_GET['mode']) { case 'home': // Do home stuff here break; case 'whatever': // Do a different page here break; case 'error': // Show your error page here break; default: header('Location: ./sw.php?mode=error'); // Sending a header does NOT end the script from processing, if you don't want the script to continue, you should use die() or exit break; }
Untested, but I believe this should work.
-
Okay so.. welcome to you for 1 year 3 months ago.
Haha, thanks!
Simple file upload problem
in PHP Coding Help
Posted
Try changing:
<Input type="file" name ="file" value="Browse" id="Ufile" style="display:none">
to:
<Input type="file" name ="file" id="Ufile" style="display:none">