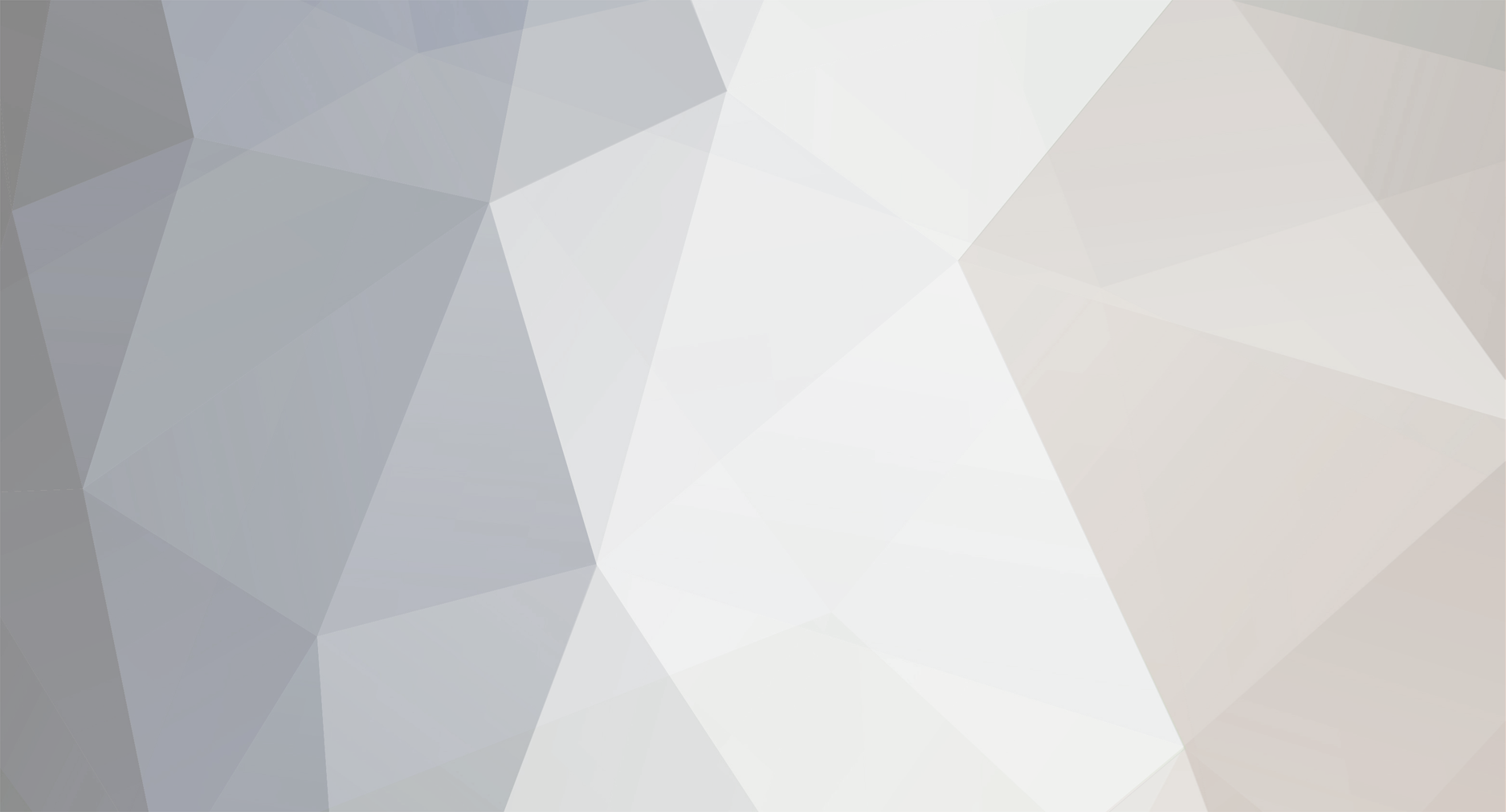
Xinil
Members-
Posts
15 -
Joined
-
Last visited
Never
Everything posted by Xinil
-
redirecting first part of a form to anotherpage and a follow up form
Xinil replied to frankstr's topic in PHP Coding Help
Store the first part of the form in hidden form fields. For example, if your first form has name="first_name" and name="last_name". Then you submit this form to go to the second one...now you'd have name="mysecondform", and name="mysecondform_option2" WITH name="first_name" type="hidden", and name="last_name" type="hidden" Now you can just submit through Post again without any problems. -
The only browsers that don't support AJAX are the ones that has Javascript disabled.
-
erikjan, performing a simple AJAX statement would be much, much less painful than storing all that information in a text file (why on earth?). You don't want to be storing any of this sensitive ORDER information anywhere that isn't secure (anywhere that isn't a database). Sessions aren't reliable and neither are cookies. I'll help you out some more. http://docs.jquery.com/Ajax#.24.get.28_url.2C_params.2C_callback_.29 Use that to call the AJAX code. Then the page you called can execute the e-mail to the user. On the callback function, do a simple javascript: document.formname.submit().
-
The difference between one huge file and one file with many includes isn't in execution time or performance, but rather security and usability. One huge file is very easy on security. If you're checking sessions, simply check the session in whatever part of the file you want. However, if you're using multiple files, you have to check session data on the included file. This is necessary because what if some user somehow finds that included file on your server? Now they can access it in a way that it wasn't originally intended (i.e. they go to menu.php instead of index.php). Authentication control is necessary on an include setup (each and every page needs it). Another issue with giant files is upload time. If you're uploading a 150Kb file and someone is trying to view the page while you're uploading it, they'll get an error. Smaller pages with includes fix this error. Smaller pages with includes also prevent bugging errors from crashing your whole site. If you include a file that has an error on it, only that page will error when called, not the whole site. So, it's really a give and take. includes = some security risk, but greater flexibility overall. Having a massive "functions.php" is perfectly normal. I don't know why anyone wouldn't have one of those. I'm referring to a whole website setup tough.
-
How would I display results in multi-column table?
Xinil replied to simcoweb's topic in PHP Coding Help
Of course, simcoweb. Just change the totalColumns to 3 and everything will work the exact same. -
How would I display results in multi-column table?
Xinil replied to simcoweb's topic in PHP Coding Help
Quick write up: $totalColumns = 5; $i = 1; echo '<table border="1">'; for ($b=0;$b<15;$b++) { if ($i == 1) echo '<tr>'; echo '<td>'; echo 'item' ,$i; echo '</td>'; if ($i == $totalColumns) { echo '</tr>'; $i = 0; } $i++; } echo '</table>'; -
You can't redirect to b.php, because then you'll lose the form $_POST data. Oh, I think I understand what you're saying. You want the page to still submit to b.php, but also send an e-mail? Not easy unfortunately, the problem is that once you submit the form, you've lost control over the user until he goes back to that form page...but you're saying you dont' have access to b.php. Soo, the only solution I can think of is AJAX. When a user clicks "submit form 2", you can have AJAX call a separate page (say c.php) and this will send an e-mail. Once AJAX has returned with a successful value (the page ran successfully), submit the form. AJAX isn't hard to learn if you use jQuery ( www.jquery.com ). It's not a beginner job though, so if you can't figure out this solution, you're pretty much out of luck (unless you want to make two submit pages, one to submit the e-mail, and then the second submits the actual form to b.php). Or, you could alternatively make a pop-up that submits an e-mail (but it pop-up blockers are enabled that won't work). So, good luck with AJAX.
-
Use your super globals. $_SERVER['DOCUMENT_ROOT'] This will never change and will always point to your root directory. Echo it out to see where it points and then change the location for each file as needed. Example: require($_SERVER['DOCUMENT_ROOT'].'/includes/myfunctions.inc.php');
-
You shouldn't do it that way then. Make it one form. Then you have two submit buttons. One with name="submit1", the other with name="submit2". Then you just add this to the page: if (isset($_POST['submit1'])) { // submit my mail() } if (isset($_POST['submit2'])) { // don't submit mail }
-
I have a project for a client that wants me to store PDF files in a zip file (5.2 now comes with zip libraries embedded). I've got the zip libraries to work, but for some reason when I create the zip file, I get a gibberish string appended to the end of the file name. Example, when I execute this code: $zip = new ZipArchive; $res = $zip->open('test.zip', ZipArchive::CREATE); if ($res === TRUE) { $zip->addFile('testfilephp.txt', 'newname.txt'); $zip->close(); echo 'ok'; } else { echo 'failed'; } When that's executed, for some reason, two files are created named: test.zip.a03440, and test.zip.b03440. If I rename those files to test.zip, I can read the archives just fine. I don't understand why it's creating this weird code on the end of the file name, any help is appreciated.
-
You aren't specifying a "where clause" in your updates. Each update you're performing is overwriting every row in the database. Example: if you have 5 rows in the table, and you perform an update WITHOUT supplying a "where" clause, each row is updated with the information provided. However, you are saying "LIMIT 1" which means only update the first row out of all rows (not the row you want it to find). so. your $do should be: $do = mysql_query("UPDATE Users SET price = '$price' WHERE username='$current' LIMIT 1") or die(mysql_error()); You're also performing 3 updates when it could just be one. Like this: $do = mysql_query("UPDATE Users SET price = '$price',area='$area',description='$description' WHERE username='$current' LIMIT 1") or die(mysql_error()); Enjoy~
-
you're already pointing the forms to different pages. Assuming you aren't nesting the forms, then they'll be submitting to "a.php" and "b.php". So long as both pages don't use the mail() function, only one page will send the mail. If you click on "submit" under Form 1, then it will submit a.php (and any code written on a.php will be executed). If you click on "submit" under Form 2, then it will run code for b.php. So, it depends on whatever you write for those two files. Action="whatever.php" is the key.
-
If you're trying to explode a string into an array, you do this: $myString = "1,2,3,4"; $newArray = explode(",",$myString); foreach ($newArray as $key => $value) { echo $value, ' - '; } Hope that makes sense.
-
Simple. Store the game data in a table in a MySQL database. Basically you have the user log in, and the other user log in. When they create a game, a row is entered into the database with this game information. So long as their session is active, it will authenticate against this row and any relevant game data should point/update it or other tables. Sessions typically should not be used to authenticate anything other than login information. Any other sensitive information should be stored elsewhere and beyond a client's control.
-
Hotlinking explained: http://en.wikipedia.org/wiki/Hot_linking Google is your friend. Now that you know this. Google for something similar to: "prevent hot linking with htaccess".