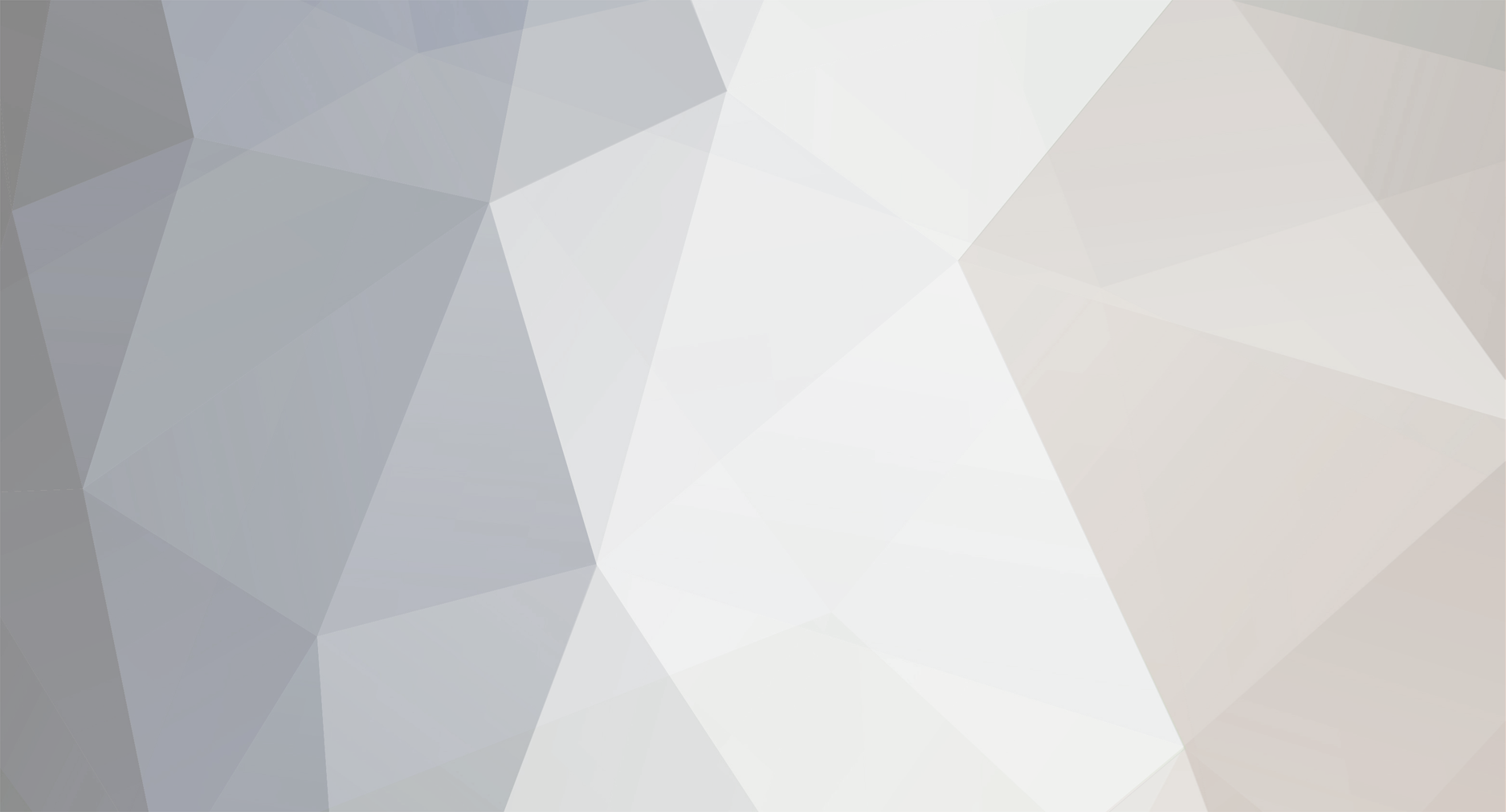
raytri
Members-
Posts
48 -
Joined
-
Last visited
Never
Everything posted by raytri
-
I was afraid of that. I'm going to mess around with some other ideas, but if I have to enter the months manually, I will. Thanks for all your help!
-
I tried the CONCAT approach, but couldn't get it to work. Again, I'm trying to do this totally in MySQL, without reference to PHP or anything like that. I want to be able to do this in direct queries against the database, not have to run it through a server-side script.
-
Hmm. The link helped me get the query to work, but only by manually plugging in a SUM(IF()) statement for each month: SELECT Account, SUM(IF(`Period Ending`='2009-12-31',`No_ Trans`,0)) As Dec09, SUM(IF(`Period Ending`='2010-01-31',`No_ Trans`,0)) As Jan10, SUM(IF(`Period Ending`='2010-03-31',`No_ Trans`,0)) As Mar10 FROM Merchant GROUP BY Account There are quite a few months, with another being added every month. So is there a way to have the query do this dynamically? (create a column for each month in the table, and have each row show the number of transactions for that account in each month).
-
Wow. What a great link! I think that solves my problem.... I'll let you know when I try to adapt it to my situation.
-
Good suggestion, but I'm just trying to pull a report. I don't want to run it through PHP. Plus I want to learn how to do this in MySQL.
-
I've been reading up on ways to turn columns into rows, but I'm just not able to turn it into working code. I have a table with data that looks like this: Account Month Transactions 100 May 2009 200 100 June 2009 180 101 May 2009 50 101 June 2009 80 101 July 2009 68 102 June 2009 400 Yes, I know this should be broken up into related tables. But this is what I have to work with. I want to pull a report that displays the data so there is one line per account, and a column for each distinct month in the database, like so: Account May 2009 June 2009 July 2009 100 200 180 0 101 50 80 68 102 0 400 0 There are a lot of months, so I'd like the query to identify each distinct month, rather than me hardcoding it into the query. I've looked at pivot tables, inner joins, etc., but I'm just not grokking what I need to do to break out the months into columns.
-
I'm just starting to explore custom error-handling routines. I've written the following, and it works. The idea is to make sure error messages aren't show to the user unless there is a fatal error, and then it's a custom message. Meanwhile, the detailed error message is e-mailed to me, both for fatal errors and warnings. Is this a good approach for error-handling code? Or am I missing something big? (I know there's some redundancy, like I could combine two of the "if" statements in the customError() function, and I'm pretty sure that any error capable of causing a shutdown is by definition serious, so the "if" statement in the fatalErrorShutdownHandler() function is unnecessary. But is the basic structure sound?) <?php //Don't show errors to user ini_set('display_errors', 0); //Trigger customer error function when a fatal error aborts the script register_shutdown_function('fatalErrorShutdownHandler'); // the function function customError($errno, $errstr, $errfile, $errline) { //For fatal errors, display custom message if($errno < 4) {ob_end_clean(); ?> <h1>Error loading page</h1> <p>The website encountered an error loading your page. The Website administrator has been notified; please try reloading the page. If that doesn't work, please try again later.</p> <p>If the error persists, please notify us at <a href="info@example.net">info@example.net</a></p> <?php } //Report both fatal errors and warnings if($errno < { //Include full URI of page that triggered the error $badpage = $_SERVER['REQUEST_URI']; error_log("Error: [$errno] $errstr in $errfile on line $errline. \n\n URI is $badpage",1, "webmaster@example.net","From: webmaster@example.net");} //If it's a fatal error, kill the rest of the page if($errno < 4) {die();} } //Triggers error reporting in the event of a fatal error shutdown function fatalErrorShutdownHandler() { //gets error that caused the shutdown $error = error_get_last(); //Only sends error message for serious errors if ($error['type'] < { customError($error['type'], $error['message'], $error['file'], $error['line']); } } //sets custom error handler for non-fatal errors set_error_handler("customError"); //buffer page output so errors can be caught before anything is output to page ob_start(); ?>
-
I have a page that lets visitors pick up to three machines from a checklist, and then generate a table comparing the features of those machines. The SQL query for the table is assembled thusly: <?php //capture ids of selected machines $atmID = $_POST['atmid']; //Assemble query variable (makes sure query works even if fewer than three machines selected), and count number of machines to get table column count $atmlist = $atmID[0]; $comparecount = 2; if($atmID[1]) {$atmlist .= ",".$atmID[1]; $comparecount ++;} if($atmID[2]) {$atmlist .= ",".$atmID[2]; $comparecount ++;} //Query for assembling comparison chart $selectSQL = "SELECT * FROM ATMcompare WHERE `atmID` IN($atmlist)"; $selectQuery = mysql_query($selectSQL); ?> So far so good. If I fetch the array from the above query, I'll have an array containing up to three subarrays, one for each machine selected. But I can't figure out how to echo the subarray values directly to a table, something like this: <?php $selectArray = mysql_fetch_array($selectQuery); ?> <tr><td><p><strong>ATM model</strong></p></td><td><?php echo $selectArray[0][1]; ?></td><td><?php echo $selectArray[1][1]; ?></td><td><?php echo $selectArray[2][1]; ?></td></tr> I've made the page work, but only by using a while statement to loop through the array for each feature, and rewinding the pointer with mysql_data_seek after each loop: <tr><td><p><strong>ATM model</strong></p></td> <?php while ($item = mysql_fetch_array($selectQuery)) { echo "<td><p><strong>".$item['Maker']." ".$item['Model']."</strong></p></td>"; } mysql_data_seek($selectQuery,0); ?> </tr> I prefer the first approach because it makes for cleaner code, appears to involve a lot less processing, and would prove I understand array structures. Any tips?
-
I figured it out. The include file containing my database login information had some legacy code intended to prevent SQL injections in POST and GET variables: //This stops SQL Injection in POST vars foreach ($_POST as $key => $value) { @$_POST[$key] = mysql_real_escape_string($value); } //This stops SQL Injection in GET vars foreach ($_GET as $key => $value) { @$_GET[$key] = mysql_real_escape_string($value); } I removed that, and now it works fine.
-
You're right; when I place the raw form on its own page and submit it to itself, it works just fine. Now I have to figure out why it doesn't work on the given page. Thanks, and if you've got any ideas as to what could cause that to break, I'm all ears.
-
Here's the div containing the generated checkbox form. Is that what you need? div><h1 class="welcomeh1" style="margin-top:20px;">ATM machines</h1> <p class="maincontentbig">Browse our selection of ATMs. You can view the entire catalog or sort by whatever option you choose.</p> <div class="formbox" style="width:130px; float:left;"> <p><strong>Choose up to three machines:</strong></p> <form method="post" action="atmcompare.php"> <p><input type="checkbox" name="atmid[]" value="6" /> Hyosung 5000CE</p> <p><input type="checkbox" name="atmid[]" value="7" /> Hyosung 1500</p> <p><input type="checkbox" name="atmid[]" value="8" /> Hyosung 1800</p> <p><input type="checkbox" name="atmid[]" value="9" /> Hyosung 2100T</p> <p><input type="checkbox" name="atmid[]" value="15" /> Hyosung 1800CE</p> <p><input type="checkbox" name="atmid[]" value="1" /> Tranax 1700/1705W</p> <p><input type="checkbox" name="atmid[]" value="2" /> Tranax 4000</p> <p><input type="checkbox" name="atmid[]" value="3" /> Triton RL1600</p> <p><input type="checkbox" name="atmid[]" value="4" /> Triton RL5000</p> <p><input type="checkbox" name="atmid[]" value="5" /> Triton RT2000</p> <p><input type="checkbox" name="atmid[]" value="14" /> Triton RL2000</p> <p><input type="checkbox" name="atmid[]" value="10" /> WRG Genesis</p> <p><input type="checkbox" name="atmid[]" value="11" /> WRG Genesis LT</p> <p><input type="checkbox" name="atmid[]" value="12" /> WRG Apollo</p> <p><input type="checkbox" name="atmid[]" value="13" /> WRG Apollo LT</p> <p style="margin-top:6px;"><input type="submit" name="submit" value="Submit" style="width:70px;" /></p> </form> </div>
-
Hi, I've read several tutorials and read through several threads here, but I can't seem to make this work. I'm putting together an interactive feature-comparison chart for products. Basically, people can check up to three machines they want to compare, hit "submit", and then the specs for the chosen machine(s) will be displayed. I've got the checkboxes being generated dynamically from the product database. It works just fine: form method="post" action="machinecompare.php"> <?php while($item = mysql_fetch_array($sqlBox)) {?> <p><input type="checkbox" name="machineid[]" value="<?php echo $item['machineID']; ?>" /> <?php echo $item['Maker']." ".$item['Model']; ?></p> <?php } ?> <p><input type="submit" name="submit" value="Submit" /></p> </form> For the form processing, I have this: $machineID = $_POST['machineid']; Which supposedly provides an array containing the values of the checked checkboxes. But when I print_r the $_POST, I get this: Array ( [machineid] => [submit] => Submit ) So apparently all it's passing is the fact that the form was submitted. If I take the array brackets off of the checkbox name, I get what you'd expect: The data is passed properly, but only for the last item checked. Array ( [machineid] => 4 [submit] => Submit ) Everything I've read suggests this should be fairly simple and automatic. So besides simple cluelessness on my part, I'm wondering if there might be something else I'm overlooking -- like a php.ini setting that causes trouble when trying to pass array data through a POST function.
-
Thanks; that "page-break-after" was what I was looking for. Typical; what I think is a PHP problem is actually a CSS problem.
-
I'm looking for pointers here, not necessarily detailed code examples. I want to solve this one myself as much as possible. I have an "online statement" site that displays account activity for customers. I have an associated "print.css" file so it can be printed out in a nice, readable format. Some of our customers have multiple accounts (one guy has around 80). For customers with multiple accounts, I have a summary display that adds up all their account activity. Good as far as it goes. But some of our customers want to be able to print out and file the statement for each individual account (at a minimum, they need to do this at tax time). The guy with 80 accounts, understandably, doesn't want to have to manually display and print 80 pages. Is there a way that I could put a "print all" button on the page, that when pressed lets the user print out statements for all of their accounts, using the format established by print.css? Ideally without having to display each page, or hit "print" in a dialog box 80 times? I was contemplating trying to write a loop that, for each of the 80 queries, would write the results into a non-viewed HTML shell, then add each page to a PDF using print.css, then give the user the print dialog box in order to print the PDF (or allow them to save the PDF to their desktop). But I'd appreciate ideas. Thanks!
-
No. I was looking at what you wrote in terms of MYSQL syntax. I'm relatively new to it, so I was thinking two things: 1. You could pull the data once and then manipulate it as a PHP array, but the code would be more complicated than just pulling it already randomly sorted from the database. I was trying to keep the code as simple as possible. 2. UNION ALL is the only way I know to make multiple passes of the same query in MYSQL, short of putting the query inside a loop or writing the query five times. Is there another way to have MYSQL pull five copies of the same query results?
-
Except that he wants to take those 12 images and repeat each image 5 times (for a total of 60 images), and have those 60 images display randomly. So he either needs to pull the data five times in MYSQL, or pull it once, duplicate the array four times in PHP, merge those into one combined array, shuffle it and then loop through it. I imagine the latter is more efficient (only one database query instead of five), but the code would be more complicated.
-
You could put the image names into a MYSQL table, and then query it five times using UNION ALL and ORDER BY RAND(): $picturequery=mysql_query("SELECT * from Pictures UNION ALL SELECT * from Pictures UNION ALL SELECT * from Pictures UNION ALL SELECT * from Pictures UNION ALL SELECT * from Pictures ORDER BY RAND()"); while ($picturearray=mysql_fetch_array($picturequery)) {echo $picturearray['PictureName'];} You can probably do the same thing more elegantly using joins, but I'm still trying to wrap my head around join syntax.
-
A (not too) manual workaround would be to simply define $page at the top of each page. So on index.php, you'd write $page = '/index.php'; Also, what do you do if you have subdirectories with their own "index.php" files?
-
The OP needs to be clearer. He's mentioned only letting them pray once per id per session, and once per id per day. The former you can do with session variables; the latter would seem to require updating the database. The mechanism ought to be similar though, using oni-kun's example. You're either writing a comma-delimited series of ids to a session variable, or you're writing them to a database cell. Then whenever they click pray, you check to see if the id has already been prayed for. Or you could be proactive, and only display the "pray" button next to ids that haven't already been prayed for. If doing the "once a day" thing, you'll also need to figure out how you want to delete the ids from the database so that a person can pray again the next day. If you want to keep it simple, just run a cron job that deletes the contents of that cell at midnight every day. If you don't like cron jobs, you could write some code that checks the time whenever the user logs in. If its the first time the user has logged in that day, erase the contents of the "already prayed for" cell.
-
Doh. Getting my PHP and Javascript mixed up.
-
Try moving the server() function up, so it's above the function call. PHP has to know about the function before it can call it.
-
Hmm. Sorry to say, I'm not sure why that's not working. I'm either missing something, or there are errors in the page elsewhere that are messing this up. Let's try a different route: 1. Delete the "redirect" line. 2. Add the following to the bottom of your send.php file: header ('Location: http://hexagon.jlkfree.com/kontakt.php?sent=sent');
-
You're welcome! Glad it worked for you.