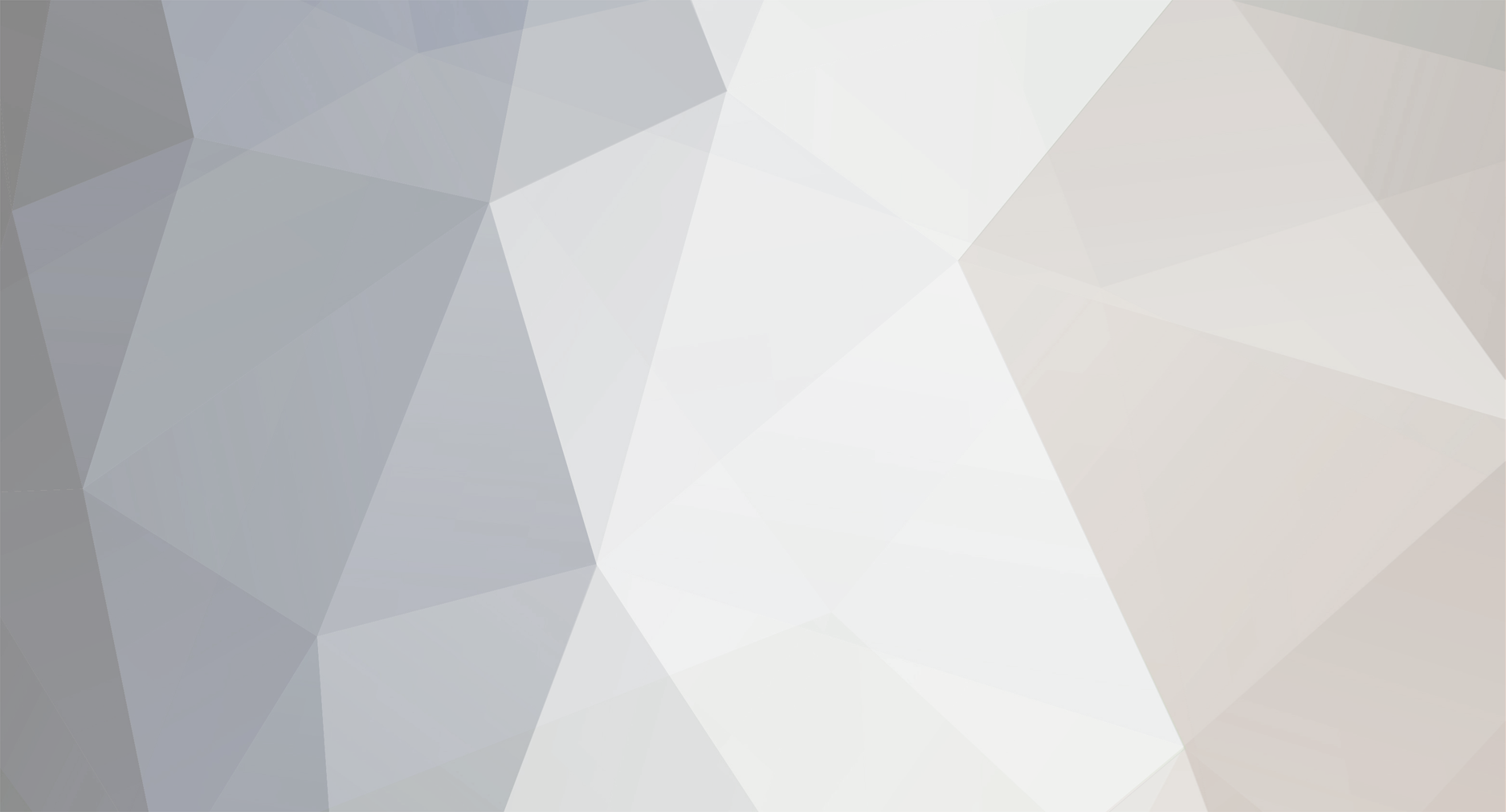
kickstart
-
Posts
2,707 -
Joined
-
Last visited
Posts posted by kickstart
-
-
Hi
I cannot think of a way of doing this in a single SQL statement. In your other thread I suggested a few other options, and I would encourage you to stop them posting in the first place rather than just suppressing their posts.
However, try this. All it is doing is building up a list of like clauses for the SQL for the excluded words (I have assumed a table of excluded words). This will be OK for a limited number of words, but if the number gets too many it will cause problems (ie, not 100% sure on MySQL, but some versions of SQL have a limit of ~255 where clauses). Also take care with the contents of the excluded words table as I have put nothing in there to prevent a potential sql injection attack.
<?php $sql = "SELECT excluded_word FROM `word_exclusion_table`"; $result = mysql_query($sql); $whereclause = ""; while ($row = mysql_fetch_assoc($result)){ $whereclause .= (($whereclause) ? ' AND ' : ' WHERE ')."title NOT LIKE '%".$row['excluded_word']."%' "; } //time to show you what you have in the database $sql = "SELECT * FROM `test_table` $whereclause ORDER BY `id` DESC LIMIT 5000;"; $result = mysql_query($sql); print " <table border=\"0\">\n"; while ($row = mysql_fetch_assoc($result)){ $title = $row['title']; $url = $row['url']; echo " <tr> <td><a href=\"$url\" target=\"http://www.freefreeads.com\">$title</a></td> </tr> "; } print " </table>\n"; ?>
All the best
Keith
-
Hi
First thing I would do is to trap the IP addresses of the posters. And then block those belonging to the spammers. Likely that if it is a bot then it is just returning the form fields, so change the names of the form fields (or if you want a bit of fun, do this dynamically, maybe based on the day of the week), which will make them have to change their script to work again.
To block the words you could compare them either with a LIKE in SQL or using a regular expression in php, dropping any record from being displayed when it contains a banned word. Probably easiest to store the list of banned words in a table, then loop through them and building up a regular expresssion string to use to checking against the posts (or you could check each one as you loop through them using strpos).
All the best
Keith
-
Hi
Have they sent you the script as well?
All the best
Keith
-
Hi
That code is looping through all the return fields when the form is submitted, and if the name starts frm_ then it uses a case statement to decide which field it is and so how to validate it.
All the best
Keith
-
Hi
Had a bit more of a play and tried test running it this time.
<?php require "auth.php"; require "config1.php"; ?> <h3>Welcome <?php echo $_SESSION['SESS_FIRST_NAME'], " ".$_SESSION['SESS_LAST_NAME'];?></h3> please select your times were you are available <html> <head> <title>My Page</title> </head> <body> <form method="post" action="<?php echo $PHP_SELF;?>"> <div align="left"><br> 9-12 Monday<input type="checkbox" name="912Monday" value="Whatever"><br> 12-4 Monday<input type="checkbox" name="124Monday" value="Whatever"><br> 9-12 Tuesday<input type="checkbox" name="912Tuesday" value="Whatever"><br> 12-4 Tuesday<input type="checkbox" name="124Tuesday" value="Whatever"><br> 9-12 Wednesday<input type="checkbox" name="912Wednesday" value="Whatever"><br> 12-4 Wednesday<input type="checkbox" name="124Wednesday" value="Whatever"><br> 9-12 Thursday<input type="checkbox" name="912Thursday" value="Whatever"><br> 12-4 Thursday<input type="checkbox" name="124Thursday" value="Whatever"><br> 9-12 Friday<input type="checkbox" name="912Friday" value="Whatever"><br> 12-4 Friday<input type="checkbox" name="124Friday" value="Whatever"><br> <input type="submit" value="submit" name="submit"> </form> <br> </div> </form> </body> </html> <?php $WhatToInsert = array(); $WhatToInsert['912Monday'] = array('\'9-10\',\'mon\'','\'10-11\',\'mon\'','\'11-12\',\'mon\''); $WhatToInsert['912Tuesday'] = array('\'9-10\',\'tue\'','\'10-11\',\'tue\'','\'11-12\',\'tue\''); $WhatToInsert['912Wednesday'] = array('\'9-10\',\'wed\'','\'10-11\',\'wed\'','\'11-12\',\'wed\''); $WhatToInsert['912Thursday'] = array('\'9-10\',\'thu\'','\'10-11\',\'thu\'','\'11-12\',\'thu\''); $WhatToInsert['912Friday'] = array('\'9-10\',\'fri\'','\'10-11\',\'fri\'','\'11-12\',\'fri\''); $WhatToInsert['124Monday'] = array('\'12-1\',\'mon\'','\'1-2\',\'mon\'','\'2-3\',\'mon\'','\'3-4\',\'mon\''); $WhatToInsert['124Tuesday'] = array('\'12-1\',\'tue\'','\'1-2\',\'tue\'','\'2-3\',\'tue\'','\'3-4\',\'tue\''); $WhatToInsert['124Wednesday'] = array('\'12-1\',\'wed\'','\'1-2\',\'wed\'','\'2-3\',\'wed\'','\'3-4\',\'wed\''); $WhatToInsert['124Thursday'] = array('\'12-1\',\'thu\'','\'1-2\',\'thu\'','\'2-3\',\'thu\'','\'3-4\',\'thu\''); $WhatToInsert['124Friday'] = array('\'12-1\',\'fri\'','\'1-2\',\'fri\'','\'2-3\',\'fri\'','\'3-4\',\'fri\''); $sql1 = "Insert Into test2 (Marker_ID, Timeslot, Days) Values "; $sql2 = ""; foreach ($WhatToInsert as $key => $value) { if (isset($_REQUEST[$key])) { foreach ($WhatToInsert[$key] as $value2) { $sql2 .= (($sql2) ? ',(' : '(')."'" .$_SESSION['login'] . "',$value2)"; } } } $sql = (($sql2) ? $sql1.$sql2 : ""); echo $sql; ?>
This should work, but you will need to change the echo of the $sql string to insteadconnect to the database and execute it.
All the best
Keith
-
Hi
The page looks like a pretty simple table with a few links at the top, and buttons used to change the sort order (no doubt it just just uses an alternative order clause on the SQL Select statement). It is probably a pretty simple ASP script and should be pretty easy to convert to ASP.
I presume the data on the page comes from a database. Any idea what database?
All the best
Keith
-
Hi
A 2 dimensional array just provides a convenient way to store the various 1 hour time slots for each of the longer time slots. The example code I gave you above should provide the basis of it. You need to declare the array and decode the input fields to decide on the value of $TickedCheckBox (the example assumes a single value for this, but you could easily loop through several selected check boxes).
In a bit more detail, to set up the insert statement to add all the individual records. This assumes that the check boxes on screen are named "912Monday", "124Monday", "912Tuesday", etc, and that the markerid is numeric and stored in $Markerid.
$WhatToInsert = array(); $WhatToInsert['912Monday'] = array(); $WhatToInsert['912Monday'][0] = '\'9-10\',\'mon\''; $WhatToInsert['912Monday'][1] = '\'10-11\',\'mon\''; $WhatToInsert['912Monday'][2] = '\'11-12\',\'mon\''; $WhatToInsert['912Tuesday'] = array(); $WhatToInsert['912Tuesday'][0] = '\'9-10\',\'tue\''; $WhatToInsert['912Tuesday'][1] = '\'10-11\',\'tue\''; $WhatToInsert['912Tuesday'][2] = '\'11-12\',\'tue\''; $WhatToInsert['912Wednesday'] = array(); $WhatToInsert['912Wednesday'][0] = '\'9-10\',\'wed\''; $WhatToInsert['912Wednesday'][1] = '\'10-11\',\'wed\''; $WhatToInsert['912Wednesday'][2] = '\'11-12\',\'wed\''; $WhatToInsert['912Thursday'] = array(); $WhatToInsert['912Thursday'][0] = '\'9-10\',\'thu\''; $WhatToInsert['912Thursday'][1] = '\'10-11\',\'thu\''; $WhatToInsert['912Thursday'][2] = '\'11-12\',\'thu\''; $WhatToInsert['912Friday'] = array(); $WhatToInsert['912Friday'][0] = '\'9-10\',\'fri\''; $WhatToInsert['912Friday'][1] = '\'10-11\',\'fri\''; $WhatToInsert['912Friday'][2] = '\'11-12\',\'fri\''; $WhatToInsert['124Monday'] = array(); $WhatToInsert['124Monday'][0] = '\'9-10\',\'mon\''; $WhatToInsert['124Monday'][1] = '\'10-11\',\'mon\''; $WhatToInsert['124Monday'][2] = '\'11-12\',\'mon\''; $WhatToInsert['124Tuesday'] = array(); $WhatToInsert['124Tuesday'][0] = '\'9-10\',\'tue\''; $WhatToInsert['124Tuesday'][1] = '\'10-11\',\'tue\''; $WhatToInsert['124Tuesday'][2] = '\'11-12\',\'tue\''; $WhatToInsert['124Wednesday'] = array(); $WhatToInsert['124Wednesday'][0] = '\'9-10\',\'wed\''; $WhatToInsert['124Wednesday'][1] = '\'10-11\',\'wed\''; $WhatToInsert['124Wednesday'][2] = '\'11-12\',\'wed\''; $WhatToInsert['124Thursday'] = array(); $WhatToInsert['124Thursday'][0] = '\'9-10\',\'thu\''; $WhatToInsert['124Thursday'][1] = '\'10-11\',\'thu\''; $WhatToInsert['124Thursday'][2] = '\'11-12\',\'thu\''; $WhatToInsert['124Friday'] = array(); $WhatToInsert['124Friday'][0] = '\'9-10\',\'fri\''; $WhatToInsert['124Friday'][1] = '\'10-11\',\'fri\''; $WhatToInsert['124Friday'][2] = '\'11-12\',\'fri\''; $sql1 = "Insert Into Appointments (Markerid, Time, day) Values "; $sql2 = ""; foreach ($WhatToInsert as $key => $value) { if (isset($_REQUEST[$key]) { foreach ($WhatToInsert[$key) as $value2) { $sql2 .= (($sql2) ? ',' : '')."($Markerid,$value2)"; } } } $sql = $sql1.$sql2.")";
Hope that gives you a few ideas.
All the best
Keith
-
Hi
It depends on how flexible you want it to be.
If you only have 2 time periods per day then I would be inclined to just store those basic time periods rather than breaking it down to individual hours.
However my assumption was that you needed to book time frames, some of which would overlap others. Eg, you might have a 9-5 Mon, and a 9-12, and a 12-5, and a 9-10, etc, so you would need to record them all (ie, I assumed you were writing something to allocate people to jobs in various time periods in the day).
It might be best to explain fully exactly what you are trying to do with the system and then someone might have an alternative idea.
All the best
Keith
-
Hi
How variable is the data? Ie, do you just have a few of these checkboxes or loads of them?
If not a massive number then I would be inclined to have a 2 dimensional array containing the time and day abbreviations, with the key of the first dimension being linked to the id of the checkbox. The build up you insert by looping through the 2nd dimension of the array for the passed 1st dimension.
For example
$WhatToInsert['9-12 Monday'][0] = '\'9-10\',\'mon\'';
$WhatToInsert['9-12 Monday'][1] = '\'10-11\',\'mon\'';
$WhatToInsert['9-12 Monday'][2] = '\'11-12\',\'mon\'';
$WhatToInsert['9-12 Tuesday'][0] = '\'9-10\',\'tue\'';
$WhatToInsert['9-12 Tuesday'][1] = '\'10-11\',\'tue\'';
$WhatToInsert['9-12 Tuesday'][2] = '\'11-12\',\'tue\'';
$sql1 = "Insert Into Appointments (AppointmentId, HourFrame, Day) Values ";
$sql2 = "";
foreach ($WhatToInsert[$TickedCheckBox) as $value)
{
$sql2 .= (($sql2) ? ',' : '')."($AppointmentId,$value)";
}
$sql = $sql1.$sql2.")"
This could be built up from data held in tables rather than hard coded if you wanted.
All the best
Keith
-
Ah I see. You don't want an OR (||) then, you want an AND (&&).
if (($win == "XP") || ($win == "Vista") || ($win == "2000") || ($win == "98") || ($win == "ME") && ($walktype == "email")) {
Give that a try.
Hi
To make the operator precedence a bit more obvious I would suggest adding a couple of extra brackets
if ((($win == "XP") || ($win == "Vista") || ($win == "2000") || ($win == "98") || ($win == "ME")) && ($walktype == "email")) {
All the best
Keith
-
Hi
There are several arrays of variables in php when a form is submitted. $_POST is just the post method ones, $_GET is the get method ones and $_REQUEST is both combined. So you could quite happily use $_POST as $field => $value.
foreach ($_REQUEST as $field => $value) is looping through all the variables in the $_REQUEST array (ie all the POST and GET items), with each turn through the loop dealing with one field with the field name in $field and the value in $value.
You cannot use your count example. You would need something like :-
$i=0; foreach ($_POST as $field => $value) { echo "$field $value <br />"; $i++ } echo $i;
This would give you a list of the field names and their values, and then the count of them at the end of it.
All the best
Keith
-
Hi
By default all the fields are in an array (well, several, $_REQUEST, or $_POST, or $_GET).
You can loop through them, eg:-
foreach ($_REQUEST as $field => $value) { // Do something here }
All the best
Keith
-
Hi
Worst is when you are using different standards at the same time on different projects.
Even M$ cannot manage to have an SQL standard between sql server and access.
All the best
Keith
-
Hi
Sorry, I have had too many varieties of SQL inflicted on me over time!
All the best
Keith
-
Hi
To me it would depend on the actual data. If it is only partially entered would it make any sense and could entry be continued at a later date?
All the best
Keith
-
Hi
I think you are confusing php and Javascript.
The fragment you posted is Javascript, while the fields you mention are (I think) the php variables that you have put the data in once the form is processed. In Javascript you would want to use the value of the field using the fields id. Eg, if you had <input type='text' id='testField' /> then you would use document.getElementById('testField').value to get its value for use in javascript.
Also an ALERT is a bit of a crude way to put up such a question. To give the users a choice you would want to use a CONFIRM (or start playing around with popup divs or dynamically creating / displaying fields from Javascript).
All the best
Keith
-
first, thanks for your reply...it partially works...when i press submit with no value...it says 1. You didn't enter a username!...but after that...on the box it appears a 1...
You are echoing the "isset" of the field. This will be true or false (ie, 1 or 0) depending on whether it has been set. However as you also have a space in there I think it will always be set, therefore checking isset will always return 1 (so next time round the field will contain a space and a 1).
p.s. i didn't understand where to do this:What is probably needed is to move the HTML form from the top of the script down to the bottom (ie, after if has processed the submit), but put it within an if statement (ie, the one given in the reply).
All the best
Keith
-
Hi
Another option might be
SELECT a.product FROM table a JOIN table b ON a.product = b.product WHERE a.category = 'Plastic' AND b.category = 'Tall'
Would be easy to add more joins (and where clauses to go with them) if there are more required categories.
All the best
Keith
-
Hi
Thanks, although part of the reason I did it was to try and get into doing some OO php.
All the best
Keith
-
Hi
Right, had a bit of a play. Think this will give you what you need, with changing the value of the input fields depending on what your user selects from the drop down fields. Not the most efficient solution if you have loads of companies.
<?php $vbCrLf = chr(13).chr(10); // open connection to MySQL server $connection = mysql_connect('localhost', 'root', 'pass') or die ('Unable to connect!'); //select database mysql_select_db('db') or die ('Unable to select database!'); //create and execute query $query = 'SELECT company, first, email, details FROM man_contacts ORDER BY company'; $result = mysql_query($query) or die ('Error in query: $query. ' . mysql_error()); //create selection list $DropDownList .= "<select name='Company' id='DropDownListId' onchange='javascript:SelectChanged();'> ".$vbCrLf; $DropDownList .= "<option value=''></option> ".$vbCrLf; while($row = mysql_fetch_row($result)) { $heading = $row[0]; $DropDownList .= "<option value='$heading'>$heading</option> ".$vbCrLf; $FirstArrayPhp .= 'FirstArray["'.$heading.'"]="'.$row[1].'";'.$vbCrLf; $EmailArrayPhp .= 'EmailArray["'.$heading.'"]="'.$row[2].'";'.$vbCrLf; $DetailsArrayPhp .= 'DetailsArray["'.$heading.'"]="'.$row[3].'";'.$vbCrLf; } $DropDownList .= "</select> ".$vbCrLf; ?> <html> <head> <title>DropDown</title> <script language="Javascript" type="text/javascript"> var FirstArray = new Array(); <?php echo $FirstArrayPhp; ?> var EmailArray = new Array(); <?php echo $EmailArrayPhp; ?> var DetailsArray = new Array(); <?php echo $DetailsArrayPhp; ?> function SelectChanged() { var Company = document.getElementById('DropDownListId').value; document.getElementById('first').value = FirstArray[Company]; document.getElementById('email').value = EmailArray[Company]; document.getElementById('company').value = DetailsArray[Company]; } </script> </head> <body> <form name="events" method="post" action="<?= $_SERVER['PHP_SELF']?>"> <table> <tr> <td> </td> <td> <?php echo $DropDownList; ?> </td> </tr> <tr> <td align="right" valign="top"><p>First Name:</p></td> <td> <input type="text" name="first" id="first" class="heading" value="<?php echo $row['first']; ?>"> </td> </tr> <tr> <td align="right" valign="top"><p>Email:</p></td> <td> <input type="text" name="email" id="email" class="date"value="<?php echo $row['email']; ?>"> </td> </tr> <tr> <td align="right" valign="top"><p>Company:</p></td> <td> <textarea name="company" id="company" class="details"value="<?php echo $row['details']; ?>"></textarea> </td> </tr> <tr> <td> </td> <td> <input name="submit" type="submit" class="submitForm" value="Submit"> </td> </tr> </table> </form> </body> </html>
I knocked up a test database and tried that and it worked fine for what I think you want.
If you used an autonumber field for the company (ie an ID field, with the company name seperate) this would avoid the possible issue here if you have duplicate company names.
By the way, the $vbCrLf is just there to force new lines and make the generated HTML more easily read. Showing my roots having done VB and ASP programming for too long.
All the best
Keith
-
Hi
You can do it manually using imagecreate(), and for a very simple graph this may be easiest. I have done it this way for simple things (eg, a graph of posts by month on a forum I run).
Since then I have started to write a php graph class. However this is a lot more difficult than I first thought it would be. Eg, if you have a line graph of values against dates, what do you do when there is no value for that date, either default the value to zero for that date or just draw a line from the value of the previous date to the value of the next date. I can forward you the graph class as it exists so far, but while is is basically all there for what I use it for I would not say it is fully debugged. And I have not done anything on pie charts in it yet.
Basically I have used php to generate a page with an image tag on the page, with the source being another php script to generate the required graph.
All the best
Keith
-
Hi
Looking at that there seem to be a couple of issues.
You are looping through populating the drop down list, but the other fields are populated from the $row outside the loop where $row has any values (added to which you have not brought back the required fields in the SQL).
Think what you need to do it use an on change event on the drop down list to trigger a javascript function, and use the javascript function to populate the other fields. To do this you would need to have a couple of arrays of the data you want to populate the fields with. For example in your loop build up a couple of strings which would be Javascript arrays, and pull the data from there.
All the best
Keith
-
Hi
Javascript is useful to save some load on your server and save the user having to wait for the server to validate the form when there are obvious errors. But it is pretty much useless to prevent anyone maliciously entering dodgy data. Once it is into php you may as well fully validate it, checking each individual field.
If you are feeling a bit masochistic then you can use Ajax to validate the form fields as they are entered. With a bit of care you can include the same php to validate the fields both via Ajax and via your main script. Can work well.
All the best
Keith
[SOLVED] syntax error
in MySQL Help
Posted
Hi
Think table is a reserved word, hence issues with a column called table.
All the best
Keith