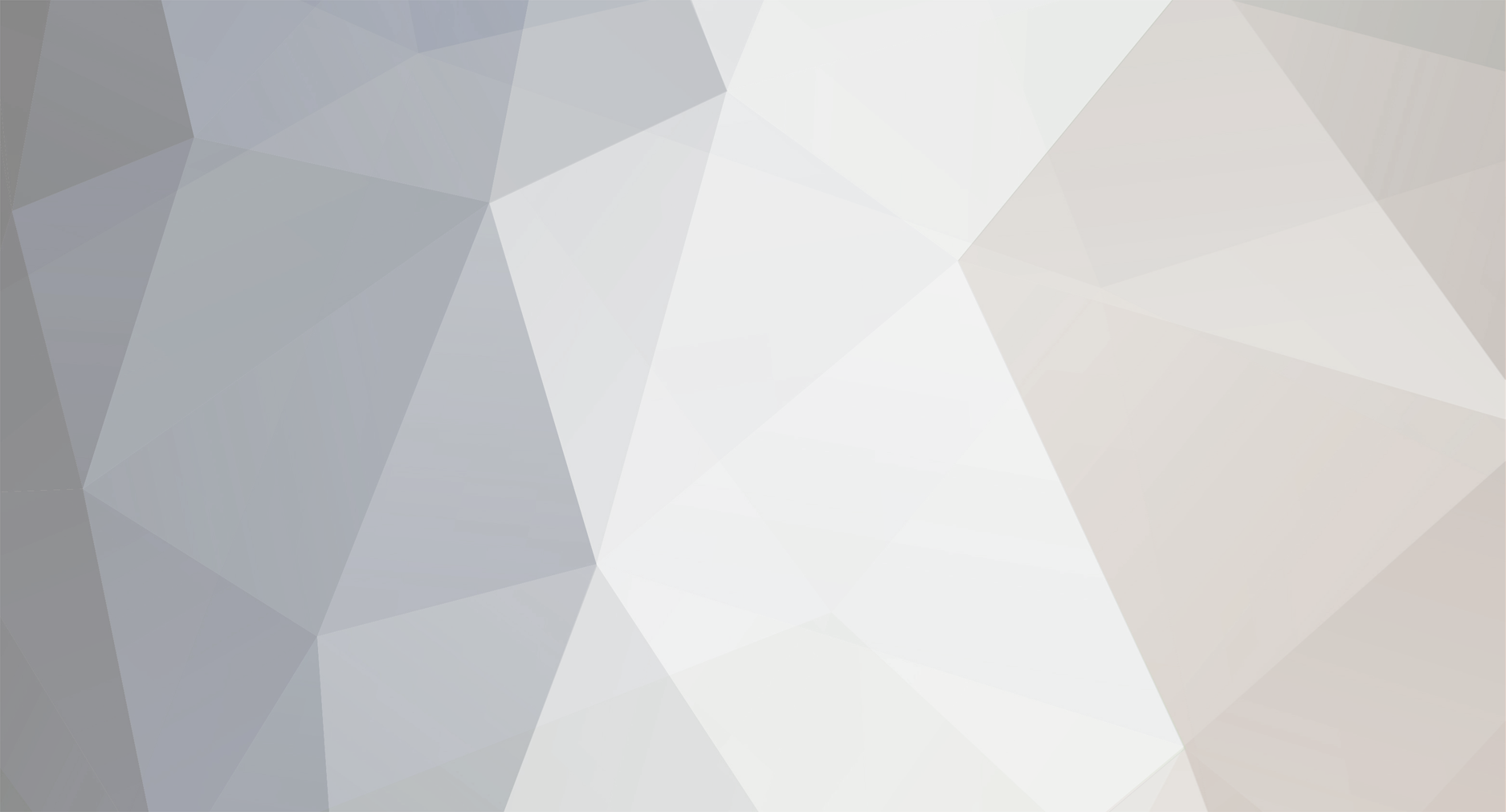
yanjchan
Members-
Posts
68 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
yanjchan's Achievements

Member (2/5)
0
Reputation
-
Hi! I wrote this as an attempt to create a simple client-server socket chat. Unfortunately, I keep getting errors about broken pipes. Any help would be much appreciated. #!/usr/local/bin/php -q <?php error_reporting(E_ALL); set_time_limit(0); ob_implicit_flush(); $address = '192.168.1.138'; $port = 31415; if (($sktMain = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) === false) { echo "Failed to create socket.\n"; echo socket_strerror(socket_last_error()) . "\n"; } socket_set_option($sktMain, SOL_SOCKET,SO_REUSEADDR, 1); if (socket_bind($sktMain, $address, $port) === false) { echo "Failed to bind to port $port.\n"; echo socket_strerror(socket_last_error()) . "\n"; } if (socket_listen($sktMain) === false) { echo "Failed to start listening on socket.\n"; echo socket_strerror(socket_last_error()) . "\n"; } $clients = array(array('sock' => $sktMain)); while (true) { print_r($clients); foreach ($clients as $client) $read[] = $client['sock']; $ready = socket_select($read, $write = NULL, $except = NULL, $tv_sec = NULL); for ($i = 0; $i < count($clients); $i++) { if (in_array($clients[$i]['sock'], $read)) { echo "Was in array\n"; $socket = $clients[$i]['sock']; if ($socket == $sktMain) { if (($client = socket_accept($sktMain)) < 0) { echo "Failed to accept socket.\n"; echo socket_strerror(socket_last_error()) . "\n"; continue; } else { array_push($clients, array('sock' => $client)); } } else { $bytes = socket_recv($socket, $buffer, 2048, 0); if ($bytes == null) { unset($clients[$i]); socket_close($socket); } else { foreach ($clients as $client) { if ($client != $sktMain) socket_write($client['sock'], $buffer, strlen($buffer)); } } } } } } ?>
-
Get div with longest length from remote webpage
yanjchan replied to yanjchan's topic in PHP Coding Help
Hi! Thanks for replying. The problem is that the DOM throws errors on things such as ul and nbsp. This is unacceptable, as for obvious reasons I cannot ask everyone who submits a site to write perfectly good XML without those elements. THanks. -
Hi! Does anyone here know how to find out which div has the most text in it on a remote webpage? For example, if the remote webpage looked like this: <html> <head> <title>Hi</title> </head> <body> <div id="big">sjdf0afh0u9 4uf0a udf8saudfo9aufosalfd</div> <div id="small">iaosjfioajdf</div> </body> </html> the PHP script would find out that the "big" div was the biggest and return its id and contents. So far, I discovered a method of doing this ... provided the site was pure XML. Unfortunately, not all webpages parsed by this script can be trusted to be as such. Thanks in advance!
-
PHP: disallow direct access from browser
yanjchan replied to Soldiers3lite's topic in PHP Coding Help
No problem! Both methods should work perfectly fine, so it's up to you to choose. Good luck with your application! Jonathan -
Using a javascript popup to control a php function
yanjchan replied to seniramsu's topic in PHP Coding Help
If you want to insert the contents of PHP variables into server-side generated javascript, you could do something like this: echo "<script type=\"text/javascript\">"; echo "var foo = {$bar}"; echo "</script>"; I think that that should work. However, I'm by no means a Javascript or PHP whiz, so forgive me if that code is wrong. -
PHP: disallow direct access from browser
yanjchan replied to Soldiers3lite's topic in PHP Coding Help
Hi! I see that you're using POST for your form. In that case, because it is very unlikely that a user will accidentally forge the data, you can just use the if statements you mentioned. To make it clearer, I suppose you could add a hidden field to your form: <input type="hidden" name="submitcheck" value="1" /> then check if the form was actually submitted using PHP: if (isset($_POST['submitcheck'])) { // do stuff here } else { // do nothing or display a message, because the form was not actually submitted. } If you are interested in preventing forged requests, then you might want to look into anti-XSRF methods. Reading this article might be a good start. Hope this helps! Jonathan -
Hi! I wasn't able to fit the whole question in the title, because it is pretty confusing. (to me - hopefully, not to you guys. ) Pretend that there are a bunch of "lines" stored in a database as having starting and ending point coordinates. For example, ID / startx / starty / endx / endy 0 / 1 / 1 / 10 / 10 However, the screen only shows an are which extends from 2, 2 to 7, 7. I could run calculations on every single row in the "line" database and determine which of those intersect with the viewport area, but that would take a long time, yes? My question is whether there is an efficient and practical way to accomplish this task. Thanks in advance!
-
Handling database updates, both timed and real-time events
yanjchan replied to kittrellbj's topic in PHP Coding Help
I apologize for the dead topic bump, but this topic is almost *exactly* similar to what I am trying to do. Would you guys recommend running a cron job every 15 seconds or so? (Actually, 4 different cron jobs executing at four different times in a minute) I've heard that updating on page load might not be very scalable. Sorry if this is in the wrong place. -
Or, does anyone know of a good tutorial that they would like to recommend? The only reputable one I've found is for PHP 4, and it doesn't work anyways.
-
I'm trying to make a PHP Socket Chat server and a C# Client, but I keep getting strange errors. This is modified from something that was meant to be used with flash. #!/usr/bin/php -q <?php /* Raymond Fain Used for PHP5 Sockets with Flash 8 Tutorial for Kirupa.com For any questions or concerns, email me at ray@obi-graphics.com or simply visit the site, www.php.net, to see if you can find an answer. */ error_reporting(E_ALL); set_time_limit(0); // Ensure that the script stays running indefinitely. ob_implicit_flush(); // Turn implicit flushing off. $address = 'icebrg.us'; // The address we are serving from. $port = 43791; // The port we are servinf from. //---- Function to Send out Messages to Everyone Connected ---------------------------------------- function send_Message($allclient, $socket, $buf) { /* This function sends a message to all of the clients. * Parameters: * $allclient = an array of all of the client sockets * $socket = the socket that is sending the message * $buf = the message buffer, contains the message */ foreach($allclient as $client) { socket_write($client, "$buf"); } } function process_Message($allclient, $socket, $buf) { $msgtype = substr($buf, 0, 3); $nicklen = substr($buf, 3, stripos($buf, ";")); $nick = substr($buf, stripos($buf, ";")+1, $nicklen); $msg = substr($buf, strpos($buf, ";")+$nicklen+1); if ($msgtype == "xit") { $index = array_search($socket, $read_sockets); unset($read_sockets[$index]); socket_close($socket); unset($sockets_ids[$index]); $sockid --; } else if ($msgtype == "whr") { send_Message($allclient, $socket, "!whr"); } else if ($msgtype == "ihr") { send_Message($allclient, $socket, $nick." is here!"); } else { send_message($allclient, $socket, $nick.": ".$msg); } } //---- Start Socket creation for PHP 5 Socket Server ------------------------------------- if (($master = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) < 0) { echo "socket_create() failed, reason: " . socket_strerror($master) . "\n"; } socket_set_option($master, SOL_SOCKET,SO_REUSEADDR, 1); if (($ret = socket_bind($master, $address, $port)) < 0) { echo "socket_bind() failed, reason: " . socket_strerror($ret) . "\n"; } if (($ret = socket_listen($master, 5)) < 0) { echo "socket_listen() failed, reason: " . socket_strerror($ret) . "\n"; } $read_sockets = array($master); $sockets_ids = array(0); $sockid = 0; //---- Create Persistent Loop to continuously handle incoming socket messages --------------------- while (true) { $changed_sockets = $read_sockets; $num_changed_sockets = socket_select($changed_sockets, $write = NULL, $except = NULL, NULL); foreach($changed_sockets as $socket) { if ($socket == $master) { if (($client = socket_accept($master)) < 0) { echo "socket_accept() failed: reason: " . socket_strerror($msgsock) . "\n"; continue; } else { $sockid ++; array_push($read_sockets, $client); array_push($sockets_ids, $sockid); socket_write($client, "$sockid"); send_Message($read_sockets, $socket, "Someone has joined"); } } else { $bytes = socket_recv($socket, $buffer, 2048, 0); if ($bytes == 0) { $index = array_search($socket, $read_sockets); unset($read_sockets[$index]); socket_close($socket); unset($sockets_ids[$index]); $sockid --; } else { $allclients = $read_sockets; array_shift($allclients); process_Message($allclients, $socket, $buffer); } } } } ?> The errors I get: Warning: socket_write(): unable to write to socket [32]: Broken pipe in /var/www/gibchat/socketShell.php on line 35 Warning: socket_recv(): unable to read from socket [104]: Connection reset by peer in /var/www/gibchat/socketShell.php on line 107 Thanks in advance for your help.
-
Flash title bar like Gmail on new chat message
yanjchan replied to yanjchan's topic in Javascript Help
I realize that I need to do that. What I need to know how to do is visually flash the title bar so it changes color, or something like that. I've begun doubting if Gmail even flashes the title bar... was I hallucinating? -
First of all, thanks for the generous help you guys have given me in the past on this forum. Second, I apologize in advance if my code is hard to read, most of it was done in a rush. Here goes: login.php <?php // create anti-csrf cookie value $hash = sha1(time().rand().strlen(rand())); $hash = substr($hash, 0, ; if (isset($_COOKIE['xsrf[0]'])) { $i = 0; while (isset($_COOKIE['xsrf['.$i.']'])) { $i++; } setcookie('xsrf['.$i.']', $hash, 0, '/citizen/', '.ch4n.net'); } else { setcookie('xsrf[0]', $hash, 0, '/citizen/', '.ch4n.net'); } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Citizen - Login</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <link rel="stylesheet" href="default.css"> </head> <body> <div class="header"><?php include("menu.html"); ?></div> <div class="body"> <?php if (!empty($_GET['errors'])): ?> <ul> <li><?php print implode("</li>\n\t<li>", explode(';', $_GET['errors'])); ?></li> </ul> <?php endif; ?> <form name="login" action="login_process.php" method="POST"> <input type="hidden" name="xsrfi" value="<?php echo $i; ?>" /> <input type="hidden" name="xsrf" value="<?php echo $hash; ?>" /> <table cellpadding="1" cellspacing="1" id="login"> <tbody> <tr class="username"> <th>Username</th> <td><input type="text" id="username" name="username" maxlength="20" /><br /></td> </tr> <tr class="password"> <th>Password</th> <td><input type="password" id="password" name="password" maxlength="20" /></td> </tr> </tbody> </table> <input type="submit" name="submit" value="Login!" /> </form> </div> </body> </html> login_process.php <?php if ($_COOKIE['xsrf['.$_POST['xsrfi'].']'] !== $_POST['xsrf'] || !isset($_COOKIE['xsrf['.$_POST['xsrfi'].']'])): $errors = "It appears you have been a victim of a browser attack! Please run a virus scan before continuing online activities.;".$_COOKIE['xsrf['.$_POST['xsrfi'].']'].";".$_POST['xsrfi'].";".$_POST['xsrf']; setcookie('xsrf['.$_POST['xsrfi'].']', sha1($hash), time()-1, '/citizen/', '.ch4n.net'); header("Location: login.php?errors=$errors"); endif; setcookie('xsrf', sha1($hash), time()-1, '/citizen/', '.ch4n.net'); require('authent.php'); $user = mysql_escape_string(htmlentities($_POST['username'])); $pass = mysql_escape_string(htmlentities($_POST['password'])); $passwordhash = hashPassword($pass); if(table_exists("user_".$user, 's2zsl9rx_citizen')): // Make a MySQL Connection require('c2db.php'); mysql_select_db("s2zsl9rx_citizen") or die(mysql_error()); $result = mysql_query("SELECT * FROM user_$user WHERE type='001'") or die(mysql_error()); $row = mysql_fetch_assoc($result); if ($row['val'] == $passwordhash): $value = $user.','.$row['val'].','.hashPassword(getip()); setcookie('citizeninfo', $value, time()+3600, '/citizen/', 'ch4n.net'); mysql_close(); header("Location: game.php"); else: $errors = 'Username and/or password are incorrect'.$row['val']; mysql_close(); header("Location: login.php?errors=$errors"); endif; else: $errors = 'Username and/or password are incorrect'; header("Location: login.php?errors=$errors"); endif; ?> Any help at all would be very much appreciated.