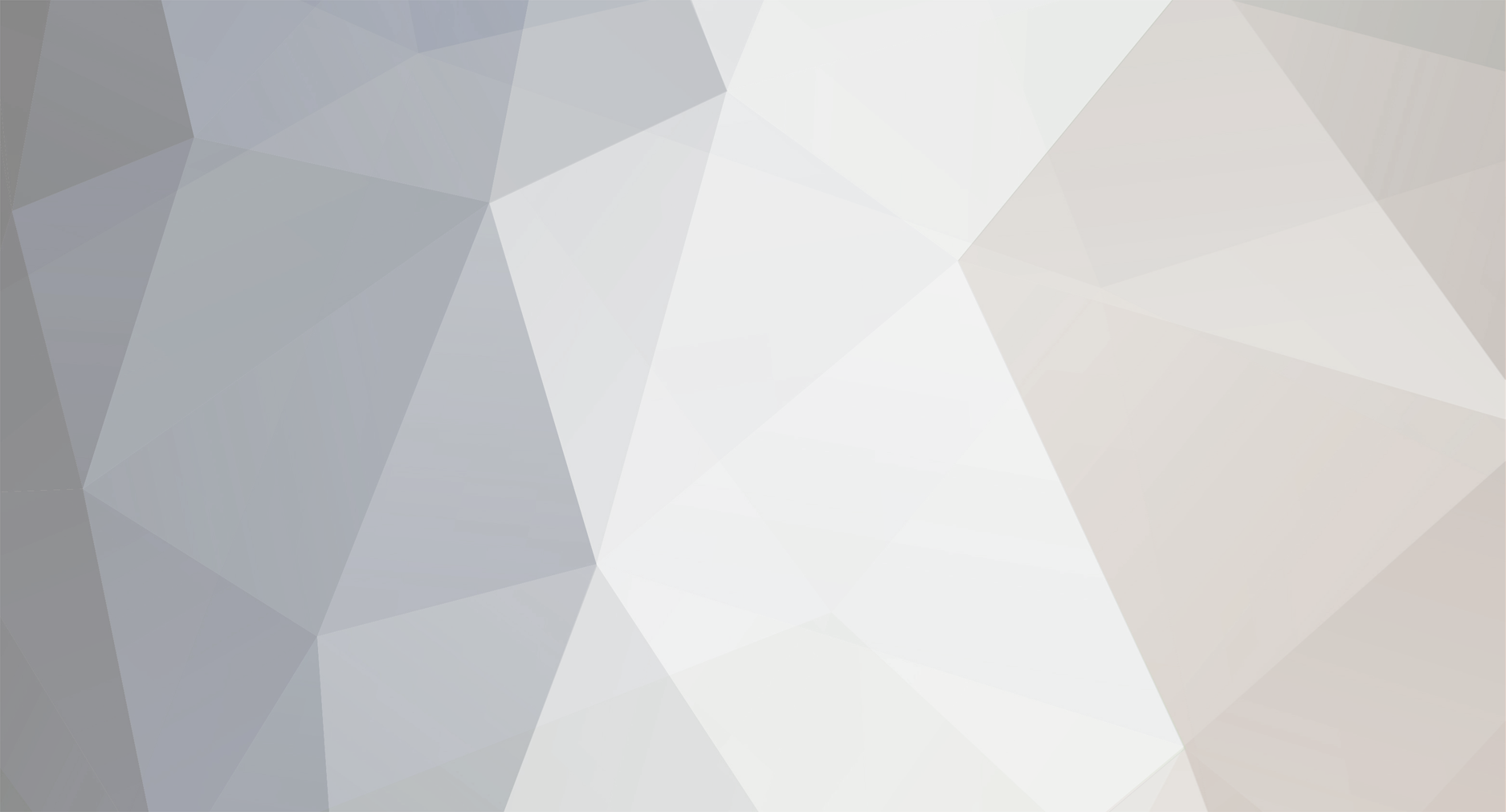
taquitosensei
-
Posts
676 -
Joined
-
Last visited
-
Days Won
2
Posts posted by taquitosensei
-
-
a boolean is 1 or 0, true or false. Usually a column labelled as "unread" would be a boolean telling you whether it had been read or not. If it's supposed to hold the user's id, you should rename it. But after looking at your table here is what the query should look like.
$stmt = $this->db->prepare("SELECT unread FROM pm WHERE unread=1 and receiver_id=:id")
This would get all of the results that were sent to your user that have not been read. You don't need the bind_result line. I believe that's a mysqli function and not needed with pdo.
-
What does the unread table look like. You only need to bind when you're putting user submitted data in your query. You don't have to do that with unread=1. Also unread shouldn't ever equal an id, because it's probably a boolean. Your query should probably look more like this
if($stmt = $this->db->prepare("SELECT unread FROM pm WHERE unread=1 and id_field=:id")) { $stmt->bindParam(':id', $id, PDO::PARAM_INT); }
-
The PR_END_OF_FILE_ERROR is either an issue with the format of your certificate or you might have an older version of nss.
-
You would need to involve javascript. You could do this almost all in javascript then do an ajax call to send the data to php. Here's a guide that shows how to listen for sound and make a reactive page. It should give you an idea of how to start. https://hackernoon.com/creative-coding-using-the-microphone-to-make-sound-reactive-art-part1-164fd3d972f3
-
1
-
-
You don't need to decode. Store the users info in your database. Pre-fill the form. Let them change the info. Then generate a new QRCode and update your database.
-
$dt4->add($dif);
I think that gives the result expected. Not sure why.
2019-07-15 12:30:00 | 2019-07-17 14:15:30 | 0 0 2 1 45 30<br>2019-08-17 01:45:30 | 2019-08-19 03:31:00 | 0 0 2 1 45 30<br>
-
Need more info. What is it supposed to do, what is it not doing. I 2nd foreach.
$files = array("file1.php","file2.php","file3.php"); foreach($files as $file) { include($file); }
-
How are you running the php script? You have to do "php -f /home/ubuntu/dev-ide/test1.php"
-
there is something wrong with $query->wheres, it's not countable. So it's not an array or not an object. Do var_dump($query->wheres); after the $query = to find out what it is and what it does contain.
-
1
-
-
I usually echo at the end of the script instead of in the loop and also send 200 http response.
$result = $page->read($_GET['pageid']); //check for data if ($result) { //page array $page_arr['pages'] = array(); while ($row =$result->fetch(PDO::FETCH_ASSOC)) { extract($row); $page_item = array( 'id'=> $id, 'title' => $title, 'pageid' => $pageid, 'pagecontent' =>html_entity_decode($pagecontent), 'mdkeywords' => $mdkeywords, 'description' =>$description, 'date_added' =>$date_added, 'hasAudio' => $hasAudio, 'excludeSearch' =>$excludeSearch ); //push to data array_push($page_arr['pages'], $page_item); } http_response_code(200); } else { $page_arr['message']="Nothing Here!!!"; } echo json_encode($page_arr);
-
I didn't even think about using the max function.
$numbers = array(1,3,7,8,10,12); foreach($numbers as $number) { if($number%2==0) { $evens[]=$number; } } $max_number = max($evens); echo $max_number;
-
$numbers = array(1,3,7,8,10,12); foreach($numbers as $number) { if($number%2==0) { if($number > $old_number) { $max_number = $number; } $old_number = $number; } } echo $max_number;
Not sure what nodejs has to do with anything. This is a php forum. Something like this would do it in php.
-
If you're not seeing the results you expect in console. I use postman for troubleshooting. You can put in the URL and the post data in json format, and run it to see what the result actually is. You could have an error in my_cart.php. You could be outputting something along with your json. Your json may not be formatted properly. I would add this
ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL);
to the beginning of my_cart.php and either view the results in development tools or download postman and try it that way.
-
1
-
-
A white screen could be a fatal error. I would turn on error reporting
put
ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL);
at the top of your index.php, if it is a fatal error, the error should display instead of the white screen.
-
1
-
-
I forgot to specify that I should get the selected country from a similar html datalist tag:
<datalist id=country_data></datalist>
And get the selected value to reduce the name options of the second datalist:<datalist id=name_data></datalist>
In other words I should connect both datalists each other.
I would suggest doing some more research. It sounds like you need to learn some basic mysql/pdo, php, and html. Here's a decent reference for PDO. There's a ton of php/html tutorials out there.
http://www.dreamincode.net/forums/topic/214733-introduction-to-pdo/
-
Well I don't have bad intentions however I understand your point of view!
It's not the intentions that count. This is unauthorized use. Without an error message, or anything other than "my script doesn't work" I'm going to assume that it's not working because of something on the IRS end.
-
I'm starting with an empty database. I want to provide 10 users credentials to a web front end. I'm going to create tables. I want the users to have access to the new tables I create. They probably should not have access to the system tables. I don't want anonymous people on the internet to be able to access the database through this web page. Please let me know if you need more details on the requirements.
I don't think anybody here is going to do the work for you. Do you have anything so far other than the requirements? Break it down one step at a time and then if you have trouble in a single spot post the code you're using and we can help.
-
Dude. That's the IRS. You don't bot the IRS. Good intentions or not.
Agreed
-
You could use something like
select c.* from customers c where not exists (select 1 from type t where c.customer_id = t.customer_id and t.type in ('macintosh'))
All this does is find the people where a record isn't found for a set of types.
Isn't that what he asked for?
This works if I Just use IN for the customers i want to find that have purchased a particular type of apple but i am trying to search for customers that have never purchased a particular apple or multiple apples from my list. For example if i select just macintosh from the list customer 1 still show up as not purchasing that apple type because they have purchased an apple that is not a macintosh. Can anyone help me figure out how i accomplish this task?
-
select c.* from customers as c LEFT JOIN type as p on c.customer_id=p.customer_id where p.customer_id not in(select customer_id from type where type in('macintosh'));
You'll have to do a nested query to select the ones that match, then select the ones that don't match from that.
-
You can always pull the info with the calendar and put it in the title attribute of the element. Instead of trying to fetch when they hover. Or am I misunderstanding what you're trying to do?
-
first...does the counter need to be 1 or 0 on the first time through. You set it to zero. Then immediately ++ I would move the $counter++; to the end of the loop. Then echo $counter; somewhere in the loop to make sure that it's actually incrementing.
-
1
-
-
you don't have a break after your default case. Not sure if that would cause this though.
-
I am in the process of writing a personal mail system for something that I am working on.
I am setting the system up that when you open the message. It shows the original message and all messages associated with the original message. I am doing this with a while loop. I do not want to, however, show the message subject with every iteration of the while loop.
How do I make it only show the subject once and run through the rest of the while loop?
This is the code that I have so far.
<div class="row compose-form"> <?php $id = $_GET['id']; $que = "SELECT mail.*, CONCAT(s_t.first, ' ', s_t.last) AS 'to', CONCAT(s_b.first, ' ', s_b.last) AS 'for' FROM mail INNER JOIN users AS s_t ON mail.sent_to = s_t.id INNER JOIN users AS s_b ON mail.sent_by = s_b.id WHERE mail.id = '$id' OR mail.replied_to = '$id' ORDER BY mail.sent DESC"; $res = mysqli_query($dbc, $que); $counter = 0; while ($message = mysqli_fetch_assoc($res)) { $counter++; ?> <div class="email-header"> <P><?php echo $subject['subject']; ?></P> </div> <div id="toggler-<?php echo $counter; ?>"> <div class="row"> <div class="col-md-12"> <div class="col-md-6"><span class="to"> <strong><?php echo $message['to']; ?></strong></span></div> <div class="col-md-6"><span class="to pull-right"> <?php echo date("m-d-Y", strtotime($message['sent']));?> (<?php echo getTimeSince($message['sent']).' ago'; ?>)</span> </div> </div> </div> <div class="toggler-hide-<?php echo $counter; ?>"> <div class="row"> <div class="col-md-12"> <div class="email-content"> <?php echo $message['body']; ?> </div> </div> </div> </div> </div> <hr class="mail-controls-divider"> <?php } ?>
You've got a counter
if($counter==0) { echo "<div class='email-header'>"; echo "<P>".$subject['subject']."</P>"; echo "</div>"; }
Limit 50 rows in search results per page
in PHP Coding Help
Posted
You can use LIMIT in mysql
select rows from table limit 1,21
would return the first 21 results. You'd have to build in some logic to determine which page you're on. Here's an example.
https://www.myprogrammingtutorials.com/create-pagination-with-php-and-mysql.html