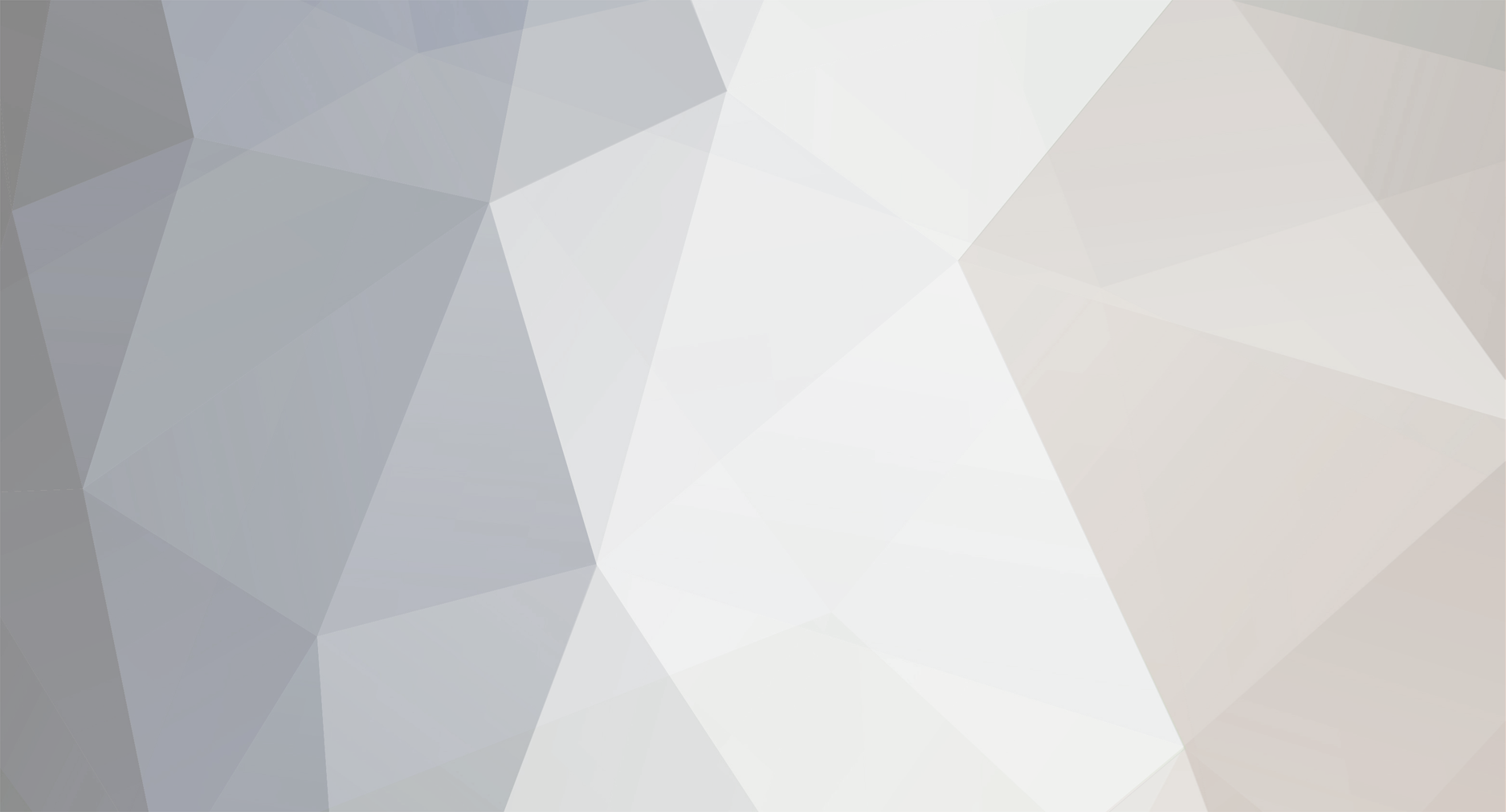
jay0316
Members-
Posts
27 -
Joined
-
Last visited
Everything posted by jay0316
-
No problem Marty. Glad I could help!
-
Hey Marty, The first example works because you're passing numbers for all your data and all your columns have 'number' types assigned to them. The second example doesn't work because this date line is a string: echo date('d M',strtotime($levelresults->timestamp) +18000); // output example: 26 Dec Since it's a string, you'll need to add quotes around it: echo "'".date('d M',strtotime($levelresults->timestamp) +18000)."'"; Then you'll need to change the column to 'string'. Give this a whirl: google.load('visualization', '1.0', {'packages':['corechart']}); google.setOnLoadCallback(drawChart); function drawChart() { var data = new google.visualization.DataTable(); data.addColumn('string', ''); data.addColumn('number', 'Time of Reading'); data.addColumn('number', 'Blood Glucose Reading'); data.addRows([ <?php $level=DB::getInstance()->query("SELECT * FROM tabbyhealth WHERE reading!=0"); foreach ($level->results() as $levelresults) { echo "["; echo "'".date('d M',strtotime($levelresults->timestamp) +18000)."'"; echo ","; echo date('H',strtotime($levelresults->timestamp) +18000); echo ","; echo $levelresults->reading; echo "],"; } ?> ]); var options = { title:'Blood Glucose Monitoring', curveType: 'function', legend: { position: 'bottom' }, width:600, height:300, hAxis: { title: 'Date' }, vAxis: { title: 'Reading and Time' } }; var chart = new google.visualization.LineChart(document.getElementById('chart_div')); chart.draw(data, options); } Here is a fiddle: http://jsfiddle.net/jay0316/pxcozvep/4/
-
Hey Marty, I had typed a larger response, but unfortunately had an issue with my browser and lost it. So, I'm going to give you an abbreviated answer. Here is a working example using your test data: http://jsfiddle.net/jay0316/pxcozvep/1/ Your data types ("string" and "number") for your columns as well as the number of columns need to match the data you are passing in data.addRows. When you use the test data you gave as an example, you are only defining 2 columns in the js, but the there are 3 pieces of data in each array that is in the data.addRows function. You would need a third column to make that work (as you can see in the fiddle link above). In the php coded version you are passing both the timestamp and glucose as "string" (because of the single quotes you are using around the <?php ?> tags, but the columns in the js code are defined as "string" and "number". These will need to match the data you're passing. Also, I'd test your php $timestamp variable by printing it out to make sure you're getting what you expect. Finally, I normally like to include the brackets with my foreach loops so it's clear where it starts and ends. There are a couple ways of doing it. One way might be creating a js variable above your google chart js code you have like so (not tested) : var rowData = [ <?php $level=DB::getInstance()->query("SELECT * FROM tabbyhealth WHERE reading!=0"); foreach ($level->results() as $levelresults) { $glucose = $levelresults->reading; $timestamp = date('d M Y h.i A',strtotime($levelresults->timestamp) +18000); echo "['".$timestamp."',".$glucose."],"; } ?> ]; and then pass the js variable to data.addRows like so: data.addRows(rowData); Hope this helps set you in the right direction. Happy Holidays!
-
I've placed an htacess file with the code below into my folder with a bunch of artfiles. I wanted to use it so that when I create an anchor tag and link to one of those files it will download the file. It works like I wanted it to (files download when I click the link); however, I have a page that I created to display some of those files on. When the htaccess file is in the folder they do not display on this page. I'm using an img tag or object tag (for swf files). On this page the files don't show or download. Is there a line of code that will use this for anchor tags only? Why does this affect the img and object tag? If not, is there a better way to do what I'm trying to do (this seemed so easy)? <FilesMatch "\.(?i:jpg|gif|png|jpeg|tif|tiff|swf|pdf)$"> Header set Content-Disposition attachment </FilesMatch>
-
I will check out the backup generator and what our DNS Service Provider has as far as maintenance page options. The power is going to be out from 7:30am to 5:00pm. So, I don't think the UPS is going to help. Thanks for the feedback guys.
-
We have our website hosted on our own internal ubuntu server. The power is going to be shutdown this weekend because the power company will be working on our power lines. What is the best way to serve up a down for maintenance page or something to our customers so that it doesn't just look like our site disappeared? The only option I've thought of so far is an external hosting account that we store some maitenance pages on and redirect our company websites to those for the weekend. Is there a better way? Thanks.
-
We are uploading product videos to youtube. On our site we have our product info stored in a database. There are a couple products that have more than one video. I'm wondering if I should store them in the record with the item. Or if they should be stored in a separate table with the item id and video id. We may eventually have some training videos etc. that could apply to several items. Any suggestions?
-
anyone? If you need more info, just let me know what you need and I'll give it to you. I could really use some help.
-
Below I have the SQL for 2 tables that are simplified versions of what I'm working with. I need to print a list of all stores where the completed by field is by "Todd". However, I need the list to always print the parent store first with the children underneath. Can I do that with the way things are setup with some combination of SQL and php? Table 1 -- -- Table structure for table `table1` -- CREATE TABLE `table1` ( `id` int(2) NOT NULL auto_increment, `parent` int(2) NOT NULL, `child` int(2) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=5 ; -- -- Dumping data for table `table1` -- INSERT INTO `table1` (`id`, `parent`, `child`) VALUES (1, 2, 3), (2, 2, 3), (3, 2, 1), (4, 4, 5); Table 2 -- -- Table structure for table `table2` -- CREATE TABLE `table2` ( `id` int(2) NOT NULL auto_increment, `store_name` varchar(255) collate latin1_general_ci NOT NULL, `completed_by` varchar(255) collate latin1_general_ci NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=7 ; -- -- Dumping data for table `table2` -- INSERT INTO `table2` (`id`, `store_name`, `completed_by`) VALUES (1, 'Walmart Supermarket', 'Todd'), (2, 'JC Penny', 'Todd'), (3, 'Wal-mart', 'Todd'), (4, 'Dollar General''s Store', 'Jeff'), (5, 'Dollar General', 'Todd'), (6, 'Giant Eagle', 'Mark'); The way I was trying to do it is by selecting all the parent stores and stores that don't have a parent child relationship: SELECT * FROM table1 WHERE NOT EXISTS ( SELECT child FROM table1 WHERE id = child ) AND completed_by = 'Todd' Then I with php i checked to see if the id was a parent, and if it was I printed the parent and then did another mysql select statement to select all the child id's and printed them out below. The problem I have is that sometimes the parent store isn't completed by "Todd", so I'm missing some of the child stores. Any ideas?
-
here are the 2 tables: dealers CREATE TABLE `dealers` (\n `Dealer_ID` mediumint(11) unsigned zerofill NOT NULL auto_increment,\n ``Dealer_Zip` varchar(255) character set latin1 default NULL,\n `Account_Number` varchar(11) character set latin1 NOT NULL,\n `Dealer_Chain` varchar(10) character set latin1 default NULL,\n `AS400_BusinessName` varchar(255) NOT NULL,\n `Dealer_BusinessName` varchar(255) character set latin1 default NULL,\n `Dealer_Username` varchar(16) character set latin1 default NULL,\n `Dealer_Password` varchar(16) character set latin1 default NULL,\n `Dealer_Address1` varchar(255) character set latin1 default NULL,\n `Dealer_Address2` varchar(50) character set latin1 default NULL,\n `Dealer_City` varchar(255) character set latin1 default NULL,\n `Dealer_State` varchar(255) character set latin1 default NULL,\n `Dealer_Country` varchar(50) character set latin1 default NULL,\n `Dealer_Phone` varchar(255) character set latin1 default NULL,\n PRIMARY KEY (`Dealer_ID`),\n UNIQUE KEY `ID_2` (`Dealer_ID`),\n KEY `ID` (`Dealer_ID`)\n) ENGINE=MyISAM AUTO_INCREMENT=1195 DEFAULT CHARSET=ascii dealers_chain_lookup CREATE TABLE `dealers_chain_lookup` (\n `ID` int(5) NOT NULL auto_increment,\n `Corporate` mediumint(11) NOT NULL,\n `Child` mediumint(11) NOT NULL,\n PRIMARY KEY (`ID`)\n) ENGINE=MyISAM AUTO_INCREMENT=60 DEFAULT CHARSET=latin1
-
Ok. I think I fixed the multiple corporate listings by changing the select to: SELECT * FROM dealers AS dlr LEFT JOIN dealers_chain_lookup AS cl ON dlr.Dealer_ID = cl.Corporate LEFT JOIN dealers AS chn ON cl.Child = chn.Dealer_ID GROUP BY dlr.Dealer_ID ORDER BY 1,2; However, I'm having an issue with the corporate store not appearing above the child store.
-
Thanks for the suggestion artacus. I modified your statement to try and make it work. So far, the corporate store is being displayed 16 times. One time for every time I put a child store in I assume because each child id in the lookup table has a corporate id attached to it. Is there something I need to adjust in my statement? SELECT * FROM dealers AS dlr LEFT JOIN dealers_chain_lookup AS cl ON dlr.Dealer_ID = cl.Corporate LEFT JOIN dealers AS chn ON cl.Child = chn.Dealer_ID ORDER BY 1, 2;
-
The MYSQL Version Number is 5.0.51a .
-
I've got a list of stores that need to be dumped into a spreadsheet using a php script. The trouble is that the php code that I found for creating a spreadsheet only excepts one select statement and I don't know how to write this in one statement. Some of the stores belong to a chain. So, there is a corporate store and then children that fall under it. Then there are also stores that are not part of a chain that need to be on the sheet. I'd like to make sure that each time a chain is listed the corporate store is first followed by it's children. All the stores will be listed alphabetically by name. table dealers - contains the store record columns Dealer_ID, Dealer_BusinessName - there is also a Dealer_Chain column that has "Corporate" or "Child" or "NULL" table dealer_chain_lookup -contains the Dealer_ID of the corporate and child store columns Corporate, Child It looks like this $result = @mysql_query($sql,$Connect) is what the script is using to pull the data for the excel document. Maybe if you don't know how to make the "select" in one statement, you may know of a way to do it in two and compile the results in the $results variable?
-
Thanks for clearing that up ignace!
-
mjdamato Thanks for that solution! Should -14 say "day" after or "days"? I saw on php.net someone used "days".
-
The following code checks the year the detail sheet was last completed against the current year. The idea was that each January it would show the user they need to complete another one. //Check Detail Date //grab year from stored date $Detail_Sheet = substr($Detail_Sheet, 0, stripos($Detail_Sheet, "-") ); //Check it against current year $y = date(Y); if ($Detail_Sheet == $y) { $d_class = "complete"; $d_icon = "images/complete.png"; } else { $d_class = "notcomplete"; $d_icon = "images/notcomplete.png"; } Now, we are allowing them 2 weeks into the new year to complete the previous year's sheet. Is there an easy way that I could modify this to still be dynamic and give them until Jan 14th each year to complete the previous year's sheet? I hope that makes sense. Thanks!
-
Thanks for the quick response. I like where you are going with being able to use the same app to view both tables. We have the server in house and have the space and privelages to do what you're saying. Are you saying to create a table with the same name, but have a column for the year and put it in a seperate database? And then the drop down would allow them to select the year they want to view?
-
Our sales reps record store information about products etc using forms that put the data into a table in phpmyadmin. Each store has its own record in the table. At the end of the year we would like to clear out the values, but keep the previous record in case we would want to use it later down the road to pull info on a store in a particular year. I was wondering if it would be better to just copy that table and give it a year (ex. "dealers_2009" ). Each year we'd be adding another table. Or would it be better to set up another table (ex. "dealer_archive") with a year column in it and transfer the records to that table each year. Obviously the easiest option would be the first, but I want to do the best option. Any recommendations?
-
Hi, I've been using php for a little over 2 years. I'd consider myself between beginner and intermediate user. I have a custom application that I developed for the company I work for. Over time I continue to get requests for new features or to make exceptions for certain senarios. I'm finding that it starts to complicate the code quite a bit, and I'm afraid that eventually it's going to get harder and harder to read and understand. In general, are there any topics or books or guidelines that I could research for keeping the code easy to manage as you continue to expand an application?
-
Hey guys, I do have a column storing when the store was added. The problem is we have to manually look at the column and compare the dates when we print out a spreadsheet of the data in the database. I'm thinking about putting in an extra column for each quarter that will have a number. 0 = store was not visited 1 = store was visited 2 = store is new Then when a new store is added I could just add a 2 to the extra column for the quarters that do not need a date. When calculating the total percentage for that quarters visits, I could subtract all the stores that have a 2 in the column from the total number of stores. That would give me an accurate percentage I believe. Does that sound like it would work? or is adding the extra columns making it harder than it is? I think it would be easier to look at 0s 1s and 2s when viewing the spreadsheet than trying to compare the dates and figure it out.
-
Our sales reps are required to visit a store once per quarter. I have an application (4 fields with submit buttons next to them) that is storing 4 visit dates (one for each quarter) in 4 columns (data type = date with default value of 0000-00-00) in a mysql database. The problem comes in when they add a new store. Let's say the new store is added during the 3rd quarter. There are not going to be visit dates for the 1st and 2nd quarter. Is there a way I can handle this so that when we look at the columns in the database, we'll be able to tell whether they just didn't visit the store or the field didn't get a value because the store was new?
-
That worked perfectly. Thanks!
-
I'm trying to use sessions for the first time. I am creating a multi page form. The form will allow users to enter a quantity of a particular kit. I have kit numbers being pulled from a database and entered as the "name" of the input fields for the quantities. On the next page I start the session and I'm trying to add those quantities to the session variables. I have the kit names in a database so I thought I'd be able to just do a while loop and have it loop through and store the values like so: //start the session session_start(); $sql = "SELECT * FROM kits"; $results = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_array($results)) { $knum = $row['number']; //register session variables session_register('$knum'); //store posted values in the session variables $_SESSION['$knum'] = $_POST['$knum']; } print $_SESSION['KCTB09']; $test = $_POST['KCTB09']; print "test = $test"; session_destroy(); ?> The $test variable works, but the session does not print anything. However if I hard code a kit name in Session like $_SESSION['KCTB09'] = $_POST['KCTB09']; , it works. Is it just not possible to store session values in a while loop using variables or am I doing something wrong?
-
I think I understand what your saying. I just don't understand how to apply it to code that makes the excel document. Are you saying that I would modify these lines: //start while loop to get data while($row = mysql_fetch_row($result)) { //set_time_limit(60); // HaRa $schema_insert = ""; for($j=0; $j<mysql_num_fields($result);$j++) { if(!isset($row[$j])) $schema_insert .= "NULL".$sep; elseif ($row[$j] != "") $schema_insert .= "$row[$j]".$sep; else $schema_insert .= "".$sep; } Thanks for the help.