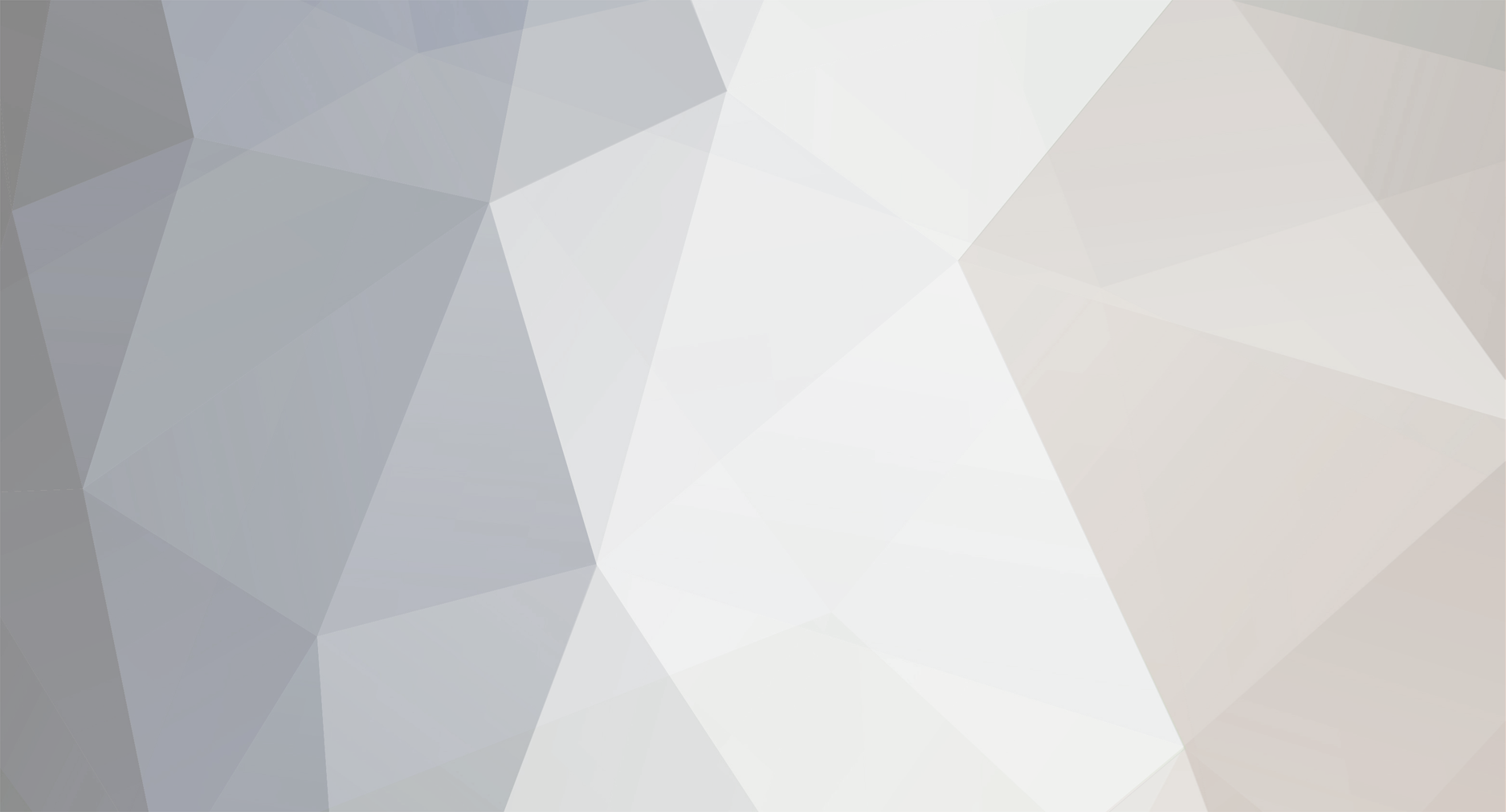
nankoweap
Members-
Posts
113 -
Joined
-
Last visited
Everything posted by nankoweap
-
how to make db config connection available throughout entire app?
nankoweap replied to ballhogjoni's topic in PHP Coding Help
i wouldn't use inheritance to acquire the functionality, but rather composition. jason -
[SOLVED] how do I notify a script when I receive an email?
nankoweap replied to ballhogjoni's topic in PHP Coding Help
if you're on a unix-based system, look into .forward files. not sure what to do on a windoze box. jason -
how to make db config connection available throughout entire app?
nankoweap replied to ballhogjoni's topic in PHP Coding Help
check out my post here: http://www.phpfreaks.com/forums/index.php/topic,249790.0.html -
this may be a good start... http://www.php.net/manual/en/refs.fileprocess.file.php jason
-
i'm a relative noob to php, but i've had zero issues with includes/requires when the paths are relative from the app's document root. i figure if it's going to search for includes from one or more directories, the doc root will be one of them. so far so good. try making your paths relative to the doc root and see what happens. jason
-
no need to go full-blown oop to get this to work. using the singleton as-is and changing the connection from "new msqli..." to "mysqli_connect..." would be a simple way to gain the advantages of a single, shared connection. in the pages that need it, just include the singleton class, get its instance and use the connection directly. jason
-
found this today... http://us.php.net/manual/en/function.sem-acquire.php using semaphores will allow your php code to effectively address concurrency by synchronizing access to a shared resource (e.g. a file used to maintain a counter/sequence).
-
regarding database access specifically... i've settled on the singleton approach. this singleton provides application-wide information plus encapsulates a connection which is lazily created. the base class of the business objects that make up the modeling layer of the application ask the singleton for its instance as well as the connection. here's a stripped down version of the singleton, AppInfo.php: <?php class AppInfo { private $mysqlHost = 'someHost'; private $mysqlUser = 'someUser'; private $mysqlPassword = 'somePass'; private $mysqlDatabase = 'someDB'; private $pageSize = 20; private $con; private function __construct() {} private function __clone() {} public static function getInstance() { if(self::$_instance === null) { self::$_instance = new self(); } self::$_instance->count += 1; return self::$_instance; } public function getMysqlHost() { return $this->mysqlHost; } public function getMysqlUser() { return $this->mysqlUser; } public function getMysqlPassword() { return $this->mysqlPassword; } public function getMysqlDatabase() { return $this->mysqlDatabase; } public function getPageSize() { return $this->pageSize; } public function getConnection() { if (is_null($this->con)) { $this->con = new mysqli($this->getMysqlHost(), $this->getMysqlUser(), $this->getMysqlPassword(), $this->getMysqlDatabase()); if ($this->con->connect_errno) { printf("Cannot connect to MySQL Server: Error Code: %s\n", $this->con->connect_error); } } return $this->con; } function __desctruct() { $this->con->close(); } } // AppInfo ?> And here's the base business object class, BaseBo.php: <?php include_once('classes/AppInfo.php'); class BaseBo { protected $appInfo; protected $con; function BaseBo() { $this->appInfo = AppInfo::getInstance(); $this->con = $this->appInfo->getConnection(); } } ?> And to round it all out, here's a snippet from a business object class, UserManager.php: <?php include_once('classes/bean/User.php'); include_once('classes/bo/ReportManager.php'); include_once('classes/bo/BaseBo.php'); class UserManager extends BaseBo { function UserManager() { $this->BaseBo(); } // bunch of user-related functions in here for logging in/out, registering, updating profiles... } ?> it's important to note that only business objects in the model access the database. anytime they need access to the connection available for servicing the current request, it's merely: $this->con the next code iteration will address and improve error/exception handling. given my rudimentary understanding of php and its architecture, this approach provides an optimal connection solution - create it lazily and share the same connection across all objects that service a request. regarding connection pooling... sorry. that's outside the realm of a php application given its inherent stateless architecture and no simple, effective way to create and share resources like connections and open files and across multiple requests. jason
-
have you tried? print($insert);
-
hmmmm.... i reckon you could explore writing a php extension that provides a control layer for your app. it may be easier to implement using some gool ol' C and the OS resources it would have ready access to. ????
-
well, you're going to have push concurrency control to something in your application. in the databaseless (is that a word?) webserver/php world, i'm not sure what can be used to reliably provide concurrency control.
-
if you're using a database (mysql, oracle...), then you could simply use the database to generate unique, incremental numbers. jason
-
try: mark1 = new GMarker(new GLatLng(openInfoWindowHtml("<?= $two ?>"), -75.240867), { icon: blueIcon});
-
well, that's not entirely true. you can introduce newlines into the body by encoding them as %0A in the url. that's a zero and not an O. of course, i'd recommend heeding gevans' words, but if all you're trying to do is get through your pascal project, then giddyup. jason p.s. pascal, eh? that brings back some memories.
-
uzzy, start at the beginning. mike posted a good starter's php tutorial. do it and go from there. forget about functions at this point until you've gained a modicum of understanding of how php can be applied to implement your web app. if you just want to peruse functions, go straight to the manual on php.net. jason
-
fair enough. i reckon i'm at the other end of the spectrum. hell, i don't touch that key unless i'm typing a tilde.
-
unless one's using keywords as table/column names, why put ticks around them at all? why not simply: mysql_query("INSERT INTO promocodes (id, code, info,createdby) VALUES ('', '$code', '$info', '$username')") or die (mysql_error()); jason
-
ok. so i've been around here for a short time and have seen a lot of php code. i've almost completed porting one of my java/jsp-based apps to php and feel i have a good understanding of how i will structure the other apps that i'll begin porting soon. they should port more quickly. one thing i've enjoyed in the java world is the strict boundaries between the MVC layers - provided it's designed and implemented that way. the model has always been implemented in a well-structured layer of components that accept data requests from the controllers, interact with the database and return results, if any. the controlling layer is a set of servlets that service each request, makes requests of the model and eventually forward "stuff" to the view. the viewing layer is a set of jsp pages responsible for user presentation. in the php world, my php world at this point , i've implemented the model in much the same way as before. this pleases me greatly as i prefer clear, well-defined application boundaries. the controlling and viewing layers, however, are physically semi-married in one php page. i don't have a real issue with this as the controlling layer should be thin and focus primarily on input validation, make requests of the model and allow the view to do its job of presentation. so the controlling layer is a short, concise and focused bit of php code at the beginning of each page. the view is the html and small bits of php used to cycle through arrays and such to build the visual components on the screen such list boxes, tables, etc. so even though i've married the VC layers in a single page, each layer is still clear and well-defined. this all pleases me. what i've seen in a lot of the code that folks post show a coupling (by printing and echoing large chunks of html) of the VC layers that would concern me greatly if it was a project i was managing technically. why? because people on projects play roles and this type of coupling tends to make programmers web-designers. only speaking for myself, this is not good because i have zero artistic capability as evidenced by the web apps i've created in the ether: http://jasonjonas.org/spot http://jasonjonas.com/rides http://jasonjonas.com/miles (this is the one i'm porting currently) http://jasonjonas.com/mtfta anyway, look at any of these apps and my lack of visual/artistic ability is evident. i can make it work, but i cannot make it look good. and that's why i'm a server-side guy. i'm good with nuts and bolts. these are all merely apps i've created and make freely available to the motorcycling community as well as anyone else who can make use of them. but with clearly defined VC layers, i could have a web-designer-type person make these pages look mucho better without having to deal with a boat load of java or php code. again, the bulk of the view is html with bits of java/php to help build some of the visual components. so my question in all of this is... if you're responsible for the design/implementation of a large php application with a good number of folks on your team, have you designed it with clear and well-defined application layer boundaries or have you, for whatever reasons, allowed some layers to overlap? if so, which ones and to what extent? what issues did this cause, if any? for the applications i've designed for my customers in the java world, these boundaries are essential in order to clearly define the roles and responsibilities of each person on the project. sorry for the long post. i've always been verbose. jason
-
did some quick research and sender is not something we can control. it's added by the mail provider. how each client interprets and displays the message to the reader isn't under our control either. how are you sending email? do you have your own mail server or...?
-
Sender must not be a header that we have control over then. unless someone else has a bright idea, i think you're forked. jason
-
interesting. at first i'd say it's an email client-ism, but at closer look i've never seen the Sender header. i'm wondering if you added Sender to the header and set its value just like the From and Reply-To if it would make it behave like you want. something like: $headers = "From: " . $_POST['email_box'] . "\r\nReply-To: " . $_POST['email_box'] . "\r\nSender: " . $_POST['email_box']; i'm not johnny-email so i don't know if this will work of fubar the whole thing, but what the hell. it's worth a try.
-
show us your modified code and the original text of the email sent which will include something like: To: [email protected] Subject: MT: Password Reset Request From: [email protected] Reply-To: [email protected] Message-Id: <[email protected]> Date: Sat, 25 Apr 2009 23:23:43 -0500 (CDT)
-
so what's the problem? add Reply-To to the header and set it to whatever email address you want. i've given you the format of the header. it's just a matter of suiting it to your application's needs. jason
-
here's how i'm setting the from and reply-to: $headers = "From: " . $appInfo->getSystemEmail() . "\r\nReply-To: " . $appInfo->getSystemEmail(); $appInfo is a singleton that stores application level configuration data. jason
-
[SOLVED] Dynamicly pulling images stored on the server
nankoweap replied to TGWSE_GY's topic in PHP Coding Help
does your application not have a database??? assuming it does, then storing the name of the selected profile picture would be a no-brainer. if your application doesn't have a database, then what are you using to store user and other application-related data? jason