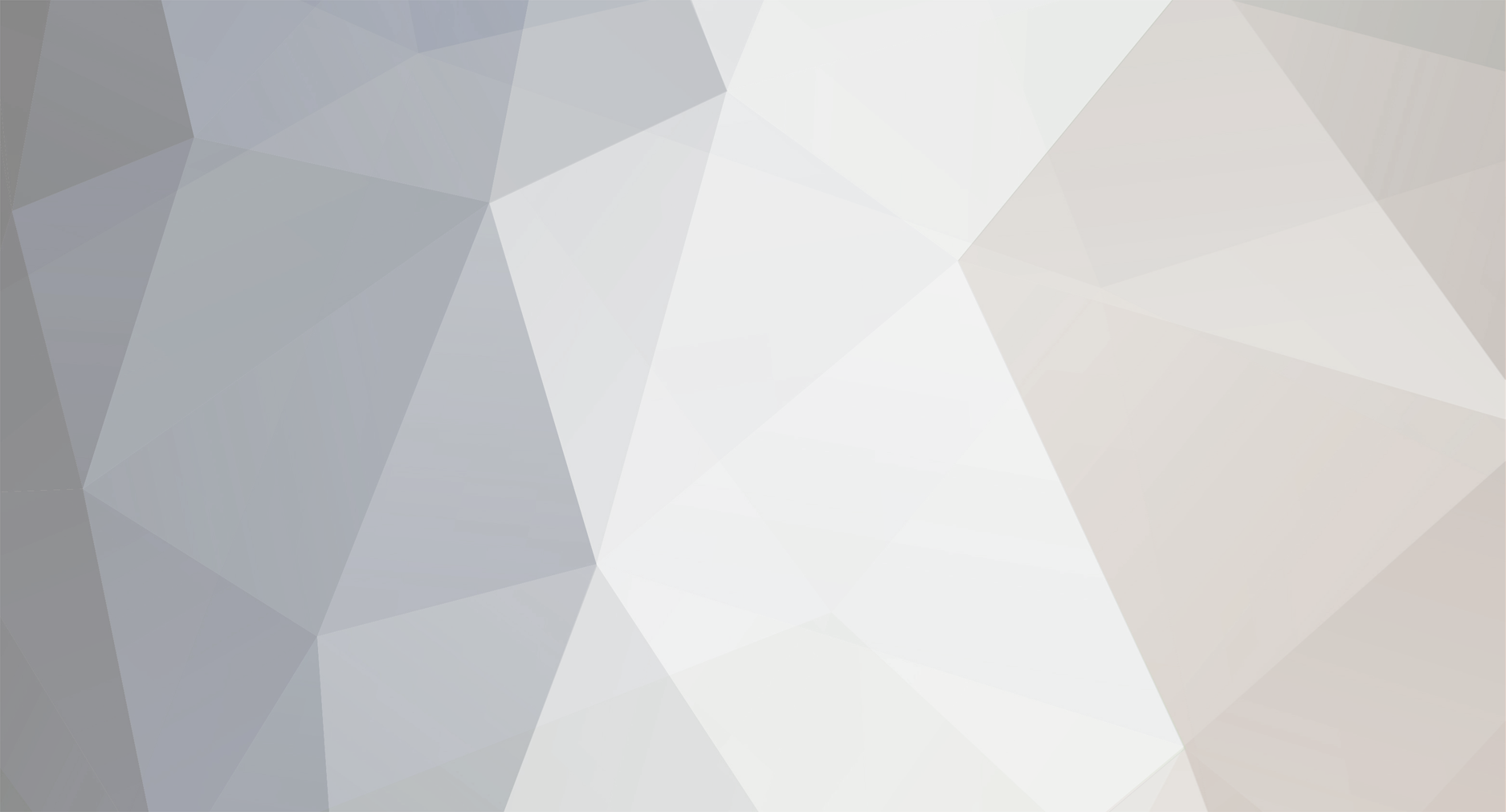
gffg4574fghsDSGDGKJYM
Members-
Posts
195 -
Joined
-
Last visited
Everything posted by gffg4574fghsDSGDGKJYM
-
It's a charset encoding issue. You will have to look at which encoding you save the file, the database data if any, the http header and the meta in the (x)html file. There is some editor and tool that allow you to save the file in different encoding you can take a look at this : http://en.wikipedia.org/wiki/Character_encoding You can setup the charset in mysql connection with this (be sure to change row to the proper charset encoding first) : <?php mysql_query("SET NAMES 'utf8';", $connect) or die ("Unable to set names."); mysql_query("SET CHARACTER SET 'utf8';", $connect) or die ("Unable to set charset."); ?> You can set the charset in http header for html with this (for xhtml it can be a lot more complicated that this though i think most people serve/view xhtml as text/html) : <?php header('Content-Type: text/html; charset=utf-8'); ?> And in html/head with this : <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> Use utf8 (unicode) or change it to whatever encoding type you want. You can use http://www.php.net/manual/en/function.htmlentities.php to convert special charaters into a ASCII readable entities which will work no matter what charset you have.
-
Add the dynamically generated javascript code inside <script> tag like that : <script type="text/javascript"> i=60; <?php echo "var minu=". $_GET['time'].";"; ?> alert(minu); </script> And view the source with your browser to see if the ouput is actually what you are expecting.
-
Why you don't look at facebook javascript to see how they done it ?
-
I'm not sure exactly what you want but i think you are looking for CONCAT : http://dev.mysql.com/doc/refman/5.0/en/string-functions.html#function_concat But if you have many website in the same table why you don't add a column like that website varchar(50) AND UPDATE customers SET referer='$_SESSION['referrer']', website='www.something.com' WHERE username='$_SESSION['userName']';
-
I hope you saved your database because you lost the ipaddress column. When you use : UPDATE weblog SET ipaddress = $ipaddress; You update all row in the database. You must tell mysql to update 1 row not the whole table with a WHERE. Now you need to recover from a backup, because all the ipaddress columns have the same value, then run a script like that : <?php $sql = "SELECT id,ipaddress FROM weblog;"; $result = mysql_query($sql); while($row = mysql_fetch_array($result)) { $ipaddress = $row['ipaddress']; $id = $row['ipd']; $sql = "UPDATE weblog SET ipaddress = INET_ATON('".$ipaddress."') WHERE id=".$id.";"; $upd = mysql_query($sql); if (!$upd) { echo "<br />Failed to update"; } else { echo "<br />Success!"; } } ?> Use id column or any other PRIMARY KEY to uniquely identify the row. Look carefully at the script i didn't test it and change id to whatever the primary key is. You still need to doing this script on the varchar until all value are converted to unsigned int, then convert it to unsigned int. You can leave it as varchar if you want it will work, but the performance will be better if you convert it to unsigned int after all data are processed.
-
MySQL refer to line 6 within the MySQL query not into the php file. It probably having something to do with a missing comma , on line 5, 7 with a MD, on line 9 and the AND on line 10. Try to get your query working into phpmyadmin before putting it into php it may help.
-
You have ip address in format '192.0.34.166' to be converted in a format like this '3221234342' ? If so you can use ip2long http://www.php.net/manual/en/function.ip2long.php Make a little php script that will loop through all your record and update them. When done only change the datatype to Unsigned INT from string. MySQL will automaticly convert the number string version to a integer and you won't lose any data. Be sure to save your table/db just in case MySQL also have built-in functions to do that : inet_ntoa http://dev.mysql.com/doc/refman/5.0/en/miscellaneous-functions.html#function_inet-ntoa inet-aton http://dev.mysql.com/doc/refman/5.0/en/miscellaneous-functions.html#function_inet-aton
-
PHP is a server side script and can't do that. You have to use a client side thing to do a popup. Use html target="_blank", javascript window.open, AJAX to bring a div in front and display the content or maybe flash or any other client side 3rd party plugin.
-
I don't think it's a php problem. Probably a html/css one. Add this between you <head></head> tag <style type="text/css"> img { margin:0; padding:0; border:0; } </style> Get very confortable with html/css before playing with php will save you from a lot of headache
-
Can I do mysql/php stuff inside of a Wordpress page???
gffg4574fghsDSGDGKJYM replied to tvance929's topic in Applications
I have suggest to find a plugin to do the datagrid for you instead of writing php code. Finding a plugin for a datagrid will be probably faster than write your own php code and it will be probably more secure and have more options that you can think of. A plugin will also probably be updated in the future to work with future version of wordpress. Easier to download the new wordpress/plugin version than working with your php code that broke with the new update. But if it works the way you want...enjoy -
It would work great. Simpler than my solution actually. something like that in products.php <?php $uri = $_SERVER['REQUEST_URI']; if (!$uri ...) /* If the URL matches the old URL pattern redirect */ { header("HTTP/1.1 301 Moved Permanently"); header("Location: http://www.yoursite.com/products/".$_GET['p']."/"); header("Connection: close"); exit(); } ..... ?> With all the sanitization and security check of course and be sure it work or you may end up in a infinite loop of redirect
-
Uploading direct in to mySQL - Help
gffg4574fghsDSGDGKJYM replied to spires's topic in PHP Coding Help
I'm not familiar with BULK INSERT (i didn't even know that exist) but i can guess mysql will need the full pathname/filename instead of only the filename. Try echo $sql; and run the sql command from phpmyadmin or in a shell to see if it work. You may want to take a look at this : http://dev.mysql.com/doc/refman/5.1/en/load-data.html If it fail i'm sure fgetcsv with a loop and lot of insert will work, i'm not sure about the performance. http://www.php.net/manual/en/function.fgetcsv.php -
Can I do mysql/php stuff inside of a Wordpress page???
gffg4574fghsDSGDGKJYM replied to tvance929's topic in Applications
While it probably can be done, i would suggest using wordpress existing tools and/or a plugin first. http://wordpress.org/extend/plugins/ Try asking google and wordpress forum, some plugin may not have the name you think or some plugin may exist outside wordpress.org If no built-in function or plugins exist that allow you to do what you want, which i doubt that, someone probably ran into a similar problem to yours and make a plugin for it. The proper way will be to write a plugins for wordpress for your need and use it instead of sneaking some code in that, some code that may break on next wordpress version update. I don't know wordpress world and plugin but you can tell exactly what your need are here, someone may know the right plugin/addon for it. -
You don't need Webmaster Tools for that. Just redirect your old page with 301 code to the new one and wait. Google will crawl your website and find them. In your products.php use something like that (don't forget to add some security check) : <?php header("HTTP/1.1 301 Moved Permanently"); header("Location: http://www.yoursite.com/products/".$_GET['p']."/"); header("Connection: close"); exit(); ?> so each time someone come to see this url http://yoursite.com/products.php?p=product-name it will be redirect to : http://www.yoursite.com/products/product-name/ in a SEO friendly way. All links in others website to yours, bookmark and robots like google won't end up in a dead link. You can handle the new page with a new php file : Options +FollowSymlinks RewriteEngine on RewriteRule ^(.*)$ prod.php?p=$1 [L] prod.php will essentially be exactly as your old products.php
-
You shouldn't post your password here LinkedIN seem to have a API you should use that instead : http://www.linkedin.com/static?key=developers_apis And the HTTP code 200 mean it work. You can remove the curl_setopt($ch, CURLOPT_HEADER, true); And try again. But it seem to use AJAX so you may need to read the javascript on linkedin and figure all the request it made to the server and try to emulate it. Lot of job and probably a bad idea since you can use the api to do the same.
-
[SOLVED] Can You Open a New Window with a PHP Redirect?
gffg4574fghsDSGDGKJYM replied to Fluoresce's topic in PHP Coding Help
Open the link in a new window/tab before redirecting.... <a href="http://www.somesite.com/visit.php?id=11" target="_blank">sometext</a> New window is a bad pratice, don't throw some javascript at it to make it worst. -
Add this little code and show us \echo $result\ output. curl_setopt($ch, CURLOPT_HEADER, true); You have at least 1 error, cookiefile and cookiejar need a filename not a directory use something like that, you make 2 variable for this and don't use the right one : $cookie_file_path = "tmp/cookie.txt"; And make a if to be sure your curl_exec work like that : $output = curl_exec($ch); $info = curl_getinfo($ch); if ($output === false || $info['http_code'] != 200) { echo "No cURL data returned for $url [". $info['http_code']. "]"; if (curl_error($ch)) echo curl_error($ch); } else { echo $output; }
-
For the ip address use $_SERVER['REMOTE_ADDR'] For the city there no function for that. You will have to find a database make a script for it. MaxMind have a nice database of IP address with ISP/City/Latitude-Longitude/... http://www.maxmind.com/app/ip-locate or you can ask google maybe someone made a API or offer a service for that
-
Sending Headers To Authenticate
gffg4574fghsDSGDGKJYM replied to elite_prodigy's topic in PHP Coding Help
I never try that but it seem that cUrl support both http authentification and HTTPS ssl support : http://www.jellyandcustard.com/2006/01/02/php-curl-http-put-ssl-and-basic-authentication/ http://www.php.net/manual/en/ref.curl.php And it will be able to do what you want without knowing exactly the http header needed for that...hope is that what you are looking for. -
[SOLVED] RSS, having problem with the text
gffg4574fghsDSGDGKJYM replied to Tiltan's topic in PHP Coding Help
It's a charset issue, try this on top of your php before anything else (even space) : <?php header('Content-Type: text/html; charset=utf-8'); ?> In the html : <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> You can also convert special characters into htmlentities like that : <?php $code = htmlentities($entry->title, ENT_NOQUOTES, "UTF-8"); ?> And better to use HTML 4.01 instead of XHTML if you serve it as text/html any way... -
You can get it by .htaccess/php .htaccess RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME} !-f RewriteRule ^(.*)$ somepage.php?page=$1 [QSA,L] Now every request that not a file or a directory will be sent to somepage.php In your php you can get it by <?php echo $_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']; ?> Or if you don't need it when the query is made you can also make a cronjob to parse the apache access_log and get all the line with a 404 response and put that in a db or whatever you want.
-
How can I save my site from SQL Injection
gffg4574fghsDSGDGKJYM replied to avijit's topic in PHP Coding Help
http://www.phpfreaks.com/tutorial/php-security -
[SOLVED] Advice For the Best Approach...
gffg4574fghsDSGDGKJYM replied to CloudSex13's topic in PHP Coding Help
I don't know how you mysql table are done, but you should do something like that : Table 1 : user user.id int user.username varchar user.password varchar .... Table 2 : note note.id int note.content text note.creator_id int (containt the user.id who created the note) ... Each page you have do display/modify a note you should add : if logged user id (data coming from SESSION) user.id == note.creator_id (data coming from the POST/GET) if it don't redirect the user. You can't trust a POST or GET form, including a hidden field form. They are easy to change. -
[SOLVED] Advice For the Best Approach...
gffg4574fghsDSGDGKJYM replied to CloudSex13's topic in PHP Coding Help
Make the page in POST instead of GET. Remove this url/folder from crawler in robots.txt Test if the currently logged user is the creator/owner of the note, if not redirect him to a profil or logout page or show a message that he can't read/modify this note because he isn't the owner. Each page (and not only this one) you should always look first if the user has the right to do what he can do on the page. Hope it answer your question.